The `int main` function serves as the entry point of a C++ program, where execution begins, and it must return an integer value to indicate the program's execution status.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is `int main`?
The `int main` function serves as the starting point for every C++ program. Every time a C++ application is executed, control is given to this function first. This makes it critical to understand its structure and role.
Importance of `int main` in C++ Programs
The `main` function dictates how the program begins executing. It signals to the operating system that this is where your application's logic starts and ends. Without a properly defined `main`, the compiler won't know where to begin, leading to compilation errors.
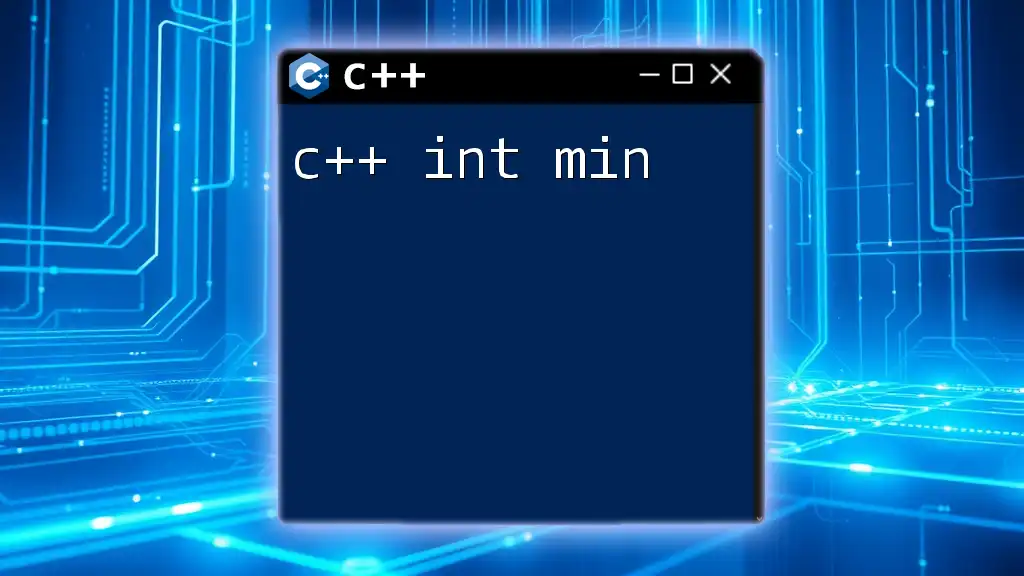
The Role of `main` in C++
Structure of a C++ Program
A basic C++ program consists of several components:
- Preprocessor directives (like `#include`)
- The `main` function itself
- Code within the `main` to perform actions
Take a look at a minimal example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Breakdown of the Example of `int main`:
- `#include <iostream>`: This directive includes the input/output stream library necessary for using `std::cout`.
- `int main()`: This defines the entry point of the program. It returns an integer to the operating system upon completion.
- `std::cout << "Hello, World!"`: This line prints "Hello, World!" to the console.
- `return 0;`: Signals that the program finished successfully.
Why `int` as a Return Type?
The return type `int` is important because it provides feedback to the operating system about the program’s execution status. By convention, returning `0` means successful execution, while returning a non-zero value indicates an error. This allows other programs, scripts, or users to determine if your program ran without issues.
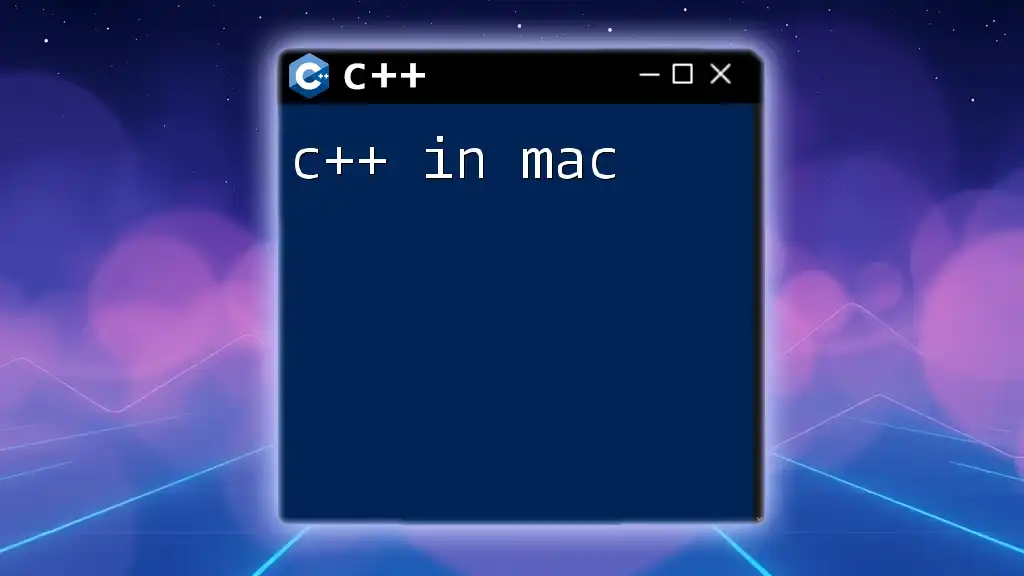
Basic Structure of a C++ Program with `int main`
Minimal Example of `int main`
To further clarify how `int main` operates, let’s examine a more straightforward program:
#include <iostream>
int main() {
// Your code here
return 0;
}
Here, while the body comment indicates where to place your code, the structure remains intact. Always ensure that a return statement is included to signify the function's completion.
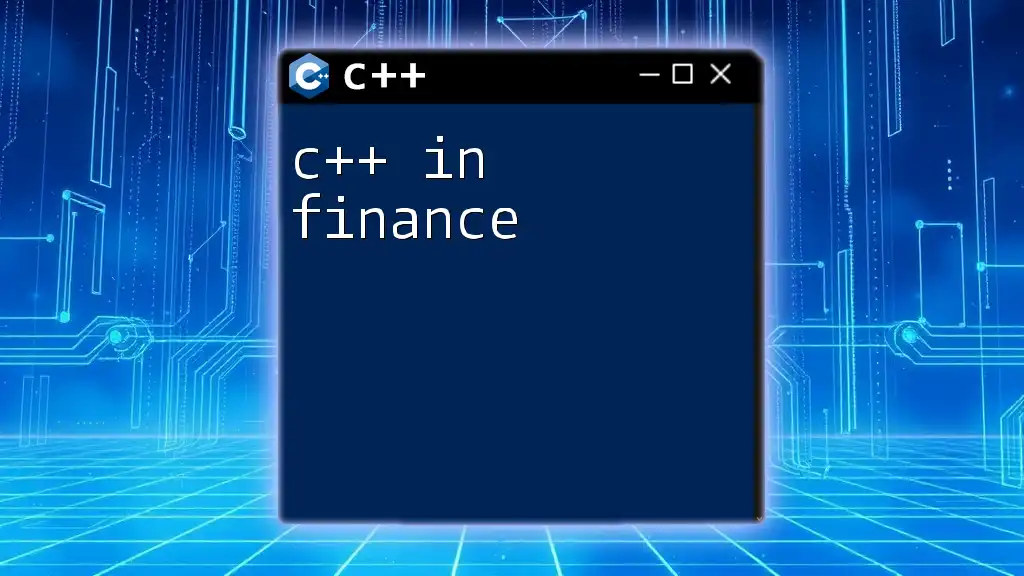
Return Values and Their Meanings
Standard Return Values
The return value of `main` can communicate the status of a program’s execution. The most common scenario is returning:
- `0`: Indicates success.
- Any other integer (e.g., `1`, `-1`, `255`, etc.): Typically indicates an error.
Custom Return Codes
While adhering to standard practices is important, sometimes you might need to inform the user about specific error states. Here’s an example scenario:
#include <iostream>
int main() {
int userInput;
std::cout << "Enter a number (0 to quit): ";
std::cin >> userInput;
if (userInput < 0) {
return 1; // Error: Negative number entered
}
return 0; // Success
}
In this example, the program communicates a specific error state if a negative number is provided.
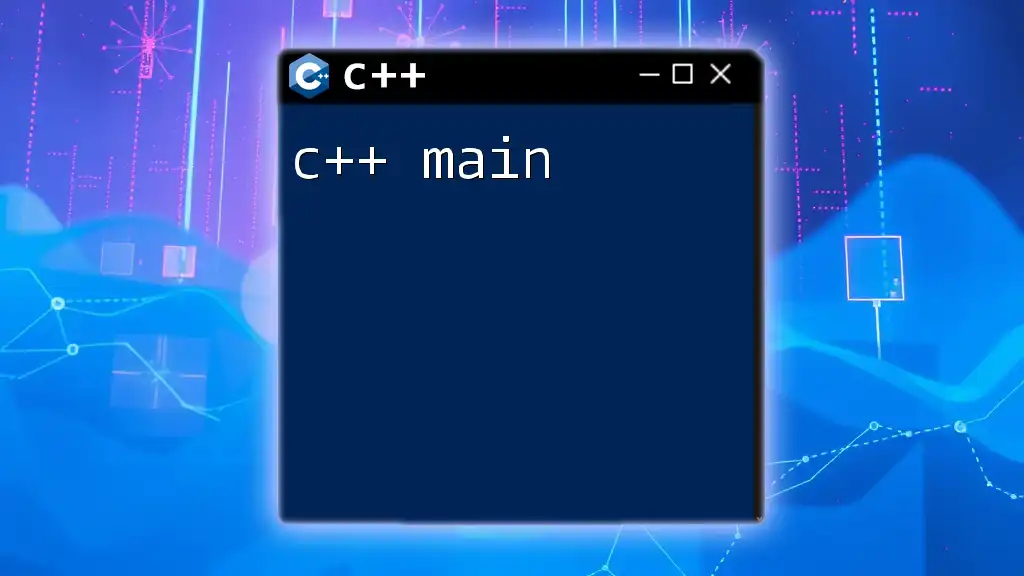
Enhanced `int main` Configurations
Using Command-Line Arguments
The `main` function can also accept command-line arguments, which allows for dynamic input during program execution. The declaration looks like this:
int main(int argc, char* argv[])
- `argc`: The number of command-line arguments.
- `argv`: An array of C-style strings representing each command-line argument.
Here’s a code snippet demonstrating how to access these arguments:
#include <iostream>
int main(int argc, char* argv[]) {
std::cout << "Number of arguments: " << argc << std::endl;
for (int i = 0; i < argc; ++i) {
std::cout << "Argument " << i << ": " << argv[i] << std::endl;
}
return 0;
}
In this version of `main`, the program iterates through the provided command-line arguments, printing each one along with its index, showcasing the power of `int main`.
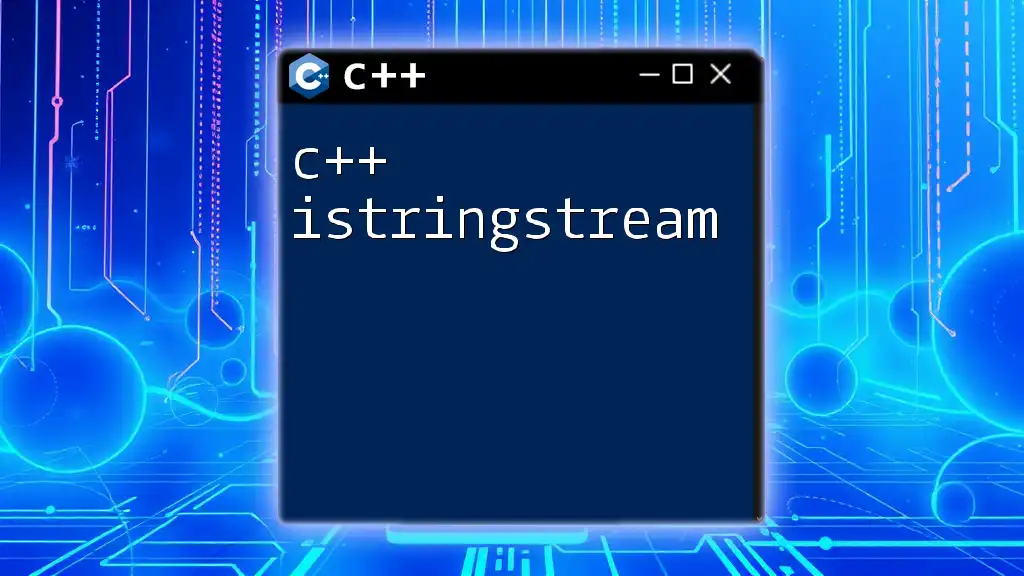
Best Practices for Writing `int main`
Clarity and Simplicity
It’s crucial to keep the `main` function clear and simple. The `main` function should do just enough to initiate your program's logic. Avoid cluttering it with too many responsibilities; instead, delegate specific tasks to other functions.
Avoiding Global Variables
While global variables can be useful, overusing them might lead to complex and hard-to-manage code. Keeping your variables local to functions (including `main`) enhances readability and prevents unexpected side effects.
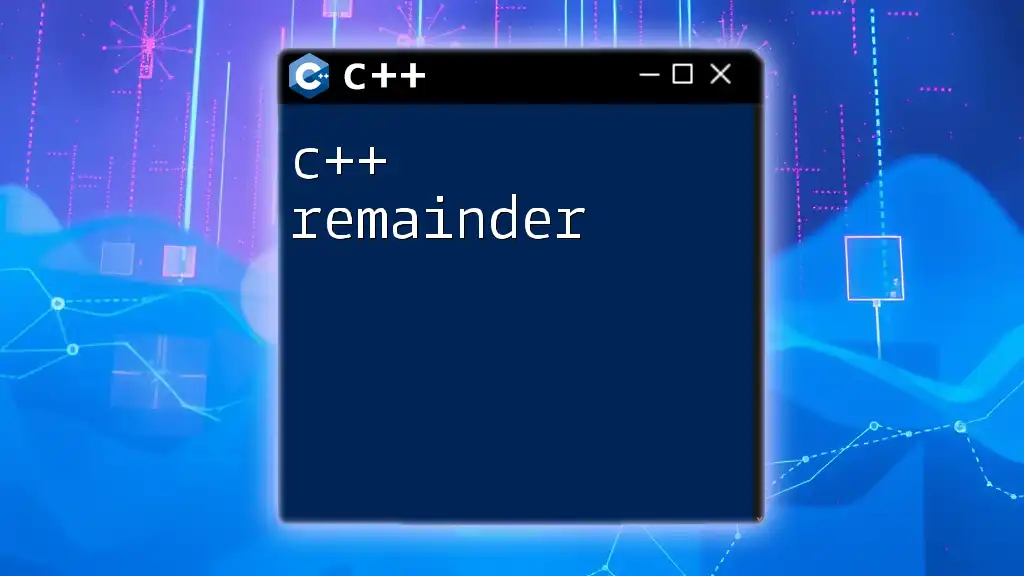
Common Mistakes to Avoid with `int main`
Incorrect Return Types
Always use `int` as the return type for `main`. Using `void main()` or another non-integer type can lead to undefined behavior in your application, affecting its reliability and compatibility across different systems.
Omitting the Return Statement
In C++, not returning an integer from `main` can yield warnings and unexpected behavior. Even if a return statement is technically optional in some modern compilers, it’s best to adhere to good programming practices by explicitly returning values.
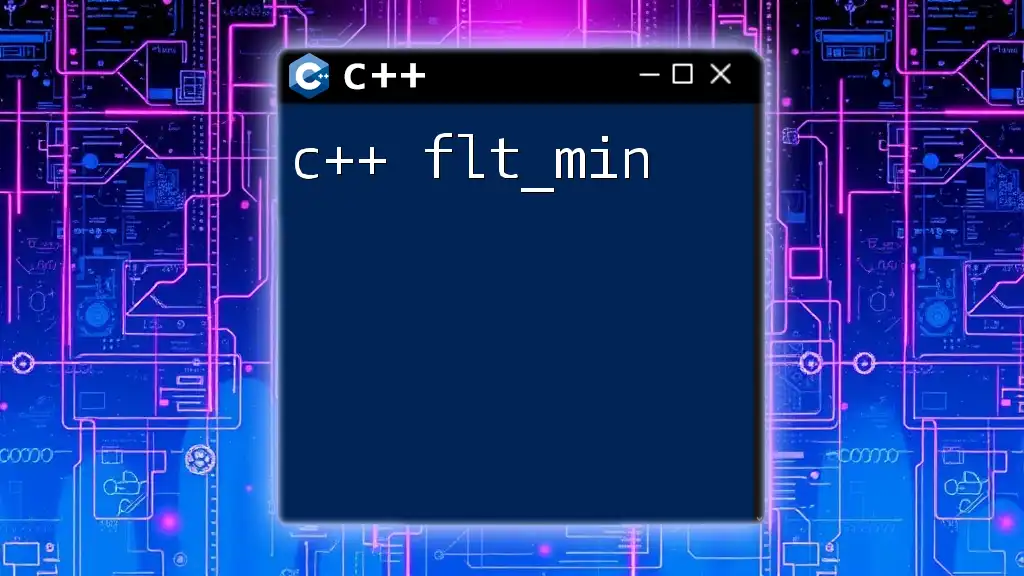
Advanced Concepts Related to `int main`
Multiple `main` Functions
In larger projects with multiple files, a clear structure becomes essential. You might need different `main` functions for testing various parts of your application. Keep in mind that only one `main` function can exist in any single compilation unit.
Overloading `main`
Although you can technically overload `main`, it’s not standard practice and generally leads to confusion. The typical declaration of `main` should always maintain its standard signature; unconventional declarations can introduce compatibility problems and complications in maintaining your code.
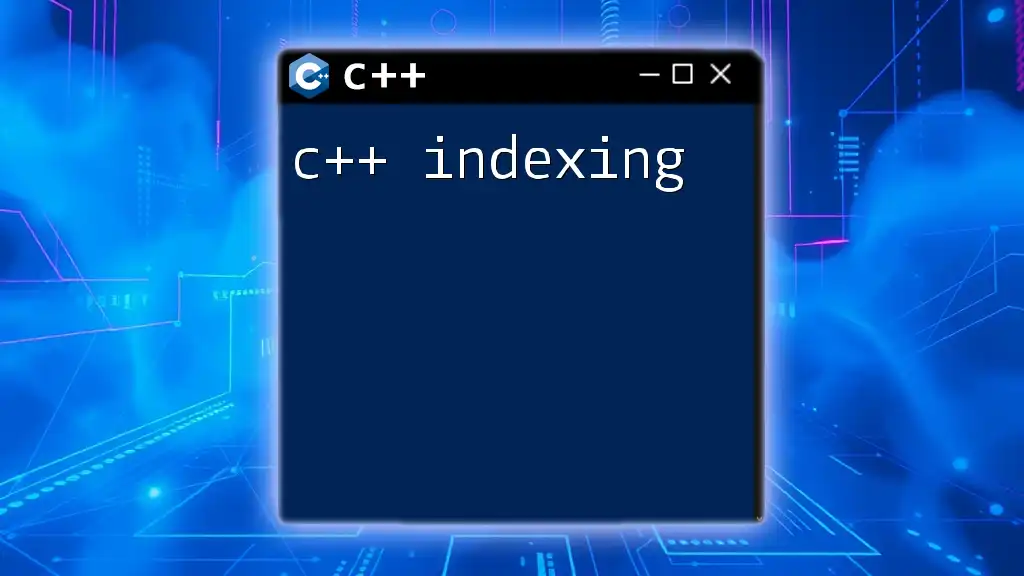
Recap of Key Points
We’ve explored the crucial aspects of the `int main` function, including its role as the entry point of C++ programs, the significance of return values, and the benefits of keeping the function clear and organized.
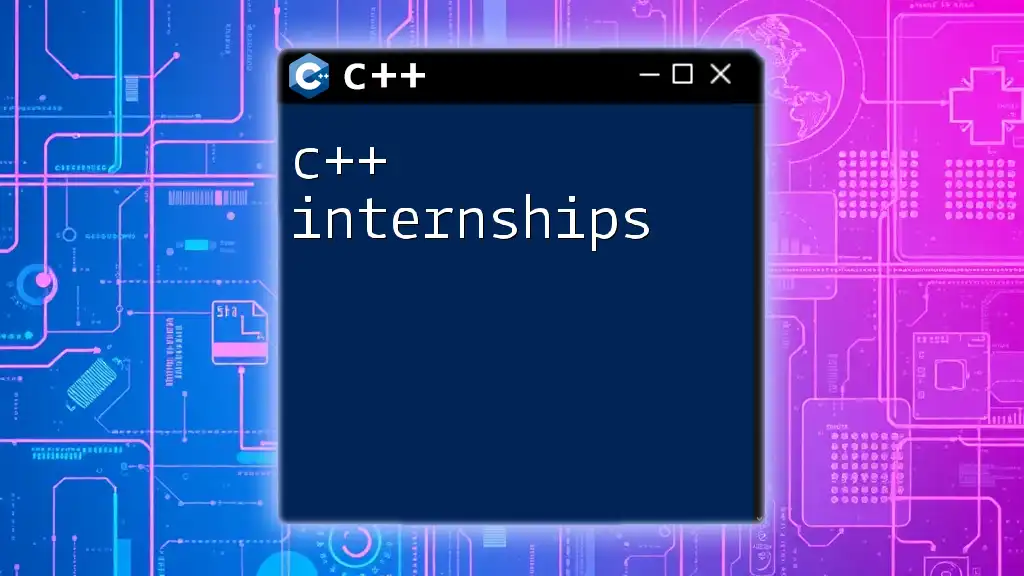
Encouragement to Experiment
Take these concepts to heart and practice writing your `int main` functions! Every time you code, experiment with different inputs, outputs, and features. Your understanding will grow as you engage more deeply with C++. Remember, the only way to truly become proficient is through practice.
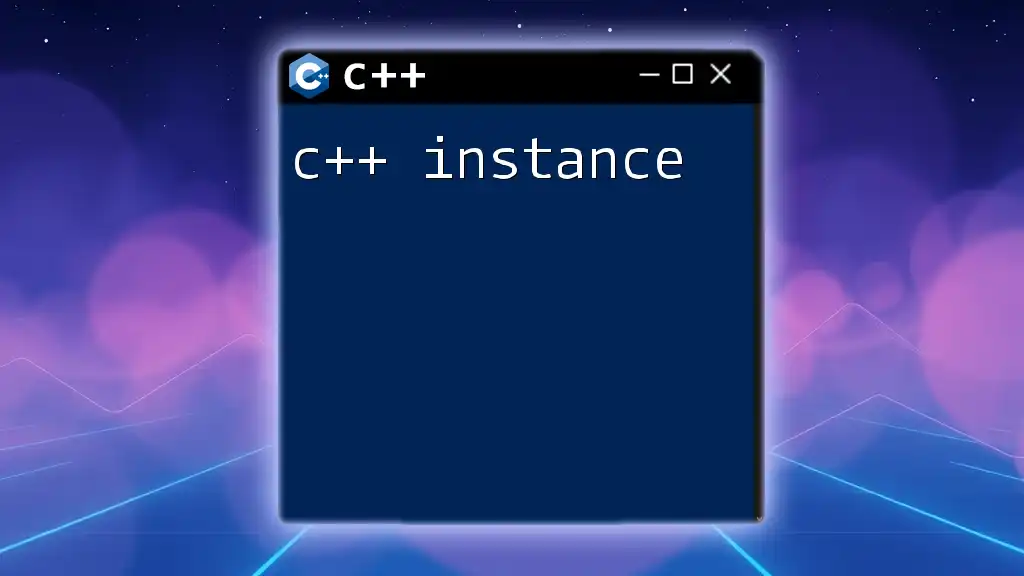
Frequently Asked Questions (FAQs)
What Happens If I Don’t Return an Integer in `main`?
Failing to return an integer from `main` could lead to compiler warnings. It’s also a good habit to keep the function consistent with its expected behavior to avoid platform-specific issues.
Why Do Some C++ Programs Use `void main()`?
Using `void main()` is not standard and can lead to unpredictable results. Adhering to the `int main` format ensures better compatibility and provides feedback on program execution.
Can I Write More Than One `main` Function?
Though you can technically define multiple `main` functions across different files in larger projects, it’s best practice to maintain a single `main` in each compiled unit. This avoids ambiguity when building and running your applications.
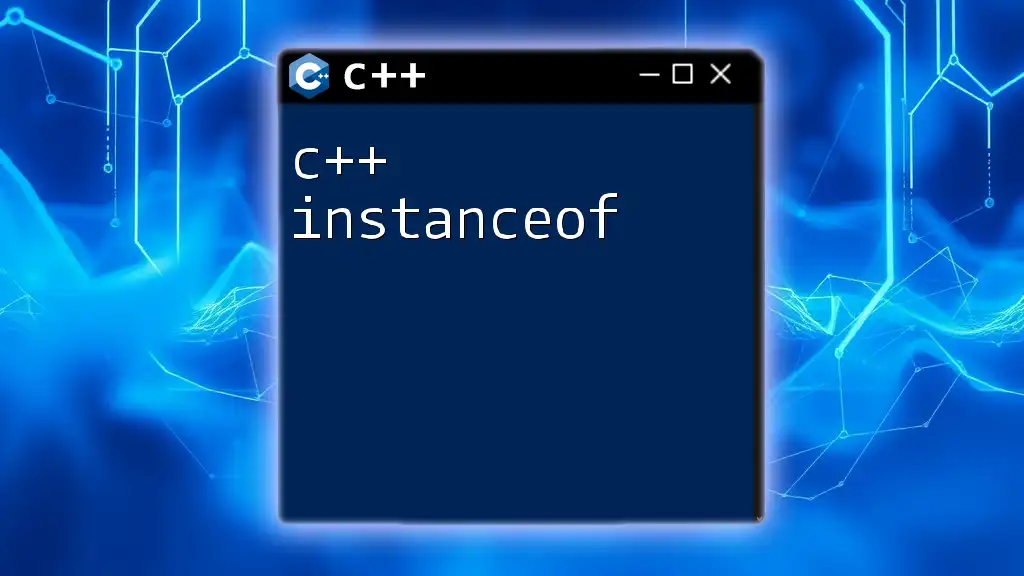
References and Further Reading
To delve deeper into the C++ programming language and the intricacies of the `int main` function, consider checking out dedicated C++ programming books, online courses, or community forums. Your journey to mastering this vital aspect of programming continues!