In C++, the `cout` object from the `<iostream>` library is used to write output to the console, allowing you to display text and variable values.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Console Output in C++
What is Console Output?
Console output is the mechanism through which a program displays information to the user in a text-based interface, commonly referred to as the command line or terminal. It plays a crucial role in programming, enabling developers to communicate with users and showcase dynamic data. Console output is fundamental for debugging purposes as it allows programmers to observe variable states, error messages, and program workflows in real time.
Why Learn C++ Console Write?
Learning C++ console write commands is essential for several reasons:
- Debugging: Outputting variable values during the execution helps identify logical errors.
- User Interaction: Many applications require user input and feedback, relying on console output for meaningful exchanges.
- Common Use Cases: Simple applications, learning environments, and demonstration scripts often utilize console output for clarity and immediacy.
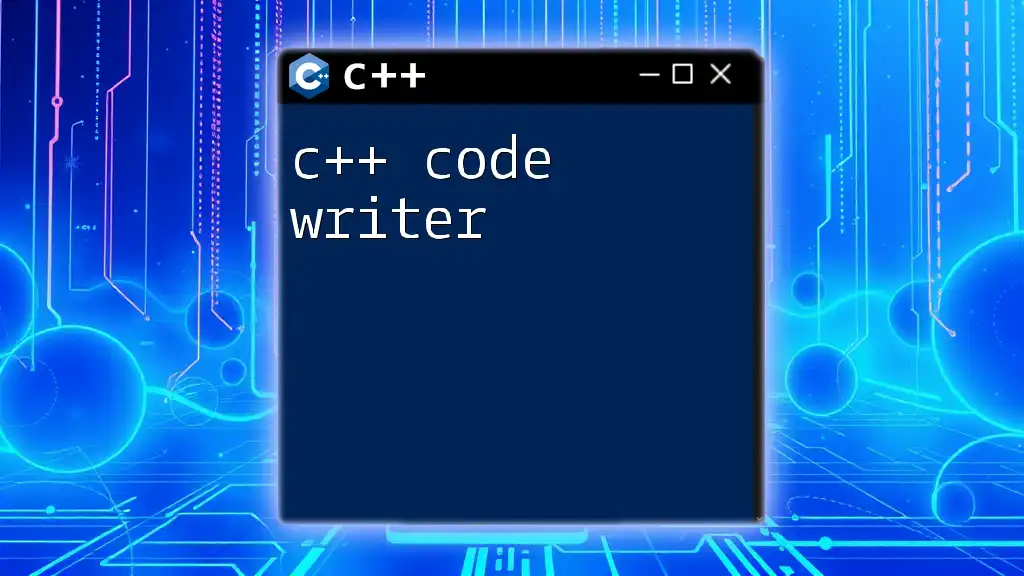
Basic Console Write Using std::cout
Introduction to std::cout
In C++, `std::cout` is the standard output stream, which is part of the `<iostream>` library. It allows programmers to send output data to the console. Understanding how to use `std::cout` effectively is foundational for any C++ developer.
The Syntax of std::cout
The basic syntax for using `std::cout` involves the insertion operator (`<<`), which directs output to the standard output stream. Here’s a simple line showing how it works:
std::cout << "Hello, World!";
Example of Basic Console Output
To illustrate how console output works, consider this minimal C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this code snippet:
- We include the `<iostream>` library, which gives us access to `std::cout`.
- In the `main()` function, we use `std::cout` to print the message "Hello, World!" to the console.
- The use of `std::endl` inserts a new line after the output, which improves readability.
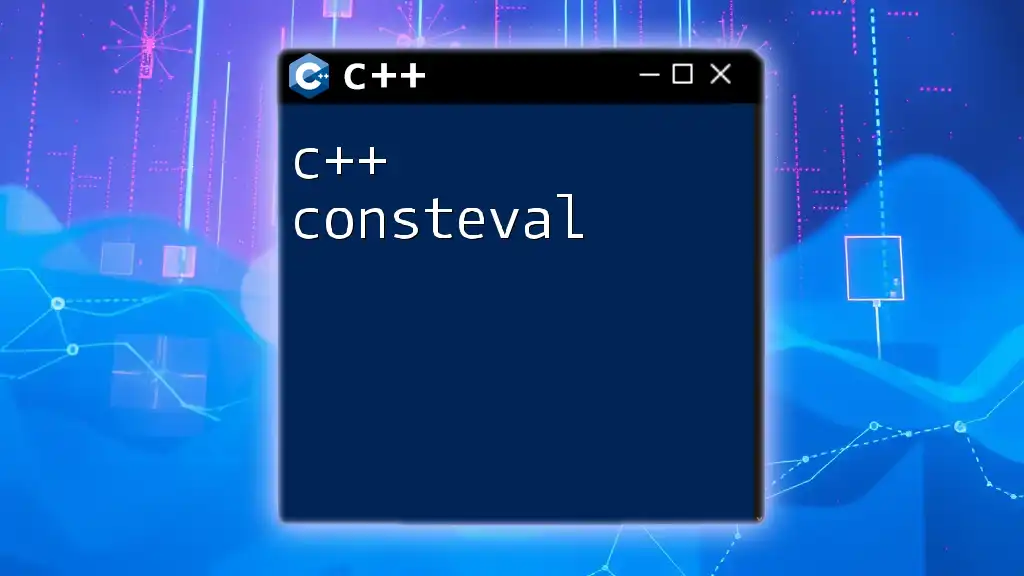
Formatting Console Output
Using std::endl
The `std::endl` manipulator is often used to add a new line after the output statement. However, it also clears the output buffer, ensuring that all data is written out at that moment. Understanding when to use `std::endl` versus the newline character (`\n`) can optimize performance in scenarios where output speed is critical.
Formatting with Manipulators
Introduction to Manipulators
Manipulators in C++ allow for the formatting of output data. Common manipulators include:
- `std::setw` for setting the width of output.
- `std::setprecision` for controlling the number of decimal places in floating-point output.
Examples of Using Manipulators
Here’s an example demonstrating how to use manipulators in a C++ program:
#include <iostream>
#include <iomanip>
int main() {
double pi = 3.14159;
std::cout << std::setprecision(3) << pi << std::endl; // Outputs: 3.14
return 0;
}
In this snippet, we see the following:
- We included `<iomanip>`, which provides functionality for manipulators.
- The `std::setprecision(3)` manipulator adjusts the output to display only three digits after the decimal point.
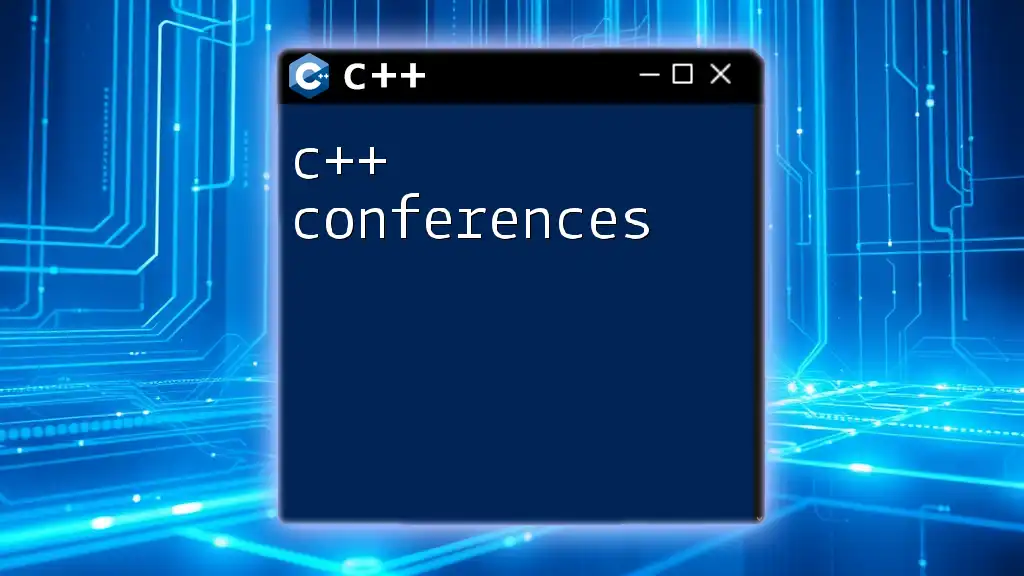
Writing Various Data Types to Console
Outputting Strings
When working with console output, we can send both C-style strings (character arrays) and `std::string` objects. Strings allow for flexible and dynamic text output. Here’s a brief example:
#include <iostream>
#include <string>
int main() {
std::string greeting = "Hello, C++!";
std::cout << greeting << std::endl;
return 0;
}
This program demonstrates:
- The use of an `std::string` variable to hold the text.
- Outputting the string directly to the console.
Outputting Numbers
C++ provides seamless capabilities for outputting multiple data types, including integers and floating-point numbers. Here’s how we can output various numeric types:
#include <iostream>
#include <iomanip>
int main() {
int number = 42;
double decimal = 3.14159;
std::cout << "Integer: " << number << ", Float: " << std::setprecision(2) << decimal << std::endl;
return 0;
}
In this example:
- We output an integer and a floating-point number together, enhancing clarity by labeling each type.
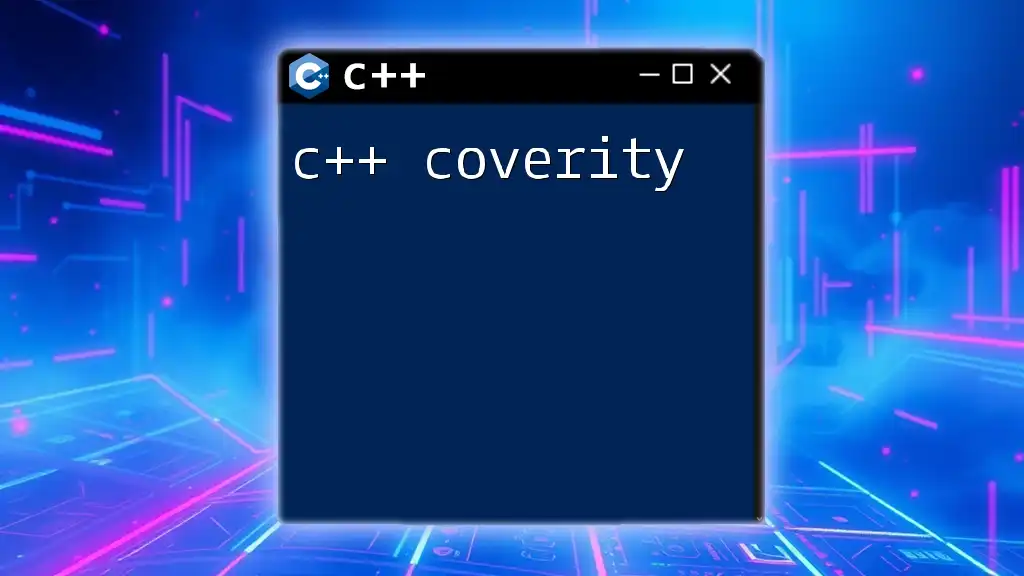
Advanced Console Output Techniques
Chaining Outputs with std::cout
One of the powerful features of `std::cout` is the ability to chain multiple output statements in one line. This reduces the number of lines and improves code efficiency. For example:
#include <iostream>
int main() {
std::cout << "My name is " << "John" << ", I am " << 30 << " years old." << std::endl;
return 0;
}
This code demonstrates how you can concatenate different data types in a single output statement, producing a clear and concise message.
Conditional Outputs
Conditional outputs enable dynamic feedback based on user input or program logic. Here’s a small program showing how to control console output based on conditions:
#include <iostream>
int main() {
int age;
std::cout << "Enter your age: ";
std::cin >> age;
std::cout << "You are " << (age >= 18 ? "an adult." : "a minor.") << std::endl;
return 0;
}
In this code snippet:
- The program prompts the user for their age and checks whether it is greater than or equal to 18.
- It uses the conditional (ternary) operator to produce different outputs based on the user’s input.
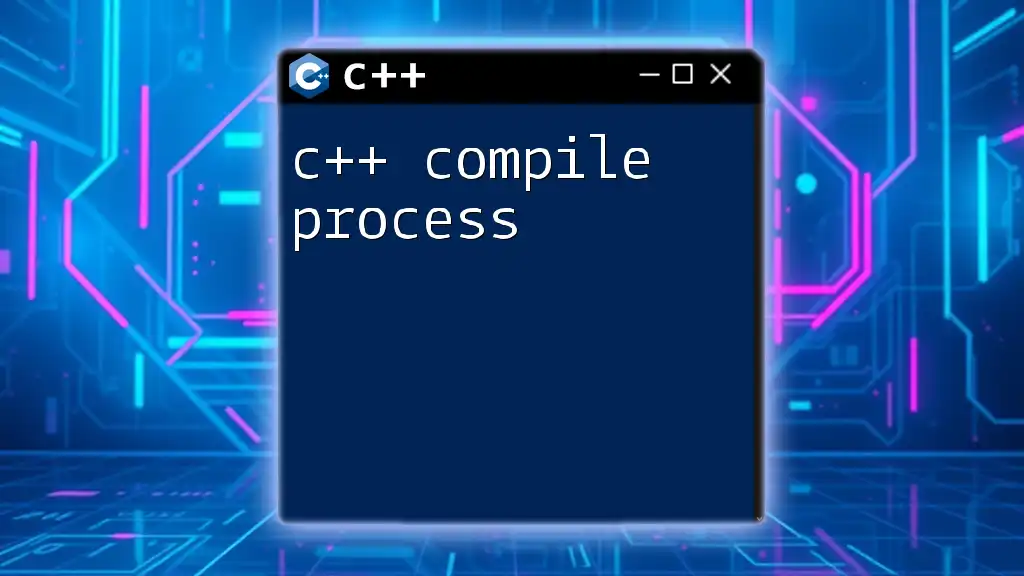
Conclusion
In this guide, we explored the essential aspects of C++ console write commands. From utilizing `std::cout` for basic output to formatting data with manipulators, the ability to effectively communicate through console output is a vital skill for any C++ programmer. Remember, practice is key. Engage with various C++ exercises to strengthen your understanding and become proficient in using console output features.
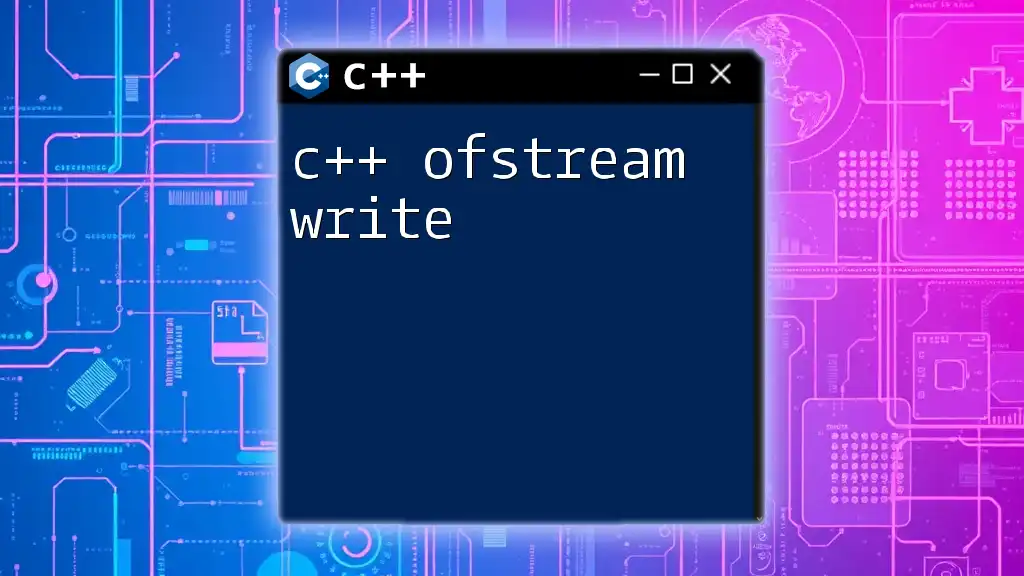
Additional Resources
To further enhance your learning experience, consider exploring online C++ documentation, picking up recommended C++ programming books, or joining C++ programming communities and forums where you can connect with other learners and experienced developers.