In C++, the `or` keyword serves as an alternative logical operator for the logical OR operation, equivalent to the `||` operator.
Here’s an example in C++:
#include <iostream>
int main() {
bool a = true;
bool b = false;
if (a or b) {
std::cout << "At least one of the conditions is true" << std::endl;
}
return 0;
}
What is the `or` Operator?
The `or` operator in C++ is a logical operator that evaluates two boolean expressions and returns `true` if at least one of the expressions is `true`. Understanding how to use this operator is crucial because it allows developers to create more complex logical conditions in their code.
In C++, the `or` operator is synonymous with the `||` operator. However, using `or` can enhance the readability of your code, especially for those who are familiar with alternative programming languages.
Syntax of the `or` Operator
The basic syntax for the `or` operator is straightforward. You can use it between any two boolean expressions, as shown below:
expression1 or expression2;
How to Use the `or` Operator
Basic Usage: To get started, let’s explore some simple examples that illustrate the functionality of the `or` operator.
#include <iostream>
using namespace std;
int main() {
bool a = true;
bool b = false;
if (a or b) {
cout << "At least one of them is true!" << endl;
}
return 0;
}
In this example, the condition `a or b` evaluates to `true` because `a` is `true`, which means the output will be "At least one of them is true!".
Combining Conditions: You can also use the `or` operator to combine multiple conditions easily.
#include <iostream>
using namespace std;
int main() {
int x = 10;
int y = 20;
if (x > 15 or y < 25) {
cout << "Condition is true!" << endl;
} else {
cout << "Condition is false!" << endl;
}
return 0;
}
In this case, the `if` condition evaluates to `true` since `y < 25`. Therefore, it prints "Condition is true!".
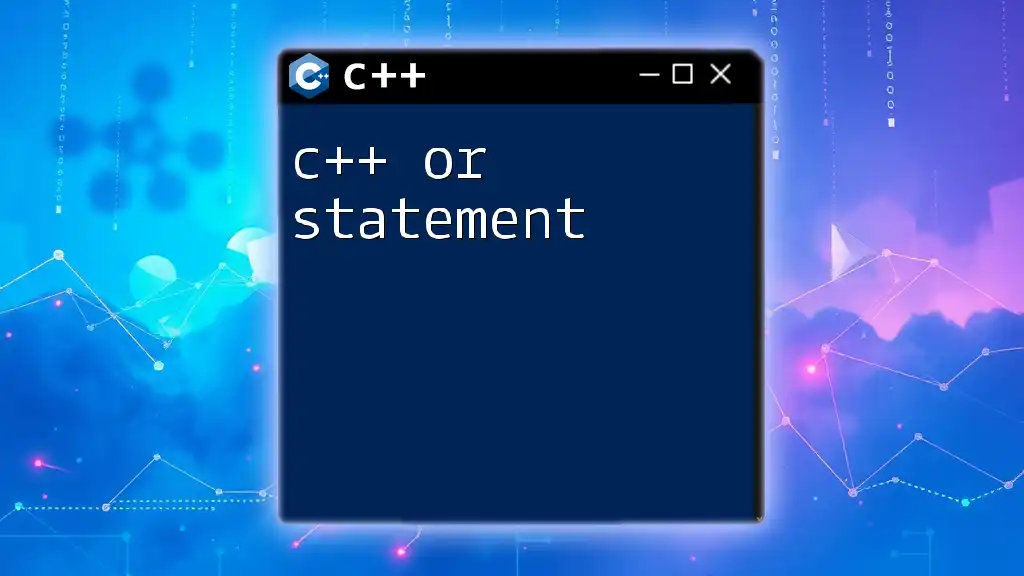
Logical Operations and Short-Circuiting
One of the powerful features of logical operators in C++, including `or`, is short-circuit behavior. This means that if the first operand evaluates to `true`, the second operand is not evaluated at all.
How Short-Circuiting Works
Consider this example:
#include <iostream>
using namespace std;
bool condition1() {
cout << "Checking condition 1" << endl;
return false;
}
bool condition2() {
cout << "Checking condition 2" << endl;
return true;
}
int main() {
if (condition1() or condition2()) {
cout << "At least one condition is true!" << endl;
}
return 0;
}
Here, `condition1()` outputs "Checking condition 1" and returns `false`. However, since `condition2()` is needed to evaluate the entire `if` condition, it outputs "Checking condition 2" and returns `true`. The final output is "At least one condition is true!".
This characteristic of short-circuiting can be particularly useful in scenarios where you want to avoid unnecessary computation or potential errors that might arise from evaluating all conditions.
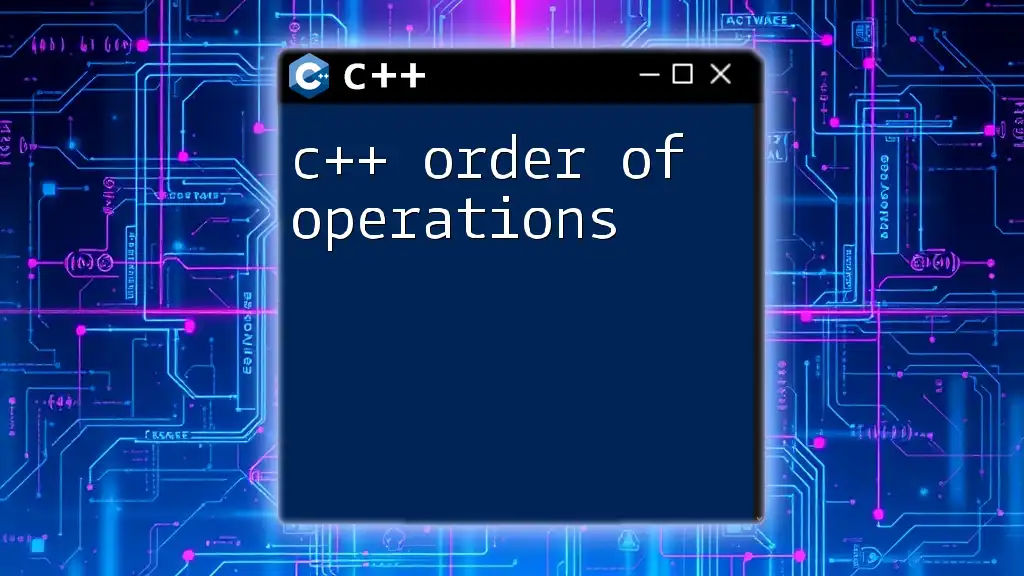
Practical Applications of the `or` Operator
Real-World Scenarios
The `or` operator frequently finds its applications in situations requiring the evaluation of multiple conditions. One common scenario is in user input validation, where multiple criteria need to be met.
Using the `or` Operator in User Authentication
Here’s a simplified example demonstrating how the `or` operator can be utilized in a user-authentication scenario:
#include <iostream>
#include <string>
using namespace std;
int main() {
string username;
string password;
cout << "Enter your username: ";
cin >> username;
cout << "Enter your password: ";
cin >> password;
if (username == "admin" or password == "12345") {
cout << "Access granted!" << endl;
} else {
cout << "Access denied!" << endl;
}
return 0;
}
In this example, the system grants access if the user enters either the correct username `"admin"` or the correct password `"12345"`. This logic allows for flexibility and ensures that users have multiple paths to access the application.
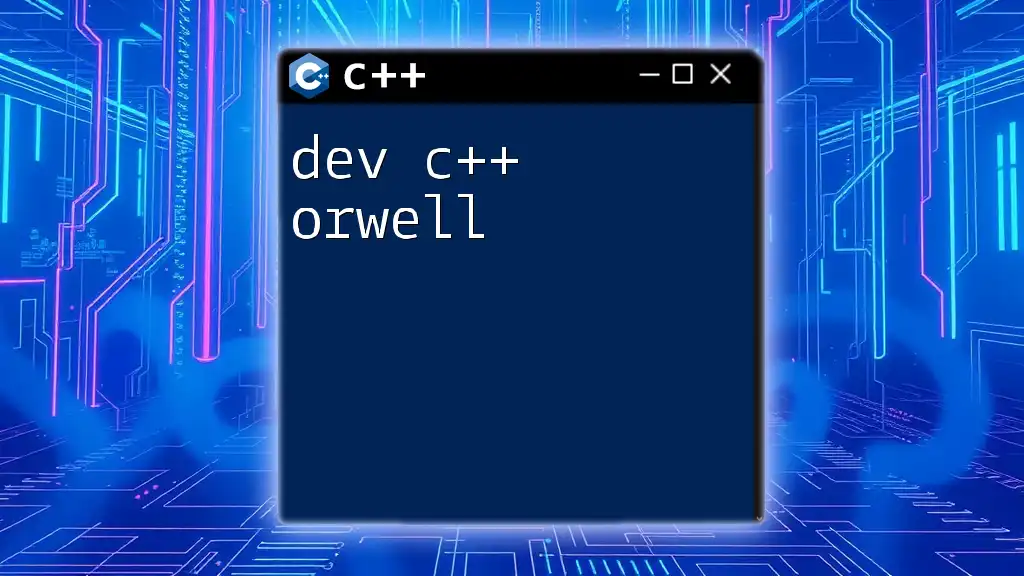
Common Mistakes with the `or` Operator
When using the `or` operator, it's easy to run into logical errors if not careful.
Overlooking Logical Errors
One common pitfall is forgetting that the `or` operator only requires one of the conditions to be `true` to succeed. This simplicity can lead to flawed logic where one might mistakenly believe both conditions need to be fulfilled.
Tips to Avoid Logical Pitfalls
- Always thoroughly understand the logic you are implementing.
- Test each condition separately if needed to confirm they behave as expected.
- Use tooltips or comments to indicate the purpose of more complex logical statements.
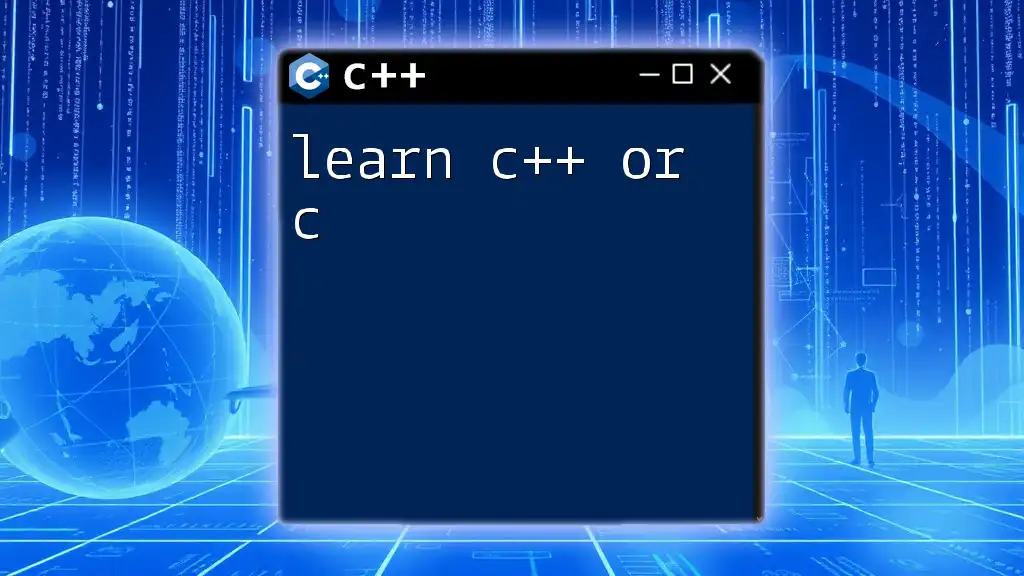
Conclusion
The `or` operator in C++ is a fundamental tool for programming effective logical conditions. It enhances code flexibility and readability while simplifying the evaluation of multiple boolean expressions. By mastering the use of the `or` operator, developers can create robust applications that accommodate various logical flow requirements. Understanding its usage, syntax, and practical applications paves the way for writing clearer and more efficient code.
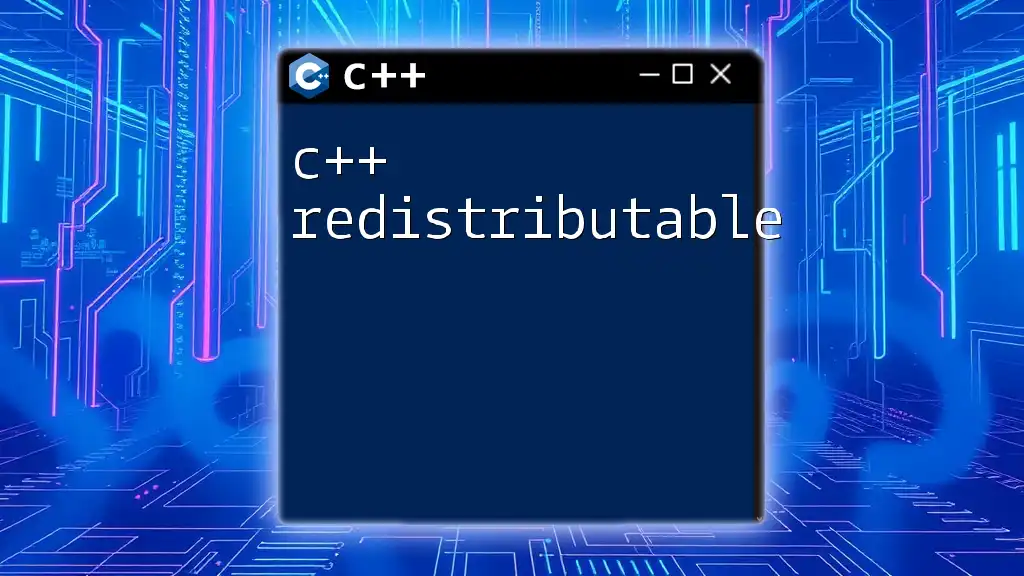
Additional Resources
For those eager to delve deeper into logical operators and C++ programming concepts, numerous resources are available to expand your knowledge. Consider exploring advanced topics about the `||` operator and bitwise operations to enrich your programming toolkit.