C++ access modifiers control the visibility and accessibility of class members, with three primary types: public, protected, and private.
class MyClass {
public:
int publicVar; // Accessible from anywhere
protected:
int protectedVar; // Accessible within the class and derived classes
private:
int privateVar; // Accessible only within the class itself
};
What are Access Modifiers in C++?
Access modifiers in C++ are keywords that set the accessibility of class members (attributes and methods). They play a crucial role in defining how and where a class's members can be accessed in your programs. The primary purpose of access modifiers is to achieve encapsulation—a fundamental principle of object-oriented programming (OOP) that helps prevent the accidental modification of data and promotes code integrity.
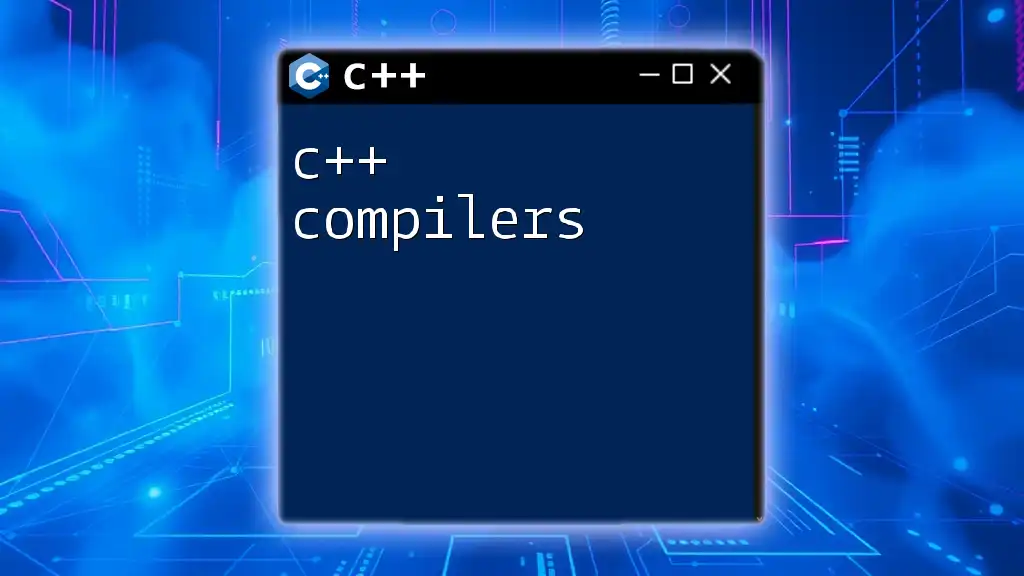
Types of Access Modifiers
Public Access Modifier
The public access modifier is the most permissive of the three main types. Members declared as public are accessible from anywhere in the program where the class object is visible.
For instance:
class MyClass {
public:
int publicVar;
void publicMethod() {
// This is a public method
}
};
In this example, both `publicVar` and `publicMethod()` are accessible from outside the class. You can instantiate `MyClass` and modify `publicVar` directly:
MyClass obj;
obj.publicVar = 10; // Accessible
obj.publicMethod(); // Also accessible
Private Access Modifier
The private access modifier restricts access to class members such that they can only be accessed within the same class. This is useful for encapsulating data, ensuring that it cannot be modified directly from outside the class.
For example:
class MyClass {
private:
int privateVar;
void privateMethod() {
// A private method
}
public:
void setPrivateVar(int val) {
privateVar = val; // Allowed
}
int getPrivateVar() {
return privateVar; // Allowed
}
};
Here, `privateVar` and `privateMethod()` are inaccessible from outside the class, ensuring that they can only be modified or accessed through the public methods `setPrivateVar()` and `getPrivateVar()`. This encapsulation is essential for maintaining the integrity of your class's data.
Protected Access Modifier
The protected access modifier strikes a balance between public and private. Members declared as protected can be accessed within the same class and by derived classes (subclasses). However, they remain inaccessible to any code outside the class hierarchy.
Consider this example:
class Base {
protected:
int protectedVar;
public:
Base() : protectedVar(0) {}
};
Derived classes can access `protectedVar`:
class Derived : public Base {
public:
void modifyVar(int val) {
protectedVar = val; // Allowed
}
};
In this case, `protectedVar` is not accessible from outside `Base` or `Derived`, ensuring a level of control while still allowing inheritance.
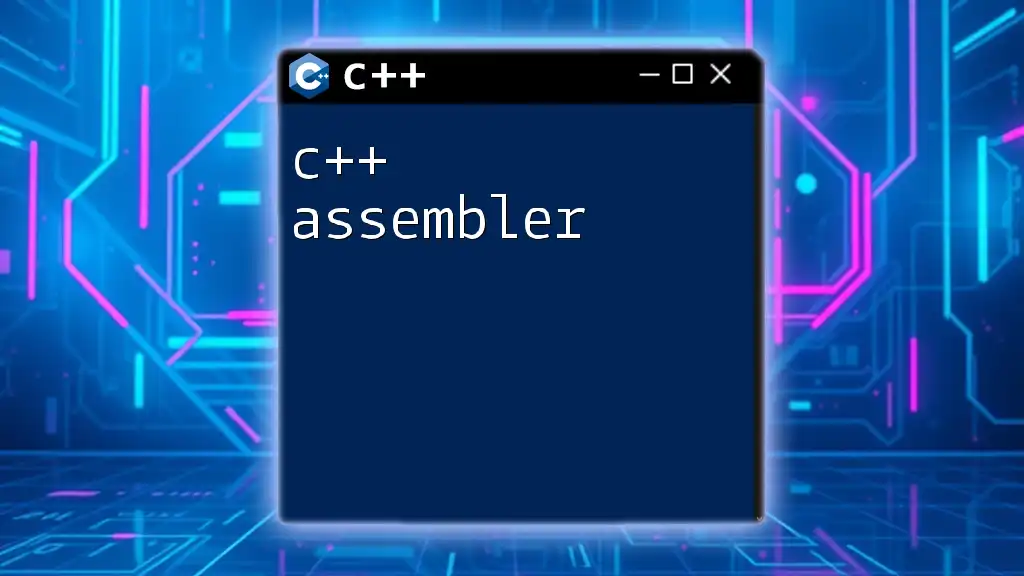
Default Access Modifier
In C++, if no access modifier is specified, the default access level for class members is private, while for struct members it is public. This implicit default access can lead to confusion, especially for those new to C++. It's crucial to be aware of this behavior to avoid unintended access control issues.
Example of default access:
class DefaultAccess {
int memberVar; // Implicitly private
};
struct DefaultStruct {
int memberVar; // Implicitly public
};
In this code, `memberVar` in `DefaultAccess` is private by default, while in `DefaultStruct`, it is public.
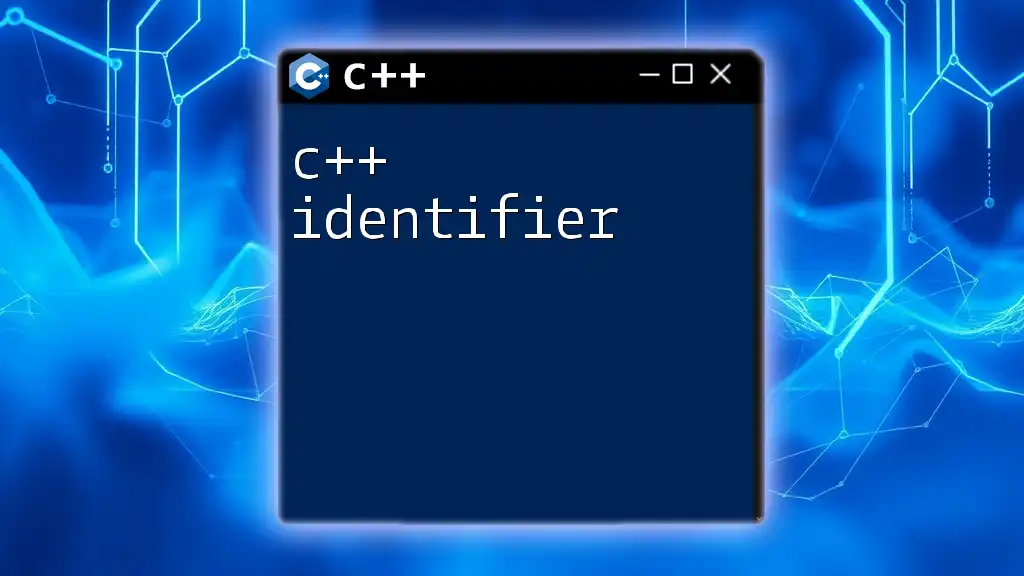
Comparison of Access Modifiers
When comparing public, private, and protected access modifiers, it's evident they serve different roles:
- Public: No restrictions; accessible from anywhere.
- Private: Restricted access; only within the class.
- Protected: Accessible in the class and derived classes but not from outside.
Choosing the right access modifier is critical for designing robust classes. Use public for interfaces and operations that should be exposed, private for internal states or functionalities that maintain the class's integrity, and protected when you want derived classes to have necessary access without exposing the data to all.
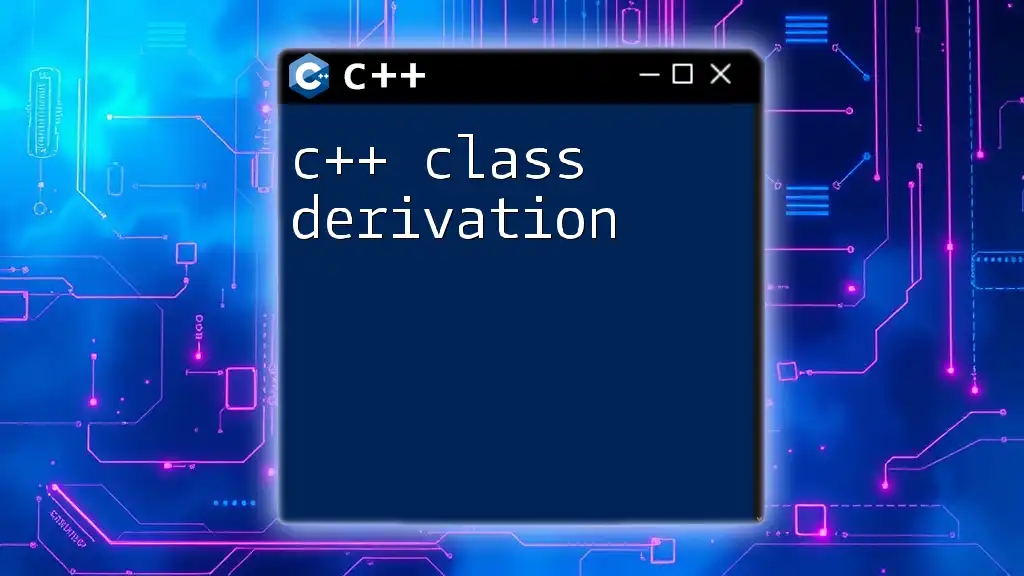
Access Modifiers in Inheritance
Inheritance and Access Modifiers
Access modifiers directly affect how base classes' members are inherited and accessed in derived classes. In C++, there are three types of inheritance: public, protected, and private.
Public Inheritance
Public inheritance keeps the base class members' access modifiers intact:
class Base {
public:
void show() {}
protected:
void display() {}
private:
void secret() {}
};
class Derived : public Base {
public:
void accessMembers() {
show(); // Accessible (public)
display(); // Accessible (protected)
// secret(); // Not accessible (private)
}
};
Protected Inheritance
Protected inheritance protects the base class members for derived classes:
class Derived : protected Base {
public:
void accessMembers() {
show(); // Accessible (protected)
display(); // Accessible (protected)
// secret(); // Not accessible (private)
}
};
Private Inheritance
Private inheritance restricts access to the base class members:
class Derived : private Base {
public:
void accessMembers() {
show(); // Accessible (private)
display(); // Accessible (private)
// secret(); // Not accessible (private)
}
};
In this scenario, the derived class can access all base members, but they cannot be accessed from outside the derived class. Understanding how to leverage these inheritance types in conjunction with access modifiers is essential for proper encapsulation and data abstraction.
Accessing Base Class Members
The ability of derived classes to access base class members depends on the access modifier used. For example, private members of the base class cannot be accessed via the derived class, emphasizing the need to utilize public and protected methods to interact with such data.
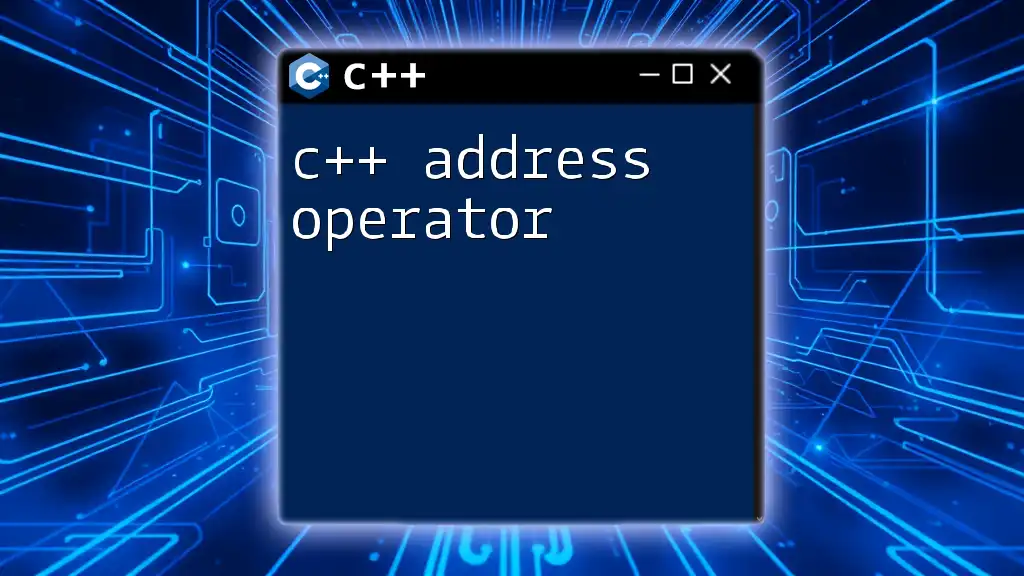
Best Practices for Using Access Modifiers
When deciding on access modifiers, follow these best practices:
- Use Private as the Default: Always start with private access to encapsulate data and expose only what is necessary.
- Favor Public Interfaces: Design class interfaces to be public for access and manipulation of data via public methods.
- Use Protected for Inheritance: Only use protected for data that should be accessed by derived classes.
- Be Consistent: Maintain consistency across your codebase to streamline understanding and management of access levels.
- Keep Security as a Priority: Consider encapsulation a security measure to protect data integrity.
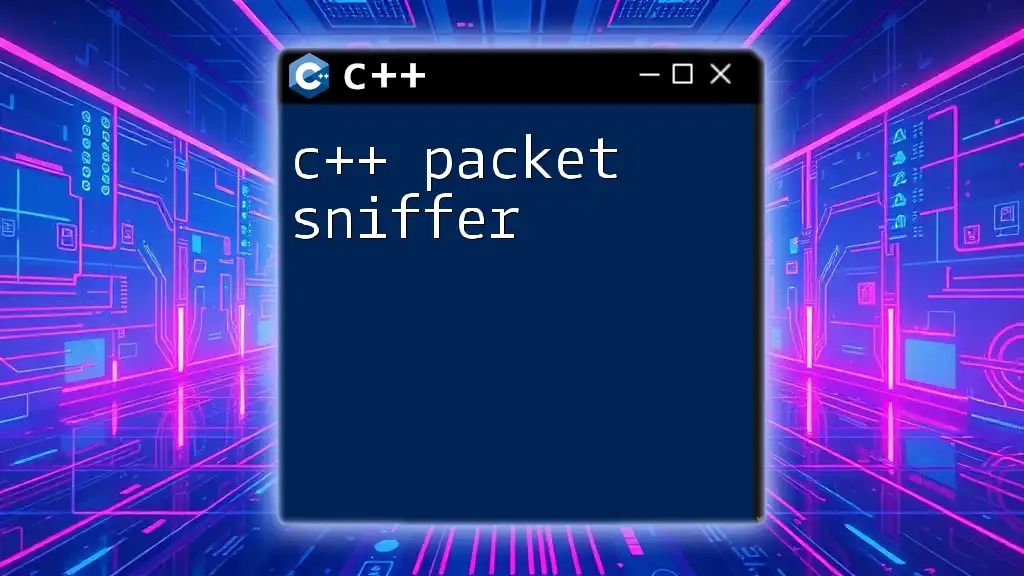
Conclusion
Understanding C++ access modifiers is paramount for effective object-oriented programming. They not only dictate the accessibility of class members but also encourage good design principles such as encapsulation and data hiding. By mastering these concepts, you enhance your ability to write robust, maintainable, and high-quality C++ code. Embrace these principles in your coding practices, and you'll pave the way for cleaner and more secure applications.