The C++ `std::format` function provides a way to format strings using a printf-like syntax, making it easier to create formatted output.
#include <iostream>
#include <format>
int main() {
int value = 42;
std::string formatted_string = std::format("The answer is: {}", value);
std::cout << formatted_string << std::endl;
return 0;
}
Understanding the Need for Formatting in C++
In programming, string formatting is crucial for outputting data clearly and meaningfully. It enhances readability and ensures that information is presented in a user-friendly manner. Legacy formatting methods such as `printf` can be cumbersome, inflexible, and prone to errors, especially for complex scenarios. This is where C++20 introduces a new solution with std::format, fundamentally changing how we format strings.
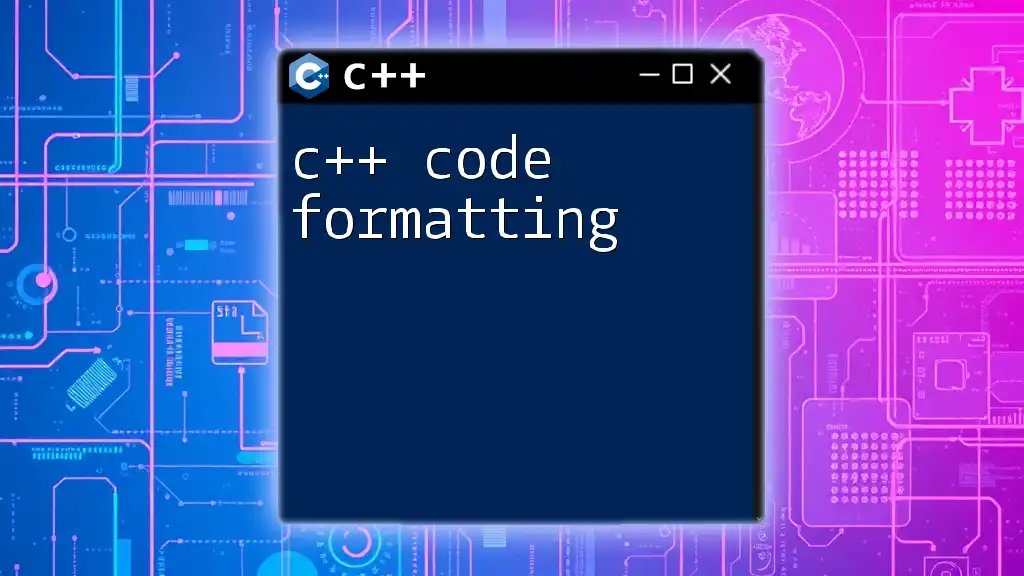
What is std::format?
The `std::format` function is part of the C++20 standard library designed for formatting strings in a more intuitive and expressive way. It allows developers to embed values directly into string literals without cumbersome syntax, leading to clearer and more maintainable code. Unlike older methods, `std::format` improves type safety and offers richer formatting options.
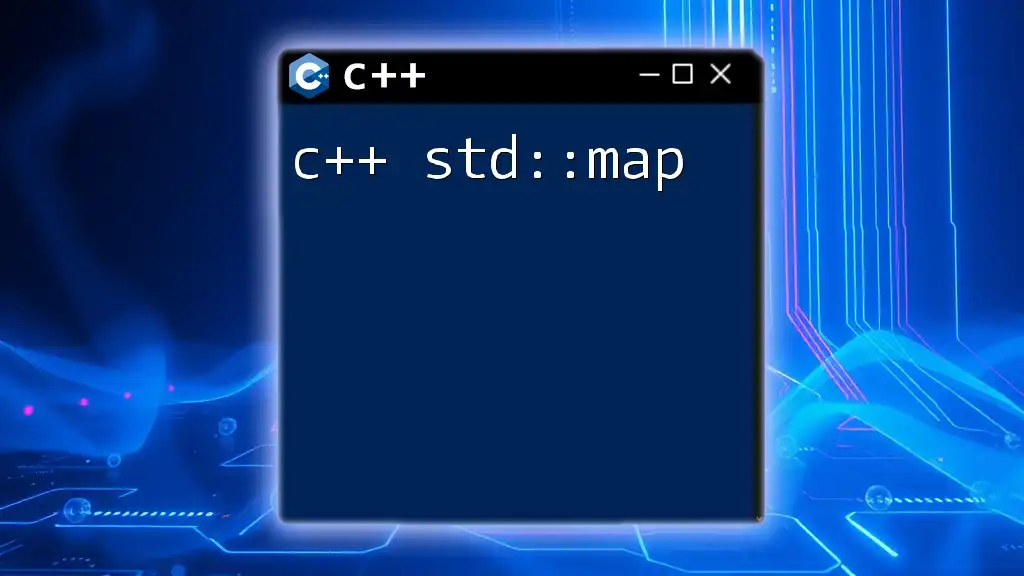
Basics of std::format
Syntax Overview
Using `std::format` is straightforward. The core syntax consists of a format string with placeholders for values. The placeholders are defined using curly braces `{}`. Here’s a simple example:
#include <format>
std::string result = std::format("Hello, {}!", "World");
In this snippet, `"Hello, {}!"` is the format string, and `"World"` replaces the placeholder `{}`.
Format Specifiers
Format specifiers allow you to control how data types are represented. Understanding these is essential for effective usage of `std::format`. Common specifiers include:
- `{}` for plain substitution (any type)
- `{:d}` for integers
- `{:f}` for floating-point numbers
- `{:s}` for strings
For instance, formatting an integer can be done as follows:
int number = 42;
std::string formatted = std::format("The answer is: {}", number);
This snippet outputs a clear message, substituting the placeholder with the value of `number`.
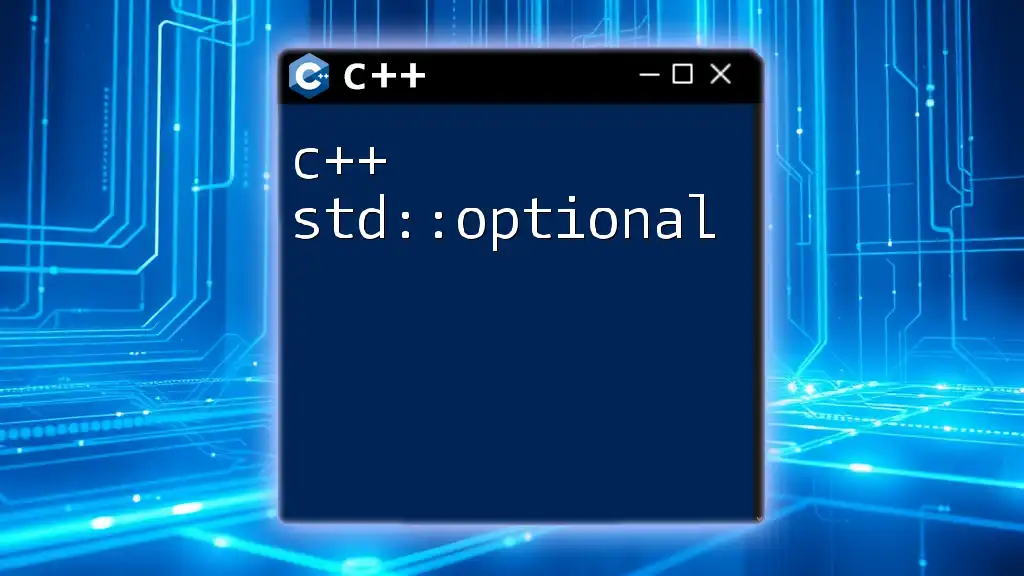
Advanced Formatting Techniques
Positional and Named Arguments
`std::format` supports positional parameters, allowing you to reference placeholders by their order in the format string. For example, the first placeholder can be referenced as `1`, the second as `2`, and so on:
std::string formatted = std::format("{:1} is the answer to life, the universe, and everything.", number);
Named arguments enhance readability. Instead of relying solely on position, you can use variable names directly in your format string, making your code even more understandable.
Formatting Options
Formatting options in `std::format` provide control over text alignment, width, and fill characters. Here’s how you can manipulate these aspects:
std::string formatted = std::format("{:<10} is left aligned", "Left");
In the above example, `:<10` ensures that the word “Left” is left-aligned within a width of 10 characters.
Custom Types and Formatters
One of the flexible features of `std::format` is the ability to create custom formatters for user-defined types. This requires specializing the `std::formatter` struct. Here’s how to implement this for a simple `Point` structure:
struct Point { int x, y; };
template <>
struct std::formatter<Point> {
constexpr auto parse(format_parse_context& ctx) { return ctx.begin(); }
template <typename FormatContext>
auto format(Point p, FormatContext& ctx) {
return format_to(ctx.out(), "({}, {})", p.x, p.y);
}
};
With this formatter, you can now format `Point` objects intuitively:
Point pt{3, 4};
std::string formatted = std::format("The point is at {}", pt);
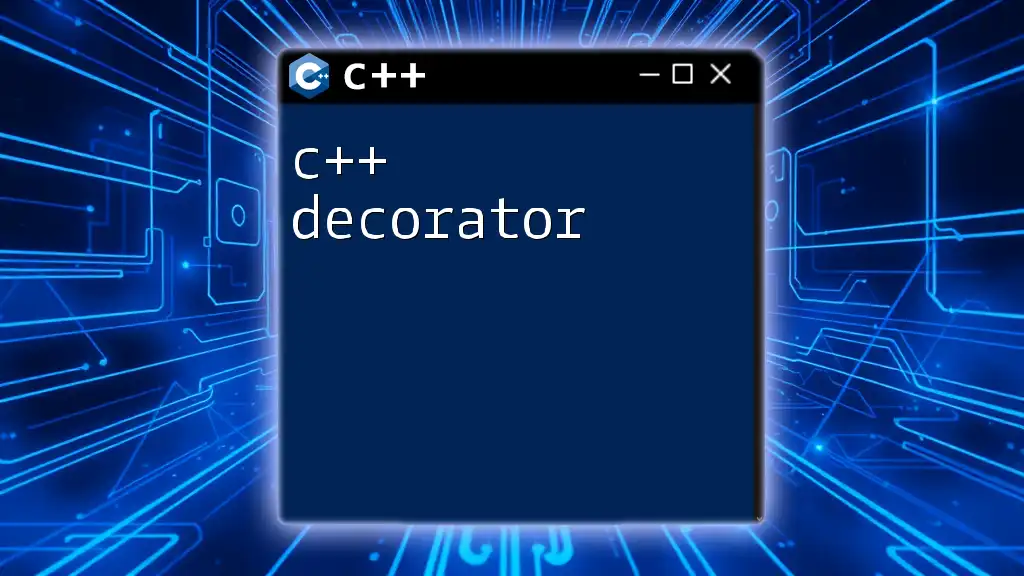
Practical Applications of std::format
File and Log Formatting
In modern applications, formatting serves a vital role in logging systems. `std::format` allows for cleaner, structured log entries that convey necessary information clearly. For example, logging application startup can look like this:
std::string log_entry = std::format("[{}] INFO: {}", current_time(), "Application started");
This approach allows developers to dynamically insert relevant data into log messages efficiently.
User Interface Development
`std::format` finds significant application in user interface development. It enables developers to update UI elements with dynamic data seamlessly. Consider the following code snippet where the score of a player is displayed:
label.setText(std::format("Score: {}", player_score));
This line dynamically updates the label whenever `player_score` changes, resulting in an engaging user experience.
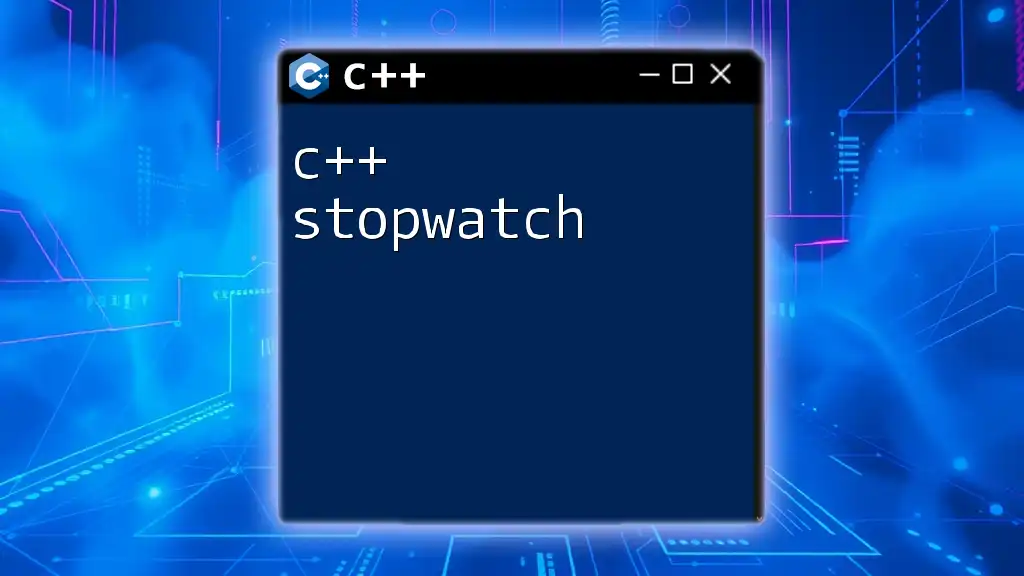
Error Handling and Performance Considerations
Handling Formatting Errors
When working with formatting, handling potential errors robustly is essential. `std::format` can throw exceptions when incorrect format specifiers or types are used. Here’s an example of error handling in action:
try {
std::string result = std::format("{:", "invalid");
} catch (const std::format_error& e) {
std::cerr << "Formatting error: " << e.what() << '\n';
}
Implementing such error handling ensures that your application can gracefully manage unexpected situations without crashing.
Performance Implications
Performance is a critical aspect of string formatting, especially in performance-sensitive applications. `std::format` offers efficiency improvements over its predecessors, but developers should still be cautious in high-frequency formatting scenarios. Profiling and optimization are recommended if formatting is a bottleneck in your application's execution.
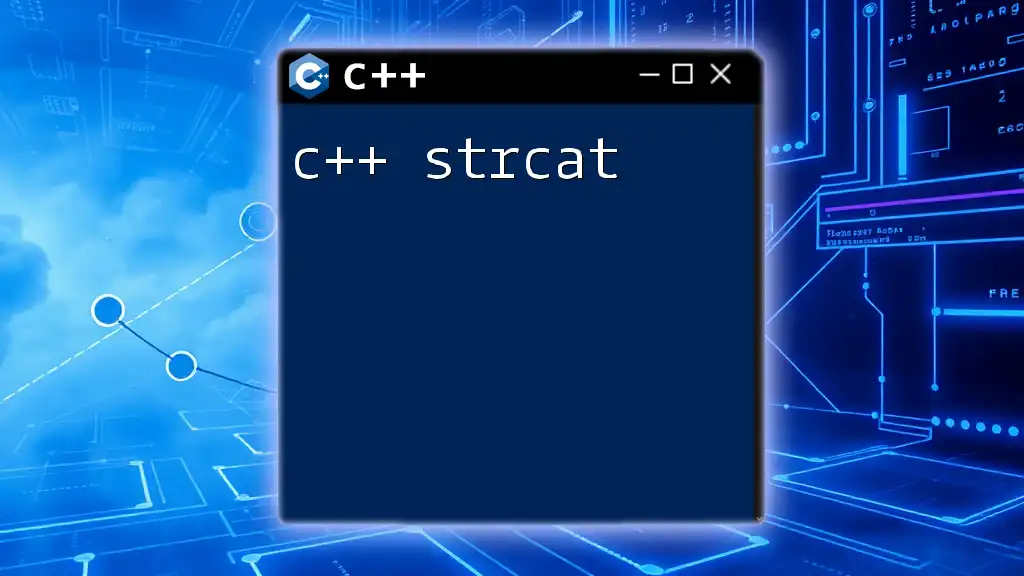
Recap of Key Points
In summary, c++ std format revolutionizes string formatting, emphasizing clarity, type safety, and flexibility. It enables developers to present information in intuitive and maintainable ways, enhancing both user experience and code quality.
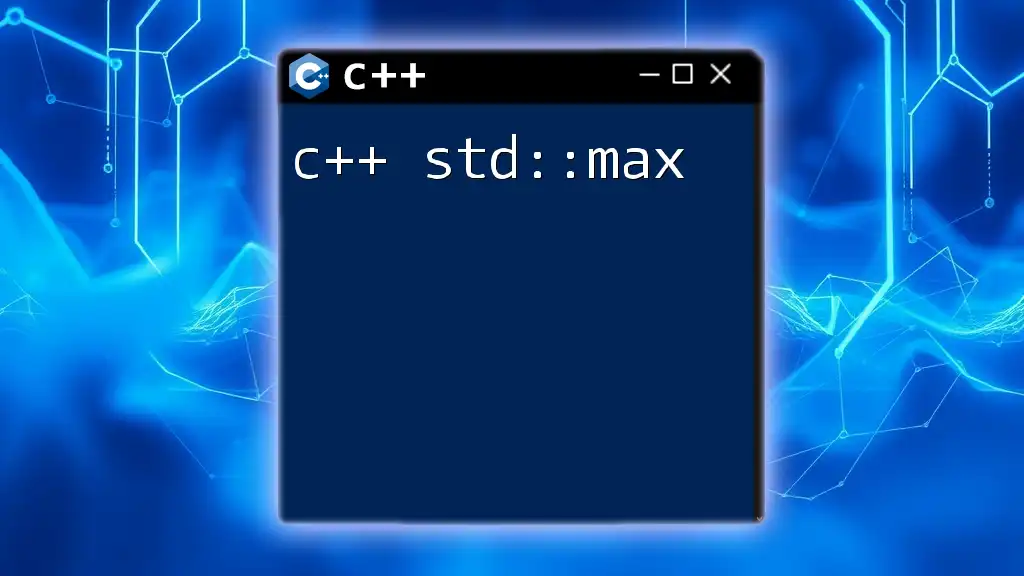
Encouragement to Practice
As you explore `std::format`, experiment with simple projects to deepen your understanding. The best way to grasp its potential is through hands-on practice. Explore other C++20 features to elevate your programming skills further.
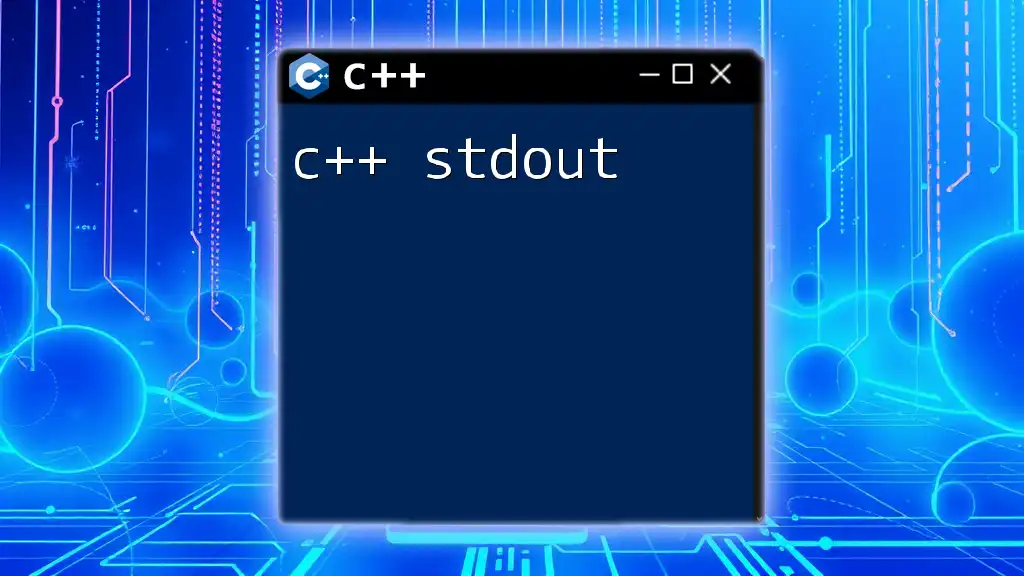
Additional Resources
For further reading on `std::format`, refer to official documentation and engaging tutorials that delve deeper into the intricacies of this powerful feature. You may also want to consider books or online courses focused on C++ best practices and advanced features to enhance your expertise.
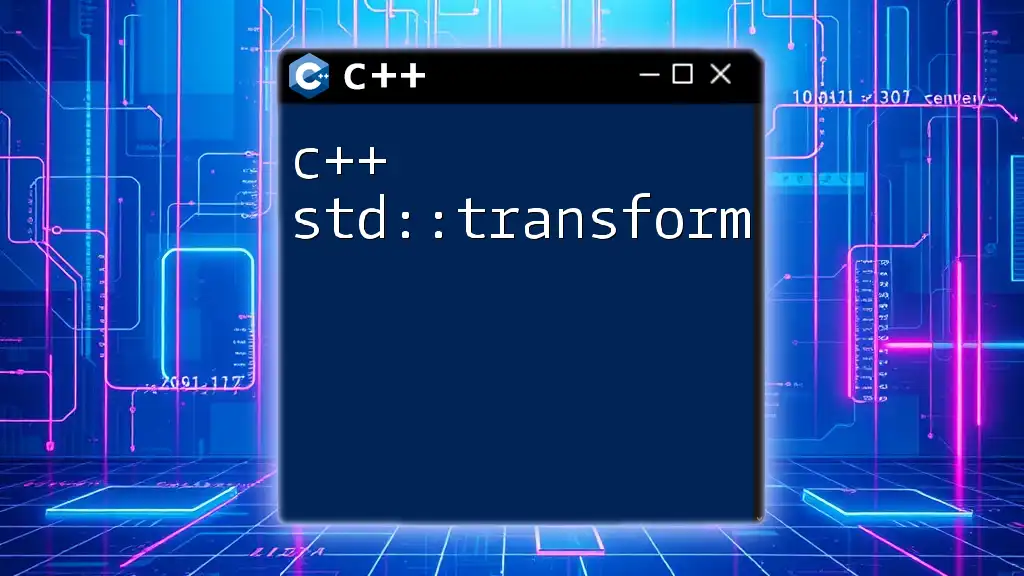
Feedback and Interaction
We invite you to share your experiences and queries regarding `std::format`. Engage with fellow learners in the comments section to foster a community of support and knowledge exchange, making learning more enriching for everyone involved.