The `usleep` function in C++ is used to suspend execution of the calling thread for a specified period, measured in microseconds.
Here’s a code snippet demonstrating its use:
#include <iostream>
#include <unistd.h>
int main() {
std::cout << "Sleeping for 2 seconds..." << std::endl;
usleep(2000000); // Sleep for 2,000,000 microseconds (2 seconds)
std::cout << "Awake!" << std::endl;
return 0;
}
Understanding `usleep`
What is `usleep`?
`usleep` is a function that allows a program to pause execution for a specified amount of time, measured in microseconds. It is defined in the `<unistd.h>` header and is commonly used in C and C++ programming to implement delays in code execution. Its primary purpose is to enable precise timing control, which is crucial in various applications, including simulations, animations, and hardware interactions.
Why Use `usleep`?
Using `usleep` can significantly enhance the performance and responsiveness of a program. It's particularly useful in scenarios where timing is critical, such as:
- Animation loops where a fixed time per frame can enhance visual fluidity.
- Polling mechanisms that check sensor states or network responses at regular intervals.
- Simulations that require synchronization with real-world time.
These use cases highlight `usleep` as an integral function for managing time-based operations effectively.

How to Use `usleep`
Basic Syntax
The basic syntax of `usleep` is straightforward:
usleep(useconds_t microseconds);
Here, `microseconds` is the duration of the sleep interval specified in microseconds.
Working with Time Values
Before using `usleep`, it's essential to understand how time is measured in this context. Time in `usleep` is defined in microseconds, where:
- 1 second = 1,000,000 microseconds
- 1 millisecond = 1,000 microseconds
This understanding allows you to convert the desired sleep duration into the appropriate value for the `usleep` function.
Example Code Snippet
Here’s a simple example that demonstrates the use of `usleep`:
#include <iostream>
#include <unistd.h>
int main() {
std::cout << "Start sleeping..." << std::endl;
usleep(1000000); // Sleep for 1 second (1,000,000 microseconds)
std::cout << "Awake now!" << std::endl;
return 0;
}
In this code snippet, the program announces the start of a sleep period, waits for 1 second, and then resumes execution, displaying that it’s awake again.
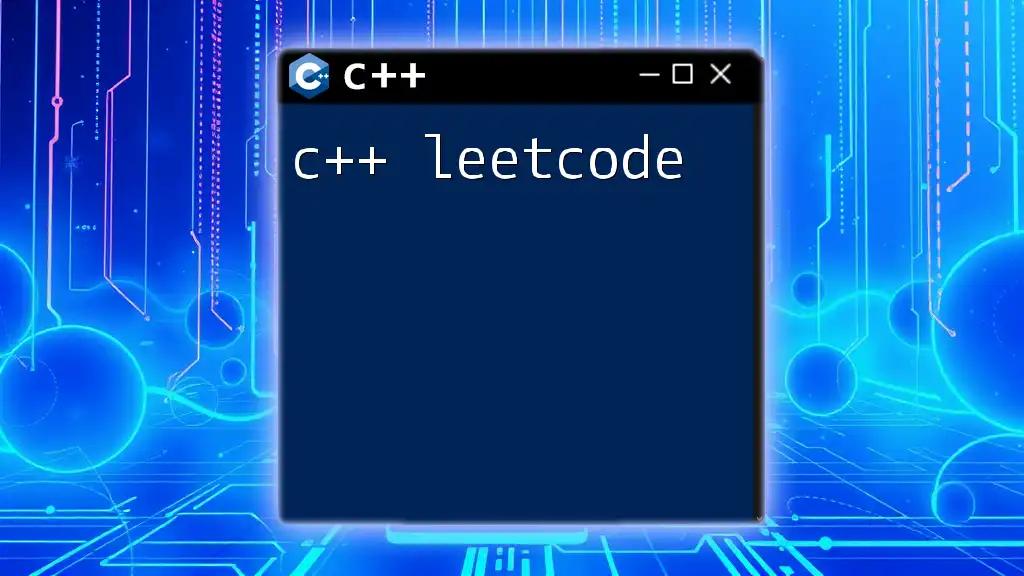
Limitations of `usleep`
Minimum Sleep Time
While `usleep` is flexible, it does have limitations regarding the minimum duration one can specify for sleep. The minimum applicable duration may vary based on the operating system and hardware. Typically, this value is around 1 microsecond, but precision might be affected by the system's scheduling algorithms.
Precision Issues
`usleep` isn’t perfectly precise. The actual sleep duration may be longer than requested due to various factors, such as:
- System Load: Heavy system load can delay the execution of the subsequent code.
- Kernel Scheduling: The exact timing can vary based on the scheduler's behavior and how the operating system prioritizes tasks.

Alternatives to `usleep`
`nanosleep`
An alternative to `usleep` is `nanosleep`, which provides higher precision for sleep periods. It allows you to specify sleep time in nanoseconds, making it suitable for applications requiring more fine-grained control over timing.
Here’s a comparison:
- `usleep`: Accepts microseconds and is simpler to use for most applications.
- `nanosleep`: More complex but allows for greater precision.
Example using `nanosleep`:
#include <iostream>
#include <time.h>
int main() {
struct timespec req = {1, 0}; // 1 second
std::cout << "Sleeping for 1 second..." << std::endl;
nanosleep(&req, NULL);
std::cout << "Awake now!" << std::endl;
return 0;
}
C++ Standard Library Alternatives
`std::this_thread::sleep_for`
With the introduction of C++11, the standard library provides a more modern approach using threads. The `std::this_thread::sleep_for` function is part of the `<thread>` header and is designed for use with `std::chrono`, providing a more C++-like interface.
Example of using `std::this_thread::sleep_for`:
#include <iostream>
#include <chrono>
#include <thread>
int main() {
std::cout << "Sleeping for 1 second..." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(1));
std::cout << "Awake now!" << std::endl;
return 0;
}
This approach not only showcases the syntax capabilities of C++11 but also promotes better readability and type safety within your code.

Practical Applications of `usleep`
Animation and Graphics
In animation, consistent frame rates are vital to rendering smooth visual effects. `usleep` is often used in game loops to create a delay that aligns frame rendering with the monitor's refresh rate, thereby ensuring a seamless visual experience for users.
Polling Processes
Consider situations where you need to poll a device or an API continuously. Using `usleep` can help regulate the polling frequency, ensuring that the system doesn’t overload the device or network with requests.
Simulations
In simulation environments, maintaining a precise temporal relationship with the real world is critical. `usleep` allows developers to implement accurate timing that reflects real-world conditions, which is essential for simulations involving physics, robotics, or any real-time data stream.
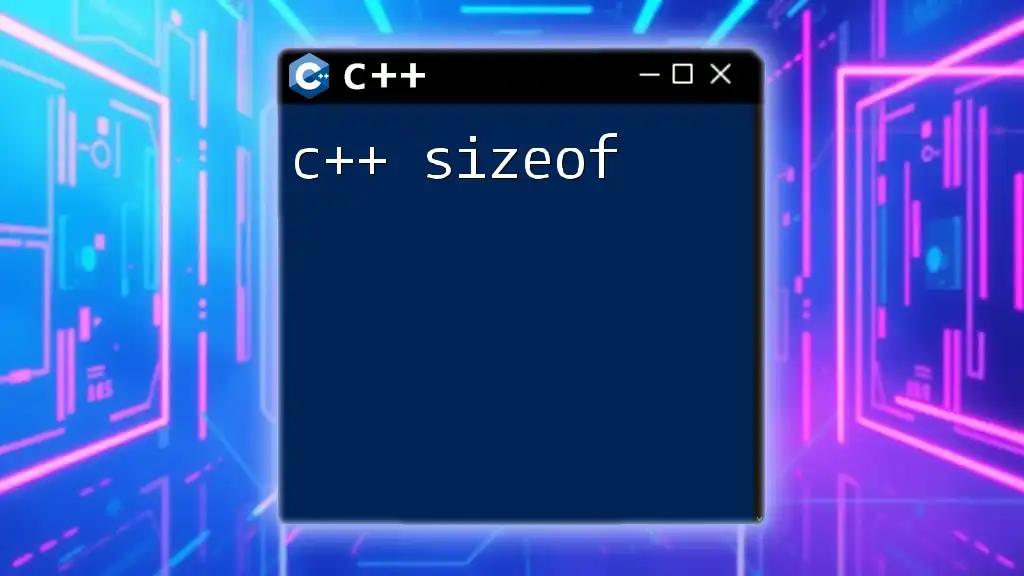
Best Practices when Using `usleep`
Avoiding Busy Waiting
Utilizing `usleep` effectively circumvents the common pitfall of busy waiting—an approach where a program continuously checks for a condition without yielding control. By incorporating `usleep`, your program can pause without using CPU cycles unnecessarily, enhancing overall efficiency.
Managing System Performance
While `usleep` can be a powerful tool, it's crucial to use it judiciously. Overusing sleep calls in larger applications can cause performance bottlenecks. Striking a balance between responsiveness and performance is vital for a smooth user experience.

Conclusion
In summary, `c++ usleep` is a simple yet powerful function that allows for precise time management in C++ programs. By understanding its capabilities, limitations, and alternatives, you can effectively utilize `usleep` to enhance your applications. Experimenting with different scenarios will provide deeper insights into how you can leverage this function in your projects.
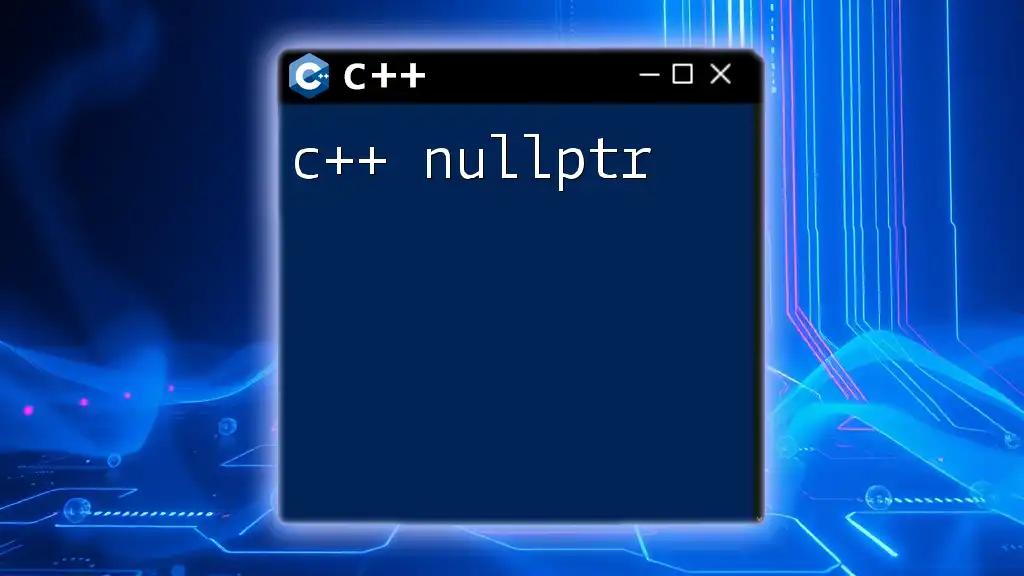
Additional Resources
Documentation
For further reading and official details, refer to the C++ documentation and resources related to `usleep`.
Community Forums
Participating in community forums can be invaluable. Engaging in discussions around C++ and `usleep` will allow you to learn from others' experiences, ask questions, and share your insights.