In C++, `auto&` is used to automatically deduce the type of a variable while ensuring it represents a reference, allowing you to work with existing objects without copying them.
Here's an example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> nums = {1, 2, 3, 4, 5};
for (auto& n : nums) {
n *= 2; // Modify the original elements
}
for (const auto& n : nums) {
std::cout << n << " "; // Outputs: 2 4 6 8 10
}
return 0;
}
Understanding C++ auto&
What is `auto&` in C++?
`auto&` is a type specifier that helps you automatically deduce the type of a variable while allowing it to reference the original variable. In simpler terms, when you declare a variable using `auto&`, you are telling the compiler to infer its type from its initializer while also creating a reference to that object. This means that any changes made to the `auto&` variable affect the original variable it references.
Importance of `auto&` in Modern C++
The significance of `auto&` in modern C++ programming cannot be overstated. It enhances code clarity, reduces redundancy, and optimizes performance.
-
Code Clarity: Using `auto&` can make your code cleaner and more readable. Instead of specifying types explicitly, which can be verbose, `auto&` simplifies declarations.
-
Performance Optimization: In scenarios involving large objects, `auto&` becomes indispensable. By using references, you avoid the overhead associated with copying objects, thus improving performance.
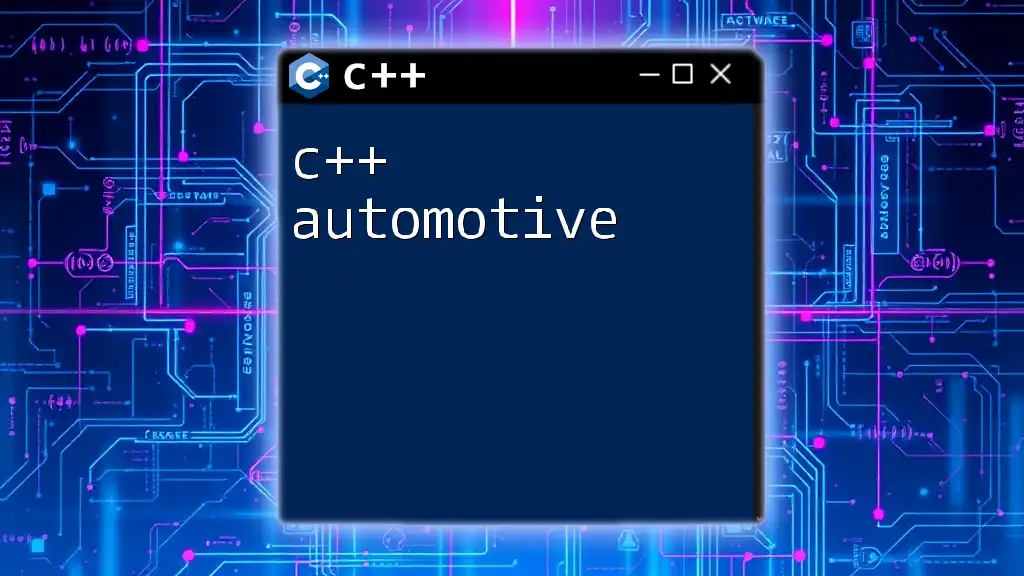
The Mechanics of `auto&`
How Does `auto&` Work?
The operation of `auto&` relies on type deduction. The C++ compiler determines the type of the variable based on the initializer's type. Below is a simple example to demonstrate this functionality:
int x = 10;
auto& ref = x; // ref is of type int&
In this case, `ref` becomes a reference to `x`. Any modifications to `ref` will be reflected in `x`.
When to Use `auto&`
Using `auto&` is best suited for specific scenarios:
-
When you want to modify existing objects: Using `auto&` allows you to make changes directly to the variable referenced, rather than working with a copy.
-
When dealing with large data: When working with large objects, referencing them via `auto&` can save both time and memory.
Common Pitfalls with `auto&`
While `auto&` is powerful, it has its pitfalls. One major risk is dangling references, which occur when you bind a reference to a temporary object or a local variable that goes out of scope. Here’s a demonstrative example:
int& getRef() {
int x = 5;
return x; // Dangerous: returns reference to local variable
}
auto& dangerousRef = getRef(); // Undefined behavior!
In this snippet, the reference `dangerousRef` points to `x`, which is a local variable. Once the function returns, `x` is destroyed, leaving `dangerousRef` as a dangling reference.
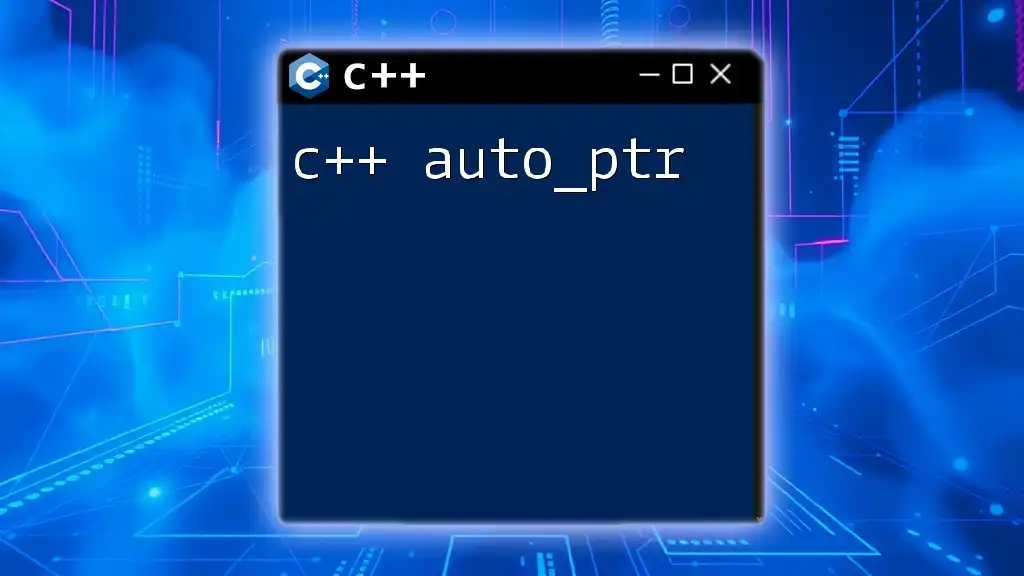
Use Cases of `auto&`
Iterating Over Containers with `auto&`
One of the most common use cases for `auto&` is iterating over STL containers. This allows you to modify the elements of the container directly, thereby improving both performance and clarity.
std::vector<int> nums = {1, 2, 3, 4, 5};
for (auto& num : nums) {
num *= 2; // Modifying elements directly
}
In this example, each element in the vector `nums` is doubled without creating copies of each element, thanks to `auto&`.
Working with Functions
When passing parameters to functions, using `auto&` can be highly beneficial. By passing variables by reference, you avoid unnecessary copying of data. Below is a function that illustrates this concept:
void increase(int& value) {
value++;
}
void processValues(std::vector<int>& vec) {
for (auto& v : vec) {
increase(v); // Directly passing by reference
}
}
In this example, `increase` modifies the passed `value` by reference. Similarly, in `processValues`, each vector element is passed by reference to the `increase` function, thus avoiding copies and improving performance.
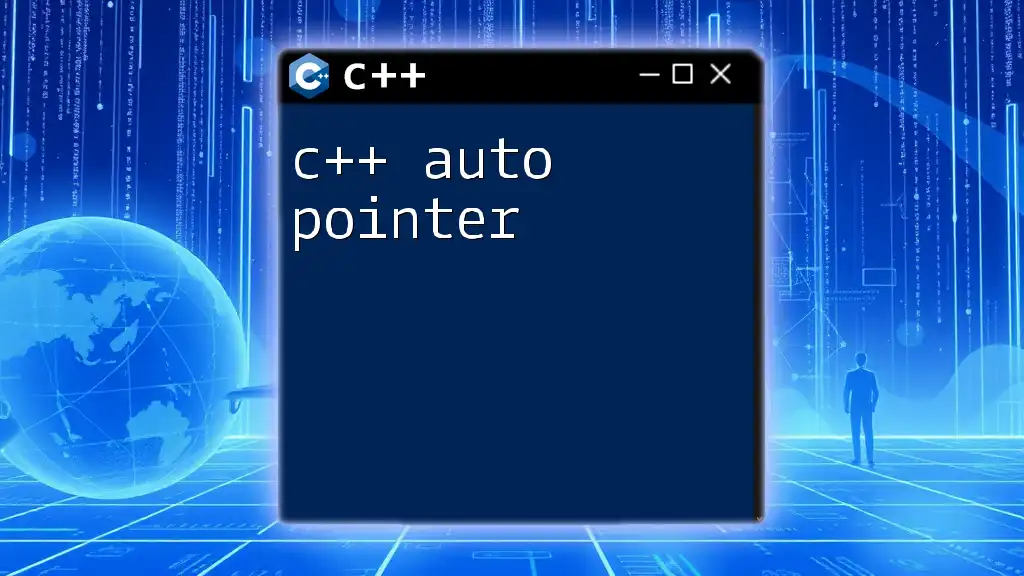
Advanced Concepts
`auto&` with Templates
The versatility of `auto&` extends into template programming. When working with templates, `auto&` accomplishes type deduction seamlessly.
template<typename T>
void printRef(T& item) {
std::cout << item << std::endl;
}
Here, any type passed to `printRef` will maintain its original reference type, allowing the function to work with both primitive types and user-defined types.
Handling Const and Non-Const References
When using `auto&`, it is vital to understand how it interacts with `const`. In scenarios where a reference is not meant to modify the original object, use `const auto&`. This provides safety while still enjoying the benefits of reference semantics.
const std::string message = "Hello, World!";
const auto& refMessage = message; // Safe and prevents modification
In this case, `refMessage` is a constant reference, which means it cannot change `message`, ensuring its integrity while allowing read access.
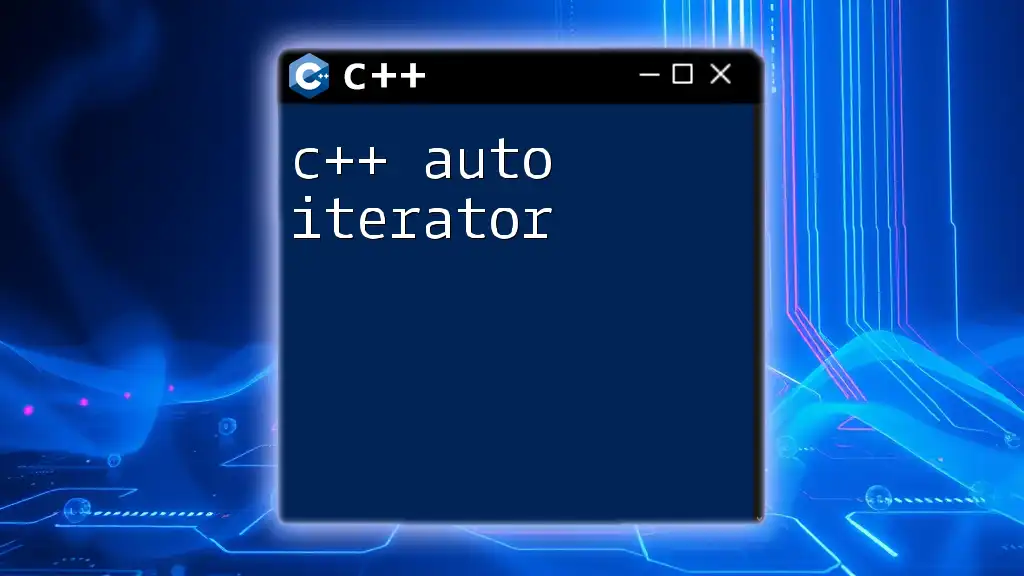
Conclusion
Recap of Key Takeaways
In summary, `auto&` serves as a vital tool in modern C++ programming. It allows for flexibility in variable declarations while promoting efficiency through reference semantics. By using `auto&`, programmers can write clearer, more efficient code without sacrificing performance.
Encouragement to Practice
I encourage you to practice using `auto&` in your own projects. Familiarizing yourself with this powerful feature will not only elevate your coding skills but also improve your coding habits. Automate type deduction and leverage references for better, cleaner, and faster C++ code.
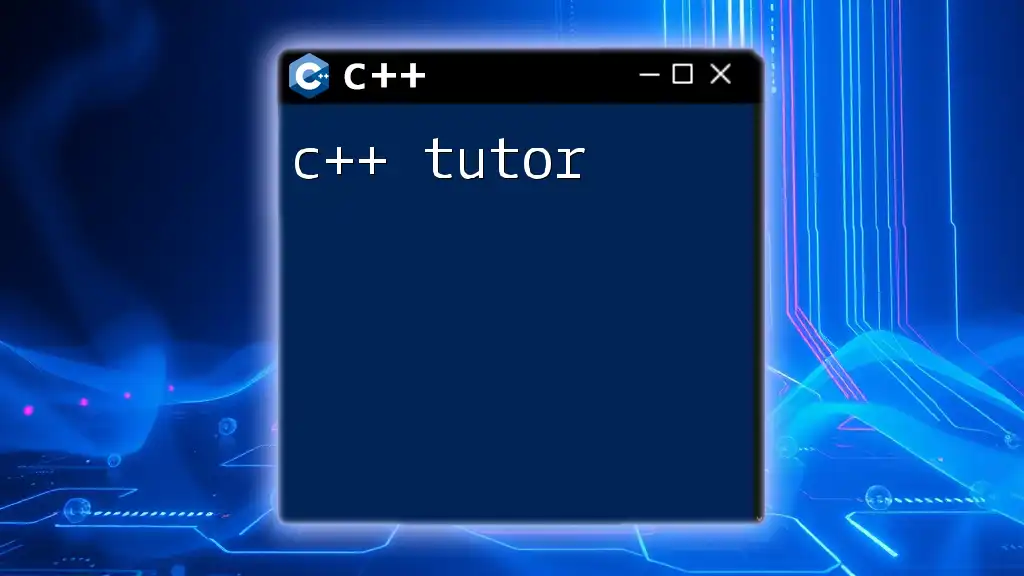
Additional Resources
Recommended Reading
To further your understanding of C++, I recommend exploring books and articles focused on modern C++ features. Resources like Effective C++ by Scott Meyers and online platforms like cppreference.com can be invaluable.
Community and Support
Joining C++ communities can provide great support as you learn. Websites such as Stack Overflow, Reddit’s r/Cplusplus, or C++ forums allow you to ask questions, share your experiences, and connect with other C++ enthusiasts. Engage with the community to deepen your knowledge and troubleshoot issues as they arise.