In C++, a permutation refers to a specific arrangement of a set of elements, and you can generate all permutations of a given collection using the `std::next_permutation` function from the `<algorithm>` header.
Here’s a simple code snippet demonstrating how to use `std::next_permutation`:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> arr = {1, 2, 3};
std::sort(arr.begin(), arr.end()); // Ensure the vector is sorted
do {
for (int num : arr) {
std::cout << num << " ";
}
std::cout << std::endl;
} while (std::next_permutation(arr.begin(), arr.end()));
return 0;
}
What is a Permutation?
A permutation refers to an arrangement of all or part of a set of objects in a specific order. In mathematics, when dealing with a collection of items, we often want to determine how these items can be ordered, which is significant not only in theoretical contexts but also in practical programming scenarios.
The importance of permutations extends into various fields such as computer science, cryptography, and operations research, where the ability to quickly generate and analyze the arrangement of data can lead to efficient solutions to complex problems.
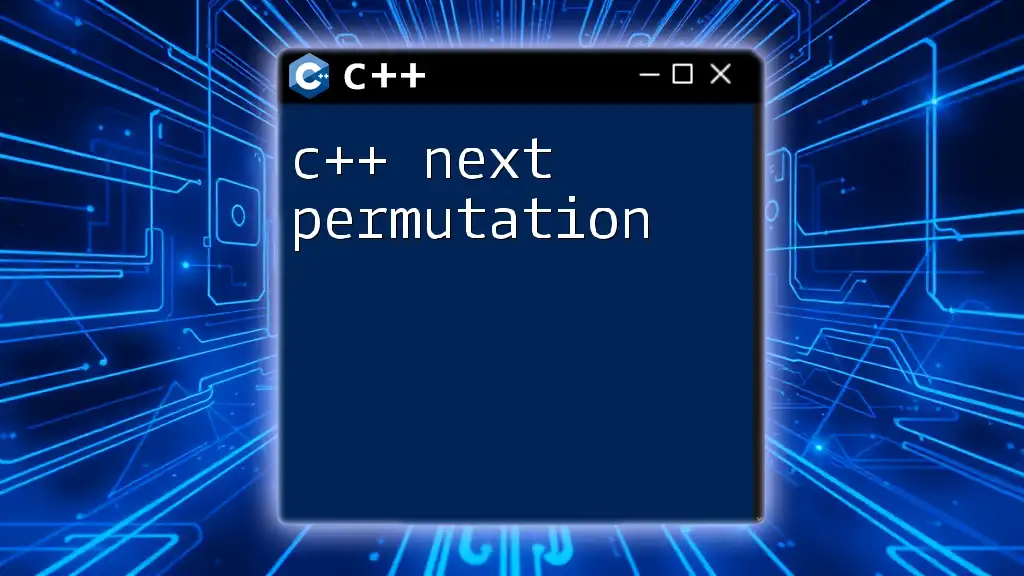
Understanding C++ Permutation
Key Concepts and Terminology
When discussing permutations in C++, it’s crucial to grasp a few basic terms:
- Factorial: The factorial of a non-negative integer \( n! \) is the product of all positive integers less than or equal to \( n \). It's a key component in calculating the number of ways to arrange \( n \) objects.
- nPr (Permutations of n items taken r at a time): This formula provides the number of ways to choose \( r \) items from \( n \) without regard to the order. It's expressed as:
\[ nPr = \frac{n!}{(n-r)!} \]
Understanding these terms provides a solid foundation for studying permutations in C++.
C++ Standard Libraries for Permutations
C++ offers a powerful Standard Template Library (STL) that includes functions useful for generating permutations. The crucial component is the `<algorithm>` header, which contains specialized algorithms to handle permutations efficiently.
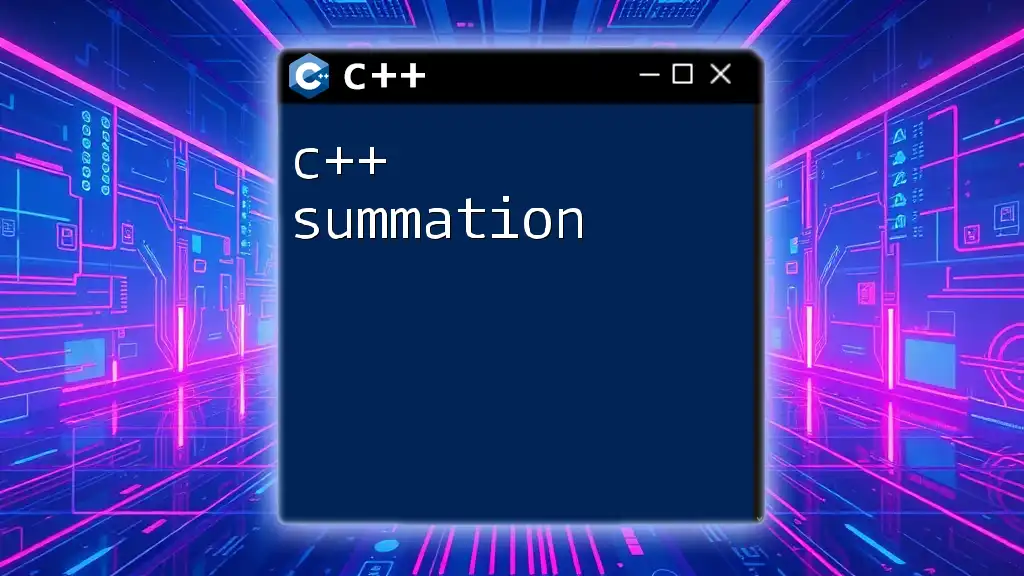
Generating Permutations in C++
Permutation using `std::next_permutation`
The C++ function `std::next_permutation` generates the next lexicographical permutation of a sequence. When applied to a series of numbers, it rearranges them into the next greater permutation. If no such permutation exists (i.e., the sequence is in descending order), the function rearranges the sequence to its lowest possible order.
How `std::next_permutation` Works
To use `std::next_permutation`, you need to invoke it on a range defined by two iterators, usually the beginning and end of a container. Here's an illustrative example:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 3};
// Generate next permutation
do {
for (int i : vec) std::cout << i << " ";
std::cout << std::endl;
} while (std::next_permutation(vec.begin(), vec.end()));
return 0;
}
In this example, the vector `{1, 2, 3}` is passed to `std::next_permutation`, generating and printing each subsequent permutation until all arrangements have been displayed.
Custom Permutation Function
While `std::next_permutation` is powerful, there may be scenarios where a custom function is warranted, particularly when you want to control the logic or format of permutation generation.
Example of a Recursive Permutation Function
A recursive function can explore every possibility by swapping elements. Here’s how you might implement it:
#include <iostream>
#include <vector>
#include <algorithm>
void permute(std::vector<int>& arr, int start) {
if (start >= arr.size()) {
for (int n : arr) std::cout << n << " ";
std::cout << std::endl;
return;
}
for (int i = start; i < arr.size(); i++) {
std::swap(arr[start], arr[i]);
permute(arr, start + 1);
std::swap(arr[start], arr[i]); // backtrack
}
}
int main() {
std::vector<int> data = {1, 2, 3};
permute(data, 0);
return 0;
}
In this code, `permute` recursively generates permutations by swapping elements and exploring further down the array. The backtracking step ensures all possible arrangements are considered.
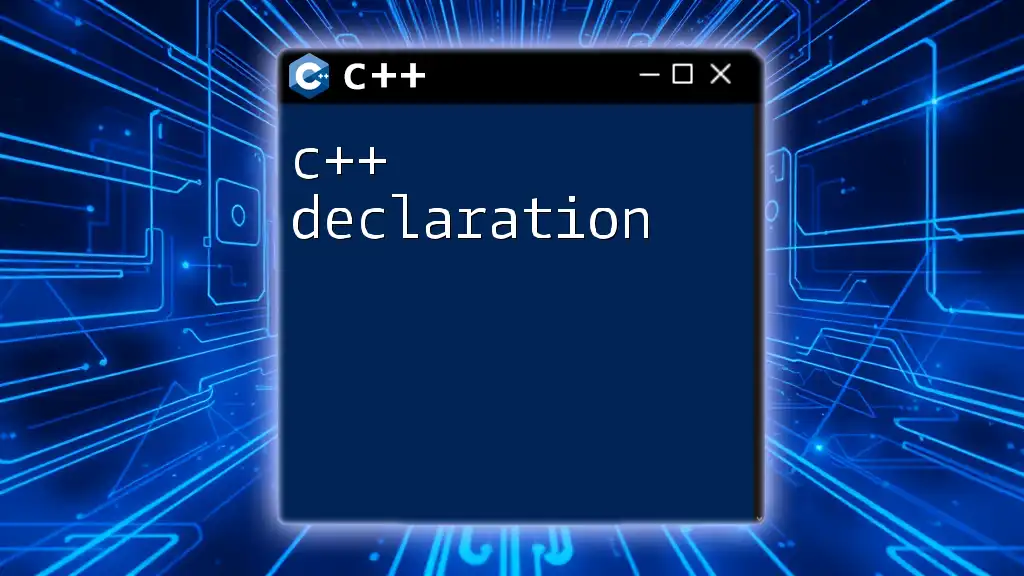
Using Permutations in Problem Solving
Case Study: Solving Combinatorial Problems
Permutations are often employed in combinatorial problems where the order of arrangement matters. A classic example is the Traveling Salesman Problem, where the goal is to determine the shortest possible route visiting a set of cities and returning to the origin.
By generating all possible permutations of city visits, you can evaluate each route’s total distance and determine the most efficient path.
Performance Considerations
Time Complexity of Permutations
The fundamental time complexity associated with generating permutations is \( O(n!) \) due to the factorial growth of possible arrangements as \( n \) increases. This complexity highlights the challenges posed by larger data sets and emphasizes the need for efficient algorithms when working with permutations in practical applications.
When to use permutations efficiently depends on the problem context—generating all possible arrangements may be feasible for small collections but should be avoided for larger sets unless necessary.
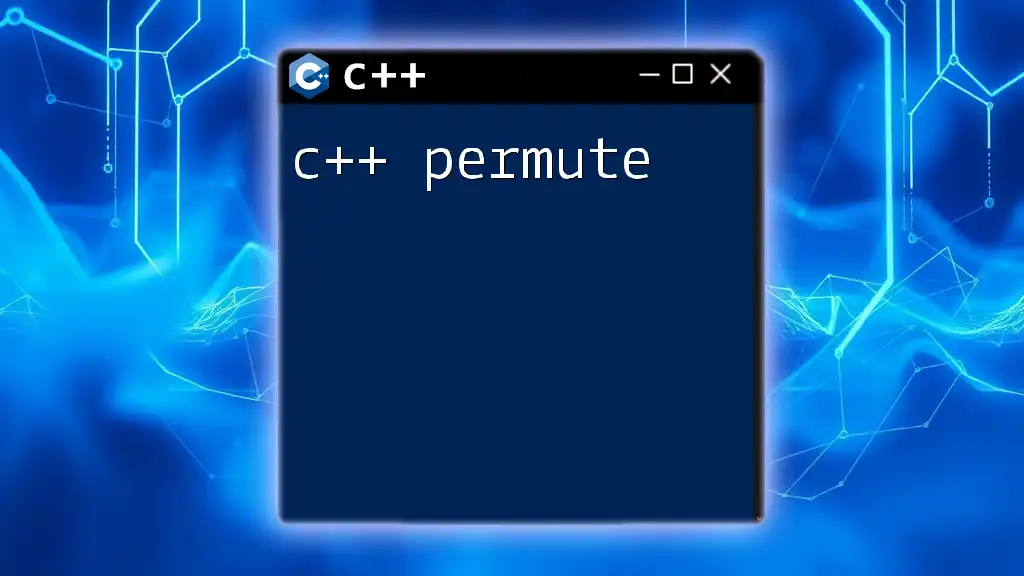
Advanced Topics in C++ Permutation
Handling Duplicates in Permutations
Problem Definition
When the input data contains duplicate elements, generating unique permutations becomes essential. The naive approach might generate redundant arrangements, inflating the output unnecessarily.
Implementation Strategy
To manage duplicates, you can utilize a set to store elements that have already been included in the current permutation.
#include <iostream>
#include <vector>
#include <algorithm>
#include <set>
void permuteUnique(std::vector<int>& nums, int start) {
if (start >= nums.size()) {
for (int num : nums) std::cout << num << " ";
std::cout << std::endl;
return;
}
std::set<int> seen;
for (int i = start; i < nums.size(); i++) {
if (seen.insert(nums[i]).second) { // only proceed if this element was not used
std::swap(nums[start], nums[i]);
permuteUnique(nums, start + 1);
std::swap(nums[start], nums[i]); // backtrack
}
}
}
int main() {
std::vector<int> data = {1, 1, 2};
permuteUnique(data, 0);
return 0;
}
In this implementation, the use of a set prevents the function from considering the same element in the same recursion depth, ensuring that only unique permutations are printed.
Optimizing Permutation Generation
For larger sets of input, performance optimization is crucial. Approaches may include using iterative methods instead of recursion to avoid stack overflow issues and to enhance performance. Moreover, leveraging algorithms such as Heap's algorithm can also facilitate efficient permutation generation.
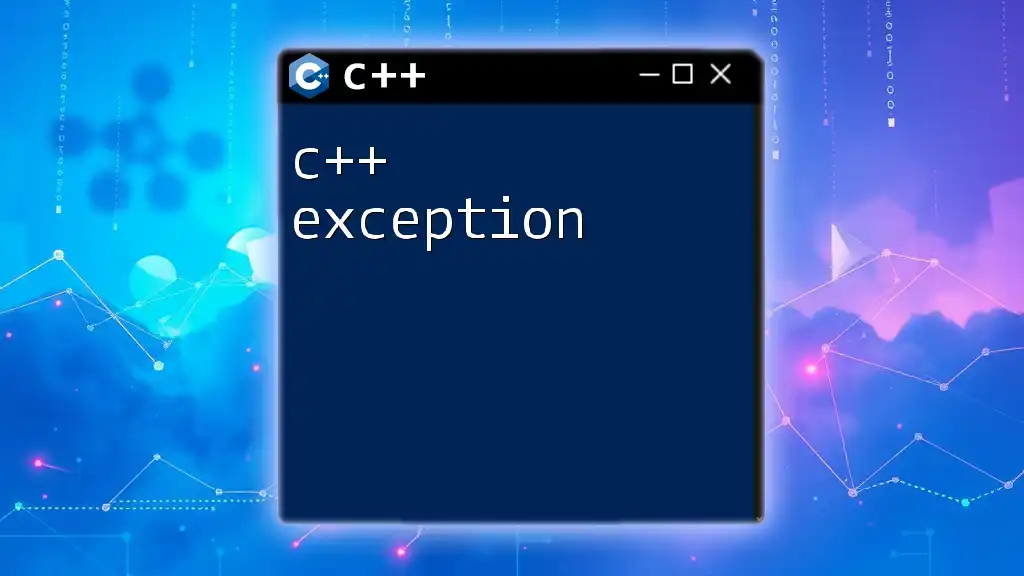
Conclusion
In sorting and ordering collections, C++ permutations provide invaluable tools for developers and data analysts alike. Understanding key concepts, employing libraries, and sometimes implementing custom solutions enable a comprehensive grasp of how to work with permutations effectively.
Encouraging hands-on practice with the provided examples and challenging problems can lead to deeper mastery of permutations in C++. Exploring these topics further will bolster one’s programming skillset and enhance problem-solving prowess in various applications.
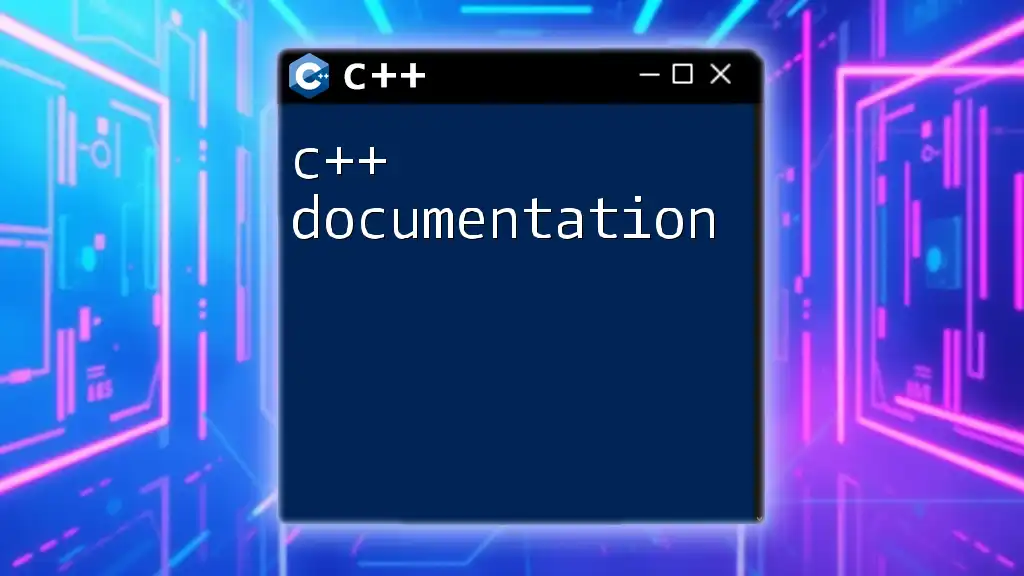
Additional Resources
For those interested in delving deeper into C++ permutations, recommended readings include experienced texts on algorithmic design and the workings of the STL. Additionally, exploring advanced C++ topics such as combinatorial algorithms and data structure optimization will be beneficial in broadening your programming knowledge.