The `windows.h` header file in C++ provides access to the Windows API, enabling developers to create applications that interact with the Windows operating system.
#include <windows.h>
int main() {
MessageBox(NULL, "Hello, World!", "Sample Message", MB_OK);
return 0;
}
Understanding Windows.h
What is Windows.h?
The Windows.h header file is a crucial component in C++ programming for Windows applications. It provides the necessary declarations for the Windows API, which allows developers to interact with the Windows operating system to manipulate windows, manage events, and perform system calls. Windows.h contains essential functions, data types, and macros used for creating GUI applications on the Windows platform.
Key Features of Windows.h
Windows.h serves as a bridge to access a wide range of functionality provided by the Windows API. Key features include system calls, message handling, and window management, which collectively enable developers to create rich and interactive desktop applications. A simple application setup can utilize these features to create and manage windows, handle user input, and respond to various system events efficiently.
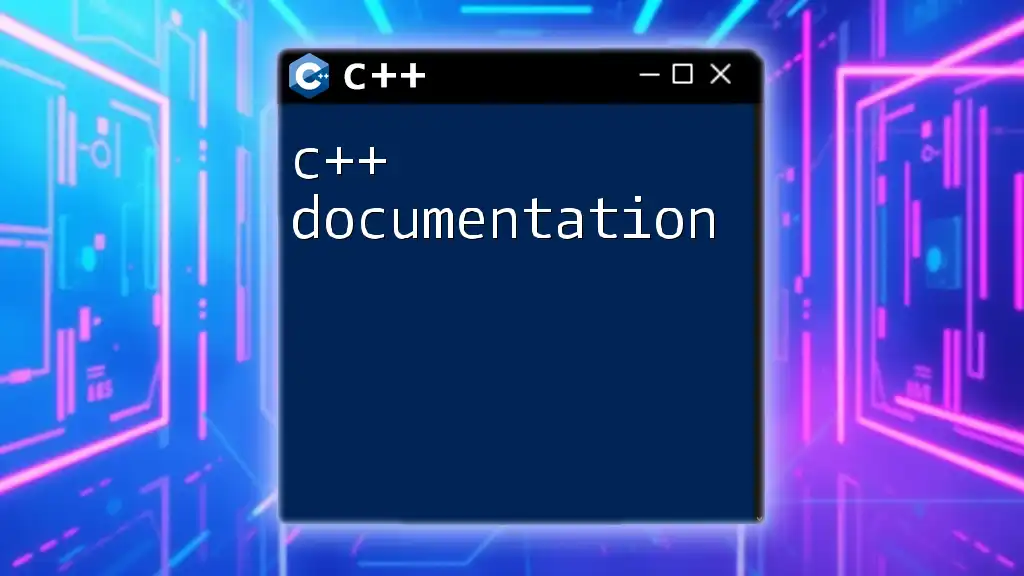
Setting Up Your Development Environment
Prerequisites
Before starting your Windows application development, ensure you have the necessary tools and software installed. Microsoft Visual Studio is the most commonly used IDE for Windows development, but alternatives like MinGW can also be used for compiling C++ programs. Installation processes typically involve downloading the IDE, setting up the appropriate C++ components, and ensuring that the Windows SDK is included.
Creating Your First Windows Application
To get started, create a basic Windows application. Here’s a step-by-step guide on how to set up a simple project using Windows.h:
- Open your IDE and create a new C++ project.
- Include the Windows.h header file at the beginning of your code.
- Implement the WinMain function to set up the application’s entry point.
Here’s a sample code snippet for a minimal Windows application that displays a message box:
#include <windows.h>
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow) {
MessageBox(NULL, "Hello, Windows!", "Welcome", MB_OK);
return 0;
}
This simple program will create a message box that greets the user upon execution.
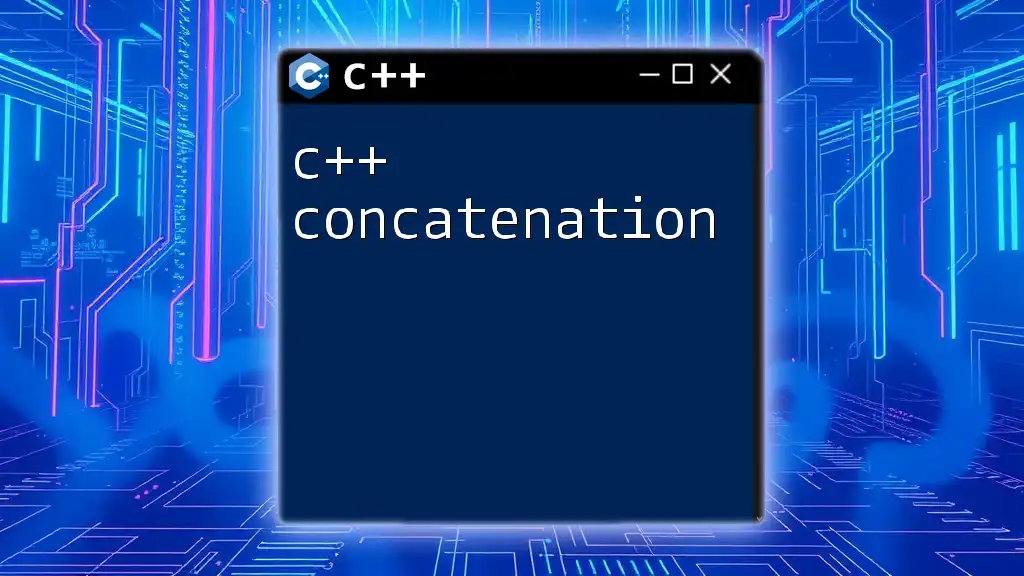
Core Components of Windows.h
Data Types in Windows.h
Understanding the data types defined in Windows.h is essential for effective programming. Common data types include:
- HINSTANCE: A handle for an instance of the application.
- HWND: A handle to a window, used extensively to manipulate Windows.
These types are fundamental when creating and interacting with Windows.
Functions in Windows.h
Windows.h provides an extensive array of functions. Some of the most important ones are:
- CreateWindow: For creating a new window.
- ShowWindow: For setting the window’s visibility.
- MessageBox: For displaying dialog boxes.
Here’s an example demonstrating how to create and show a window:
HWND hwnd = CreateWindowEx(
0,
"WindowClass",
"Sample Window",
WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT,
NULL, NULL, hInstance, NULL
);
ShowWindow(hwnd, nCmdShow);
As shown, CreateWindowEx enables customization of the window's styles and properties.
Windows Message Loop
The message loop is the heart of a Windows application, allowing it to process events such as keyboard input, mouse movements, and system commands. A proper message loop looks like this:
MSG msg;
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
The loop continuously retrieves messages from the queue and dispatches them to the appropriate window procedure, facilitating a responsive user interface.
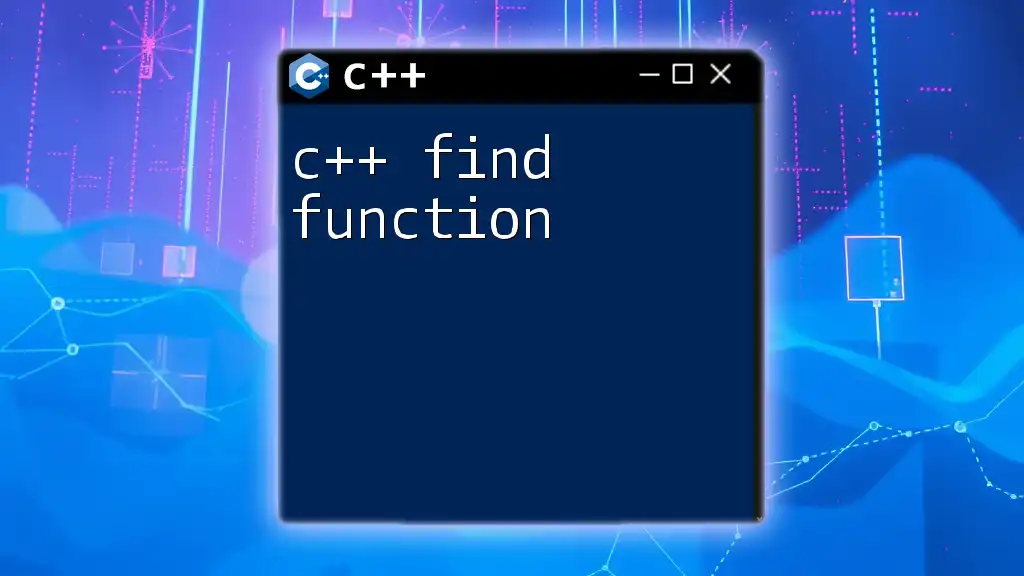
Handling Windows Messages
What are Windows Messages?
Windows messages are communication between the operating system and the application. Each window has a unique message queue, and messages are identified by constants like WM_PAINT, WM_DESTROY, etc. This message-driven architecture is vital for managing interactions within your application.
Writing a Message Procedure
A Window Procedure (WndProc) is a function that processes messages sent to a window. It’s essential for handling user inputs and system notifications. Here's how to define a basic WndProc function:
LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam) {
switch (msg) {
case WM_PAINT:
// Handle paint event
break;
case WM_DESTROY:
PostQuitMessage(0);
break;
default:
return DefWindowProc(hwnd, msg, wParam, lParam);
}
return 0;
}
In this example, the WndProc function responds to paint and destroy messages, ensuring the application can render graphics and exit gracefully.
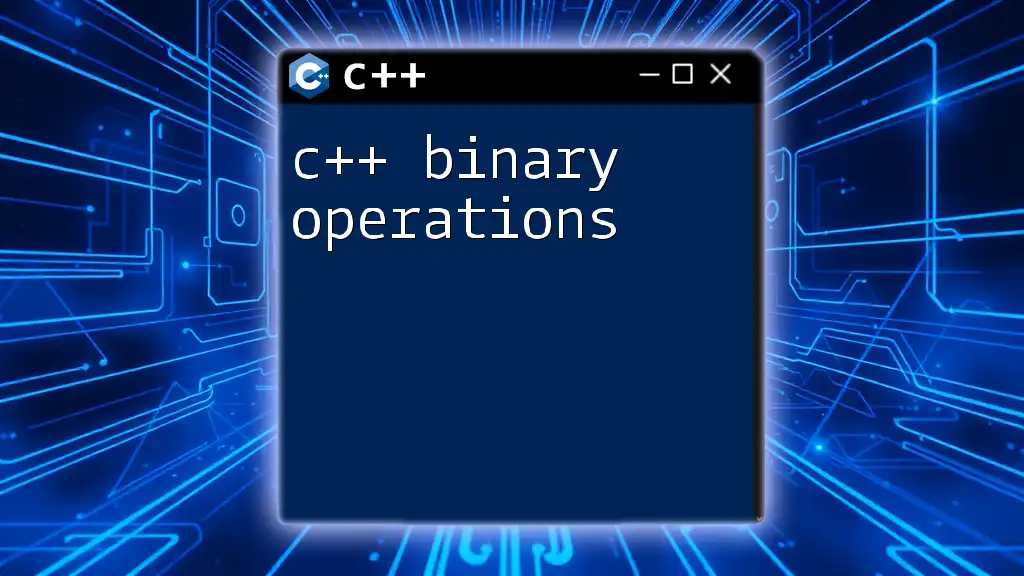
Advanced Topics
Working with Graphics
The Graphics Device Interface (GDI) in Windows.h allows for drawing graphics and text. GDI provides functions to render shapes, images, and text onto the application's window. A simple example is using BeginPaint and EndPaint in the WM_PAINT message to perform custom painting.
Multithreading with Windows.h
Multithreading is critical for creating responsive applications. CreateThread allows you to run multiple threads concurrently, improving performance. Here is a basic outline of how to create a new thread:
DWORD WINAPI ThreadFunction(LPVOID lpParam) {
// Thread-specific code
return 0;
}
// Creating a thread
HANDLE hThread = CreateThread(NULL, 0, ThreadFunction, NULL, 0, NULL);
Using threads helps manage complex tasks without freezing the GUI, but it's important to use synchronization techniques to avoid race conditions.
File and Path Management
Interacting with the file system in Windows is straightforward using functions defined in Windows.h. For example, the CreateFile function opens files for reading, writing, or both. Here’s how you can use it:
HANDLE hFile = CreateFile("example.txt", GENERIC_READ, 0, NULL, OPEN_EXISTING, FILE_ATTRIBUTE_NORMAL, NULL);
This snippet attempts to open a file and returns a handle that can be used to perform further file operations.
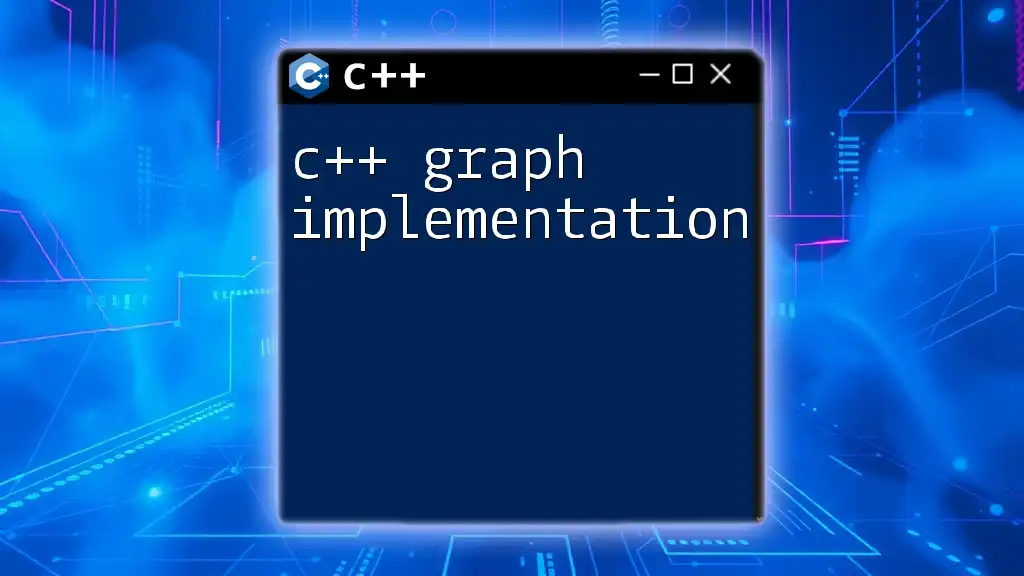
Best Practices for Using Windows.h
Code Organization
Effective code organization is vital for maintaining readability and manageability in your Windows applications. Keep related functions together, separate file handling logic, and manage resources appropriately. By structuring your code into modules, you can simplify debugging and updates.
Error Handling
Proper error handling in Windows applications enhances stability. Checking for invalid handles and using GetLastError can provide insights into what went wrong during API calls. For instance:
if (hFile == INVALID_HANDLE_VALUE) {
DWORD dwError = GetLastError();
// Handle error accordingly
}
Incorporating comprehensive error handling ensures your application can manage unexpected issues gracefully.
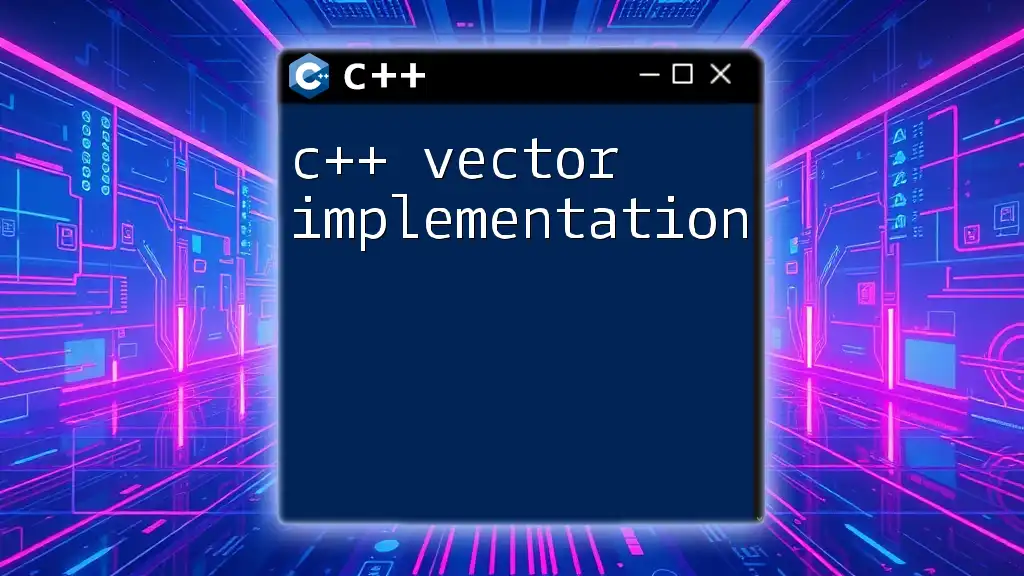
Conclusion
In this guide to C++ Windows.h documentation, we've explored the essentials of using Windows.h to build Windows applications. Understanding its data types, functions, and message handling mechanisms empowers developers to create interactive applications effectively. By following best practices, you can enhance the quality and maintainability of your code. Ready to dive deeper into programming? Join [Your Company Name] for more tutorials and resources!
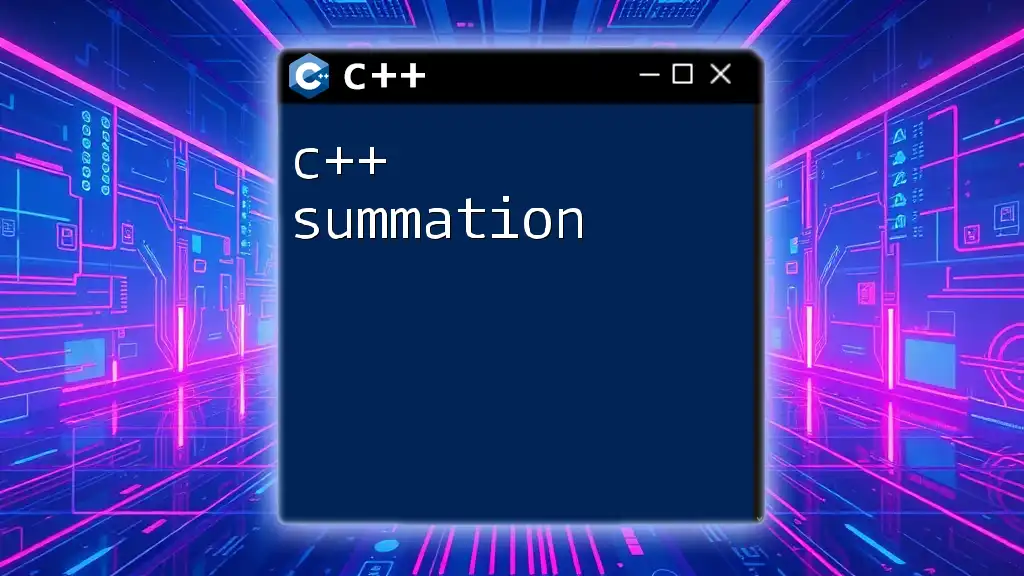
Additional Resources
To expand your knowledge further, consult the official Microsoft documentation on the Windows API, and consider reading recommended books on C++ programming and Windows development. Online courses can also provide structured learning paths tailored to your needs.