C++ automotive refers to the application of C++ programming in the automotive industry, particularly for creating software that controls vehicle systems and enhances functionalities.
Here's a simple example demonstrating a basic C++ structure for an automotive application that manages vehicle speed:
#include <iostream>
class Vehicle {
public:
void setSpeed(int s) {
speed = s;
std::cout << "Vehicle speed set to: " << speed << " km/h" << std::endl;
}
private:
int speed;
};
int main() {
Vehicle myCar;
myCar.setSpeed(100);
return 0;
}
Understanding the Role of C++ in Automotive
What Makes C++ Valuable for Automotive Software?
C++ holds a prominent position in the automotive industry due to its high performance, object-oriented programming capabilities, and ability to handle real-time processing effectively.
-
Performance: C++ allows automotive applications to run efficiently with minimal overhead, making it ideal for the resource-constrained environments typically found in embedded systems. Its capability for both high-level abstraction and low-level memory manipulation can lead to optimized code that runs faster and uses less memory.
-
Object-Oriented Programming: The OOP paradigm enables developers to create reusable code components, which simplifies the management of complex software architectures. This is particularly advantageous in automotive software, where different subsystems need to be integrated seamlessly. Class hierarchies can be built to encapsulate functionality and create a modular system that is more straightforward to maintain and extend.
-
Real-Time Processing: In many automotive applications, timely execution of tasks is critical. C++ allows for precise control over system resources, enabling developers to create real-time applications that meet strict timing requirements. This is essential for control systems, where delays can lead to unsafe operational states.
Common Applications of C++ in Automotive
C++ finds applications in various domains within the automotive industry. Some of the most common include:
-
Embedded Systems: These are dedicated computer systems designed to perform specific tasks within a vehicle, such as controlling the engine or managing infotainment systems. For instance, many Electronic Control Units (ECUs) in vehicles run on C++ due to its ability to yield efficient and reliable execution.
-
Control Systems: C++ is widely used for developing control algorithms that regulate vehicle dynamics, including steering, braking, and acceleration. These systems often require real-time processing capabilities that C++ supports.
-
Simulation and Modeling: Design engineers use C++ for simulation applications to model vehicle dynamics, aerodynamics, and other factors critical to automotive design. High-fidelity simulations enable designers to test and iterate on their designs before physical prototypes are built, saving time and resources.

C++ Programming Concepts for Automotive Development
Key C++ Features for Automotive Applications
Memory Management
Efficient memory management is essential when developing software for automotive applications, particularly in embedded environments where resources are limited. C++ provides developers with control over memory allocation and deallocation, allowing for careful handling of system resources.
For example, instead of using dynamic memory allocation, which can lead to fragmentation issues, developers can often use fixed-size arrays or statically-allocated objects. Here’s a code snippet showing how to manage memory allocation:
class Sensor {
private:
int dataBuffer[100]; // Fixed-size array for storing sensor data
public:
void collectData(); // Method to collect data into the buffer
};
Concurrency and Multithreading
As automotive systems become more complex, the need for concurrency and multithreading increases. C++ offers robust libraries for managing threads and ensuring thread safety in critical applications.
Here's an example demonstrating basic thread handling in C++:
#include <thread>
#include <iostream>
void sensorTask() {
// Simulated sensor task
std::cout << "Sensor data being processed." << std::endl;
}
int main() {
std::thread sensorThread(sensorTask);
sensorThread.join(); // Wait for thread to complete
return 0;
}
Important Libraries and Frameworks
AUTOSAR
The AUTOSAR (AUTomotive Open System ARchitecture) standard is crucial within the automotive software domain. It defines a standard architecture for automotive software components, promoting interoperability and reusability. C++ plays a key role in implementing AUTOSAR components, where software modules can be developed following standard interfaces and protocols.
Boost Libraries
The Boost libraries are powerful tools that extend C++ functionality with a broad range of features, including data structures, algorithms, and utilities applicable in automotive development. Libraries such as Boost.Asio for network programming or Boost.Thread for multithreading can significantly simplify complex tasks within automotive applications.
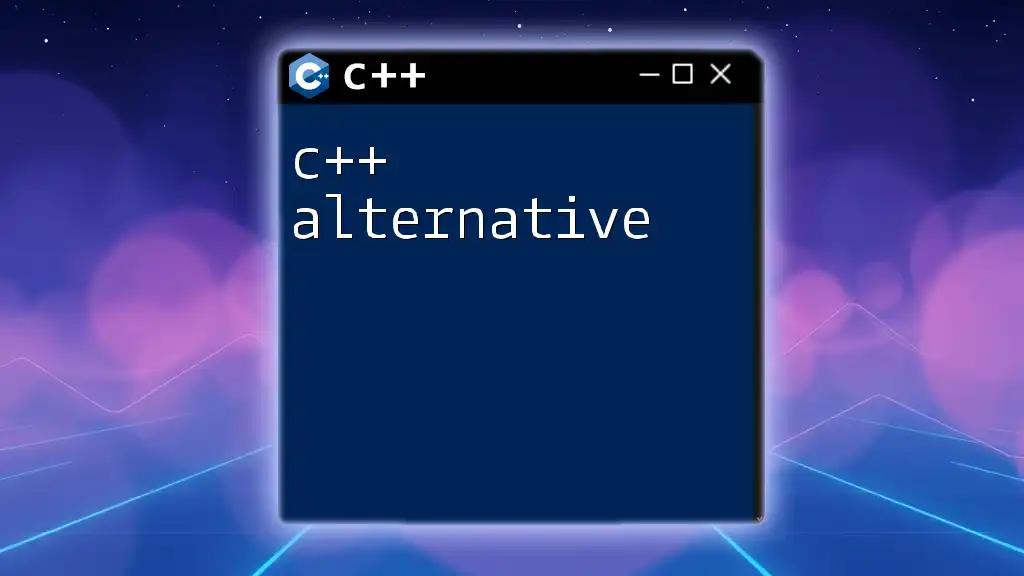
Practical Examples of C++ in Automotive
Example 1: CAN Bus Communication
The Controller Area Network (CAN) is a fundamental communication protocol used in automotive electronics. C++ can be utilized to send and receive messages over a CAN bus, essential for interoperability among various ECUs.
Here’s a simple code snippet showing how to send a message via CAN:
#include <canlib.h>
void sendMessage() {
CanLib can;
can.openChannel(0); // Open CAN channel 0
can.write(0x123, "Test Message", 12); // Send a message
}
Example 2: Vehicle Control System Simulation
C++ is often used to simulate vehicle control systems to understand their behavior under different conditions. This includes acceleration, steering, and braking mechanisms. Below is an example of encapsulating vehicle behaviors in a C++ class:
class Vehicle {
public:
void accelerate() {
// Code to increase speed
std::cout << "Vehicle is accelerating." << std::endl;
}
void brake() {
// Code to decrease speed
std::cout << "Vehicle is braking." << std::endl;
}
};

Best Practices for C++ Automotive Development
Safety and Reliability Considerations
Given the critical nature of automotive applications, adhering to safety standards is paramount. One widely recognized guideline is MISRA C++, which provides recommendations for coding practices to enhance code safety and reliability. Following these guidelines can minimize risks associated with software defects in safety-critical systems.
Regular testing and validation processes, such as static analysis and unit testing, are crucial for ensuring the robustness of C++ applications in automotive development.
Optimizing Performance
With performance being a critical requirement in automotive applications, developers must utilize profiling tools to identify performance bottlenecks. Common optimization strategies include:
- Avoiding unnecessary copying of objects: Utilize move semantics introduced in C++11.
- Using inline functions: Reduce function call overhead for small functions.
- Minimizing dynamic memory allocation: This can often lead to fragmentation in real-time systems.
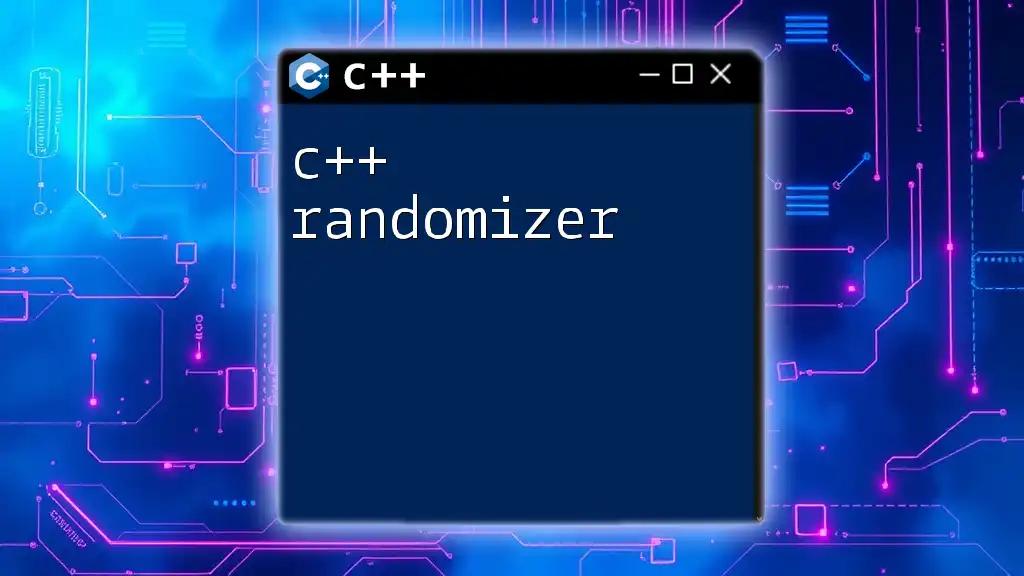
Future of C++ in the Automotive Industry
Emerging Technologies
The automotive landscape is evolving with the rise of electric vehicles (EVs) and autonomous vehicles. C++ will remain fundamental in developing software for these advancements due to its performance and reliability. The complexity of algorithms required for electric drivetrains and self-driving systems will continue to rely heavily on C++ capabilities.
Continuous Learning and Adaptation
As the automotive sector advances, the demand for skilled C++ developers will grow. Continuous learning through resources such as online courses, developer forums, and contribute to open-source projects will help developers stay abreast of new tools and technologies.
In conclusion, C++ is intrinsic to the automotive industry's future, combining performance, safety, and flexibility to tackle the challenges of modern vehicle systems. Embracing C++ in automotive applications can lead to innovation and safety enhancements that drive the industry forward.