C++ ENet is a networking library that simplifies the creation of reliable and ordered packet communication, ideal for real-time applications like games.
Here’s a simple example demonstrating how to initialize an ENet host and create a client connection:
#include <enet/enet.h>
int main() {
enet_initialize(); // Initialize ENet
ENetHost* client = enet_host_create(NULL, 1, 2, 0, 0); // Create a client host
// ... additional code for connection and handling ...
enet_host_destroy(client); // Clean up
enet_deinitialize(); // Deinitialize ENet
return 0;
}
Understanding ENet
What is a Networking Library?
Networking libraries are essential tools in game development, allowing developers to manage communication between clients and servers seamlessly. They abstract the complexities of network protocols and provide robust functionalities, enabling developers to focus on game logic rather than low-level networking details.
Core Features of ENet
ENet stands out among networking libraries due to several key features:
-
Reliable Communication:
ENet ensures that packets sent over the network arrive without loss. It combines the benefits of UDP's speed with the reliability usually associated with TCP. -
Connection-Oriented Communication:
Unlike stateless protocols, ENet's connection-oriented model facilitates a persistent link between clients and servers. This model is crucial for multiplayer scenarios where consistent interaction is needed. -
Packet Sequencing and Ordering:
ENet guarantees that packets arrive in the same order they were sent. This feature is particularly important in real-time environments, preventing out-of-sequence data from causing gameplay inconsistencies.
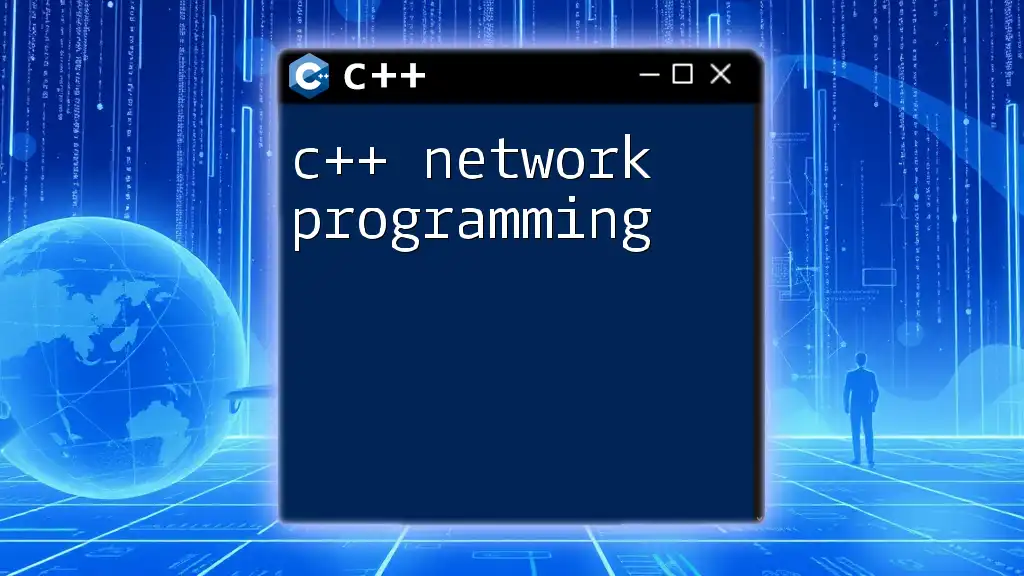
Setting Up ENet
Installing ENet
To start using C++ ENet, follow these steps:
-
Download the Library:
You can obtain the latest version of ENet from its [official repository](https://github.com/ccws/enet). -
Compile the Library:
Use the following command to compile the source code, depending on your system:cmake . make
-
Linking with a C++ Project:
Make sure to link the ENet library in your project settings. For example, add `-lenet` to your compilation command.
Initial Setup in Your C++ Project
Create a new C++ project, ensure it includes headers for ENet, and configure your build system. Here’s a basic structure:
- Create a directory for your project.
- Inside, create a `src` folder for source files and include the ENet header files.
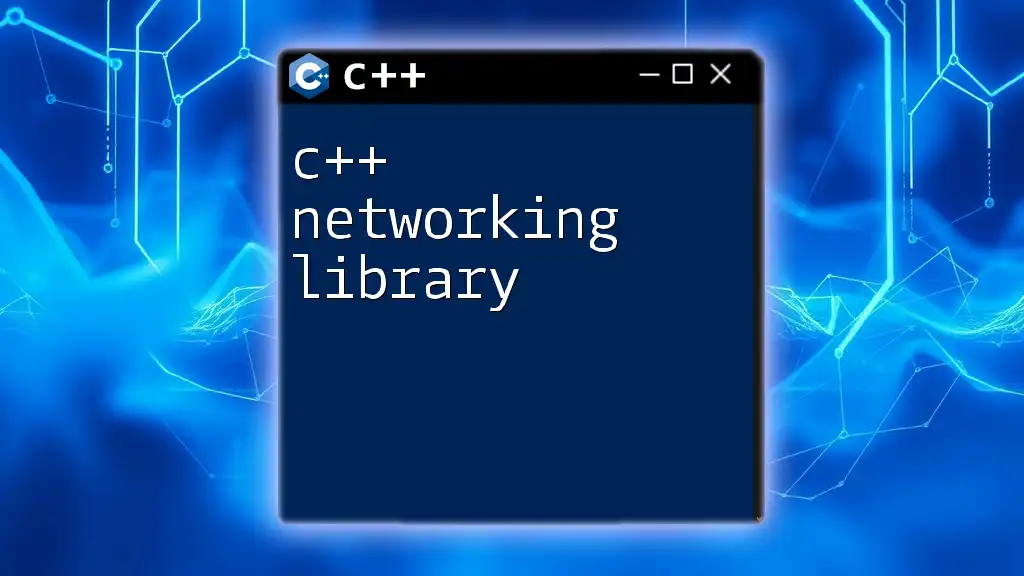
Basic Concepts
Creating a Host
To create a server host in ENet, you must initialize the library and set up the host parameters. Here’s a fundamental snippet:
#include <enet/enet.h>
int main() {
if (enet_initialize() != 0) {
fprintf(stderr, "An error occurred while initializing ENet.\n");
return EXIT_FAILURE;
}
ENetHost* server = enet_host_create(NULL, 32, 2, 0, 0);
if (server == NULL) {
fprintf(stderr, "An error occurred while trying to create an ENet server host.\n");
return EXIT_FAILURE;
}
// Your server loop here
enet_host_destroy(server);
enet_deinitialize();
return EXIT_SUCCESS;
}
This code initializes ENet, creates a server capable of handling 32 clients, and prepares two channels for communication.
Connecting to a Host
A client can connect to a server using the following straightforward code snippet:
ENetHost* client = enet_host_create(NULL, 1, 2, 0, 0);
ENetPeer* peer = enet_host_connect(client, &address, 2, 0);
if (peer == NULL) {
fprintf(stderr, "No available peers for initiating an ENet connection.\n");
return EXIT_FAILURE;
}
Here, `address` is an `ENetAddress` struct configured with the server's IP and port.
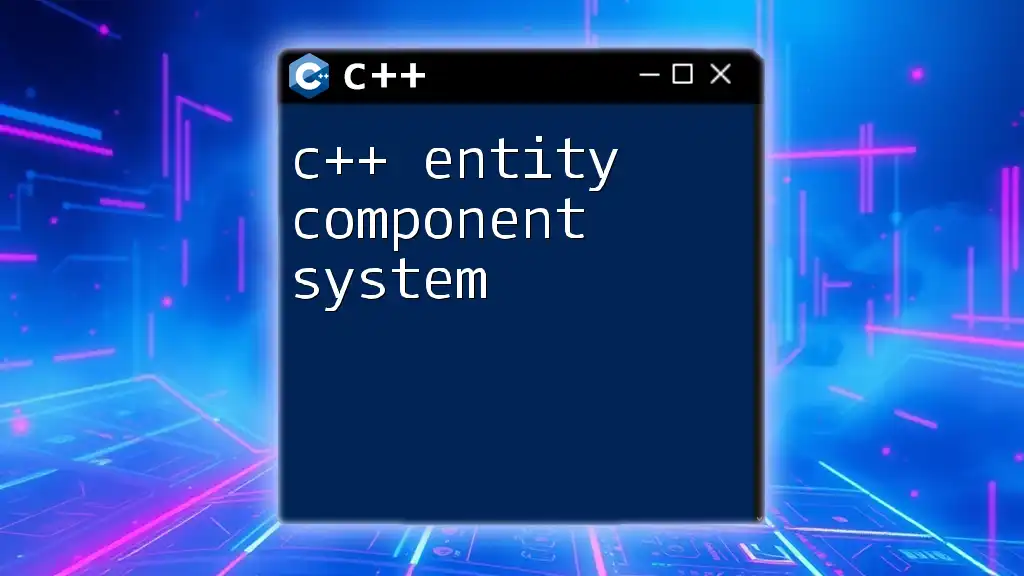
ENet Packet Handling
Sending and Receiving Packets
To send a packet in ENet, define it and use the `enet_host_broadcast` function or send it directly to a peer:
ENetPacket* packet = enet_packet_create("Hello, World!", strlen("Hello, World!") + 1, ENET_PACKET_FLAG_RELIABLE);
enet_host_send(server, peer, packet);
To receive packets, implement the following:
ENetEvent event;
while (enet_host_service(server, &event, 1000) > 0) {
switch (event.type) {
case ENET_EVENT_TYPE_RECEIVE:
printf("%s\n", (char*)event.packet->data);
enet_packet_destroy(event.packet);
break;
}
}
This code listens for incoming packets and prints the message.
Reliability and Performance
When sending packets, ENet offers various flags. For instance, using `ENET_PACKET_FLAG_RELIABLE` guarantees packet delivery, but for scenarios such as real-time updates (like player positions), you might prefer `ENET_PACKET_FLAG_UNSEQUENCED` or no flags at all for performance.
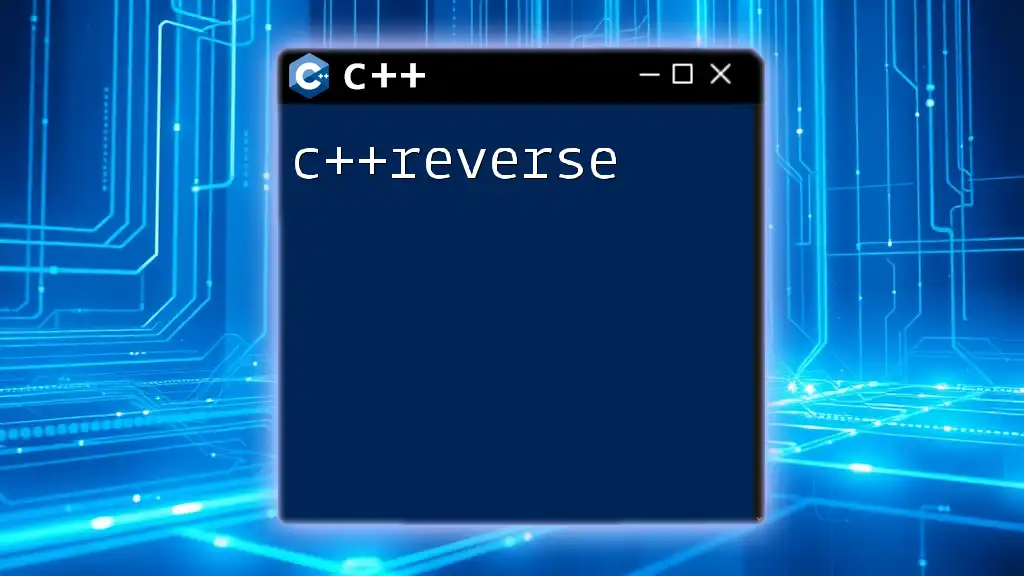
Working with Channels
What are Channels?
Channels in ENet allow developers to categorize data streams. This categorization enables the separation of critical game messages from less crucial updates, optimizing bandwidth usage and maintaining performance.
Creating and Using Channels
To create and use channels in your application, use the channel identifier when sending packets:
enet_host_send(server, peer, packet, 1); // The '1' refers to the channel number.
You’ll need to ensure that the client and server know which channels to use for specific messages.
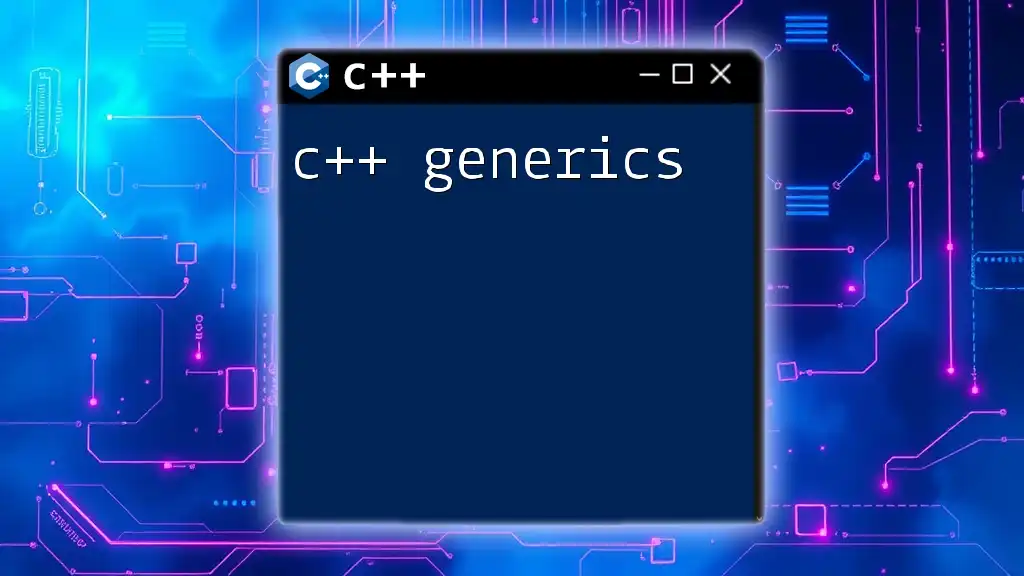
Advanced Features
Handling Multiple Clients
To manage multiple client connections effectively, maintain an array or list of `ENetPeer` pointers. This allows you to track active connections and send messages to specific clients. Ensure that you handle connection events properly:
case ENET_EVENT_TYPE_CONNECT:
printf("A new client connected.\n");
break;
case ENET_EVENT_TYPE_DISCONNECT:
printf("A client disconnected.\n");
break;
Implementing a Basic Game Loop
Integrate a simple game loop that keeps your server responsive:
while (true) {
while (enet_host_service(server, &event, 1000) > 0) {
// Handle your events here.
}
// Game logic updates, sending periodic updates to clients, etc.
}
This structure ensures your server continues to handle events while managing game logic effectively.
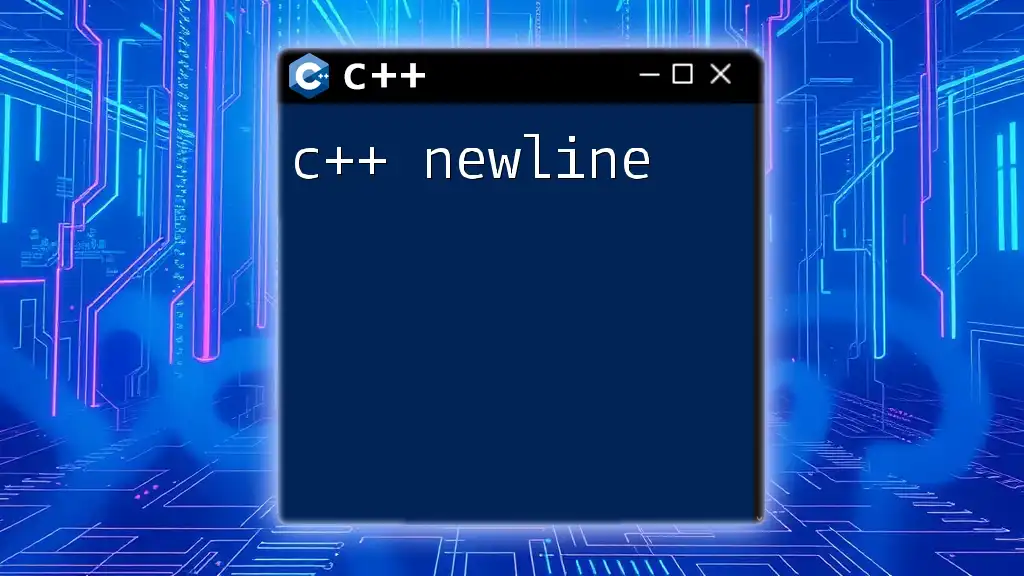
Debugging and Troubleshooting
Common Issues and Solutions
When working with C++ ENet, you may encounter issues such as failure to connect or packet loss. Ensure that:
- Firewalls are not blocking the ports.
- The server is reachable, and clients are using the correct IP and port.
- Proper error handling is in place to catch and log networking errors.
Resources for Further Learning
Engage with the community through forums and documentation. Exploring the [official ENet documentation](https://enet.bespin.org/) will deepen your understanding and expose you to advanced techniques.
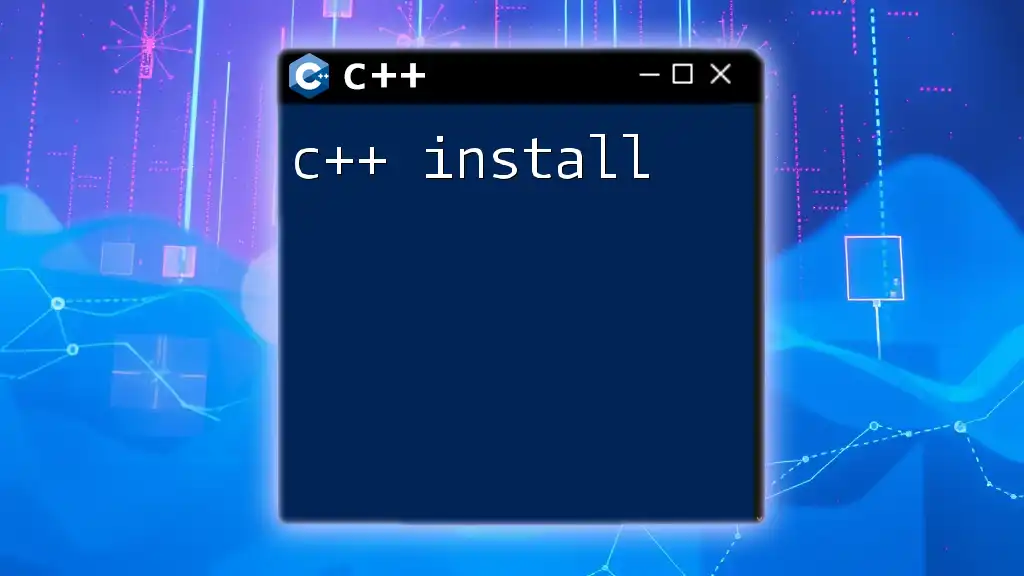
Conclusion
Through this guide on C++ ENet, you now understand the core functionalities and setup procedures for creating robust multiplayer applications. With the concepts of hosts, packets, channels, and game loops, you are equipped to begin your multiplayer journey. As you continue experimenting with C++ ENet, you'll discover the vast potential of networking in game development.
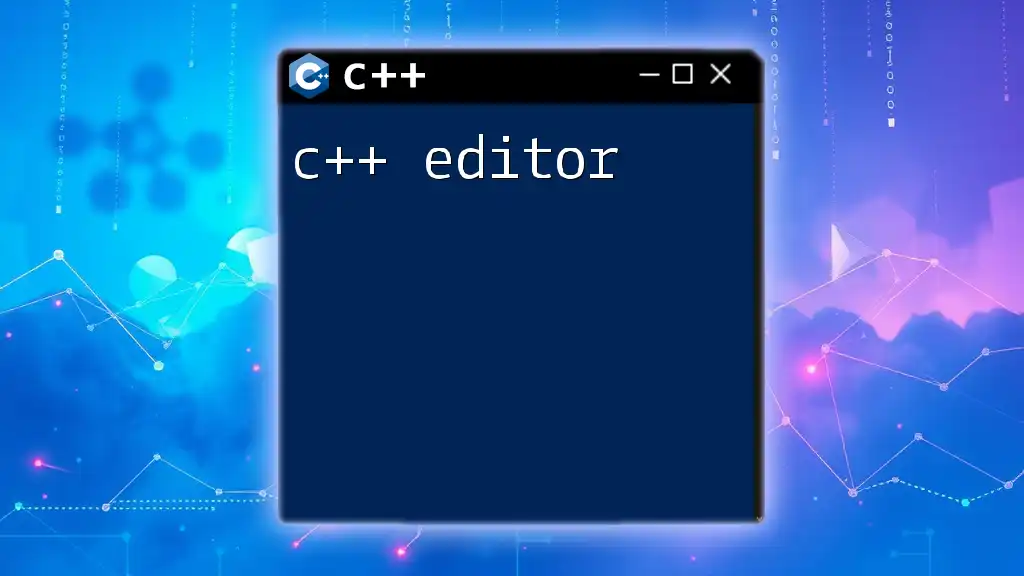
Additional Resources
For further exploration, visit the official ENet documentation and participate in community forums where you can share your experiences and learn from others. Happy coding!