The C++ Entity Component System (ECS) is a design pattern that promotes composition over inheritance, organizing code into reusable components and entities while improving performance and flexibility in game development or simulations.
#include <iostream>
#include <vector>
#include <unordered_map>
#include <memory>
class Component {
public:
virtual ~Component() = default;
};
class Position : public Component {
public:
float x, y;
Position(float x, float y) : x(x), y(y) {}
};
class Velocity : public Component {
public:
float vx, vy;
Velocity(float vx, float vy) : vx(vx), vy(vy) {}
};
class Entity {
std::unordered_map<std::type_index, std::shared_ptr<Component>> components;
public:
template<typename T>
void AddComponent(T component) {
components[typeid(T)] = std::make_shared<T>(component);
}
template<typename T>
std::shared_ptr<T> GetComponent() {
return std::static_pointer_cast<T>(components[typeid(T)]);
}
};
int main() {
Entity player;
player.AddComponent(Position(0.0f, 0.0f));
player.AddComponent(Velocity(1.0f, 0.0f));
auto pos = player.GetComponent<Position>();
std::cout << "Player Position: (" << pos->x << ", " << pos->y << ")\n";
return 0;
}
Understanding Entity Component Systems in C++
What is an Entity Component System (ECS)?
An Entity Component System (ECS) is an architectural pattern that is particularly effective in game development and other areas involving complex entity behavior. The ECS paradigm shifts focus from traditional Object-Oriented Programming (OOP) methods, which often rely on inheritance and tightly coupled classes, to a more flexible and modular approach.
ECS consists of three main parts:
- Entities: Basic objects with unique IDs.
- Components: Data containers for specific attributes.
- Systems: Logic that processes entities with specific components.
Historically, the ECS approach emerged as a solution to the limitations of OOP, allowing for increased modularity and reusability.
Core Concepts of ECS
Entities
Entities in ECS are simple, unique identifiers that serve as containers for components. They don't contain any behavior themselves, allowing for great flexibility.
Components
Components are the building blocks of functionality in ECS. They consist of plain data that describes various attributes of the entity. For instance, a `PositionComponent` might contain coordinates, while a `VelocityComponent` might define speed and direction.
Systems
Systems represent the logic that operates on the entities equipped with specific components. For example, a `MovementSystem` might read from both `PositionComponent` and `VelocityComponent` to update the position of entities based on their velocity.
The relationship between entities, components, and systems is crucial to understanding ECS as it allows for robust interactions without the need for complex hierarchies.
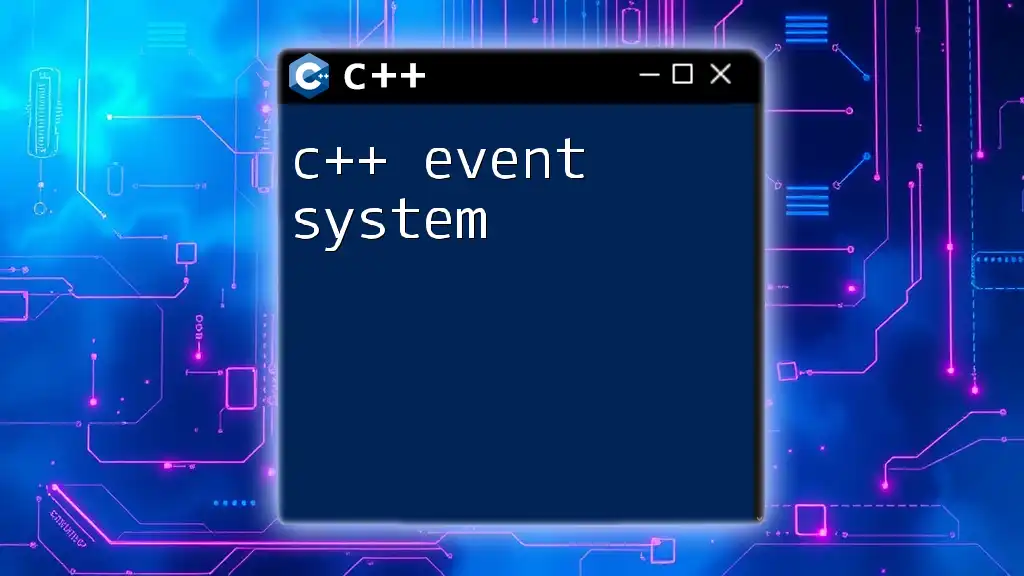
Building a Basic ECS in C++
Setting Up Your C++ Environment
To get started with implementing a C++ Entity Component System, you'll need a suitable C++ development environment. This can include IDEs like Visual Studio, CLion, or Code::Blocks, and a compiler like GCC or Clang.
Designing the ECS Structure
Defining the Entity Class
Here’s how you can create a straightforward `Entity` class:
class Entity {
public:
int id; // Unique ID for the entity
};
This class is simple yet critical, as it allows you to manage a collection of entities.
Creating the Component Base Class
Next, develop a base class for components:
class Component {
public:
virtual ~Component() {}
};
The `Component` class acts as a base for all specific component types, ensuring a unified interface.
Implementing Specific Components
Each component should serve a specific purpose. Below are examples of a `PositionComponent` and a `VelocityComponent`:
- Position Component:
class PositionComponent : public Component {
public:
float x, y;
};
- Velocity Component:
class VelocityComponent : public Component {
public:
float vx, vy;
};
These components provide the data needed to dictate the behavior of the associated entities.
Implementing the ECS Manager
Creating the ECSManager Class
The next logical step is to create the `ECSManager`, which manages entities and their components:
class ECSManager {
std::unordered_map<int, std::shared_ptr<Entity>> entities;
std::unordered_map<std::type_index, std::vector<std::shared_ptr<Component>>> components;
};
This class should include methods for adding and managing entities, as well as associating components with them.
Adding and Managing Entities
The ECSManager should allow for easy addition and retrieval of entities. This promotes efficient management of multiple entities throughout your application.
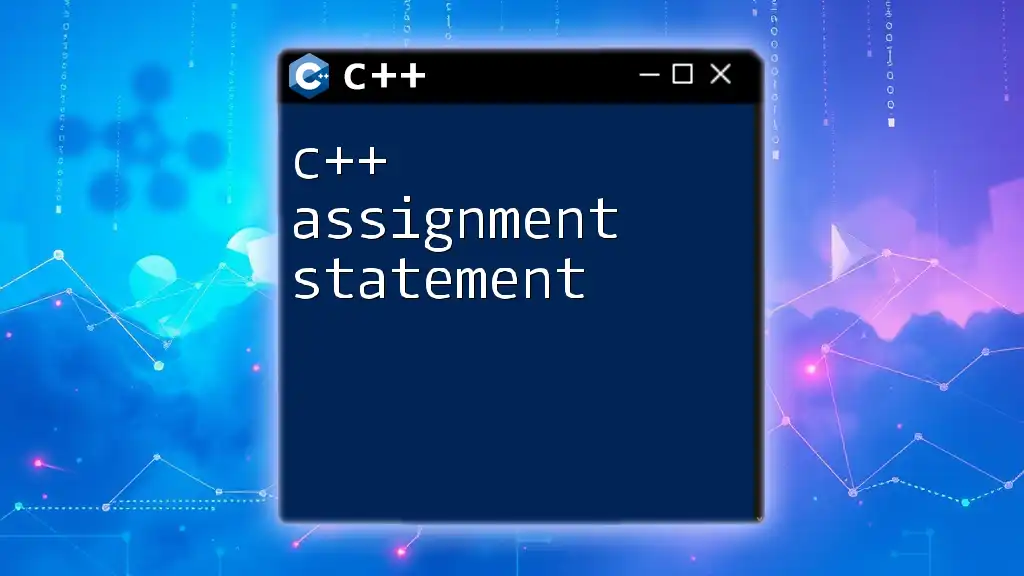
Systems: How They Work in ECS
What is a System?
A system in ECS is a piece of functionality that processes entities possessing specific components. Systems are where the logic of your application resides, distinctly separating data from behavior.
Creating Systems in C++
Implementing a Movement System
Here, we can create a simple movement system:
void MovementSystem(ECSManager& ecs) {
for (const auto& entity : ecs.getEntities()) {
auto pos = entity->getComponent<PositionComponent>();
auto vel = entity->getComponent<VelocityComponent>();
if (pos && vel) {
pos->x += vel->vx;
pos->y += vel->vy;
}
}
}
In this system, we process each entity and update its position based on its velocity.
Implementing Other Common Systems
You may want to implement additional systems like:
- Physics System: Handle collision detection and response.
- Rendering System: Manage the display of entities.
Each system works independently, enhancing modularity.
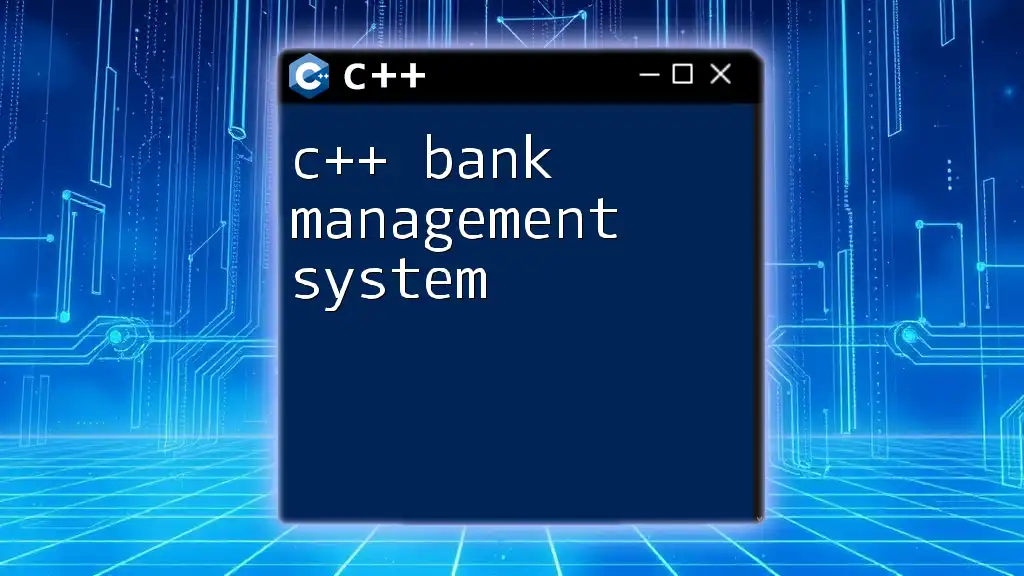
Performance Considerations in C++ ECS
Memory Management Strategies
Efficient memory management is pivotal in ECS, especially in performance-sensitive scenarios like gaming.
- Dynamic Memory Management: Utilize dynamic allocation for components, pairing them with smart pointers to manage lifetime automatically.
- Static Memory Management: For small, fixed-sized components, using stack allocation can yield performance benefits.
Optimizing Component Access
Implementing a data-driven design can significantly improve performance. Systems should ideally operate on contiguous memory segments, so consider data layout strategies that enhance cache coherence. Additionally, caching frequently accessed components can reduce lookup times in your ECS.
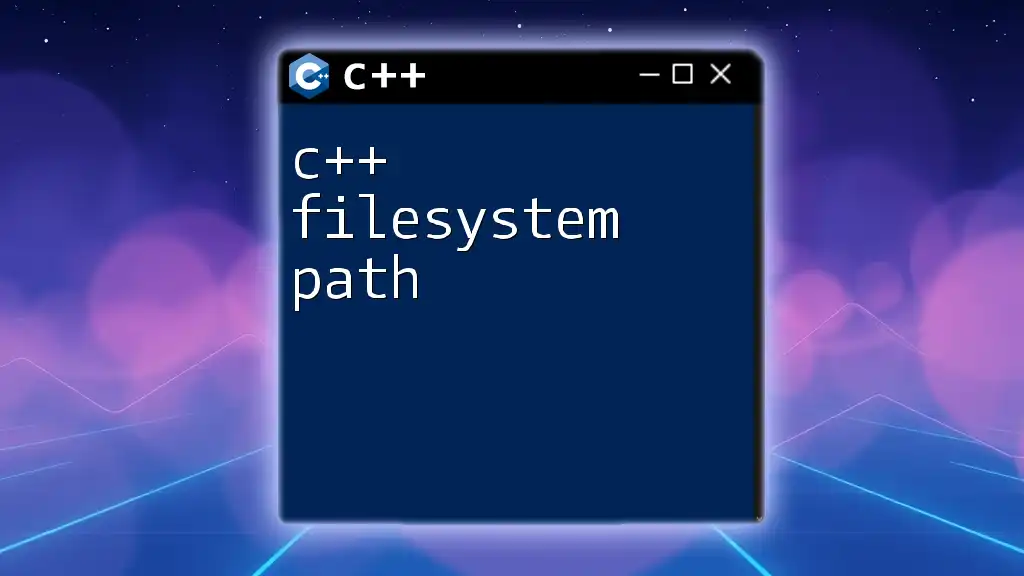
Advanced ECS Concepts
Event Systems in ECS
An event system can improve the flexibility of your ECS architecture. Events allow different systems to communicate without needing direct references to each other. You can define an `Event` class and implement a simple event dispatcher to manage event propagation.
Example Use Cases of ECS
Consider various use cases for ECS, especially in different domains:
- Game Development: ECS facilitates the implementation of complex game mechanics and interactions.
- Simulation Applications: Useful in scenarios requiring realistic physics and behavioral modeling.
- Real-time Rendering Techniques: ECS can be instrumental in separating rendering logic from game mechanics.
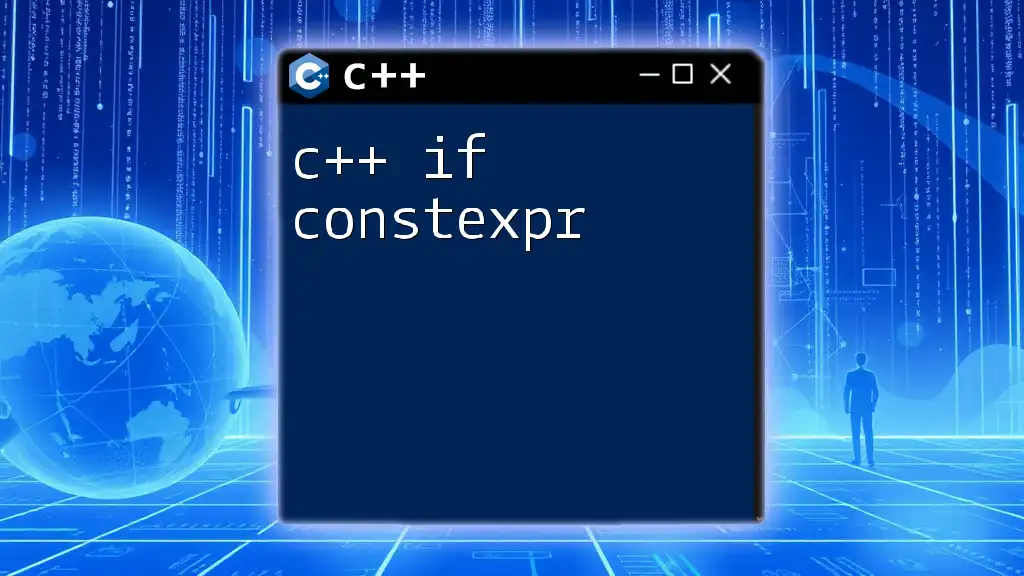
Conclusion
Advantages of Using C++ ECS
Utilizing the C++ Entity Component System provides significant advantages:
- Scalability: Adding new entities and behaviors can be done with minimal changes.
- Flexibility: Systems can be modified or replaced without impacting the entire architecture.
- Separation of Concerns: ECS promotes a clean separation between data and behavior, enhancing maintainability.
Further Learning Resources
For those eager to deepen their understanding, consider exploring:
- Books: “Game Programming Patterns” by Robert Nystrom.
- Online Courses: Platforms like Udemy and Coursera offer various courses related to ECS and game development.
- Helpful Libraries and Frameworks: Investigate existing ECS frameworks like EnTT or Artemis-CPP to see professional implementations.
Final Thoughts
Experimenting with the C++ Entity Component System can lead to innovative designs and enhanced performance in your applications. Don’t hesitate to dive in and join the community to share ideas, best practices, and to seek support. Embrace the ECS paradigm, and elevate your programming skills to new heights!