C++ development on Mac involves setting up a development environment using tools like Xcode or command-line compilers to create and run C++ programs efficiently.
Here's a simple code snippet that demonstrates a basic C++ program:
#include <iostream>
int main() {
std::cout << "Hello, Mac users!" << std::endl;
return 0;
}
Setting Up Your Development Environment on macOS
Choosing the Right Hardware
When it comes to C++ development on Mac, selecting the right MacBook is crucial for ensuring an optimal development experience. A MacBook with at least 8GB of RAM is generally recommended for basic programming tasks, while 16GB or more is ideal for handling larger projects or multiple applications simultaneously. Opting for a model with an M1 or M2 chip can provide impressive performance boosts, given their efficiency and speed in compilation tasks.
Installing Xcode
Xcode is Apple’s official IDE for macOS and is highly suitable for C++ development. It comes bundled with various tools necessary for code compilation, debugging, and deployment.
To install Xcode, follow these steps:
- Open the App Store on your Mac.
- Search for “Xcode” and click the download button.
- Once downloaded, follow the installation prompts to set it up.
Installing Command Line Tools
Command Line Tools include compilers and other utilities required for C++ development. Installing these tools is simple and can be accomplished using the Terminal.
To install Command Line Tools, use the following command:
xcode-select --install
This command prompts a window to install the necessary tools required for building and running C++ programs from the Terminal.
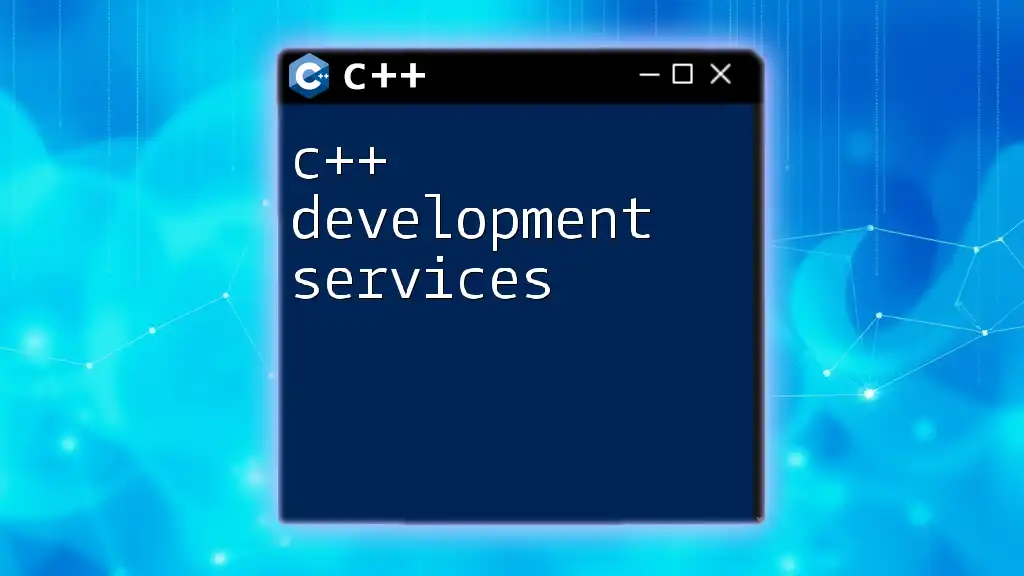
Configuring Your C++ IDE on MacBook
Alternatives to Xcode
While Xcode is an excellent choice for many developers, it’s not the only option. Several other IDEs are popular among C++ programmers on Mac, including:
- CLion: A cross-platform IDE that is well-regarded for its smart C++ editor features.
- Code::Blocks: A lightweight open-source IDE that supports multiple compilers.
- Visual Studio Code: A free, extensible code editor that can be customized for C++ development using extensions.
Setting Up Xcode for C++ Development
Once Xcode is installed, the next step is to create a new C++ project.
- Open Xcode and select “Create a new Xcode project.”
- Choose “Command Line Tool” from the macOS section.
- Select C++ from the “Language” dropdown and set your project name.
Configuring Build Settings
After your project is created, navigate to your project settings. Here, configure build options under the “Build Settings” tab to enable optimization levels and debug settings that suit your development needs.
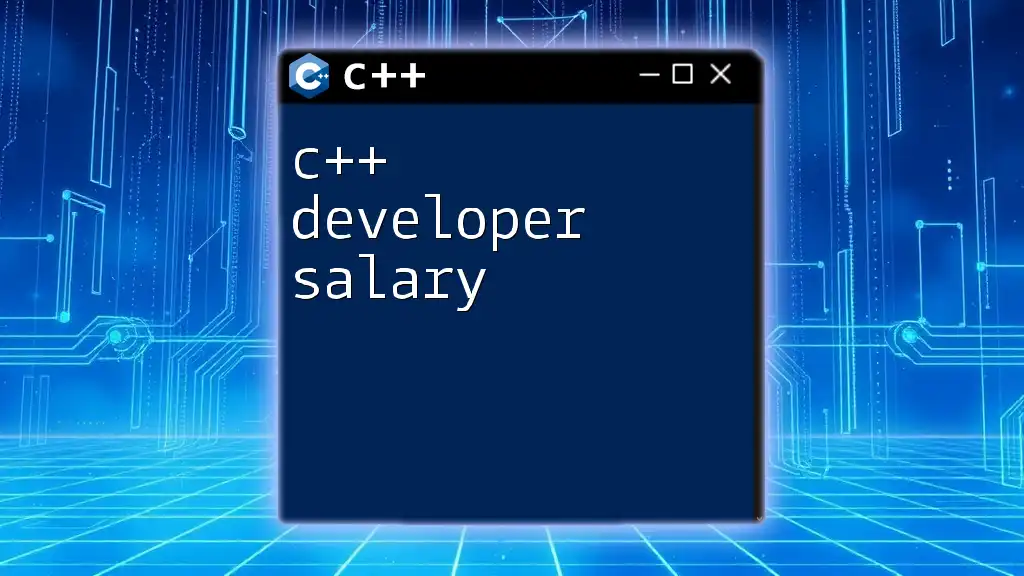
Writing Your First C++ Program on Mac
Hello World: The Classic Example
Your journey into C++ development on Mac begins with the classic "Hello World" program. This simple program serves as a foundational building block for understanding the structure of C++ code.
Step-by-Step Coding Example
Here's how to write your first C++ program in Xcode. In the main.cpp file generated by Xcode, input the following code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Explanation of Each Part of the Code
- `#include <iostream>`: This line includes the Input/Output stream library, necessary for using `std::cout`.
- `int main()`: The main function is the entry point of any C++ program.
- `std::cout << "Hello, World!" << std::endl;`: This statement outputs the text "Hello, World!" to the console.
- `return 0;`: Indicates that the program has completed successfully.
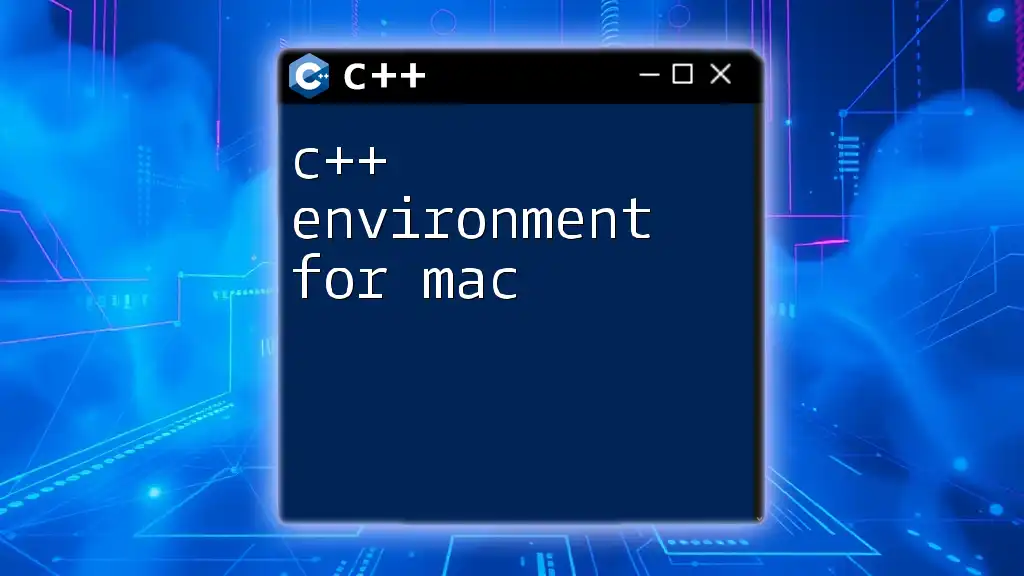
Compiling and Running C++ Programs on Mac
Using Xcode
To build and run your project in Xcode:
- Click on the play button (Run) in the top left corner.
- The debugger will execute your code, and you should see "Hello, World!" printed in the output console.
Using the Command Line
For those who prefer working directly in the Terminal, you can compile and run C++ programs without the IDE. Open Terminal, navigate to your project directory, and use the following commands:
To compile:
g++ -o HelloWorld HelloWorld.cpp
This command compiles `HelloWorld.cpp` into an executable named `HelloWorld`.
To run the program:
./HelloWorld
This executes the compiled program, displaying the output in your terminal.
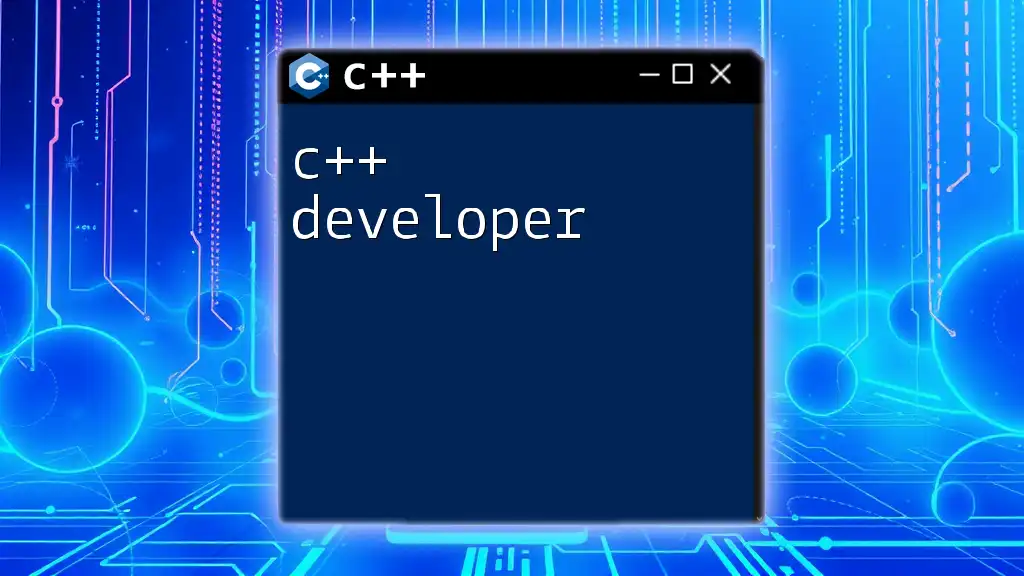
Debugging C++ Code on macOS
Built-In Debugging Tools in Xcode
Xcode provides a powerful debugging environment with features such as breakpoints, step execution, and a memory graph. To set a breakpoint, simply click in the gutter next to the line of code where you want to pause execution, allowing you to inspect variables and control the flow of the program.
Using gdb with Command Line
For those who prefer command-line debugging, you can set up gdb. First, install gdb using Homebrew:
brew install gdb
Once installed, you need to codesign gdb for it to work properly on macOS.
To run gdb against your executable:
gdb ./HelloWorld
In gdb, use basic commands such as `break` to set breakpoints, `run` to start execution, and `print` to display variable values.
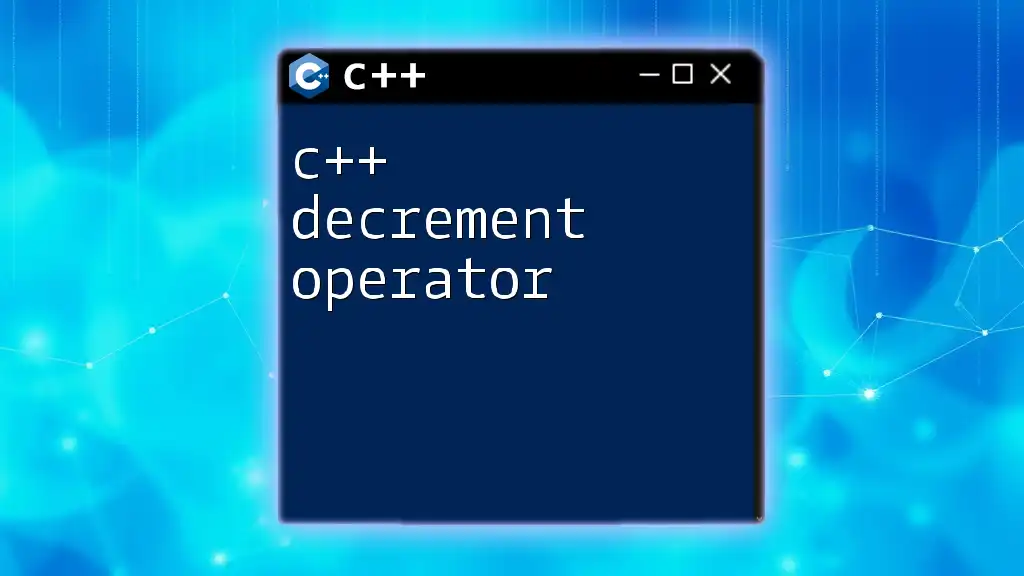
C++ Libraries and Frameworks for Mac
Popular C++ Libraries
Leveraging libraries can save time and enhance the functionality of your applications. Some widely-used libraries include:
- Boost: A collection of libraries that extend the functionality of C++.
- Qt: Particularly used for GUI application development.
- OpenCV: Ideal for computer vision projects.
Installing Libraries
One easy way to manage libraries is through Homebrew. If you haven't already installed Homebrew, you can set it up with the following command:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
Once installed, you can install libraries like Boost using:
brew install boost
Using libraries appropriately can greatly enhance development efficiency.
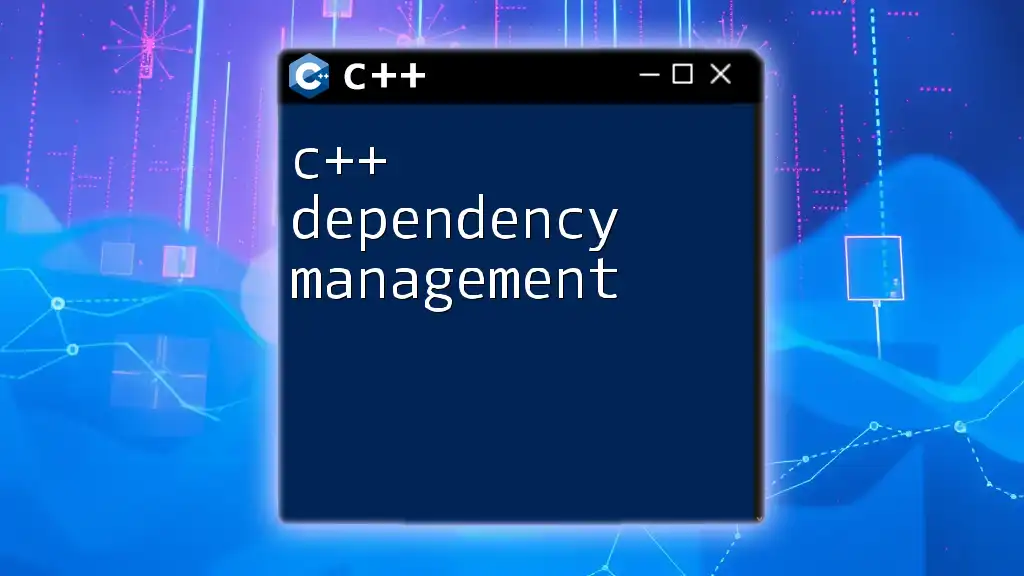
Best Practices for C++ Development on MacBook
Code Organization
Maintaining a well-structured codebase is essential for readability and maintenance. Organize your files into folders based on features or modules. Always use meaningful filenames and consistent naming conventions to improve code clarity.
Version Control with Git
Implementing version control is crucial for managing code changes. To set up Git on macOS:
- Install Git either through Homebrew or directly from the official Git website.
- Initialize a Git repository in your project directory:
git init
Use commands like `git add` and `git commit` to track changes in your code.
Frequent Coding Mistakes to Avoid
Common pitfalls in C++ can lead to frustrating debugging sessions. Some frequent mistakes include:
- Memory Management Issues: Always ensure proper allocation and deallocation of memory to prevent memory leaks.
- Undefined Behavior: Understand the significance of initialization and the consequences of accessing uninitialized variables.
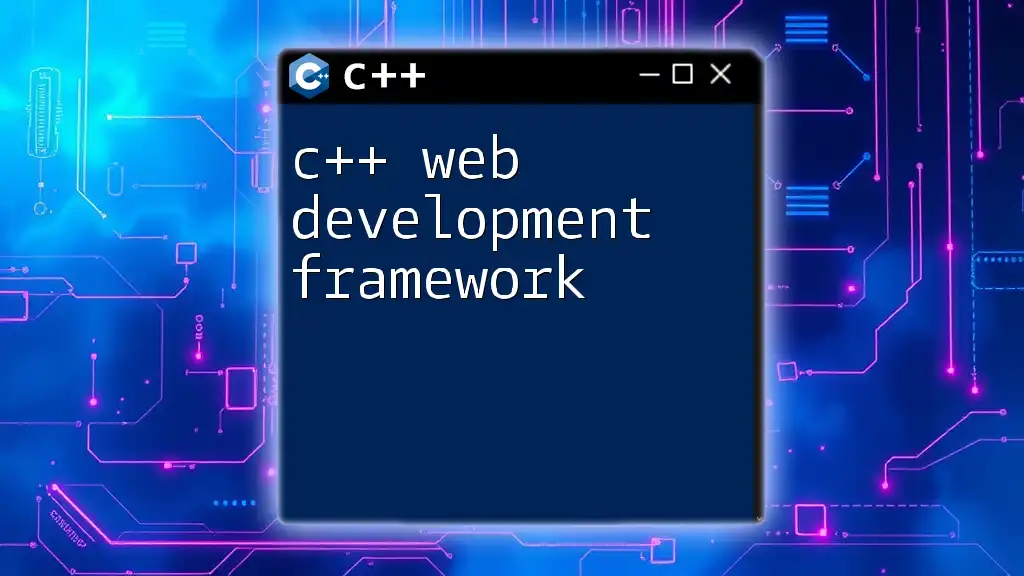
Conclusion
In conclusion, C++ development on mac is a rewarding endeavor with various tools and resources available to streamline your learning and coding experience. By setting up your environment correctly, understanding the language structures, and adopting best practices, you’ll be well on your way to becoming a proficient C++ developer. Remember to explore additional resources and keep practicing your skills for continuous improvement in leading-edge development.
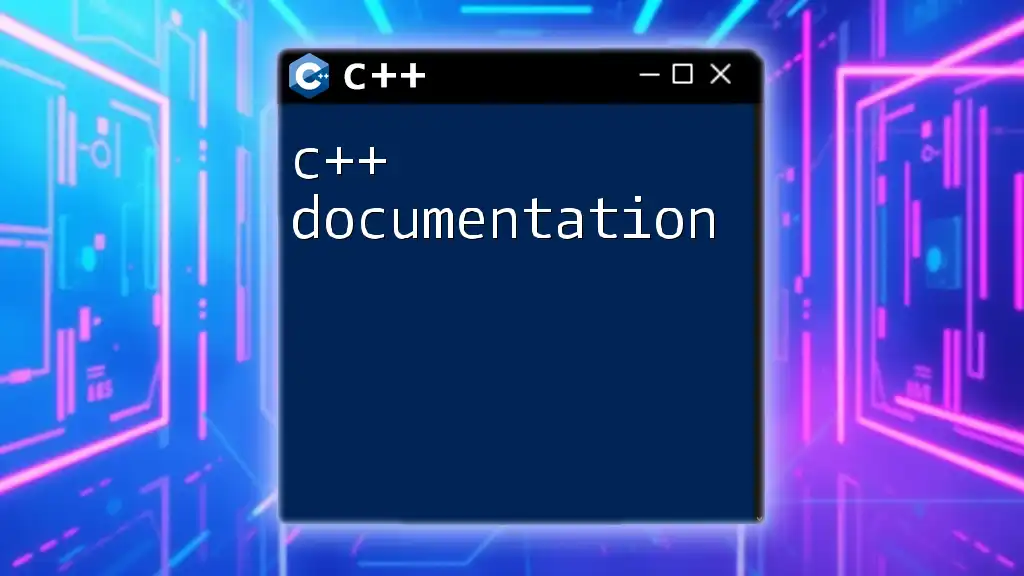
Additional Resources
For further learning about C++ development specifically tailored for Mac systems, consider exploring recommended books, online courses, and tutorials to deepen your understanding and enhance your programming skills.