Kafka C++ refers to the use of the Apache Kafka client library in C++ for producing and consuming messages efficiently from Kafka topics.
Here’s a simple example of producing a message to a Kafka topic using the C++ client:
#include <librdkafka/rdkafkacpp.h>
int main() {
RdKafka::Conf *conf = RdKafka::Conf::create(RdKafka::Conf::ConfType::CONF_GLOBAL);
conf->set("bootstrap.servers", "localhost:9092", errstr);
RdKafka::Producer *producer = RdKafka::Producer::create(conf, errstr);
RdKafka::Topic *topic = RdKafka::Topic::create(producer, "my_topic", nullptr, errstr);
producer->produce(topic, RdKafka::Topic::PARTITION_UA, RdKafka::Producer::RK_MSG_COPY,
"Hello, Kafka!", strlen("Hello, Kafka!"), nullptr, nullptr);
producer->poll(0);
delete topic;
delete producer;
delete conf;
return 0;
}
Understanding Apache Kafka
What is Apache Kafka?
Apache Kafka is a distributed event streaming platform capable of handling trillions of events a day. It is designed to provide high throughput and fault tolerance, making it an integral component in various real-time data processing scenarios. Common use cases include log aggregation, stream processing, and building real-time data pipelines.
In a microservices architecture, Kafka acts as a central communication hub, allowing services to publish and subscribe to streams of records, which facilitates job decoupling and scaling.
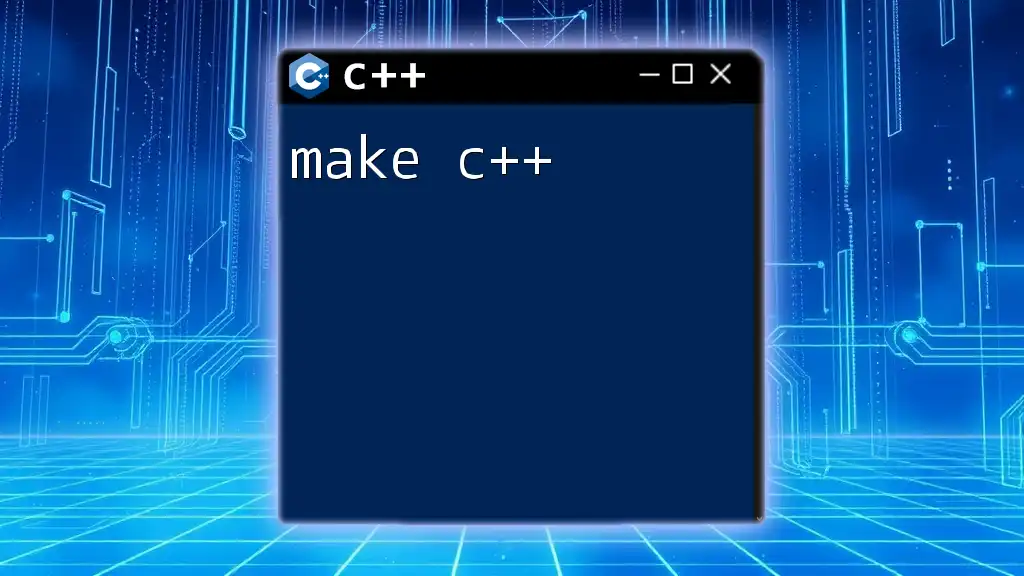
Overview of C++ Programming Language
Why C++?
C++ is a powerful programming language known for its performance and efficiency. It provides low-level memory manipulation capabilities alongside high-level abstractions, making it suitable for implementing complex systems that require fine-tuning. Applications of C++ span across various domains, from system programming to game development, making it an attractive choice for building high-performance applications that interact with Kafka.
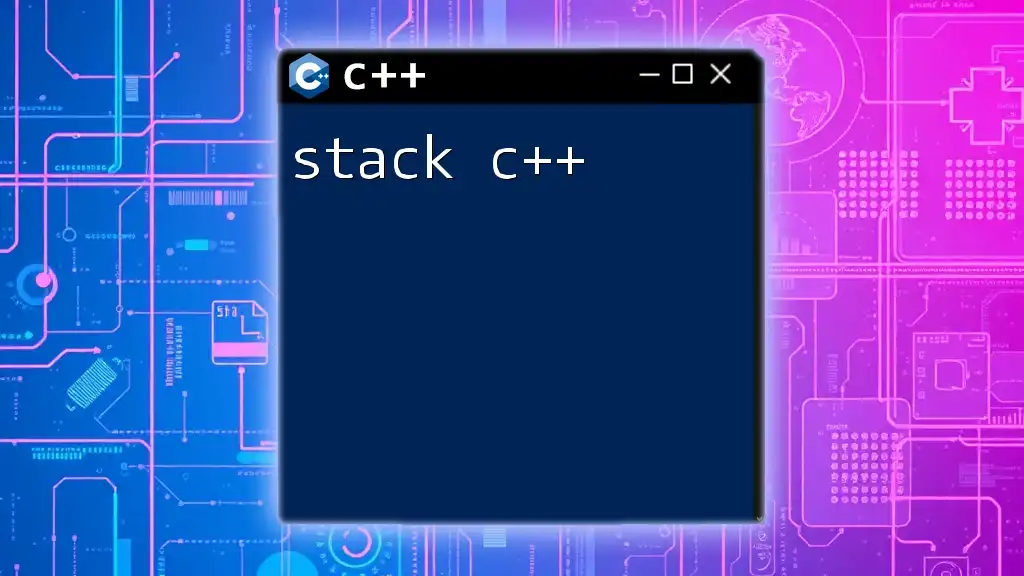
Setting Up Your Kafka Environment
Installing Apache Kafka
Installation Steps
To work with Kafka in C++, you first need to install Apache Kafka:
- Downloading Kafka: Obtain the latest Kafka release from the official Apache website.
- Configuring Kafka Server: Follow the setup instructions to configure server properties, such as Zookeeper and Kafka broker settings.
- Starting Kafka Server: Run the Zookeeper instance and the Kafka server using terminal commands.
Setting Up C++ Development Environment
Choosing the Right C++ Compiler
Selecting a reliable C++ compiler is crucial for development. Options include GCC, Clang, and Microsoft Visual Studio Compiler (MSVC). Each offers varying features, so choose one that matches your development requirements.
Installing Dependencies
Ensure you have the necessary libraries installed for Kafka. One of the most recommended libraries is librdkafka, which provides a C and C++ client for Kafka. Other essential tools include CMake and Boost, which can help with building your C++ applications.
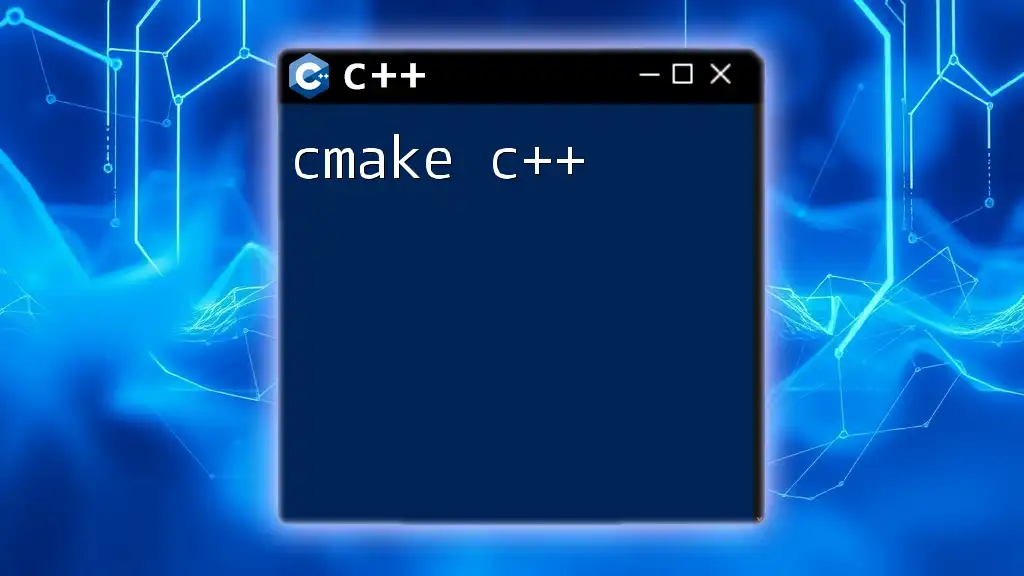
Working with Kafka in C++
Understanding Kafka Client Libraries for C++
When working with Kafka in C++, one popular choice is librdkafka, a robust client library designed to maximize Kafka's capabilities while providing simplicity for developers. It has a rich set of features, including producing and consuming messages, topic management, and configuration options.
Configuring the Kafka C++ Producer
Creating a producer in C++ is straightforward with the existing client libraries. Here’s how you can implement a basic Kafka producer:
#include <librdkafka/rdkafkacpp.h>
int main() {
// Kafka Producer Configuration
RdKafka::Conf *conf = RdKafka::Conf::create(RdKafka::Conf::ConfType::CONF_GLOBAL);
std::string errstr;
conf->set("bootstrap.servers", "localhost:9092", errstr);
// Create Producer
RdKafka::Producer *producer = RdKafka::Producer::create(conf, errstr);
// Sending a message
std::string topic_name = "my_topic";
RdKafka::Topic *topic = RdKafka::Topic::create(producer, topic_name, nullptr, errstr);
producer->produce(topic, RdKafka::Topic::PARTITION_UA, RdKafka::Producer::RK_MSG_COPY, "Hello Kafka", 10, nullptr, nullptr);
// Clean up
delete topic;
delete producer;
delete conf;
return 0;
}
In this code snippet:
- We first create a configuration object, specifying the Kafka broker with `bootstrap.servers`.
- A producer instance is then created, which is used to send messages to a specified topic.
- The `produce` method sends a message containing the string "Hello Kafka" to the topic in a designated partition.
Configuring the Kafka C++ Consumer
Setting up a Kafka consumer involves subscribing to topics and polling for messages. Below is a simple implementation:
// Kafka Consumer Configuration
RdKafka::Conf *conf = RdKafka::Conf::create(RdKafka::Conf::ConfType::CONF_GLOBAL);
std::string errstr;
conf->set("group.id", "my_group", errstr);
conf->set("bootstrap.servers", "localhost:9092", errstr);
// Create Consumer
RdKafka::Consumer *consumer = RdKafka::Consumer::create(conf, errstr);
// Subscribing to topics
std::vector<std::string> topics = {"my_topic"};
consumer->subscribe(topics);
// Polling for messages
RdKafka::Message *msg = nullptr;
consumer->poll(1000); // Wait for a message
if ((msg = consumer->consume(1000)) != nullptr) {
std::cout << "Received message: " << static_cast<char *>(msg->payload()) << std::endl;
}
// Clean up
delete consumer;
delete conf;
return 0;
In this example:
- We configure the consumer with `group.id` for load balancing and `bootstrap.servers` to specify the Kafka brokers.
- We subscribe to a topic and use the `poll` method to receive messages.
- The `consume` function is invoked to retrieve a message from the topic, which gets printed to the console.
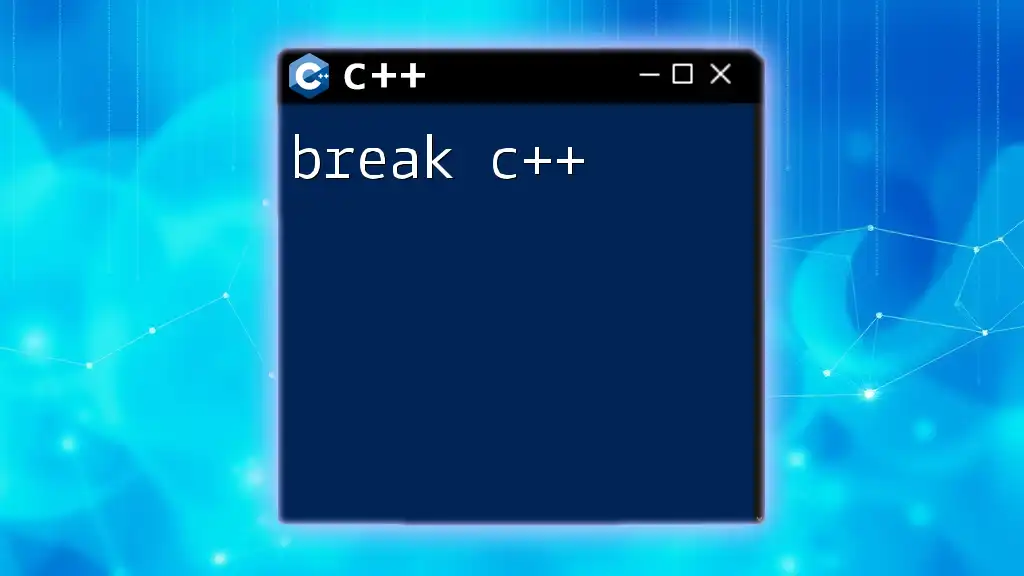
Best Practices for Working with Kafka in C++
Error Handling and Debugging
Errors can arise during production and consumption that need to be effectively handled. Common issues include connection problems and misconfigurations. Implement comprehensive error logging to troubleshoot, using librdkafka's built-in logging features for identifying problems in your setup.
Improving Performance
Enhancing Kafka's performance can significantly impact your application's efficiency. Batch processing and asynchronous calls are effective strategies for boosting throughput. You can increase the `batch.size` configuration in your producer to send larger batches of messages and set up asynchronous message production to reduce latency.
Here's a quick example of how to set batch processing:
conf->set("batch.size", "16384", errstr); // Set batch size to 16KB
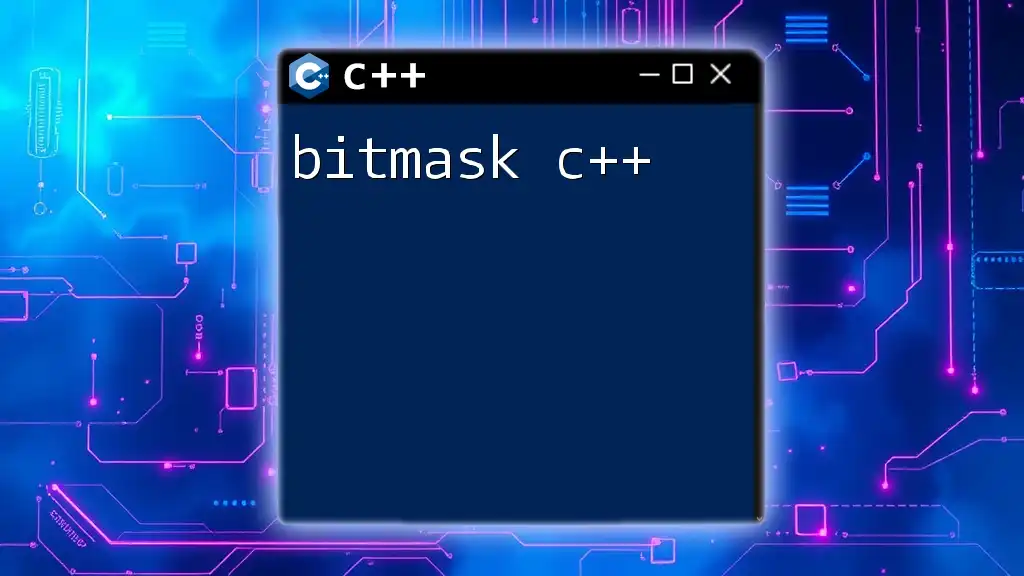
Real-World Use Cases and Applications
Scenarios for Using Kafka with C++
Kafka and C++ integration can serve many scenarios:
- Microservices Communication: Using Kafka as a message broker to facilitate communication between different microservice components.
- Real-Time Data Processing: Processing streams of data in real-time, such as sensor data in IoT applications.
- Logging and Monitoring Systems: Aggregating log data from multiple services into a centralized Kafka topic for real-time monitoring and analysis.
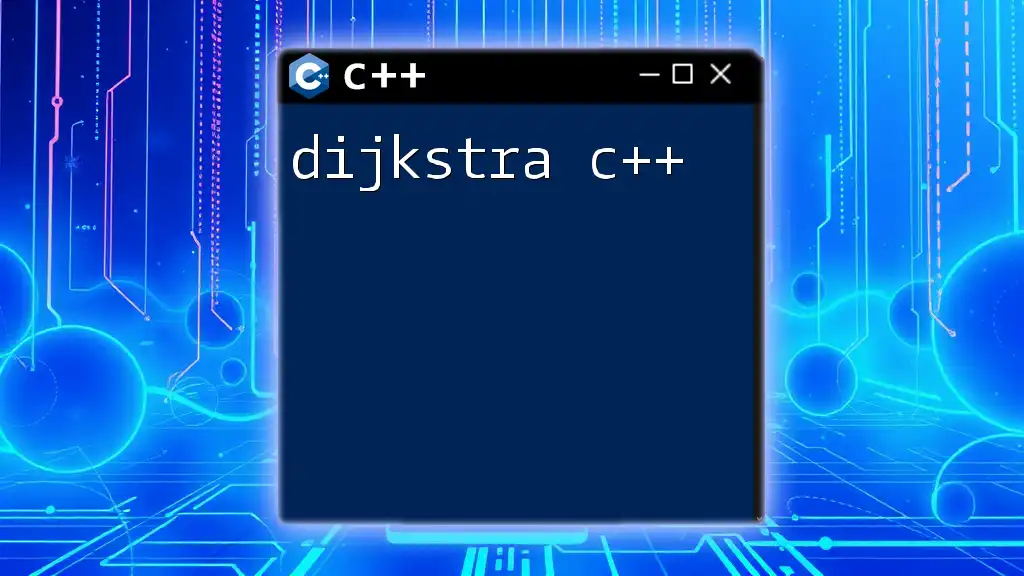
Conclusion
In this article, we've explored the core concepts and practices of working with Kafka in C++. Understanding the integration of kafka c++ enhances the ability to build robust applications capable of handling distributed data streams. Utilizing Kafka's features allows developers to create scalable services that can process high volumes of data efficiently.
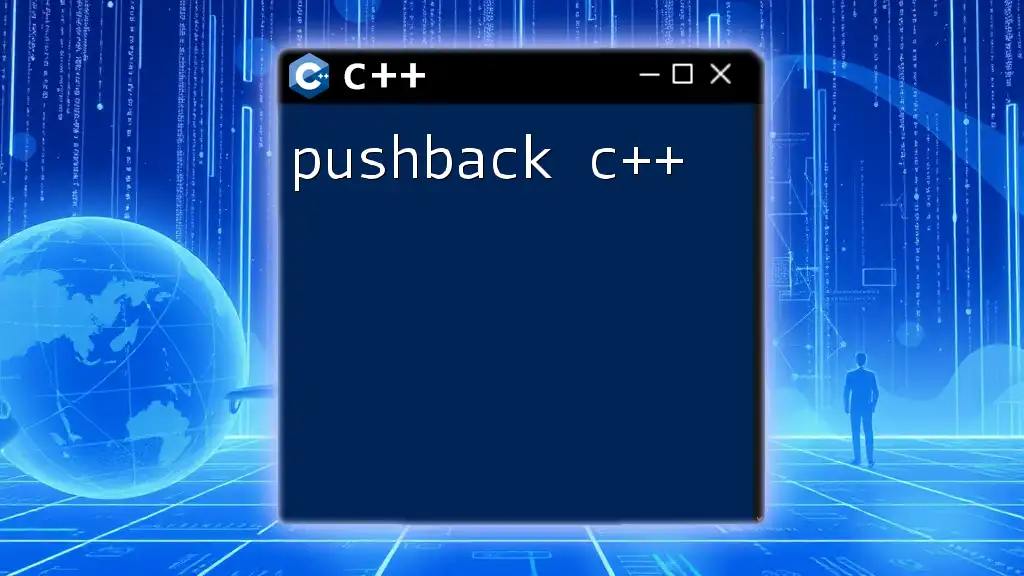
Additional Resources and Tools
For further reading, consult the Kafka Official Documentation, which offers comprehensive insight into advanced configurations and scenarios. Libraries like librdkafka have their own documentation and examples for quick reference. For community support, consider engaging with forums and platforms dedicated to Kafka and C++.
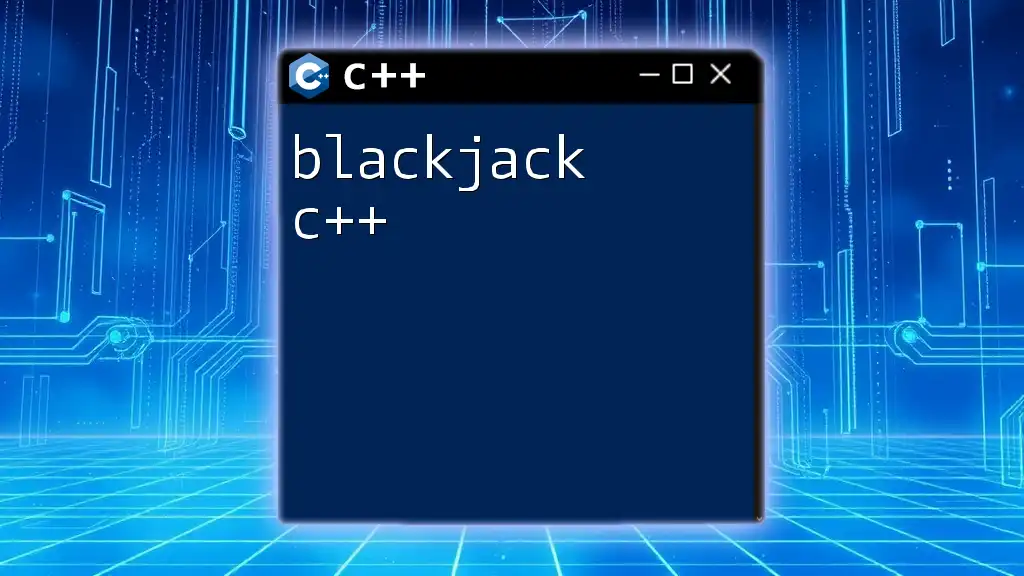
Call to Action
Experiment with the provided examples and explore the various capabilities of Kafka in your C++ applications. Engage with the community, share your experiences, and enhance your projects leveraging the power of Kafka!