C++ is widely utilized in the finance sector for its performance efficiency and ability to handle complex calculations quickly, making it ideal for high-frequency trading and risk management systems.
Here’s a simple code snippet demonstrating how to calculate the compound interest, a common financial calculation, using C++:
#include <iostream>
#include <cmath>
int main() {
double principal = 1000.0; // Initial investment
double rate = 0.05; // Annual interest rate
int time = 10; // Number of years
double compoundInterest = principal * pow((1 + rate), time);
std::cout << "Compound Interest: " << compoundInterest << std::endl;
return 0;
}
Understanding C++’s Role in Finance
What Makes C++ Ideal for Finance?
C++ is deeply embedded in the finance sector due to its unique capabilities. Performance stands out as a key attribute; being a compiled language, it allows programs to execute faster than interpreted languages. This speed is particularly critical in environments like algorithmic trading, where decisions must be made in microseconds.
C++ also offers a robust way to handle precision, which is vital in finance where even small inaccuracies can lead to significant financial losses. The language supports various data types and complex calculations, making it suitable for financial simulations, risk assessments, and complex algorithms.
Additionally, C++'s rich libraries such as QuantLib and Boost provide extensive resources specifically designed for financial applications. These libraries offer functionalities ranging from quantitative finance to financial derivatives, greatly enhancing productivity and efficiency for developers.
Common Use Cases in Finance
C++ shines in multiple facets of finance, with some notable use cases including:
- Algorithmic Trading: Building high-frequency trading systems that rely on complex algorithms to execute trades at optimal times.
- Risk Management: Utilizing simulation techniques to evaluate and minimize financial risks.
- Financial Modeling: Developing models that inform decisions regarding asset pricing, interest rates, and portfolio management.
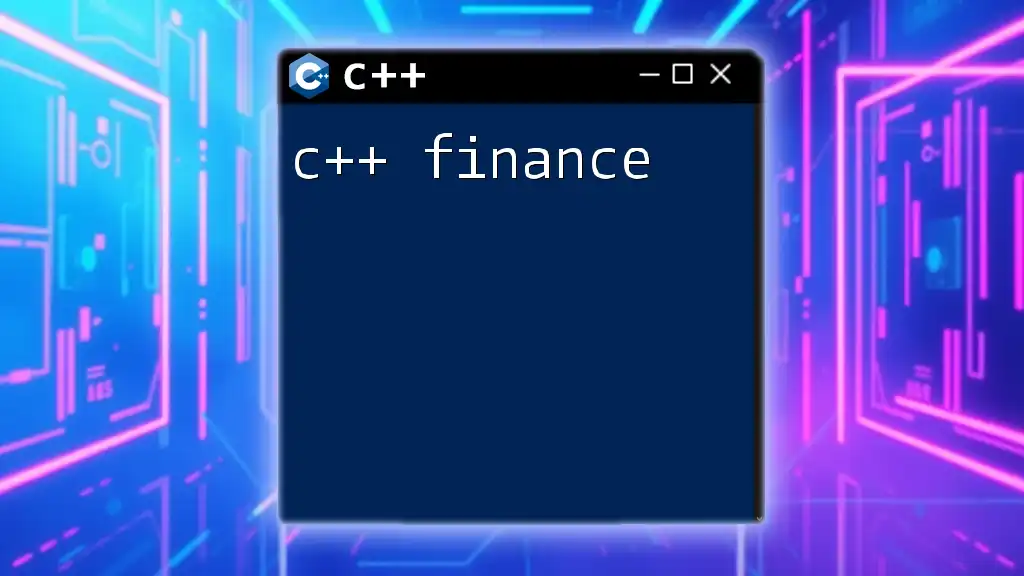
Setting Up a C++ Development Environment
Installing a C++ Compiler
To dive into C++ in finance, the first step is to set up a suitable development environment. One needs to install a C++ compiler. Commonly used compilers include GCC, Visual Studio, and MSVC.
To provide a quick reference, here’s how to install g++ on a Debian-based system:
# For Debian-based systems, install g++
sudo apt install g++
Recommended Development Tools
Choosing the right development tools can substantially enhance your coding experience. Integrated Development Environments (IDEs) such as Visual Studio, Code::Blocks, and Eclipse provide useful features like debugging, code completion, and project management. Implementing a debugger, such as GDB, can also help identify and resolve issues effectively during development.
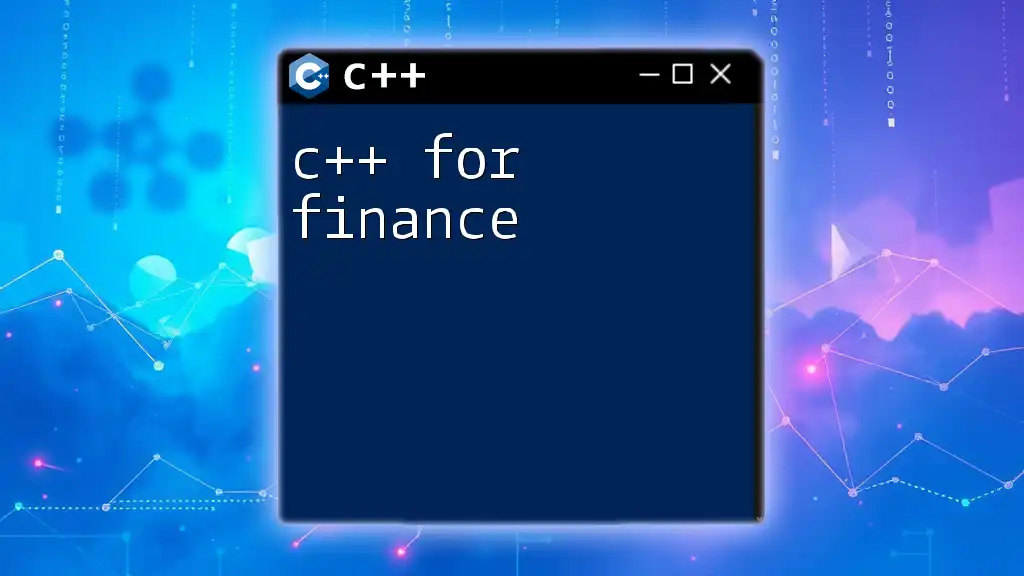
Core C++ Concepts for Financial Applications
Object-Oriented Programming (OOP) in Finance
One of the cornerstones of C++ is its support for Object-Oriented Programming (OOP). This paradigm is particularly useful in finance for modeling complex financial instruments and maintaining data integrity through encapsulation.
To illustrate, consider a basic class for a financial instrument:
class FinancialInstrument {
public:
double price;
double quantity;
FinancialInstrument(double p, double q) : price(p), quantity(q) {}
double getValue() {
return price * quantity;
}
};
This example highlights how C++ can represent a financial product through properties (price and quantity) and methods (calculating total value).
Templates and Generic Programming
Another compelling feature of C++ is the ability to use templates, which allow developers to create generic functions and classes. This promotes code reusability and enhances type safety.
Here’s a simple example of a template function for calculating the value of a financial transaction:
template<typename T>
T calculateValue(T price, T quantity) {
return price * quantity;
}
This function can handle various data types, making it versatile for different financial calculations.
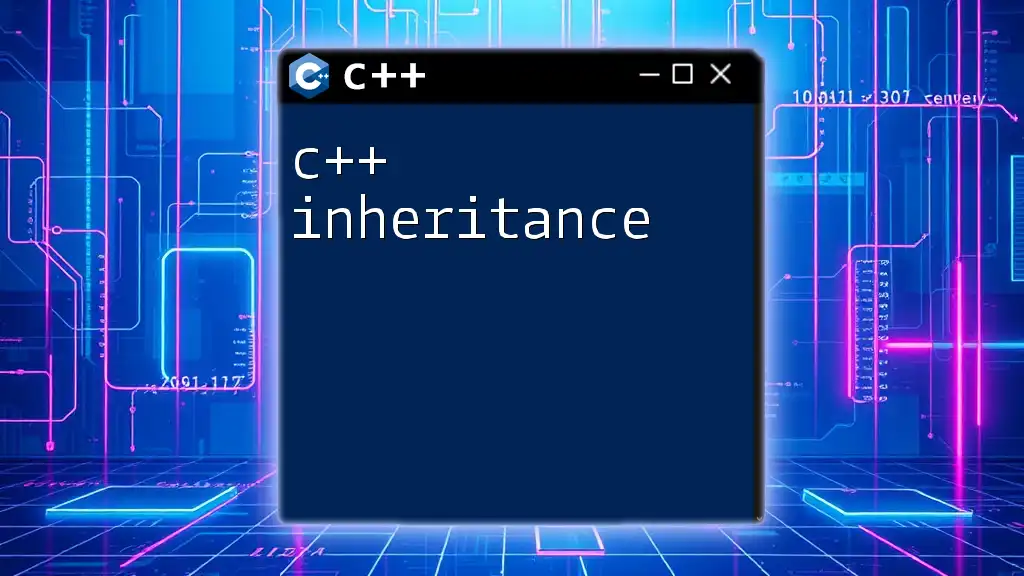
Advanced C++ Techniques in Finance
Using STL (Standard Template Library)
The Standard Template Library (STL) is a powerful ally in C++ in finance. It provides a range of data structures like vectors, lists, and maps, which can streamline data management and retrieval processes.
For instance, using vectors to store stock prices allows for efficient data handling:
#include <vector>
std::vector<double> stockPrices = {100.0, 102.5, 101.0};
double averagePrice(const std::vector<double>& prices) {
double sum = 0;
for (double price : prices) {
sum += price;
}
return sum / prices.size();
}
With STL, managing dynamic data becomes easier, allowing financial analysts to focus more on analysis rather than implementation details.
Exception Handling for Robust Financial Software
In the finance industry, robust error handling is imperative to prevent catastrophic failures in applications. C++ offers structured exception handling to manage errors gracefully.
Consider the following example that demonstrates how to implement a basic exception handling mechanism:
class FinancialException : public std::exception {
const char* what() const noexcept override {
return "Financial error occurred!";
}
};
void processTransaction(double amount) {
if (amount < 0) {
throw FinancialException();
}
// Transaction processing logic
}
With such a structure, the code can respond effectively to unexpected inputs or errors, ensuring safer financial operations.
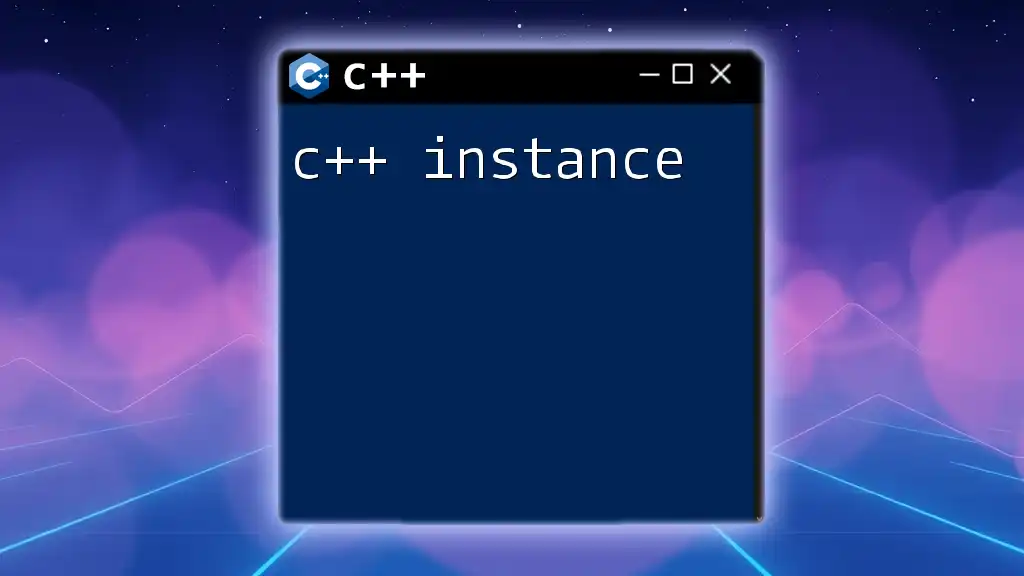
Practical Applications of C++ in Finance
Building a Simple Stock Price Tracker
Let’s put theory into practice by outlining how to build a simple stock price tracker. This application allows users to add stock prices and calculate their average, demonstrating foundational C++ techniques.
The overall architecture involves defining classes for the tracker and implementing relevant functions. Basic functionalities include:
- Adding stock prices to a vector.
- Calculating and retrieving the average stock price.
Here’s a structural overview of the code:
class StockTracker {
private:
std::vector<double> prices;
public:
void addPrice(double price) {
prices.push_back(price);
}
double getAverage() {
return averagePrice(prices);
}
};
This code snippet illustrates a straightforward approach to managing stock prices, paving the way for more complex financial applications.
Implementing a Basic Risk Assessment Tool
To further bolster your skills in C++ in finance, developing a basic risk assessment tool can greatly enhance understanding. This tool can analyze volatility and provide insights into potential risks using historical financial data.
Key elements of the tool include:
- Data Collection: Use various methods to fetch and store historical stock prices.
- Risk Metrics Calculation: Implement calculations of Value at Risk (VaR) and standard deviation to analyze and quantify asset risks.
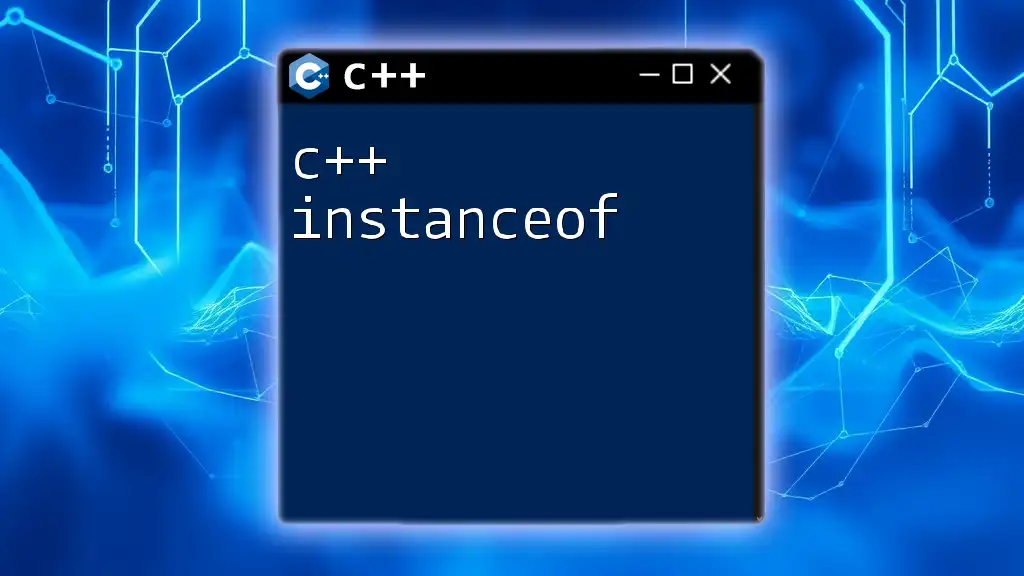
Conclusion
Throughout this guide, we have explored the manifold applications of C++ in finance and how its unique features cater specifically to the needs of the financial sector. The performance, precision, and libraries available in C++ make it an excellent choice for developing software solutions that drive financial decision-making.
Looking forward, as financial technology continues to evolve, the relevance of C++ in fintech and algorithmic trading is likely to expand, offering exciting new opportunities for those willing to invest their time in mastering this language.
Engagement is the essence of growth. If you feel inspired, we encourage you to share your projects, queries, and experiences of utilizing C++ in finance, fostering an enriching community of learners and innovators.
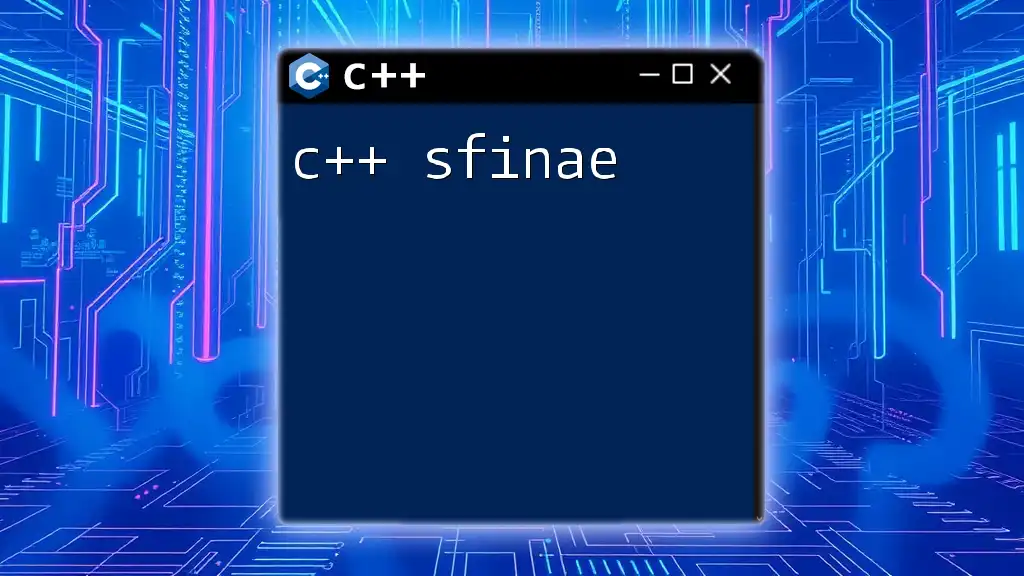
Additional Resources
Books and Online Courses
Consider diving deeper with recommended literature and online platforms that specialize in C++ and its applications in finance.
Communities and Forums
Engage with developer communities and forums to exchange ideas, challenges, and solutions in the domain of C++ in finance.
Seeking knowledge is a continual journey; let’s embark on it together!