A finite state machine (FSM) in C++ is a computational model used to design algorithm representations where an entity transitions between a finite number of states based on inputs.
Here's a simple example of a FSM implementation in C++:
#include <iostream>
#include <string>
enum class State { START, STOP };
class FSM {
public:
State currentState;
FSM() : currentState(State::START) {}
void transition(const std::string& input) {
switch (currentState) {
case State::START:
if (input == "go") {
currentState = State::STOP;
std::cout << "Transitioning to STOP state.\n";
}
break;
case State::STOP:
if (input == "reset") {
currentState = State::START;
std::cout << "Transitioning to START state.\n";
}
break;
}
}
};
int main() {
FSM fsm;
fsm.transition("go"); // Output: Transitioning to STOP state.
fsm.transition("reset"); // Output: Transitioning to START state.
return 0;
}
Understanding FSM
A Finite State Machine (FSM) is a computational model consisting of a limited number of states, transitions between those states, and actions that occur in response to events. FSMs are foundational in computer science, playing critical roles in various applications, from game design to robotics and user interface management.
The concept of FSMs can be traced back to the early days of automata theory. They provide a simple yet powerful way to model the behavior of a system where the state at any given time determines the response to future inputs. By leveraging FSMs in C++, developers can create clear and maintainable code structures that encapsulate the state-dependent behavior of their applications.
How FSMs Work
At its core, an FSM operates through the following essential components:
- States: These define when a system is in a particular configuration or condition.
- Transitions: These are rules that dictate how and when the system moves from one state to another, usually triggered by specific events.
- Events: Inputs or conditions that trigger transitions between states.
To visualize this, you can think about a traffic light. The traffic light can be RED, YELLOW, or GREEN. It transitions from RED to GREEN when an event occurs (e.g., timer expiry), then switches to YELLOW before returning to RED.
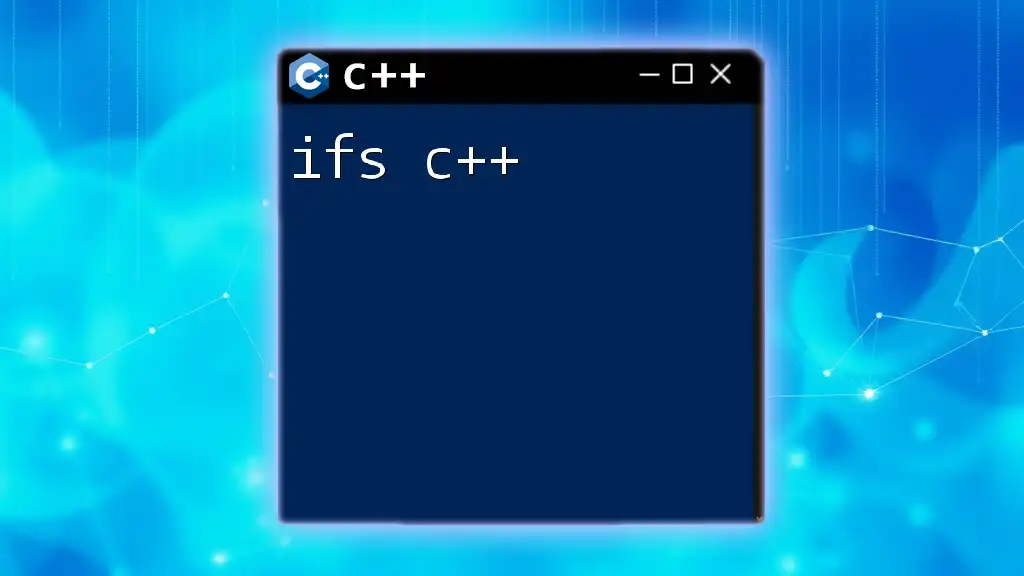
Basics of FSM in C++
Key Concepts of Finite State Machines
Understanding FSMs requires a grasp of three essential concepts:
- States: These represent the distinct conditions or statuses of a system. For example, in a traffic light system, the states consist of RED, YELLOW, and GREEN.
- Transitions: The mechanism that facilitates movement from one state to another. Each transition is typically triggered by an event.
- Events: The triggers that make transitions occur. For a traffic light, the event might be a timer expiring or sensor indications.
Types of FSM
FSMs can be classified into two main types:
-
Deterministic FSM: In this type, each state is associated with exactly one transition for each possible event. This ensures predictability in the behavior of the system.
-
Non-deterministic FSM: Here, a state may have multiple transitions for a given event. This introduces ambiguity, requiring additional rules to resolve which transition to take.
Understanding the differences between these two types forms the basis for implementing FSMs that fit specific system requirements.
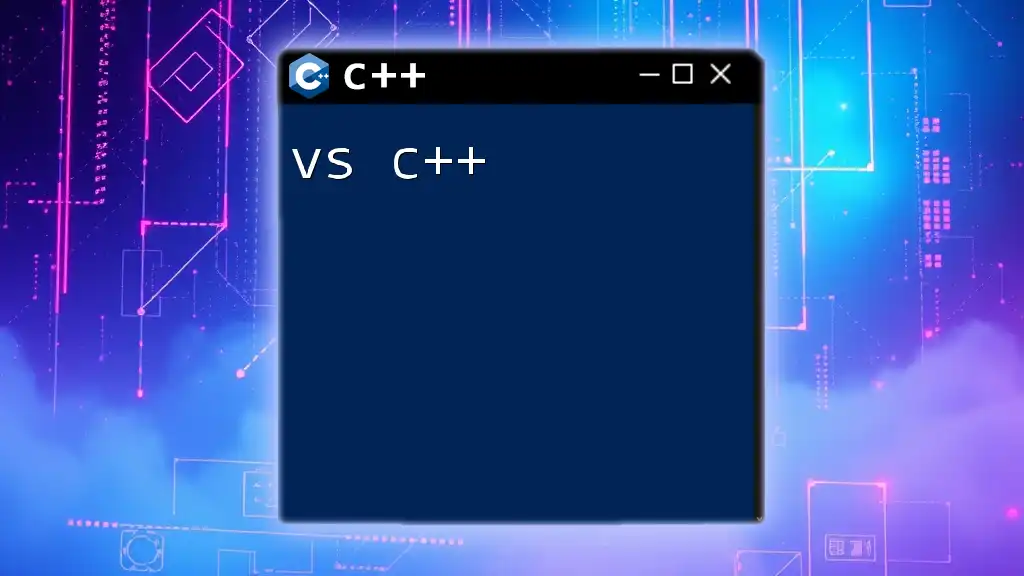
Implementing FSM in C++
Setting Up Your C++ Environment
Before diving into coding, it's crucial to set up your C++ environment properly. Ensure you have a modern compiler (like g++) and an IDE or text editor that you are comfortable with. Consider using tools such as CMake for project management.
Designing a Simple FSM
When designing an FSM, follow these general steps:
-
Define the States: List all the possible states your FSM can exist in.
-
Outline Transitions: Specify what events cause the FSM to transition from one state to another.
-
Implement the Logic: Convert your design into code.
Let's transform this into C++ code by creating a simple traffic light FSM.
class TrafficLight {
public:
enum class State { RED, YELLOW, GREEN };
enum class Event { CHANGE };
TrafficLight() : currentState(State::RED) {}
void trigger(Event event) {
switch (currentState) {
case State::RED:
if (event == Event::CHANGE) currentState = State::GREEN;
break;
case State::GREEN:
if (event == Event::CHANGE) currentState = State::YELLOW;
break;
case State::YELLOW:
if (event == Event::CHANGE) currentState = State::RED;
break;
}
}
State getState() const { return currentState; }
private:
State currentState;
};
In this implementation, we define an enum class for the states (RED, YELLOW, GREEN) and another for the events (CHANGE). The trigger function manages state transitions based on the current state and the incoming event.
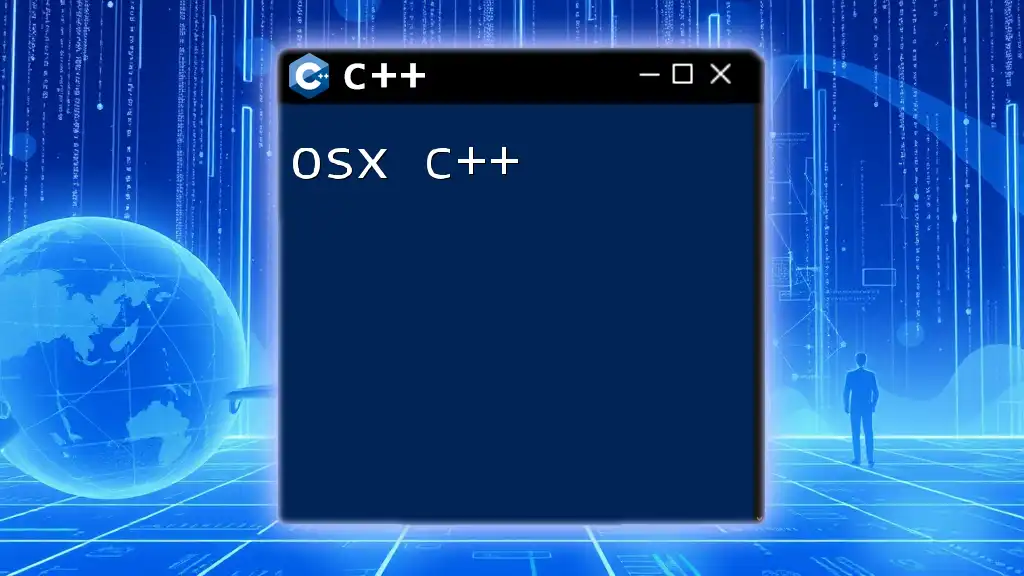
Advanced Concepts in C++ State Machines
Hierarchical State Machines
Hierarchical State Machines (HSMs) build upon basic FSM concepts by allowing states to have substates, creating levels within which transitions can occur. This level of abstraction helps manage complex systems, where states may have their transitions.
By nesting states, organizations can model scenarios where common functionality is inherited from a parent state, reducing code duplication and enhancing clarity.
Event-driven FSMs
Event-driven FSMs improve responsiveness by relying on an event loop that processes events in a queue. This model is particularly useful in applications that require real-time interaction, such as GUIs or online games.
This architecture allows for effective state management, enabling the system to remain responsive while processing multiple inputs simultaneously.
Combining FSM with Other Design Patterns
FSMs can be integrated with other design patterns to enhance flexibility and functionality:
-
Observer Pattern: This pattern allows FSMs to notify listeners of state changes, enabling decoupled communication between different system parts.
-
Strategy Pattern: Utilize this pattern for dynamic state handling, where strategies can be swapped out based on current system states.
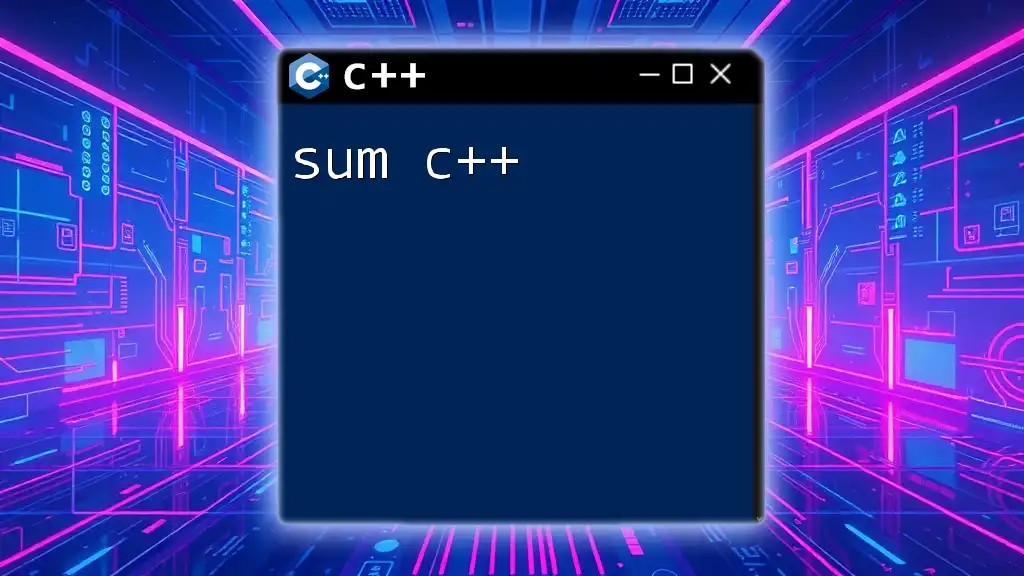
Use Cases of FSM in C++
Real-world Applications
-
Games: FSMs are commonly employed to manage player states, character behavior, and game mechanics, enabling seamless gameplay transitions and interactions.
-
Robotics: Control systems in robotics often leverage FSMs to navigate different operational states, such as idle, moving, or executing tasks.
-
GUI Applications: User interfaces benefit from FSMs by managing views or application states, effectively capturing user interactions.
Case Study: Building a Simple Game State Machine
Let's take a look at how to create a simple game state machine that manages transitions between different game states.
class Game {
public:
enum class GameState { MENU, PLAYING, PAUSED, GAME_OVER };
void changeState(GameState newState) {
currentState = newState;
}
private:
GameState currentState;
};
The `Game` class shown above contains an enum class for the possible game states (MENU, PLAYING, PAUSED, GAME_OVER) and a method to change the state. This structure allows for clear management of what the game is doing at any point in time.
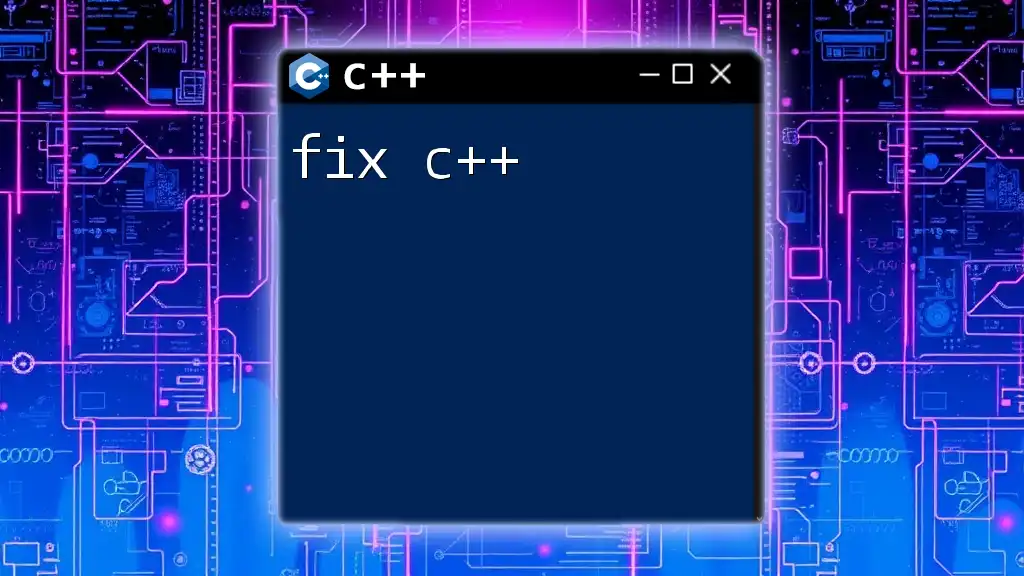
Best Practices for FSM in C++
Tips for Effective FSM Design
To create robust FSM applications, consider these best practices:
-
Keep States and Transitions Clear: Ensure that the purpose of each state and transition is understood.
-
Avoid Complex State Logic: Maintain simplicity to reduce the chances of errors and make debugging easier.
-
Use Clear Naming Conventions: This enhances code readability and helps others understand your logic quickly.
Common Pitfalls to Avoid
While designing FSMs, be mindful of the following pitfalls:
-
Overcomplicating State Transitions: Simplicity is key. Avoid convoluted transitions that can confuse future developers (or yourself).
-
Ignoring Edge Cases: Ensure that transitions cover all possible events to avoid unexpected behavior.
-
Failing to Test Transition Conditions: Comprehensive testing is essential to guarantee that state transitions function as intended under various scenarios.
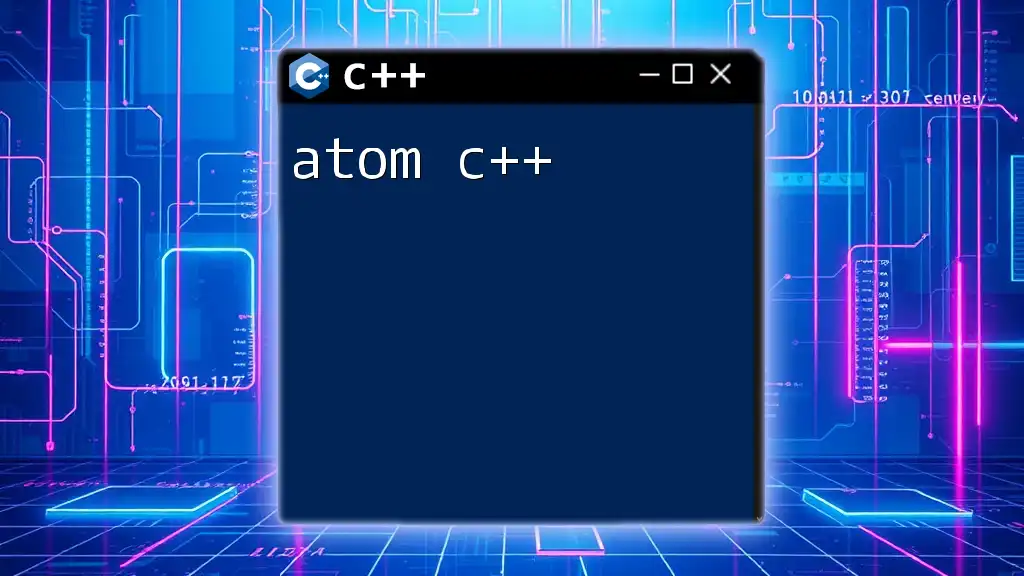
Conclusion
The integration of FSM in C++ represents a powerful approach to managing state-dependent behaviors within applications. As technology evolves and projects grow in complexity, mastering FSMs will become increasingly important for developers.
Learning to implement and utilize FSMs effectively not only enhances system architecture but also ensures code remains readable and manageable, aligning with best practices in software design.
As a call to action, experiment with FSMs in your projects, leveraging their capabilities to streamline complex workflows and user interactions. Consider diving into additional resources and tutorials to broaden your understanding and expertise in applying FSM concepts in C++ effectively.
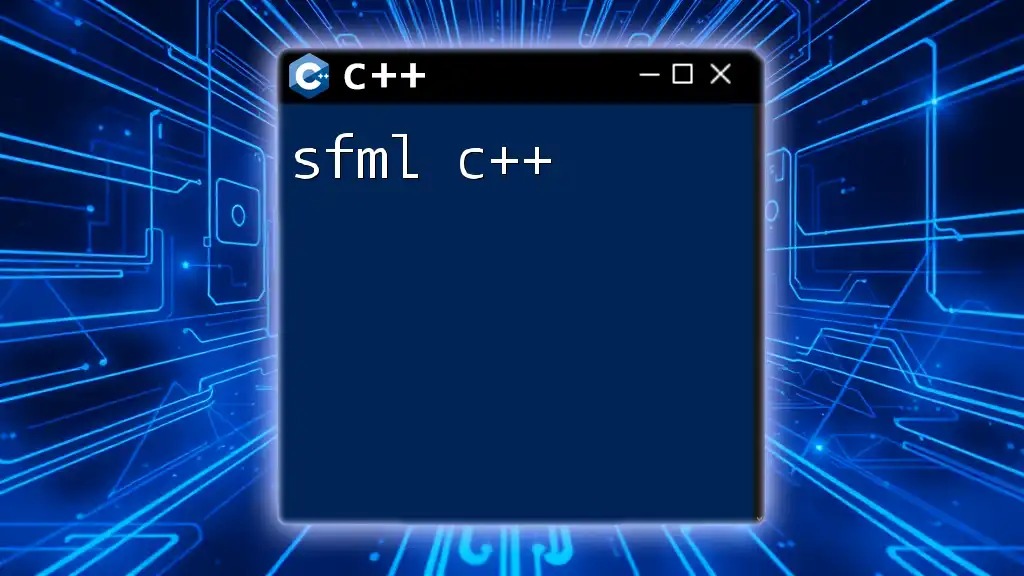
References
- Recommended books, articles, and online courses are available for those seeking to deepen their knowledge in FSM and C++ software design.