A C++ code writer is a tool or script designed to assist programmers in generating C++ code quickly and efficiently. Here’s an example of a simple C++ program that outputs "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is a C++ Code Writer?
A C++ code writer is a programmer skilled in writing software applications using the C++ programming language. This role is crucial in various industries where performance and efficiency are paramount. A proficient C++ code writer understands the language's syntax and paradigms, enabling them to create clean, maintainable, and efficient code.
Skill Sets Required
To become an effective C++ code writer, one must possess a solid foundation in essential programming concepts such as variables, data types, loops, and conditionals. Familiarity with C++ syntax is critical, as it differs in several ways from other programming languages. Furthermore, skills in debugging, code optimization, and a good grasp of object-oriented programming are invaluable in producing high-quality code.
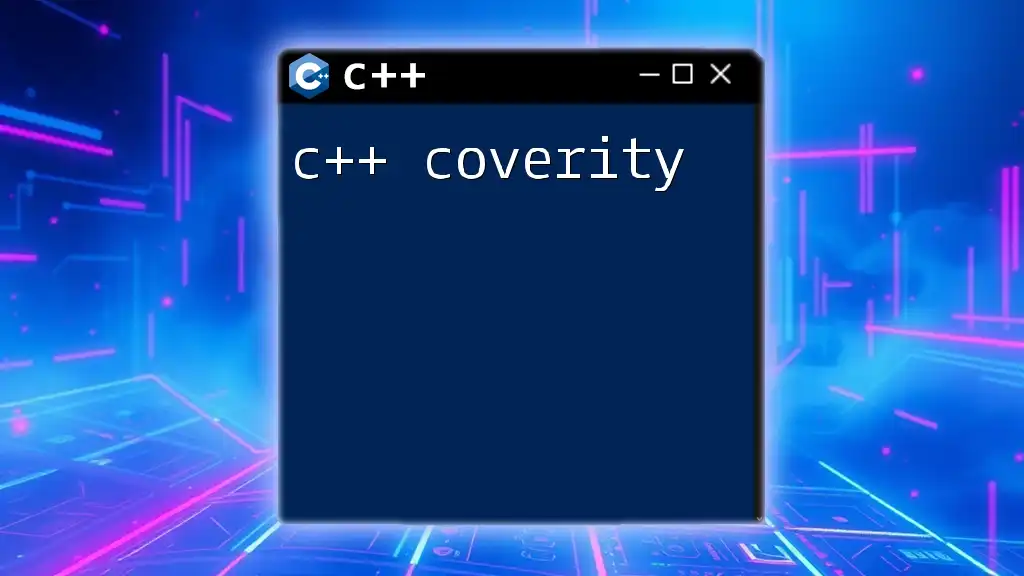
Understanding C++ Basics
Installing C++ Environment
Before diving into C++ development, you must set up the appropriate environment. This generally includes selecting a compiler and an Integrated Development Environment (IDE).
Compilers and IDEs
Popular C++ compilers include GCC, Clang, and Microsoft Visual C++. Each has unique strengths; for instance, GCC is widely used in Linux environments, while Visual C++ integrates seamlessly with Windows applications. For IDEs, options like Code::Blocks, Visual Studio, and CLion offer comprehensive tools to make your coding experience more enjoyable.
Setting Up Your First C++ Project
To create your first C++ program, follow these steps:
- Install your chosen compiler and IDE.
- Create a new project in your IDE.
- Write the basic "Hello World" program, which serves as an excellent starting point.
Here is the code snippet to create this simple program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
C++ Syntax and Structure
Understanding the structure and syntax of C++ is paramount for a C++ code writer.
Basic Syntax Rules
C++ syntax consists of various elements, including variable declarations, data types (like int, float, and char), and operators. The main function is the entry point of any C++ program.
Control Structures
Control structures such as if statements, loops (for, while), and switch cases are fundamental for creating dynamic and responsive programs.
Here’s an example of a simple loop:
for (int i = 0; i < 5; i++) {
std::cout << i << " ";
}
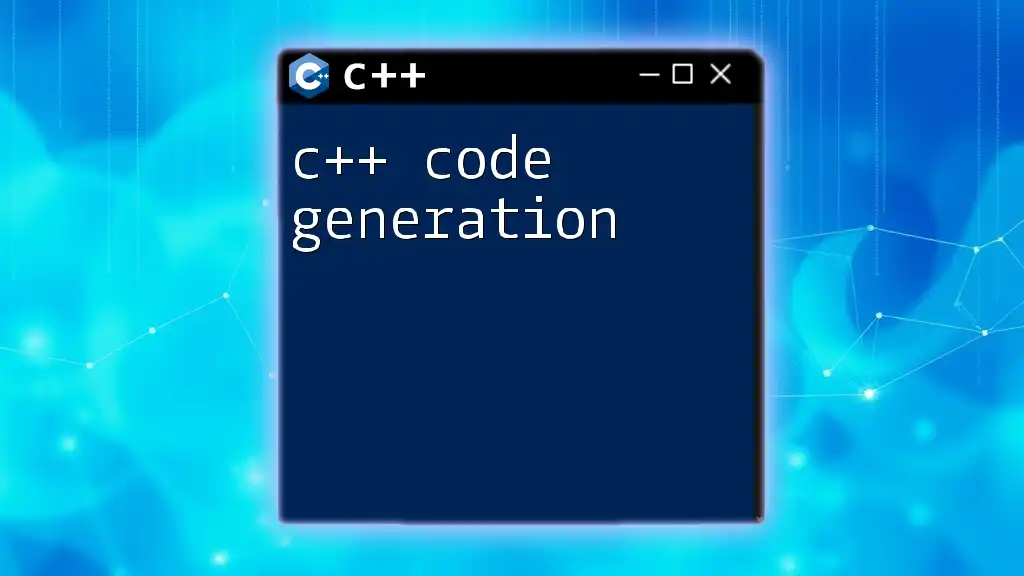
Writing Efficient C++ Code
Code Organization Techniques
Good code organization is vital for maintainability and clarity.
Modular Programming
Modular programming allows you to break up complex tasks into smaller, manageable functions. C++ supports this through the use of functions and header files, which can be included in multiple source files.
Commenting and Documentation
Commenting your code is not merely good practice; it is essential for maintaining readability. Use comments to explain complex logic. A well-documented codebase helps others—and yourself—understand your work when revisiting it later.
Best Practices for C++ Code Writing
Naming Conventions
Choose descriptive names for variables and functions. Consistency in naming helps improve code readability. For instance, use camelCase for variable names and PascalCase for function names.
Avoiding Common Mistakes
Memory management is a common pitfall for many C++ programmers. Always ensure that dynamically allocated memory is freed appropriately to avoid memory leaks.
Here’s an example of a simple function that calculates the square of a number:
int calculateSquare(int number) {
return number * number;
}
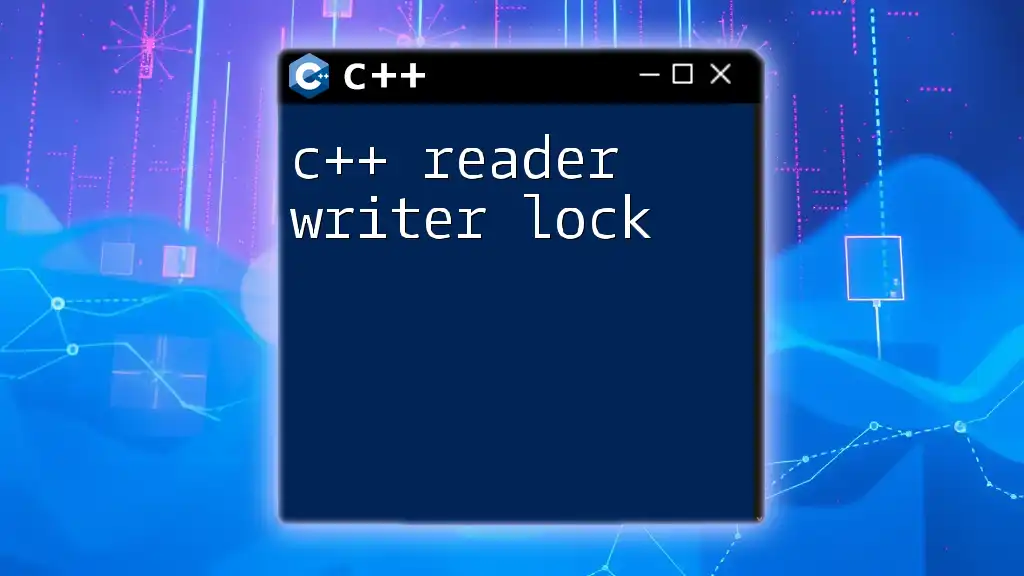
Tools and Resources for C++ Code Writers
Development Tools
Using development tools can significantly enhance your productivity as a C++ code writer.
Version Control Systems
Version control systems like Git allow you to track changes in your codebase over time. They facilitate collaboration and make it easier to revert to earlier code versions if necessary.
Debugging Tools
Debugging is a critical part of programming. Tools like gdb and Valgrind can help identify issues in your code and ensure it runs smoothly.
Online Resources
Leverage online resources to bolster your C++ learning.
Websites and Forums
Visit sites like cppreference.com for detailed C++ documentation and Stack Overflow for community support and solutions to coding issues.
Online Courses and Tutorials
Consider enrolling in online courses that offer structured and comprehensive learning experiences.
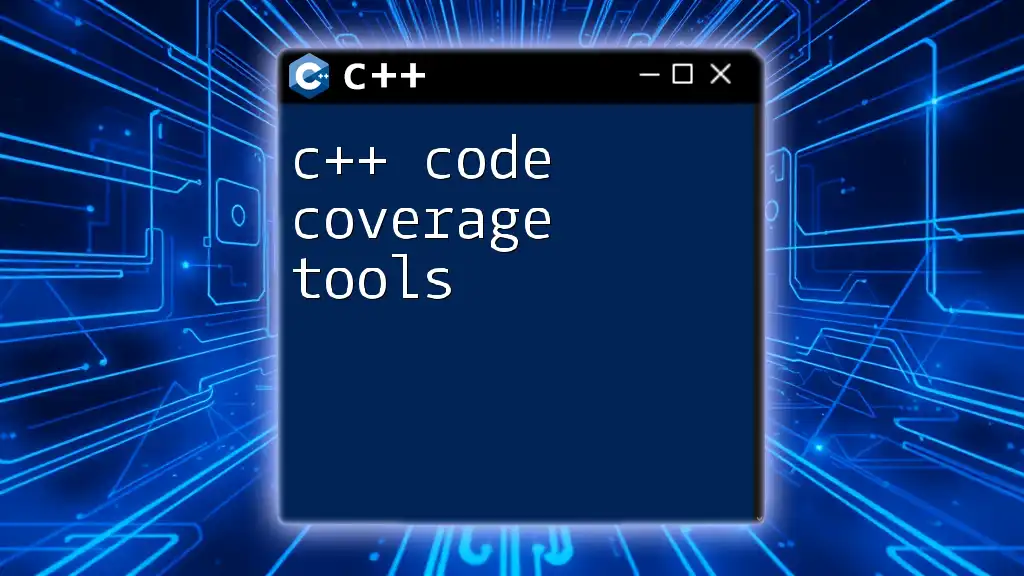
Common Challenges in C++ Programming
Understanding Pointers and Memory Management
Pointers are a unique feature of C++ but can be intricate for beginners. They allow for direct memory manipulation but require careful handling to avoid errors.
Working with Libraries and Frameworks
C++'s Standard Template Library (STL) provides a wide range of data structures and algorithms, simplifying many programming tasks. Familiarity with libraries like Boost can further enhance your coding efficiency.
Here’s how to use STL efficiently:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (auto num : numbers) {
std::cout << num << " ";
}
}
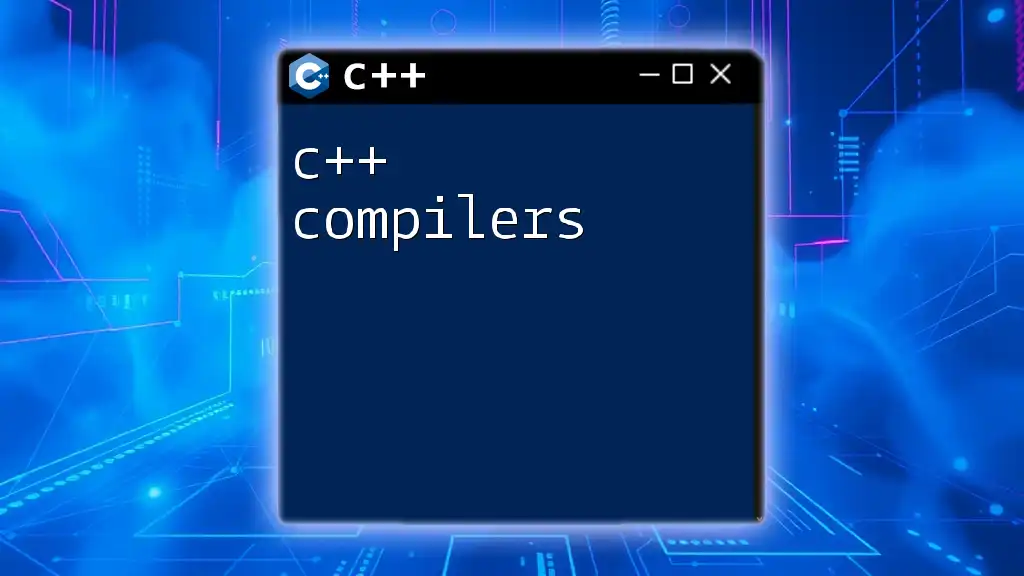
Real-World Applications of C++
Industries Commonly Using C++
C++ is a versatile language utilized in various fields, including game development, telecommunications, finance, and high-performance computing. Its ability to manage hardware resources effectively makes it highly sought after.
Case Studies
Companies like Microsoft and Google use C++ extensively in various projects, highlighting its reliability and efficiency in developing robust applications.
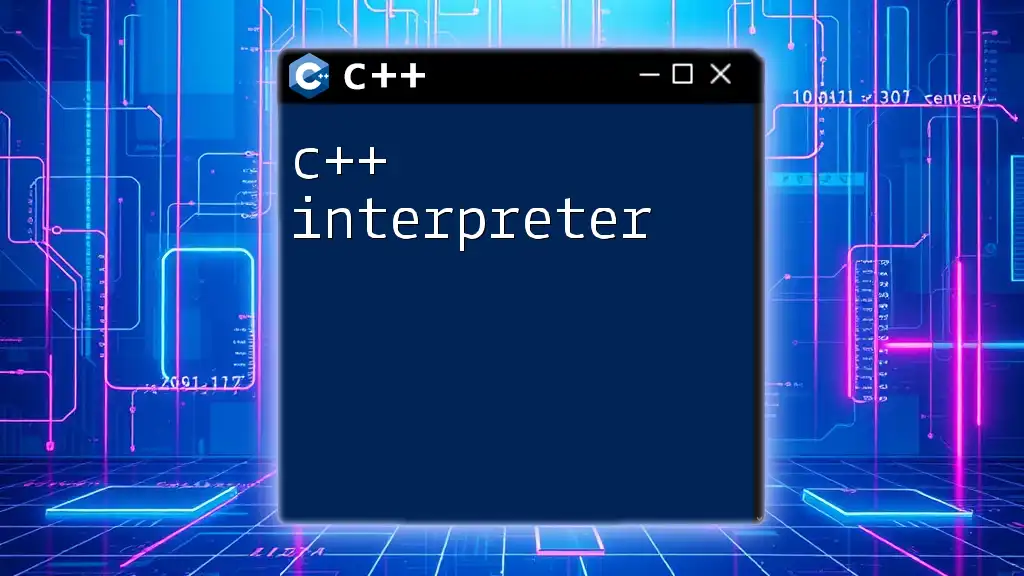
Conclusion
Becoming a proficient C++ code writer requires dedication and continuous practice. Understanding the fundamentals, embracing best practices, and utilizing available tools and resources is essential for mastering this powerful language.
As you embark on your journey to becoming a skilled C++ programmer, remember that practice is the key to success. Keep exploring, coding, and seeking knowledge, and you'll find your skills and confidence growing in this dynamic field.