The `offsetof` macro in C++ is used to determine the byte offset of a member variable within a specified structure or class, allowing for low-level memory manipulation and access.
#include <iostream>
#include <cstddef> // for offsetof
struct MyStruct {
int a;
double b;
};
int main() {
std::cout << "Offset of 'b' in MyStruct: " << offsetof(MyStruct, b) << " bytes" << std::endl;
return 0;
}
Understanding Memory Layout in C++
Memory organization is crucial in C++ programming, as it directly affects performance, efficiency, and the behavior of your applications. Understanding how memory is allocated and organized can help in crafting optimized and bug-free code.
In C++, memory is divided into two main areas: the stack and the heap. The stack is used for static memory allocation where variables are created and destroyed in a last-in, first-out order. On the other hand, the heap is used for dynamic memory allocation, allowing for the allocation of memory at runtime.
Alignment and Padding Concepts
When dealing with structures, compilers often add padding to ensure that members are aligned to specific byte boundaries for efficient access. This means that the actual size in memory of structures may exceed the sum of their individual member sizes due to this padding. Understanding alignment and padding is essential when using `offsetof` to avoid confusion about the actual memory layout.
How Structures Utilize Memory
Structures combine different data types into a single type, leading to contiguous memory allocation. For example, consider the following structure:
struct Example {
int intValue;
char charValue;
double doubleValue;
};
In this example, the compiler determines the memory layout, considering the sizes of `int`, `char`, and `double`, along with any necessary padding. Visualizing the layout could be beneficial; for example, the `intValue` may start at an offset of 0 bytes, with the `charValue` placed after it, potentially needing additional padding to align `doubleValue` properly.
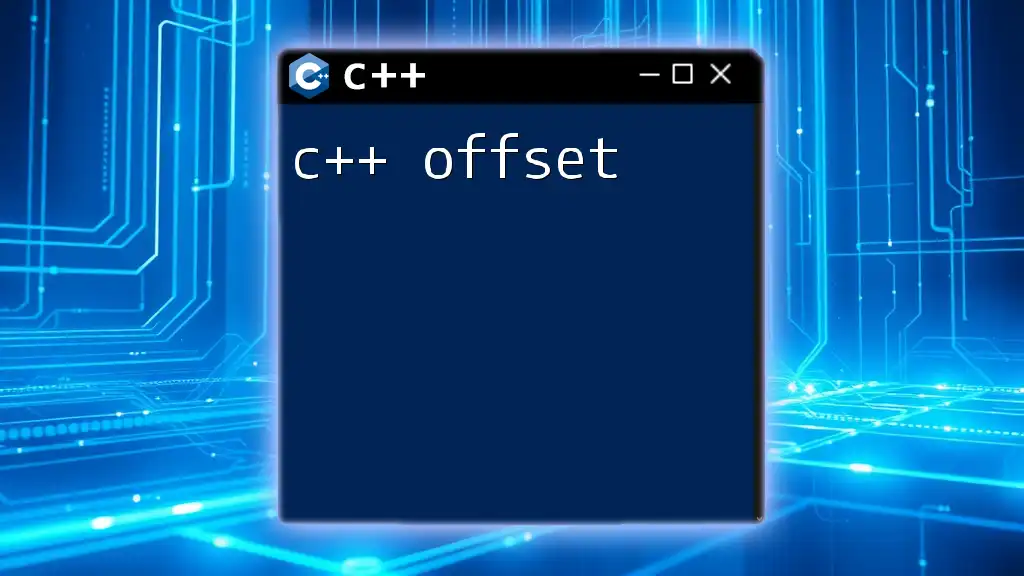
What is `offsetof`?
The `offsetof` macro is a functionality provided in C++ that allows programmers to determine the offset of a specific member within a structure or class. It illustrates the byte offset from the beginning of the struct to the target member.
To utilize `offsetof`, you need to include the `<cstddef>` header file. The syntax for `offsetof` is straightforward:
offsetof(type, member)
Code Example of `offsetof`
Here’s a practical example to illustrate how to use the `offsetof` macro:
#include <cstddef>
#include <iostream>
struct Example {
int intValue;
char charValue;
double doubleValue;
};
int main() {
std::cout << "Offset of intValue: " << offsetof(Example, intValue) << " bytes\n";
std::cout << "Offset of charValue: " << offsetof(Example, charValue) << " bytes\n";
std::cout << "Offset of doubleValue: " << offsetof(Example, doubleValue) << " bytes\n";
return 0;
}
Running this code will output the byte offsets of the structure members, allowing you to understand how they are laid out in memory. The results will reveal critical insights into the structure’s memory organization.
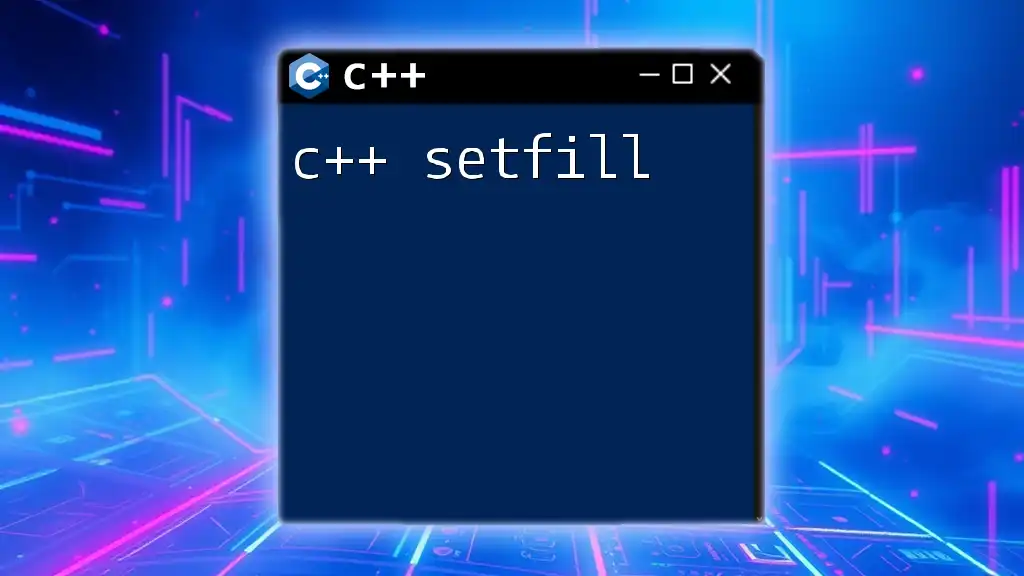
How to Use `offsetof`
Using `offsetof` is simple, yet powerful. Here’s a step-by-step guide on implementing it effectively:
-
Include the Necessary Header: Always ensure to include `<cstddef>` at the beginning of your program.
-
Implement the Macro: Use `offsetof` in your code to find the offsets of various members within your structs/classes. This can assist in debugging and optimizing memory usage.
-
Practical Scenarios: Here are some scenarios where `offsetof` shines:
- Serializing Data: When preparing data for networks or file I/O, knowing the exact offsets can help in structuring packets correctly.
- Interfacing with Hardware: Hardware often requires specific layouts; using `offsetof` assists in configuring structures that communicate with hardware efficiently.
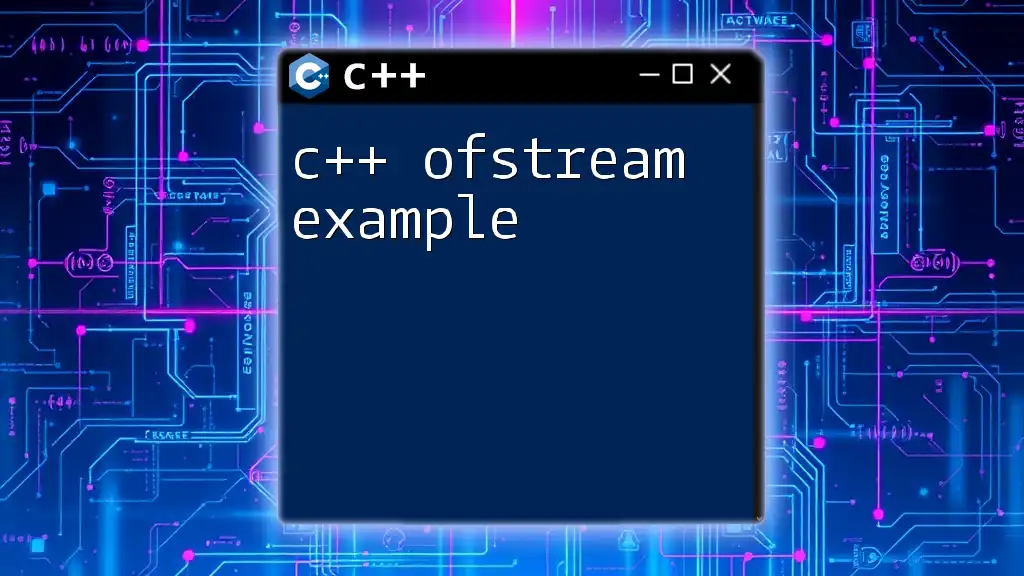
Common Pitfalls and Best Practices
While using `offsetof` can be beneficial, some common pitfalls should be avoided:
-
Misusing `offsetof` with Non-standard-layout Types: Misapplying `offsetof` on structures with virtual functions or derived classes can lead to undefined behavior. Only use it on standard-layout types.
-
Proper Struct Definitions: Ensure that your structs are defined clearly and adhere to standard layout rules. Misalignment caused by incorrect struct definitions can lead to misleading offset values.
Examples of Mistakes
Example 1: Non-standard Layout Structures
struct NonStandard {
char c;
virtual void func() {}
};
// Using offsetof here will lead to undefined behavior
std::cout << offsetof(NonStandard, c) << std::endl; // Undefined behavior
In this example, attempting to use `offsetof` on a structure with virtual functions will yield undefined behavior, underscoring the need for caution.
Example 2: Padding and Alignment Issues
struct Aligned {
char c;
int i; // Expecting offsets without understanding padding can lead to confusion
};
Understanding padding is crucial here as the actual offset of `i` may not be immediately intuitive. Without accounting for the padding added by the compiler, calculations based solely on member sizes may result in incorrect assumptions.
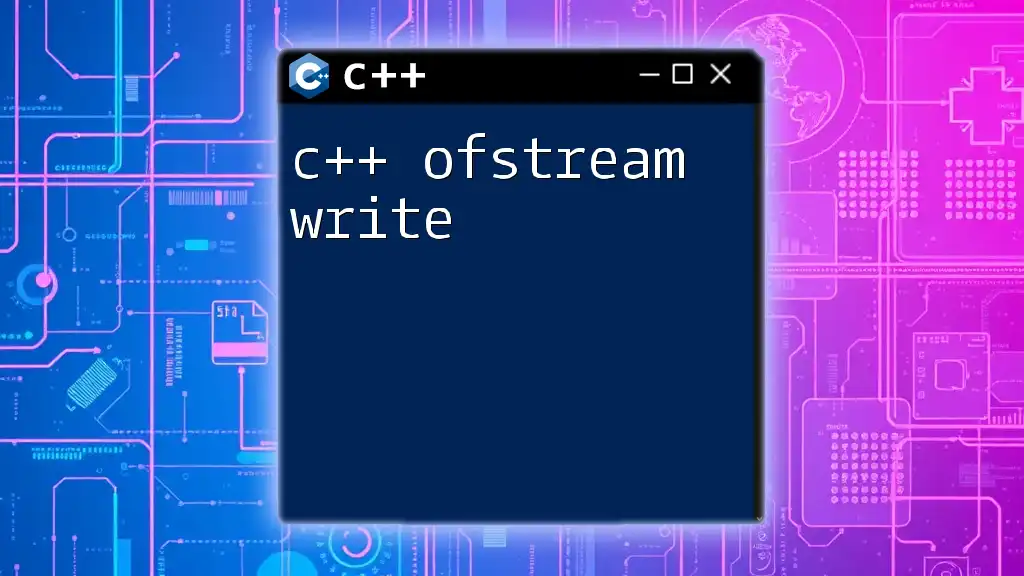
Alternatives to `offsetof`
Aside from `offsetof`, modern C++ provides alternatives like `std::addressof` that may serve specific use cases better. However, when working directly with offsets, `offsetof` remains a reliable choice when used with caution.
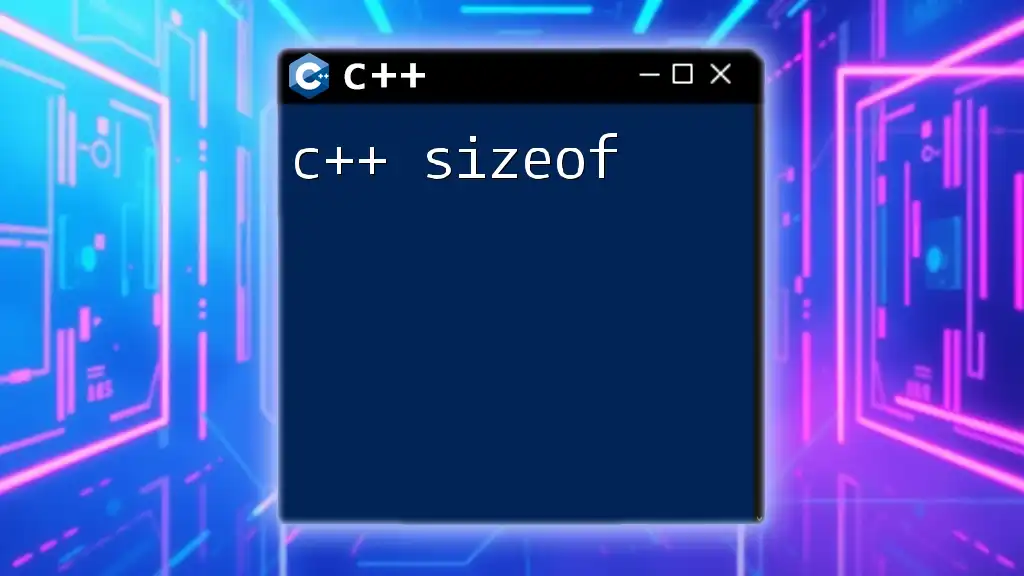
Conclusion
Understanding the C++ `offsetof` macro is vital for programmers aiming to have a more profound knowledge of memory layout and data structures. It empowers you to make informed decisions when crafting efficient and performance-oriented code. As you explore and experiment with the examples provided, take note of the behavior and results of your implementations.
Feel free to share your experiences or questions regarding `offsetof`, and continue enhancing your understanding of C++. The more you practice, the better equipped you'll be for complex programming challenges.
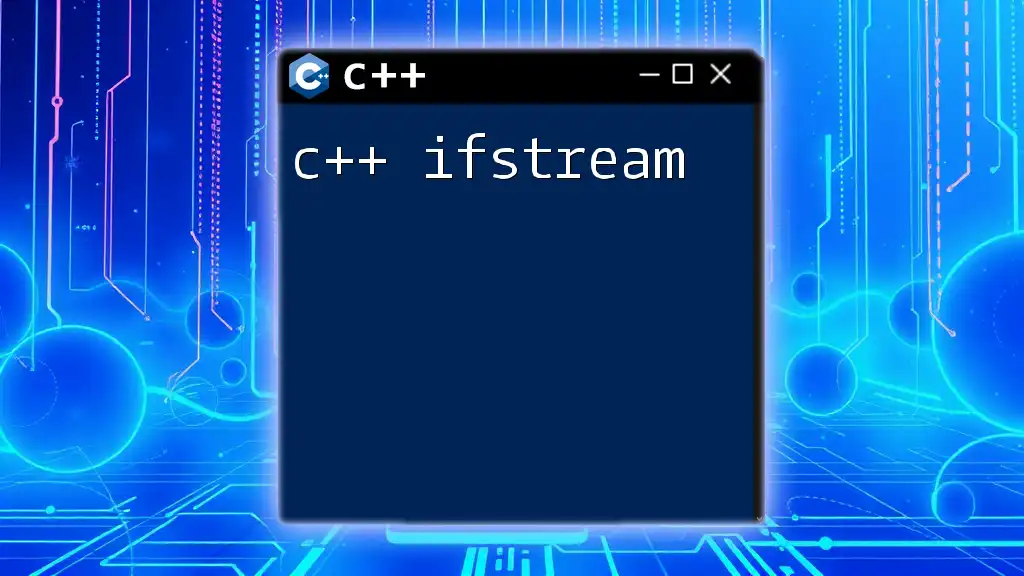
Additional Resources
To further your knowledge, consider exploring the following resources:
- C++ Standard Library documentation
- Online forums and communities dedicated to C++ (e.g., Stack Overflow, C++ subreddits)
- Recommended literature that dives deeper into memory management and optimization in C++ programming.
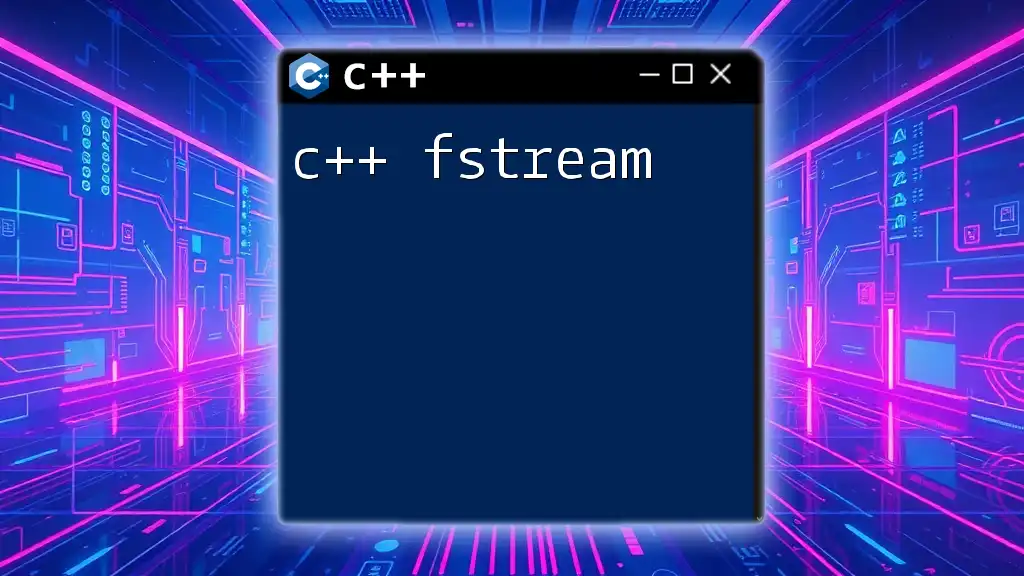
FAQs about `offsetof`
-
What happens if you use `offsetof` on a pointer? Using `offsetof` on pointers generally leads to logical errors, as it’s designed to work with types and their member variables.
-
Is there a specific C++ standard where `offsetof` was introduced? `offsetof` was introduced in the C standard and has been available in C++ since the early versions, maintaining consistent support across different compilers.
-
Can `offsetof` be used on classes that have private members? Yes, `offsetof` can be used on classes with private members as long as those members are part of a standard layout. However, accessing them outside class methods will require appropriate access specifiers.
Using the `offsetof` macro effectively enhances your C++ programming toolkit, paving the way for more efficient and structured code development.