In C++, "fallthrough" occurs when control passes from one case of a switch statement to the next without a break statement, allowing multiple cases to execute sequentially.
Here's an example of C++ fallthrough in action:
#include <iostream>
int main() {
int value = 2;
switch (value) {
case 1:
std::cout << "Value is 1" << std::endl;
break;
case 2:
std::cout << "Value is 2" << std::endl;
// Intentional fallthrough
case 3:
std::cout << "Value is 3" << std::endl;
break;
default:
std::cout << "Value is unknown" << std::endl;
}
return 0;
}
In this example, if `value` is 2, the output will be:
Value is 2
Value is 3
What is Fallthrough in C++?
Definition of Fallthrough
In C++, fallthrough refers to a behavior in switch statements where control passes from one case to the next without encountering a `break` statement. This feature allows for multiple case statements to share the same code, effectively enabling the flow of execution through several blocks of code until a break or the end of the switch statement is reached.
Historical Context
Fallthrough originated in the C programming language, where it was a common technique for executing shared logic among several cases. With the transition to C++, the fundamental mechanics of fallthrough remained unchanged, but the language introduced more features and stricter syntactical rules to improve code readability and maintainability.
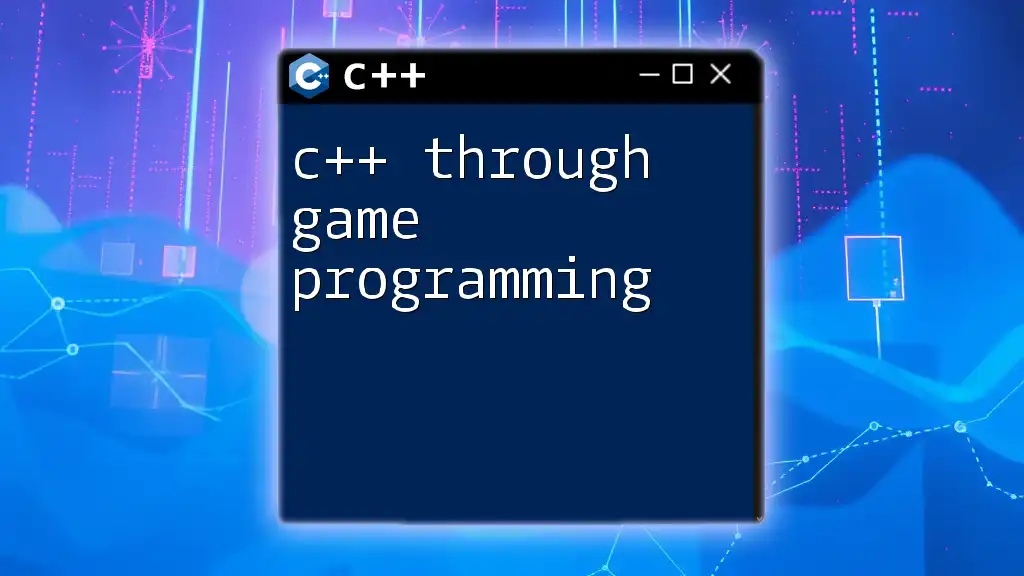
The Mechanics of Fallthrough
Understanding Switch Statements
A switch statement is a control flow structure that allows the variable's value to be compared against a list of possible matches, known as cases. Here's the basic syntax:
switch(expression) {
case constant:
// code to execute
break;
// other cases...
default:
// optional default code
}
When a match is found, the corresponding block of code executes. If there is no `break`, execution continues into the next case, which is the essence of fallthrough.
How Fallthrough Occurs
When control falls through from one case to another, it can lead to multiple cases sharing logic seamlessly:
#include <iostream>
using namespace std;
void testFallthrough(int value) {
switch(value) {
case 1:
cout << "Value is 1" << endl;
case 2:
cout << "Value is 2" << endl;
case 3:
cout << "Value is 3" << endl;
break;
default:
cout << "Value is unknown" << endl;
}
}
int main() {
testFallthrough(2);
return 0;
}
In this example, if you call `testFallthrough(2)`, the output will include text from both case 2 and case 3 because there is no break after case 2. This illustrates how fallthrough allows multiple cases to run sequentially.
When Does Fallthrough Occur?
Fallthrough can be beneficial in certain scenarios, such as when multiple cases share the same logic. For example, if multiple values correspond to the same action, you can represent this compactly:
void testChainedCases(int day) {
switch(day) {
case 1:
case 2:
case 3:
cout << "Weekday" << endl;
break;
case 4:
case 5:
case 6:
case 7:
cout << "Weekend" << endl;
break;
default:
cout << "Invalid day" << endl;
}
}
Here, cases 1, 2, and 3 all lead to "Weekday," thus simplifying code.
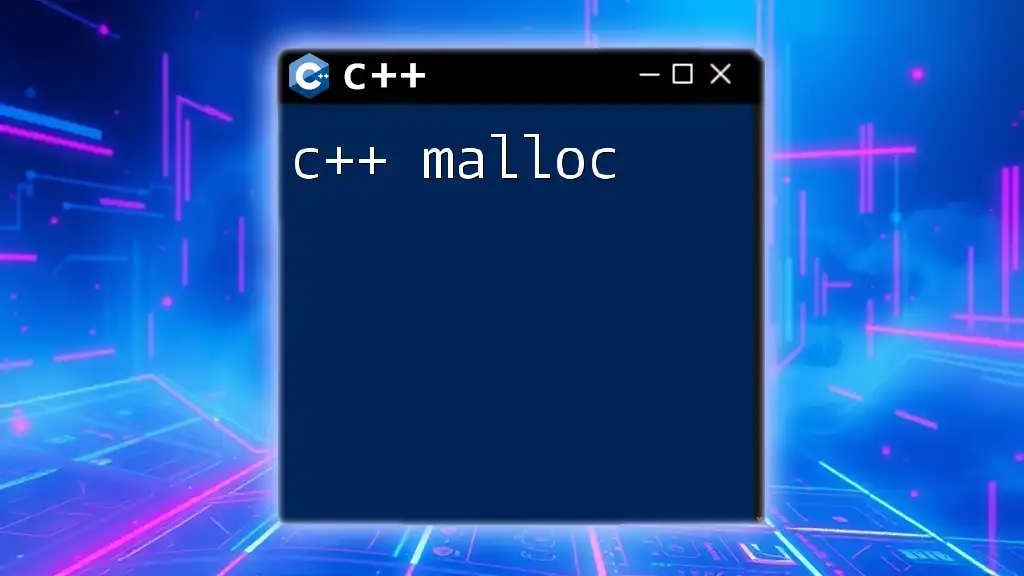
Example of Fallthrough
Basic Fallthrough Example
Let’s look deeper into our earlier example of fallthrough. As noted, calling `testFallthrough(2)` will yield:
Value is 2
Value is 3
This shows how control passed through case 2 into case 3 due to the absence of a `break`. Understanding this behavior can help you structure switch statements in a more organized way, utilizing fallthrough where appropriate.
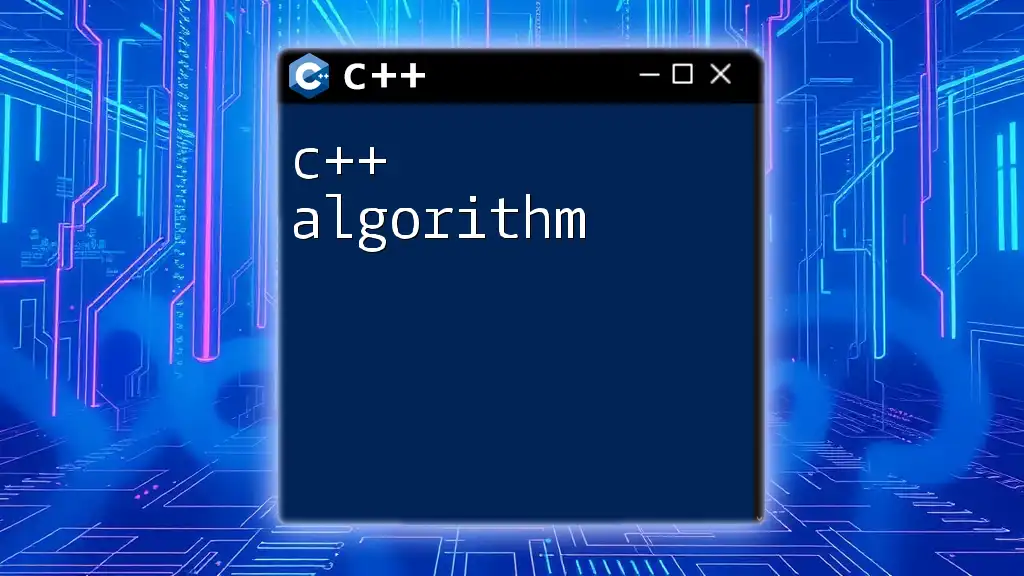
Common Pitfalls of Fallthrough
Missing Break Statements
One of the most common pitfalls with fallthrough is forgetting `break` statements. If a `break` is missing intentionally or due to oversight, it can lead to unexpected behavior, making the code hard to debug. Always be cautious and aware of where your execution paths lead, especially in a complex switch statement.
Misunderstandings in Intent
It's essential to clarify when fallthrough is intentional versus accidental. Readers of your code should easily grasp your intentions. Documenting your code with comments and using break statements appropriately can create clarity.
Using Attributes to Indicate Intent
In modern C++ (C++17 and beyond), you can use the [[fallthrough]] attribute to specify that fallthrough is intentional. Here’s how it works:
switch(value) {
case 1:
cout << "Case 1" << endl;
[[fallthrough]];
case 2:
cout << "Case 2" << endl;
break;
}
This not only makes your code more explicit but also informs readers and compilers that you've deliberately chosen fallthrough. Good documentation practices can significantly enhance code maintainability and comprehension.
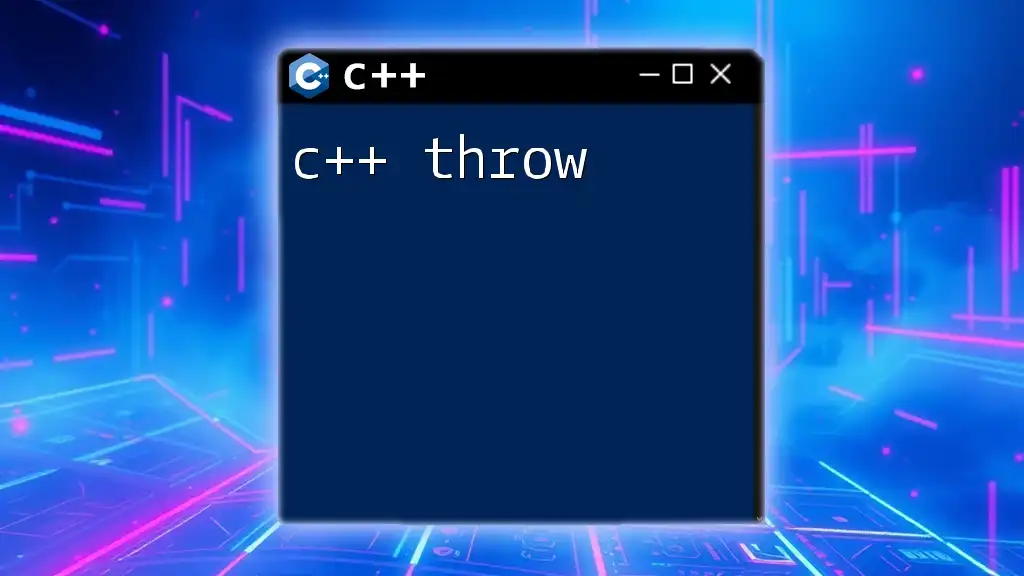
Alternatives to Fallthrough
Avoiding Fallthrough with If-Else Statements
While fallthrough can reduce redundancy, it’s crucial to consider alternatives. If the logic is sufficiently complicated or the cases diverge significantly, using `if-else` statements can provide clearer intent:
if (value == 1) {
cout << "Value is 1" << endl;
} else if (value == 2) {
cout << "Value is 2" << endl;
} else {
cout << "Value is unknown" << endl;
}
Such an approach can make it easier to follow the code's flow, particularly for those less familiar with C++ fallthrough behavior.
Using Functions for Complex Logic
For those situations when many cases require significantly different behavior, it’s often wise to extract logic into functions. This will not only promote cleaner code but also increase its reusability. By breaking down complex switch statements into well-defined functions, you can improve maintainability and enhance collaborative coding efforts.
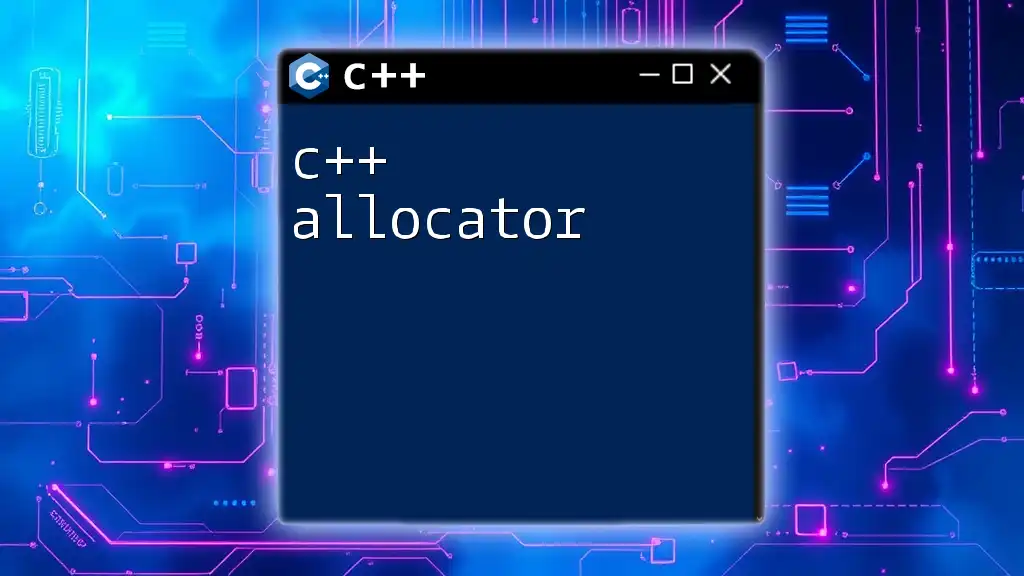
Conclusion
Understanding C++ fallthrough is essential for writing effective switch statements. While fallthrough can simplify code logic, it requires careful consideration and frequent documentation to ensure clarity and prevent unintended consequences. Experimenting with fallthrough will deepen your understanding and help you leverage this valuable feature effectively in your C++ projects.
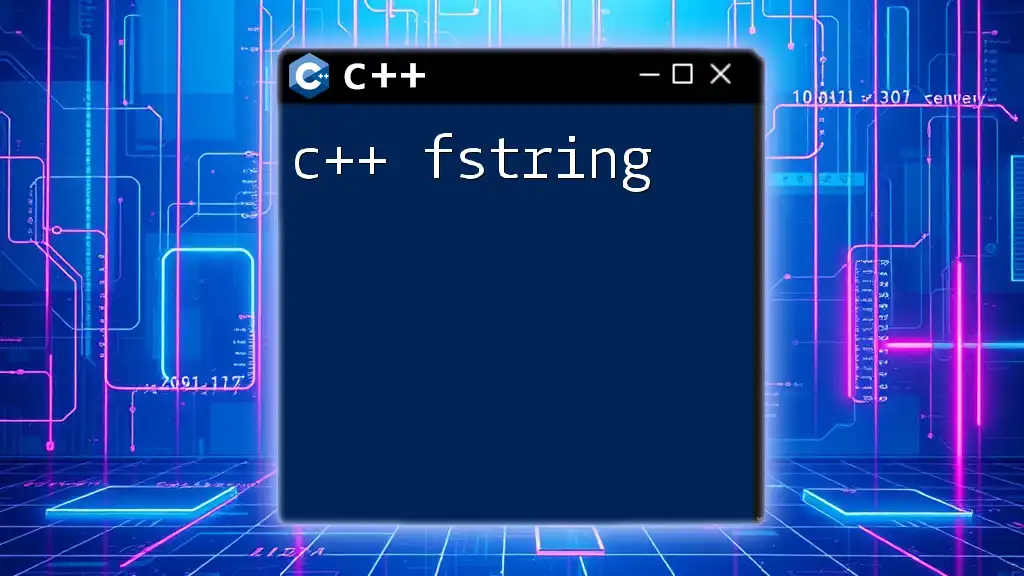
Additional Resources
To deepen your knowledge further, consider exploring additional articles or books focused on C++ programming. Online tutorials related to control flow and community forums can serve as excellent resources for discussing best practices and addressing any lingering questions you may have.