In C++, header files (with a .h extension) declare functions, classes, and variables for use in multiple source files (with a .cpp extension), promoting code reusability and organization.
Here's a simple example of how to use a header and CPP file:
header file (example.h):
#ifndef EXAMPLE_H
#define EXAMPLE_H
void greet(); // Function declaration
#endif // EXAMPLE_H
source file (example.cpp):
#include <iostream>
#include "example.h"
void greet() { // Function definition
std::cout << "Hello, World!" << std::endl;
}
int main() {
greet(); // Calling the function
return 0;
}
What are Header Files?
Definition of Header Files
In C++, header files serve as an essential blueprint for your code. These files typically contain declarations of functions, classes, and variables that can be shared between multiple source files. When you separate your declarations into header files, it allows for a more organized and manageable codebase. Header files by themselves do not contain actual implementations; instead, they provide information that can be leveraged in different source files (CPP files).
Common File Extensions
Header files commonly use the following file extensions:
- .h: Traditionally used for C and C++ header files.
- .hpp: Often used in C++ to emphasize that it contains C++ code.
Purpose of Header Files
Header files serve multiple purposes in C++, making them vital for efficient programming.
-
Declarations vs. Definitions: Declarations inform the compiler about the structure and types of functions and classes but do not include their implementations, which are left for CPP files. This separation simplifies the compilation process.
-
Facilitating Code Reusability: By declaring functions and classes in header files, you can include them in multiple CPP files, reducing redundancy.
-
Keeping Code Organized: Header files help you manage large projects by allowing you to separate different functionalities into distinct files, making it easier to navigate your code.
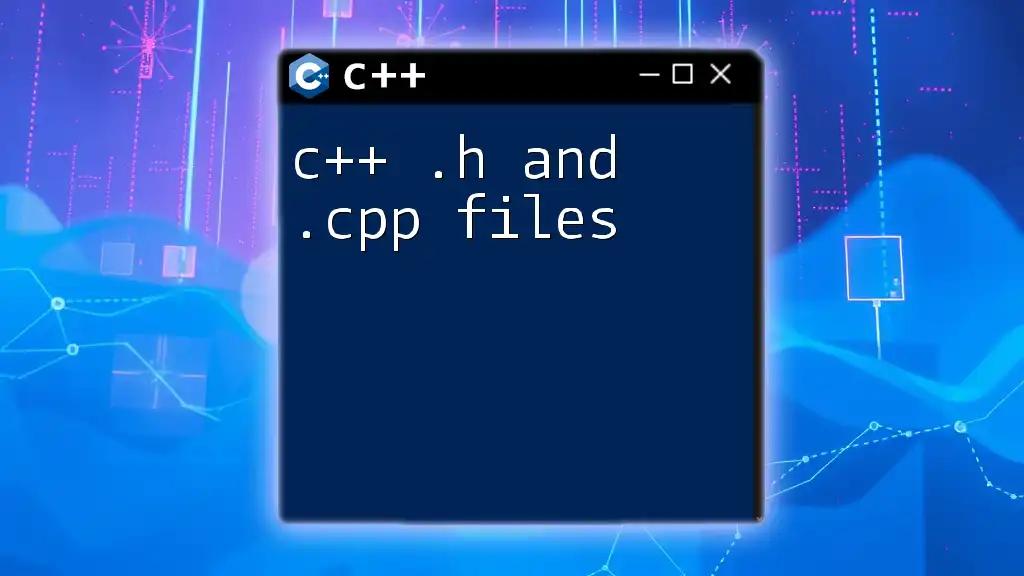
Structure of a Header File
Basic Components
A typical header file will include:
-
Includes and Guards: This prevents double inclusion of the same header file, which can lead to compilation errors. The guards are defined using `#ifndef`, `#define`, and `#endif` directives.
-
Function Declarations: These allow you to describe the functions without defining them.
-
Class Declarations: Classes can be declared, specifying their public and private members.
Example of a Simple Header File
Here’s an example of a simple header file:
#ifndef MYHEADER_H
#define MYHEADER_H
void sayHello();
class MyClass {
public:
void display();
};
#endif // MYHEADER_H
In this example:
- The guards ensure that the content between `#ifndef` and `#endif` is only included once, preventing duplicate definitions.
- The function `sayHello()` is declared, as well as a class `MyClass` with a public method `display()`.
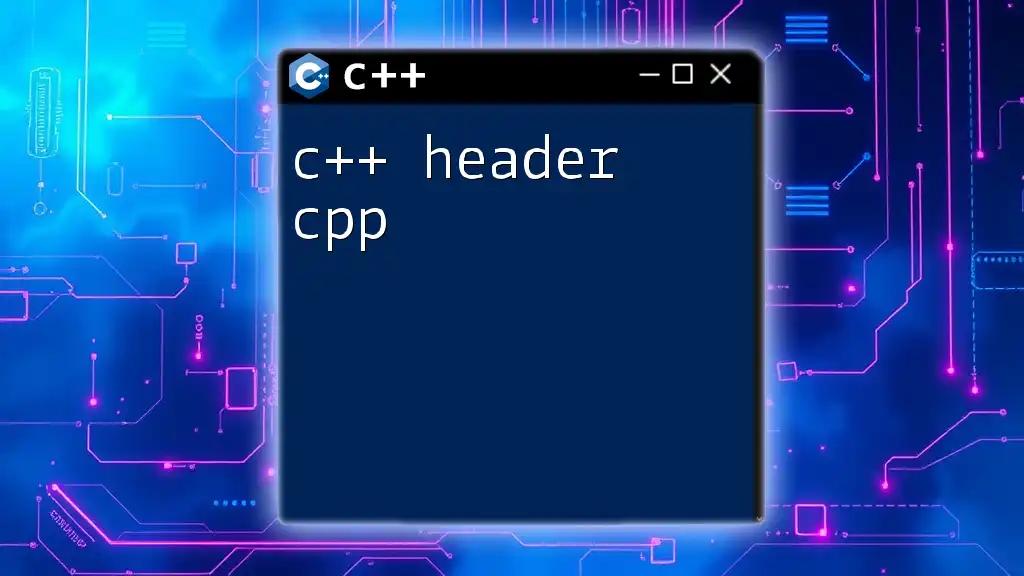
What are CPP Files?
Definition of CPP Files
CPP files, short for C++ source files, contain the implementation of the functions and classes that are declared in the header files. The CPP file is where you determine the behavior of your program, making it the engine that drives your application.
Common File Extensions
CPP files typically use the .cpp extension, signaling to the compiler that they contain C++ code.
Purpose of CPP Files
The prime role of CPP files is to provide the implementations for the declarations made in header files. This separation streamlines the compilation process, as it allows the compiler to process the interface (header files) independently from the implementation details (CPP files).
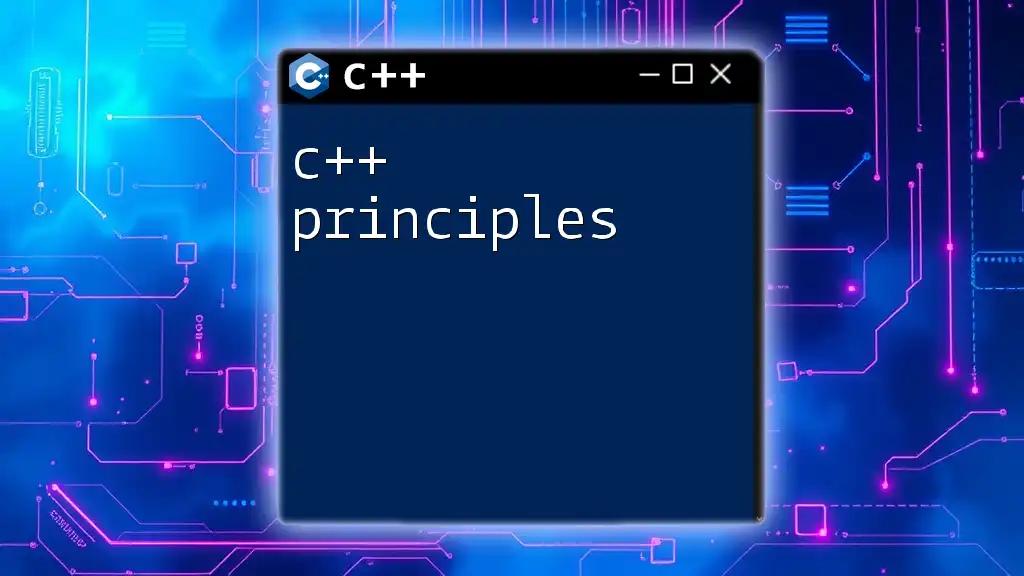
Structure of a CPP File
Basic Components
A CPP file will usually contain:
-
Preprocessor Directives: To include the relevant header files and libraries.
-
Include Statements: These link your CPP file to necessary header files.
-
Main Function: This is the entry point of the C++ application.
Example of a Simple CPP File
Here’s how a simple CPP file is structured:
#include "MyHeader.h"
#include <iostream>
void sayHello() {
std::cout << "Hello, World!" << std::endl;
}
void MyClass::display() {
std::cout << "Displaying MyClass!" << std::endl;
}
int main() {
sayHello();
MyClass myObject;
myObject.display();
return 0;
}
In this example:
- The line `#include "MyHeader.h"` connects the CPP file to the declared functions and classes in the header file.
- The main function calls `sayHello()` and creates an object of `MyClass` to call the `display()` function, showcasing how implementations are built on declarations.

How to Include Header Files in CPP Files
Using #include Directive
Including header files in your CPP files is straightforward. You utilize the `#include` directive, which tells the compiler to include the content of the specified header file. For standard library headers, use angle brackets (`<>`), and for custom header files, use quotes (`""`).
#include "MyHeader.h"
This directive allows the functions and classes declared in `MyHeader.h` to be accessible in your CPP file.
Understanding Include Guards
Include guards are a critical feature for preventing multiple inclusions of the same header file, which can lead to redefinition errors. The structure looks like this:
#ifndef MYHEADER_H
#define MYHEADER_H
// Declarations go here
#endif // MYHEADER_H
With this, the contents of your header file will only be included the first time the compiler encounters it.
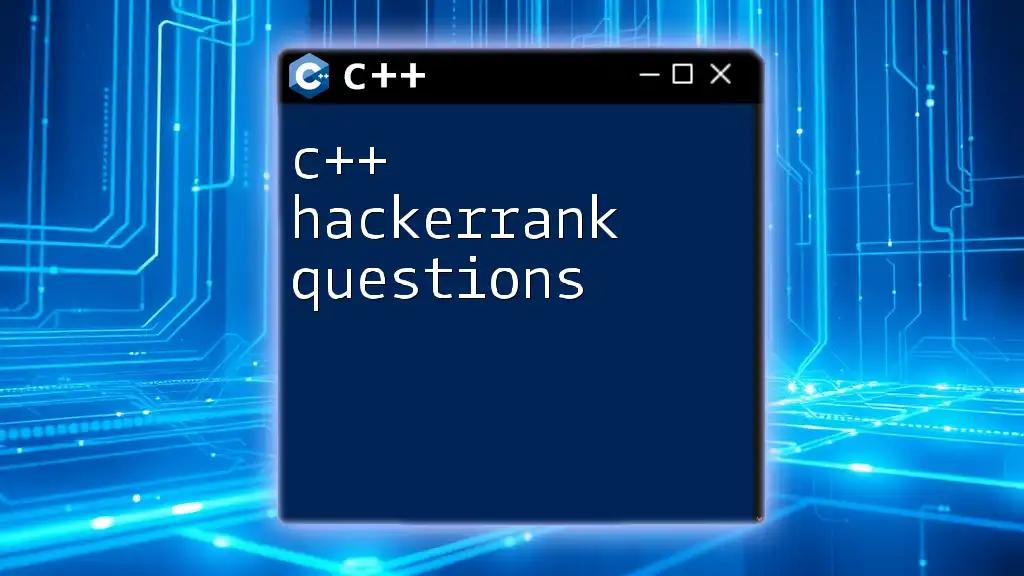
Best Practices for Organizing Header and CPP Files
Naming Conventions
Using consistent and descriptive naming conventions can significantly enhance code readability. For instance, if your header file is called `MyClass.h`, your corresponding implementation file should be named `MyClass.cpp`. This convention makes it clear which implementation corresponds to which declaration.
Avoiding Circular Dependencies
Circular dependencies can occur if two header files include each other. This can lead to compilation issues. You can avoid this by utilizing forward declarations—declaring a class before defining it—to break the circular dependency.
Documentation and Comments
Good commenting practices cannot be overstated. Documenting your header files clearly, especially describing the purpose of various functions and classes, enhances maintainability. Using tools like Doxygen can further help automate the generation of documentation.

When to Use Header Files vs. CPP Files
Scenarios for Using Header Files
You should use header files when you need to declare functions and classes that will be used in multiple source files. This is particularly useful for libraries and modules that can be reused across different parts of your application.
Scenarios for Using CPP Files
Use CPP files to implement the logic, defining the declared functions and classes. The main function, which serves as the entry point of your program, should also be placed in a CPP file.

Conclusion
In summary, understanding C++ header and CPP files is paramount for writing efficient and organized code. By leveraging header files for declarations and CPP files for implementations, we can create a clean separation of concerns, fostering reusable and maintainable code. Adopting best practices in naming conventions, avoiding circular dependencies, and documenting your code will set a strong foundation for your programming endeavors.
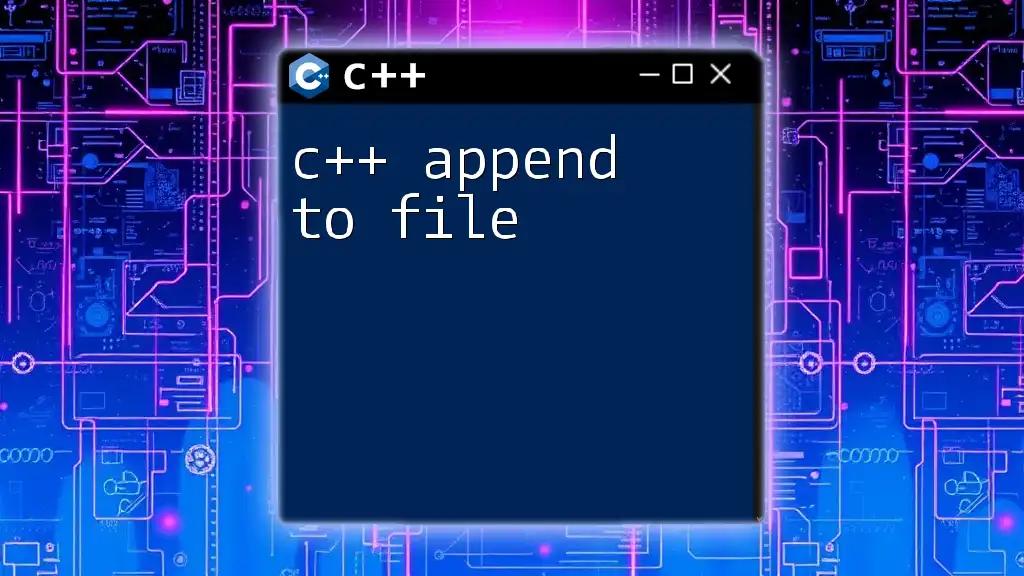
Additional Resources
To further your learning, consider checking out recommended books, online courses, and useful resources that delve deeper into header and CPP file structures in C++.

FAQs
The FAQs section can address common questions about C++ header and CPP files, offering quick tips for troubleshooting issues that may arise during development. This will help solidify the knowledge gained through the article and assist in practical applications.