An adjacency matrix in C++ is a two-dimensional array used to represent a finite graph, where each element indicates whether pairs of vertices are adjacent or not.
#include <iostream>
#include <vector>
int main() {
// Example graph with 4 vertices
const int V = 4;
std::vector<std::vector<int>> adjMatrix(V, std::vector<int>(V, 0));
// Adding edges: 0-1, 0-2, 1-3
adjMatrix[0][1] = 1;
adjMatrix[0][2] = 1;
adjMatrix[1][3] = 1;
// Displaying the adjacency matrix
for (const auto &row : adjMatrix) {
for (int val : row) {
std::cout << val << " ";
}
std::cout << std::endl;
}
return 0;
}
What is an Adjacency Matrix?
An adjacency matrix is a powerful data structure used to represent graphs. It provides a clear and concise way to indicate the relationships between vertices (or nodes) within the graph. Each entry in the matrix indicates whether pairs of vertices are adjacent or not, which is essential for various graph algorithms.
Benefits of Using Adjacency Matrices
Using an adjacency matrix offers several advantages:
-
Quick Edge Lookup: You can quickly check if an edge exists between any two vertices by simply accessing the corresponding matrix entry. This operation is \( O(1) \), making it very efficient.
-
Simplicity: The matrix representation is straightforward and easy to understand, especially for smaller graphs.
However, it’s important to consider space complexity. The adjacency matrix requires \( O(V^2) \) space, where \( V \) is the number of vertices. This can become impractical for large, sparse graphs, where an adjacency list might be more efficient.
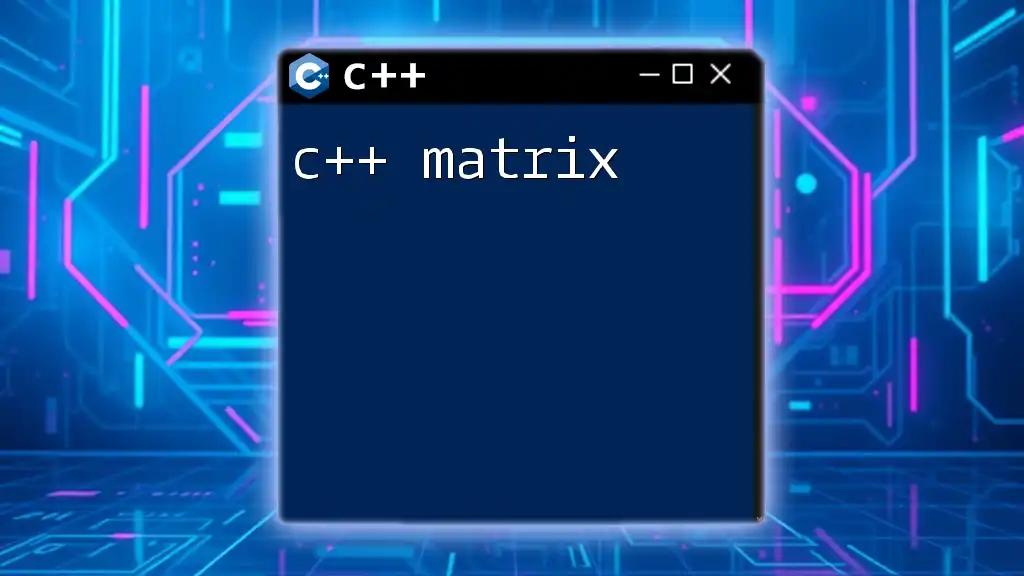
Understanding Graphs
Types of Graphs
Graphs can vary significantly based on their properties:
-
Directed vs. Undirected Graphs: In a directed graph, edges have a direction (from vertex A to vertex B). In contrast, undirected graphs have edges that indicate a bidirectional relationship between vertices.
-
Weighted vs. Unweighted Graphs: Weighted graphs assign a value (weight) to each edge, indicating the cost, distance, or capacity, whereas unweighted graphs treat all edges equally.
Representation of Graphs
While there are several ways to represent graphs (like adjacency lists and edge lists), an adjacency matrix is particularly useful when quick access to edge information is needed.
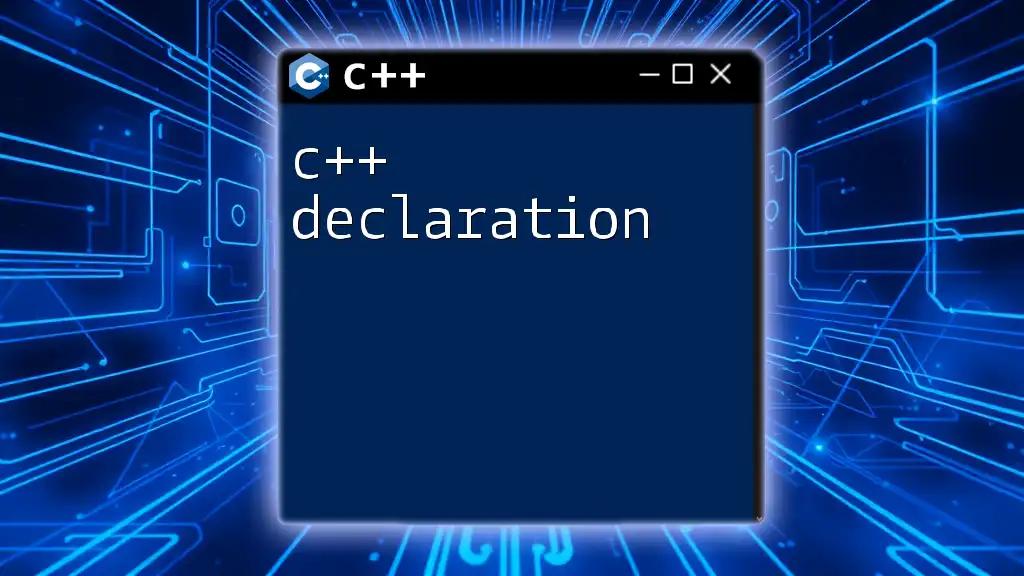
Creating an Adjacency Matrix in C++
Defining the Matrix
To represent a graph using an adjacency matrix in C++, you first declare a 2D array. This array needs to be of size \( V \times V \) (where \( V \) is the number of vertices). Each element in the array will either be `0` (no edge) or `1` (edge exists).
const int MAX_VERTICES = 100;
int adjacencyMatrix[MAX_VERTICES][MAX_VERTICES] = {0};
Initializing the Matrix
Before using the matrix, it should be initialized to ensure all values are set to `0`.
void initializeMatrix(int matrix[MAX_VERTICES][MAX_VERTICES], int vertices) {
for(int i = 0; i < vertices; i++) {
for(int j = 0; j < vertices; j++) {
matrix[i][j] = 0;
}
}
}
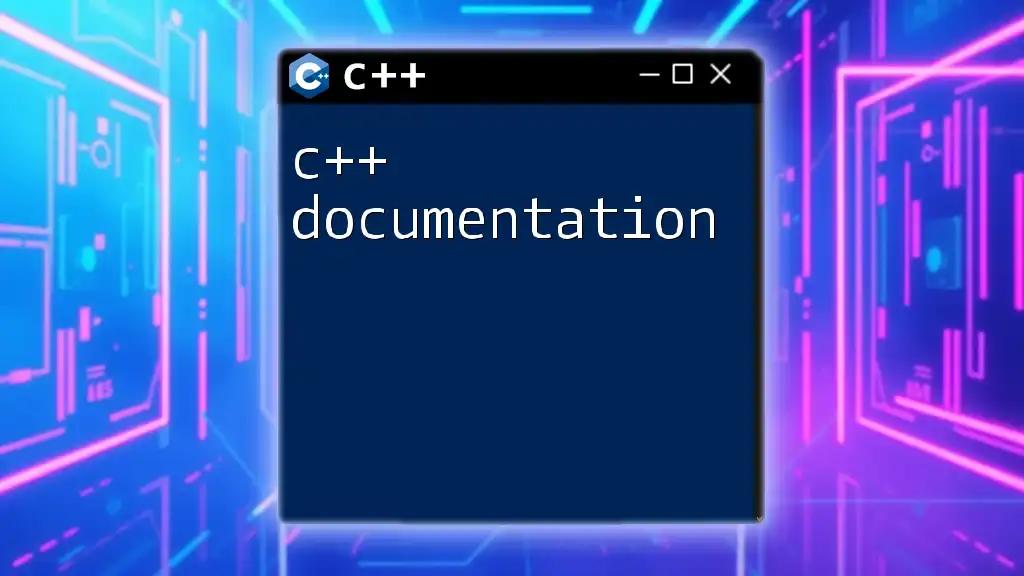
Populating the Adjacency Matrix
Inserting Edge in an Undirected Graph
To add edges to an undirected graph, set both `matrix[start][end]` and `matrix[end][start]` to `1`.
void addEdge(int matrix[MAX_VERTICES][MAX_VERTICES], int start, int end) {
matrix[start][end] = 1;
matrix[end][start] = 1; // For undirected graph
}
Inserting Edge in a Directed Graph
For directed graphs, only set the `matrix[start][end]` to `1`.
void addDirectedEdge(int matrix[MAX_VERTICES][MAX_VERTICES], int start, int end) {
matrix[start][end] = 1;
}
Inserting Weighted Edges
When working with weighted graphs, you can store the weights in the adjacent matrix.
void addWeightedEdge(int matrix[MAX_VERTICES][MAX_VERTICES], int start, int end, int weight) {
matrix[start][end] = weight; // Weight can be any integer
}
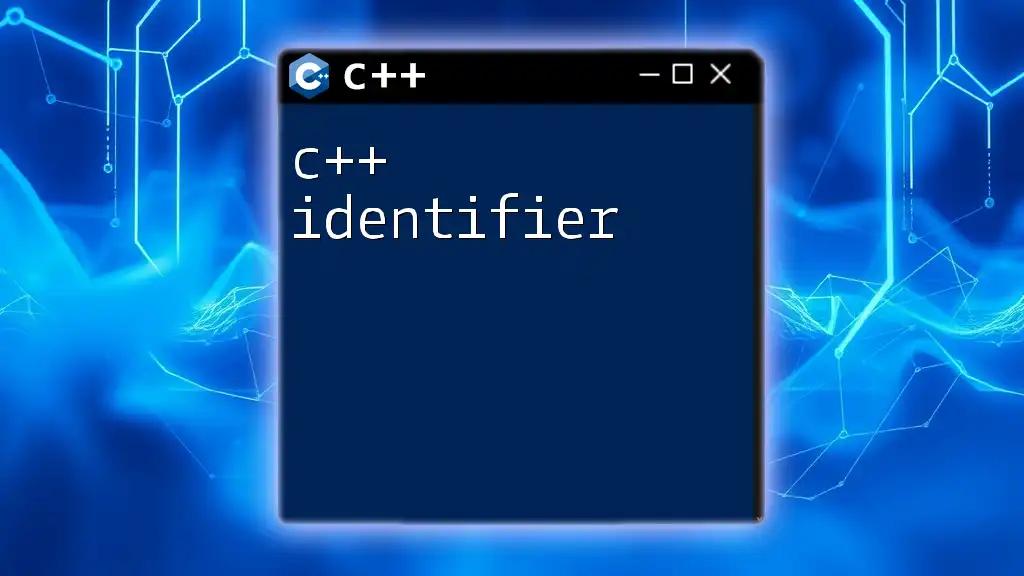
Traversing the Adjacency Matrix
Depth-First Search (DFS) Implementation
DFS is a popular graph traversal technique. It explores as far as possible along each branch before backtracking. Here’s a basic implementation:
void DFS(int matrix[MAX_VERTICES][MAX_VERTICES], int visited[], int vertex, int vertices) {
visited[vertex] = 1;
cout << vertex << " ";
for (int i = 0; i < vertices; i++) {
if (matrix[vertex][i] == 1 && !visited[i]) {
DFS(matrix, visited, i, vertices);
}
}
}
Breadth-First Search (BFS) Implementation
BFS traverses the graph level by level, ensuring that it visits all neighbors before moving on to the next level. Here’s how you can implement BFS:
void BFS(int matrix[MAX_VERTICES][MAX_VERTICES], int start, int vertices) {
queue<int> q;
bool visited[MAX_VERTICES] = {false};
q.push(start);
visited[start] = true;
while (!q.empty()) {
int vertex = q.front();
q.pop();
cout << vertex << " ";
for (int i = 0; i < vertices; i++) {
if (matrix[vertex][i] == 1 && !visited[i]) {
q.push(i);
visited[i] = true;
}
}
}
}
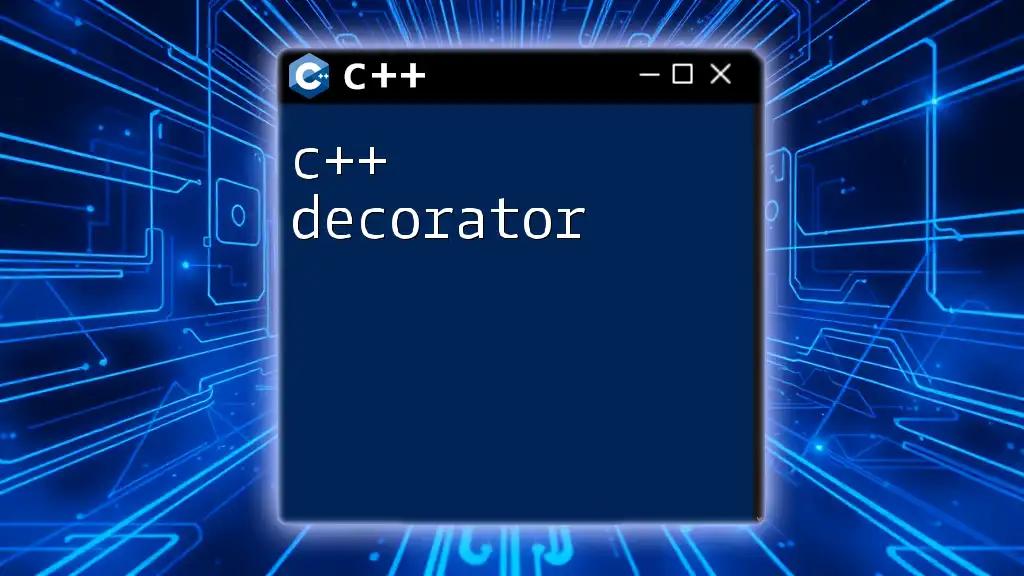
Recap of Key Takeaways
In summary, the C++ adjacency matrix is a great option for visually and logically representing graphs. Its ability to quickly check for edge existence makes it valuable for many graph algorithms. However, be mindful of space considerations, especially with large graphs.

Additional Resources for Learning C++ Graph Algorithms
For those eager to dive deeper into graph theory and algorithms in C++, many online courses and textbooks provide excellent information and hands-on exercises. Websites such as Coursera, Udacity, and books like "Introduction to Algorithms" by Cormen et al. are excellent starting points.

FAQs About Adjacency Matrix in C++
What is the time complexity of using an adjacency matrix? The time complexity for operations like edge insertion, deletion, or check is \( O(1) \), but traversing all edges takes \( O(V^2) \).
Can an adjacency matrix be used for sparse graphs? While technically feasible, it’s inefficient due to its space requirements. An adjacency list is more suitable for sparse graphs.
How does the adjacency matrix compare to the adjacency list? While an adjacency matrix provides fast edge lookup, it consumes more space, making an adjacency list more efficient for sparse graphs. The choice depends on specific use cases and requirements.

Join Our C++ Learning Platform
If you want to master the use of C++ adjacency matrix and other critical graph representation techniques, consider signing up for our classes. With a focus on practical coding skills and quick learning, we’re here to help you succeed!