A C++ tutor provides targeted instruction on using C++ commands effectively, enabling learners to write efficient code quickly.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Simple output command
return 0;
}
What is C++?
C++ is a powerful, versatile programming language that has stood the test of time. Originally designed by Bjarne Stroustrup at Bell Labs in the early 1980s, C++ emerged as an extension of the C programming language and has since evolved into a robust language that supports both procedural and object-oriented programming paradigms.
The Evolution of C++
Over the decades, C++ has undergone several enhancements through standardized versions, continually improving upon its performance and features. The introduction of key features like templates, namespaces, and exception handling has allowed C++ to remain relevant in modern software development.
Why Choose C++?
Choosing C++ opens the door to a wide array of applications across various industries, from game development to software engineering and systems programming. Its efficiency in handling low-level operations and capability for object-oriented design makes it a favorite among developers. Additionally, the vibrant community and extensive libraries available facilitate continuous learning and resource gathering.
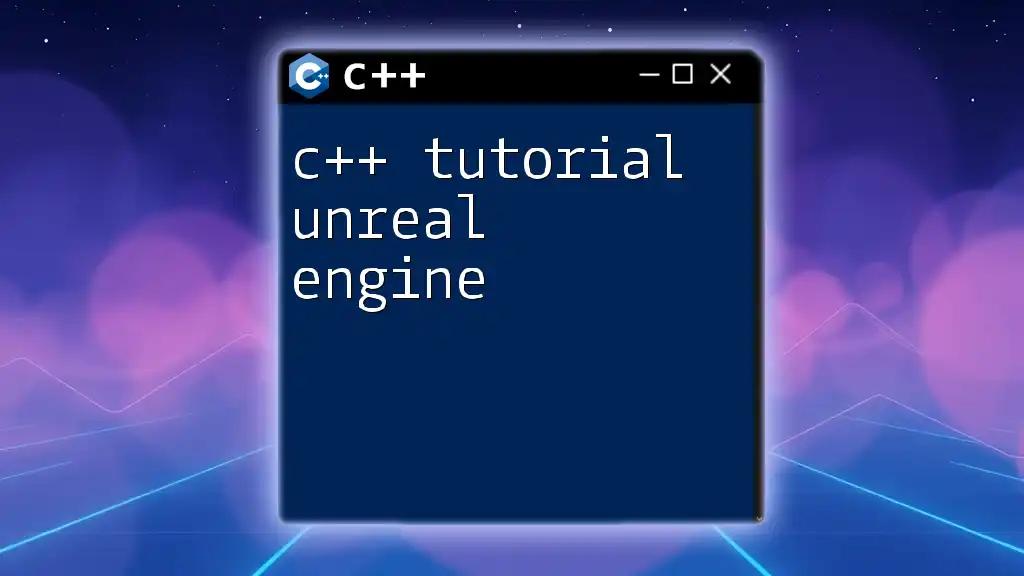
Getting Started with C++
Embarking on your C++ journey is an exciting step, and setting up your development environment is critical to ensure a smooth learning process.
Setting Up Your Development Environment
To get started, you'll need to choose a development environment and install a C++ compiler. Popular Integrated Development Environments (IDEs) like Visual Studio, Code::Blocks, and CLion provide excellent platforms for writing and debugging code.
You’ll also need a compiler, such as `g++` or `clang++`, which will convert your C++ code into executable programs. Once your environment is configured, you will be ready to dive deep into coding.
Writing Your First C++ Program
Creating your first C++ program is an exhilarating experience! The classic "Hello, World!" example serves as a perfect introduction:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code snippet, `#include <iostream>` is a preprocessor directive that includes the Input/Output stream library, allowing you to use `std::cout` to print the output to the console.
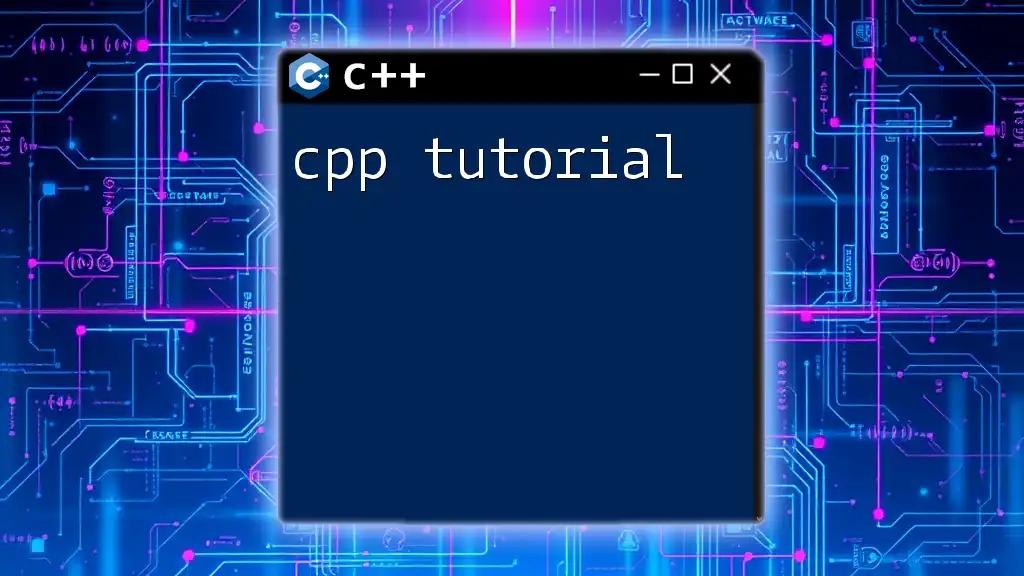
Core C++ Concepts
Understanding the fundamental concepts of C++ is essential for any aspiring programmer.
Variables and Data Types
Variables in C++ are used to store data. Before using a variable, you must declare it with a specified data type, such as:
int age = 25;
float salary = 50000.50;
Common data types include:
- int for integers
- float for floating-point numbers
- char for characters
- bool for boolean values, representing true or false
Control Structures
Control structures determine the flow of your program’s execution.
Conditional Statements
Conditional statements, such as `if`, `else if`, and `else`, allow your program to make decisions based on conditions.
For instance:
if (age > 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Minor" << std::endl;
}
In the example above, the condition checks if `age` is greater than 18, allowing the program to respond appropriately.
Loops
Loops enable repetitive execution of code. Common loop types include `for` and `while`.
Here’s an example of a `for` loop that prints numbers from 0 to 4:
for (int i = 0; i < 5; i++) {
std::cout << i << std::endl;
}
Functions
Functions organize your code into reusable blocks. You define a function once and can call it multiple times, which enhances both readability and maintainability.
Here’s a simple function that adds two integers:
int add(int a, int b) {
return a + b;
}
C++ also supports function overloading, allowing functions to have the same name with different parameter types.
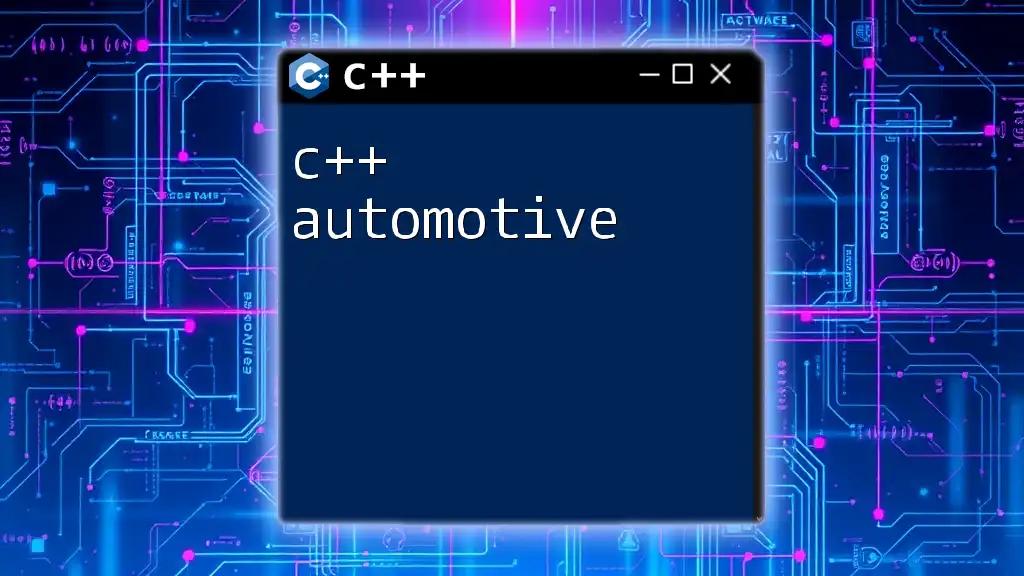
Working with Object-Oriented Programming
One of C++’s powerful features is its support for object-oriented programming (OOP).
Understanding Classes and Objects
A class is a blueprint for creating objects, which are instances of classes. Here’s an example:
class Car {
public:
void drive() {
std::cout << "Driving..." << std::endl;
}
};
In this case, `Car` is a class, and its method `drive` can be used by any object created from that class.
Inheritance and Polymorphism
Inheritance allows one class (derived class) to inherit properties and methods from another (base class), facilitating code reuse.
Consider the following example:
class Vehicle {
public:
void honk() {
std::cout << "Honking!" << std::endl;
}
};
class Truck : public Vehicle {
public:
void haul() {
std::cout << "Hauling cargo!" << std::endl;
}
};
Here, `Truck` inherits from `Vehicle`, accessing its `honk` method while also having its own method `haul`.
Polymorphism enables the same function to behave differently based on the object invoking it. This concept is vital for designing flexible and extensible systems.

Advanced C++ Concepts
As you become more comfortable with C++, venturing into advanced concepts will greatly enhance your programming capabilities.
Pointers and Dynamic Memory Management
Pointers store the memory address of a variable. Understanding pointers is crucial for dynamic memory management, allowing you to allocate and deallocate memory during runtime.
Here’s a simple example of using a pointer:
int* ptr = new int; // Allocate memory
*ptr = 10; // Assign value
delete ptr; // Free memory
In this snippet, we allocate memory for an integer and later free it. Proper memory management helps prevent memory leaks, which can deteriorate application performance.
Templates and the Standard Template Library (STL)
C++'s templates allow you to create generic functions and classes. This feature enhances code reuse and type safety.
The Standard Template Library (STL) provides built-in data structures, like vectors and maps, which simplify complex operations. Here's an example of using a vector:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
STL effectively enhances the programmer's efficiency by providing pre-built implementations for commonly used algorithms and data structures.
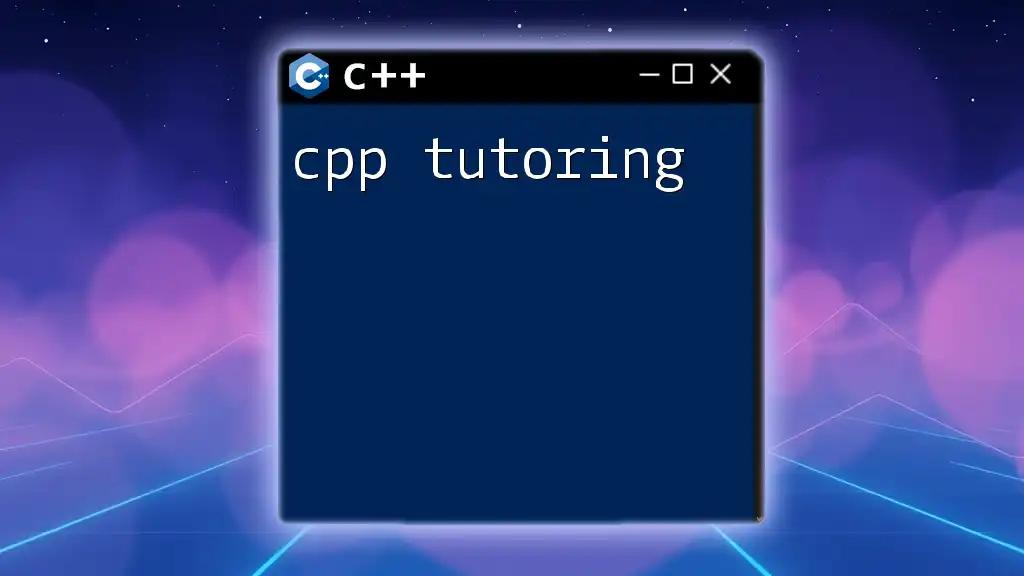
Best Practices for C++ Programming
Writing clean and maintainable code is crucial for long-term project success.
Writing Clean and Maintainable Code
Code readability is key. Make sure your code is easily understandable by using clear variable names and consistent formatting. Regularly documenting your code with comments helps others (and your future self) comprehend your logic.
Common Mistakes to Avoid
While coding in C++, beginners often fall into pitfalls including:
- Memory leaks: Failing to release dynamically allocated memory.
- Misusing pointers: Not understanding pointer arithmetics or dereferencing NULL pointers.
- Scope and lifetime issues: Overlooking how variable scope can affect program functionality.
By being aware of these common challenges, you can write more robust code.

How to Find a C++ Tutor
Finding the right C++ tutor can significantly accelerate your learning process.
Identifying Your Learning Goals
Understand what specific topics you wish to cover and your preferred style of learning—whether it's hands-on exercises, theoretical discussions, or a mix of both.
Where to Look for C++ Tutors
Several avenues offer opportunities to discover C++ tutors:
- Online platforms: Websites like Udemy, Coursera, and Codecademy provide various C++ courses tailored to different skill levels.
- Local programming schools: Search for coding boot camps in your area that offer C++ programming classes.
- Forums and community boards: Platforms like Stack Overflow and Reddit can also connect you with tutors or mentors.

Conclusion
In conclusion, becoming proficient in C++ is a rewarding journey that enriches your programming skills. The role of a C++ tutor can provide personalized guidance, helping you navigate the complexities of the language. With the right resources, lessons, and dedication, you're well on your way to mastering C++. Embrace the challenge, and the rewards will follow as you expand your programming repertoire.
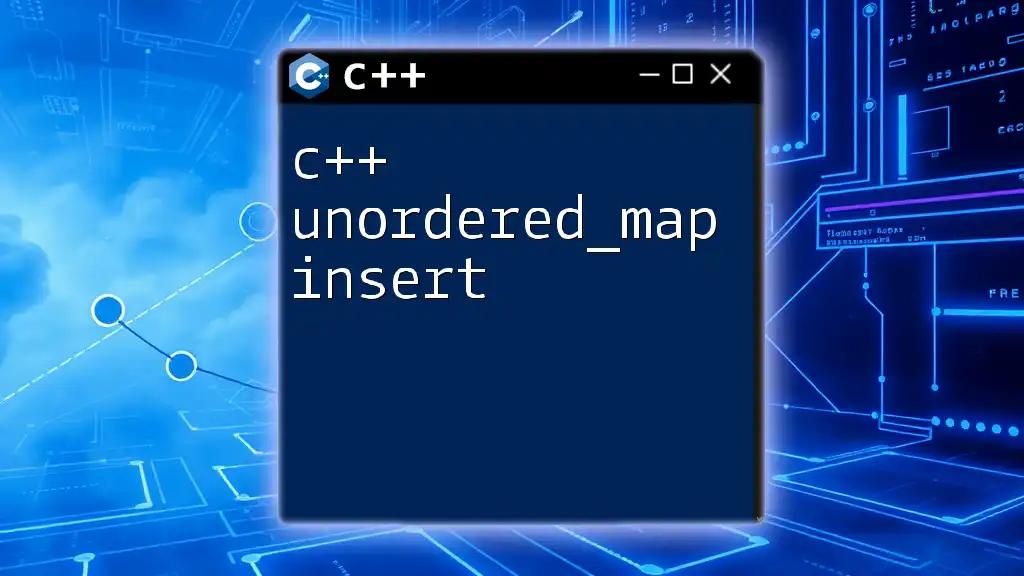
FAQs
What is the Average Duration for Learning C++ with a Tutor?
The duration varies based on your current knowledge and learning pace, but typically it may range from a few weeks to several months for substantial mastery.
Can I Learn C++ Online?
Absolutely! Numerous online platforms offer C++ courses, providing flexibility to learn at your own pace.
How Much Does C++ Tutoring Typically Cost?
Costs can vary widely depending on the tutor’s expertise, lesson frequency, and location. Expect to find tutoring services ranging from $20 to $100 per hour.