The `showpoint` manipulator in C++ forces the display of the decimal point and trailing zeros for floating-point numbers, ensuring that they are presented in a consistent format.
Here’s an example code snippet that demonstrates its usage:
#include <iostream>
#include <iomanip>
int main() {
double num1 = 5.0;
double num2 = 6.75;
std::cout << std::showpoint; // Enable showpoint
std::cout << "Number 1: " << num1 << std::endl; // Outputs 5.0
std::cout << "Number 2: " << num2 << std::endl; // Outputs 6.75
return 0;
}
What is the `showpoint` Manipulator?
In C++, the `showpoint` manipulator is used to control the output format of floating-point numbers. It specifically enables the display of the decimal point for numbers that would normally be formatted without one. This is particularly useful in ensuring consistency, especially when dealing with mixed data types in output statements.
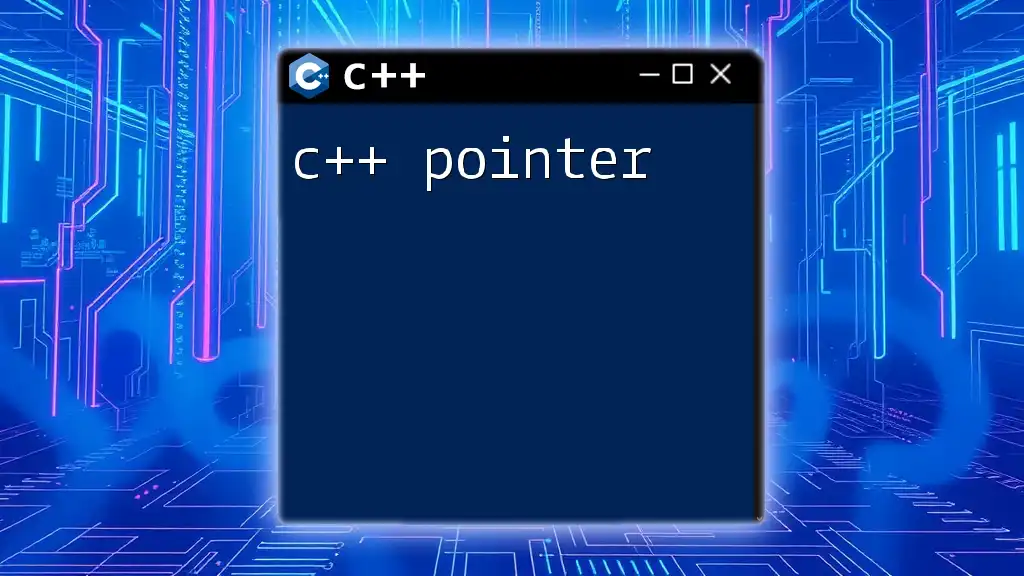
Why Use `showpoint`?
Using `showpoint` can significantly enhance the readability and clarity of your output. Without this manipulator, numbers like `10.0` would simply be displayed as `10`, losing the indication that they are indeed floating-point values. By retaining the decimal, readers can easily identify the type of the number, which is vital when presenting numerical data.
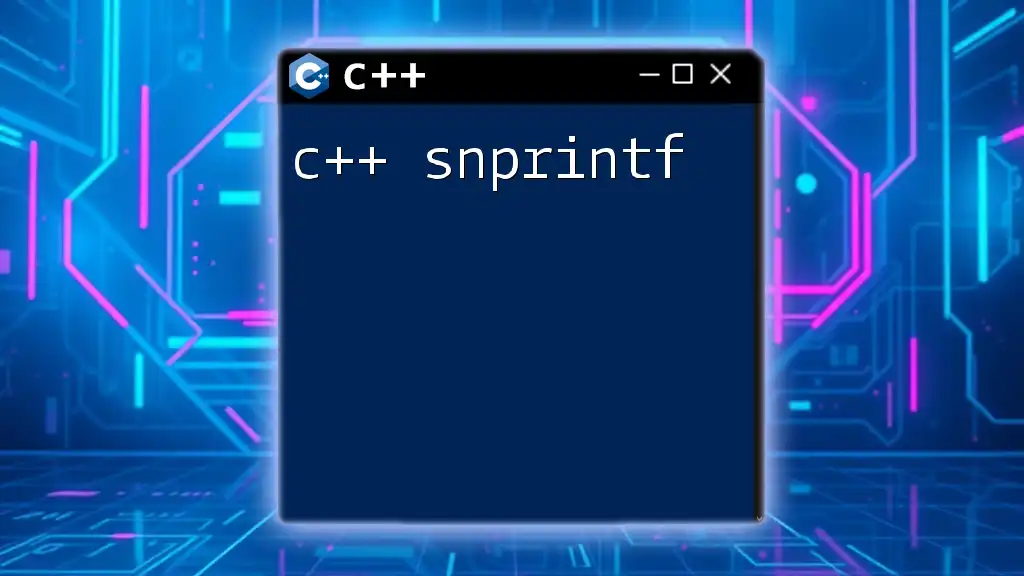
What are I/O Manipulators?
I/O manipulators are special functions that can be included in input/output statements to modify how data is displayed. They play a crucial role in formatting output in a way that is clear and convenient for the user.
Common Manipulators in C++
Some commonly used manipulators in C++ include:
- `fixed`: Displays numbers in fixed-point notation.
- `scientific`: Displays numbers in scientific notation.
- `setprecision`: Sets the number of digits to be displayed after the decimal point.
These manipulators can be combined with `showpoint` to create tailored output formats.
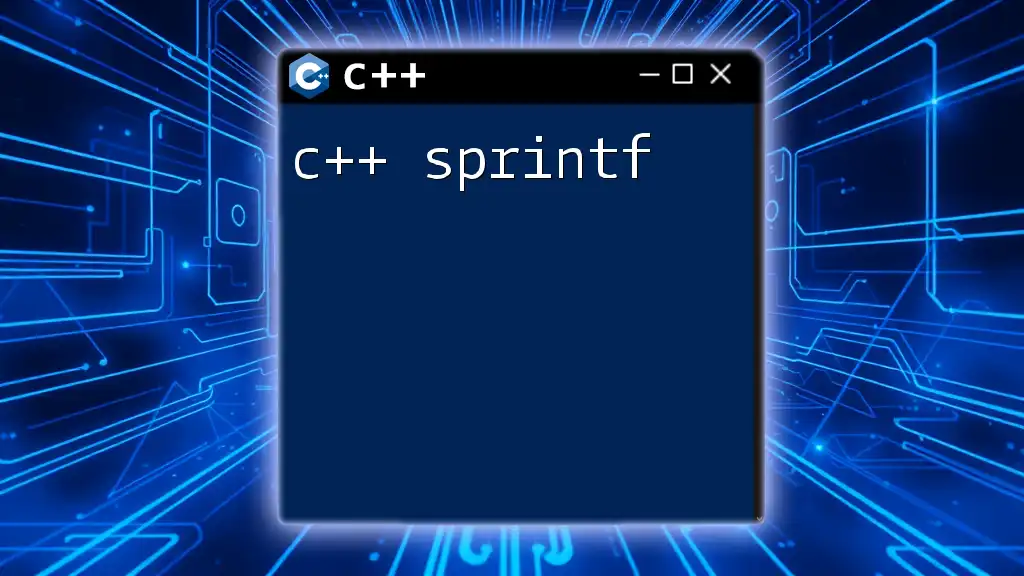
Functionality of `showpoint`
The fundamental role of `showpoint` is to force the output of floating-point numbers to show a decimal point, even if the number is a whole number. This ensures that the type information is visible.
Syntax of `showpoint`
Here's a simple example illustrating the syntax for using `showpoint`:
#include <iostream>
#include <iomanip> // for std::showpoint
int main() {
double num = 123.0;
std::cout << std::showpoint << num; // Outputs: 123.000000
return 0;
}
In this example, without the `showpoint` manipulator, the output would have simply been `123`.
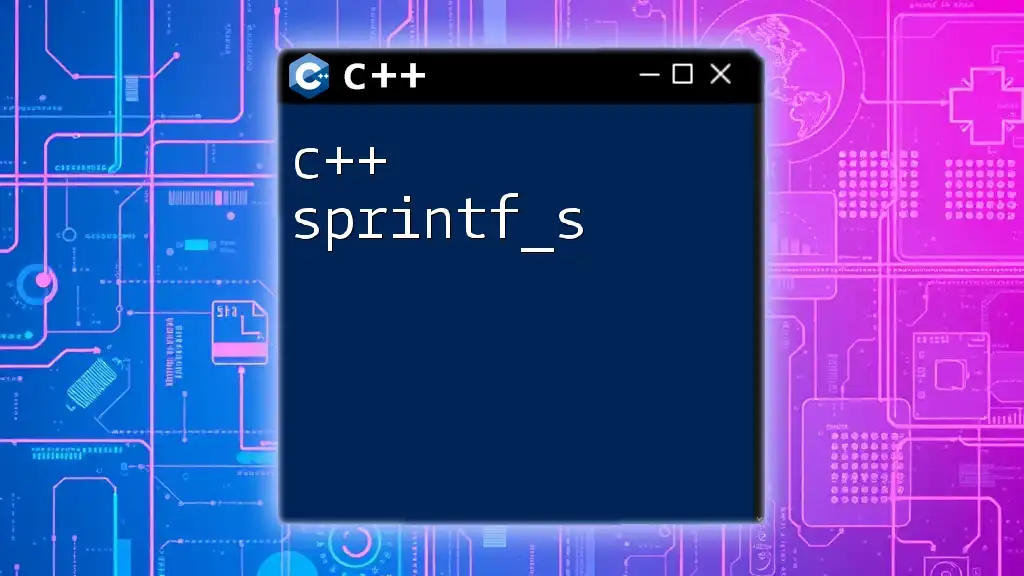
When to Use `showpoint`
Using `showpoint` is particularly beneficial in the following scenarios:
- Reports and Financial Statements: When displaying financial figures that require clarity in decimal points.
- Scientific Calculations: When precision is crucial, and one must ensure that outputs are interpreted correctly.
Example Scenarios
Here’s a code snippet demonstrating typical use cases of `showpoint`:
#include <iostream>
#include <iomanip>
int main() {
double value1 = 10.0;
double value2 = 20.12345;
// Without showpoint
std::cout << "Without showpoint:\n";
std::cout << value1 << "\n" << value2 << "\n";
// With showpoint
std::cout << std::showpoint; // Enable showpoint
std::cout << "With showpoint:\n";
std::cout << value1 << "\n" << value2 << "\n";
return 0;
}
In this example, the use of `showpoint` changes the output of `value1` from `10` to `10.000000`, fostering clarity.
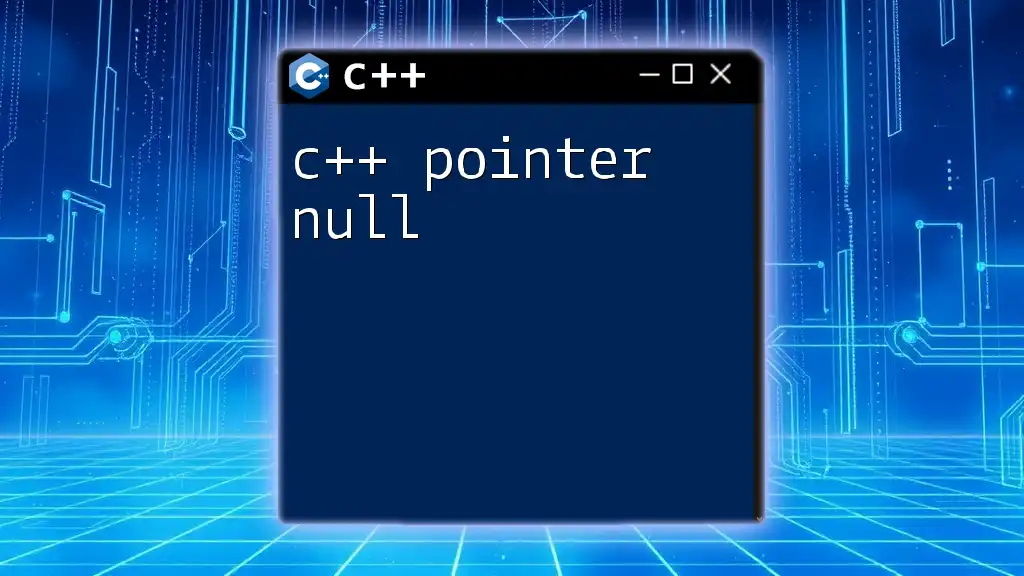
Using `showpoint` with `fixed` and `scientific`
The `showpoint` manipulator can be combined with other manipulators to achieve desired formatting. For instance, integrating it with `fixed` or `scientific` allows for precise control over how the output is displayed.
Example of Combined Use
#include <iostream>
#include <iomanip>
int main() {
double num = 1.23e2;
std::cout << std::showpoint << std::fixed << num << "\n"; // Use fixed format
std::cout << std::showpoint << std::scientific << num << "\n"; // Use scientific format
return 0;
}
This code will show the number in both fixed-point and scientific formats, demonstrating the flexibility of `showpoint` in making the output visually coherent.
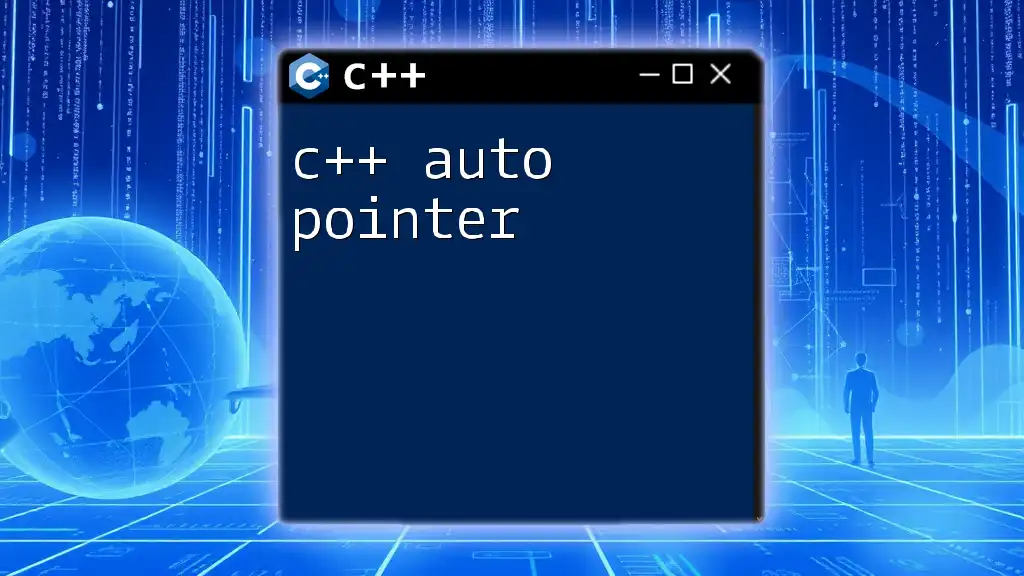
Enhanced Output Clarity
One of the primary advantages of employing `showpoint` is the clarity it brings to numerical data. Numbers that might otherwise appear ambiguous are clearly formatted, allowing for easier interpretation.
In financial reporting, for example, having a consistent display of numbers such as currencies ensures that users do not misread values due to lack of a decimal point.
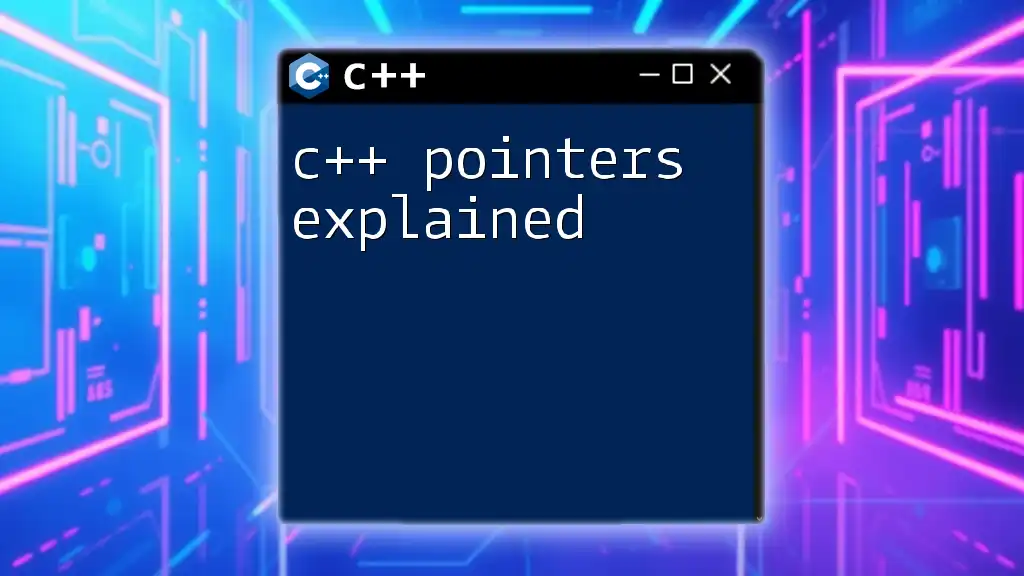
Limitations and Considerations
While `showpoint` is a powerful tool for formatting, there are certain considerations:
When Not to Use `showpoint`
Using `showpoint` may not be suitable in all contexts. For instance, when outputting results where decimal precision is irrelevant, adding unnecessary zeros could lead to confusion. Avoid it in cases where conciseness is more important than clarity.
Impact on Performance
Although the performance impact is generally negligible in most applications, excessive use of multiple manipulators can lead to less efficient code. Be mindful of your formatting, and only use `showpoint` when necessary.
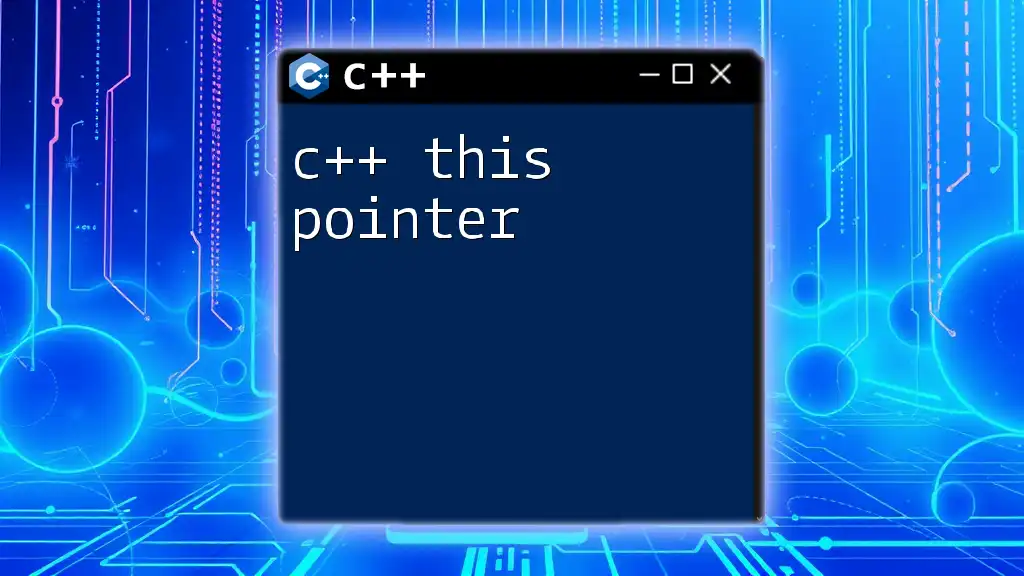
Recap of `showpoint` Importance
In summary, understanding and effectively using the `showpoint` manipulator enhances the clarity of floating-point output in C++. It serves as a straightforward yet impactful way of indicating numerical types, encouraging precision and readability in various applications.
Call to Action
Try incorporating `showpoint` into your own C++ code. Experiment with different manipulators to see how they affect your output, and share your experiences with others seeking to enhance their C++ formatting skills.
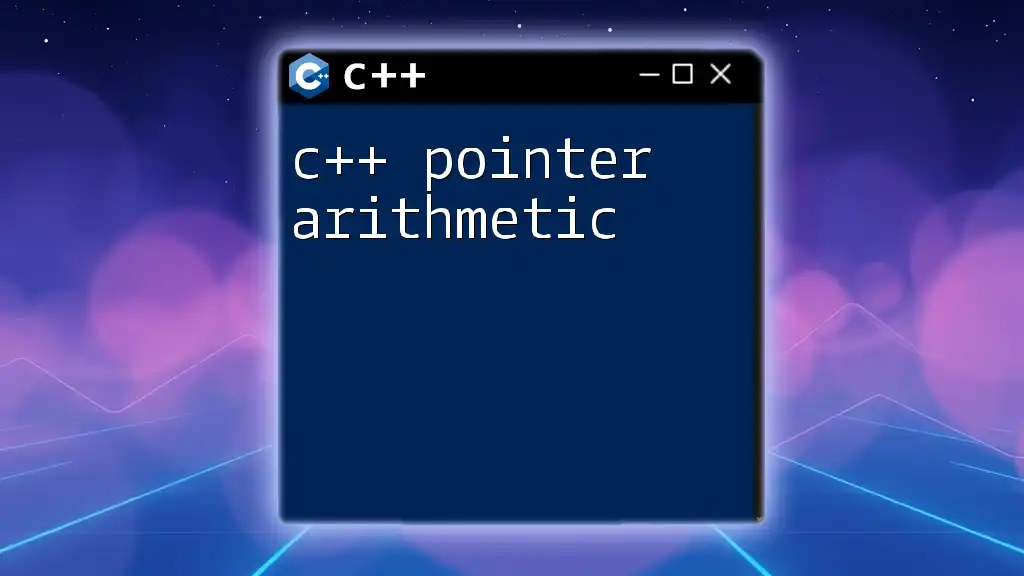
Further Learning Materials
For those interested in continuing their education in C++ programming and formatting techniques, consider exploring books and online tutorials focusing on I/O manipulations in C++. Engaging in community forums can also provide valuable insights from fellow developers seeking to master these skills.