C++ can be utilized in finance for tasks such as modeling financial instruments, risk analysis, and algorithmic trading due to its performance and efficiency.
Here's a simple code snippet that calculates the compound interest, which is often used in financial calculations:
#include <iostream>
#include <cmath>
double compoundInterest(double principal, double rate, int time) {
return principal * pow((1 + rate / 100), time);
}
int main() {
double principal = 1000.0; // initial amount
double rate = 5.0; // annual interest rate
int time = 10; // time in years
std::cout << "Compound Interest: " << compoundInterest(principal, rate, time) << std::endl;
return 0;
}
What is C++ Finance?
C++ finance refers to the utilization of the C++ programming language for developing financial applications and models. This discipline encompasses a range of tasks, from algorithmic trading systems to risk management frameworks. By leveraging C++, developers can create high-performance solutions that are critical in the fast-paced financial environment.
Why Use C++ for Financial Applications?
Performance and Speed
C++ is renowned for its superior performance and low-latency capabilities, making it ideal for high-frequency trading (HFT) applications where milliseconds can mean significant financial gains or losses. The language provides access to low-level system resources, which enables programmers to optimize their code for maximum efficiency.
Memory Management
C++ offers direct control over memory allocation and management. This feature is particularly advantageous in financial applications where managing large datasets, such as market histories and price feeds, is essential. For example, memory can be dynamically allocated when needed and released when no longer required, vastly improving performance.
Here is a simple example demonstrating dynamic memory allocation in C++:
#include <iostream>
int main() {
double* portfolioAllocations = new double[10]; // Allocate memory for 10 allocations
// Use the allocated memory here...
delete[] portfolioAllocations; // Free the memory
return 0;
}
Complex Financial Algorithms
The library ecosystem in C++ is robust, featuring dedicated libraries like QuantLib that aid in financial computations, providing a vast array of functions to solve complex financial problems efficiently. These libraries simplify tasks like option pricing or fixed income analytics that would otherwise be cumbersome to implement from scratch.
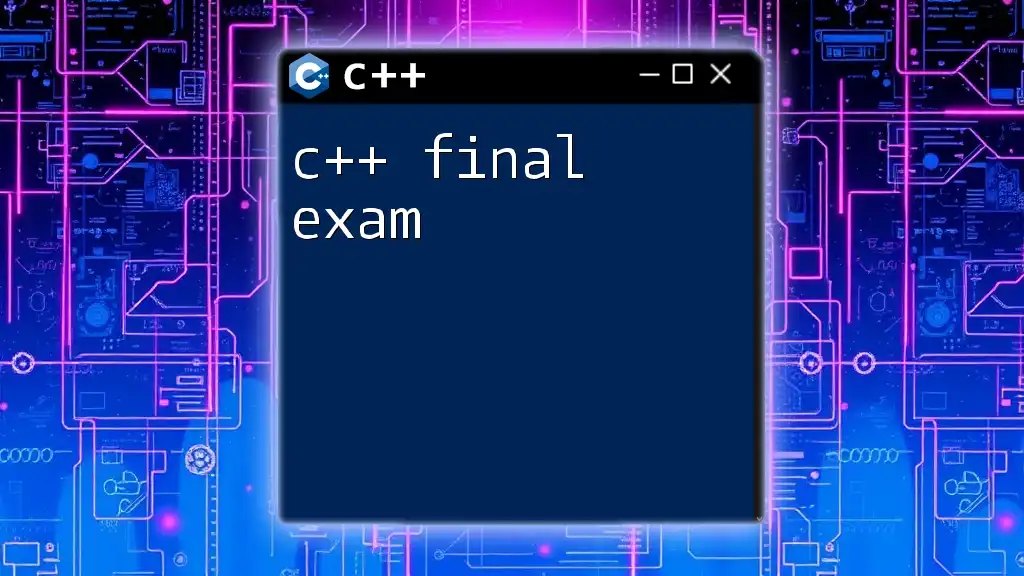
Core Concepts of C++ in Finance
Data Types and Structures
Using Standard Data Types
Understanding fundamental data types is vital for effective financial computations. Floating-point types, such as `float` and `double`, are frequently used for calculations involving prices, returns, and interest rates. Correct usage of these types ensures that precision is maintained during calculations.
Complex Data Structures
C++ provides a rich set of data structures that can be leveraged to create sophisticated financial models. For instance, using a `std::map` allows developers to maintain portfolio holdings indexed by asset types:
#include <iostream>
#include <map>
#include <string>
int main() {
std::map<std::string, double> portfolio;
portfolio["AAPL"] = 150.0; // Share price for Apple
portfolio["GOOGL"] = 2800.0; // Share price for Google
// Iterate through portfolio
for (const auto& asset : portfolio) {
std::cout << "Asset: " << asset.first << " Price: $" << asset.second << std::endl;
}
return 0;
}
Object-Oriented Programming in Finance
Classes and Objects
Encapsulating financial instruments as classes helps manage complexity. For instance, a simple stock class can be implemented to represent key properties:
#include <iostream>
#include <string>
class Stock {
private:
std::string name;
double price;
public:
Stock(std::string n, double p) : name(n), price(p) {}
double getPrice() const { return price; }
void setPrice(double newPrice) { price = newPrice; }
void display() const {
std::cout << "Stock: " << name << ", Price: $" << price << std::endl;
}
};
Inheritance and Polymorphism
C++’s support for inheritance and polymorphism fosters flexible code design. For instance, financial instruments such as stocks and bonds can inherit from a base class:
class FinancialInstrument {
public:
virtual void display() const = 0; // Pure virtual function
};
class Bond : public FinancialInstrument {
private:
double yield;
public:
Bond(double y) : yield(y) {}
void display() const override {
std::cout << "Bond Yield: " << yield << "%" << std::endl;
}
};
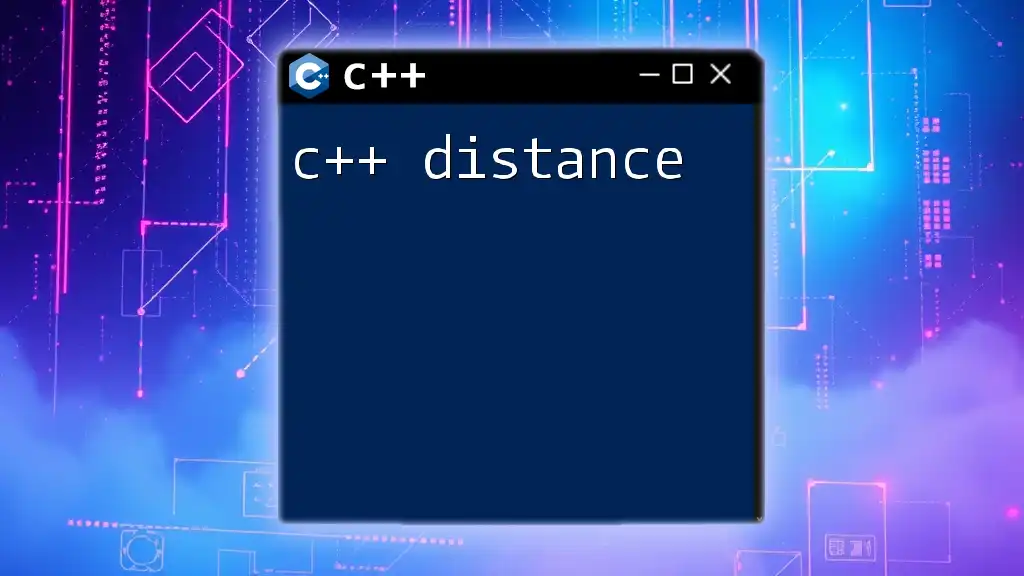
Financial Model Development
Time Value of Money
Understanding Present and Future Value
The concept of time value of money (TVM) is fundamental in finance. It expresses the idea that a dollar today is worth more than a dollar in the future due to its potential earning capacity.
The formula for calculating future value (FV) is:
\[ FV = PV \times (1 + r)^n \]
Where:
- PV = Present Value
- r = interest rate
- n = number of periods
Here’s how you can implement this in C++:
#include <cmath>
#include <iostream>
double calculateFutureValue(double presentValue, double rate, int years) {
return presentValue * pow(1 + rate, years);
}
Annuities and Loans
An annuity is a series of equal payments made at regular intervals. Understanding how to calculate the present value of an annuity is essential. The formula for present value of an annuity is:
\[ PVA = PMT \times \frac{1 - (1 + r)^{-n}}{r} \]
Here’s a C++ function to calculate this:
double calculatePVA(double payment, double rate, int periods) {
return payment * (1 - pow(1 + rate, -periods)) / rate;
}
Risk Management Models
Value at Risk (VaR)
Value at Risk (VaR) is a quantitative measure of the risk of loss on an investment. A basic approach to estimate VaR is using Monte Carlo simulations, which assess portfolio risk based on historical data.
Here’s a simple snippet to demonstrate a Monte Carlo method for estimating VaR:
#include <vector>
#include <cstdlib>
#include <ctime>
double simulatePortfolioValue(const std::vector<double>& returns) {
// Simulate a portfolio value based on historical returns
double portfolioValue = 1000000; // Initial investment
for (const double& r : returns) {
portfolioValue *= (1 + r);
}
return portfolioValue;
}
Stress Testing and Scenarios
Stress testing evaluates how financial instruments respond under extreme conditions. One way to conduct stress tests in C++ is to simulate shocks to portfolio values by modifying market data inputs.
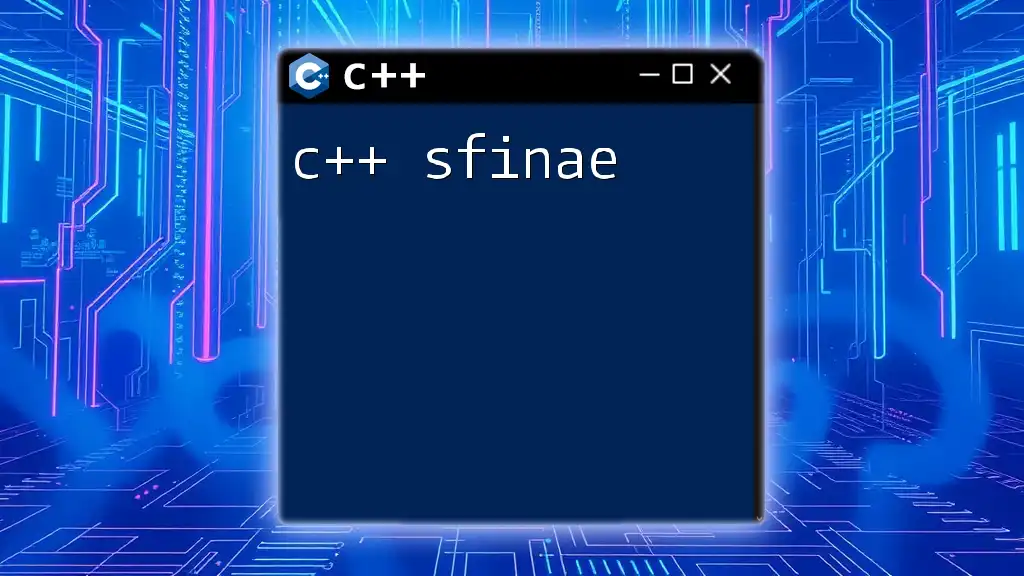
Advanced Techniques in C++ Finance
Using Libraries and Frameworks
Boost Libraries
Boost provides a variety of free, peer-reviewed libraries that extend C++. Its applications in finance include date/time calculations, financial time series manipulation, and statistical distributions.
QuantLib for Financial Derivatives
QuantLib is a comprehensive library that supports complex quantitative analyses. It plays a significant role in pricing financial derivatives and managing risk.
Here’s an example of how to use QuantLib for pricing a simple option:
#include <ql/quantlib.hpp>
// Code using QuantLib to price options goes here...
Concurrent Programming in Finance
Multithreading
In finance, the ability to run multiple threads concurrently is crucial for processing large datasets. C++ offers several methods for multithreading, including the `<thread>` library, allowing developers to build efficient systems for trading and risk management.
#include <iostream>
#include <thread>
void fetchMarketData(int thread_id) {
std::cout << "Fetching market data in thread: " << thread_id << std::endl;
// Fetching logic here...
}
int main() {
std::thread t1(fetchMarketData, 1);
std::thread t2(fetchMarketData, 2);
t1.join();
t2.join();
return 0;
}
Optimization Techniques
Using libraries like OpenMP can significantly enhance performance through parallel computing. Optimizing a portfolio simulation using OpenMP can reduce computation time dramatically.
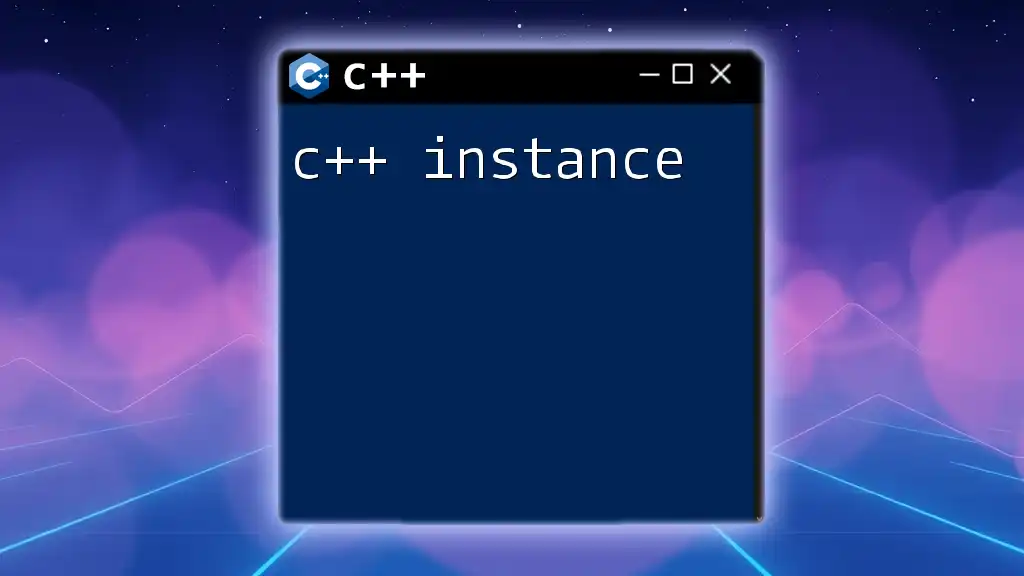
Real-World Applications of C++ in Finance
Algorithmic Trading
Building a Trading Bot
Algorithmic trading involves automated trading based on pre-defined criteria. The core components of a trading system include market data acquisition, signal generation, risk management, and execution.
Here’s a skeleton code structure for a trading bot:
class TradingBot {
public:
void run() {
// Code logic for the trading algorithm
}
};
Backtesting Strategies
Backtesting assesses the viability of a trading strategy by applying it to historical data. Implementing a backtesting framework in C++ allows traders to optimize their algorithms efficiently.
Risk Analytics Software
Tools for Risk Assessment
C++ is widely employed in developing risk analytics software like Value-at-Risk models and stress testing tools. The ability to handle complex calculations and large datasets makes C++ a preferred choice for financial institutions.
Case studies have shown that companies utilizing C++ for risk analytics can achieve enhanced risk assessment capabilities, minimizing potential losses during market downturns.
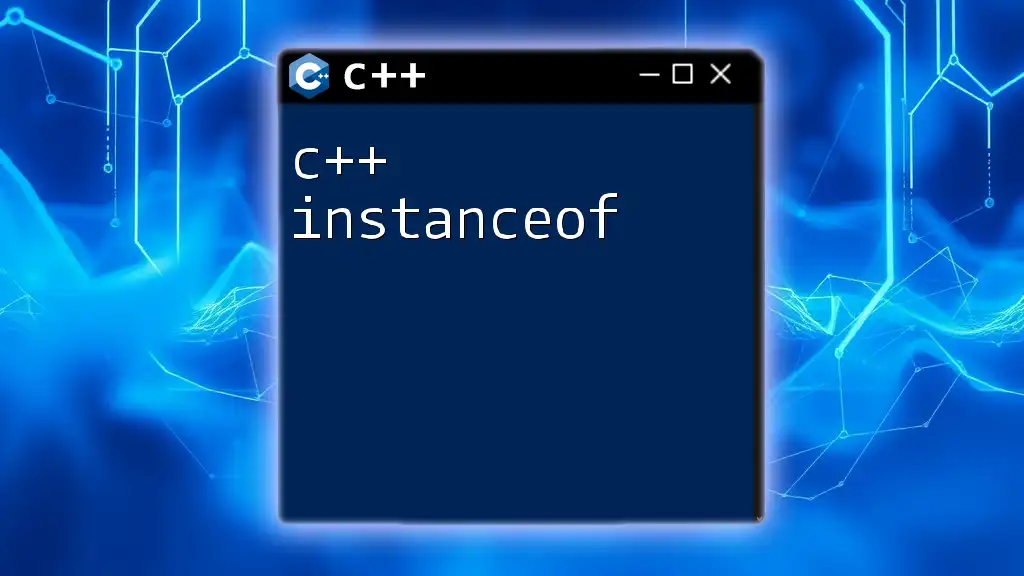
Learning Resources and Community
Recommended Books and Online Courses
Books on C++ for Finance
A selection of titles focusing on C++ in finance can provide valuable insights and depth. Look for publications that address both theoretical and practical applications. Recommended authors include Paul Wilmott, and Sergio M. Focardi.
Community and Forums
Where to Connect with Other C++ Finance Developers
Engaging with communities like Stack Overflow or Reddit's r/cpp and r/quant can facilitate networking and learning. Collaborating with peers enhances knowledge and can lead to opportunities in the financial tech space.
Attending Meetups and Conferences
Conferences and meetups focused on C++ or finance tech provide platforms for discussion, learning, and collaboration. These events can introduce you to industry professionals and leaders in financial technology.
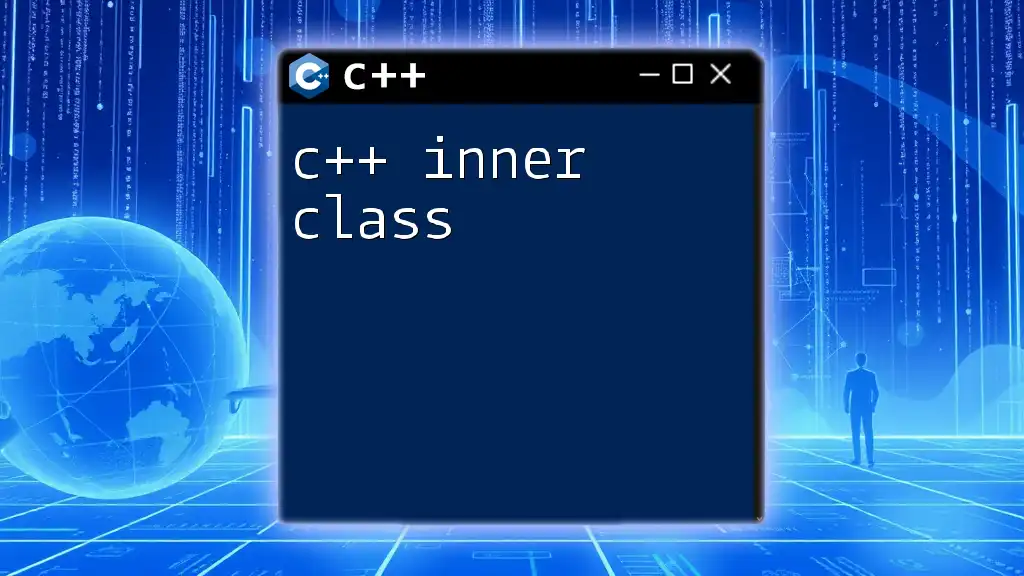
Conclusion
In summary, C++ finance represents the intersection of programming and financial theory, enabling the development of advanced, performance-oriented solutions. Mastering C++ can significantly enhance your capabilities in the finance sector, from real-time trading systems to comprehensive risk management frameworks.
As you journey into C++ finance, continually explore new resources, engage with communities, and experiment with practical projects to solidify your understanding and skills.