To set up a C++ development environment on a Mac, you can install Xcode and the necessary command-line tools, then create a simple C++ program using the following code snippet:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Development Environment
What is a C++ Environment?
A C++ environment is a setup that consists of various components that enable you to write, compile, and execute C++ code efficiently. It generally includes a compiler, an Integrated Development Environment (IDE) or a text editor, build tools for managing project compilation, and debuggers for troubleshooting code errors. An optimized C++ environment enhances your productivity and streamlines the development process, allowing you to focus on writing code rather than managing configurations.
Key Components of C++ Setup
The essential components required for setting up a C++ environment on macOS are:
- Compiler: Translates your C++ code into machine-readable code. Common choices include Clang and GCC.
- IDE/Text Editor: Provides an interface to write your code. Popular options include Xcode, CLion, and Visual Studio Code.
- Build Tools: Manage the compilation process and help organize your project. Make and CMake are widely used.
- Debugger: A tool that helps in identifying and fixing code bugs. GDB and LLDB are popular debugging tools available for macOS.
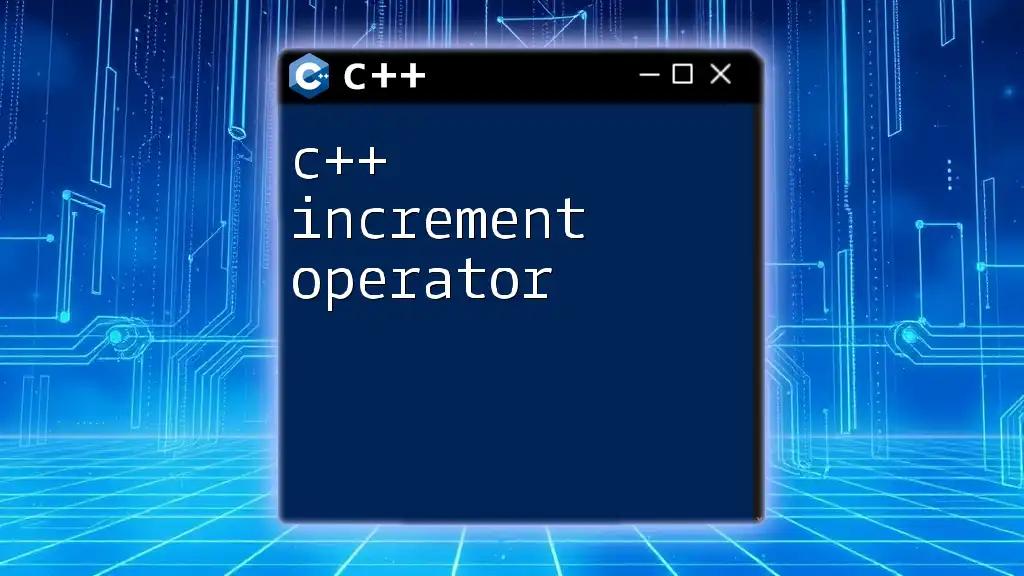
Prerequisites for Setting Up C++ on macOS
System Requirements
Before diving into installation, ensure your system meets the minimum requirements for running a C++ environment on macOS. While most modern Macs will suffice, check that your macOS version is up-to-date to avoid compatibility issues.
Installing Xcode Command Line Tools
Xcode includes the necessary tools to compile C++ programs, but you can also install just the command line tools to save space.
To install Xcode command line tools, open a terminal and execute:
xcode-select --install
This command prompts the installation of essential tools, including Clang.
Checking Your Installation
Once the installation is complete, verify it by checking the path of Xcode:
xcode-select -p
To confirm that the `g++` compiler is available and functioning, run:
g++ --version
This command should return the version of the installed compiler.
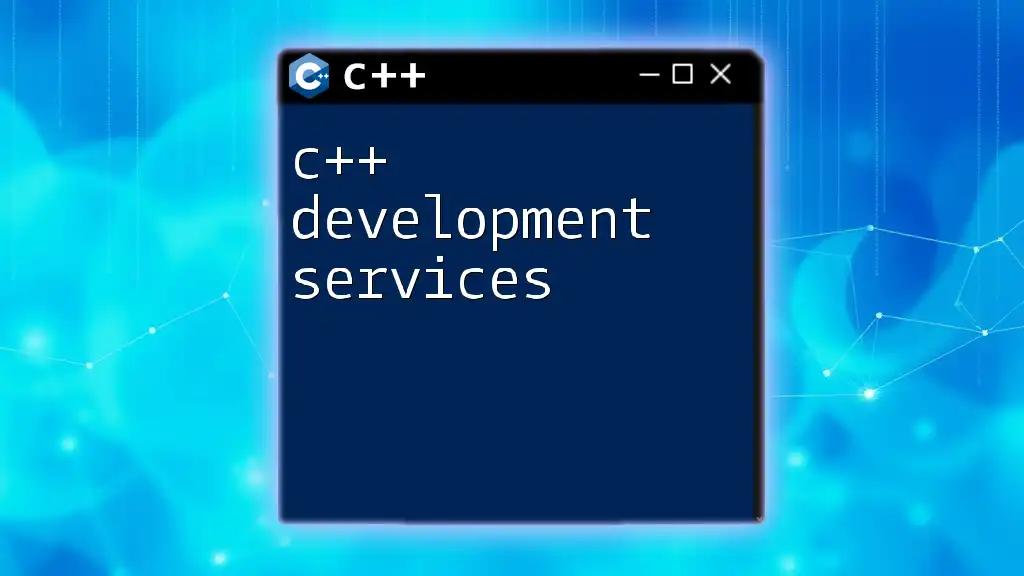
Choosing a C++ Compiler on macOS
Common Compilers for C++
C++ development on macOS primarily revolves around two compilers: Clang and GCC.
- Clang: This is the default compiler on macOS, known for its fast compilation speeds and better error messages.
- GCC: If you prefer using GCC instead, you can install it via Homebrew, a package manager for macOS.
To install GCC, use the following command:
brew install gcc
Setting Up Compiler Options
Understanding compiler flags is crucial for fine-tuning your compilation process. For example, you might want to compile a simple program `hello.cpp` into an executable named `hello`:
g++ -o hello hello.cpp
In this example, `-o` specifies the output file name.
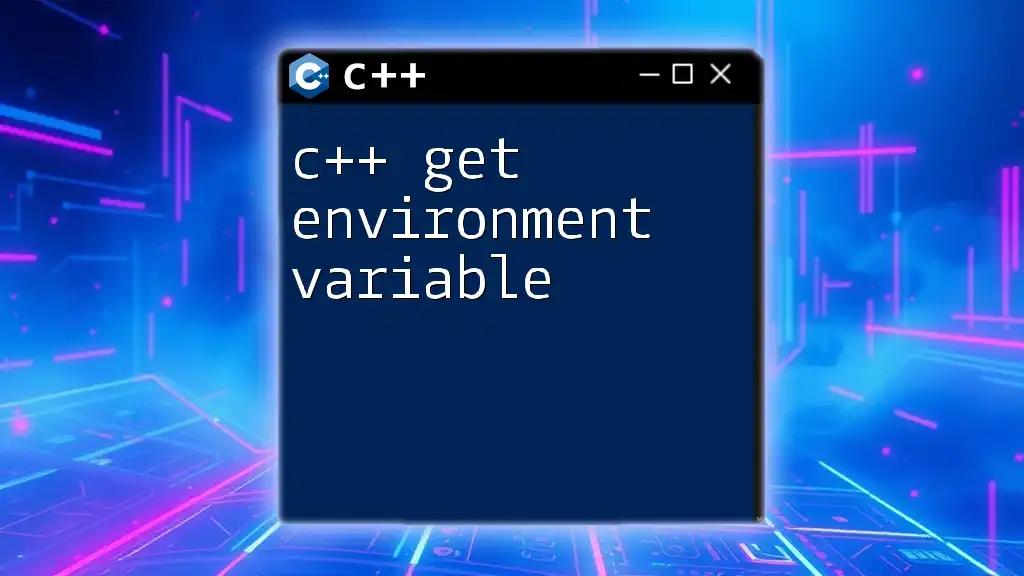
Integrated Development Environments (IDEs) and Text Editors
Recommended IDEs for C++ on macOS
There are several IDEs you can choose from, each with its advantages:
- Xcode: A robust IDE that integrates well with macOS. It supports C++ and provides a rich feature set for development. You can create a new project using the template for C++.
- CLion: A powerful IDE from JetBrains which provides excellent code assistance and debugging tools, albeit as a paid option.
- Visual Studio Code: A lightweight and highly customizable text editor. It requires additional extensions to function as a C++ development environment effectively.
Basic Configuration of IDEs
Setting up Xcode for C++ Development
To set up Xcode for C++:
- Open Xcode and create a new project.
- Select "Command Line Tool" under macOS.
- Choose C++ as the language.
Create a simple "Hello World" program in your `main.cpp` file:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Run the project using the play button in Xcode.
Configuring Visual Studio Code for C++
To configure Visual Studio Code for C++ development:
- Install VS Code from the [official website](https://code.visualstudio.com/).
- Add necessary extensions such as:
- C/C++ by Microsoft: Provides debugging and code navigation.
- Code Runner: Enables running code snippets efficiently.
- Set up a `tasks.json` file to compile and run your code easily within VS Code.
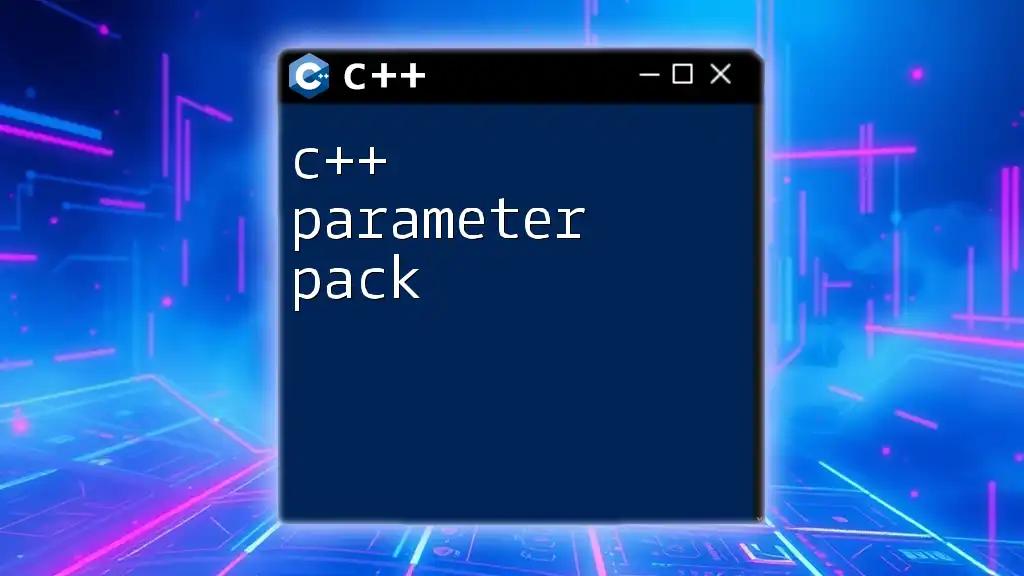
Build Tools for C++ Projects
Introduction to Build Systems
Build systems manage the compilation process of software development. They automate the creation of executables from your source code. By managing dependencies and compilation configuration, build systems help save time on repetitive tasks.
Popular Build Systems for C++
Two popular build systems are Make and CMake:
- Make: A classic tool for project builds, using Makefiles to define build rules.
- CMake: A more modern, cross-platform tool that generates native makefiles or project files for different IDEs.
Installing CMake
To leverage CMake in your Mac setup, install it via Homebrew:
brew install cmake
Example: Using CMake for a Simple Project
Creating a simple CMake project involves setting up your project folder with a `CMakeLists.txt` file. Here's a basic example:
-
Create a folder structure:
HelloWorld/ CMakeLists.txt hello.cpp
-
Write the `CMakeLists.txt` file:
cmake_minimum_required(VERSION 3.10)
project(HelloWorld)
add_executable(hello hello.cpp)
- Compile the project by navigating to the folder in the terminal and running:
cmake .
make
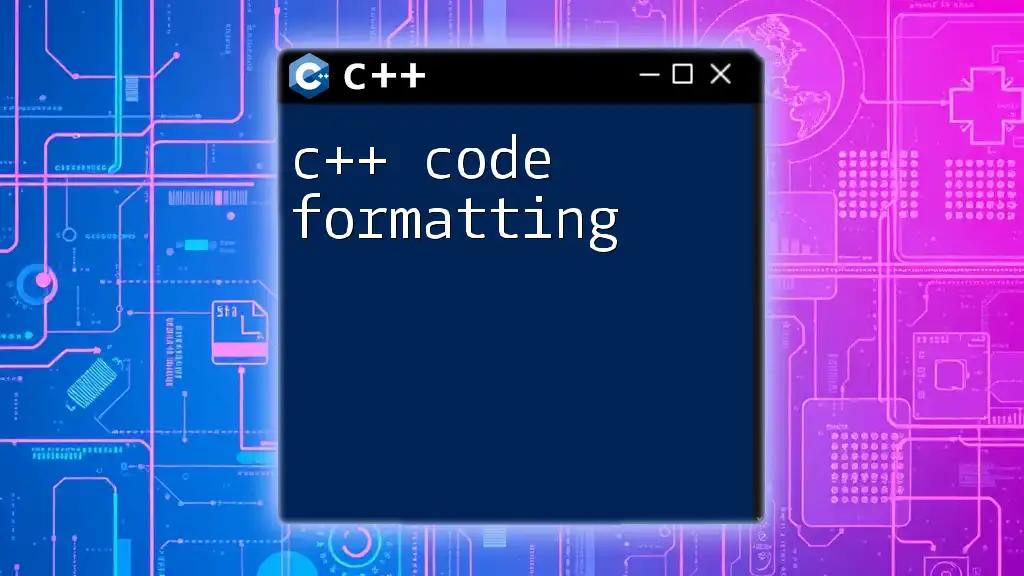
Debugging C++ Code on macOS
Importance of Debugging
Debugging enables you to identify and fix code errors, ensuring your programs run smoothly. Without proper debugging tools and practices, even small mistakes can lead to significant issues in your code.
GDB and LLDB
Among the debugging tools available for macOS, GDB (GNU Debugger) and LLDB (LLVM Debugger) are widely used. GDB is powerful but may require additional configuration. LLDB, the default for Xcode, is easier to use in a macOS-centric environment.
To install GDB, use:
brew install gdb
Sample Debugging Session
You can compile a C++ program with debugging symbols to make debugging more effective. Use the following command:
g++ -g -o hello hello.cpp
To start debugging with LLDB:
lldb ./hello
This will open the LLDB console, where you can set breakpoints, run your program, and inspect variables.
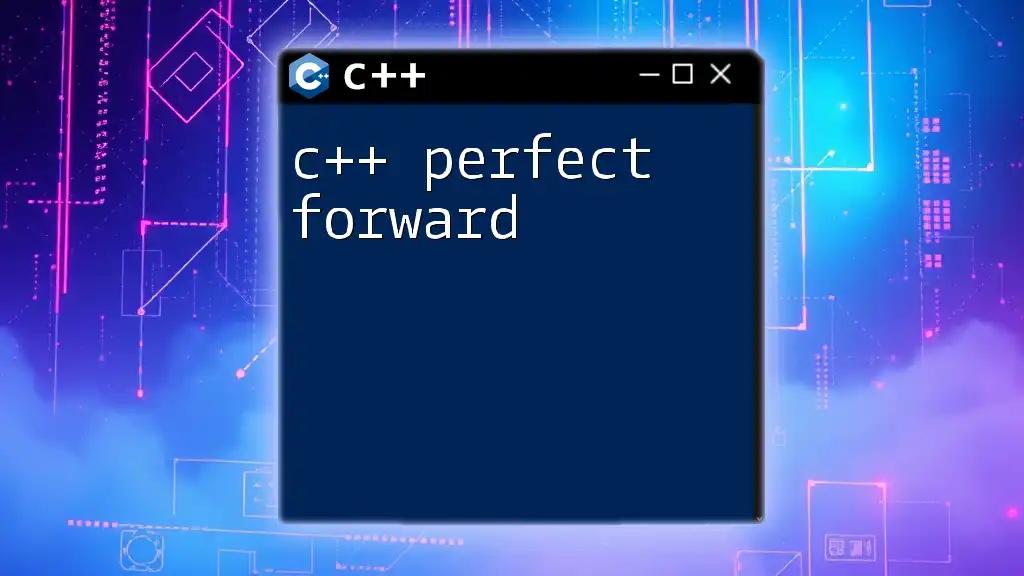
Tips and Best Practices for C++ Development on macOS
Organizing Your Projects
A well-organized project structure promotes maintainability and collaboration. Using clear naming conventions for directories and files can make navigation easier. Employing version control systems like Git is essential for tracking changes and collaborating with others.
Code Quality and Style Guides
Maintaining code quality through consistent style is vital for readability and maintainability. Following established C++ style guides, such as Google's C++ Style Guide, can help achieve this. Tools like clang-format can automate code formatting, while cpplint can check style conformance.
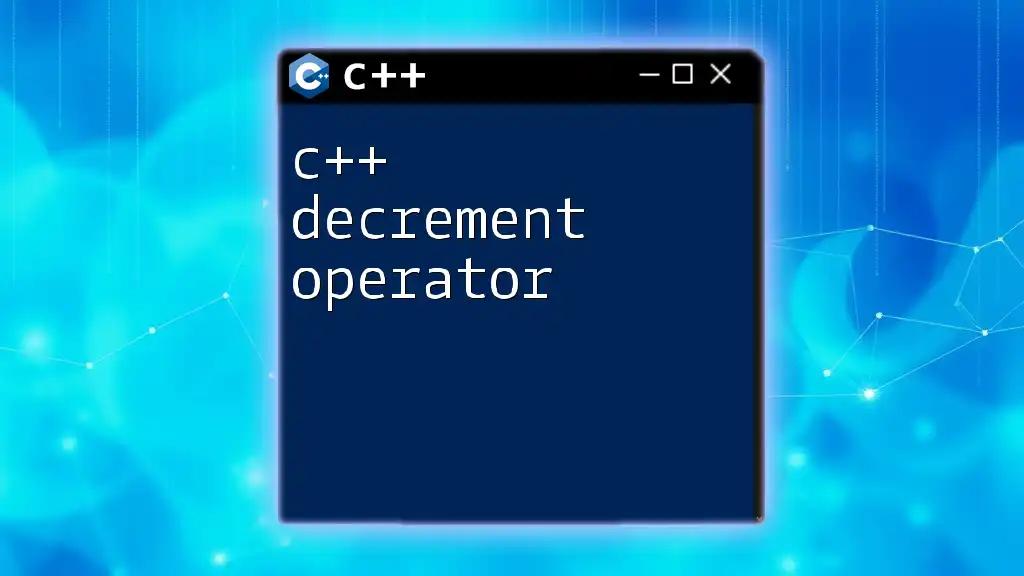
Conclusion
Setting up a C++ environment for Mac can be straightforward if you follow the right steps and use the appropriate tools. By understanding the components of a C++ setup, choosing the right IDE, and utilizing build and debugging tools, you can streamline your development process. As you progress, don't hesitate to explore more advanced tools and practices that will enhance your coding efficiency. Start coding in C++ today and take your software development skills to new heights!
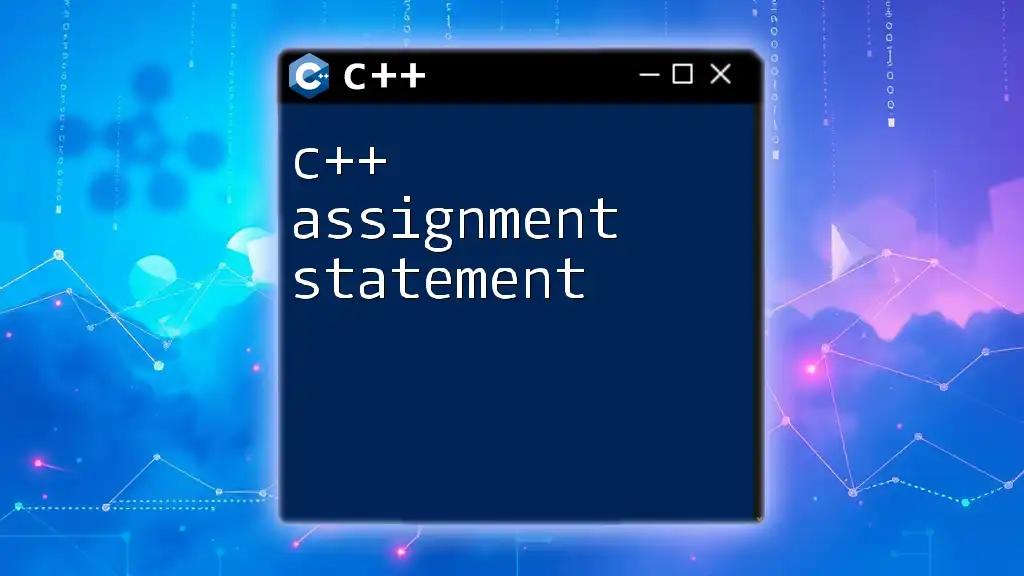
Additional Resources
For further learning, consider exploring recommended C++ books, online courses, and forums that can provide valuable insights and community support as you continue your journey into C++ programming.