C++/CLI is an extension of C++ designed to work with the .NET framework, allowing developers to write managed code that interacts seamlessly with .NET libraries.
Here’s a simple code snippet demonstrating the use of C++/CLI to create a .NET application that outputs "Hello, World!":
#include <iostream>
using namespace System;
int main()
{
Console::WriteLine("Hello, World!");
return 0;
}
Understanding the C++ and .NET Integration
What is C++/CLI?
C++/CLI is a variant of C++ designed specifically for the .NET framework. It acts as a bridge that enables native C++ code to interact with managed .NET code. Through C++/CLI, developers can leverage the power of C++ for performance-intensive tasks while taking advantage of the extensive functionalities offered by .NET libraries.
One of the core distinctions between C++ and C++/CLI lies in how memory management is handled. In C++, developers manually manage memory using pointers, whereas C++/CLI integrates garbage collection features that simplify memory management, making development easier and less error-prone.
The Role of Common Language Runtime (CLR)
The Common Language Runtime (CLR) is a crucial component of the .NET framework that manages code execution. It provides services such as memory management, security, exception handling, and type safety, thus enabling seamless interaction between different programming languages.
When you write C++/CLI code that utilizes .NET libraries, it is the CLR that ensures managed code runs safely within the .NET environment. For instance, when a C++/CLI application accesses objects created in C# or VB.NET, the CLR allows these interactions to happen without requiring additional work from the developer.
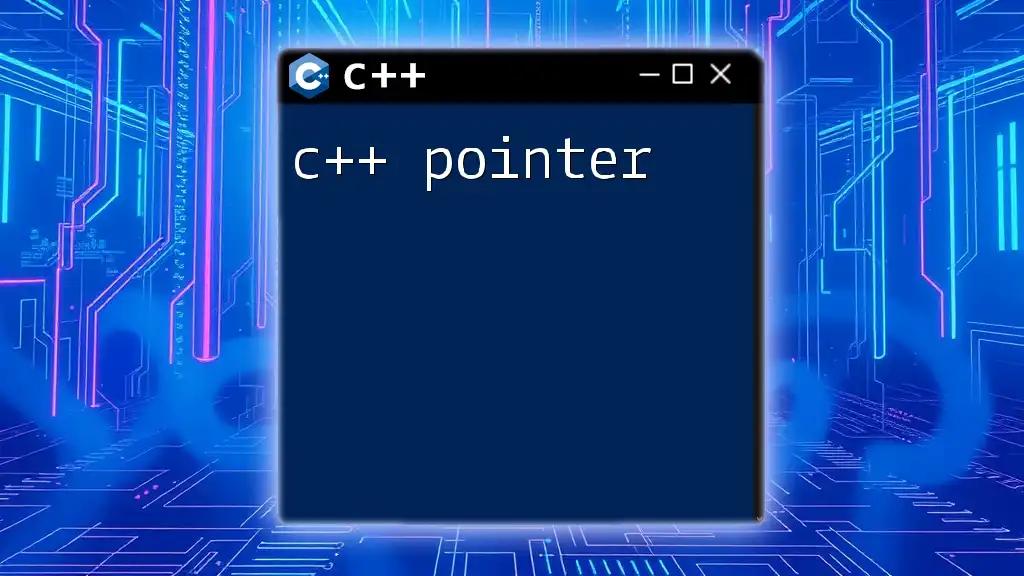
Setting Up Your Development Environment
Required Tools and Software
To start using C++ dotnet effectively, the following software and tools are essential:
- Visual Studio: The primary IDE for developing C++/CLI applications.
- .NET SDK: Make sure you have the latest .NET SDK installed.
- Windows SDK: Provides functionalities if you're developing Windows-specific applications.
Configuring Your Project for C++/CLI
To create a C++/CLI project in Visual Studio:
- Launch Visual Studio and select Create a new project.
- Choose CLR Empty Project, which sets up the necessary configurations for C++/CLI development.
- Configure your project properties by navigating to Project > Properties:
- Go to Configuration Properties > General.
- Ensure that Common Language Runtime support is set to either /clr or /clr strictly.
This setup gives you a solid foundation to start coding in the C++/CLI environment.
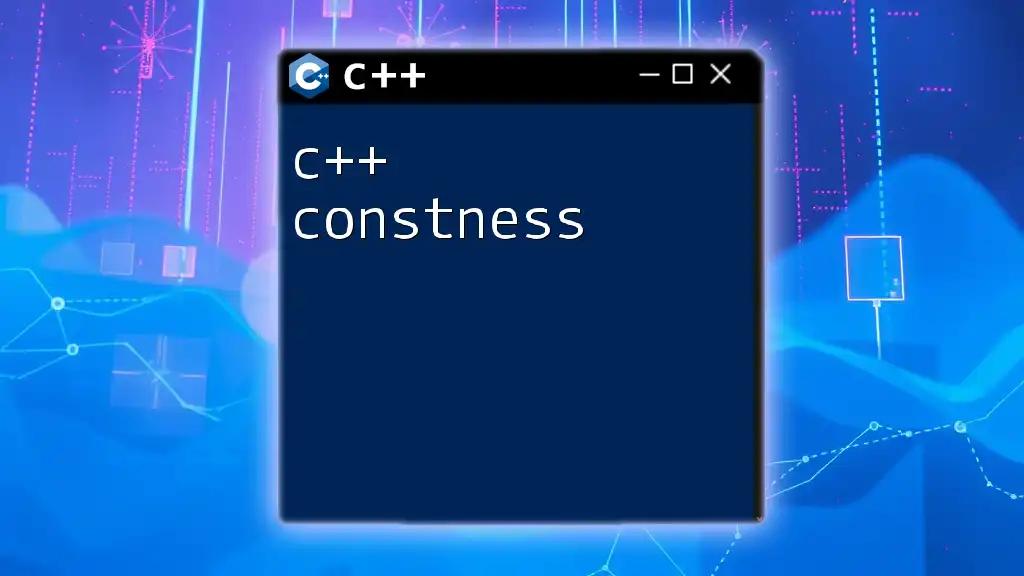
Core Concepts of C++ and .NET
Managed vs. Unmanaged Code
Understanding the distinction between managed and unmanaged code is vital for C++ dotnet development.
- Managed Code: This code runs under the supervision of the CLR, allowing automatic memory management and enhanced security features. C++/CLI compiles to managed code.
- Unmanaged Code: Here, the code runs directly on the operating system, and developers must handle memory management manually.
For example, consider the following simple code snippets to demonstrate the difference:
// Managed code example using C++/CLI
public ref class MyClass {
public:
void MyMethod() {
// Managed operation
}
};
// Unmanaged code example
class MyUnmanagedClass {
public:
void MyMethod() {
// Unmanaged operation
}
};
Using .NET Libraries in C++
One of the standout features of C++ dotnet is the ability to reference and utilize .NET libraries in your C++ applications. To do this, you can follow these steps:
- In your project, right-click on References and choose Add Reference.
- Select desired .NET assemblies, such as `System.Windows.Forms`.
Here’s a simple C++/CLI example that demonstrates calling a .NET library to show a message box:
#include <Windows.h>
using namespace System;
using namespace System::Windows::Forms;
void ShowMessage() {
MessageBox::Show("Hello from C++/CLI!", "C++ DotNet Example");
}
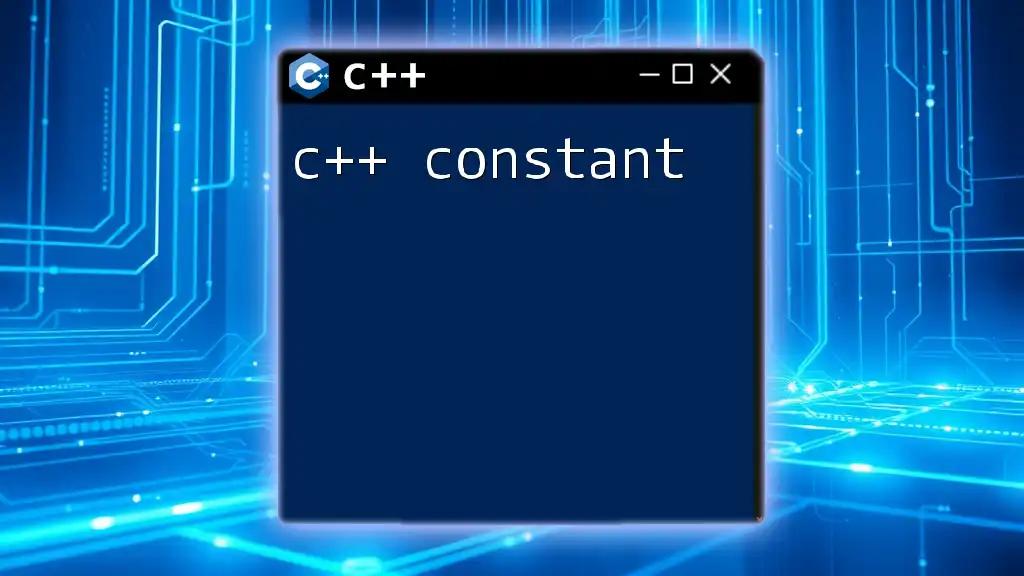
Practical Applications of C++ in .NET
Creating Windows Forms Applications
Windows Forms is a UI framework under .NET that allows developers to create desktop applications with rich user interfaces. The integration of C++ with Windows Forms opens doors for building powerful GUIs.
To create a simple Windows Forms application using C++/CLI, follow these steps:
- Create a new CLR Windows Forms Application project in Visual Studio.
- Add a button to the form via the Designer.
- Double-click the button to create an event handler:
private: System::Void button1_Click(System::Object^ sender, System::EventArgs^ e) {
MessageBox::Show("You clicked the button!", "Event Click");
}
Developing Web Applications with ASP.NET and C++
Leveraging ASP.NET for web application development with C++/CLI serves to harmonize the performance of C++ with the robustness of the .NET web framework. You can build and deploy sophisticated web applications with ease.
To create a simple ASP.NET MVC application using C++/CLI, you might start with:
- Set up your project as an ASP.NET MVC Application in Visual Studio.
- Define a controller with an action method:
public ref class HomeController : public Controller {
public: ActionResult Index() {
return View();
}
};
This code snippet represents a basic controller within an ASP.NET MVC setup, portraying how C++/CLI can be used to manage web requests.
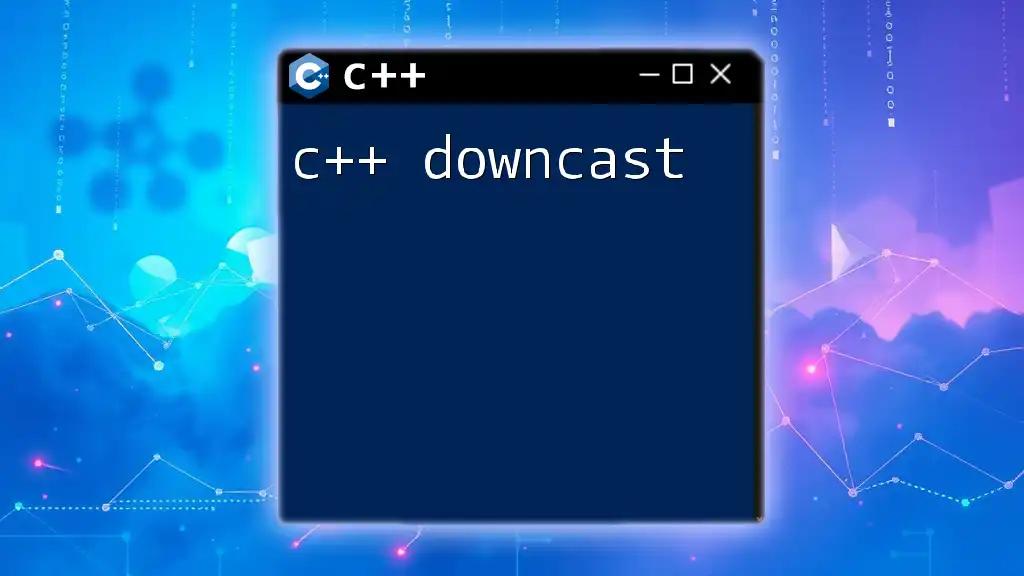
Error Handling in C++ and .NET
Exception Handling Techniques
Exception handling is critical in any programming environment, and C++/CLI provides robust ways to manage exceptions. The structure is similar to standard C++, but the mechanics differ slightly due to managed code.
C++/CLI employs `try-catch` blocks to catch exceptions from both managed and unmanaged code:
try {
// Code that may throw an exception
} catch (Exception^ ex) {
MessageBox::Show("An error occurred: " + ex->Message, "Error");
}
This approach ensures that errors are gracefully handled, allowing for a better user experience.
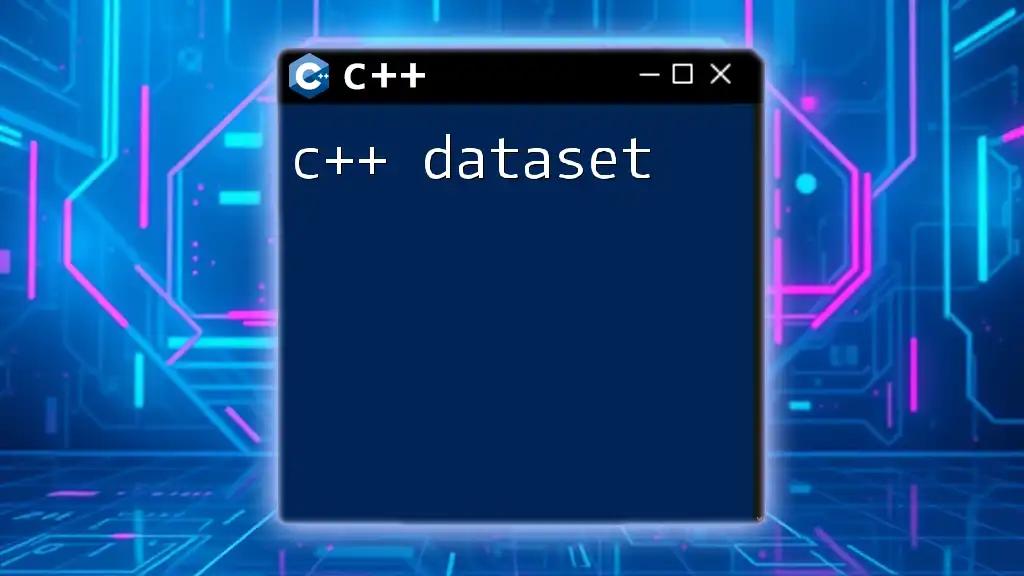
Best Practices for C++ and .NET Development
Performance Optimization Tips
When working with C++ dotnet, optimizing performance can make a significant difference. Here are a few best practices:
- Minimize Pointer Use: The more you can rely on managed references rather than pointers, the more you benefit from garbage collection.
- Avoid Excessive Allocations: Reuse objects when possible instead of constantly allocating and deallocating memory.
For example, consider using `List<T>` for collections instead of unmanaged arrays to take advantage of built-in memory management.
Debugging and Testing Strategies
Debugging plays a vital role in guaranteeing application performance and stability. Visual Studio provides robust debugging tools such as breakpoints, variable watches, and memory profiling tools. Additionally, leveraging automated unit testing frameworks can lead to more maintainable code.
Integrate unit tests with your C++/CLI projects using frameworks that support .NET, such as NUnit or MSTest.
[TestClass]
public ref class MyTests {
[TestMethod]
void TestMethodExample() {
// Your test assertions
}
};
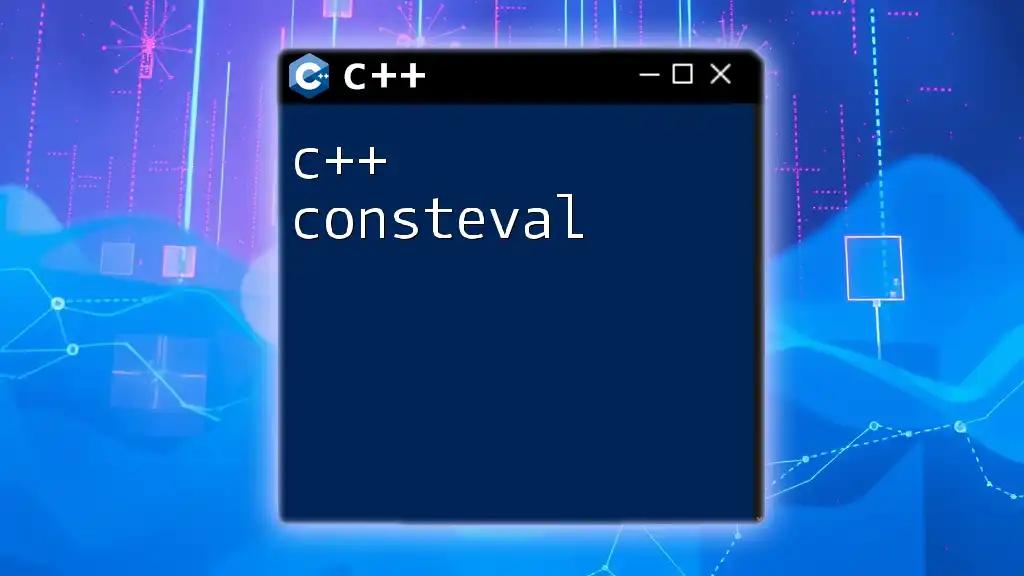
Conclusion
The combination of C++ dotnet opens up vast possibilities for developers, enabling them to harness the strength of both C++ and the .NET framework. By understanding the integration, setup, and core concepts outlined in this guide, you can effectively build dynamic applications that leverage both worlds.
As you continue your journey in C++ and .NET development, keep exploring resources and practical exercises to further deepen your knowledge and skills. Happy coding!

References and Resources
- Microsoft Documentation: Official materials on C++/CLI and .NET.
- Online Courses: Recommended platforms for learning full-stack development with C++ and .NET.
- Community Forums: Engaging with fellow developers on platforms like Stack Overflow or GitHub for collaborative problem-solving.