C++ dependency management involves organizing and resolving the relationships between various code files and libraries to ensure smooth compilation and linkage of a program.
Here's an example of how to include a library in your C++ project using `#include`:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
Understanding Dependencies in C++
What is C++ Dependency Management?
C++ dependency management refers to the process of handling external libraries, frameworks, and modules your project relies on to function effectively. Every C++ project is likely to utilize multiple dependencies, ranging from small utility libraries to larger frameworks that introduce complex functionalities. Proper management of these dependencies is crucial for ensuring that your application builds consistently, runs reliably, and can be maintained over time.
Types of Dependencies
Static Dependencies
Static dependencies occur when a library is linked into your application during compile time. This means the entire library becomes part of your executable. One advantage is that it can simplify deployment since you do not have to distribute additional DLLs or shared libraries. However, this can increase the size of your executable and make it harder to update individual components.
Example:
If you include a static library in your project, your code might look like this:
#include "mylib.h"
// Some code that utilizes mylib
When compiled, the `mylib` code will be embedded within your executable.
Dynamic Dependencies
Dynamic dependencies are linked to your application at runtime, typically through shared libraries or DLLs. This leads to a smaller executable size, as the shared library's code is not included in your application’s binary. However, managing these dependencies requires careful tracking of library versions and ensuring they are present on the target machine.
Example:
Using a dynamic library often involves specifying it in your build system:
#include <mylib.h>
// Function call to a method in mylib
At runtime, the executable dynamically links to `mylib`, which must be available on the system.
Common Scenarios for Dependencies
C++ projects often require various dependencies based on their functionality. Common scenarios include:
- External Libraries: Many projects leverage well-established libraries like Boost, OpenCV, or even third-party APIs. Properly managing these ensures your project remains aligned with updates and bug fixes.
- Inter-project Dependencies: For larger systems, you might have multiple interconnected C++ projects. Coordinating dependencies between them is crucial to maintain project integrity and consistency.
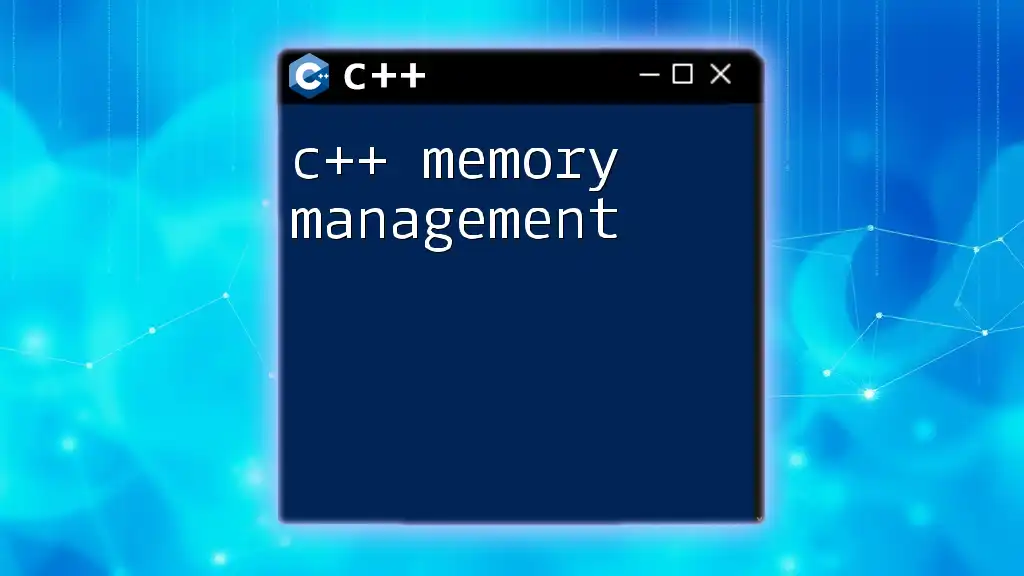
Popular Dependency Management Tools
CMake
CMake is a cross-platform build system generator that simplifies the process of managing project builds and dependencies. It enables developers to declare dependencies declaratively using `CMakeLists.txt` files.
Configuration Example:
cmake_minimum_required(VERSION 3.10)
project(MyProject)
find_package(MyLibrary REQUIRED)
target_link_libraries(MyProject MyLibrary::MyLibrary)
Here, `find_package` locates `MyLibrary`, and `target_link_libraries` links it against `MyProject`, ensuring that the library's functionalities are available.
Conan
Conan is a C/C++ package manager designed to handle binary packages. It facilitates versioning, build configurations, and dependency resolution.
Setting up Conan:
conan new mylib/0.1 -t # Creates a new library
conan install . --build=missing # Installs dependencies
This command sets up your library and manages its dependencies effectively.
vcpkg
vcpkg is a tool for managing C++ libraries on Windows, Linux, and macOS. It integrates seamlessly with CMake, making it easier to bring external libraries into your project.
Integration Example:
vcpkg install fmt # Installing a library
cmake -DCMAKE_TOOLCHAIN_FILE=<vcpkg-root>/scripts/buildsystems/vcpkg.cmake
By using vcpkg, you're ensuring that all dependencies are handled smoothly.
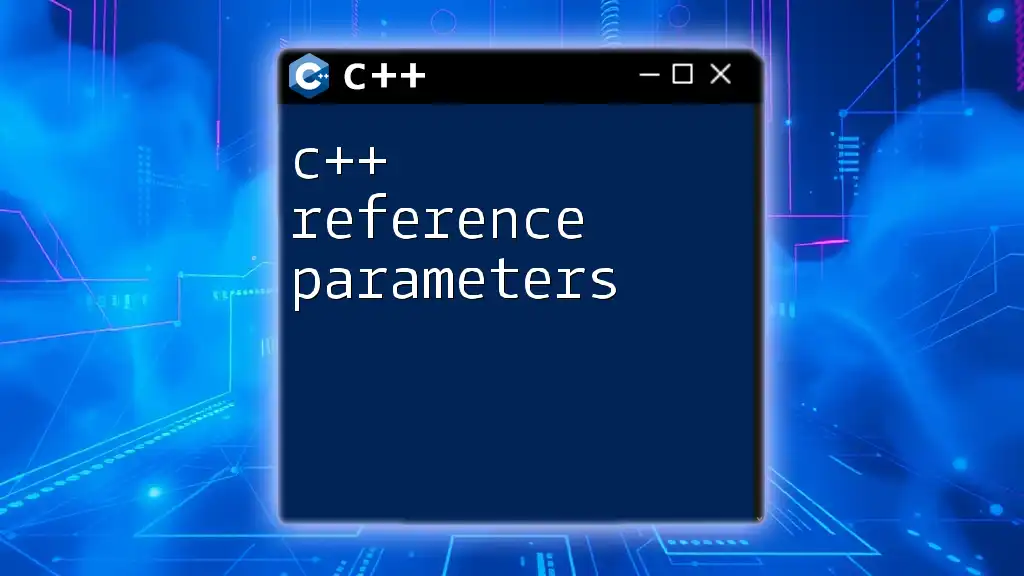
Setting Up Dependency Management in Your C++ Project
Choosing the Right Tool for Your Needs
Selecting the right dependency management tool depends on various factors, such as your project's size, scope, and specific requirements. Evaluate the community support available for each tool and the ecosystem they provide.
Creating a Dependency Manifest
A dependency manifest is a file that lists and describes all the dependencies your project uses. This document can be invaluable for maintaining your project, as it serves as a single source of truth. It's recommended to include the following elements in your manifest:
- Library Name and Version: To ensure you are using the correct versions.
- License Information: To abide by licensing agreements.
- Installation Instructions: Clear guidelines for how to install dependencies.
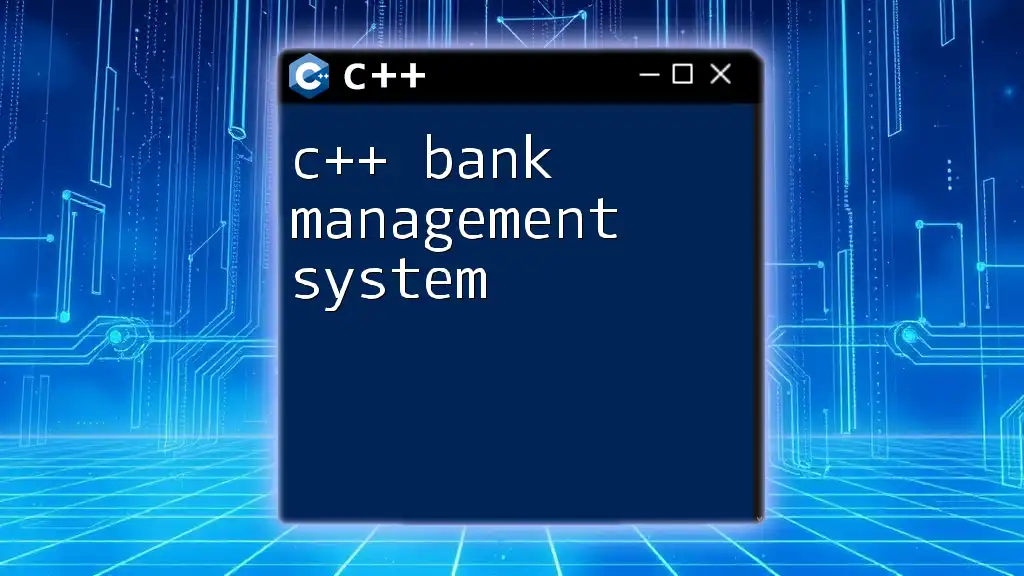
Managing Version Conflicts
Understanding Semantic Versioning
Semantic Versioning is a versioning scheme that consists of three parts: major, minor, and patch. It provides clarity on the nature of changes in each version. When managing dependencies, following semantic versioning helps you avoid breaking changes that could affect your project.
Resolving Version Conflicts
When different packages depend on conflicting versions of the same library, you can face challenging dependency resolution issues. It is crucial to adopt strategies to manage these conflicts effectively. Tools like Conan provide mechanisms for resolving such conflicts by specifying required versions:
# Example conanfile.txt with version conflict resolution
[requires]
fmt/7.1.3
[options]
fmt:shared=True
This ensures that your project can resolve dependencies correctly.
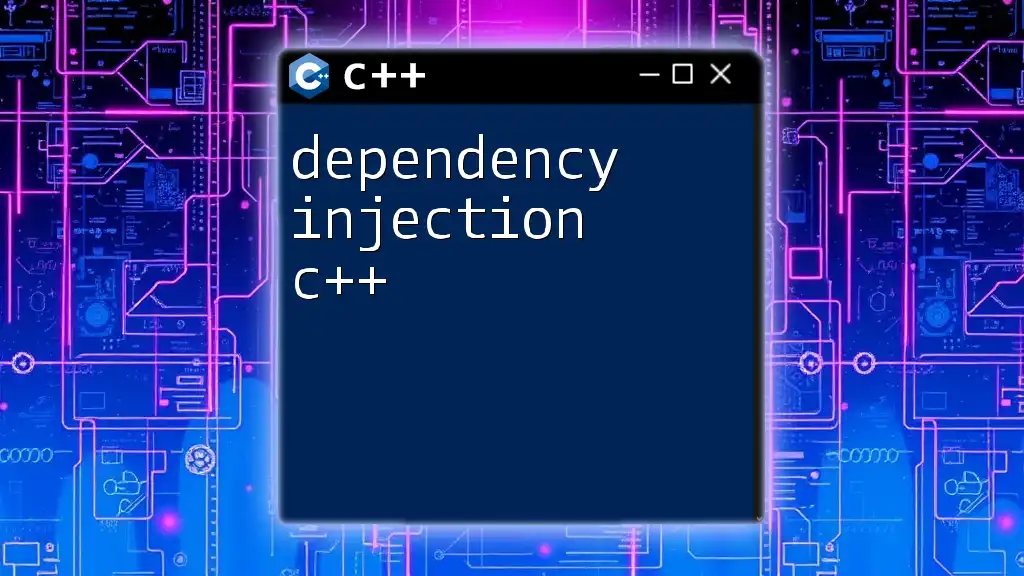
Best Practices for C++ Dependency Management
Keep Dependencies Updated
Maintaining updated libraries is essential in avoiding security vulnerabilities and ensuring optimal performance. Regularly check for updates in dependencies, and consider utilizing tools to automate this process.
Minimize Dependency Footprint
Reducing the number of dependencies can lead to simpler builds and fewer potential conflict scenarios. Wherever possible, prefer header-only libraries that do not require linking to external binaries.
Testing and Validation
Comprehensive testing is vital, especially when managing multiple dependencies. Ensure to thoroughly test all paths where your dependencies are utilized. Consider incorporating Continuous Integration (CI) practices to automate the testing of dependency resolutions.
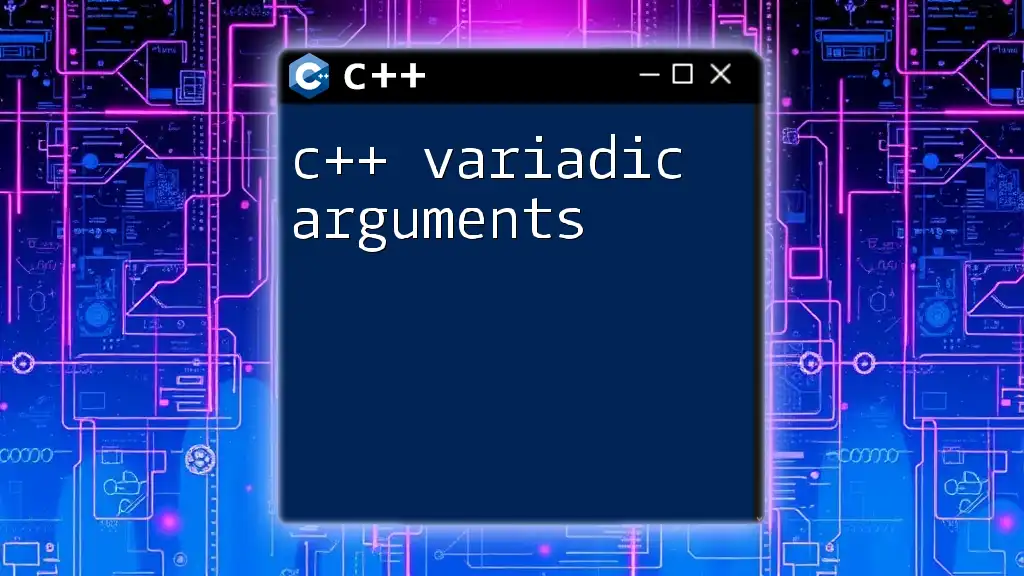
Troubleshooting Common Dependency Issues
Common Error Messages and Solutions
Often, C++ developers encounter error messages related to missing dependencies or version conflicts. Some common examples include missing headers or library files, often accompanied by error codes. A systematic approach to troubleshooting can help resolve these quickly.
Practical Tips:
- Use verbose output options in your build system to gain more insight into what might be going wrong.
- Ensure that the correct paths are set in your project configuration.
Debugging Dependency Resolution Issues
When facing dependency resolution failures, make use of available tools and methods for tracking down resolution issues. For instance, Conan offers commands that provide insight into dependency trees, allowing you to understand what libraries are being pulled in.
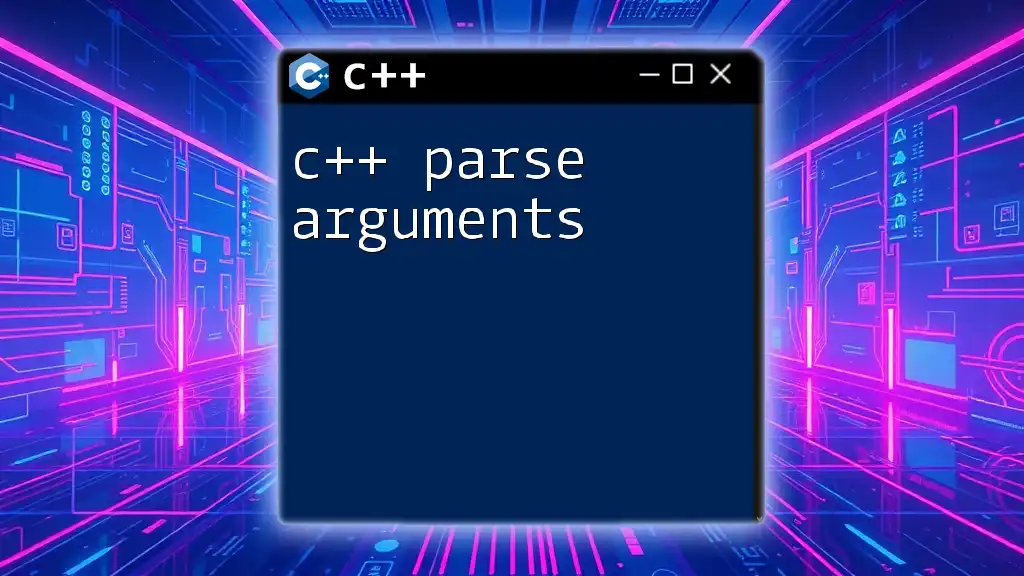
Conclusion
Effective C++ dependency management is integral to modern software development. By carefully selecting tools, maintaining versioning discipline, and implementing best practices, you can stay agile and responsive to changes in your project’s needs. Dive into dependency management to enhance the stability, performance, and maintainability of your C++ applications and take your development skills to the next level!
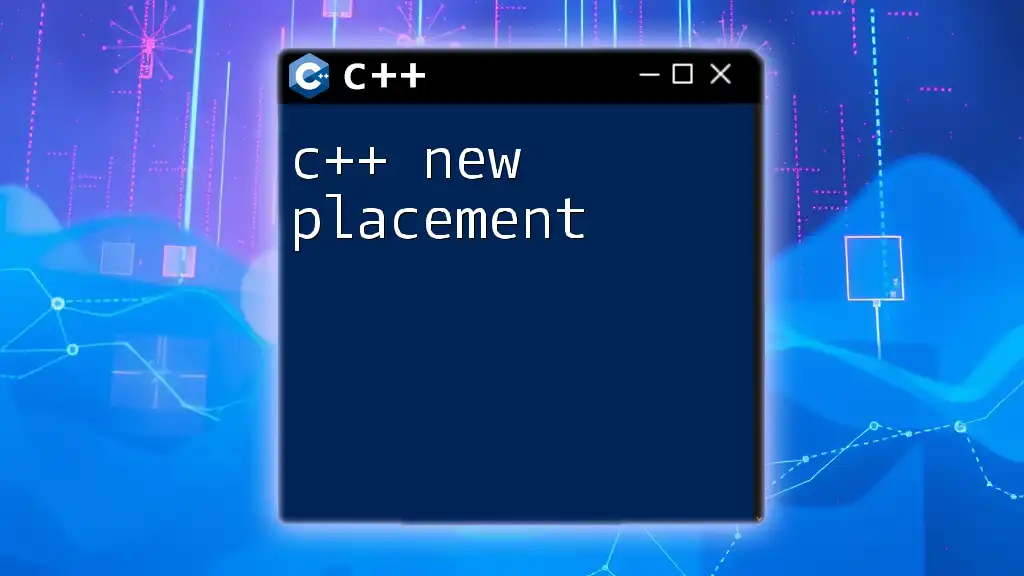
Additional Resources
For further exploration into C++ dependency management, consider delving into reputable books and websites dedicated to C++ development and package management tools. This continuous learning will empower you to navigate the ever-evolving landscape of C++ programming effectively.