In C++, `float` and `double` are data types used to represent decimal numbers, with `float` offering single-precision (typically 32 bits) and `double` providing double-precision (typically 64 bits) for greater accuracy.
Here's a code snippet demonstrating their usage:
#include <iostream>
using namespace std;
int main() {
float floatNum = 5.75f; // Single precision
double doubleNum = 5.75; // Double precision
cout << "Float: " << floatNum << endl;
cout << "Double: " << doubleNum << endl;
return 0;
}
Understanding Floating-Point Representation
What are Float and Double?
In C++, float and double are two data types used to represent floating-point numbers, which are numbers that can have decimal points. The float data type is typically used when you need to save memory and when precision is less critical, while double is used when dealing with a wider range of values or when higher precision is required.
Key Differences between float and double:
-
Precision:
- Float provides about 7 decimal places of precision.
- Double provides about 15-16 decimal places of precision.
-
Memory Size:
- Float takes up 4 bytes.
- Double takes up 8 bytes.
This distinction makes choosing the right type important depending on the requirements of your application.
Memory Allocation
Understanding memory allocation for these types clarifies their fundamental differences.
- A float occupies 4 bytes in memory. This means it can store less information than a double, limiting its use in high-precision calculations.
- A double, with its 8 bytes of memory, can hold much larger numbers and offers more precision.
This added precision is critical when you’re performing calculations needing high accuracy, such as in scientific simulations.
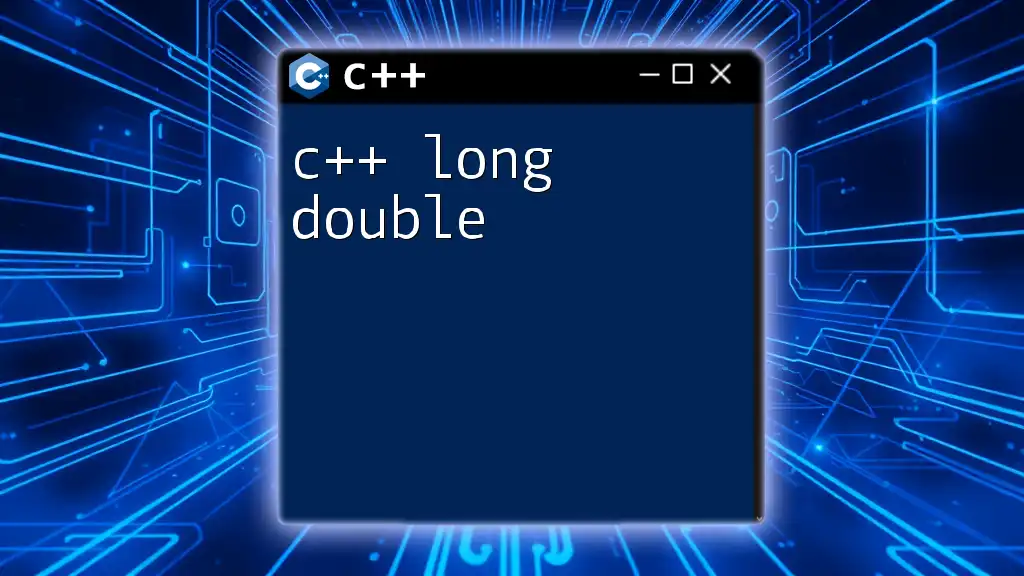
Theoretical Background
Floating-Point Precision
Floating-point arithmetic can introduce complexities due to rounding errors and precision loss.
- Significant Digits: The number of significant digits in a floating-point number indicates its precision. While float can handle a limited set, double allows for more extensive computations due to its higher significant digits count.
The internal representation of floating-point numbers consists of a mantissa (the significant digits) and an exponent (which scales the number). This representation is based on the IEEE 754 standard, widely used in programming languages.
Example of Floating-Point Imprecision:
float sumFloat = 0.1f + 0.2f; // Might not equal 0.3f
double sumDouble = 0.1 + 0.2; // More likely to equal 0.3
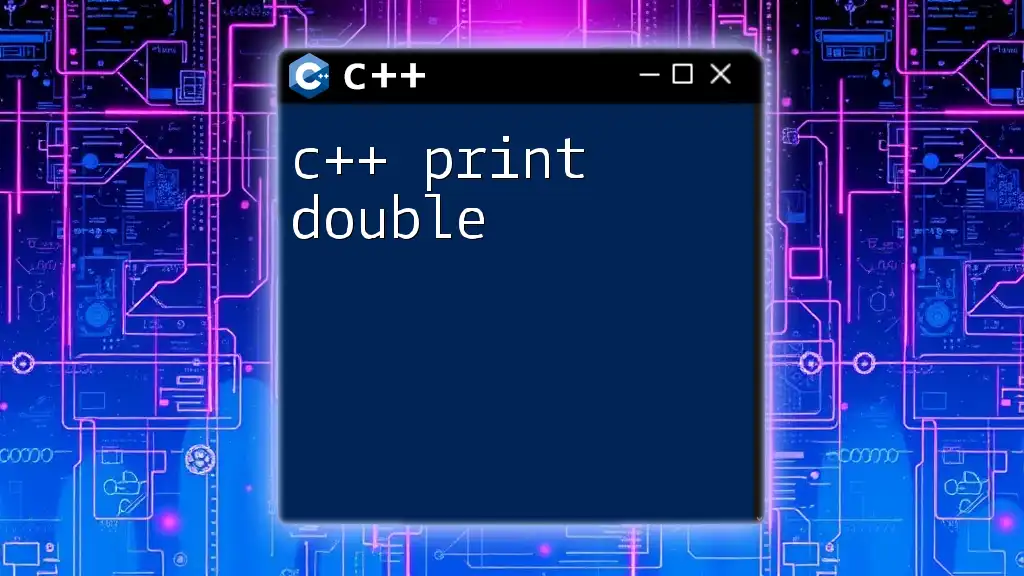
How to Use Float and Double in C++
Declaration and Initialization
Declaring and initializing float and double variables in C++ is straightforward.
Syntax for declaring:
float myFloat = 3.14f; // f suffix indicates a float
double myDouble = 3.14159; // Default is double
Basic Operations
You can perform typical arithmetic operations with both floats and doubles. However, always be cautious about precision:
float resultFloat = myFloat * 2.0f; // Works fine
double resultDouble = myDouble / 2.0; // High precision
Type Conversions
Type conversion allows you to switch between float and double. Be aware that converting from float to double keeps the value same but may result in precision loss when converting from double to float:
double newDouble = static_cast<double>(myFloat);
float newFloat = static_cast<float>(myDouble);
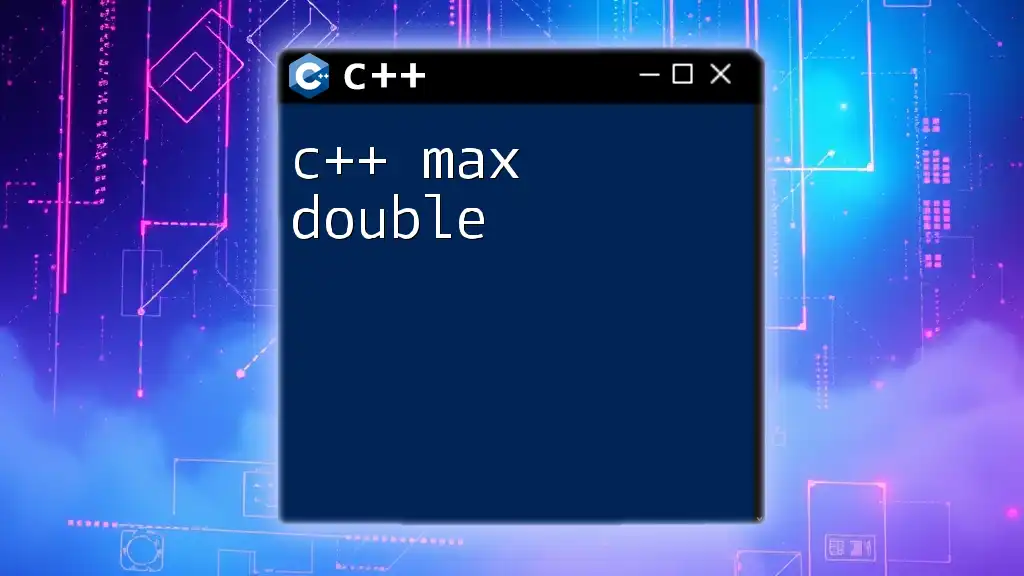
Practical Applications
Use Cases for Float
Using `float` can be ideal in contexts like game development or graphics programming, where speed is crucial, and slight precision loss is tolerable. For example, graphics engines often handle significant numbers of calculations quickly, making `float` the preferred choice.
float x = 1.0f / 3.0f; // Representation in graphics
Use Cases for Double
In contrast, `double` shines in scientific computations, financial calculations, and situations where accuracy is vital. The precision of `double` minimizes the risk of significant errors impacting outcomes.
double gravitationalConstant = 9.80665; // High precision needed
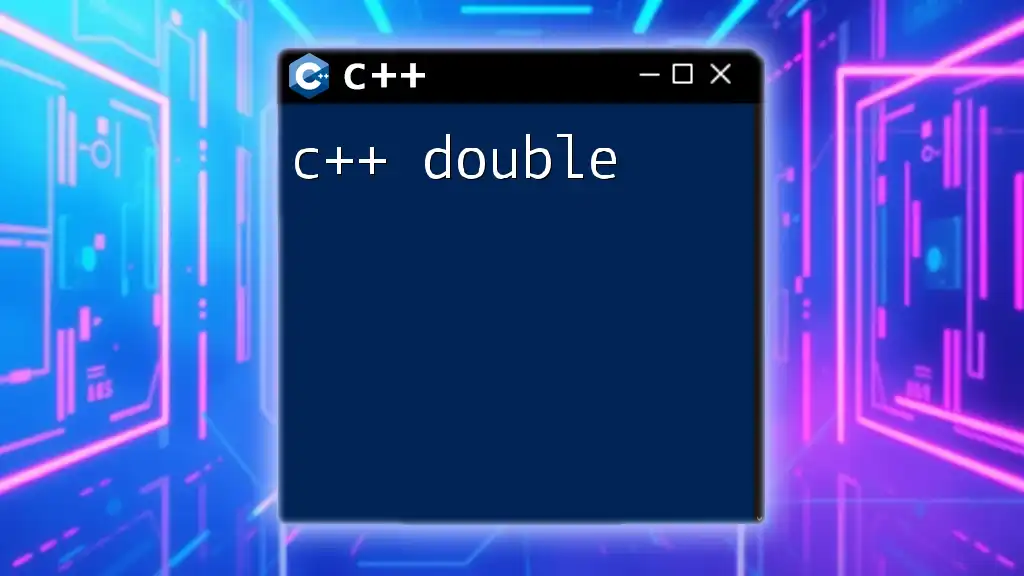
Common Pitfalls
Understanding Precision Loss
A common mistake is assuming that adding small float values will yield a precise result. The following example demonstrates how this can lead to inaccuracies:
float a = 0.1f + 0.2f; // Might not equal exactly to 0.3f
Best Practices to Avoid Issues
- Use double for critical calculations – This minimizes rounding errors.
- Avoid comparing floats directly – Instead, use a tolerance level (epsilon) to determine closeness:
if (fabs(a - b) < epsilon) {
// Considered equal
}
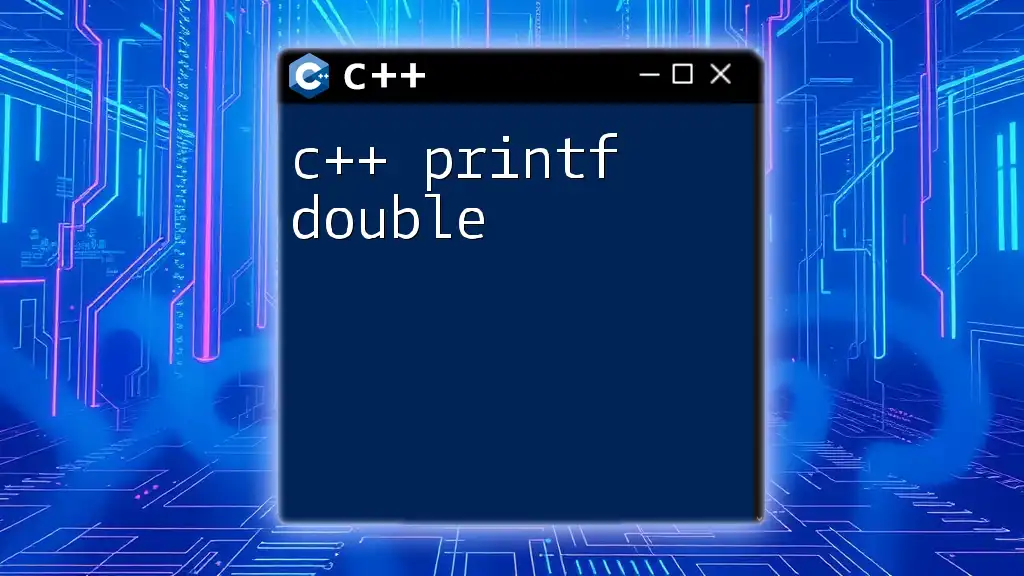
Performance Considerations
Speed vs Precision
While `float` is often faster due to smaller memory and faster processing, double can still be acceptable in many scenarios, especially with modern CPUs. The crucial element is to weigh performance against the required precision.
If the application requires a high rate of calculations but tolerates minor inaccuracies, `float` could be the choice. Conversely, for applications demanding rigorous accuracy, prioritize `double`, even if execution might slow down slightly.
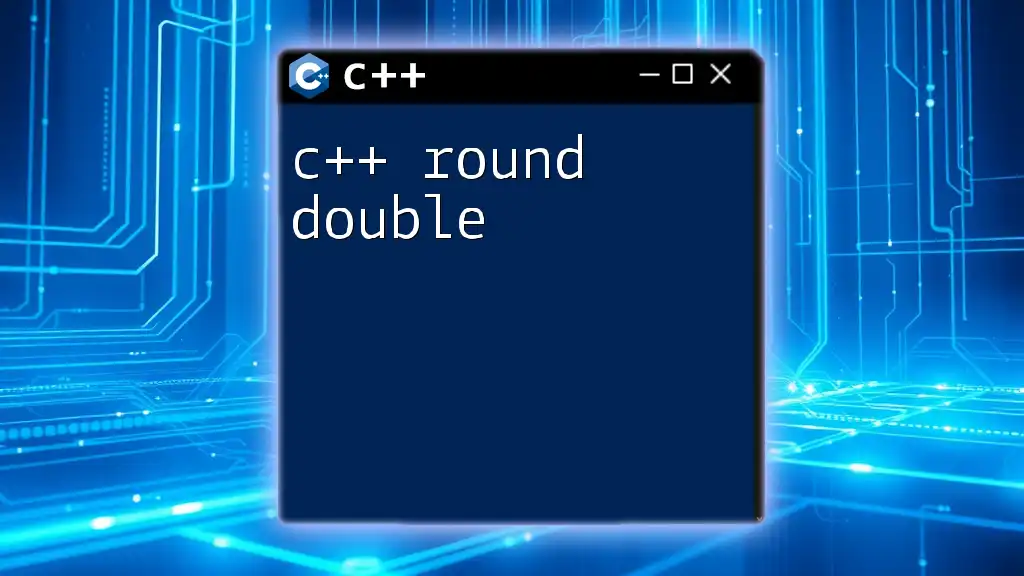
Advanced Topics
Floating-Point Comparisons
Careful comparisons between float and double values are essential to avoid bugs in logic. Logical equality may not yield the expected results due to precision loss. Instead, consider the following method to perform comparisons:
float a = 0.3f;
float b = 0.1f + 0.2f;
const float epsilon = 0.0001f;
if (fabs(a - b) < epsilon) {
// Considered equal
}
Utilizing epsilon allows for a practical approach to accounting for small errors introduced by floating-point arithmetic.
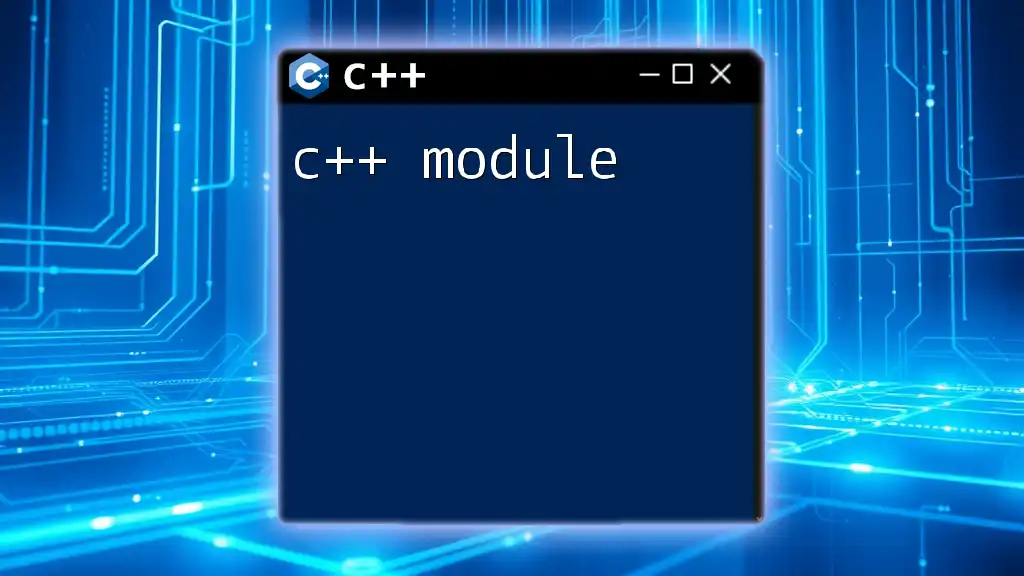
Conclusion
In summary, understanding c++ float double types is critical for effective programming in C++. This comprehensive guide has outlined their differences, appropriate applications, and best practices. By selecting the right type based on precision needs and performance constraints, you can enhance the accuracy and efficiency of your code.
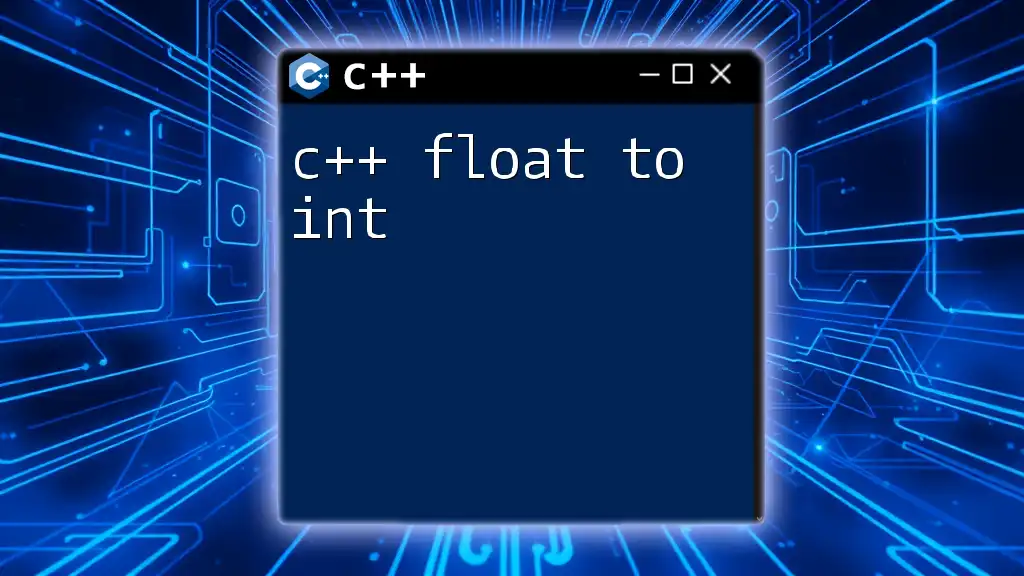
Additional Resources
For further reading and resources, consider exploring the official C++ documentation, textbooks on data types, and online tutorials dedicated to floating-point arithmetic and its implications in programming.
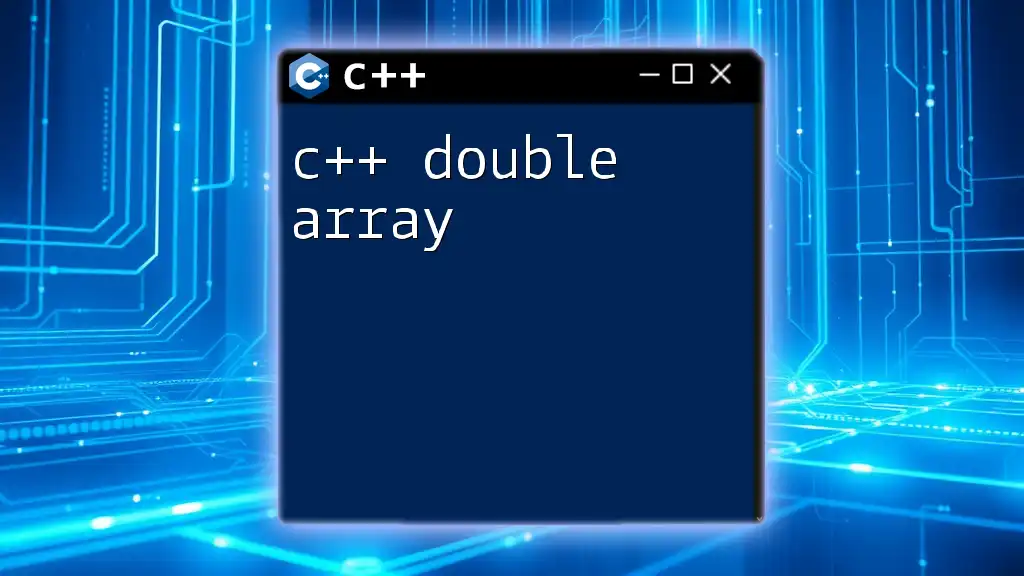
Call to Action
Join our learning community today! We invite you to participate in workshops or join online courses to deepen your understanding of C++ programming. Feel free to leave your comments or questions below; we would love to hear from you!