The `printf` function in C++ is used to output formatted text to the console, and it can display double precision floating-point numbers using the `%f` format specifier.
#include <stdio.h>
int main() {
double num = 3.14159;
printf("The value of num is: %.2f\n", num);
return 0;
}
Understanding the `printf` Function
What is `printf`?
The `printf` function is a powerful tool in C++ for generating formatted output. It is derived from the C programming language and is widely used for its flexibility in displaying data. Unlike `cout`, which is part of the C++ Standard Library and relies on operator overloading, `printf` uses a format string to determine how to print each argument.
Why Use `printf` for Doubles?
When handling floating-point numbers, particularly double values, `printf` provides several advantages:
- Precision Control: You can specify the exact number of decimal places, allowing for precise formatting of numerical data.
- Formatted Output: It enables the printing of numbers in various styles, such as fixed-point notation, scientific notation, and more.
- Performance: In some cases, `printf` can outperform C++ streams in terms of speed, especially for large amounts of output.

Syntax of `printf`
Basic Syntax
To use the `printf` function, you need to follow its basic syntax:
printf(format_string, arguments);
The `format_string` contains the specifications for how the arguments will be displayed.
Format Specifiers
Understanding format specifiers is vital for using `printf` effectively. They dictate how each argument should be formatted. For printing double values, the primary specifier is `%f`:
- `%f`: Used for printing a floating-point number.
- `%e`: Displays a number in scientific notation.
- `%g`: Automatically chooses between `f` and `e` based on the value and precision.
Additional Specifiers
Let’s delve deeper into using these specifiers with examples:
double num = 123456789.123456;
// Basic float representation
printf("Default format: %f\n", num);
// Scientific notation
printf("Scientific notation: %e\n", num);
// General format
printf("General format: %g\n", num);
Each of these specifiers formats the double value in a distinct manner, demonstrating `printf`'s versatility.

Printing Double Values
Basic Printing of Doubles
Printing a basic double is straightforward. Here's how to do it:
double num = 123.456;
printf("The number is: %f\n", num);
This will output the floating-point representation of `num` with six decimal places by default.
Controlling Precision
Precision control is essential when you need a specific number of decimal places. You can specify this in the format string using a period followed by a number. For example:
printf("Number with 2 decimal places: %.2f\n", num);
The output of this command will be:
Number with 2 decimal places: 123.46
Field Width and Alignment
Controlling the field width allows you to format output neatly. You can specify the total width of the output, which can help in aligning numbers in a column format. Here’s an example:
printf("Number with width of 10: |%10.2f|\n", num);
This will print the number with a total width of 10 characters, right-aligned:
Number with width of 10: | 123.46|
Printing Scientific Notation
To print double values in scientific notation, use the `%e` specifier. For example:
printf("Scientific notation: %e\n", num);
This outputs:
Scientific notation: 1.234568e+02
Conditional Output with Double Values
Using conditional statements can help format double values dynamically based on their properties. For example:
if (num < 1000) {
printf("Value: %.2f\n", num);
} else {
printf("Value in scientific notation: %e\n", num);
}
This code checks if `num` is less than 1000 and prints it in fixed-point format or scientific notation as necessary.

Common Pitfalls and Best Practices
Common Errors in printf
While `printf` is powerful, it can lead to errors if not used correctly. Some common pitfalls include:
-
Mismatch of Format Specifiers: Using the wrong specifier for the data type can lead to undefined behavior or inaccurate output.
int a = 5; printf("The number is: %f\n", a); // Incorrect usage
-
Forgetting to Include the Correct Format: Always ensure that your format string matches the type of variable passed.
Best Practices for Formatting
To maintain consistency and readability:
- Use Precision Wisely: Avoid excessive precision unless necessary; it can clutter your output.
- Consistent Formatting: Maintain a consistent format across your program to enhance readability.
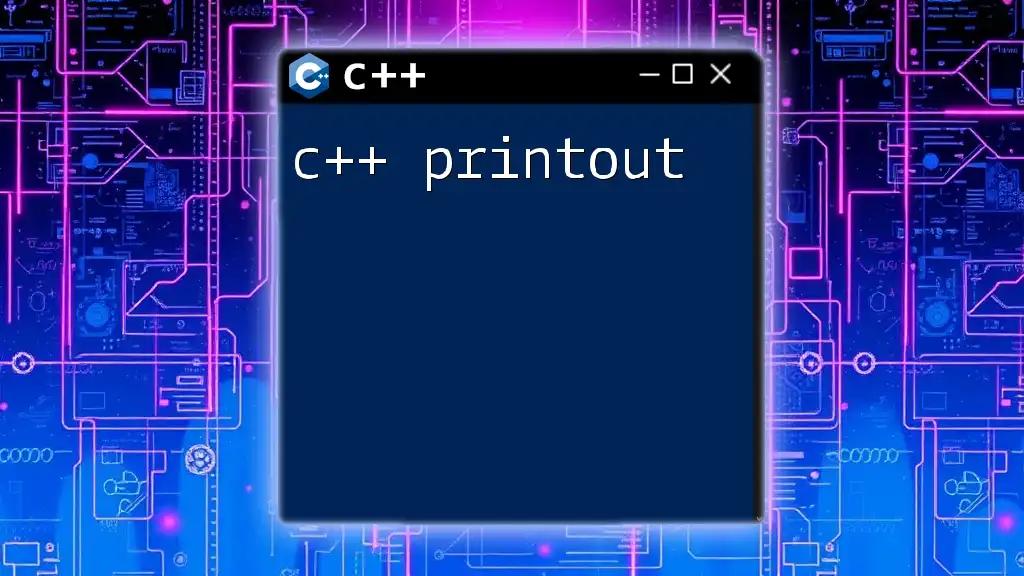
Conclusion
Mastering the `printf` function, especially for double types, can significantly improve your ability to control output formatting in C++. Ensuring precision and alignment can lead to better readability and professionalism in your coding projects.

Additional Resources
For those looking to expand their knowledge further, consider exploring C++ reference manuals or online tutorials focused on advanced formatting techniques. Engaging in community forums can also provide insights and tips from fellow coders.

Frequently Asked Questions
Can `printf` handle all types of floating point values?
Yes, `printf` can handle both `float` and `double` types, although it's preferable to use `double` since `float` values are automatically promoted to `double` when passed to the function.
What happens if I input a very large double value?
`printf` can handle large double values, but if the value exceeds the floating point limits, it may result in infinite or overflow representations.
When should I prefer `cout` over `printf`?
You might prefer `cout` when working with C++ features that require type safety or when embedding non-numeric data within your output, as it integrates naturally with C++’s object-oriented paradigm.