In C++, a `double` is a data type used to represent floating-point numbers with double precision, allowing for more accurate representation of decimal values. Here's a simple code snippet demonstrating its use:
#include <iostream>
using namespace std;
int main() {
double pi = 3.14159;
cout << "Value of pi: " << pi << endl;
return 0;
}
Understanding C++ Data Types
The Role of Data Types in Programming
In programming, data types define the nature of the data that can be stored and manipulated within a program. They specify the operations that can be performed on the data and the amount of memory allocated to them. Understanding data types is crucial for ensuring efficiency and correctness in your code.
Types of Data Types
In C++, data types are generally categorized into primitive types (such as integers, floats, and characters) and composite types (like arrays and structures). Each type has its own specific properties, including size, value range, and precision.
Overview of Floating-Point Types
Floating-point types are essential for representing real numbers, which include numbers that have fractional parts. In C++, the two primary floating-point types are `float` and `double`.
The key difference between them lies in their precision and memory usage. While a `float` generally provides up to 7 decimal digits of precision, a `double` typically offers between 15 to 17 decimal digits, making it suitable for operations requiring greater accuracy.
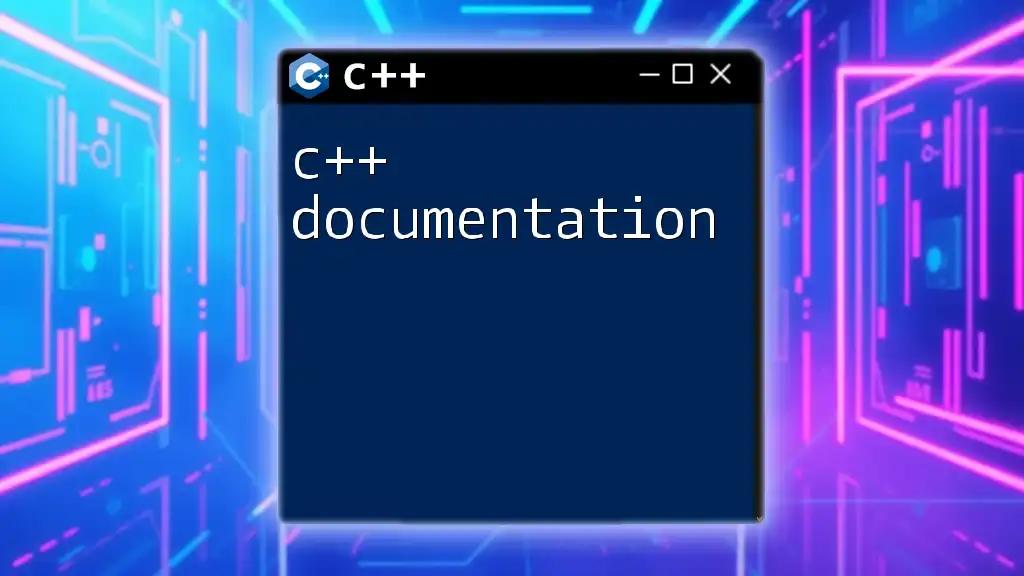
C++ Double: An In-Depth Look
Defining Double in C++
The term double in C++ signifies a data type that allows for the storage of double-precision 64-bit binary values, enabling the representation of vast ranges of numbers with high precision.
To declare a double in C++, you can use the following syntax:
double myDouble; // Declaration
Characteristics of Double Type
A double in C++ is characterized by its enhanced precision and range. It provides a precision of approximately 15-17 decimal places and occupies 8 bytes (64 bits) of memory, enabling programmers to work with very small or very large numbers efficiently.
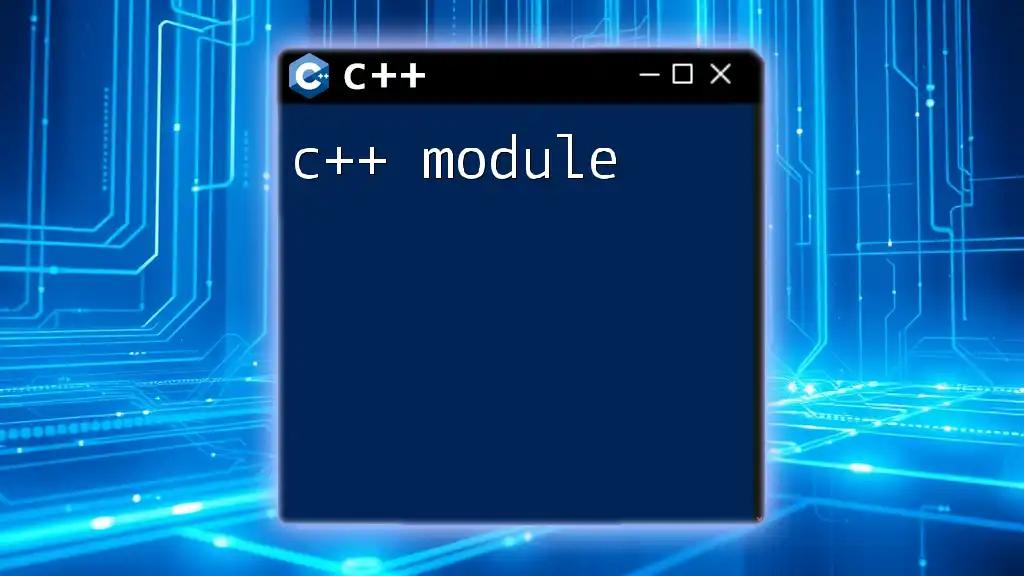
Practical Usage of C++ Doubles
Declaring and Initializing Doubles
Declaring and initializing a double variable is straightforward. You can either declare it without an initial value or assign a value during the declaration. Here’s an example:
double pi; // Declaration without initialization
double pi = 3.141592653589793; // Declaration with initialization
You can also initialize doubles with various values:
double speedOfLight = 299792458.0; // Speed of light in meters per second
Operations with Doubles
Basic Arithmetic Operations
Doubles can be used in arithmetic operations just like other data types. Here are a few examples:
Addition and Subtraction:
double a = 5.5;
double b = 2.2;
double sum = a + b; // sum = 7.7
double difference = a - b; // difference = 3.3
Multiplication and Division:
double product = a * b; // product = 12.1
double division = a / b; // division = 2.5
Complex Operations
To perform more complex mathematical operations, you can utilize the `<cmath>` library, which provides a wide range of functions for scientific calculations.
Here’s an example of calculating the square root:
#include <cmath>
double squareRoot = sqrt(16.0); // squareRoot = 4.0
Type Conversion
In C++, it's crucial to understand type conversion, particularly when working with different data types. You can perform both implicit and explicit conversions.
Implicit Conversion occurs automatically when you assign a value from a smaller data type to a larger one:
int integerNumber = 5;
double convertedDouble = integerNumber; // Implicit conversion
If you need to convert a double back to an integer, you will require explicit conversion:
double myDouble = 9.7;
int myInteger = static_cast<int>(myDouble); // Explicit conversion
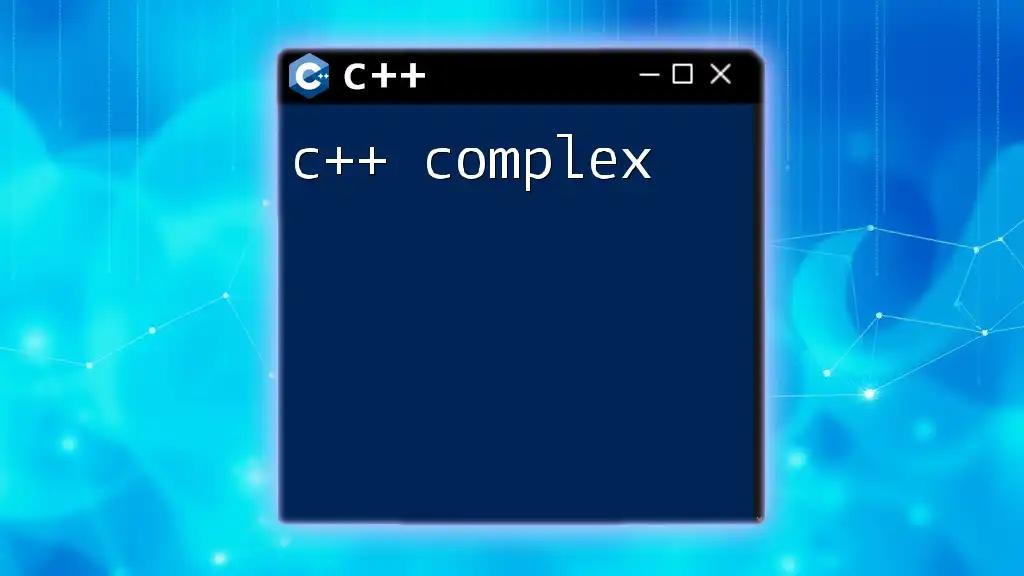
Best Practices in Using Doubles
When to Use Double Over Float
Choosing between float and double depends largely on your precision needs. If your application demands higher precision in calculations, such as financial applications or scientific computing, you should opt for a double due to its ability to handle greater precision with less risk of truncation errors.
Handling Precision Issues
Despite the advantages, floating-point types, including doubles, have limitations regarding precision. Over time, you may encounter floating-point errors—small discrepancies that arise due to how numbers are represented in memory.
You can control the number of decimal places printed using std::setprecision from `<iomanip>`:
#include <iostream>
#include <iomanip>
double myDouble = 3.141592653589793238;
std::cout << std::fixed << std::setprecision(2) << myDouble; // Controls output to 2 decimal places

Common Errors with Doubles
Common Mistakes
One of the common pitfalls when working with doubles is overflow and underflow. Overflow occurs when a number exceeds the maximum limit, while underflow happens when a number is too small to be represented.
Another frequent issue arises when comparing doubles directly. Due to rounding errors in floating-point arithmetic, two doubles that you think are equal might not be. For example:
double x = 0.1 + 0.2; // Might not equal to 0.3 due to precision issues
if (x == 0.3) {
// This condition may not hold true
}
Debugging Tips
When encountering issues with doubles, consider using debugging tools that can help you identify conversion errors or unexpected behavior. Good practices include validating your data and using assertions to check the outcomes of your computations during development.
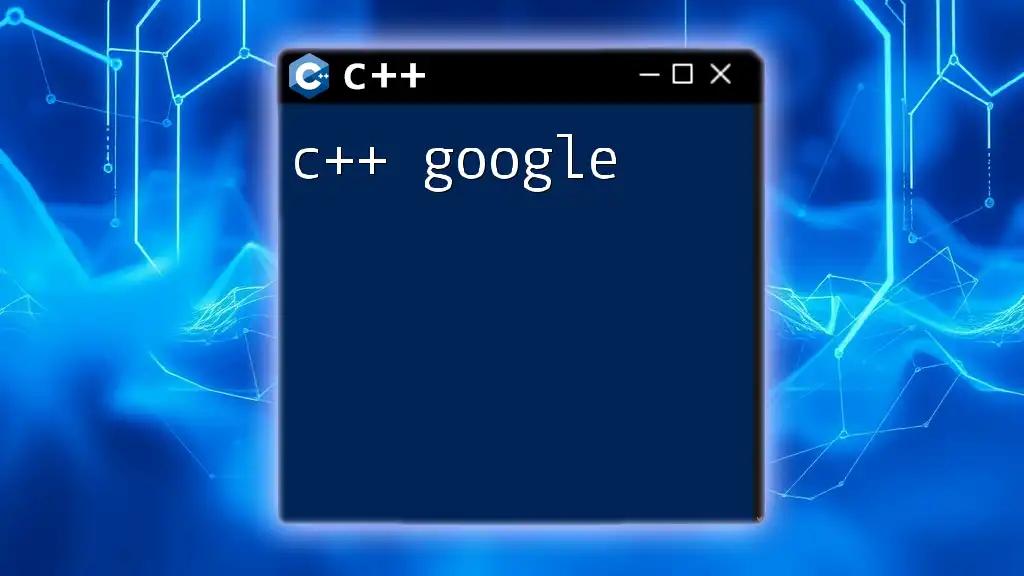
Conclusion
Recap of C++ Doubles
C++ doubles play a crucial role in numerical computations, allowing developers to handle large and precise floating-point numbers. Understanding their characteristics, operations, and best practices can significantly enhance your programming capability.
Additional Resources
For continued learning, consider exploring additional resources that focus on C++ data types, floating-point arithmetic, and advanced mathematical libraries.

Call to Action
Start practicing with doubles in your projects today! Engage with our platform for more hands-on tutorials on C++ and its commands, and enhance your programming skills!