In C++, the distance between two iterators can be calculated using the `std::distance` function from the `<iterator>` header, which returns the number of elements between them.
#include <iostream>
#include <vector>
#include <iterator>
int main() {
std::vector<int> vec = {10, 20, 30, 40, 50};
auto start = vec.begin();
auto end = vec.end();
std::cout << "Distance: " << std::distance(start, end) << std::endl;
return 0;
}
Understanding the Concept of Distance in C++
In C++, the term distance usually refers to the measurement between two points. This concept is fundamental in various applications, from geometry to data patterns and even real-world scenarios like navigation systems. Understanding distance is crucial for programmers, as it influences algorithms and data handling.
Types of Distance Calculations
Euclidean Distance
Euclidean distance is a well-known way to determine the straight-line distance between two points in Euclidean space. The formula to calculate Euclidean distance is given as follows:
$$ d = \sqrt{(x_2 - x_1)^2 + (y_2 - y_1)^2} $$
To implement this in C++, you can utilize the standard library's mathematical functions. Below is a simple example of how to calculate Euclidean distance:
#include <cmath>
#include <iostream>
// Function to calculate Euclidean distance
double euclideanDistance(double x1, double y1, double x2, double y2) {
return std::sqrt(std::pow(x2 - x1, 2) + std::pow(y2 - y1, 2));
}
// Example usage
int main() {
double dist = euclideanDistance(1.0, 2.0, 4.0, 6.0);
std::cout << "Euclidean Distance: " << dist << std::endl;
return 0;
}
In this implementation, the Euclidean distance function calculates the distance based on the provided coordinates and utilizes the `sqrt` and `pow` functions for mathematical operations. Euclidean distance is particularly useful in scenarios where you need to determine the "as-the-crow-flies" distance, making it integral in game development and simulations.
Manhattan Distance
Conversely, Manhattan distance measures distance along axes at right angles. It is often used in grid-like pathfinding algorithms, such as in urban navigation systems. The formulation here is:
$$ d = |x_2 - x_1| + |y_2 - y_1| $$
Here’s how you can implement Manhattan distance in C++:
#include <iostream>
#include <cmath>
// Function to calculate Manhattan distance
double manhattanDistance(double x1, double y1, double x2, double y2) {
return std::abs(x2 - x1) + std::abs(y2 - y1);
}
// Example usage
int main() {
double dist = manhattanDistance(1.0, 2.0, 4.0, 6.0);
std::cout << "Manhattan Distance: " << dist << std::endl;
return 0;
}
In the above code, the `manhattanDistance` function computes the distance by taking the absolute differences of the x and y coordinates. This method is especially useful when navigating through blocks in city layouts or grid-based games, offering clarity on potential paths that a movement could take.
Hamming Distance
When dealing with strings, Hamming distance becomes relevant. It measures the difference between two strings of equal length by counting the positions at which the corresponding characters differ. This distance is often applied in error detection and correction algorithms.
Here’s a practical implementation of Hamming distance in C++:
#include <iostream>
#include <string>
// Function to calculate Hamming distance
int hammingDistance(const std::string& str1, const std::string& str2) {
if (str1.length() != str2.length()) {
throw std::invalid_argument("Strings must be of the same length");
}
int distance = 0;
for (size_t i = 0; i < str1.length(); ++i) {
if (str1[i] != str2[i]) {
distance++;
}
}
return distance;
}
// Example usage
int main() {
int dist = hammingDistance("karolin", "kathrin");
std::cout << "Hamming Distance: " << dist << std::endl;
return 0;
}
In the example above, the Hamming distance function iterates through each character of the two strings, comparing them and increasing the distance count for each differing pair. Hamming distance is especially useful in the realm of telecommunications and information theory, helping identify errors in transmitted data.
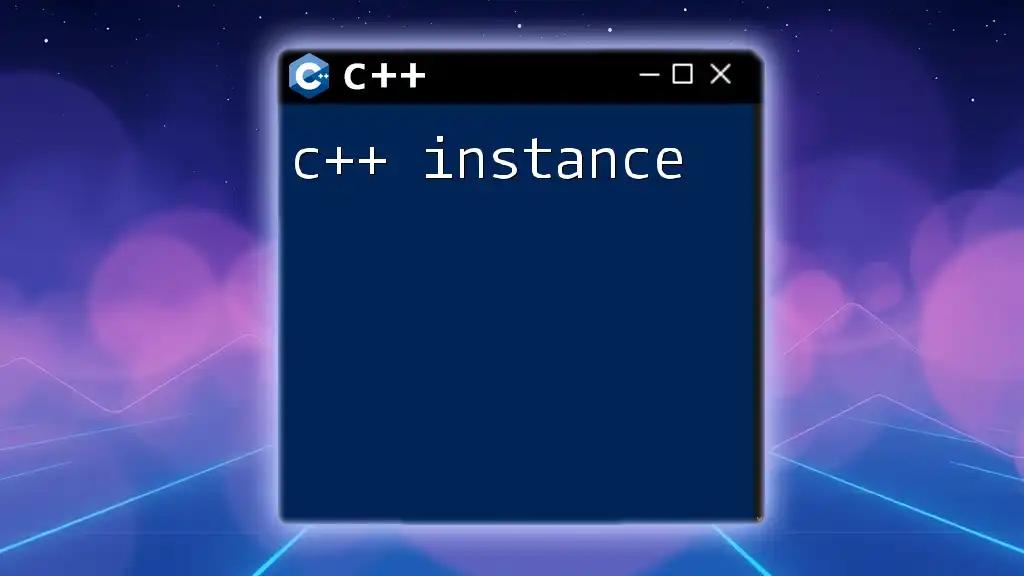
Advanced Distance Calculations
Chebyshev Distance
Chebyshev distance extends the concept of distance to a maximum factor. It is defined as the maximum difference among the coordinates. The formula for Chebyshev distance is:
$$ d = max(|x_2 - x_1|, |y_2 - y_1|) $$
This distance metric is typically used in environments like chess, where a piece can move in multiple directions and the diagonal moves have the same weight as horizontal or vertical moves.
Minkowski Distance
Minkowski distance is a generalized measurement that behaves like both Euclidean and Manhattan distances. It comprises all possible distance scenarios using a parameter \( p \). The formula is as follows:
$$ d = \left( \sum_{i=1}^{n} |x_i - y_i|^p \right)^{\frac{1}{p}} $$
For \( p=1 \), it reduces to Manhattan distance, and for \( p=2 \), it becomes Euclidean distance. It's a highly versatile formula that can be customized based on specific needs.
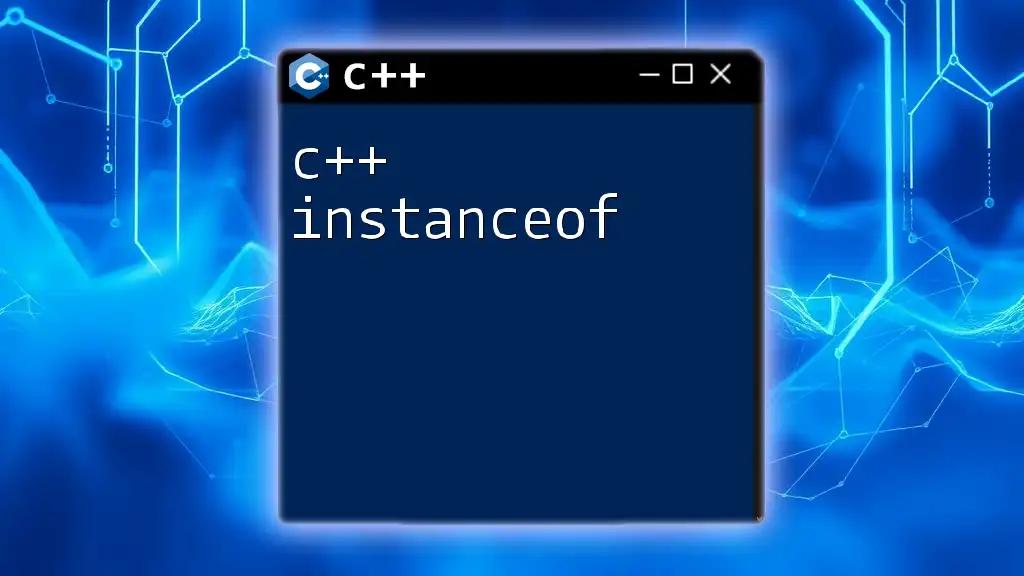
Using Distance in Algorithms
K-Means Clustering
Distance calculations play a vital role in K-Means clustering algorithms. The algorithm relies heavily on determining the centroid distance from various points to group them effectively. Selecting the right distance metric could dramatically affect the performance and accuracy of clustering results.
Nearest Neighbor Search
Another important application of distance is in the K-Nearest Neighbors (K-NN) algorithm. K-NN utilizes distance to classify data into different categories.
In a simple implementation, you'd typically define a distance function and use it to find the closest neighbors of a point. Below is a simple prototype:
#include <vector>
#include <algorithm>
// Example structure to represent a point in 2D space
struct Point {
double x, y;
};
// Basic Euclidean distance function
double euclideanDistance(Point a, Point b) {
return std::sqrt(std::pow(b.x - a.x, 2) + std::pow(b.y - a.y, 2));
}
// Function for K-NN algorithm skeleton
std::vector<Point> kNearestNeighbors(std::vector<Point>& points, Point target, int k) {
std::vector<std::pair<double, Point>> distances;
for (const auto& point : points) {
double distance = euclideanDistance(point, target);
distances.emplace_back(distance, point);
}
std::sort(distances.begin(), distances.end());
std::vector<Point> neighbors;
for (int i = 0; i < k; i++) {
neighbors.push_back(distances[i].second);
}
return neighbors;
}
In this example, the `kNearestNeighbors` function calculates the distances of all points in a dataset to a target point, sorts them, and retrieves the k-nearest neighbors. Distance metrics directly influence not just the results but also the efficiency of the algorithm.
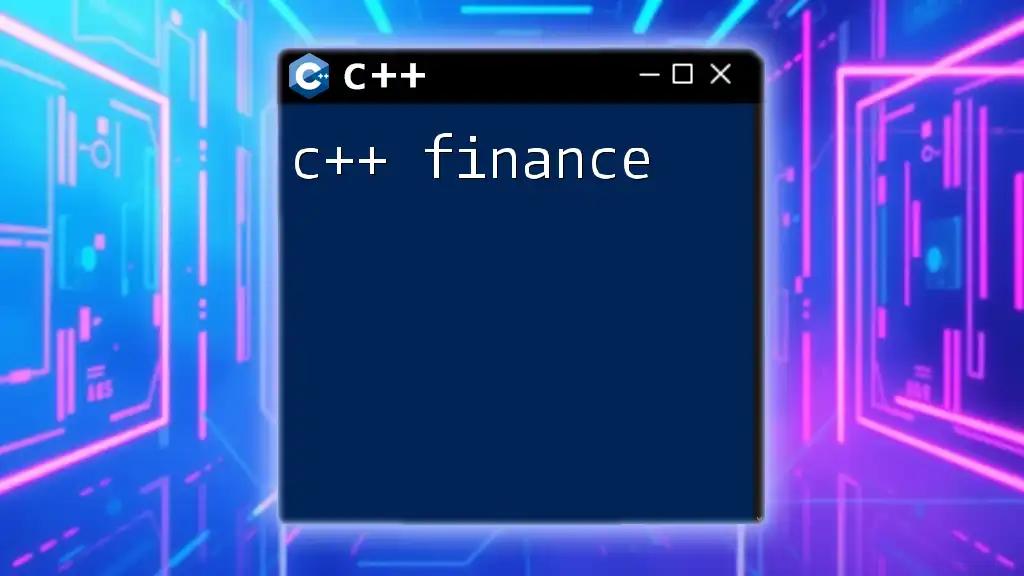
Optimizing Distance Calculations
As with many computational problems, optimizing your distance calculations can save precious time and resources. Some common pitfalls include unnecessary recalculations and inefficient algorithms, both of which can be avoided by employing techniques such as caching results and using efficient data structures, such as trees or hash maps.
Further Techniques
Utilizing preprocessing to calculate distances between points or even leveraging parallel processing can significantly reduce the time complexity of your algorithms while ensuring precise calculations. In large data applications, these optimizations can drastically enhance performance.
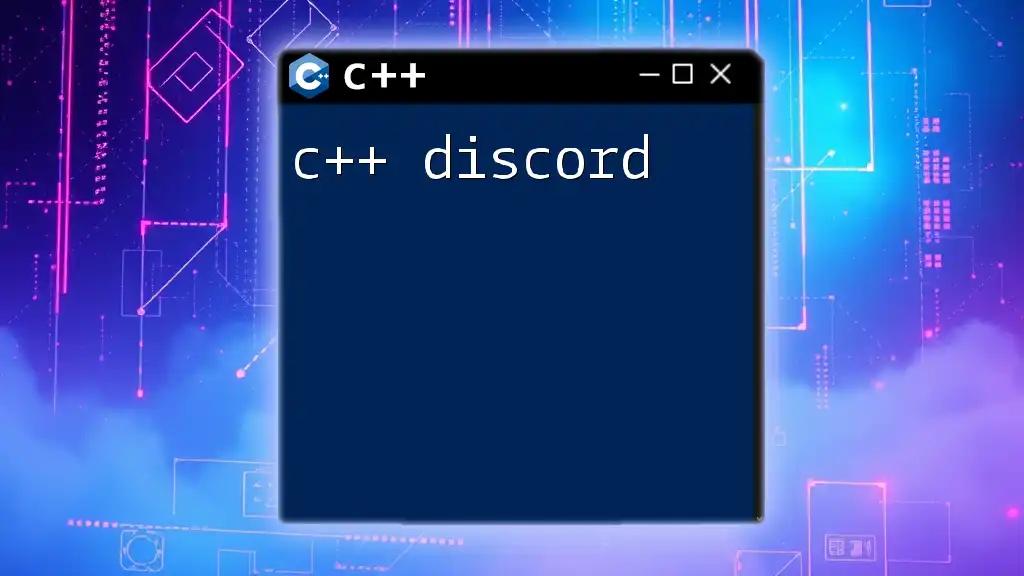
Conclusion
Understanding C++ distance calculations is crucial for diversifying your programming toolkit. Whether you're working on geometric algorithms or developing a simple game, knowing when and how to apply these distance measures will enhance your code’s efficiency and effectiveness. From Euclidean to Hamming, each distance measure serves distinct purposes, aiding in problem-solving across various domains.
Endeavor to implement these concepts in your own projects, as practical experience will solidify your understanding and open up new avenues in your programming journey.