The `fprintf` function in C++ is used to output formatted data to a specified file stream, allowing for precise control over the text and its layout.
#include <cstdio>
int main() {
FILE *file = fopen("output.txt", "w");
if (file != NULL) {
fprintf(file, "Hello, %s! You have %d new messages.\n", "Alice", 5);
fclose(file);
}
return 0;
}
What is `fprintf`?
The `fprintf` function in C++ is a powerful tool used for formatted output to a file or output stream. It belongs to the family of `printf` functions, which handle formatted output, but with a specific focus on writing to file streams instead of the standard output (console). Understanding `fprintf` is crucial for anyone looking to handle file I/O effectively in their applications.
In comparison to other output functions, `fprintf` provides a way to format strings in a highly customizable manner, enabling the inclusion of multiple data types in a single output statement. This versatility is vital in situations where you need to present data in a structured way.
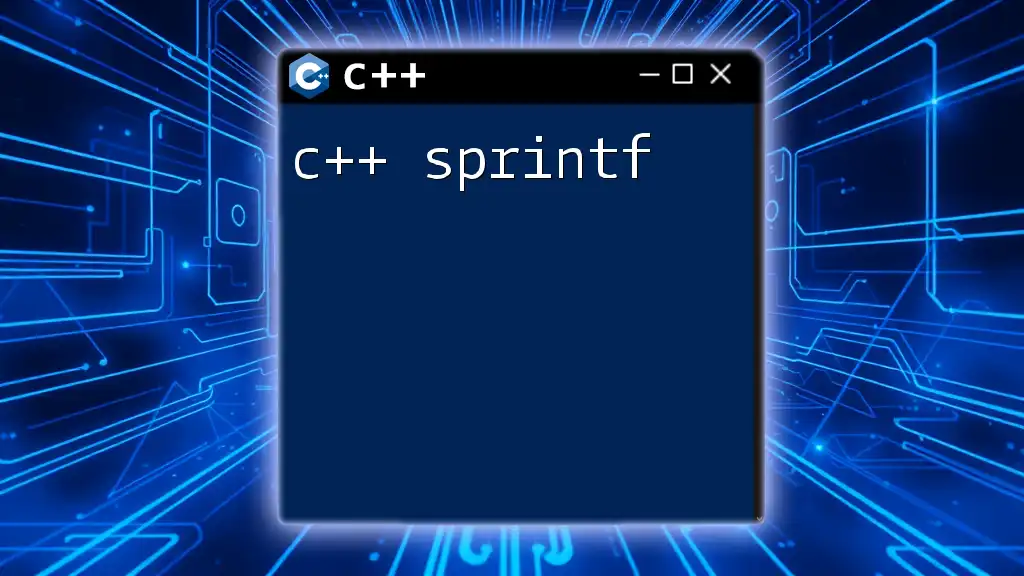
Importance of Formatted Output
Formatted output plays a significant role in programming as it enhances the readability and presentation of data. When dealing with files, especially when generating reports or logging information, the ability to format the output can be the difference between clear communication and disorganization.
Real-world applications of `fprintf` include:
- Logging: Writing debug information into log files.
- Data export: Generating CSVs or other forms of structured textual data for applications.
- Reports: Creating formatted reports that capture essential data in a readable format.
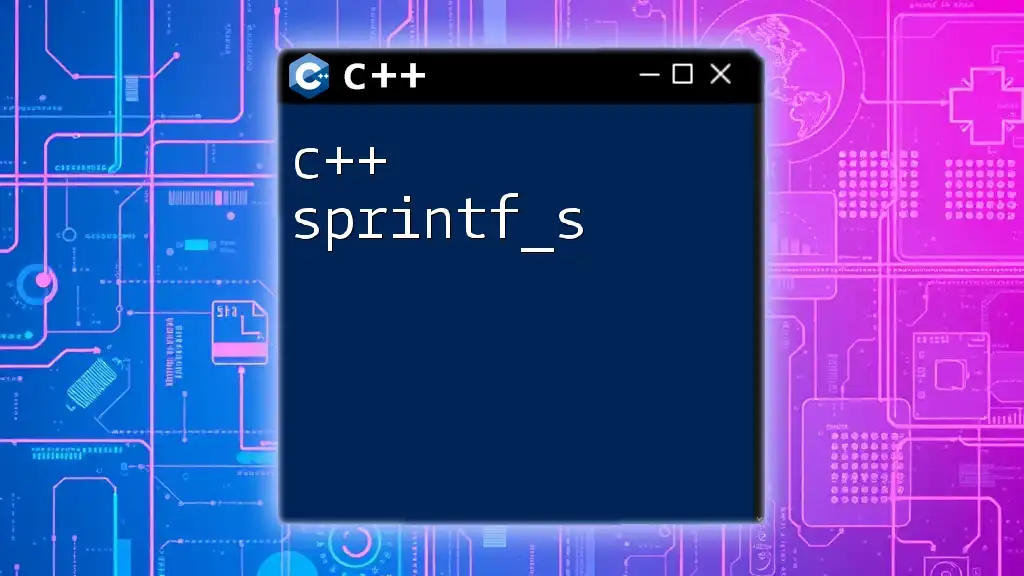
Understanding the Syntax of `fprintf`
The basic syntax of the `fprintf` function is:
fprintf(file_pointer, format_string, value1, value2, ...);
Parameters Explained
File pointer: This parameter points to the file where the output will be directed. It is essential to acquire a valid file pointer using the `fopen()` function, which opens the file for reading or writing.
Format string: The format string contains text and format specifiers (like `%d` for integers, `%f` for floating-point numbers, and `%s` for strings). The format specifiers dictate how the subsequent arguments are to be formatted when written to the output.
Additional arguments: These are the variables whose values are inserted into the format string. You can pass multiple variables to create a structured output.
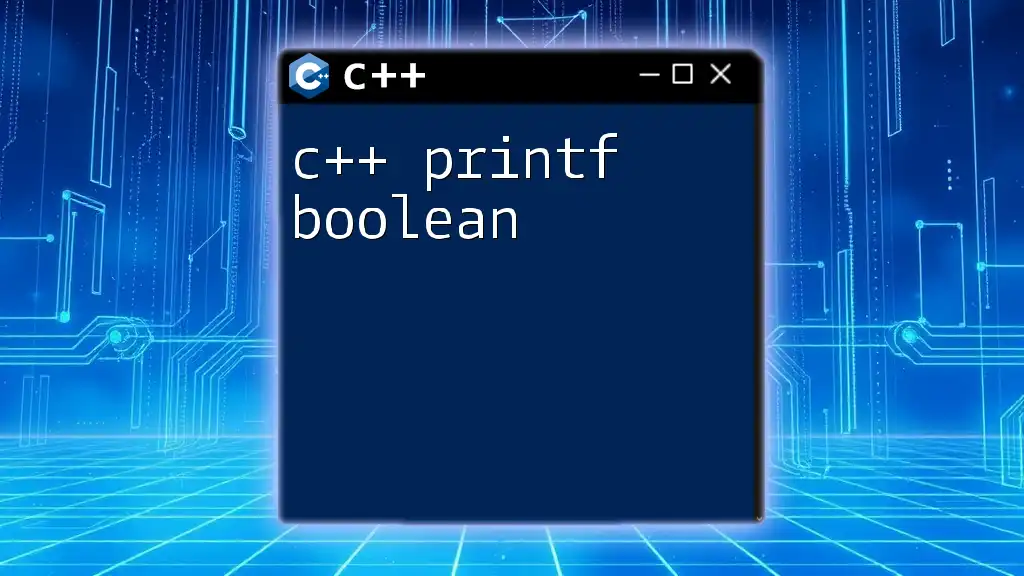
The Format Specifiers
Common format specifiers include:
- Integers: Use `%d` or `%i` to format integer values.
- Floating-point numbers: Use `%f` for fixed-point notation, `%e` for scientific notation, and `%g` for the most compact representation.
- Strings: Use `%s` to display strings.
Formatting Options
In addition to the basic specifiers, you can control the appearance of the output using formatting options such as width and precision modifiers. Flags like `-`, `+`, `0`, and `#` further customize how your output is displayed, making it more user-friendly and visually appealing.
For instance, you can specify width to ensure numbers align nicely:
fprintf(file, "%10d %5.2f\n", 123, 456.789);
In this example, the integer will be right-aligned within a width of 10, and the floating point will display two decimal places within a width of 5.
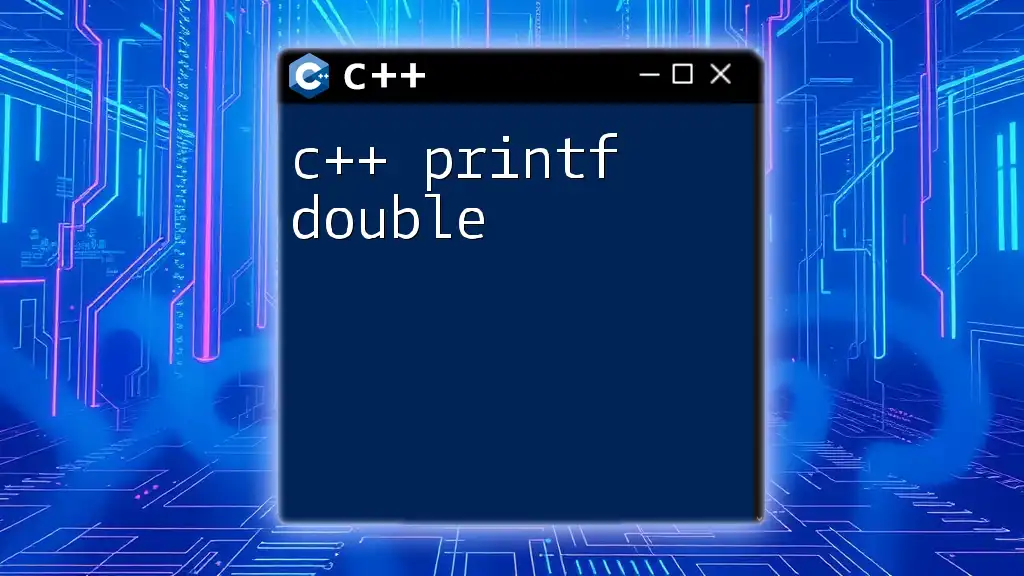
File I/O Operations Using `fprintf`
Opening a File for Writing
Before you can use `fprintf`, you must open a file using the `fopen()` function. Choose the appropriate mode based on your needs, such as `"w"` for writing new content or `"a"` for appending to existing content.
Writing to a File
To write formatted data to a file, use `fprintf` in the following manner:
FILE *file = fopen("output.txt", "w");
if (file != NULL) {
fprintf(file, "Hello, World! Number: %d\n", 42);
fclose(file);
}
In this snippet, we open a file called `output.txt`, write a formatted string that includes an integer, and then close the file. Ensuring that the file pointer is valid is crucial to prevent runtime errors.
Handling Errors
When using `fprintf`, be aware of potential errors. For example, trying to write to a file that hasn’t been opened properly will cause the program to fail. Always check the validity of the file pointer before attempting operations.
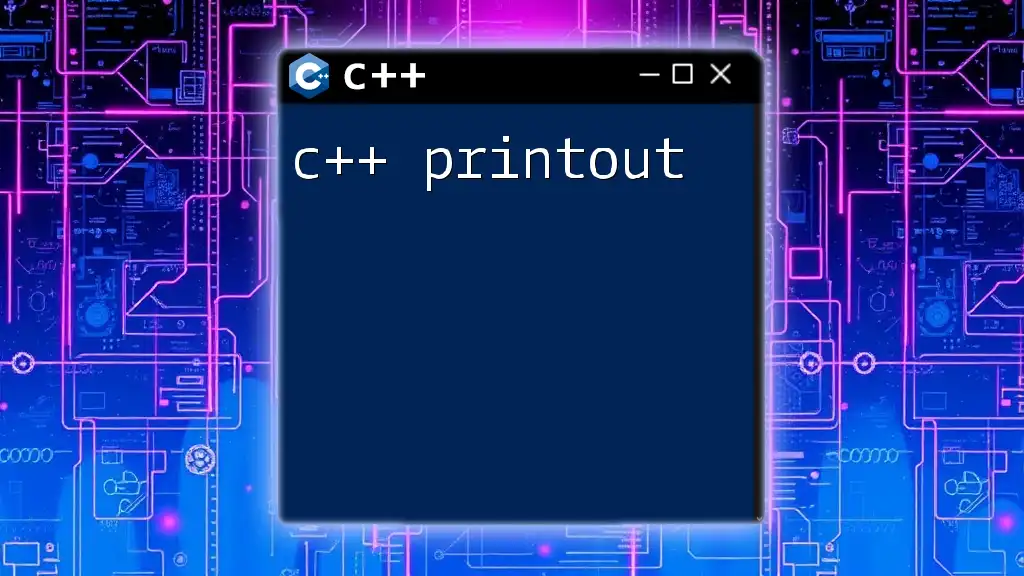
Advanced Usage of `fprintf`
Custom Formatting
One of the powerful features of `fprintf` is its ability to format custom data types such as structures. For instance:
struct Person {
char name[50];
int age;
};
Person p = {"Alice", 30};
FILE *file = fopen("output.txt", "a");
if (file) {
fprintf(file, "Name: %s, Age: %d\n", p.name, p.age);
fclose(file);
}
In this example, we define a `Person` structure and write its attributes to a file using `fprintf`.
Formatted Output in Console vs. Files
While you can use `fprintf` to send formatted output to both the console and files, the nuances differ. For console output, you may use `printf`; however, `fprintf` provides the flexibility to log or store output in files as needed. This is particularly useful for debugging and creating logs, where preserving output is essential.
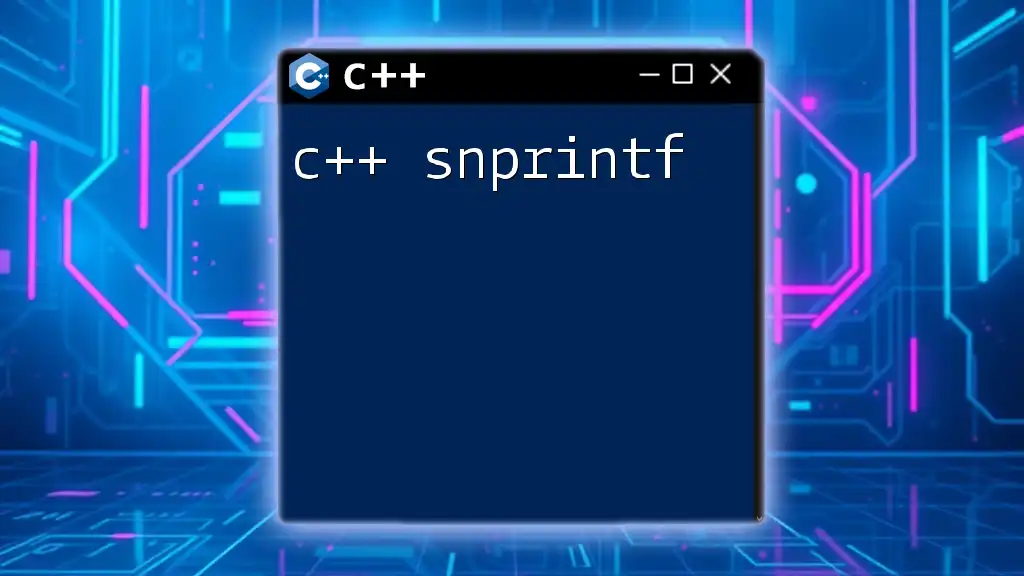
Best Practices for Using `fprintf`
Memory Management
Always ensure proper closure of file pointers when done with writing. Utilize `fclose()` to prevent memory leaks and ensure all data is flushed to disk.
Debugging Output
When debugging, use `fprintf` to print out variable states, errors, or flow control messages. This can save time and clarify the application’s functioning.
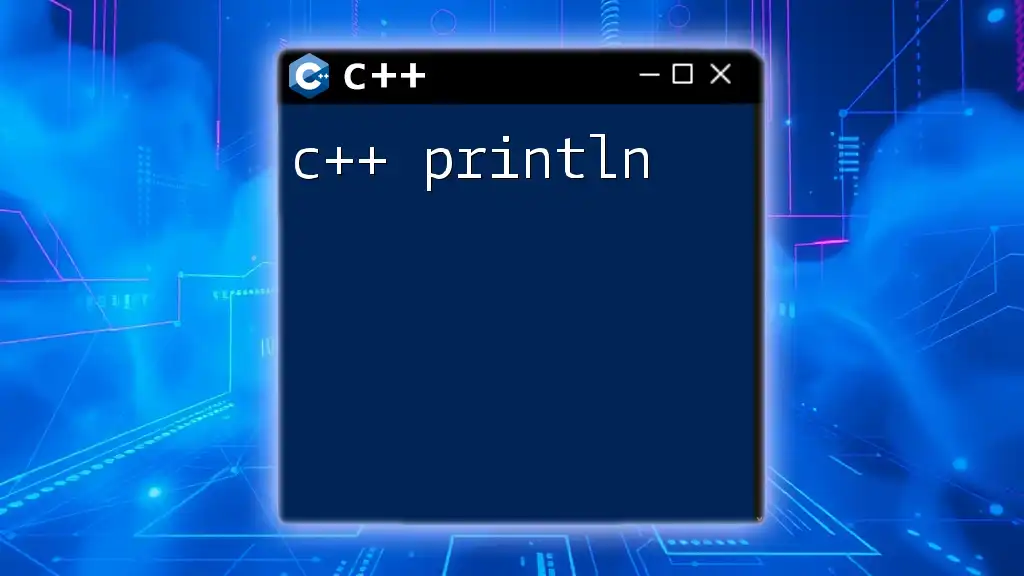
Conclusion
The use of `fprintf` in C++ is an indispensable skill for programmers who wish to manage formatted file I/O effectively. By understanding its syntax, format specifiers, and error handling, you can produce clean, readable files for various applications. Emphasizing best practices will enhance code reliability and maintainability.
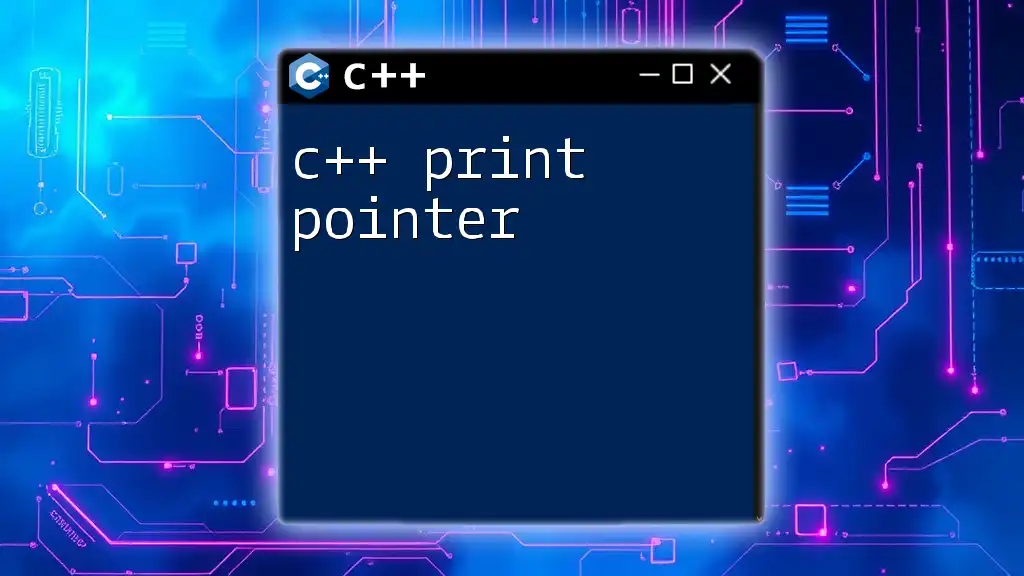
Frequently Asked Questions (FAQs)
What is the difference between `printf` and `fprintf`?
`printf` outputs to the console, while `fprintf` directs output to a specified file stream.
Why is it necessary to close a file after writing?
Closing a file frees up system resources and ensures that all data is properly written and saved.
Can I use `fprintf` without opening a file?
No, you must first open a file and obtain a valid file pointer before writing with `fprintf`.