A Java to C++ translator is a tool that converts Java code into equivalent C++ code to facilitate easier cross-language programming.
Here's a simple code snippet illustrating the translation of a Java class to C++:
// Java Code
class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
// Equivalent C++ Code
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Understanding Java and C++
Differences Between Java and C++
Java and C++ are both powerful programming languages, but they have fundamental differences that affect how code is written and executed.
Memory Management
Java has a built-in garbage collection system that automatically manages memory, freeing up allocated space when objects are no longer in use. In contrast, C++ requires manual memory management, meaning developers must explicitly allocate and deallocate memory. This requires a deeper understanding of how memory operates but allows more control and potentially better performance when done correctly.
Object-Oriented Principles
Both languages support object-oriented programming, but their approaches to inheritance and polymorphism differ. For instance, Java uses interfaces and abstract classes extensively, while C++ allows multiple inheritance, which can complicate the design but offers greater flexibility.
Syntax Variations
While the syntax of Java and C++ is similar because both are influenced by C, there are notable differences, especially in how data types and functions are declared.
Example: Variable Declarations in Java vs. C++
int count = 10; // Java
int count = 10; // C++
As shown, basic variable declaration is similar, but deeper concepts like function overloading or operator overloading significantly differ.
When to Consider Conversion
A java c++ translator is particularly useful in several situations.
Performance Needs
If an application is performance-critical and Java’s garbage collection is causing latency issues, C++ can be a better choice due to its compiled nature and manual memory management.
System Level Programming
If you're working with low-level system programming, such as operating systems or hardware interaction, C++ is often the preferred language for its direct memory access capabilities.
Access to C/C++ Libraries
For projects that rely on C or C++ libraries, converting Java code allows developers to leverage existing powerful libraries not available in Java.
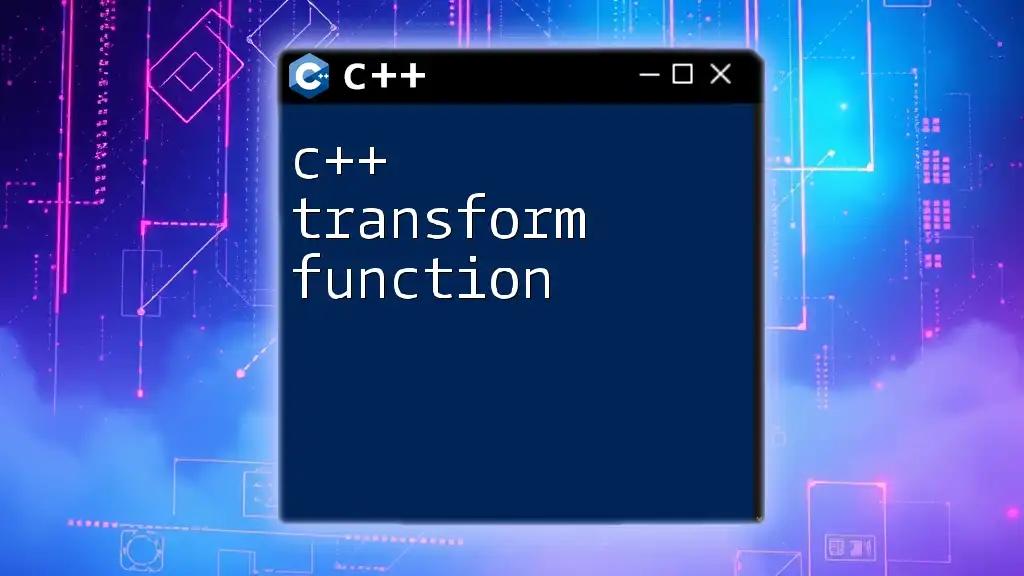
How to Convert Java to C++
Key Concepts in Translation
Understanding how to translate specific elements is crucial:
Data Types
Java's data types need to be mapped to their C++ counterparts.
- Java `int` corresponds to C++ `int`
- Java `float` aligns with C++ `float`
- Java `String` can be represented as `std::string` in C++
Example conversion:
String text = "Hello, World!"; // Java
std::string text = "Hello, World!"; // C++
Control Structures
Control flow is similar, but syntax can differ.
Example: Translating a Java for-loop to C++: Java:
for (int i = 0; i < 10; i++) {
System.out.println(i);
}
C++:
for (int i = 0; i < 10; i++) {
std::cout << i << std::endl;
}
Class and Object Translation
When converting classes, pay attention to constructors, destructors, and access modifiers.
Java:
class Dog {
String name;
Dog(String name) {
this.name = name;
}
void bark() {
System.out.println("Woof");
}
}
C++:
class Dog {
std::string name;
public:
Dog(std::string name) : name(name) {}
void bark() {
std::cout << "Woof" << std::endl;
}
};
Tools for Java to C++ Conversion
Automated java c++ translator tools exist, which can simplify the task. However, they have limitations.
- Pros: Quick conversion and capable of handling large codebases.
- Cons: Generated code may not be idiomatic C++ and might require significant tweaks.
It's advisable to review the output of these tools and understand the converted code, rather than blindly relying on them.
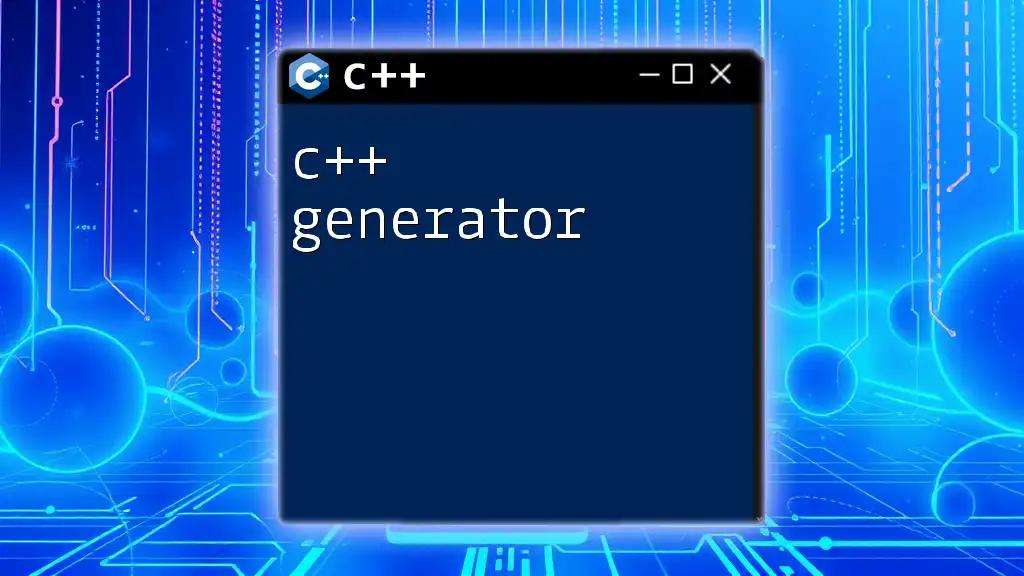
Step-by-Step Guide for Manual Conversion
Step 1: Analyze the Java Code
Before starting the conversion, analyze the Java code thoroughly to understand the logic and functionality. This allows for more accurate translation and ensures the final C++ code meets the desired requirements.
Step 2: Create a C++ Project Structure
Establishing a clear project structure is essential. You should set up headers and source files appropriately. C++ uses namespaces instead of Java's packages.
Example: Translating a package to a namespace Java:
package com.example;
C++:
namespace Example {
// C++ code goes here
}
Step 3: Manual Code Translation
Translate the code segment by segment, paying close attention to the logic flow and syntax.
Example: A simple Java program and its C++ equivalent Java:
public class Main {
public static void main(String[] args) {
Dog myDog = new Dog("Rex");
myDog.bark();
}
}
C++:
#include <iostream>
#include <string>
class Dog {
public:
std::string name;
Dog(std::string name) : name(name) {}
void bark() {
std::cout << "Woof" << std::endl;
}
};
int main() {
Dog myDog("Rex");
myDog.bark();
return 0;
}
Step 4: Testing the C++ Application
Once the translation is completed, it’s vital to test the C++ application thoroughly.
Running Unit Tests
Implement unit tests to ensure that the translated code behaves as intended. This often requires writing new tests tailored for C++.
Handling Issues and Debugging
Common issues may arise during translation, resulting in errors or unexpected behavior. Be prepared for debugging and refining the code once the basics are in place.
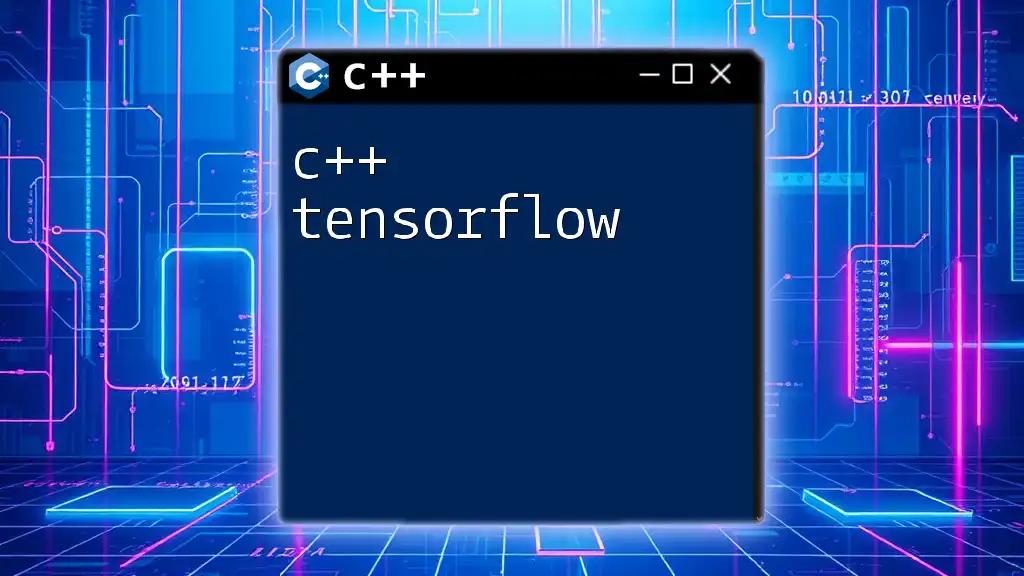
Best Practices for Java to C++ Conversion
Keep Code Simple and Readable
One of the most important principles during conversion is clarity. Aim to keep the code simple, which often translates to better performance and maintainability. Use C++ idioms when appropriate to leverage the language's strengths.
Documentation and Comments
As you convert code, maintain thorough documentation on what changes were made and why. Commenting on complex conversions can help you and others understand the reasoning behind specific implementations.
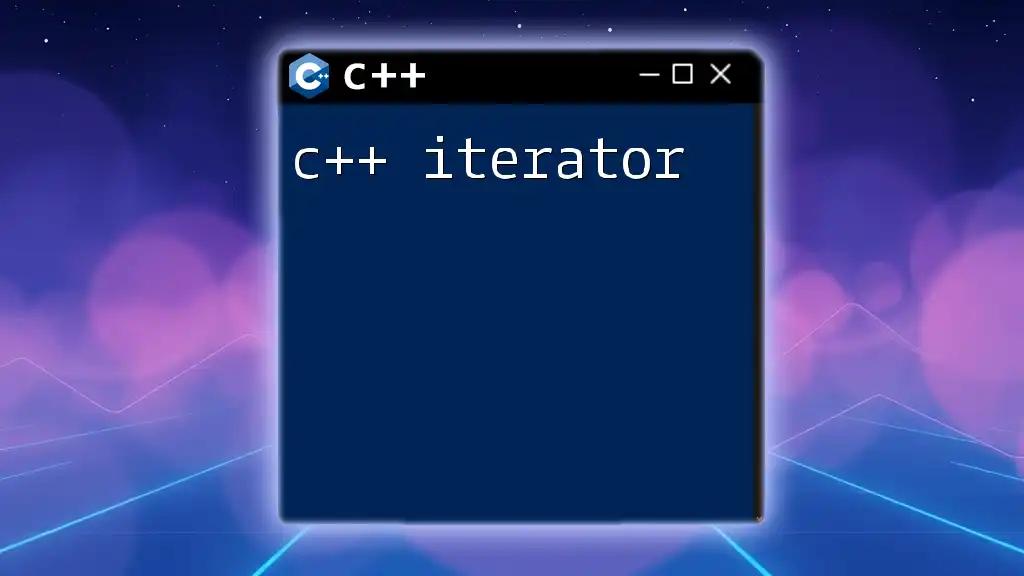
Common Challenges in Translation
Converting from Java to C++ is not without its challenges.
Syntax Errors
One common issue arises from mismatches in syntax between the two languages. Watch for missing semicolons or incorrect brackets that don’t translate well between languages.
Library and API Differences
Java's rich library ecosystem means that certain functions may not have direct C++ equivalents. Understanding these differences in APIs is essential for successful conversion.
Performance Bottlenecks
After converting, don’t forget to analyze the performance of your C++ application. Due to differences in how Java and C++ handle memory, you might encounter performance bottlenecks that weren't present in the Java version.
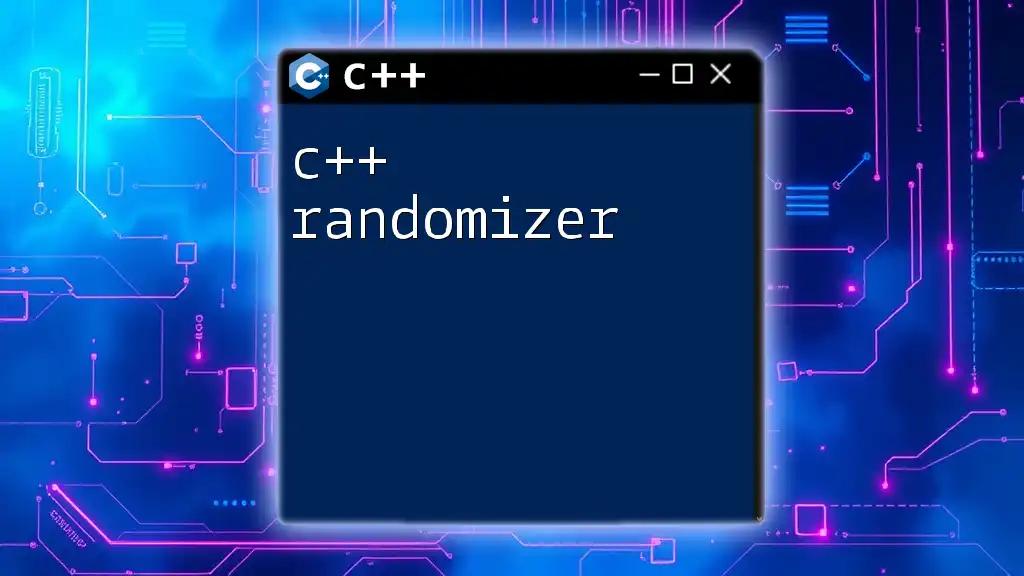
Conclusion
The java c++ translator concept is both a necessity and a challenge for those transitioning between these languages. By developing a strong understanding of both Java and C++, you can successfully navigate their differences, ensuring a smooth and efficient translation process.
Make sure to take advantage of resources and communities for ongoing support, and don’t hesitate to reach out with your questions or for further learning opportunities. The journey of becoming proficient in translating between Java and C++ will enhance your programming capabilities and broaden your skill set in software development.