The `std::this_thread::sleep_for` function in C++ is used to block the execution of the current thread for a specified duration, which can be useful for creating delays in your program.
#include <iostream>
#include <thread>
#include <chrono>
int main() {
std::cout << "Waiting for 2 seconds...\n";
std::this_thread::sleep_for(std::chrono::seconds(2));
std::cout << "Continue execution.\n";
return 0;
}
Understanding C++ Threads
What are Threads?
In programming, threads are the smallest units of processing that can be scheduled by an operating system. They enable a program to perform multiple tasks concurrently, leading to improved responsiveness and resource utilization. Multithreading is key for applications that require asynchronous operations, allowing different parts of your program to execute independently.
The Role of `<thread>` Library in C++
The `<thread>` library in C++ provides a powerful set of tools for managing threads. It allows developers to create and manage threads and provides synchronization mechanisms to prevent data races. Key features include thread creation, joining threads, and sleeping or delaying the execution—effectively facilitating a multithreaded programming model.
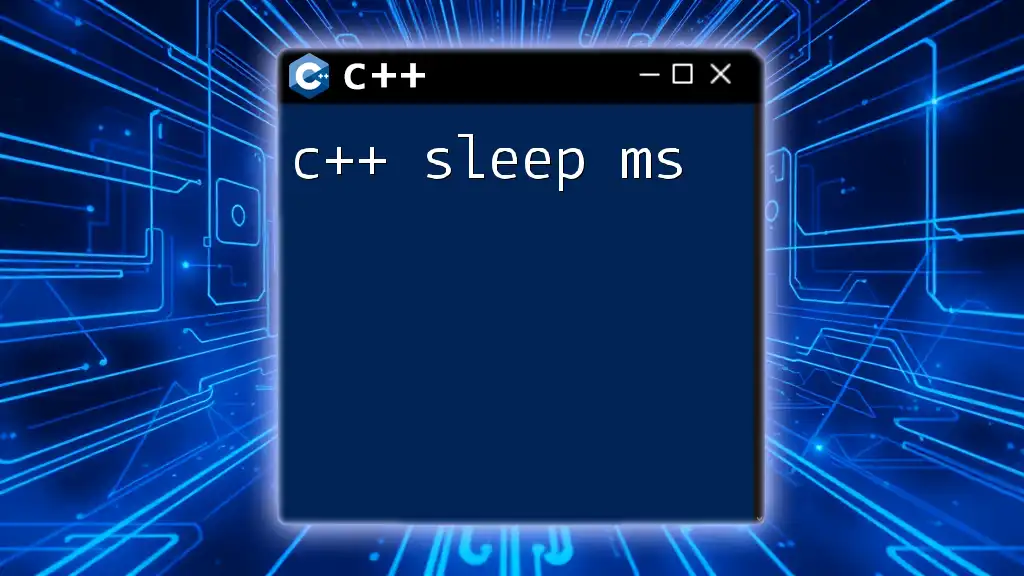
Introduction to `sleep_for`
What is `sleep_for`?
The `sleep_for` function is a part of the `<thread>` library that allows a thread to pause its execution for a specified period. This is particularly useful in scenarios where you want to delay execution, simulate workload, manage timing, or coordinate the execution of multiple threads.
Syntax of `sleep_for`
The syntax of `sleep_for` is straightforward:
std::this_thread::sleep_for(std::chrono::duration);
It requires a duration parameter, which specifies how long the thread should sleep.
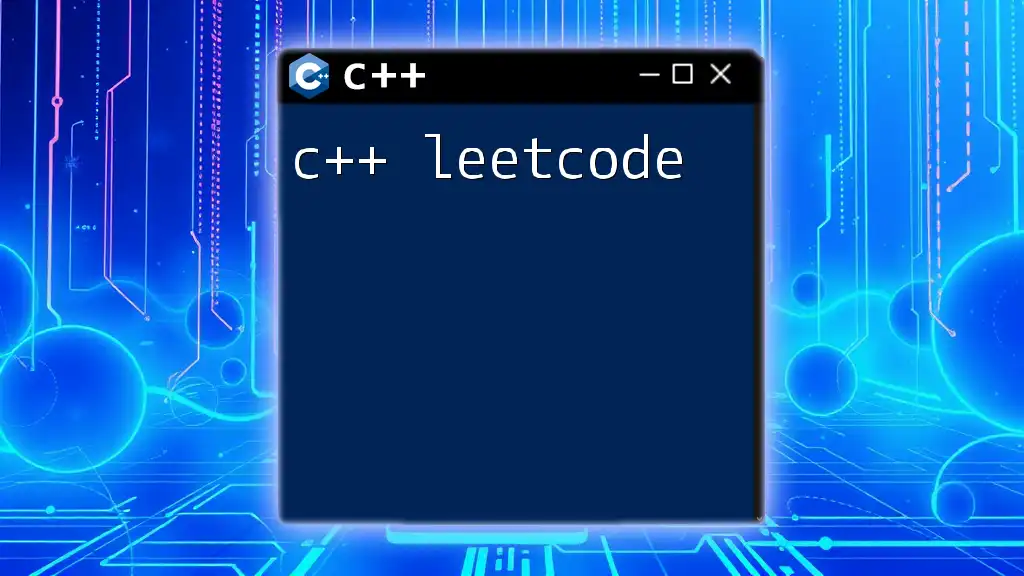
Importing Required Headers
Necessary Headers for `sleep_for`
To make use of `sleep_for`, you need to include the appropriate headers at the beginning of your C++ program:
#include <iostream>
#include <thread>
#include <chrono>
- `<iostream>` provides functionalities for output and input.
- `<thread>` is necessary for working with threads and the `sleep_for` function.
- `<chrono>` is used for time representation.
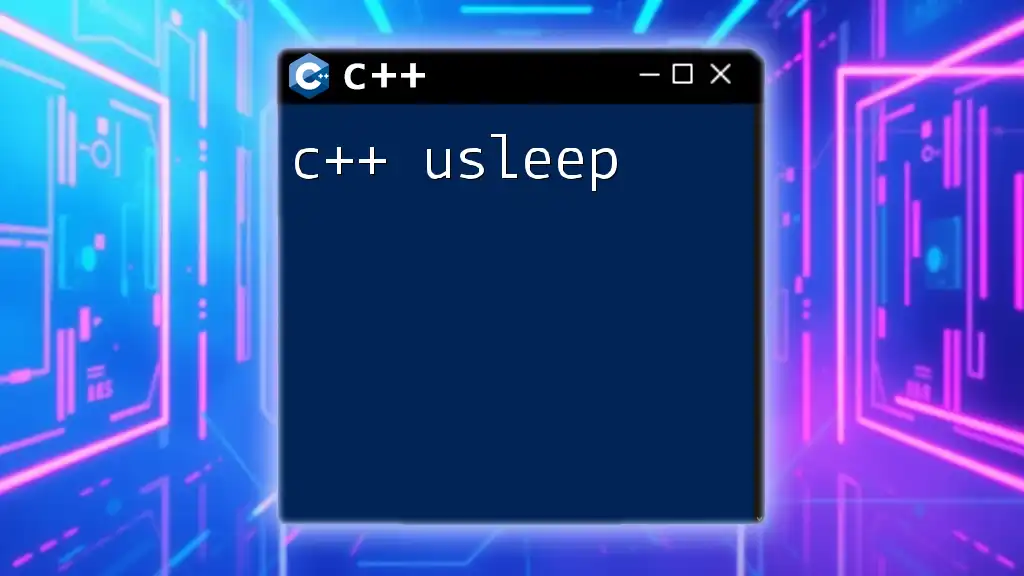
How to Use `sleep_for`
Basic Example
Let’s start with a simple example that demonstrates the functionality of `sleep_for`:
#include <iostream>
#include <thread>
int main() {
std::cout << "Sleeping for 1 second..." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(1));
std::cout << "Awake!" << std::endl;
return 0;
}
In this snippet:
- First, we print a message indicating that we will sleep for 1 second.
- The call to `std::this_thread::sleep_for(std::chrono::seconds(1));` puts the thread into sleep for 1 second.
- After waking, another message indicates the completion of the sleep.
Advanced Example: Multiple Threads
You can also use `sleep_for` in a multithreaded context:
#include <iostream>
#include <thread>
void threadFunction(int id) {
std::cout << "Thread " << id << " is starting." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(2));
std::cout << "Thread " << id << " is done." << std::endl;
}
int main() {
std::thread t1(threadFunction, 1);
std::thread t2(threadFunction, 2);
t1.join();
t2.join();
return 0;
}
In this example:
- We define a function `threadFunction` that takes an integer id.
- Inside this function, we simulate some work using `sleep_for` for 2 seconds.
- We create two threads, each of which runs the `threadFunction`.
- The `join` method ensures that the main thread waits for both threads to finish before proceeding.
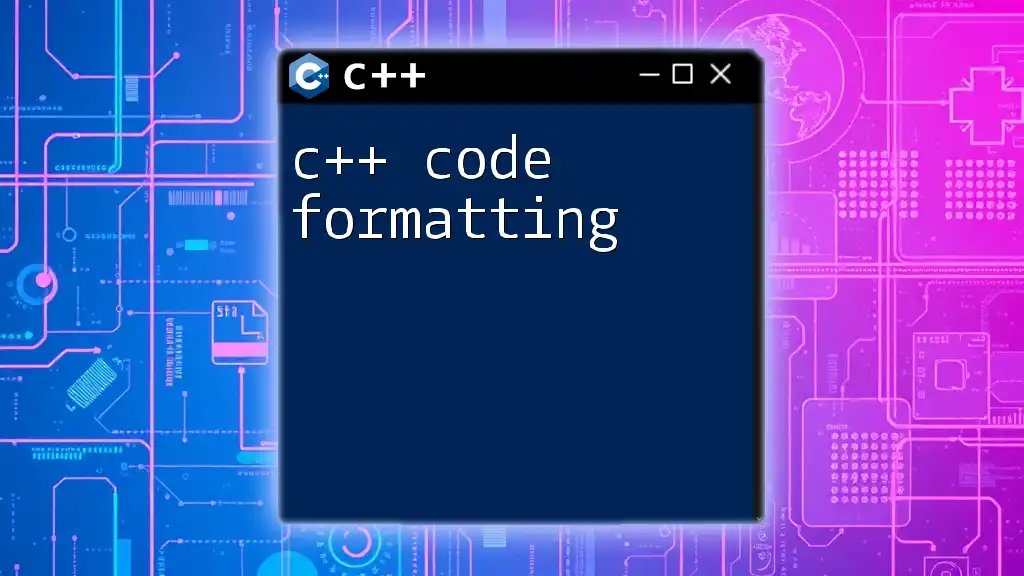
`sleep_for` with Different Time Units
Working with `std::chrono`
The `std::chrono` library allows you to specify sleep durations using multiple units such as seconds, milliseconds, microseconds, and nanoseconds, giving you flexibility based on your application's needs.
Implementation Examples
Using Milliseconds
You can specify time in milliseconds easily:
std::this_thread::sleep_for(std::chrono::milliseconds(500));
In this case, the execution halts for 500 milliseconds.
Using Microseconds and Nanoseconds
Similarly, for microseconds and nanoseconds:
std::this_thread::sleep_for(std::chrono::microseconds(100));
std::this_thread::sleep_for(std::chrono::nanoseconds(1000));
Here, the program will sleep for 100 microseconds and 1000 nanoseconds, respectively. These short durations can be useful for timing-critical applications where precision is essential.
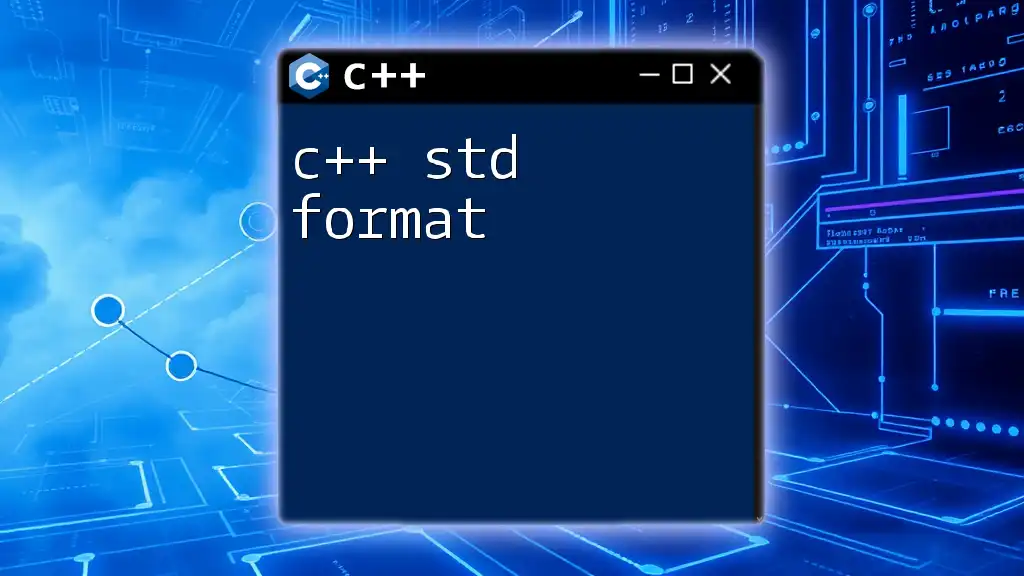
Common Mistakes with `sleep_for`
Overuse of `sleep_for`
One common pitfall is the overuse of `sleep_for`, which can severely impact application performance. Limiting the frequency and duration of sleeps is crucial for maintaining responsiveness in your application. It's essential to consider alternative approaches such as event handling instead of sleeping, especially in interactive applications.
Misunderstanding Time Units
Another frequent mistake is misunderstanding the time units. Using the wrong unit can lead to unexpected behavior. Always ensure you are using the correct `chrono` duration type for your needs—whether seconds, milliseconds, or microseconds— to avoid these pitfalls.
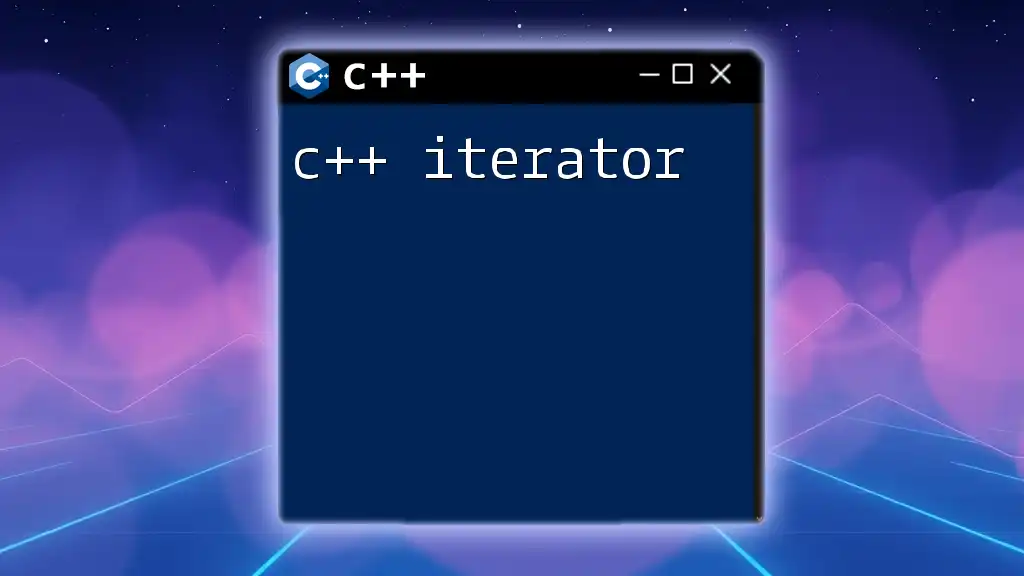
Conclusion
In summary, the `sleep_for` function in C++ is a powerful tool for managing thread execution. By allowing threads to pause for defined durations, it helps control the timing of operations within your application. Understanding how to effectively utilize `sleep_for` can enhance your multithreading capabilities and improve the overall performance of your C++ programs.
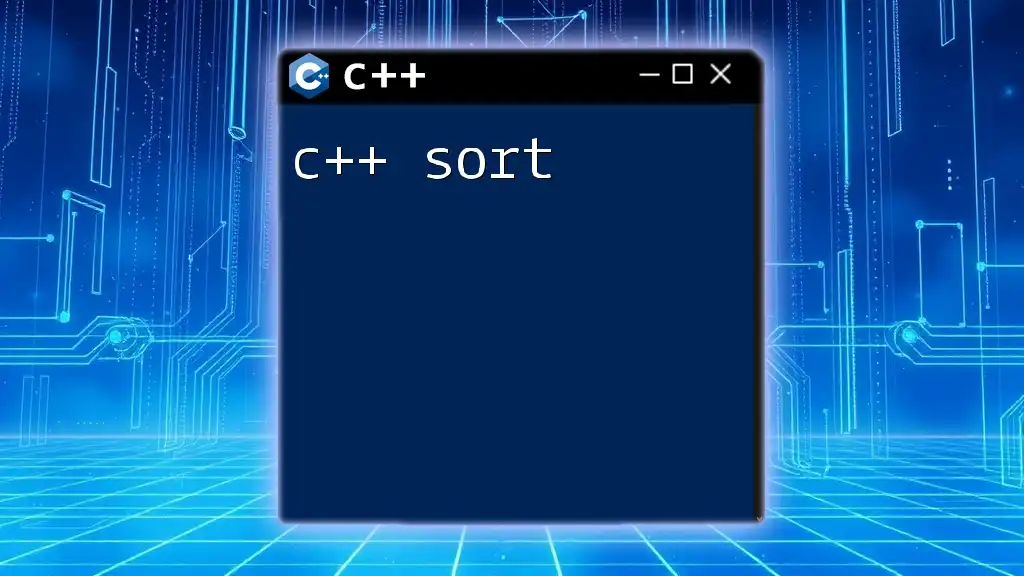
Additional Resources
For further learning, explore more about C++ multithreading concepts and best practices. Online resources such as tutorials, documentation on the `<thread>` library, and community forums can provide valuable insights for honing your skills.
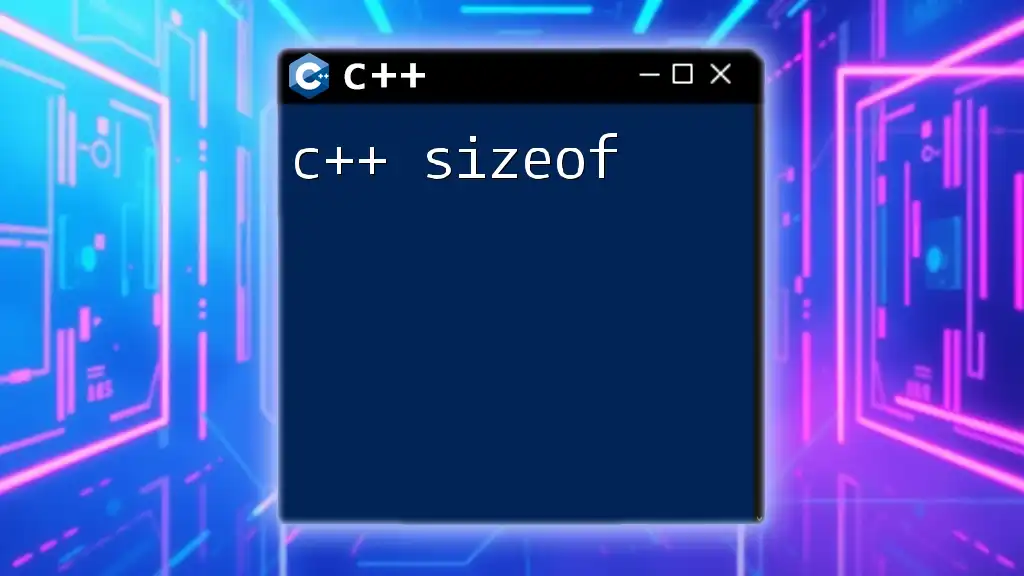
Frequently Asked Questions
What is the Difference Between `sleep` and `sleep_for`?
The `sleep` function is simpler and typically sleeps for a fixed duration, while `sleep_for` provides more flexibility, allowing you to specify exact durations using various units.
Can `sleep_for` Handle Different Time Formats?
Yes, `sleep_for` can handle multiple time formats provided through the `std::chrono` library, allowing programmers to specify sleep durations in seconds, milliseconds, microseconds, or nanoseconds.
Is it Possible to Interrupt `sleep_for`?
While `sleep_for` can't be interrupted directly, you can implement cooperative multitasking with conditions or flags to signal threads to wake up early by structuring your code logically around sleep durations.