In C++, rethrowing an exception allows you to catch an exception and then pass it up the call stack without losing the original error context, as shown in the following example:
#include <iostream>
#include <stdexcept>
void functionA() {
throw std::runtime_error("Original Exception");
}
void functionB() {
try {
functionA();
} catch (...) {
std::cout << "Caught exception in functionB, rethrowing..." << std::endl;
throw; // Rethrow the caught exception
}
}
int main() {
try {
functionB();
} catch (const std::runtime_error& e) {
std::cout << "Caught rethrown exception: " << e.what() << std::endl;
}
return 0;
}
Understanding Exceptions in C++
What is an Exception?
An exception is an unexpected or exceptional event that occurs during the execution of a program. In C++, exceptions are used to manage errors gracefully without crashing the program. They allow developers to separate error-handling code from regular code, leading to cleaner and more maintainable applications.
There are two main types of exceptions:
- Standard exceptions: Provided by the standard library, such as `std::runtime_error`, `std::logic_error`, etc.
- Custom exceptions: User-defined exceptions created for specific application logic.
The Exception Handling Mechanism
In C++, exception handling is achieved using the `try`, `catch`, and `throw` keywords.
- Try Block: Wraps code that might potentially throw an exception.
- Catch Block: Specifies how to handle a particular type of exception thrown by the try block.
The order of catch blocks matters; if multiple catch statements exist, the compiler will check them in the order they appear. The first matching catch will handle the exception.
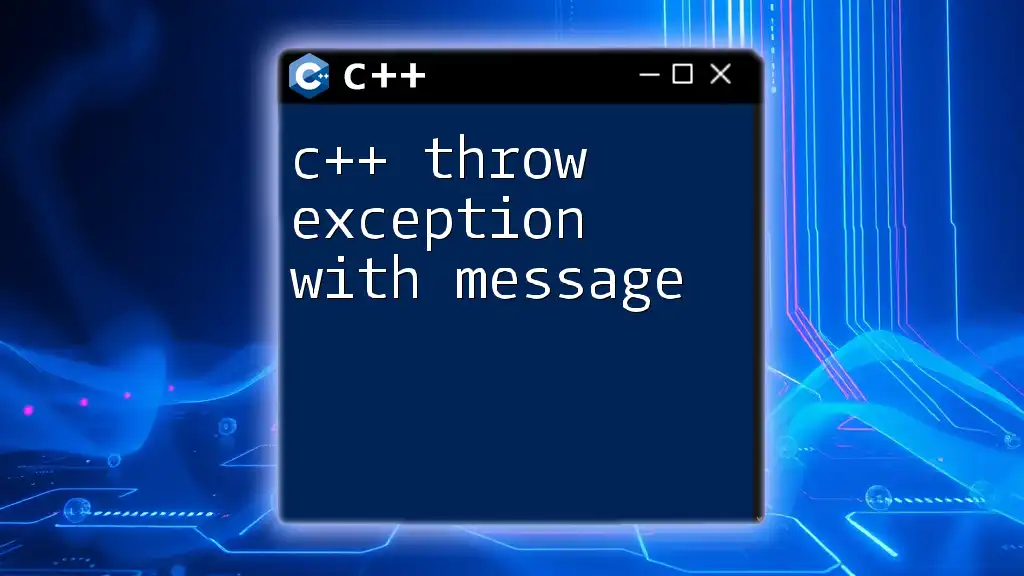
The Concept of Rethrowing Exceptions
What Does Rethrowing Mean?
Rethrowing an exception allows a function that has caught an exception to pass it along to the next layer of code. This is crucial for context preservation. If a catch block can provide additional context or handling, it can rethrow the original exception or throw a new one that encapsulates the original exception.
When to Rethrow an Exception
- Preserving Context: Often, the context in which an exception occurs can be as important as the exception itself. By rethrowing, you can maintain this context for better debugging or logging.
- Adding Additional Information: If you catch an exception and recognize it could benefit from additional context, rethrowing allows you to enrich the exception before passing it.
Syntax of Rethrowing Exceptions
You can rethrow an exception in two main ways:
- Using `throw;` without any arguments, which rethrows the currently handled exception.
- Using `throw exception;` to throw a new exception, potentially with a modified message or type.
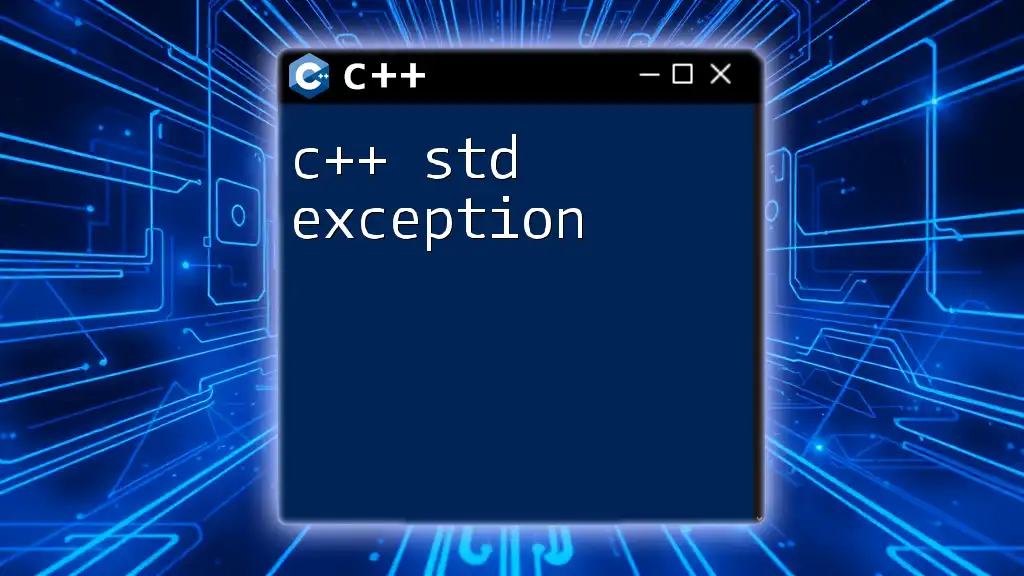
How to Rethrow Exceptions in C++
Basic Example of Rethrowing an Exception
Here’s a straightforward example demonstrating the concept of rethrowing.
try {
// Code that may throw exceptions
throw std::runtime_error("An error occurred");
} catch (const std::exception& e) {
std::cerr << "Caught an exception: " << e.what() << std::endl;
throw; // Rethrowing the same exception
}
In this snippet, if an exception (`std::runtime_error`) is thrown, it will be caught as a `std::exception`, logged, and then rethrown so that it can be handled by another layer of code.
Advanced Example: Adding Context
You may want to provide more context when rethrowing an exception. Here’s how to do it effectively:
try {
// Code that may throw exceptions
throw std::runtime_error("An error occurred");
} catch (const std::runtime_error& e) {
std::cerr << "Caught a runtime_error: " << e.what() << std::endl;
throw std::runtime_error("Context: " + std::string(e.what())); // Rethrowing with additional info
}
In this scenario, if a `std::runtime_error` is caught, the code adds context before rethrowing a new `std::runtime_error`, thus providing more information for the eventual handler.
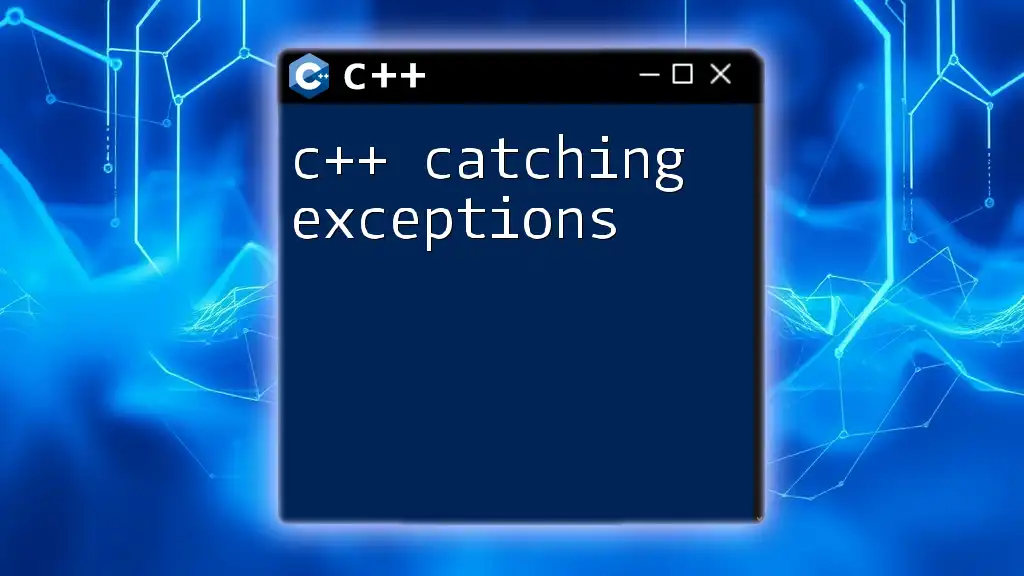
Best Practices for Rethrowing Exceptions
When to Avoid Rethrowing Exceptions
While rethrowing can be valuable, there are situations where it might not be the best choice:
- Performance Considerations: Frequent rethrowing, especially in heavy computational loops, could incur performance penalties.
- Multiple Layers of Catch Blocks: If the same exception is rethrown multiple times down nested catch blocks, it can lead to confusion and obfuscate the original source of the error.
Using `std::exception_ptr` for Advanced Exception Handling
For cases where you need to capture and rethrow exceptions without losing context, C++ provides `std::exception_ptr`. This facilitates safer error handling across threads.
Capture and Rethrow Example
std::exception_ptr eptr;
try {
// Code that may throw exceptions
} catch (...) {
eptr = std::current_exception(); // Capture the current exception
}
// Later in the code, we can rethrow
if (eptr) {
std::rethrow_exception(eptr); // Rethrow the caught exception
}
This method allows you to defer the handling of an exception, sharing it across threads without losing the original stack trace.
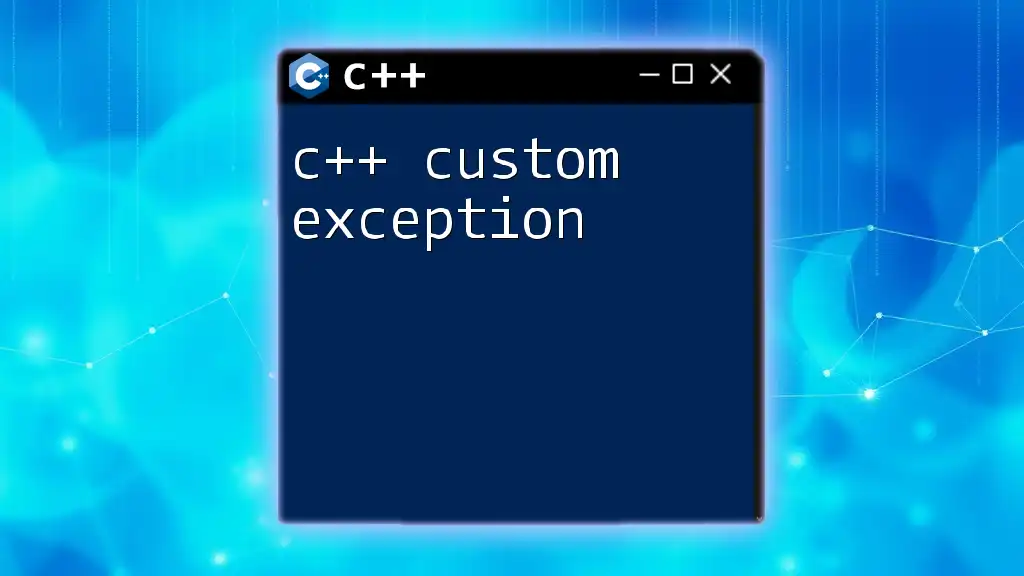
Common Pitfalls in Rethrowing Exceptions
Misunderstanding Rethrow Syntax
A common mistake involves confusing `throw;` with `throw exception;`. Using `throw;` rethrows the current exception, while `throw exception;` creates a new exception.
Losing Stack Trace Information
Improper exception handling might lead to a loss of stack trace information, making debugging difficult. Always aim to rethrow the original exception or enhance it with context to preserve relevant information.
Performance Implications of Deep Nesting
Deeply nested catch blocks can complicate the control flow of handling exceptions. Ensure that layers of exception management are necessary to avoid performance issues.
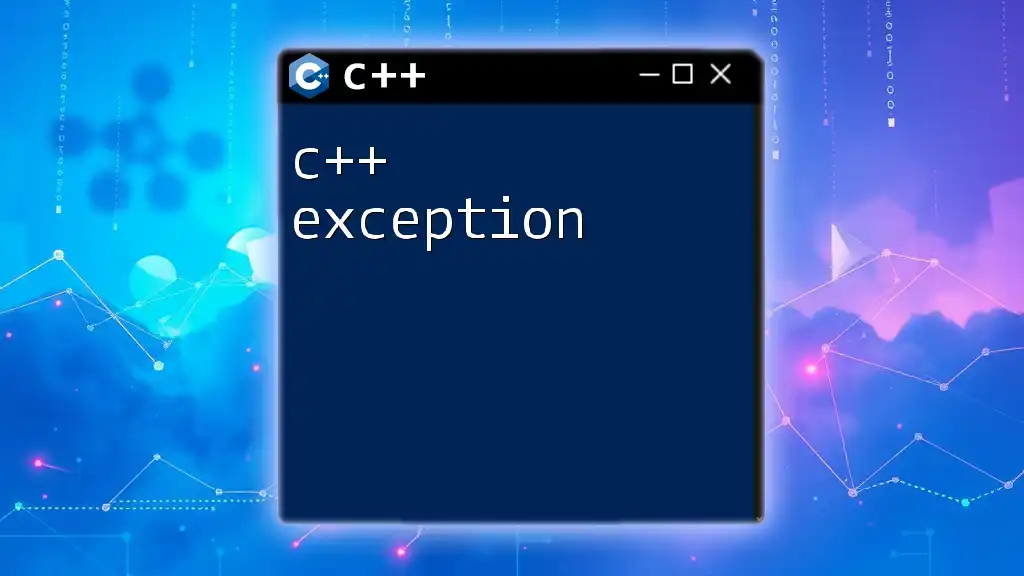
Debugging Rethrown Exceptions
Strategies for Identifying Issues
When debugging issues related to rethrown exceptions, consider:
- Using Debuggers: Step through your code and watch the flow of exceptions.
- Logging: Implement comprehensive logs that capture when exceptions are thrown and caught, along with relevant context.
Example of a Debugging Scenario
try {
// Simulated problematic code that throws an exception
throw std::runtime_error("Simulated error");
} catch (const std::exception& e) {
std::cerr << "Error occurred: " << e.what() << std::endl;
throw; // Rethrowing for outer handling
}
In this scenario, if a simulated error occurs, it gets logged, and the exception is rethrown for possible handling at a higher level, allowing further examination of the issue.
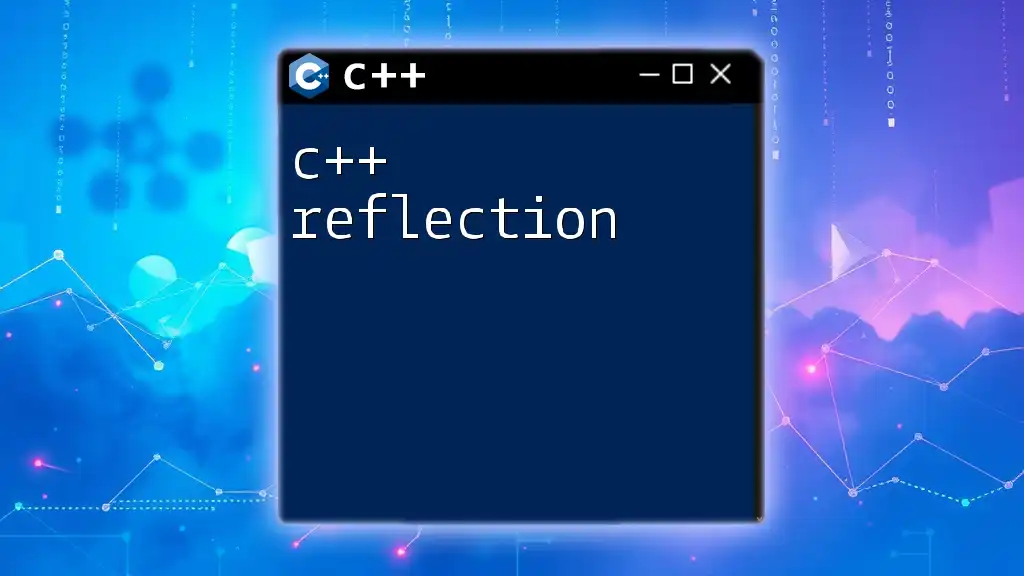
Conclusion
In summary, C++ rethrow exception plays a vital role in effective error handling. Understanding when and how to rethrow exceptions not only improves your application's robustness but also aids in tracing and debugging.
Utilizing rethrowing helps maintain context and create meaningful error messages, enabling better handling of exceptions throughout your code. As you continue exploring C++, practice the examples outlined here to deepen your understanding of this powerful feature.