In C++, the `CreateWindow` function is used to create a window by specifying its class, title, dimensions, and other properties.
HWND hwnd = CreateWindow(
"WindowClass", // Window class name
"My C++ Window", // Window title
WS_OVERLAPPEDWINDOW, // Window style
CW_USEDEFAULT, // X position
CW_USEDEFAULT, // Y position
800, // Width
600, // Height
nullptr, // Parent window
nullptr, // Menu
hInstance, // Instance handle
nullptr // Additional application data
);
Understanding Windows Programming Basics
Introduction to WinAPI
To begin with, WinAPI, also known as the Windows Application Programming Interface, is a crucial system for developing Windows applications using C++. It provides a set of functions that allow programmers to interact with the Windows operating system to create and manage windows, handle events, and perform various other tasks. Using WinAPI for window management offers numerous advantages, such as fine control over window behavior and a rich set of functionality crucial to application development.
Setting Up Your Development Environment
Before diving into C++ and the `CreateWindow` function, it’s essential to set up your development environment properly. You may use integrated development environments (IDEs) such as Visual Studio or MinGW.
- Create a new C++ project for a Windows application.
- Ensure you include necessary headers and libraries in your project. Typically, you need to include:
#include <windows.h>
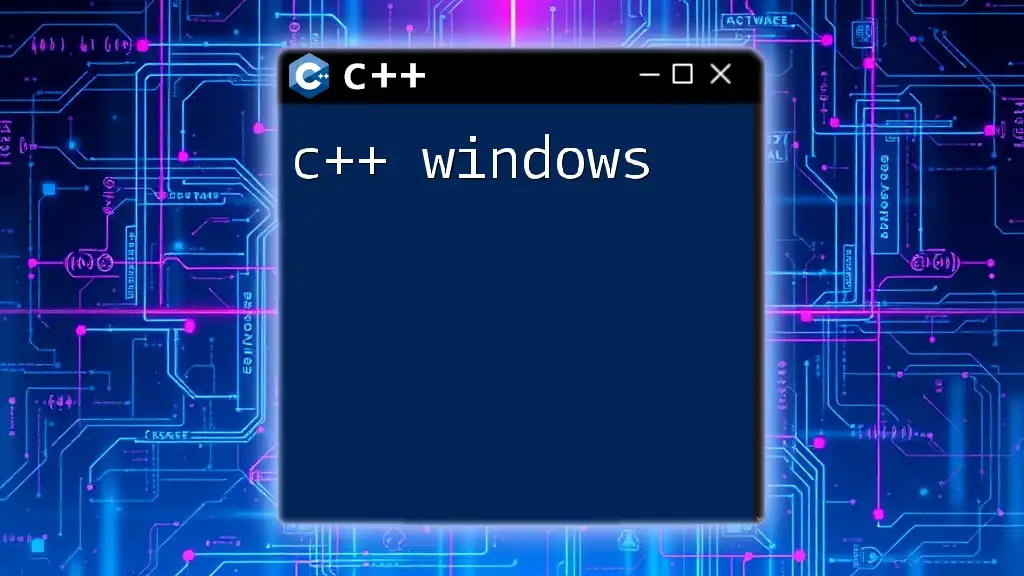
What is CreateWindow?
Definition and Purpose
The `CreateWindow` function is a fundamental WinAPI function that facilitates the creation of a new window. It plays a critical role in setting up the graphical user interface (GUI) of applications. This function is especially important for developers looking to manage multiple windows or customize window behavior in their applications.
Differences between CreateWindow and CreateWindowEx
While `CreateWindow` is widely used, it is essential to understand how it differs from `CreateWindowEx`. The latter allows for extended styles, giving developers greater flexibility in defining how their windows behave, such as transparency and layered windows.
Parameters of CreateWindow
The `CreateWindow` function has several parameters, each serving a specific purpose:
- hInstance: The instance handle for the application, usually obtained via `GetModuleHandle(NULL)`.
- lpClassName: The class name for the new window, which must be previously registered.
- lpWindowName: The title of the window, displayed in the window’s title bar.
- dwStyle: The window style, which determines the appearance and behavior of the window.
- x and y: The position of the new window on the screen.
- nWidth and nHeight: The window's width and height dimensions, in pixels.
- hWndParent: Handle to the parent window, if applicable.
- hMenu: Handle to a menu associated with the window.
- lpParam: Pointer to additional parameters that can be accessed in the window procedure.
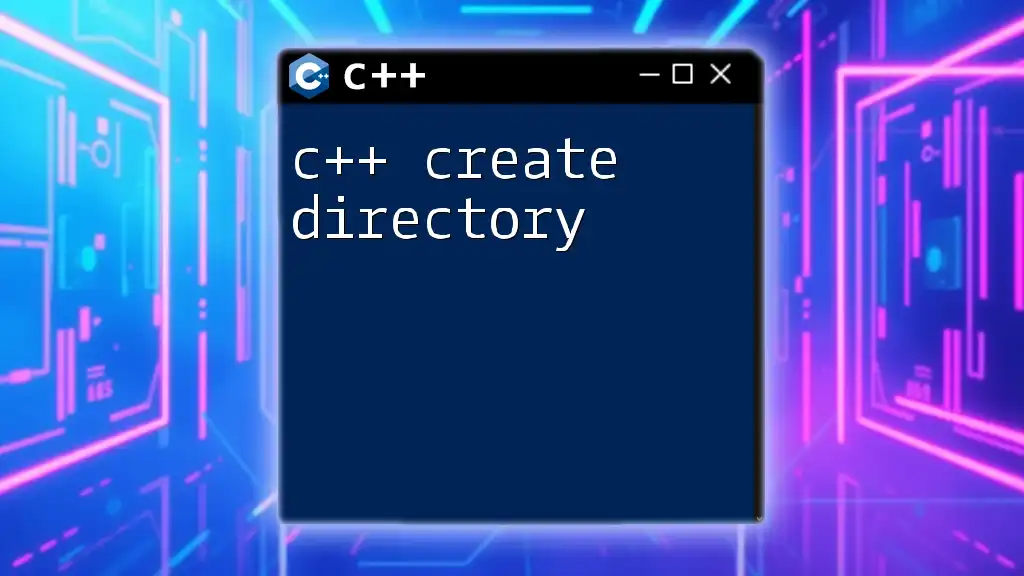
Step-by-Step Guide to Creating a Window
Step 1: Registering a Window Class
Before creating a window, you must register a window class using the `WNDCLASS` structure. This tells the operating system how to handle windows of this class.
WNDCLASS wc = {0};
wc.lpfnWndProc = WindowProc; // Pointer to the window procedure
wc.hInstance = hInstance;
wc.lpszClassName = "MyWindowClass";
RegisterClass(&wc);
Step 2: Creating the Window
Once the window class is registered, you can create the window yourself using the `CreateWindow` function.
HWND hwnd = CreateWindow(
"MyWindowClass",
"Hello, Windows!",
WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT,
CW_USEDEFAULT,
500,
400,
NULL,
NULL,
hInstance,
NULL);
Step 3: Displaying the Window
After you've created your window, the next step is to make it visible on the screen. You do this by calling `ShowWindow` and `UpdateWindow`.
ShowWindow(hwnd, SW_SHOW);
UpdateWindow(hwnd);
Step 4: Message Loop
A critical concept in Windows programming is the message loop, which processes events (messages) sent to your window. It keeps your application responsive to user inputs.
MSG msg;
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
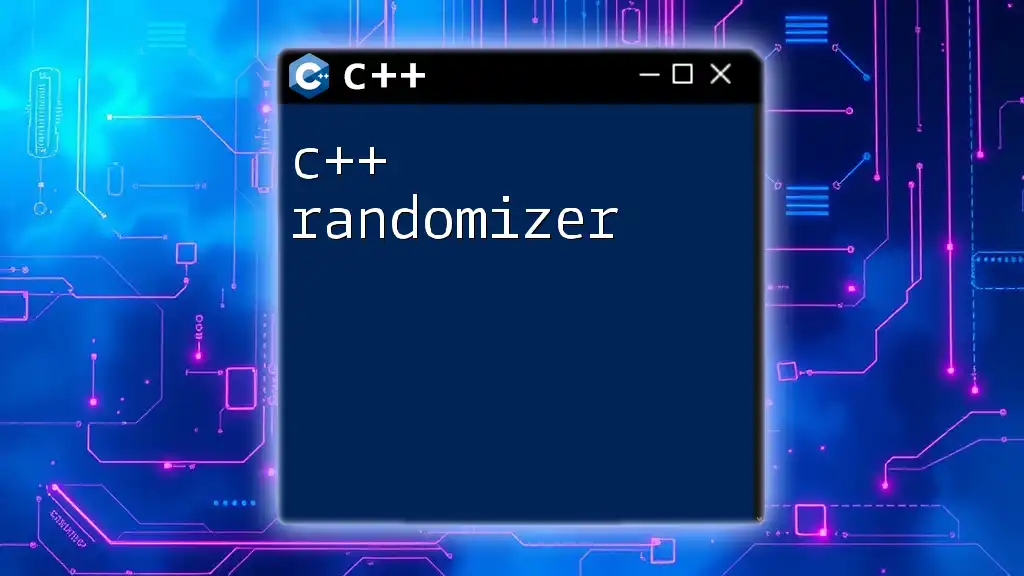
Customizing the Window
Changing Window Styles
Customizing window styles can significantly alter how your application appears and interacts with users. Common styles include:
- WS_BORDER: Adds a border to the window.
- WS_CAPTION: Adds a title bar.
- WS_VISIBLE: Makes the window visible upon creation.
Handling Window Messages
Windows communicates with your application using messages. You will need to handle these in your window procedure, typically defined as `WndProc`.
LRESULT CALLBACK WindowProc(HWND hwnd, UINT uMsg, WPARAM wParam, LPARAM lParam) {
switch (uMsg) {
case WM_DESTROY:
PostQuitMessage(0);
return 0;
// Handle other messages...
}
return DefWindowProc(hwnd, uMsg, wParam, lParam);
}
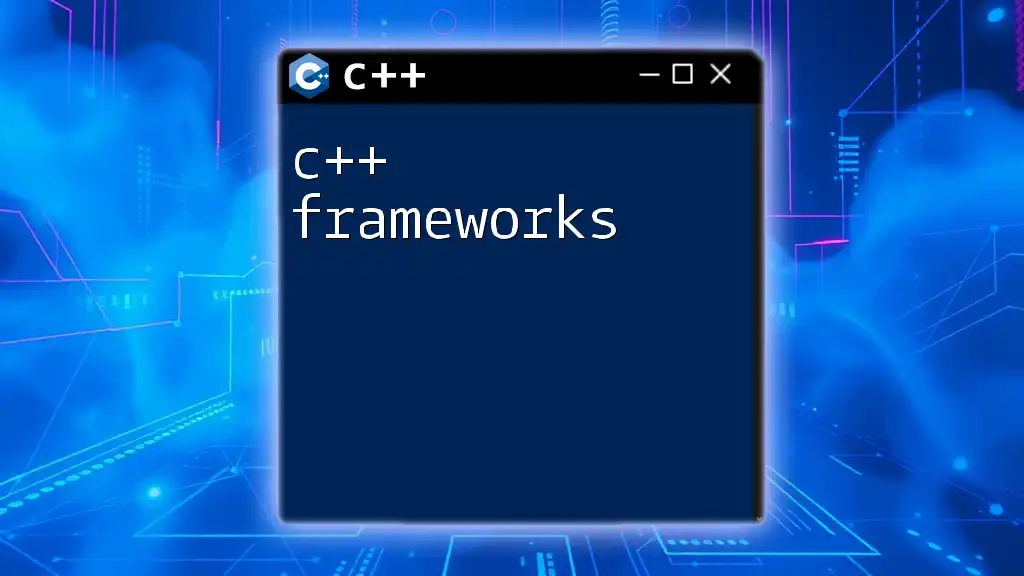
Common Issues and Troubleshooting
Debugging Window Creation Errors
When working with the `CreateWindow` function, you may encounter various errors. Common issues include incorrect class names or unregistered window classes. It’s vital to handle these errors gracefully. Utilizing `GetLastError()` can help you diagnose problems and log error codes to identify their cause.
Performance Considerations
To ensure user satisfaction and a fluid experience, adhere to best practices when designing your windows. Avoid blocking the message loop with long operations. Instead, offload intensive tasks into separate threads or use timers to keep your application responsive.
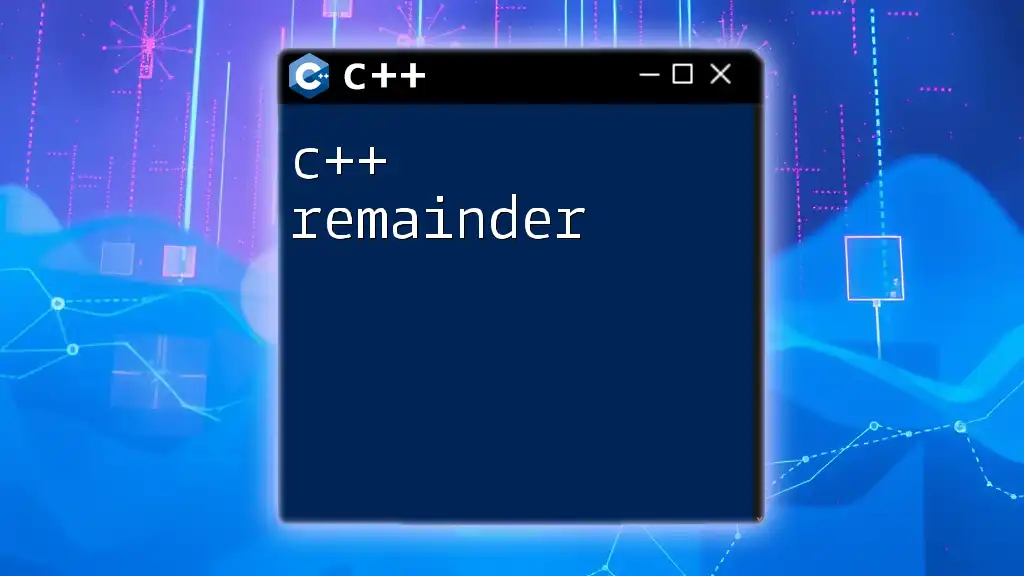
Conclusion
In this comprehensive guide, we’ve walked through the key aspects of using `CreateWindow` in C++. Through understanding the WinAPI, registering window classes, and managing message loops, you can create functional Windows applications that respond effectively to user interactions. We encourage you to explore this topic further, experiment with different window styles, and become proficient in building rich graphical user interfaces with C++.
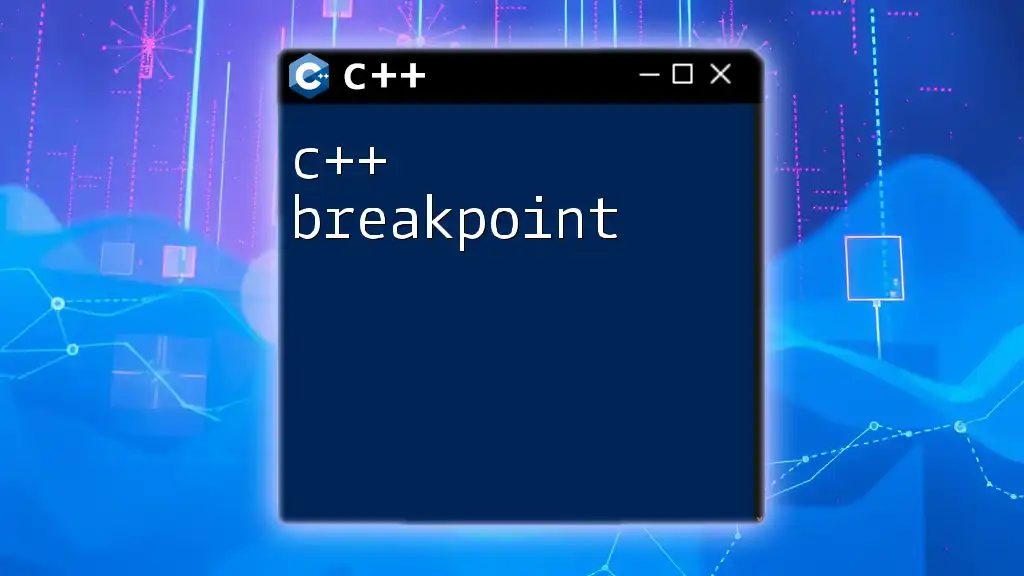
Additional Resources
For further reading and exploration, consider browsing the official Microsoft documentation on WinAPI, engaging with community forums, or diving into recommended books and online courses focused on C++ Windows programming. These are invaluable resources to solidify your knowledge and enhance your skills.
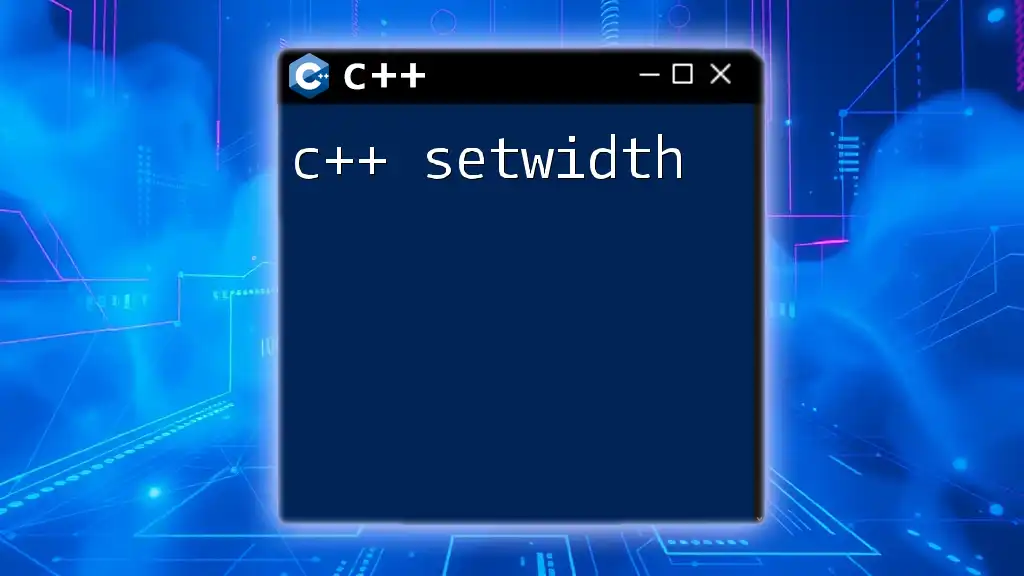
FAQs
What is the difference between CreateWindow and CreateWindowEx?
`CreateWindowEx` is an extended version of `CreateWindow`, allowing for more customizable window styles. It’s essential when you need features like layered windows or transparency.
Can I create a window without a message loop?
Creating a window without a message loop is technically possible, but it will not be interactive. The application won’t be able to respond to user inputs or various events.
How do I add controls (buttons, text boxes, etc.) to my window?
Adding controls to your window requires creating child windows using `CreateWindow` or `CreateWindowEx`, where the parent handle is set to the main window’s handle. You can specify the control type in the class name parameter.