A C++ reader-writer lock is a synchronization primitive that allows multiple threads to read shared data concurrently while ensuring exclusive access for writing, thereby optimizing performance in scenarios where reads are more frequent than writes.
Here's a simple example of using a reader-writer lock in C++:
#include <iostream>
#include <shared_mutex>
#include <thread>
#include <vector>
std::shared_mutex rw_lock;
int shared_data = 0;
void reader(int id) {
std::shared_lock<std::shared_mutex> lock(rw_lock);
std::cout << "Reader " << id << " read: " << shared_data << std::endl;
}
void writer(int id) {
std::unique_lock<std::shared_mutex> lock(rw_lock);
shared_data += 1;
std::cout << "Writer " << id << " updated data to: " << shared_data << std::endl;
}
int main() {
std::vector<std::thread> threads;
for (int i = 0; i < 5; ++i) {
threads.emplace_back(reader, i);
threads.emplace_back(writer, i);
}
for (auto& t : threads) {
t.join();
}
return 0;
}
Understanding the Basics of C++ Synchronization
Concurrency in C++ is an essential aspect of modern applications, especially with the rise of multi-core processors. Understanding how to manage multiple threads efficiently is crucial when dealing with shared resources.
Common Synchronization Mechanisms
To effectively manage access to resources in a multi-threaded environment, various synchronization mechanisms are employed. The most common include mutexes and condition variables. Mutexes provide a way to ensure that only one thread can access a particular piece of code at a time, while condition variables enable threads to wait for certain conditions to be met before proceeding.
However, these basic mechanisms have their drawbacks. Mutexes can lead to degradation in performance, especially in scenarios where multiple threads are primarily reading data rather than writing it. This is where reader-writer locks can come into play. They allow multiple readers to access shared data concurrently while ensuring that only one writer can modify the data at any given moment.
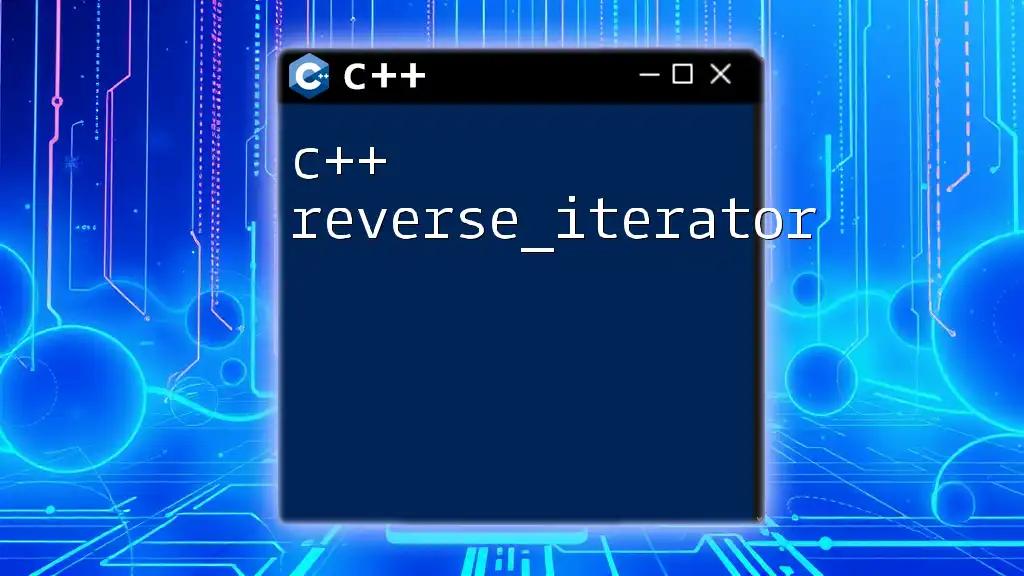
C++ Reader-Writer Locks Explained
Key Concepts
At its core, a C++ Reader-Writer Lock allows multiple threads to read data while preventing write access during reading. Conversely, when a thread wishes to write, it must ensure exclusive access to the data, blocking any potential readers during this time. This dual-access mechanism provides a balanced approach to concurrency management.
How Reader-Writer Locks Work
Reader-Writer locks function by maintaining two separate modes of access: shared (for readers) and exclusive (for writers). In scenarios with high read operations, allowing multiple readers to access the shared resource simultaneously can vastly improve performance. However, when a writer needs to update the resource, it must achieve exclusive access, blocking all other reading or writing threads.
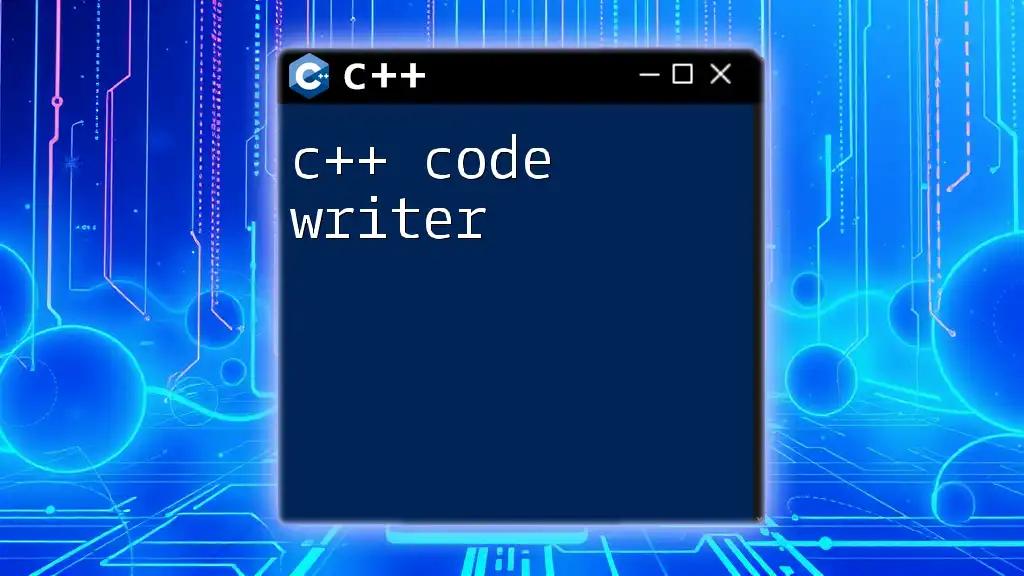
Implementing a C++ Read-Write Lock
To implement a C++ Reader-Writer Lock, the C++ standard library provides a convenient class known as std::shared_mutex. This class facilitates concurrent read access while still safeguarding the integrity of data during write operations.
Code Example: Simple Reader-Writer Lock Implementation
Here’s an example that demonstrates a basic implementation of a read-write lock using `std::shared_mutex`:
#include <iostream>
#include <shared_mutex>
#include <thread>
class SharedData {
public:
void read() {
std::shared_lock<std::shared_mutex> lock(mutex_);
// Perform read operations
std::cout << "Reading data...\n";
// Simulate reading delay
std::this_thread::sleep_for(std::chrono::milliseconds(100));
}
void write() {
std::unique_lock<std::shared_mutex> lock(mutex_);
// Perform write operations
std::cout << "Writing data...\n";
// Simulate writing delay
std::this_thread::sleep_for(std::chrono::milliseconds(100));
}
private:
std::shared_mutex mutex_;
};
void reader(SharedData& data) {
data.read();
}
void writer(SharedData& data) {
data.write();
}
Understanding `std::shared_mutex`
The `std::shared_mutex` class is a powerful tool for implementing reader-writer locks in C++. It allows multiple threads to acquire shared locks while only one thread can hold an exclusive lock. This not only improves efficiency in read-heavy scenarios but also provides a straightforward interface for thread safety in your applications.
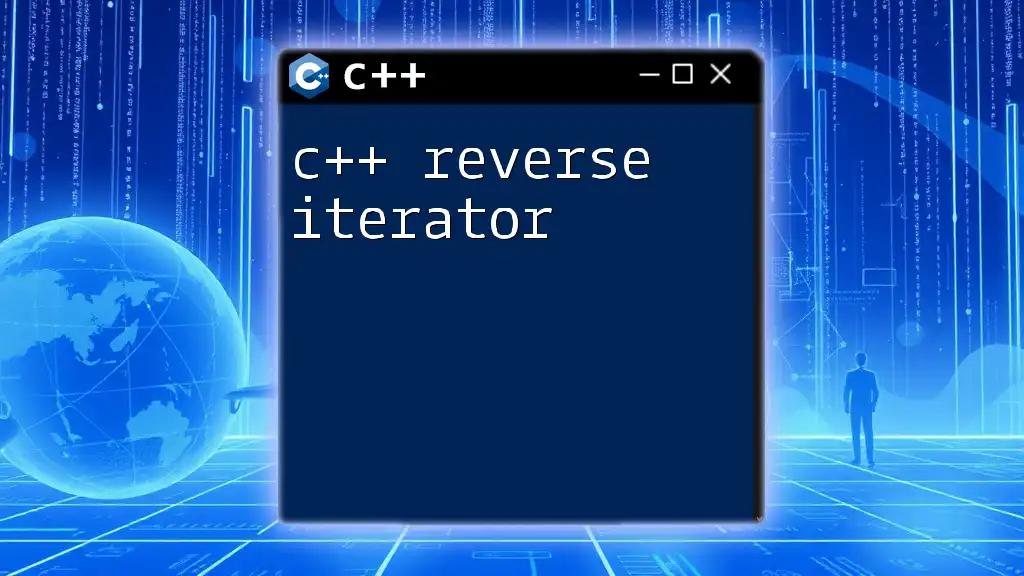
Practical Applications of Reader-Writer Locks
Use Cases in Real-World Applications
C++ Reader-Writer Locks are particularly useful in applications that handle a significant amount of read operations, such as:
- Databases: Many database engines utilize reader-writer locks to allow multiple users to read records simultaneously, while ensuring data integrity during insertions or updates.
- Caching Systems: In a caching scenario, readers can frequently access cached data without requiring a lock, only acquiring one when the cache needs to be updated.
Examples of Efficiency Gains
In many cases, the performance improvements gained by allowing multiple readers can be substantial. For instance, when testing a read-write scenario with hundreds of concurrent threads, reader-writer locks can drastically reduce the time it takes to complete all operations compared to a traditional mutex setup.
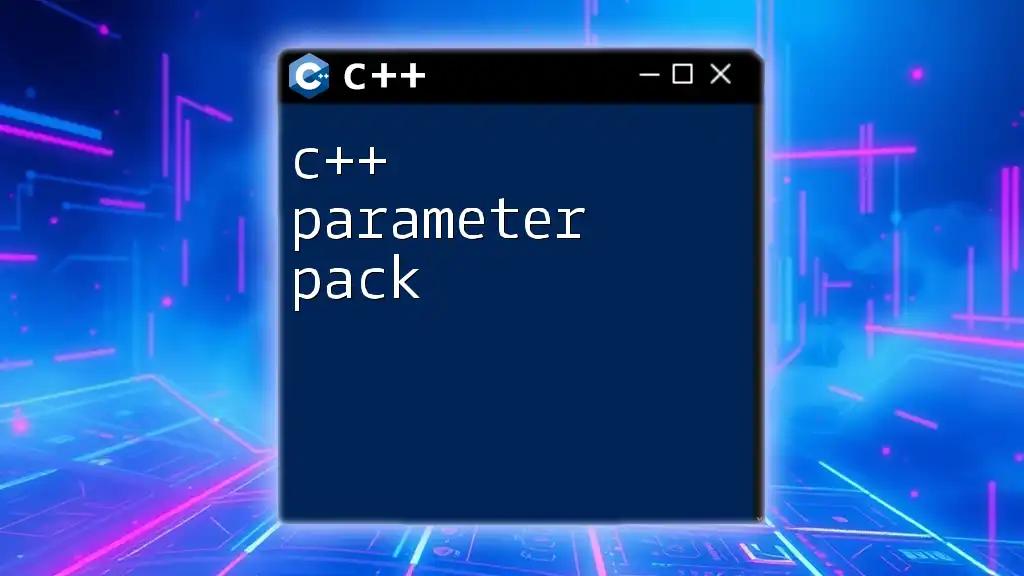
Handling Deadlocks and Race Conditions
Common Issues with Reader-Writer Locks
While reader-writer locks simplify synchronization in certain contexts, they can introduce complexity and potential issues. For instance, the potential for deadlocks exists if threads are not managed properly—particularly when both readers and writers compete for resources.
Best Practices
To avoid these issues, follow best practices such as:
- Lock Ordering: Establish a well-defined ordering for acquiring locks to prevent deadlock scenarios. Always try to acquire reader and writer locks in the same order across all threads.
- Clear Access Patterns: Maintain clarity about how threads access shared resources. Use documentation and code comments to describe expected behavior and access logic.
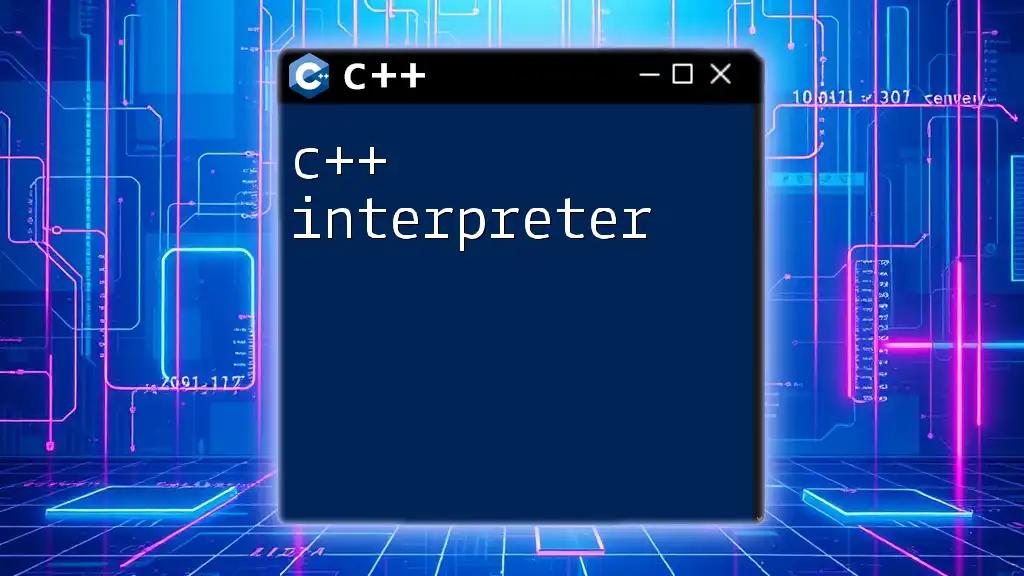
Performance Considerations
Measuring Lock Contention
Understanding how often locks are being contended will provide insights into your program’s performance. Lock contention refers to the situation where multiple threads attempt to acquire locks simultaneously, which may lead to delays in execution.
Choosing Between Reader-Writer Locks and Other Synchronization Methods
The decision to use a reader-writer lock versus other synchronization methods involves balancing complexity and efficiency. If your application primarily reads data, a reader-writer lock might save significant time. Conversely, if write operations are more frequent, the overhead of managing a reader-writer lock may outweigh its benefits.
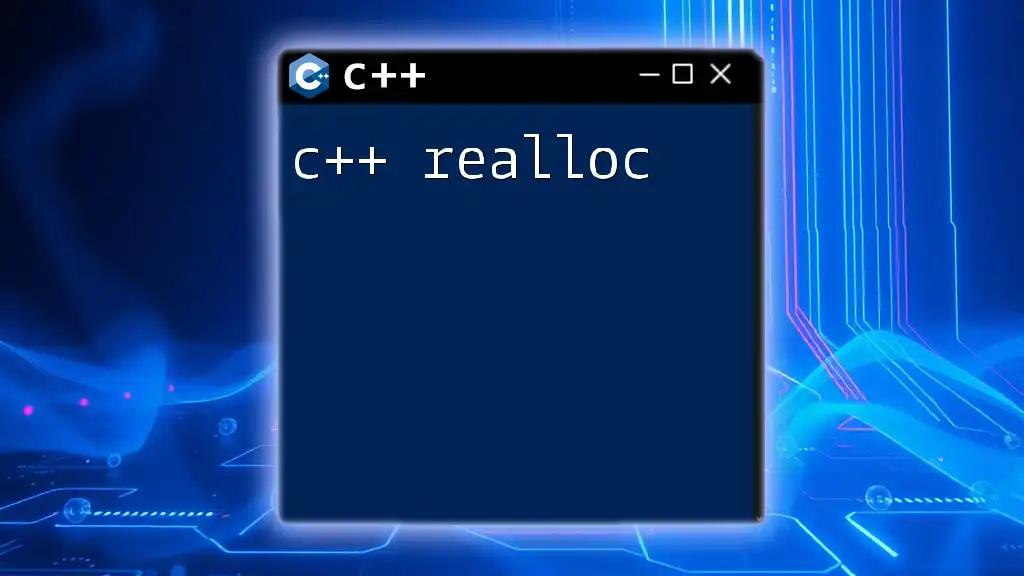
Conclusion
In summary, a C++ Reader-Writer Lock provides an elegant solution for managing concurrent read and write access to shared resources, optimizing performance in read-heavy applications. By implementing best practices and understanding the potential pitfalls, developers can significantly enhance the efficiency of their multi-threaded applications.
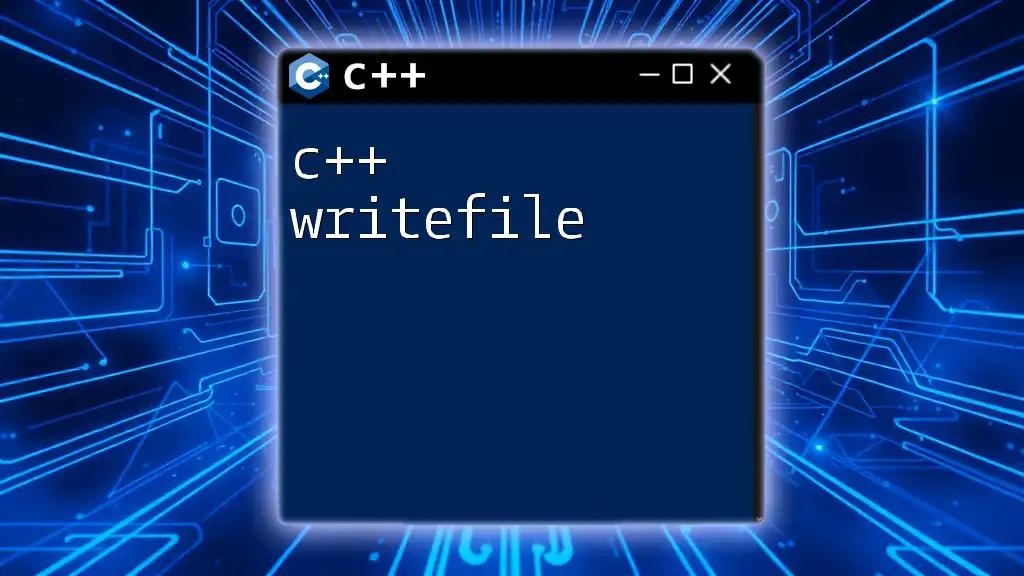
Additional Resources
For further learning, consider exploring books, articles, and online tutorials that delve deeper into concurrency, threading, and C++ lock mechanisms. Engaging in community forums and discussion groups can also provide valuable insights and support as you navigate the complexities of C++ concurrency.

Call to Action
We encourage you to join the conversation! Share your questions or experiences related to using C++ reader-writer locks in the comments section below. Your insights could help others in the community as they explore concurrency in their own projects.