`c++filt` is a command-line tool that demangles C++ symbol names, converting them from their encoded format back into readable function and variable names.
Here's a code snippet demonstrating how to use `c++filt`:
echo "_Z3addii" | c++filt
This will output:
add(int, int)
Understanding Name Mangling
What is Name Mangling?
Name mangling is a process where the compiler generates unique names for functions, variables, and classes in languages that support features like function overloading and namespaces. In C++, this is crucial for distinguishing between multiple instances of a function sharing the same name but differing by their parameter types.
Why is Name Mangling Necessary?
Name mangling becomes essential in C++ due to its support for:
- Function Overloading: Functions can share the same name as long as their parameter types differ. The compiler needs to create unique identifiers to avoid confusion during linking.
- Namespaces and Classes: Multiple functions and classes may exist with the same names across different namespaces or classes. The compiler distinguishes these by modifying the names.
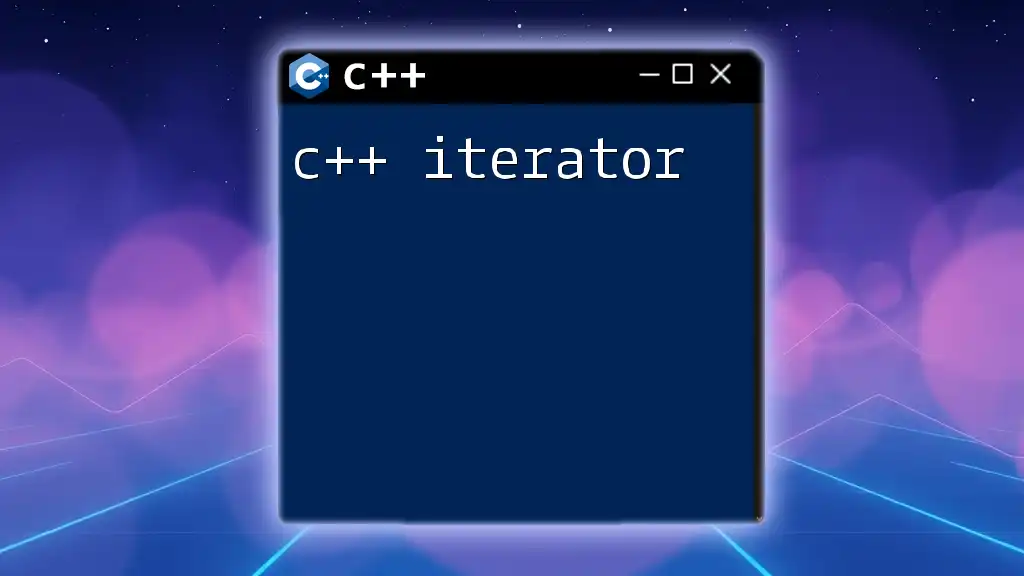
Introduction to c++filt
What is c++filt?
c++filt is a command-line tool specifically designed to demangle (or unpack) the mangled names generated by the C++ compiler. When you encounter a mangled name in error messages or logs, c++filt will turn it back into a human-readable format, making debugging significantly easier.
History and Evolution of c++filt
c++filt was developed as part of the GNU Binutils project. Its purpose has evolved over the years to accommodate changes in the C++ standard, reflecting the ongoing advancements in the language. This evolution has ensured that c++filt remains a reliable tool for developers needing to decode complex C++ function signatures.
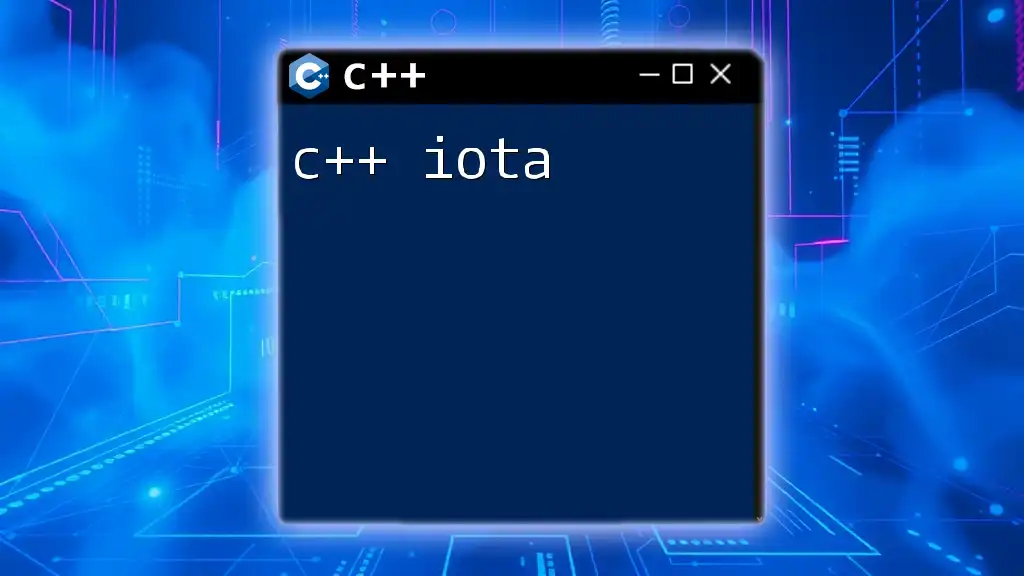
Using c++filt: A Step-by-Step Guide
Installing c++filt
Installing c++filt is straightforward and varies slightly depending on your operating system:
-
Linux: Most Linux distributions offer c++filt as part of the binary packages. Use your package manager to install:
sudo apt-get install binutils
-
macOS: Install it using Homebrew:
brew install binutils
-
Windows: You can find c++filt as part of MinGW or Cygwin installations. Install the corresponding package to include it.
Basic Syntax of c++filt Command
The primary command to use c++filt is simple:
c++filt <mangled_name>
Replace `<mangled_name>` with the name you want to demangle.
Common Use Cases
Demangling Function Names
To demangle a function name, simply provide it as an argument to c++filt. For example:
c++filt _Z7myFunctioni
The output will be:
myFunction(int)
This shows you the clear function signature, helping you understand its parameters.
Demangling Class Names
You can also use c++filt to demangle class names. For example:
c++filt _ZN3Foo3barEv
This will output:
Foo::bar()
Again, you receive clarity on how the class and its member functions are structured.
Batch Processing
c++filt can handle multiple mangled names, allowing batch demangling. You can use a command like this:
printf "_Z7myFunctioni\n_ZN3Foo3barEv\n" | c++filt
This will output:
myFunction(int)
Foo::bar()
This feature is particularly useful for processing logs or error messages that contain several mangled names.
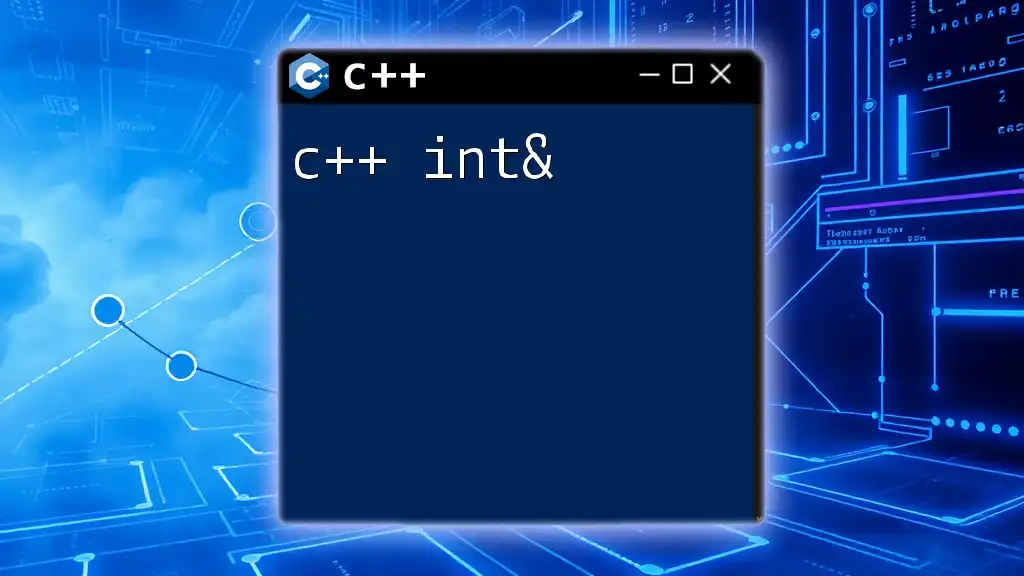
Advanced Features of c++filt
Additional Command-Line Options
c++filt offers several command-line options to enhance its usability. Some common options include:
- `-g`: Suppress the output of the internal demangling symbols.
- `-n` or `--no-demangle`: Prevents any demangling of names, outputting them as-is.
Using these options can tailor the output to your specific debugging or analysis needs. For instance:
c++filt -g <mangled_name>
Integrating c++filt into Build Processes
It’s often advantageous to include c++filt in your build processes, making it easier to identify issues during compilation. For example, in a Makefile, you might include a target to demangle names after building your program:
demangle: my_program
@echo "Demangling function names..."
c++filt < mangled_names.txt > demangled_names.txt
This ensures that every time you build your program, you also get the demangled output.
Using c++filt with Other Tools
c++filt can complement debugging tools like gdb. When gdb encounters a mangled name, you can use c++filt directly in your debugging session. For instance:
(gdb) print _Z7myFunctioni
You would receive an output indicating this is a function call, and using c++filt will clarify the signature further.
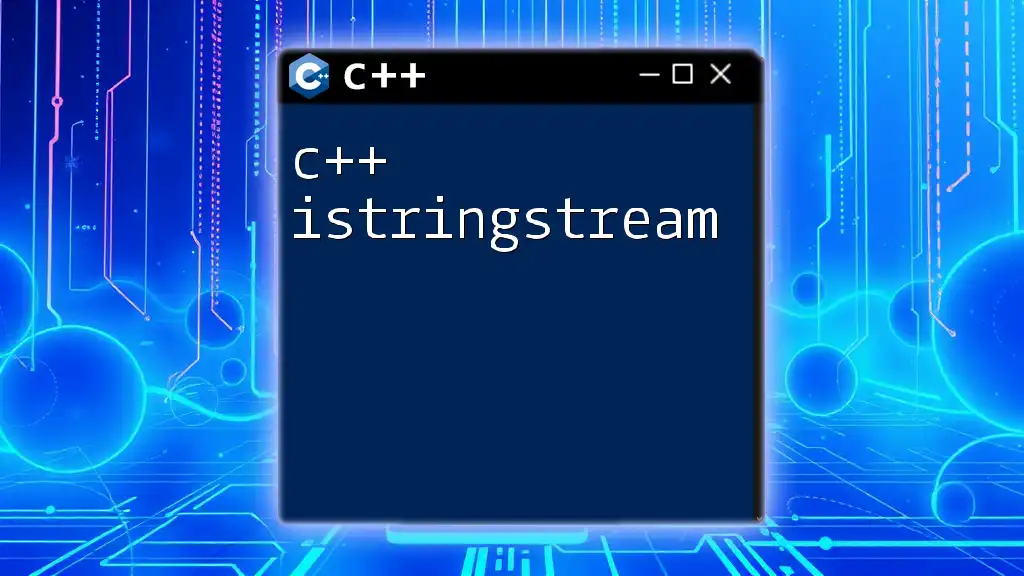
Troubleshooting Common Issues
Common Demangling Problems
While c++filt is generally reliable, there are instances where it may not perform as expected:
- Invalid Mangled Names: If the mangled name is incorrectly formatted, c++filt will return an error.
- Compiler-specific Mangling: Different compilers (GCC vs. Clang) might have different mangling strategies, leading to confusion if names were generated across compiler boundaries.
Solutions and Tips
- Verify Mangled Names: Double-check the mangled names from the output you received to ensure they were copied correctly.
- Consult Compiler Documentation: If you’re encountering consistent issues, refer to your compiler’s documentation for specifics on its name mangling schemes.
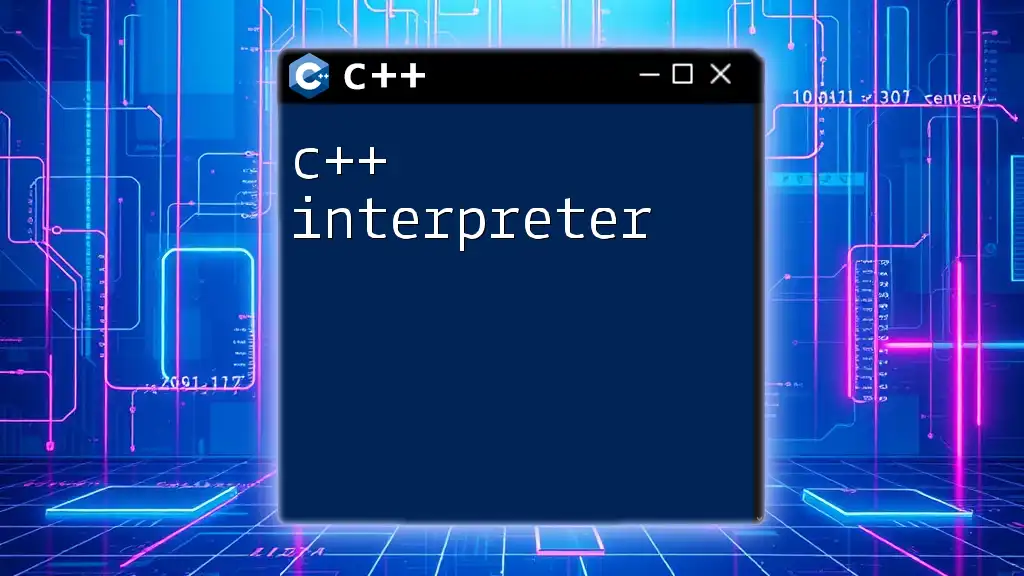
Conclusion
The c++filt tool significantly simplifies the process of debugging C++ programs by allowing developers to demangle complex function and class names easily. By understanding the importance of name demangling and employing c++filt effectively, you can streamline your debugging efforts and enhance your programming efficiency.
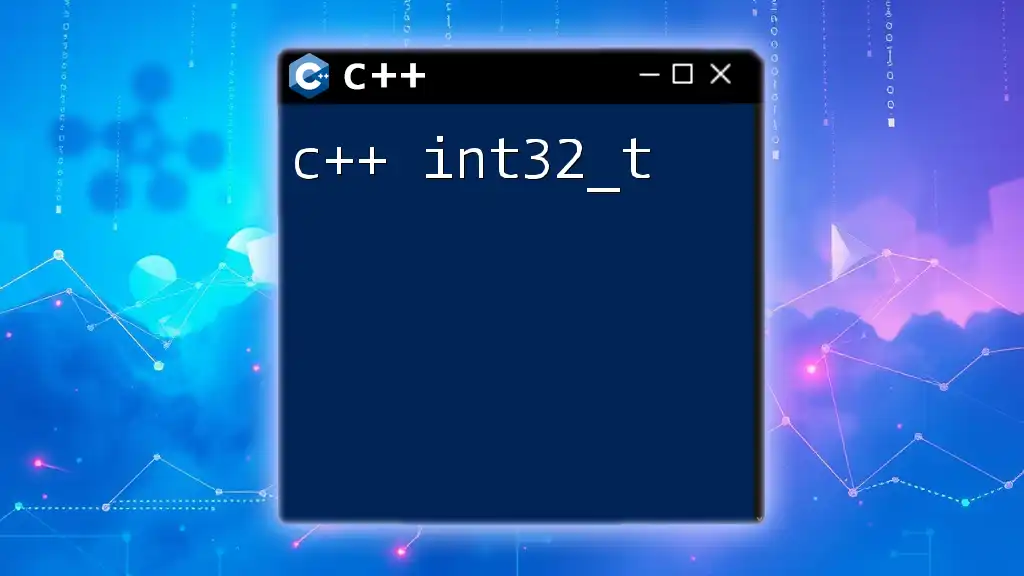
Additional Resources
For those looking to deepen their understanding of c++filt, consider exploring the official GNU documentation and tutorials available online. These resources provide great insights into additional features and advanced usage scenarios.
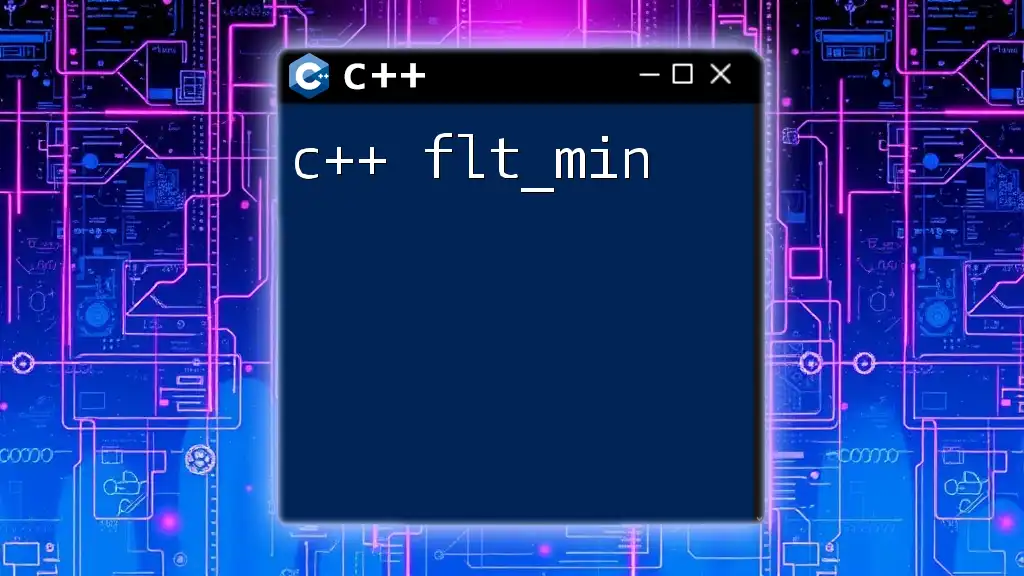
FAQs
What is the difference between c++filt and other filtering tools? c++filt is specialized for C++ name demangling, while other filtering tools may not support the complexities associated with C++ name mangling.
Can c++filt be used with other programming languages? c++filt is specifically designed for C++ and may not function correctly with mangled names from other languages.
Where can I find more examples of using c++filt? Documentation provided within the GNU Binutils package and various online C++ programming resources offer practical examples and usage scenarios.