In C++, the `ofstream` class is used to create files and write data to them, allowing you to store output from your program in a specified file.
Here's a simple example of using `ofstream` to write text to a file:
#include <fstream>
int main() {
std::ofstream outFile("example.txt");
if (outFile.is_open()) {
outFile << "Hello, World!" << std::endl;
outFile.close();
}
return 0;
}
Understanding ofstream
What is ofstream?
In C++, `ofstream` is a class specifically designed for output file stream operations. It allows you to create and manipulate files by writing data to them, performing essential tasks in scenarios such as logging, data storage, and configuration management. `ofstream` operates primarily in write mode, meaning it can overwrite existing files or create new ones if they do not already exist.
It’s crucial to understand that `ofstream` is just one part of the family of file handling classes, which includes `ifstream` for input file streams and `fstream` that supports both input and output on the same file.
Key Characteristics of ofstream
`ofstream` is characterized by several important features:
-
Writing Capabilities: The primary purpose of `ofstream` is to facilitate writing operations, allowing for the insertion of data directly into files.
-
Buffering and Performance Considerations: `ofstream` employs buffering to optimize write operations, which can enhance performance when working with large amounts of data. Understanding buffering can aid in efficient file handling.
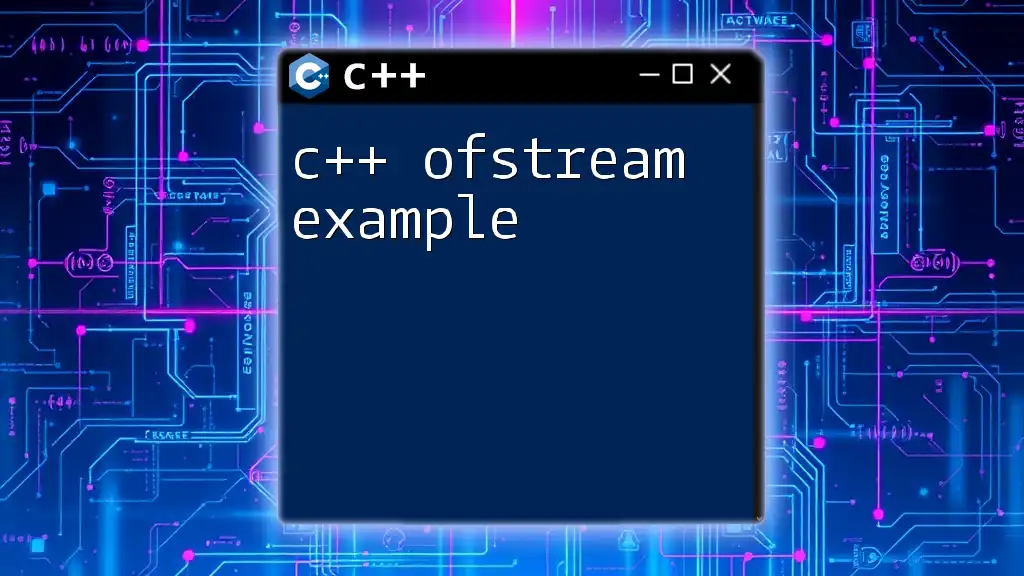
Getting Started with ofstream
Including the Required Header File
To use `ofstream`, you must include the appropriate header file at the beginning of your program:
#include <fstream>
This line allows your program access to the file stream functionalities necessary for file creation and manipulation.
Creating an ofstream Object
Declaring an `ofstream` object is a straightforward process. Here is a simple declaration:
std::ofstream outFile;
This line initializes an `ofstream` variable named `outFile`, which you can then use to open and write data to files.
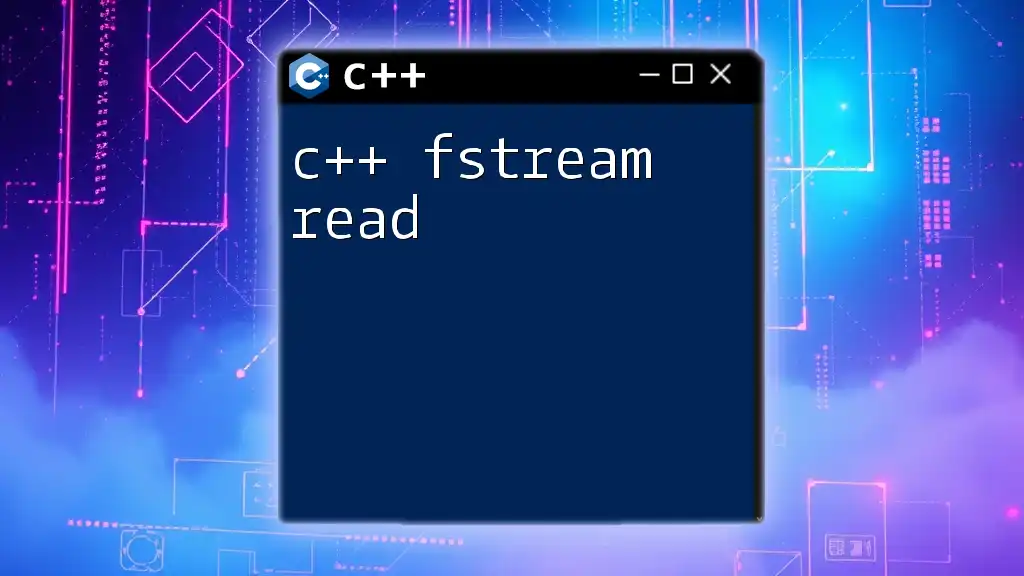
Opening a File using ofstream
Syntax to Open a File
To begin writing to a file, you need to open it using the `.open()` method provided by `ofstream`. The syntax is as follows:
outFile.open("filename.txt");
Here, `"filename.txt"` is the name of the file you want to create or modify.
Modes of Opening a File
When opening a file, you can specify different modes, which determine how the file will be accessed:
-
ios::out: This is the default mode for `ofstream`, allowing data to be written to the file.
-
ios::app: This mode opens the file in append mode, meaning that new data will be added to the end of the file, preserving existing content.
-
ios::trunc: If this mode is specified, any existing content in the file will be deleted when it is opened.
Here’s an example demonstrating the opening of a file in append mode:
outFile.open("filename.txt", std::ios::out | std::ios::app);
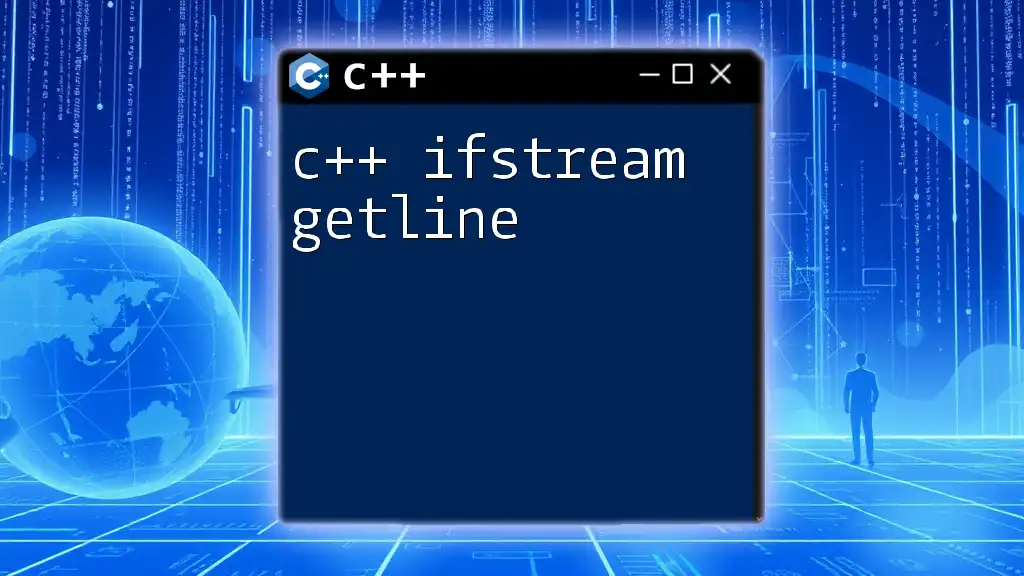
Writing to a File
Using the Insertion Operator
The `<<` operator is used with `ofstream` to write data into a file. For example:
outFile << "Hello, World!" << std::endl;
This statement writes "Hello, World!" followed by a newline to the file.
Writing Different Data Types
`ofstream` supports writing various data types, including strings, integers, and floating-point numbers. Below is an example of how to write multiple data types:
std::string name = "John Doe";
int age = 30;
outFile << "Name: " << name << ", Age: " << age << std::endl;
In this code snippet, both a string and an integer are formatted into a single line that is then written to the file.
Formatting Output
For more advanced formatting, C++ provides manipulators such as `std::setw` and `std::setprecision`. Here’s how you can use these manipulators for writing formatted numbers:
#include <iomanip>
outFile << std::fixed << std::setprecision(2) << 123.456 << std::endl;
This code will output the number with two decimal points.
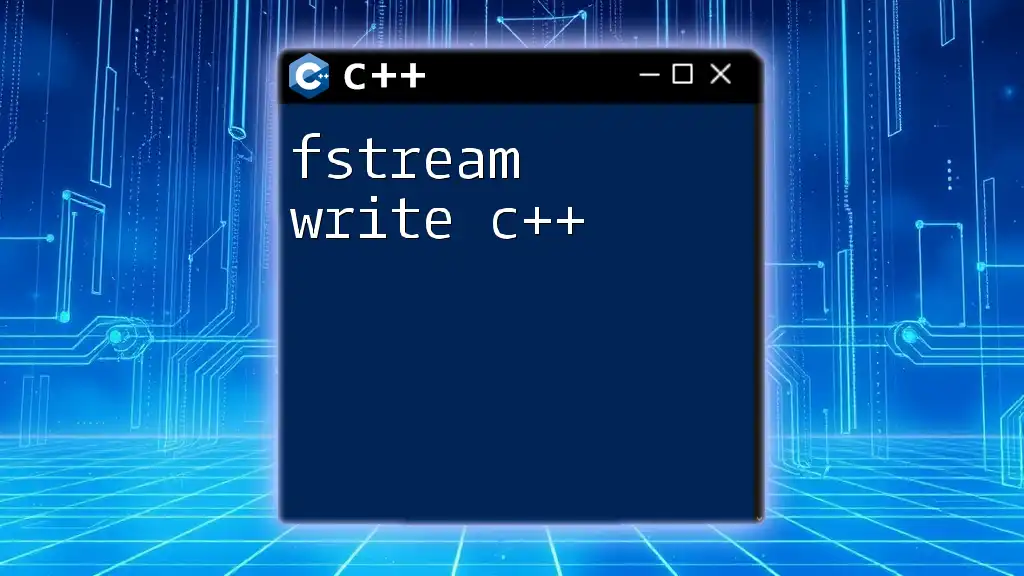
Closing a File
Importance of Closing Files
Once you’ve completed writing to a file, it’s essential to close it using the `.close()` method. This step ensures that all data is flushed from buffers and saved properly.
outFile.close();
Failing to close a file can lead to data loss or corruption, making it a crucial part of file management.
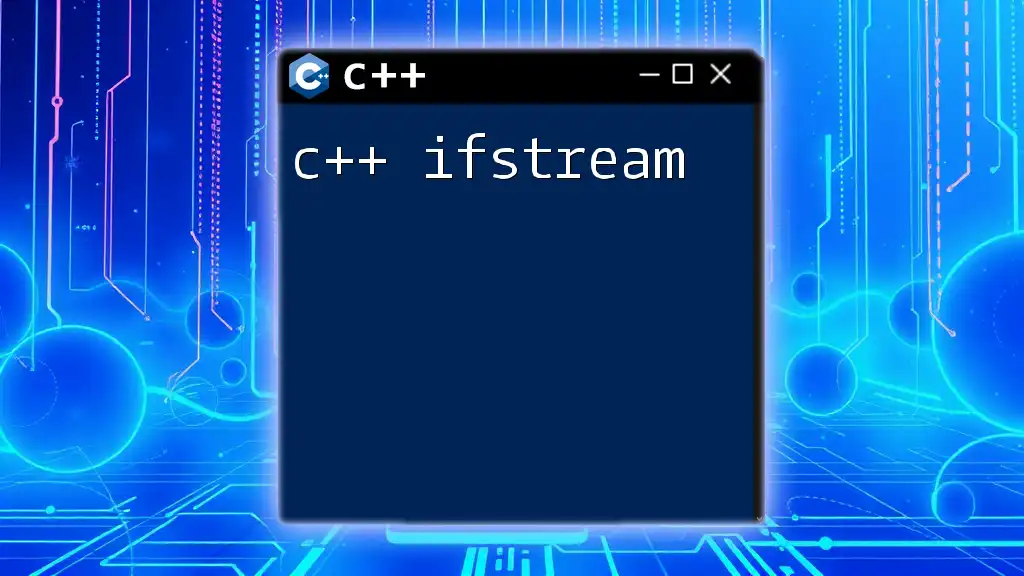
Error Handling with ofstream
Checking if the File is Open
Before performing any write operations, it is prudent to verify that the file has been opened successfully:
if (!outFile.is_open()) {
std::cerr << "Error: File could not be opened." << std::endl;
}
This check helps prevent errors that may arise from attempting to write to an unopened or non-existent file.
Handling Write Errors
To ensure data integrity, it’s important to verify write operations. The `fail()` method checks for write errors:
if (outFile.fail()) {
std::cerr << "Error during write operation." << std::endl;
}
Implementing such checks can help catch issues early and maintain robustness.
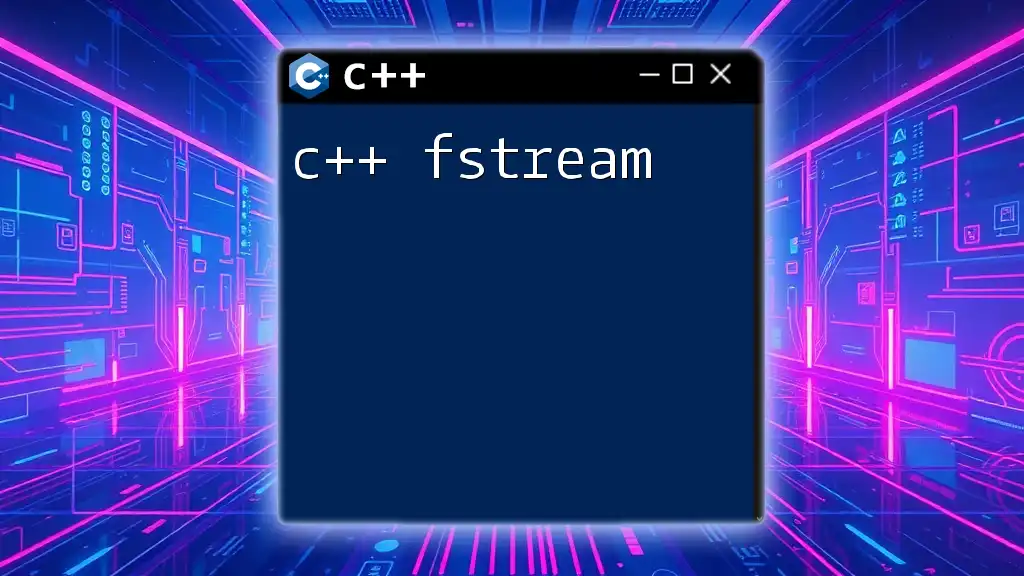
Best Practices when Using ofstream
Proper Resource Management
Always remember to close files to free up system resources. Following the RAII (Resource Acquisition Is Initialization) principle aids in preventing resource leaks.
Avoiding Hardcoding File Names
For greater flexibility, consider utilizing configuration files or command-line parameters to specify file names. This practice makes your code more adaptable and easier to maintain.
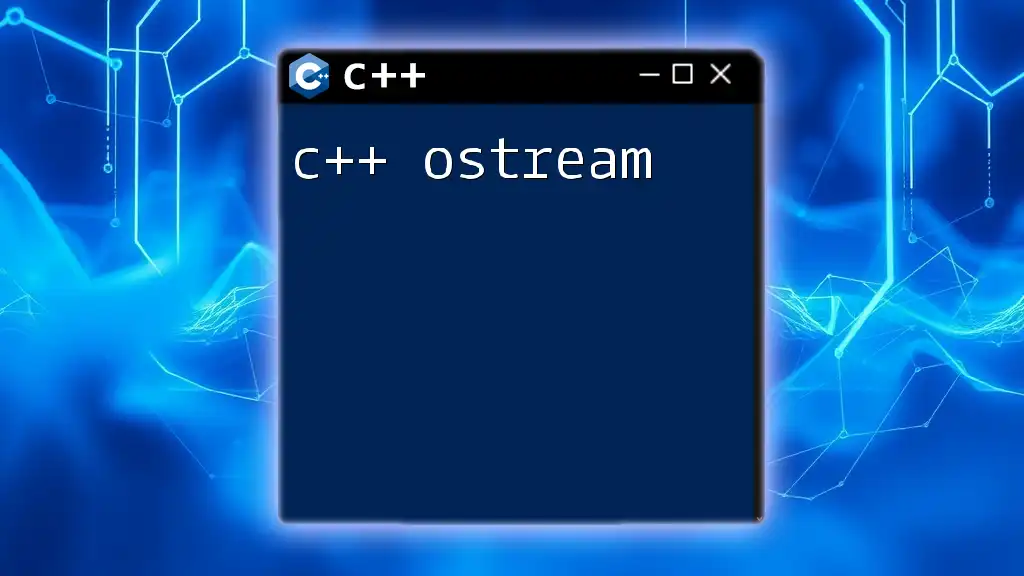
Common Mistakes & Troubleshooting
Forgetting to Close Files
One of the most frequent pitfalls is neglecting to close a file. Always ensure `outFile.close()` is called before your program ends to safely save data.
Not Checking if File is Open
Failure to check if a file is open might lead to write failures, causing unexpected behavior in your application.
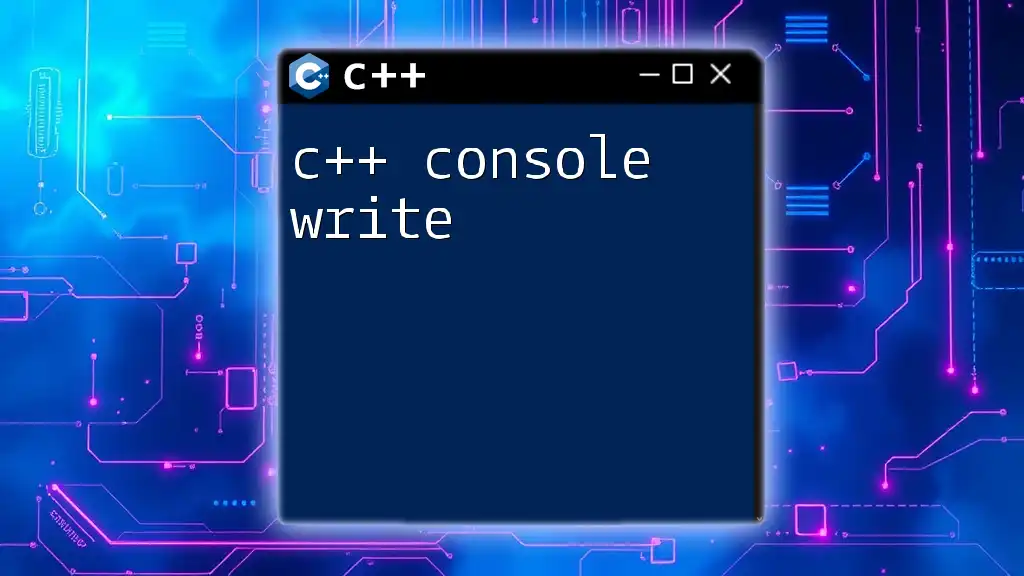
Conclusion
In summary, mastering c++ ofstream write operations is crucial for efficient file handling in modern C++ programming. Understanding how to open files, write various data types, format output, and handle errors will equip you with the skills necessary to confidently manage file operations. The key to success lies in practice, so experiment with these techniques to refine your file handling capabilities.
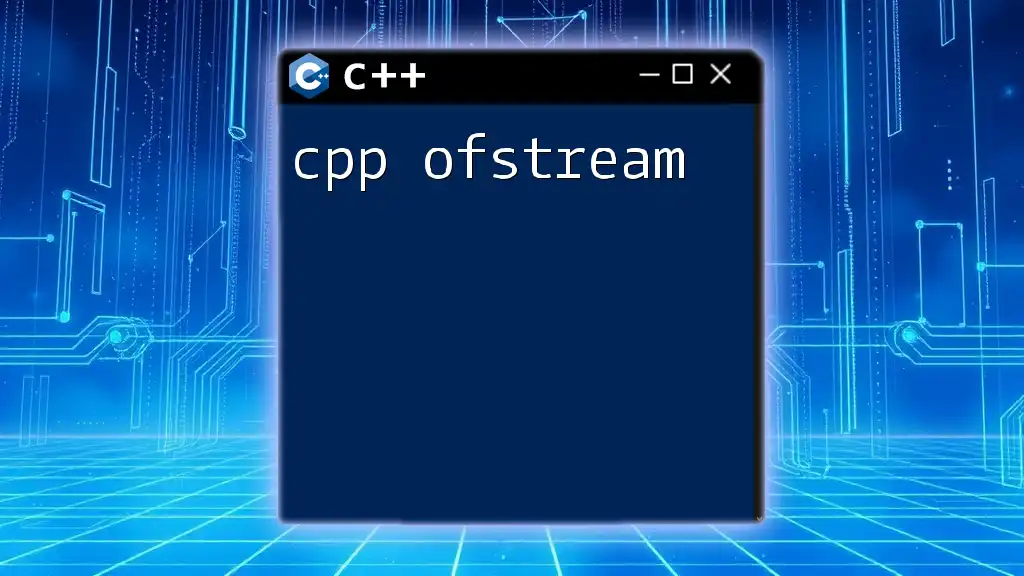
Additional Resources
References for Further Learning
For more insights and comprehensive learning materials, explore C++ documentation, tutorials, and forums.
Code Snippets Repository
Consider utilizing coding platforms or GitHub repositories that offer a plethora of examples and challenges related to file handling in C++. Engaging with these resources will significantly enhance your proficiency with `ofstream` and overall C++ file operations.