C++ HackerRank questions typically challenge users to solve algorithmic problems using efficient programming techniques and coding practices.
Here’s a simple C++ code snippet that demonstrates how to find the maximum element in an array, which is a common type of challenge on platforms like HackerRank:
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> arr = {1, 5, 3, 9, 2};
int maxElement = arr[0];
for(int i = 1; i < arr.size(); i++) {
if(arr[i] > maxElement) {
maxElement = arr[i];
}
}
cout << "Maximum Element: " << maxElement << endl;
return 0;
}
Understanding HackerRank
What is HackerRank?
HackerRank is an online platform dedicated to coding and technical skill development through practice and competition. It offers a vast collection of coding challenges that enable users to enhance their programming abilities across various domains, including algorithms, data structures, artificial intelligence, and even specific languages like C++. By tackling these challenges, programmers can sharpen their skills in a hands-on manner, which is crucial for excelling in real-world software development tasks.
Benefits of Solving HackerRank Questions
Participating in C++ HackerRank questions carries numerous benefits:
- Improves Problem-Solving Skills: Frequent practice helps you develop a systematic approach to breaking down problems and formulating solutions.
- Prepares for Technical Interviews: Many tech companies use platforms like HackerRank to conduct programming assessments for candidates, making this practice valuable.
- Validates Coding Proficiency: Successfully completing challenges can be a confidence booster and provide evidence of your coding skills for potential employers.
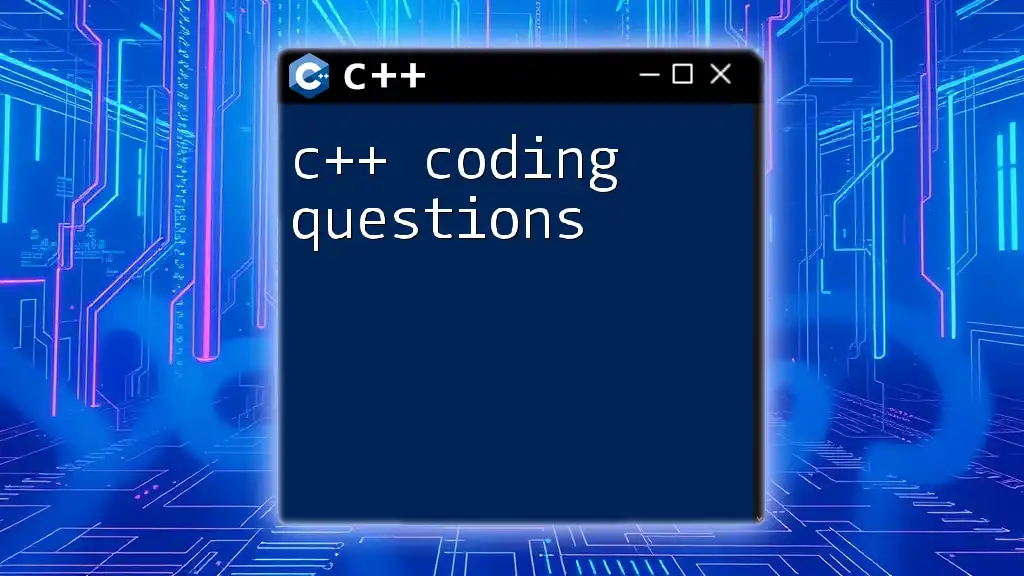
Getting Started with C++ on HackerRank
Setting up Your HackerRank Profile
To begin utilizing HackerRank for solving C++ questions, you first need to create an account. The process is straightforward:
- Visit the HackerRank Website: Navigate to the main page and click on the 'Sign Up' button.
- Fill in Your Details: Register by providing your email and creating a password.
- Choose C++ as Your Primary Language: After registration, select C++ from the list of programming languages. This ensures that you're set up to receive challenges tailored to your preferred language.
Navigating the C++ Challenges
Once registered, the next step is to explore the available C++ challenges. HackerRank offers a variety of problems ranging from easy to hard.
- Beginner-Friendly Options: If you're new to C++, it’s wise to start with simpler challenges to build your confidence and grasp the fundamentals.
- Skill Selection: Based on your current knowledge, you can choose challenges that focus on specific topics such as loops, functions, or data structures.
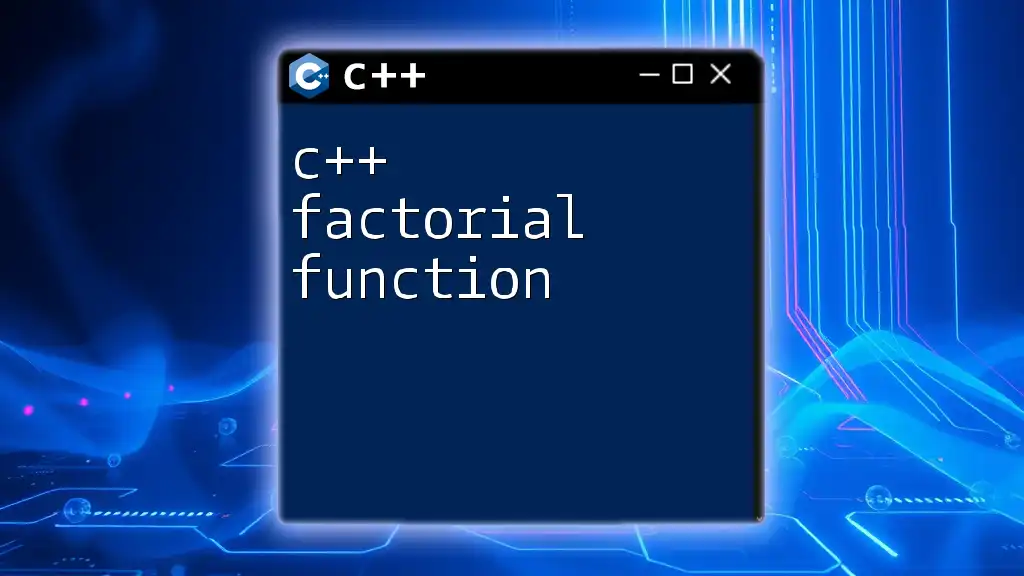
Common C++ HackerRank Questions
Easy Level Questions
One of the most beginner-friendly challenges on HackerRank is the "Say Hello, World!" task. This task introduces you to the essentials of syntax and output in C++.
Example Question: "Say Hello, World!"
- Task Explanation: Your objective is to output the text "Hello, World!".
- Code Snippet:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
- Explanation: In this code, we include the `<iostream>` header for input and output capabilities. The `main()` function is the entry point. We use `cout` to print the string followed by an end line indication.
Medium Level Questions
As you become comfortable with basic tasks, you can tackle medium-level questions that require a deeper understanding of conditional logic and loops.
Example Question: "Conditional Statements"
- Task Explanation: You need to determine if a given integer meets specific conditions to classify it as "Weird" or "Not Weird."
- Code Snippet:
#include <iostream>
using namespace std;
int main() {
int n;
cin >> n;
if (n % 2 == 1) {
cout << "Weird" << endl;
} else if (n >= 2 && n <= 5) {
cout << "Not Weird" << endl;
} else if (n >= 6 && n <= 20) {
cout << "Weird" << endl;
} else {
cout << "Not Weird" << endl;
}
return 0;
}
- Explanation: This code evaluates whether the integer `n` is odd or falls within certain ranges to categorize it. The `if-else` structure helps you navigate through the different conditions.
Hard Level Questions
Once you're well-versed in basic and intermediate challenges, it's time to tackle hard-level questions, which often revolve around more complex algorithms and data structures.
Example Question: "Binary Search"
- Task Explanation: Implement a binary search algorithm to find a target element in a sorted array.
- Code Snippet:
#include <iostream>
using namespace std;
int binarySearch(int arr[], int size, int target) {
int left = 0, right = size - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1; // Not found
}
- Explanation: This code establishes a binary search algorithm, which works by dividing the search interval in half. If the element is found, its index is returned; otherwise, an indication of "not found" is given.
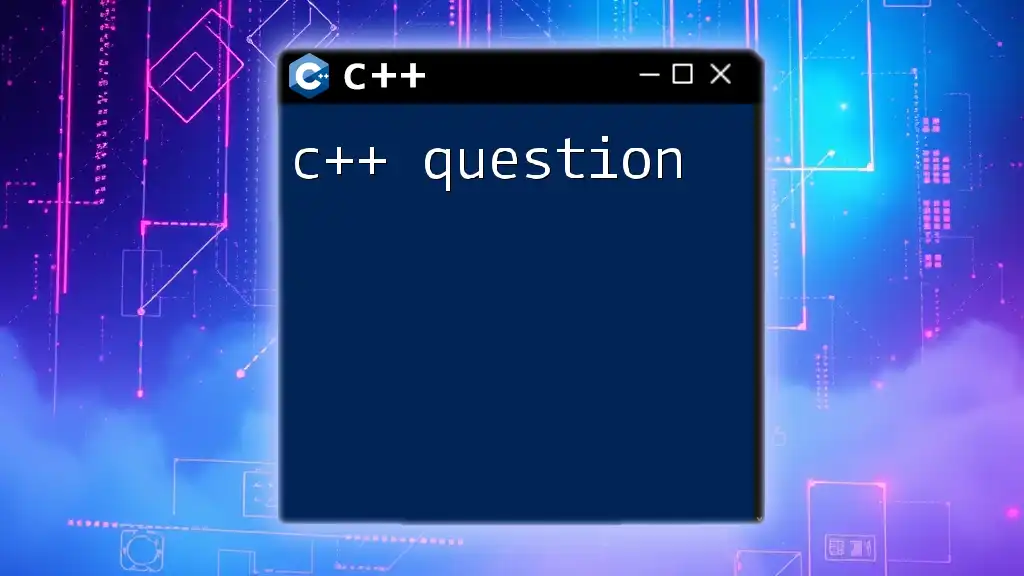
Strategies for Solving HackerRank C++ Questions
Understanding the Problem Statement
Before diving into coding, it’s essential to thoroughly read and understand the problem statement. Take your time to identify:
- Input and Output Requirements: Pay attention to what is expected in terms of input format and the desired output.
- Constraints: Be aware of any limitations or specific conditions that need to be met.
Writing Efficient C++ Code
Coding efficiently is crucial, especially in competitive programming. Here are essential tips:
- Optimize Time Complexity: Aim for solutions that run within the time limits set by the challenges.
- Reduce Space Complexity: Utilize data structures wisely to minimize the memory footprint of your program.
Debugging Common Issues
When tackling C++ HackerRank questions, debugging becomes a vital skill. Common pitfalls include:
- Runtime Errors: Often caused by accessing out-of-bounds indices or inappropriate data types. Always validate your inputs.
- Syntax Errors: Double-check your syntax, such as mismatched braces and missing semicolons, which can lead to failure during test cases.
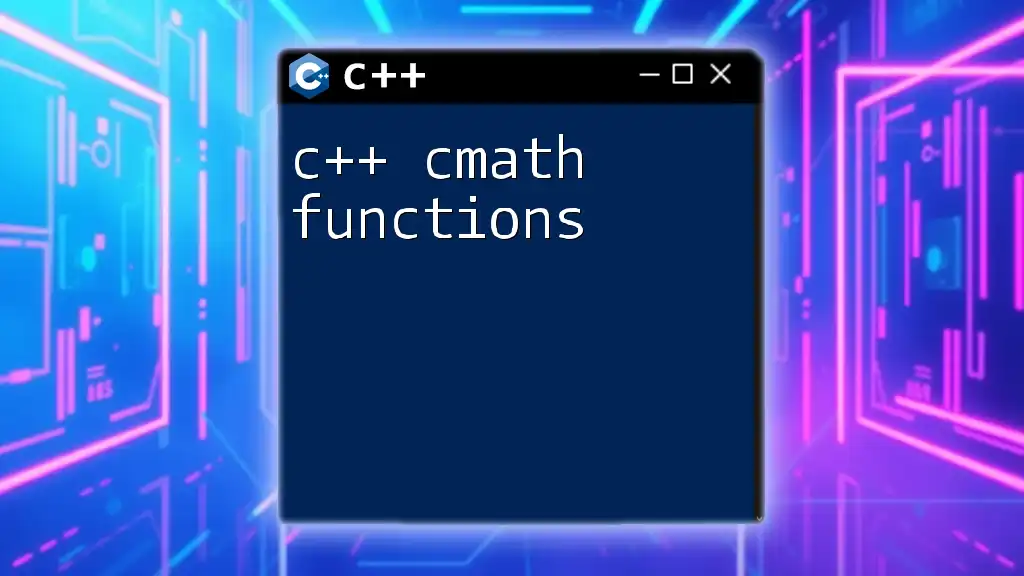
Conclusion
Mastering C++ HackerRank questions is an excellent way to sharpen your programming skills and prepare for the tech industry. By solving a variety of challenges, you will build a solid foundation in C++ while enhancing your problem-solving capabilities. Don't hesitate to share your experiences in the comment section, as the journey of coding is best enjoyed when shared with others.
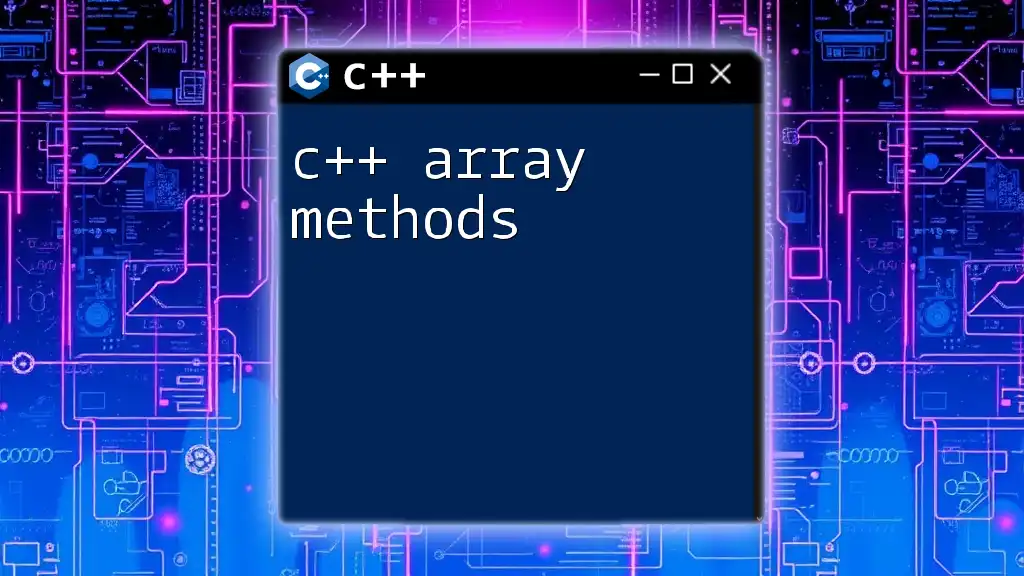
Additional Resources
Recommended Practice Websites
While HackerRank is a fantastic starting point, consider supplementing your practice with other platforms like LeetCode, Codewars, and Codecademy that provide additional C++ challenges and learning materials.
Community and Forums
Engaging with the programming community can significantly enhance your learning. Websites and forums like Stack Overflow, Reddit's r/programming, and even HackerRank's own community page are valuable resources where you can ask questions, share strategies, and connect with fellow coders. Joining a community can offer support and motivation as you take on the challenges that lie ahead.