C++ catching exceptions allows you to handle runtime errors gracefully using try-catch blocks to prevent program crashes.
#include <iostream>
#include <stdexcept>
int main() {
try {
throw std::runtime_error("An error occurred");
} catch (const std::runtime_error& e) {
std::cerr << "Caught an exception: " << e.what() << std::endl;
}
return 0;
}
Understanding Exceptions in C++
What are C++ Exceptions?
Exceptions in C++ are a mechanism for handling unexpected situations that arise during runtime, allowing developers to manage errors in a controlled way. Unlike regular errors that can be fixed at compile-time, exceptions occur due to unforeseen circumstances, such as invalid user input, out-of-bounds array access, or resource allocation failures.
This distinction is crucial because it enables programs to recover gracefully from errors without crashing, thereby enhancing robustness and user experience.
Types of Exceptions
In C++, exceptions can generally be categorized into:
-
Built-in Exceptions: These are predefined exceptions provided by the C++ Standard Library. For instance, `std::exception` is the base class for all standard exceptions, and classes like `std::runtime_error` and `std::out_of_range` represent specific types of runtime errors.
-
User-defined Exceptions: Developers can create custom exception classes tailored to specific application needs. This is particularly useful when standard exceptions do not adequately represent an error condition.
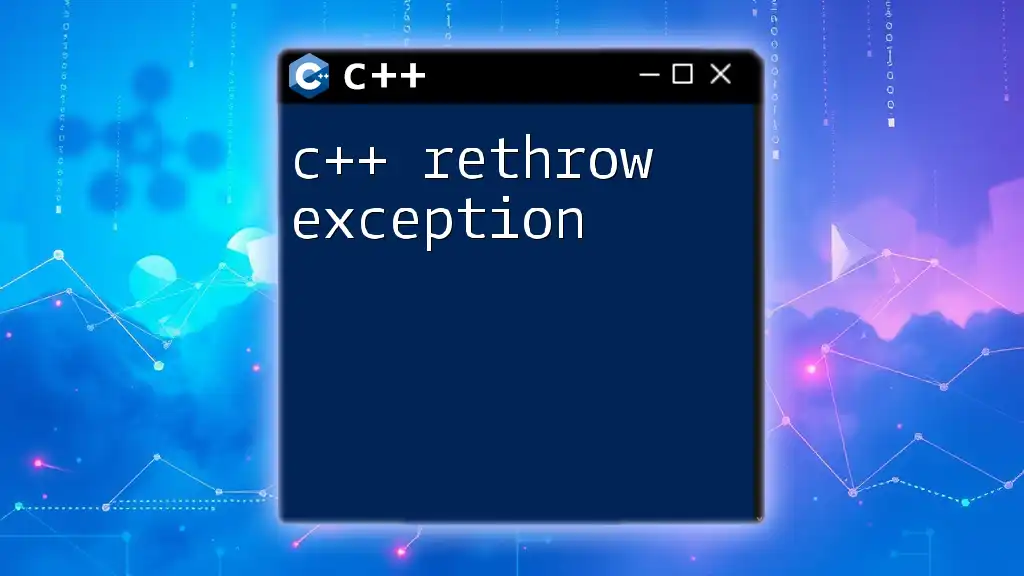
The Basics of Exception Handling
Using Try-Catch Blocks
The cornerstone of exception handling in C++ is the try-catch block. Here’s how it works:
- try: This block contains code that may throw an exception.
- catch: This block defines how to handle the exception if it occurs.
- throw: Used to signal that an exception has occurred.
The following is a basic syntax example of using try-catch:
try {
// Code that may throw an exception
} catch (const std::exception& e) {
// Code that handles the exception
}
When to Use Exception Handling
Exception handling is essential when:
- There is a reasonable chance that an operation may fail.
- Maintaining flow control is crucial after an error occurs.
- You need to ensure resources are adequately freed after an error.
However, it's important to be cautious, as extensive use of exceptions can lead to performance overhead. Therefore, keep exception handling minimal and relevant to critical error management code.
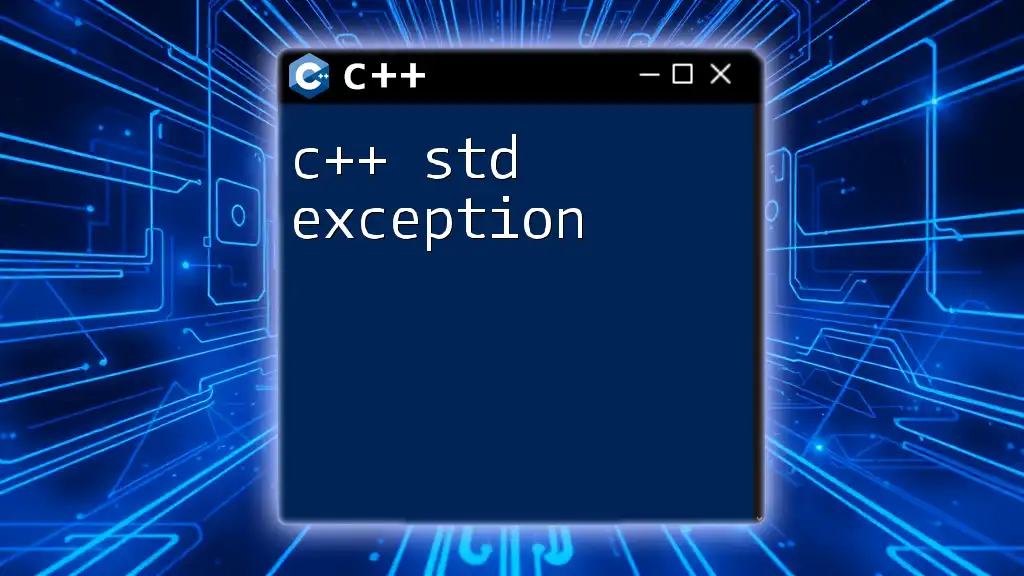
C++ Exception Handling Example: The Try-Catch Mechanism
Exception Handling Example in C++
Here’s a practical example that illustrates how exceptions are caught and handled in C++:
#include <iostream>
#include <stdexcept>
void mightGoWrong() {
throw std::runtime_error("Something went wrong!");
}
int main() {
try {
mightGoWrong();
} catch (const std::runtime_error& e) {
std::cerr << "Caught an exception: " << e.what() << std::endl;
}
return 0;
}
Explaining the Example
In this code:
- The `mightGoWrong()` function explicitly throws a `std::runtime_error` using the `throw` keyword.
- The `main()` function includes a `try` block that invokes `mightGoWrong()`.
- If an exception is thrown, control flows to the `catch` block, which handles it by displaying an error message.
This example illustrates the simplicity of exception handling: isolate error-prone code with try blocks and elegantly manage exceptions with catch blocks.
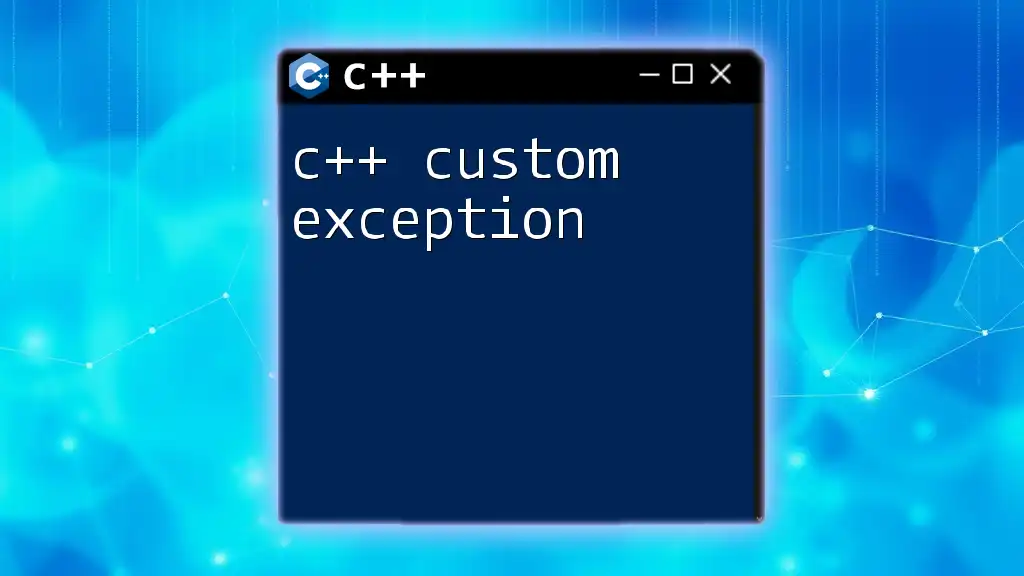
Advanced Exception Handling Techniques
C++ Catch All Exceptions
Sometimes, you may want to handle all exceptions without caring for their specific types. This can be done using a generic catch block:
catch (...) {
// Handle all other exceptions
}
This catch-all syntax is useful when you want to ensure your application continuously runs without crashing, but be cautious as it doesn’t give specifics about the error.
Re-throwing Exceptions
In certain scenarios, you might catch an exception but determine that it can’t be handled appropriately in that context. In such cases, it’s essential to re-throw the exception so that higher-level code can handle it.
try {
// Some code
} catch (const std::exception& e) {
// Handle the exception
throw; // re-throws the same exception
}
Re-throwing preserves the original exception type and context, making it easier to diagnose issues later.
Creating Custom Exceptions
Creating custom exceptions allows you to encapsulate domain-specific error information. This can significantly enrich the debugging experience in complex applications.
class CustomException : public std::exception {
public:
const char* what() const noexcept override {
return "This is my custom exception!";
}
};
When defining a custom exception, it's a good practice to override the `what()` method to provide meaningful error messages.
When to Use Custom Exceptions
Use custom exceptions when you need to signal specific error states unique to your application. This practice enhances code clarity and maintainability since it quickly informs users about what went wrong.
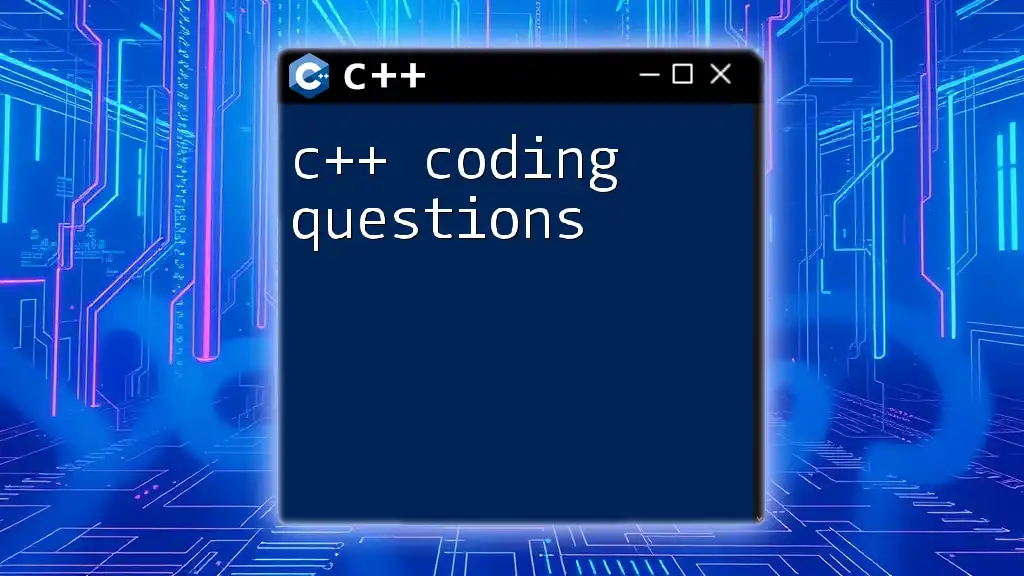
Common Mistakes in Exception Handling
Avoiding Common Pitfalls
When working with exception handling, be wary of these common mistakes:
- Not catching exceptions by reference: Always catch exceptions using reference types (e.g., `const std::exception&`) instead of by value to avoid slicing and reduce overhead.
- Ignoring the exception type: Catching all exceptions without any type checking can make it difficult to manage errors properly.
- Not cleaning up resources properly: Ensure that resources, such as file handles or memory allocations, are freed. Using RAII (Resource Acquisition Is Initialization) and smart pointers can help manage this automatically.
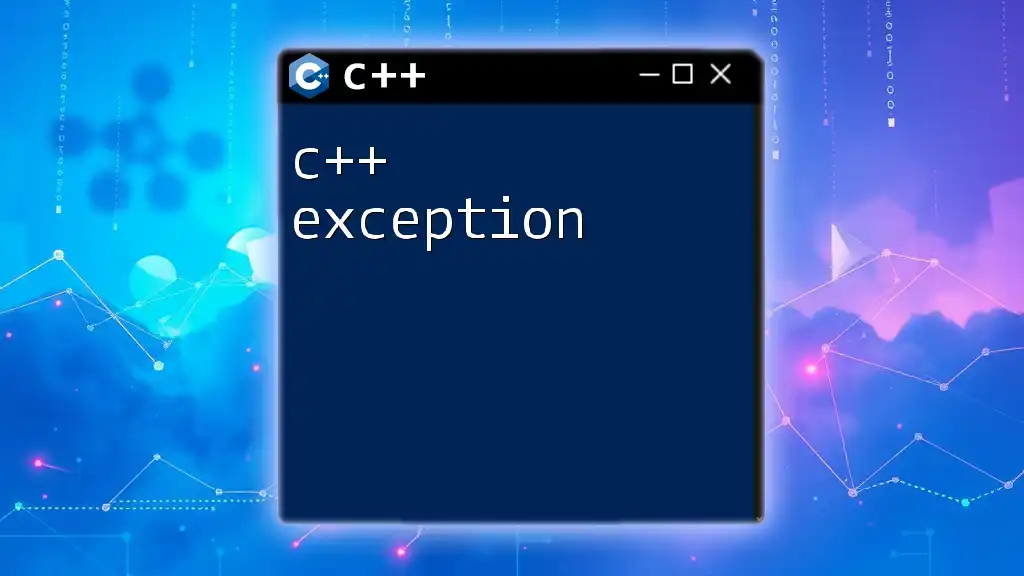
Best Practices for Exception Handling in C++
Best Practices Using Try-Catch
- Keep try blocks small: Limit the amount of code inside try blocks. This makes it easier to identify the source of errors.
- Catch only those exceptions you can handle: Handling errors you cannot address will lead to confusion and potentially mask critical issues.
- Document exceptions in function signatures: Clearly state which functions may throw exceptions in order to inform users of your code about the potential for errors.
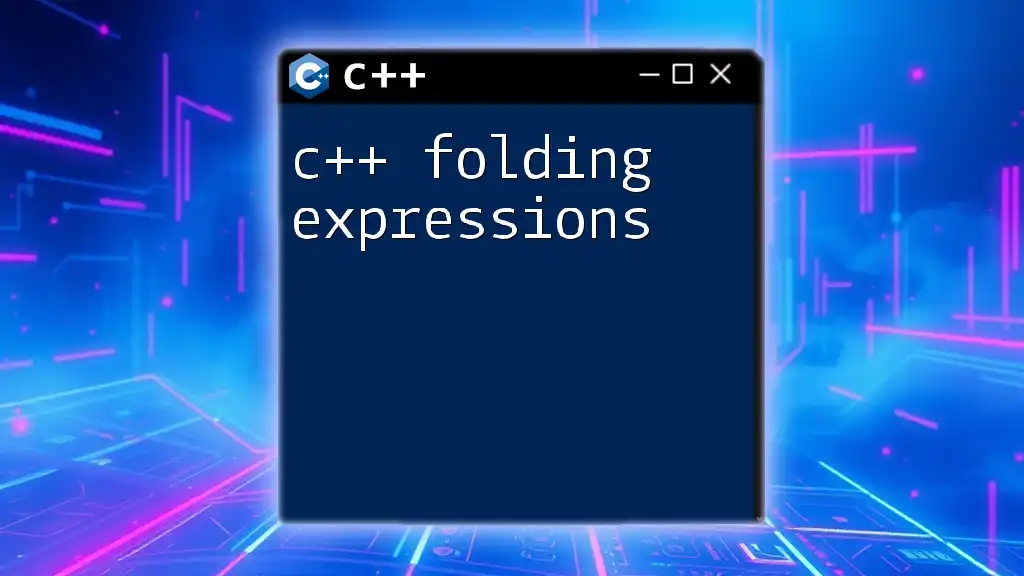
Conclusion
C++ exception handling is a powerful tool that, when used correctly, can significantly enhance the robustness of your applications. By managing runtime errors effectively, you not only improve the user experience but also maintain cleaner and more maintainable code.
Encouraging hands-on practice and exploration will allow you to master the art of catching exceptions in C++, turning potential problems into manageable code paths. A well-implemented exception handling strategy can differentiate between a crashing application and a responsive, resilient one.