In C++, a header file is used to declare the functions, classes, and variables that can be shared between multiple source files, promoting modularity and reusability in programming.
// example_header.h
#ifndef EXAMPLE_HEADER_H
#define EXAMPLE_HEADER_H
void exampleFunction();
#endif // EXAMPLE_HEADER_H
Introduction to C++ Headers
What is a Header File?
A header file in C++ is a file that usually has a `.h` or `.hpp` extension, designed to contain declarations of functions, classes, variables, and constants. These files serve as an interface, allowing code in different source files to communicate with each other. They play an essential role in organizing code, promoting reusability, and facilitating modular programming.
Why Use Header Files?
The use of header files fosters better organization of code. By encapsulating declarations in a separate file, developers can keep the implementation details out of the main file. This not only enhances readability but also allows programmers to reuse the declared components across multiple files without duplication, making future maintenance significantly easier.
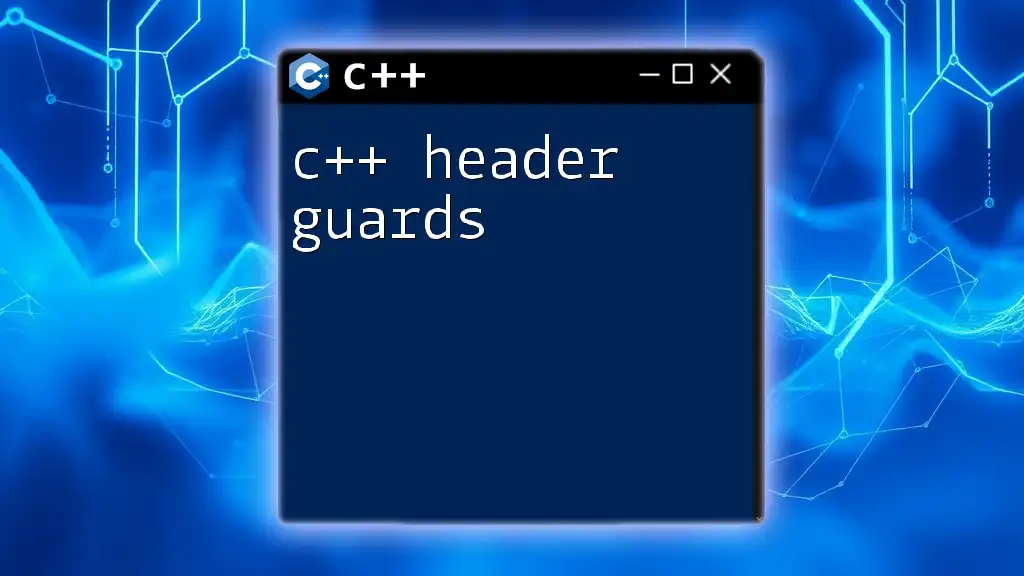
Structure of a C++ Header File
Basic Syntax of a Header File
Every header file needs a structure that ensures it is included only once in a compilation process. This prevents errors related to multiple definitions. The conventional way to do this involves using include guards. Here's a typical setup:
#ifndef HEADER_NAME_H
#define HEADER_NAME_H
// Declarations and definitions
#endif // HEADER_NAME_H
This snippet checks if `HEADER_NAME_H` is defined. If it’s not, it defines it and includes the content of the file. If the file is included multiple times, the guard prevents the contents from being processed more than once.
Common Components of a Header File
Header files often contain:
- Function declarations: These inform the compiler about functions defined in other files without needing their complete implementation.
- Class definitions: They provide the structure for objects, including members and methods.
- Constants and macros: Useful for defining values and reusable code snippets globally.
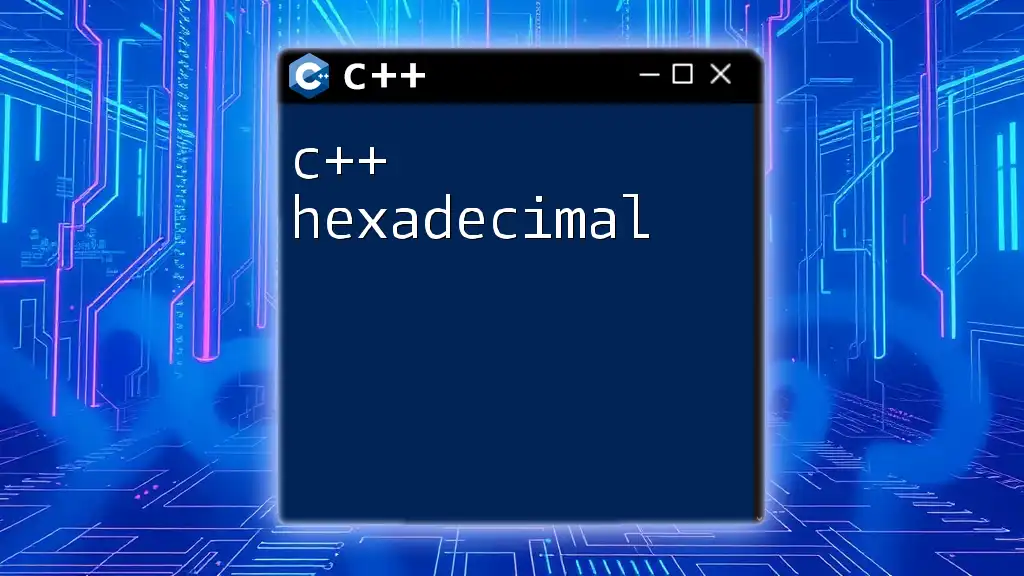
Creating and Organizing Header Files
When to Create a Header File?
Creating a header file becomes beneficial when a function or class needs to be accessed by multiple source files. Generally, if you find yourself duplicating declarations, that’s a sign that a header file would improve your code structure.
Best Practices for Header Files
To ensure maintainability and clarity in your projects, consider these best practices:
- Use clear and descriptive naming conventions for header files, such as `Calculator.h` rather than `file1.h`.
- Apply a modular design where each header file corresponds to a specific component or module of the application, preventing clutter and confusion.
- Minimize dependencies between headers. If possible, include only what is necessary to reduce compilation times and interdependencies.
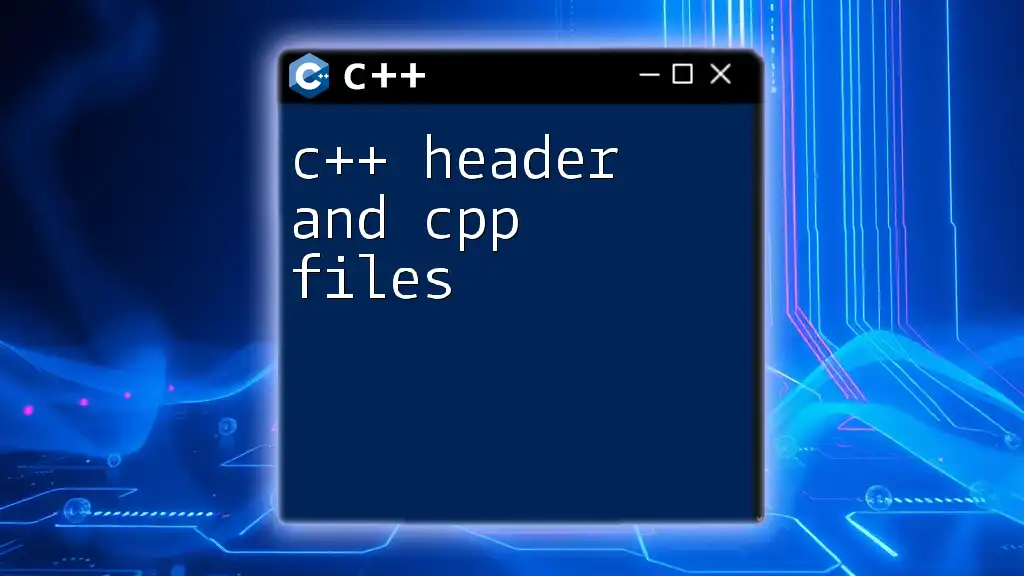
Including Header Files in C++
Using the Preprocessor Directive
To bring a header file into your source code, the `#include` preprocessor directive is used. The syntax can look like this:
#include "myHeader.h" // For local files
#include <iostream> // For standard libraries
Using quotes indicates that the compiler should look for `myHeader.h` in the local directory, while angle brackets suggest that it should search in standard library directories.
Differences Between Angle Brackets and Quotes
When to use one over the other often depends on the location of the header file. Use quotes for user-defined headers that reside in the same directory as your source file, while angle brackets should be applied to standard or library headers that are located in standard directories.
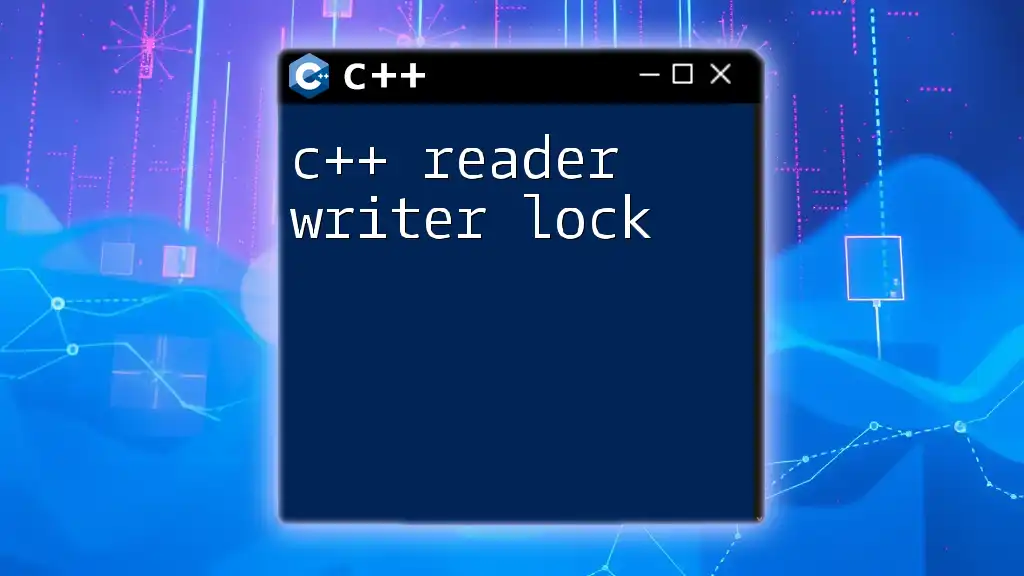
Common Use Cases for Header Files
Defining Classes and Structs
Header files are frequently used for defining classes. Consider this example that shows how to create a simple class in a header file:
// MyClass.h
class MyClass {
public:
void display();
};
This definition outlines a class named `MyClass` that includes a single public method `display()`, which could later be implemented in a corresponding `.cpp` file.
Function Declarations
Header files are also ideal for declaring functions. For instance, you might have a separate header file for various mathematical operations:
// MathFunctions.h
float add(float a, float b);
float subtract(float a, float b);
This approach allows you to call `add` and `subtract` in your main program without needing to know their implementations.
Constants and Macros
Constants and macros can also be defined in header files to promote consistency across your application:
// Constants.h
const double PI = 3.14159;
#define MAX_SIZE 100
By including this header, you have `PI` and `MAX_SIZE` accessible in any file that includes `Constants.h`.
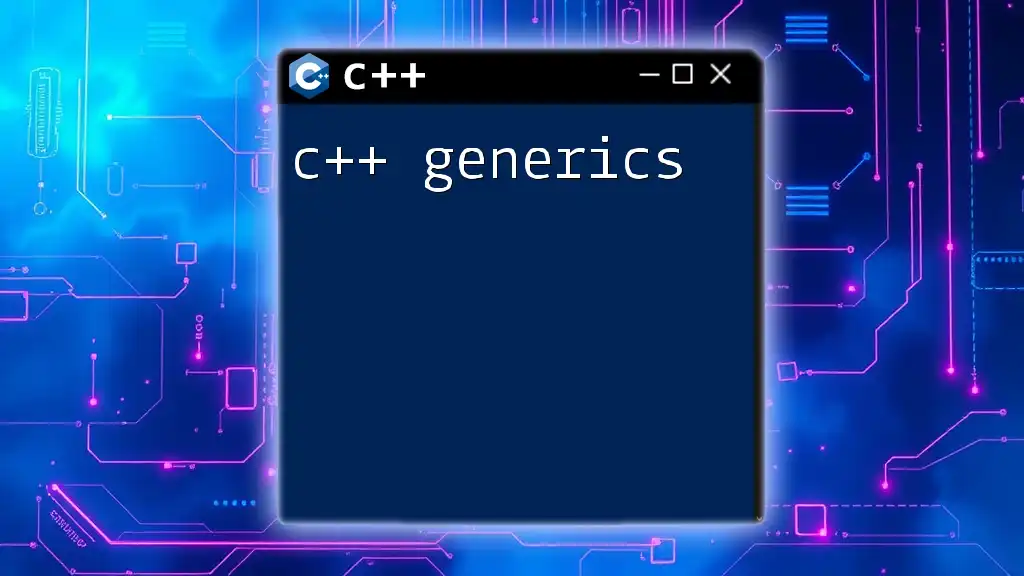
Common Mistakes and How to Avoid Them
Missing Include Guards
A common oversight is neglecting to add include guards, which can lead to issues with multiple definitions. Always ensure include guards are present in your header files to shield against this.
Circular Dependencies
Circular dependencies arise when two header files include each other, leading to compilation errors. To resolve this, use forward declarations where possible, and structure your includes wisely to avoid such pitfalls.
Overusing Header Files
While header files are invaluable, overusing them can lead to confusion. Avoid cluttering your project with unnecessary headers—only include what’s needed to maintain clarity and coherence.
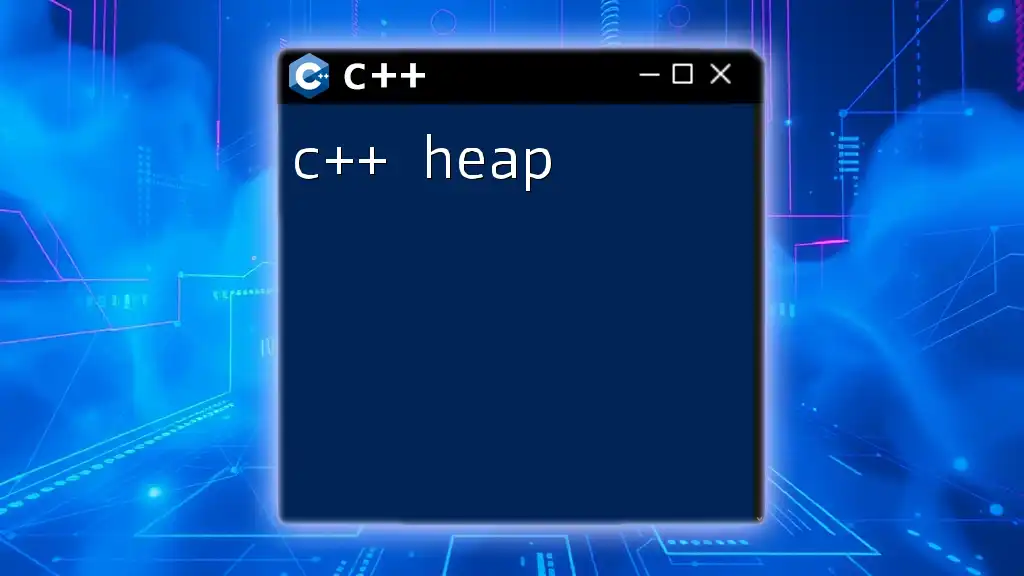
Header Files in Standard Libraries
Understanding the Standard C++ Library
The Standard C++ Library features a multitude of headers, each serving a purpose. Widely used headers include:
- `<iostream>`: for input and output operations
- `<vector>`: for utilizing dynamic arrays
- `<string>`: to work with strings conveniently
Example of Using Standard Header Files
A classic example demonstrating the use of a standard header file would be:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this snippet, the program utilizes the `<iostream>` header to output a greeting message to the console.
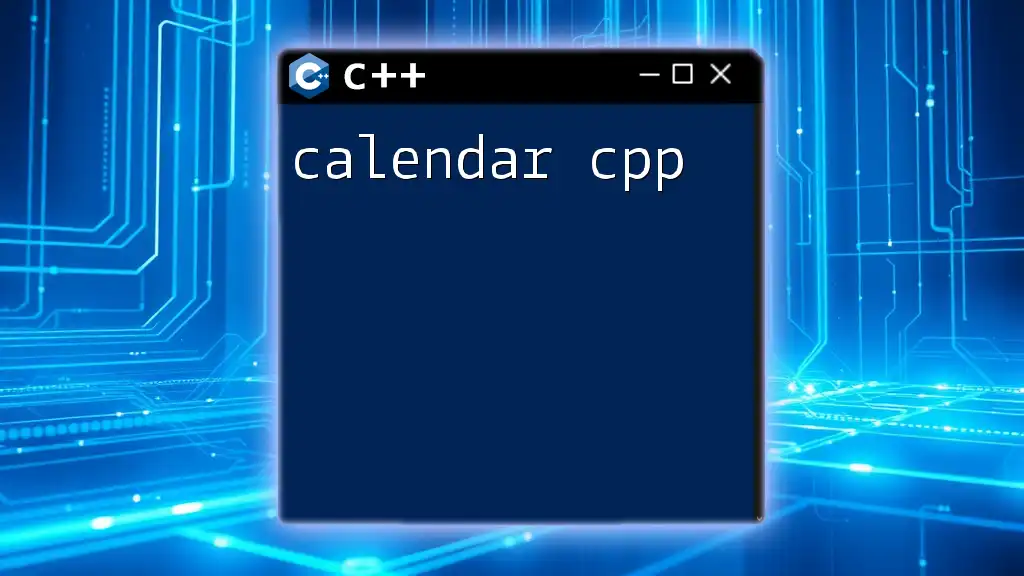
Conclusion
In summary, understanding and utilizing C++ header files is crucial for optimal code organization, reusability, and modular programming. By adhering to best practices, including judicious use of include guards and modular structures, you can significantly enhance your coding efficiency and maintainability. Don't forget to practice these concepts in your coding projects to solidify your understanding!
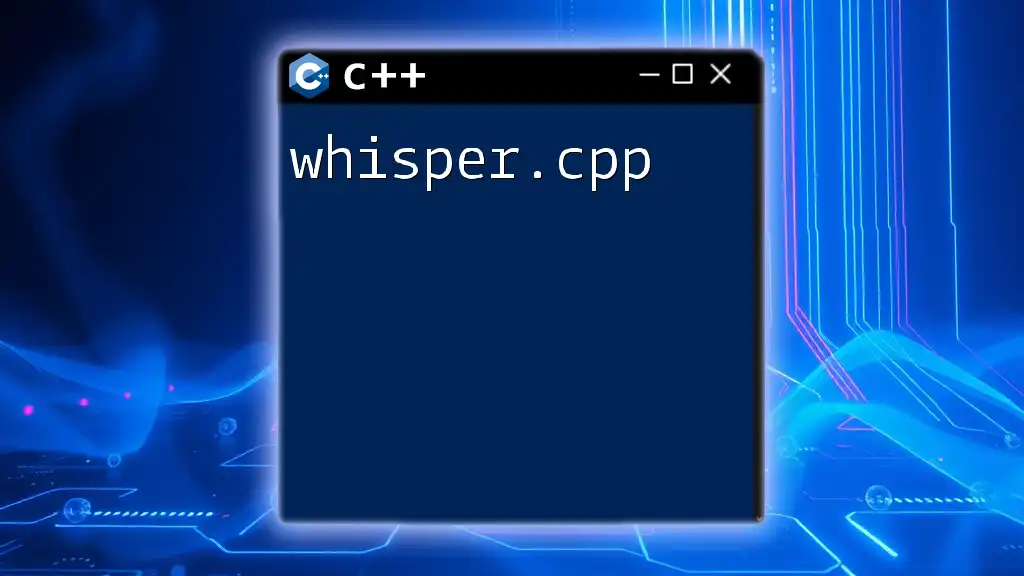
Additional Resources
For further reading and practice, you might want to explore some books and tutorials on C++. Joining online C++ forums and communities can also be beneficial for learning and exchanging knowledge. Always refer to documentation resources like cppreference.com for the most reliable information on C++ programming.