The C++ `next_permutation` function rearranges the elements in a range to the next lexicographical permutation, returning `true` if such a permutation exists or `false` if it is already the last permutation.
#include <algorithm>
#include <vector>
#include <iostream>
int main() {
std::vector<int> nums = {1, 2, 3};
std::next_permutation(nums.begin(), nums.end());
for (int num : nums) {
std::cout << num << " ";
}
return 0;
}
Understanding the Concept of Next Permutation
What is a Permutation?
Permutations are arrangements of a set of elements in different orders. For example, given the set {1, 2, 3}, the permutations include {1, 2, 3}, {1, 3, 2}, {2, 1, 3}, and so forth. Understanding permutations is crucial in programming, especially for combinatorial problems where the order of elements matters.
Definition of Next Permutation
The next permutation refers to the lexicographically next greater permutation of a sequence of numbers. In simpler terms, it means finding the "next" arrangement of the digits or elements that would come immediately after the current arrangement in an ordered list. If no such arrangement exists (i.e., the sequence is in descending order), the next permutation will be the lowest possible order (ascending).
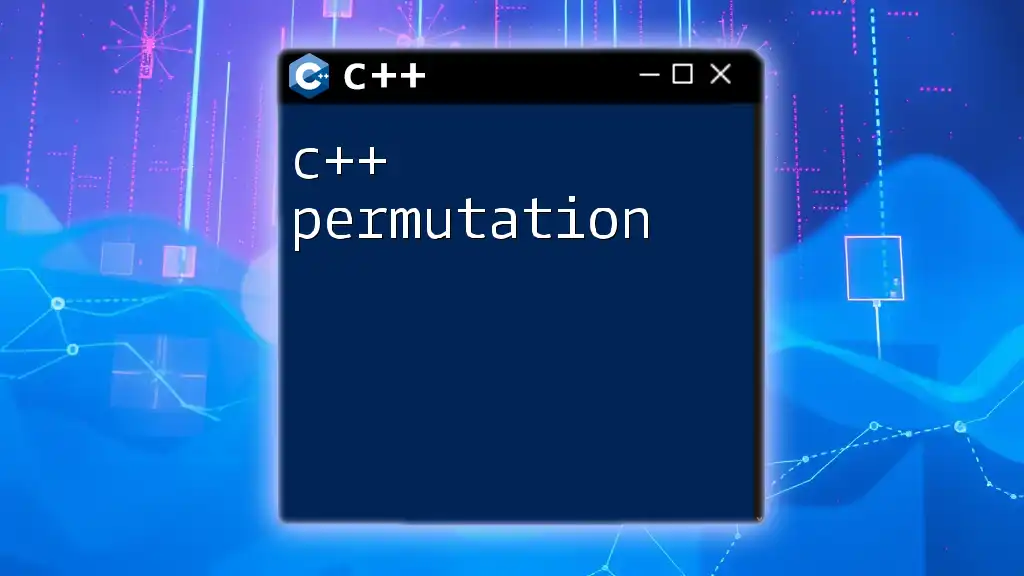
Why Learn Next Permutation in C++
Real-life Applications
The concept of permutations plays a vital role in solving various problems in computer science and mathematics. For instance, it can be applied in fields like:
- Game development: Determining possible scenarios or moves.
- Cryptography: Understanding potential key combinations.
- Optimization problems: Finding the best arrangement in resource allocation.
Optimization and Efficiency
Learning how to efficiently compute the next permutation is essential because simple brute-force methods can become computationally expensive, especially for larger datasets. Using the `next_permutation` function allows developers to avoid unnecessary calculations and get results more rapidly.
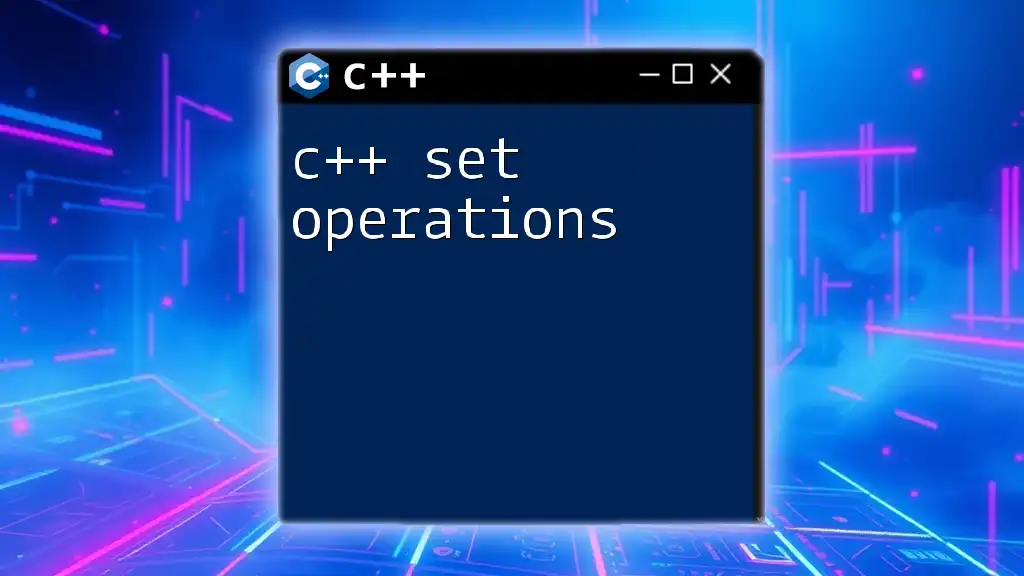
The Standard Template Library (STL) and Next Permutation
Overview of the Standard Template Library
The Standard Template Library (STL) in C++ provides various data structures and algorithms to facilitate programming tasks. It includes functions that streamline operations for manipulating data, especially when it comes to permutations.
Using the `next_permutation` Function in C++
The `next_permutation` function from the STL simplifies the task of obtaining the next permutation.
Syntax:
bool next_permutation(BidirectionalIterator first, BidirectionalIterator last);
To use this function, you need to include the necessary header:
#include <algorithm>
#include <vector>
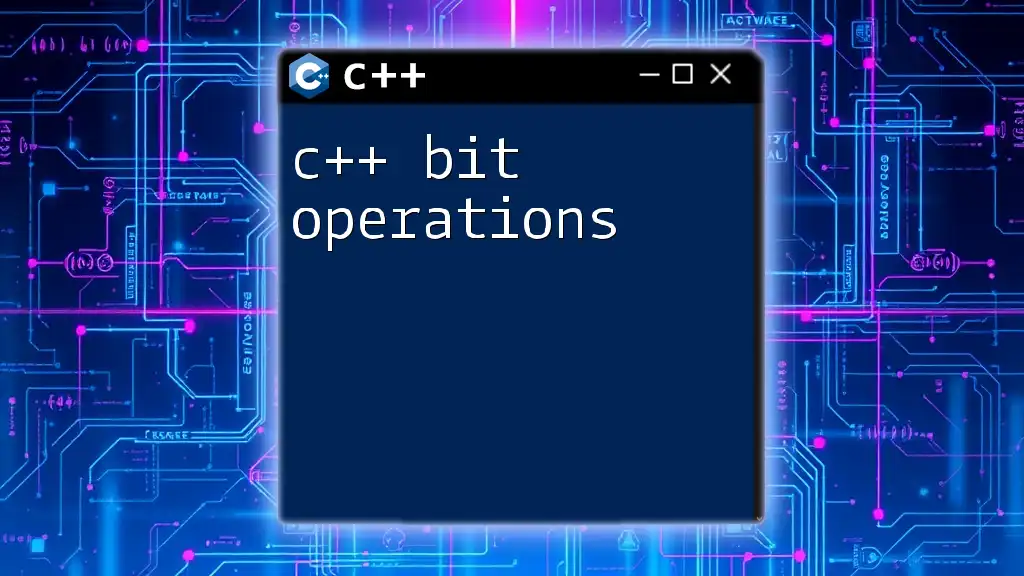
How to Use `next_permutation` in C++
Basic Syntax and Parameters
The `next_permutation` function takes two parameters: the beginning and end of the sequence. It rearranges the elements into the next lexicographical permutation. If such a permutation does not exist, it rearranges the elements into the smallest permutation (sorted order).
Consider the following code snippet demonstrating basic usage:
std::vector<int> nums = {1, 2, 3};
std::next_permutation(nums.begin(), nums.end());
Here, the vector `nums` will be rearranged to its next permutation.
Step-by-Step Explanation of the Algorithm
The algorithm for finding the next permutation consists of three steps:
-
Find the rightmost ascent: Search through the sequence from the back to find the first pair of elements that are in increasing order. This identifies the pivot point where the order needs to be altered.
-
Locate the successor to the pivot: Once the pivot is identified, find the smallest element on the right side of the pivot that is larger than the pivot itself. This element will be swapped with the pivot to form the next permutation.
-
Swap and reverse: Swap the identified pivot with its successor. Then, reverse the order of the elements to the right of the pivot to ensure the next permutation is the smallest possible arrangement.
Illustrative Example: Let's say we have the following array: `{1, 2, 3}`.
- The rightmost ascent is `2` and `3`.
- The smallest element larger than `2` on its right side is `3`, so we swap these two, resulting in `{1, 3, 2}`.
- Since we’re now finished with the left part of the array, we simply sort the right side (or reverse, as it’s already in descending order). The result is the next permutation.
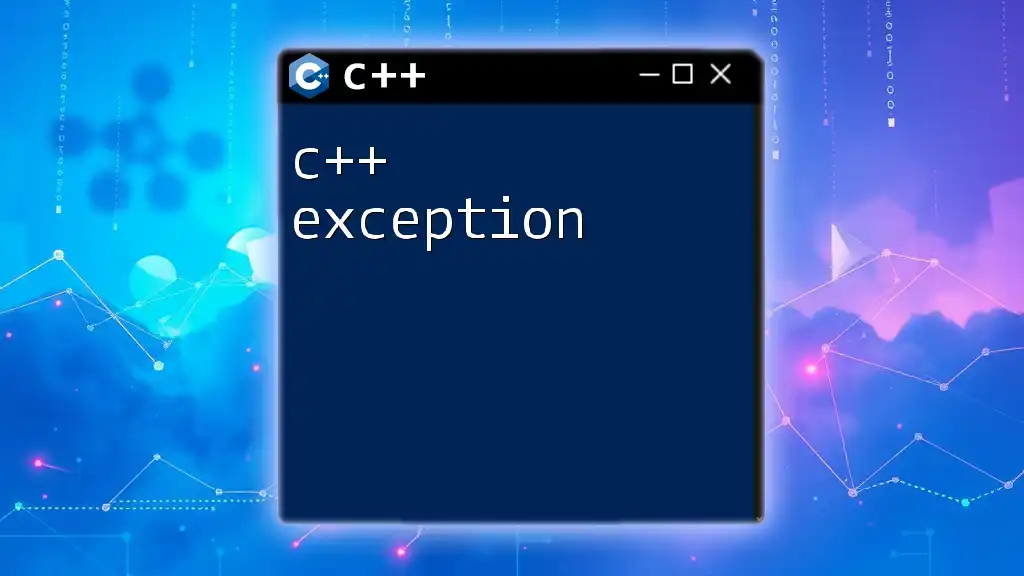
Detailed Breakdown of the Next Permutation Algorithm
Visualizing the Steps
Visual aids can significantly enhance understanding, but for now, let's elaborate on the steps with comments in C++ code.
std::vector<int> nums = {1, 2, 3};
// Step 1: Find the first decreasing element (pivot)
auto it = std::find_if(nums.rbegin(), nums.rend() - 1, std::greater<int>());
if (it != nums.rend() - 1) {
// Step 2: Find the successor
auto next = std::find_if(nums.rbegin(), it + 1, std::greater<int>());
// Step 3: Swap them
std::iter_swap(it.base() - 1, next.base() - 1);
// Reverse the order after pivot
std::reverse(it.base(), nums.end());
}
In this code, we maintain clarity through comments, ensuring that each part is understood without visual aids.
Edge Cases
When using c++ next permutation, it can be useful to recognize edge cases. For instance, if the input vector is sorted in descending order (e.g., `{3, 2, 1}`), `next_permutation` will rearrange it to ascending order `{1, 2, 3}`, signaling that there are no higher permutations available.
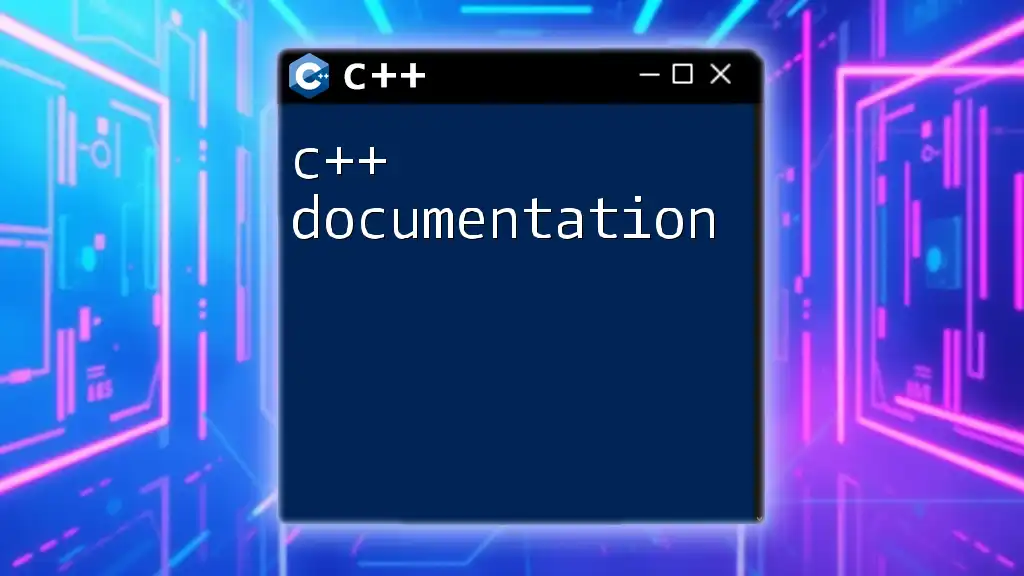
Practical Examples
Example 1: Generating Permutations of a Set of Integers
Here’s how you can generate all permutations of a set using `next_permutation` in a loop:
std::vector<int> nums = {1, 2, 3};
do {
// Print current permutation
for (int n : nums) std::cout << n << " ";
std::cout << std::endl;
} while (std::next_permutation(nums.begin(), nums.end()));
This code will output all permutations in lexicographic order. Each time `next_permutation` is called, it rearranges `nums` to the next possible arrangement until no more arrangements are left.
Example 2: Using Next Permutation with Strings
The `next_permutation` function is not limited to integers. It can also be applied to strings, enhancing its versatility in managing different datasets.
std::string s = "abc";
std::next_permutation(s.begin(), s.end());
std::cout << s; // Output will be "acb"
In this example, the string "abc" is transformed into its next lexicographic arrangement, demonstrating that `next_permutation` works uniformly across various data types.
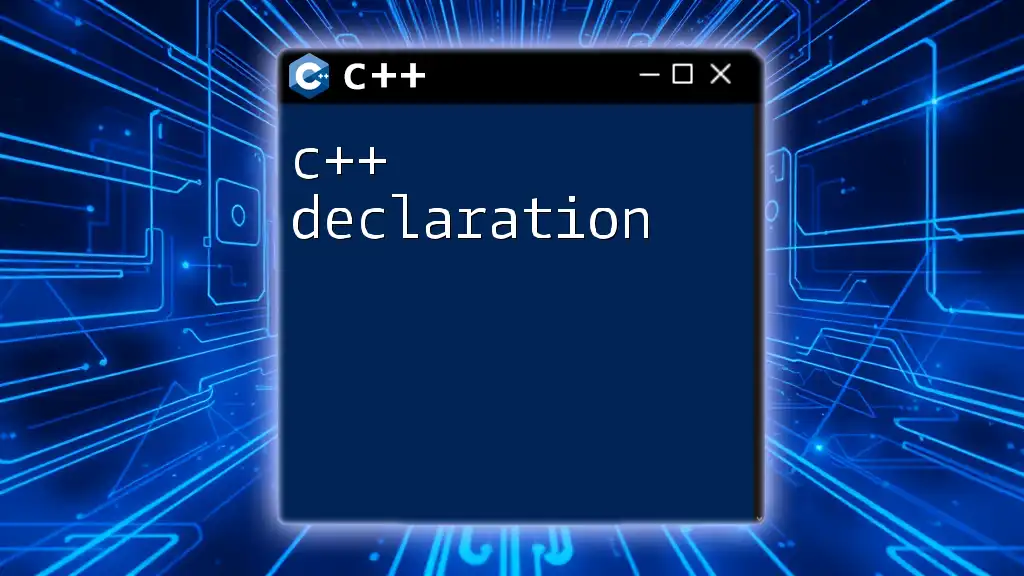
Common Pitfalls and Troubleshooting
Understanding Limitations
Despite its utility, `next_permutation` may lead to erroneous results if used incorrectly, such as failing to sort data beforehand. Not recognizing that it operates on a mutable array can potentially yield unexpected behavior.
Best Practices
To utilize `next_permutation` effectively:
- Always ensure your data is properly organized.
- Be mindful of the edge cases mentioned.
- Use meaningful variable names and sufficient comments in your code for better readability.
Following these practices will minimize errors and help you leverage the power of this function efficiently.
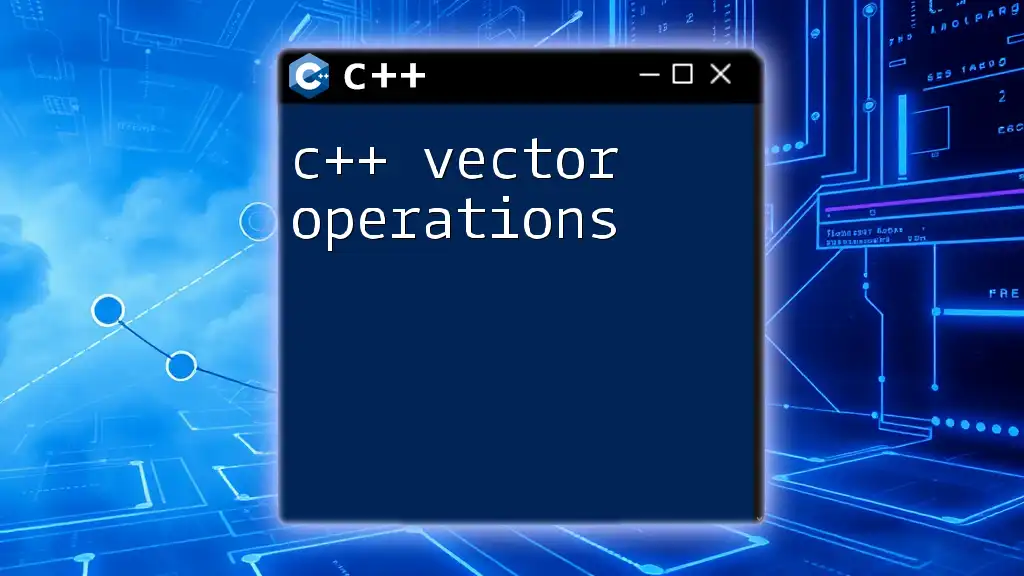
Conclusion
Understanding c++ next permutation not only enhances your programming skills but also offers a deep dive into combinatorial problems. Effectively using the `next_permutation` function can save time and effort through efficient algorithm design.
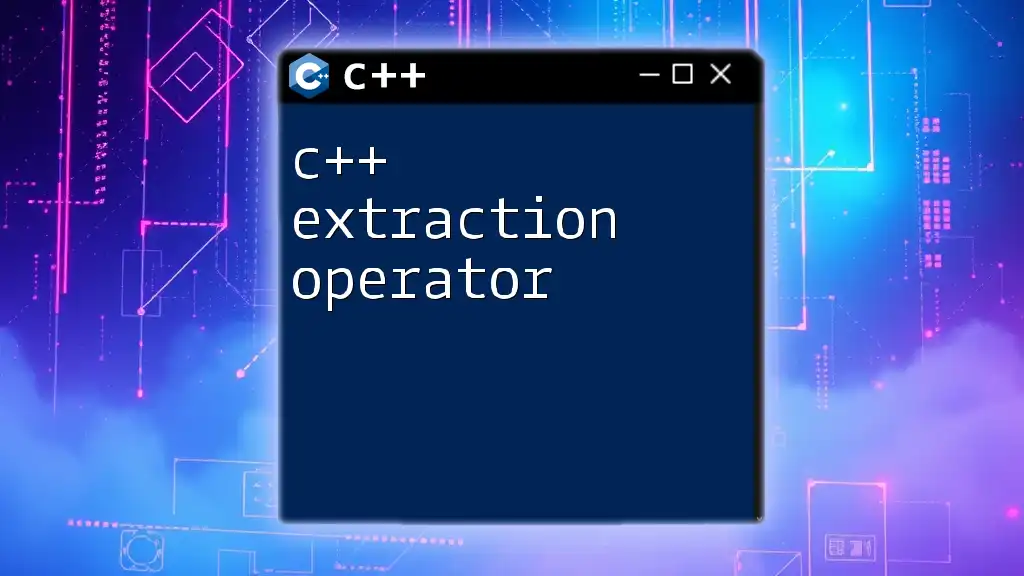
Additional Resources
For further reading, it's recommended to explore:
- The official C++ documentation on `std::next_permutation`.
- Books on algorithms that cover permutations, combinations, and their applications in programming.
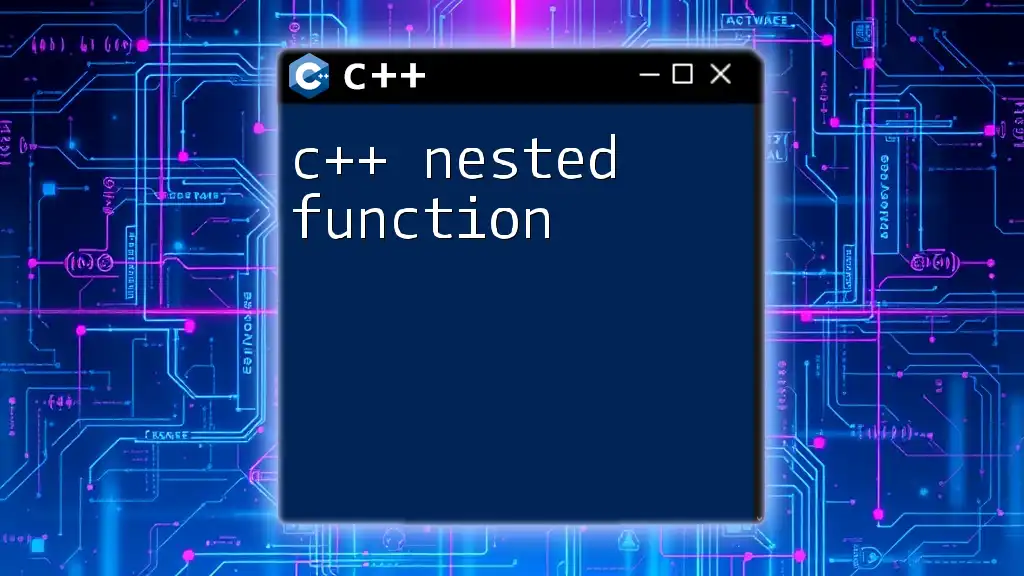
Call to Action
Take the next step in your C++ journey by experimenting with `next permutation` in various scenarios. Stay connected and subscribe to our platform for concise programming tutorials tailored just for you!
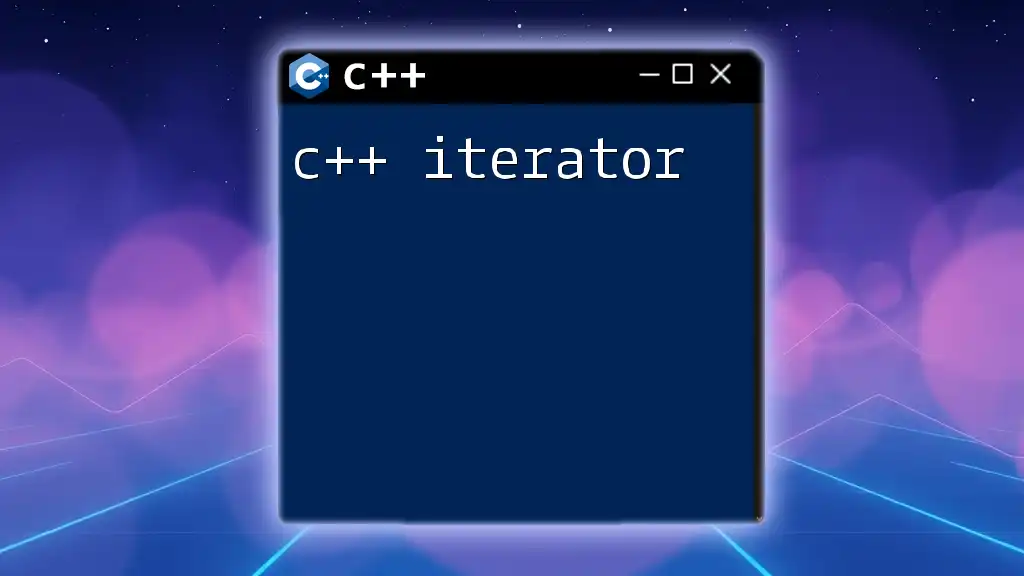
FAQs
-
What happens when there are no available next permutations? If the current arrangement is the highest possible (i.e., sorted in decreasing order), `next_permutation` will reset the arrangement to the lowest order (sorted in increasing order).
-
Can I use `next_permutation` with custom data types? Yes, as long as you provide a comparison operator for your custom type, `next_permutation` can be utilized effectively.
By mastering the `next_permutation` function, you're equipping yourself with a powerful tool in your C++ arsenal, ready to tackle an array of combinatorial problems with confidence!