In C++, the remainder of a division operation can be calculated using the modulo operator `%`, which returns the remainder when one integer is divided by another.
Here’s a simple code snippet demonstrating its use:
#include <iostream>
int main() {
int dividend = 10;
int divisor = 3;
int remainder = dividend % divisor;
std::cout << "The remainder of " << dividend << " divided by " << divisor << " is " << remainder << std::endl;
return 0;
}
Understanding Remainder
The concept of a remainder emerges from basic arithmetic, specifically when we divide one integer by another. In simple terms, the remainder is what is left after the division has occurred. For example, if you divide 5 by 2, the quotient is 2, and the remainder is 1 (because 5 = 2 × 2 + 1).
In programming, especially in C++, the remainder operation is crucial for numerous applications, such as conditional logic, looping, and algorithm development. The C++ language provides an operator specifically for this purpose, known as the modulus operator.
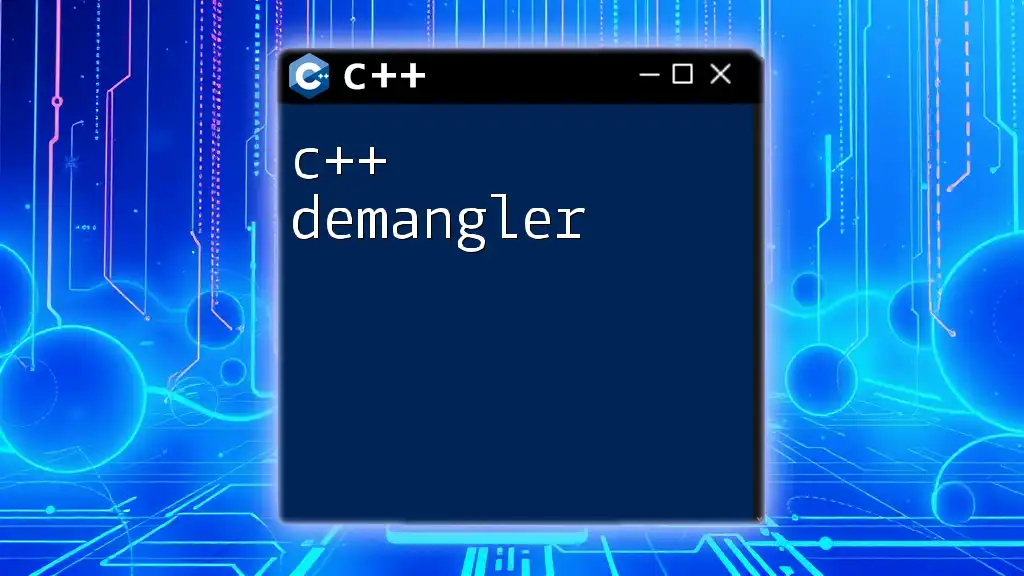
The Remainder Operator (%)
What is the Remainder Operator?
In C++, the modulus operator is represented by the symbol %. It yields the remainder resulting from the integer division of one number by another. When used in a C++ expression, the syntax is straightforward:
int result = a % b;
Here, the value of `result` will contain the remainder when `a` is divided by `b`.
How the Remainder Works
To truly understand how the modulus operator works, let’s consider a few straightforward examples:
-
Example 1: `5 % 2`
Here, 5 divided by 2 gives a quotient of 2 with a remainder of 1. Thus:
int remainder1 = 5 % 2; // remainder1 will be 1
-
Example 2: `10 % 4`
In this case, 10 divided by 4 results in a quotient of 2 with a remainder of 2. Therefore:
int remainder2 = 10 % 4; // remainder2 will be 2
Common Use Cases
The modulus operator plays a key role in several practical programming scenarios, including checking for even or odd numbers. Here’s how you can implement that in C++:
if (number % 2 == 0)
std::cout << "Even";
else
std::cout << "Odd";
In the example above, the number is checked for evenness by utilizing `number % 2`. If the result is 0, the number is even; if it's 1, the number is odd.
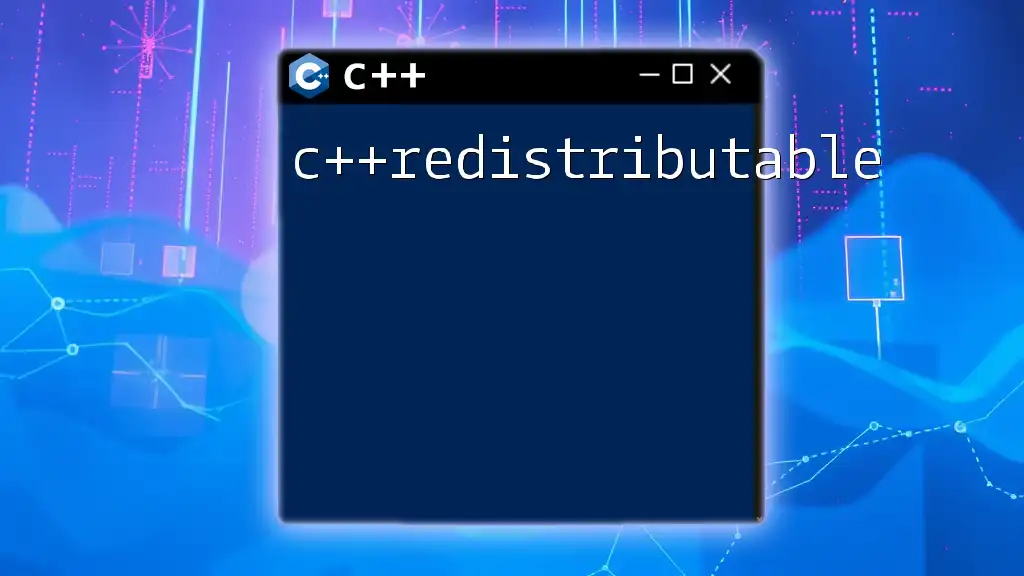
Advanced Use Cases
Practical Applications
Beyond simple checks, the remainder operator can be crucial in more complex functionality. For instance, using it in loops to create cyclic behavior can be very effective.
Consider implementing a circular queue:
int queue[5];
int front = 0, rear = 0;
rear = (rear + 1) % 5; // Circular movement
In this code, the queue size is effectively limited to 5, and the rear index wraps around to the beginning of the array when it reaches the end, thanks to the modulus operation.
Understanding Negative Remainders
A notable nuance in C++ is how it handles negative numbers. For example:
int remainder = -5 % 3; // remainder will be -2
In this case, the remainder is negative, which might differ from the expectations of those familiar with other programming languages. Understanding this behavior is critical for avoiding confusion in your programs.
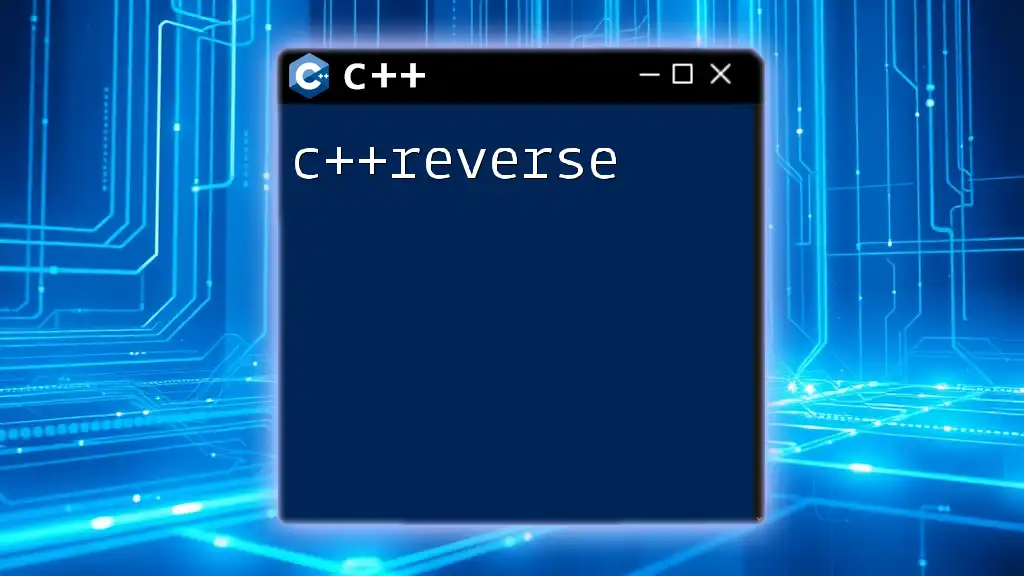
Combining Remainders with Other Data Types
Sometimes, you may need to work with floating-point numbers. In C++, the standard modulus operator does not work directly with them, but you can use the fmod function from the `<cmath>` library, which provides the same concept for floats:
double remainder = fmod(5.5, 1.0);
This would yield a remainder of 0.5. Using the appropriate function ensures accuracy when dealing with different data types.
Remainders in User-Defined Functions
Creating your own functions that use the remainder concept can streamline your code and improve readability. Here’s a simple example of a function that calculates the remainder:
int calculateRemainder(int dividend, int divisor) {
return dividend % divisor;
}
This function takes two integer inputs and returns their remainder, demonstrating the reusability and encapsulation of the modulus operation.
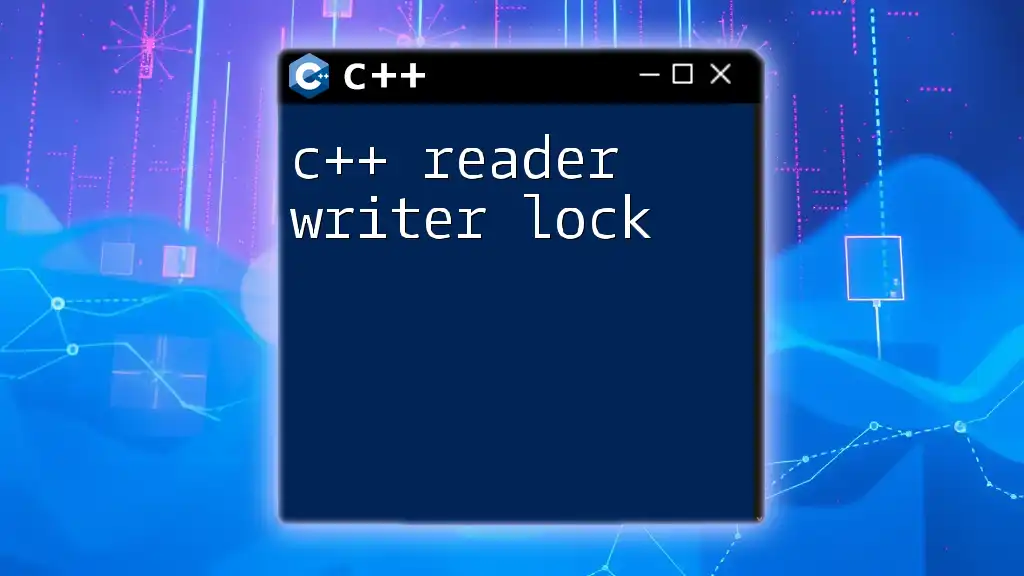
Best Practices
When using the remainder operator, keeping a few best practices in mind can help you avoid common pitfalls:
- Always consider edge cases, such as what happens when the divisor is zero. In C++, dividing by zero will throw a runtime error.
- Use descriptive variable names to make your code transparent. This will help others (and future you) understand your logic at a glance.
- Be mindful of integer versus floating-point division. Always verify what type of data you are working with and ensure that functions like `fmod` are used appropriately for floating-point numbers.
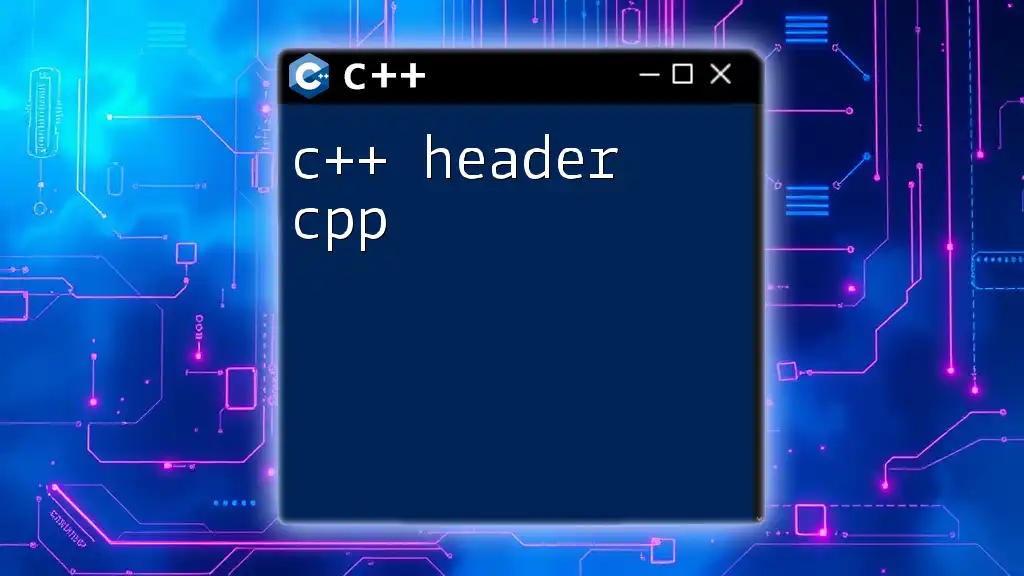
Conclusion
The C++ remainder operator is a fundamental tool that, when properly understood and applied, can enhance your programming skill set. By mastering its uses, from basic checks to more complex applications like circular queues, you can write more efficient code. Practice with these concepts will deepen your understanding and proficiency in C++.
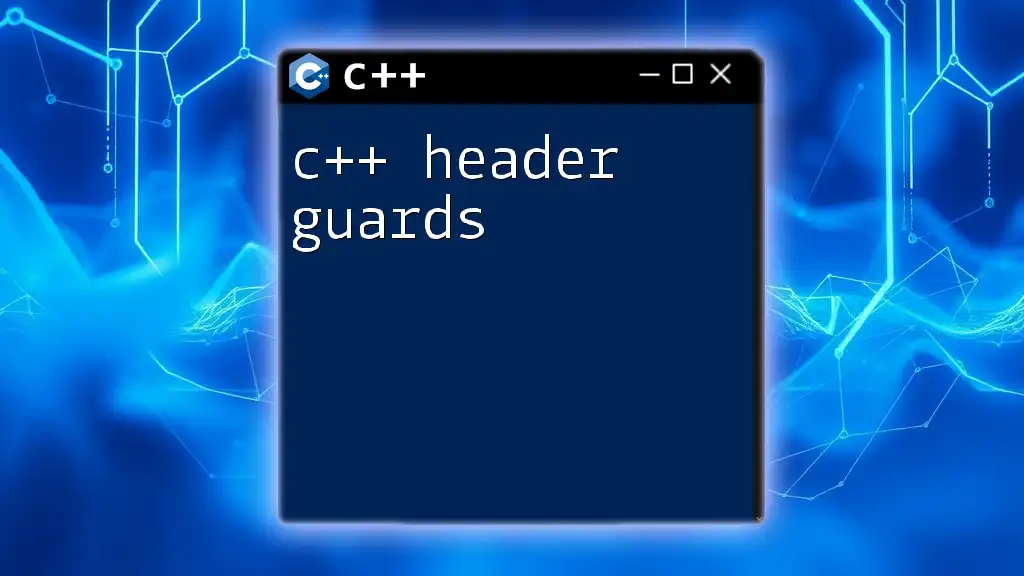
Additional Resources
To further your learning, consider exploring online C++ compilers for hands-on exercises. The official C++ documentation provides valuable insights into operator usage, and various tutorials will help reinforce these concepts. Additionally, reading recommended books on C++ programming can deepen your expertise in the language.