In this post, we'll explore essential C++ coding questions to enhance your understanding and proficiency in using C++ commands effectively.
Here’s a basic example of a C++ program that outputs "Hello, World!" to demonstrate basic syntax:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Coding Questions
What are C++ Coding Questions?
C++ coding questions are specific problems or challenges designed to assess a programmer's understanding of the C++ language and programming concepts. These questions test knowledge on fundamental topics such as data structures, algorithms, object-oriented programming, and memory management. They can be practical applications, theoretical concepts, or problems requiring algorithmic solutions.
Importance of C++ Coding Questions in Interviews
In the fast-paced tech industry, interviews often hinge on the candidate's ability to solve coding problems efficiently. C++ coding questions provide insights into a programmer's problem-solving skills, coding proficiency, and grasp of C++ concepts. Success in these interviews can significantly influence career opportunities and positions within a company.
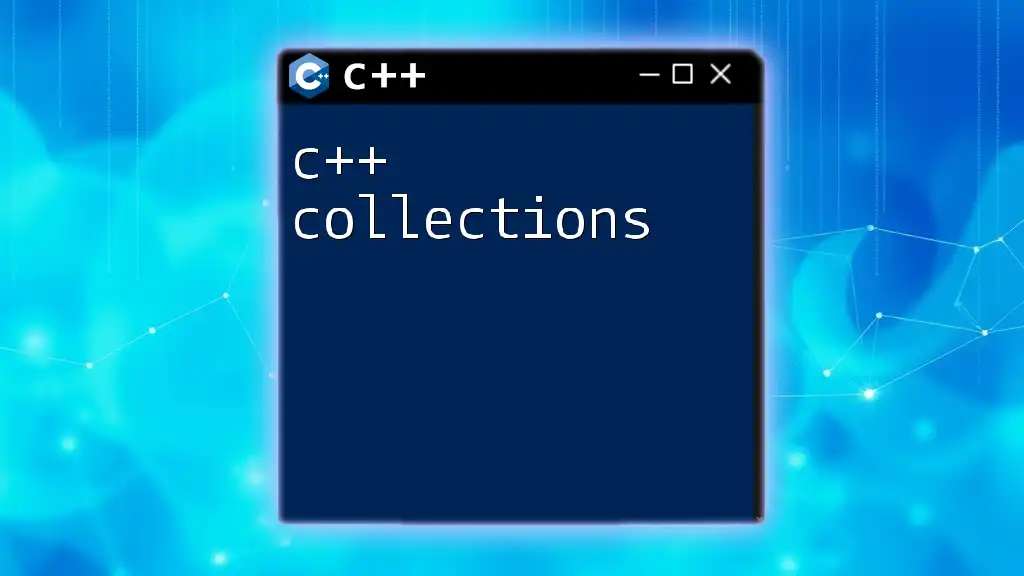
Common Categories of C++ Coding Questions
Data Structures and Algorithms
Data structures and algorithms form the backbone of many programming challenges. Understanding both basic data structures (like arrays, linked lists, and trees) and algorithms (such as searching and sorting) is crucial.
Example Question: "Implement a binary search algorithm."
Binary search is an efficient algorithm to find an item from a sorted list of items. Here’s how it can be implemented in C++:
int binarySearch(int arr[], int size, int target) {
int left = 0, right = size - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1; // Target not found
}
Object-Oriented Programming Concepts
Object-oriented programming (OOP) is another fundamental aspect of C++. It allows programmers to create complex systems through encapsulation, inheritance, and polymorphism.
Example Question: "Explain the concept of polymorphism in C++ with a code example."
Polymorphism allows methods to do different things based on the object it is acting upon, which is a cornerstone of OOP. The following example demonstrates polymorphism:
class Base {
public:
virtual void show() { cout << "Base class show() called."; }
};
class Derived : public Base {
public:
void show() override { cout << "Derived class show() called."; }
};
void demonstratePolymorphism(Base* base) {
base->show();
}
In this example, the `show` method behaves differently based on whether it is called on a `Base` or `Derived` class object.
Memory Management
C++ provides fine-grained control over memory use, which is both powerful and potentially risky. Understanding pointers, dynamic memory allocation, and deallocation is vital to prevent memory leaks.
Example Question: "What is a memory leak, and how can you prevent it in C++?"
A memory leak occurs when a program allocates memory but fails to release it back to the system after it is no longer needed. To prevent memory leaks, always ensure that every `new` operation is paired with a corresponding `delete` operation. Here’s a simple demonstration:
void memoryLeakExample() {
int* ptr = new int(10);
// Use the allocated memory
// Memory leak if 'delete ptr' is not called
delete ptr; // Memory is freed
}
C++ Standard Library
The C++ Standard Library (STL) offers pre-written classes and functions that simplify programming tasks. Familiarity with STL components, including vectors, maps, and algorithms, can significantly boost productivity.
Example Question: "How do you use a `std::vector` in C++?"
Vectors are dynamic arrays that can resize themselves automatically. Here's a basic example:
#include <vector>
#include <iostream>
void manipulateVector() {
std::vector<int> numbers = {1, 2, 3};
numbers.push_back(4);
for (int num : numbers) { std::cout << num << " "; }
}
In this snippet, we initialize a vector, add an element, and then print its contents.
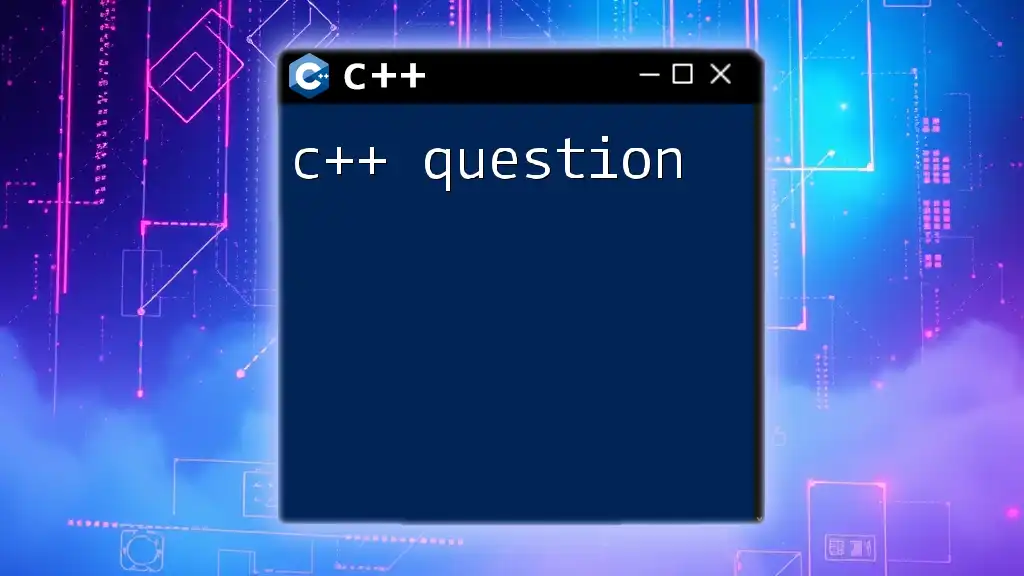
Preparing for C++ Coding Interviews
Practice Makes Perfect
The best way to prepare for coding interviews is through rigorous practice. Utilize coding platforms like LeetCode, HackerRank, and CodeSignal. These platforms offer a plethora of C++ coding questions that help you simulate real interview conditions.
Mock Interviews and Peer Reviews
Engaging in mock interviews with peers is invaluable. It provides real-time feedback and mimics the high-pressure environment of actual coding interviews. Peer reviews of your code also foster constructive criticism, helping you to refine your thinking and approach.
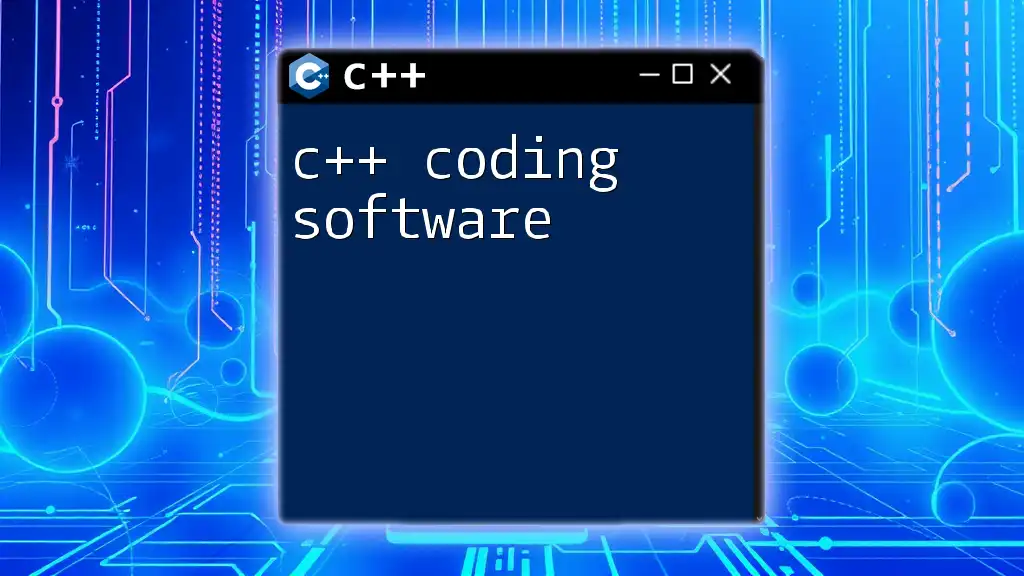
Strategies for Answering C++ Coding Questions
Breaking Down the Problem
When faced with a complex coding question, taking a moment to understand and break the problem down into smaller, manageable parts can help. Identify the inputs and outputs, and note any constraints. This systematic approach often leads to clearer solutions.
Writing Clear and Efficient Code
Always aim for clarity and efficiency in your code. Write code that not only works but is also easily understandable. Following conventions and clean coding practices goes a long way in demonstrating professionalism.
Example: Compare the following poorly written function to a cleaner version.
Poorly written:
int f(int a) {
return a*a*a;
}
Cleaner version:
int cube(int number) {
return number * number * number;
}
The latter is easier to read and understand.
Testing Your Code
Unit testing is crucial in validating the correctness of your solution. Consider using frameworks like Google Test to automate testing in C++. Effective testing can catch edge cases that may cause your code to fail.
Example of a simple test case:
#include <cassert>
void test_cubicFunction() {
assert(cube(3) == 27);
assert(cube(-3) == -27);
}
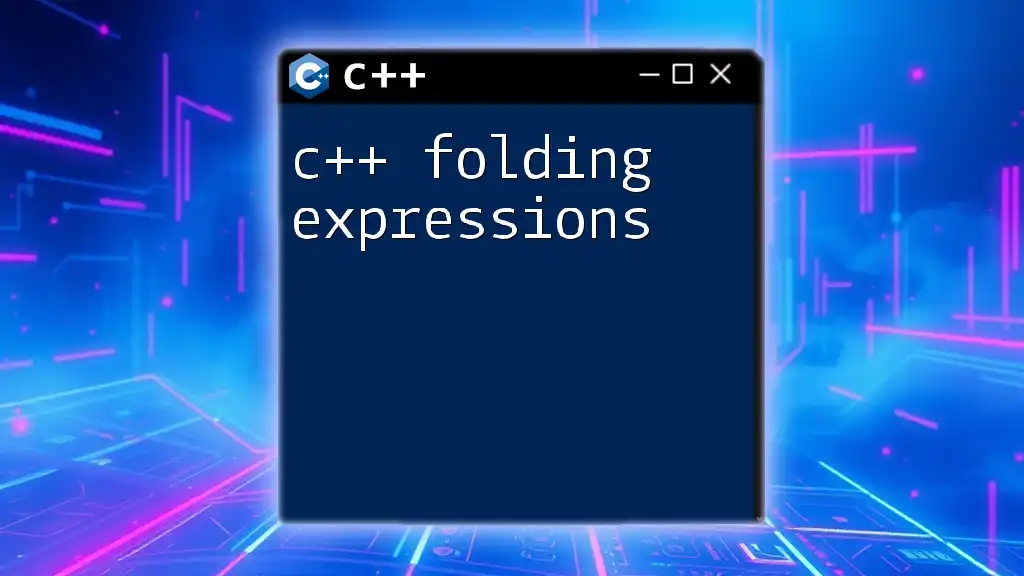
Conclusion
Mastering C++ coding questions is a critical aspect of preparing for technical interviews. By understanding various categories of questions and practicing effectively, you can improve your coding skills and increase your confidence as a programmer. Utilize resources, engage in mock interviews, and maintain a relentless pursuit of understanding to enhance your C++ expertise. Remember, consistent practice is key to success in overcoming coding challenges and impressing potential employers.
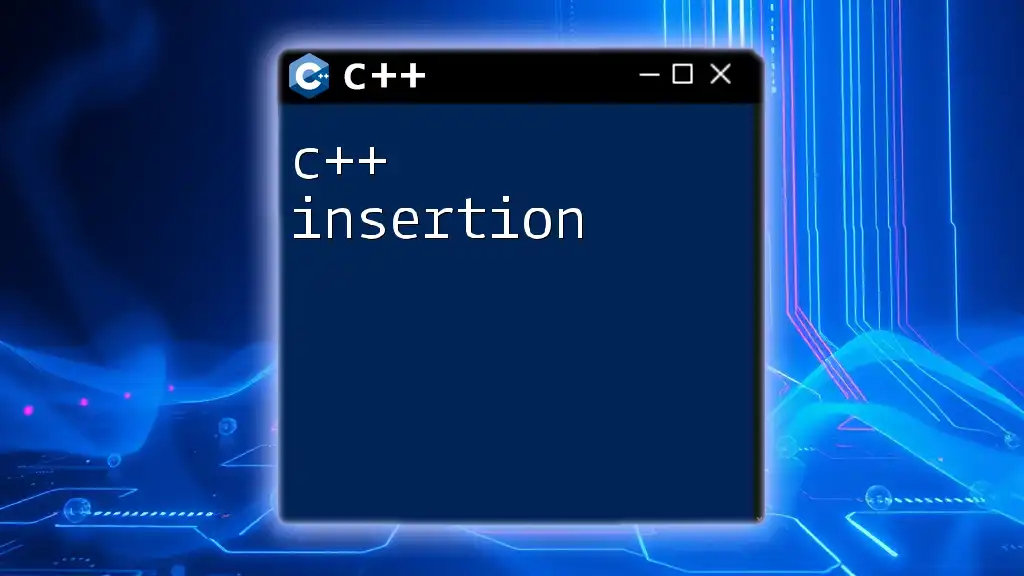
Additional Resources
To deepen your knowledge and skills, consider exploring the following resources:
- Recommended books on C++ programming.
- Online courses for comprehensive learning.
- Communities for peer support and feedback in coding practices۔