In C++, the `short` keyword is used to define variables with a smaller range of integer values, typically occupying 2 bytes of memory.
short myNumber = 32767; // A short variable that can store a value from -32,768 to 32,767
Understanding C++ Syntax
C++ programs have a fundamental structure that comprises headers, functions, and various components that work together to perform tasks. Every standard C++ program begins with an `#include` directive, followed by a `main` function. This function serves as the entry point of the program. Mastering this basic syntax allows you to build upon more complex commands easily.
Basic Structure of C++ Programs
A typical C++ program has the following components:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this example, the output statement `cout << "Hello, World!" << endl;` showcases how to print text to the console. This is a foundational command in C++, illustrating its clarity and simplicity.
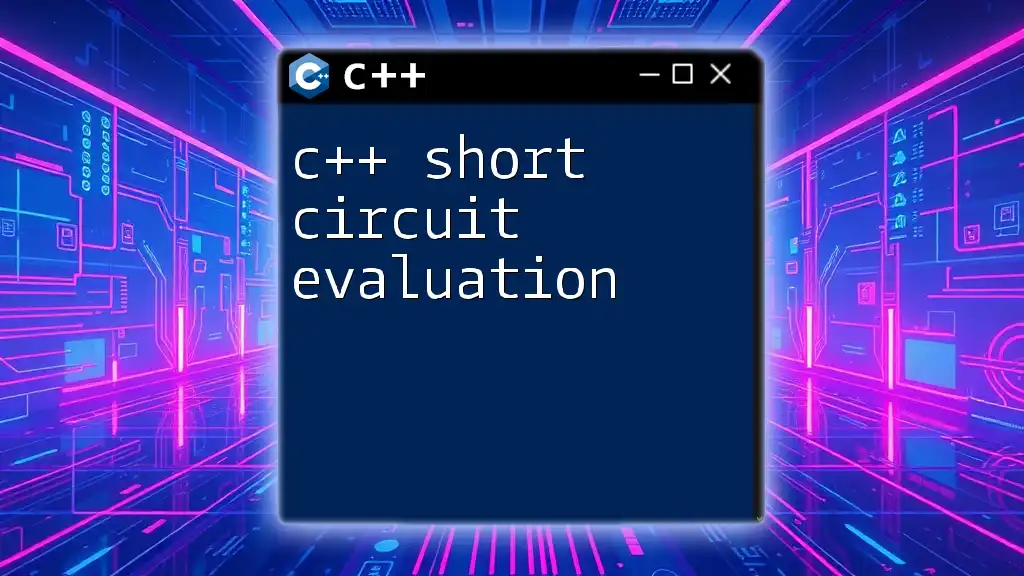
Key C++ Commands
Short Command Techniques
One of the keys to writing efficient C++ code lies in keeping your commands short and to the point. It not only boosts readability but also cuts down on development time. Below are some ways to utilize short C++ commands effectively.
Shortening Output Statements
Using `cout` does not have to be verbose. You can format your output strings to enhance clarity and conciseness. For instance, you can simplify your output statements by employing escape sequences like `\n` for new lines instead of the more verbose `endl`.
cout << "Result: " << result << "\n"; // Instead of cout << "Result: " << result << endl;
Minimizing Input Handling
User input handling can also be condensed. Instead of breaking your input into multiple statements or lines, you can use a single line to read a value:
int number; cin >> number; // Quick input statement without complexity
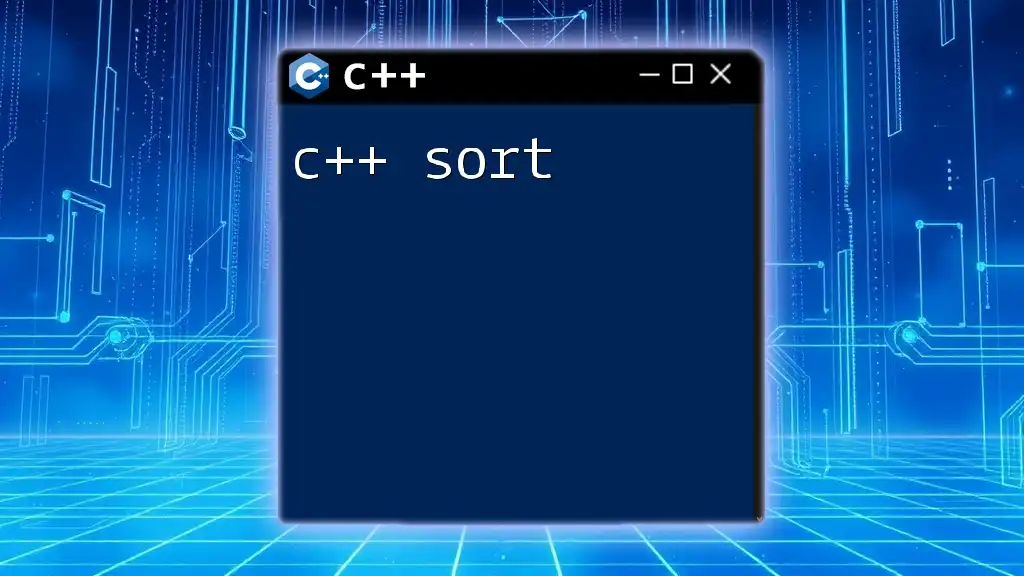
Utilizing Shortcuts in C++
Relying on Standard Template Library (STL)
The Standard Template Library (STL) is a treasure trove of functions and data structures that can significantly shorten your code. C++ provides several pre-built libraries that help you avoid manual implementations. For example, using `vector` instead of traditional arrays can minimize code and make it more manageable.
Compact Conditional Statements
Using conditional statements doesn’t always require lengthy blocks of code. The ternary operator is a concise way to handle conditional assignments or evaluations.
int max = (a > b) ? a : b; // Quick max function using the ternary operator
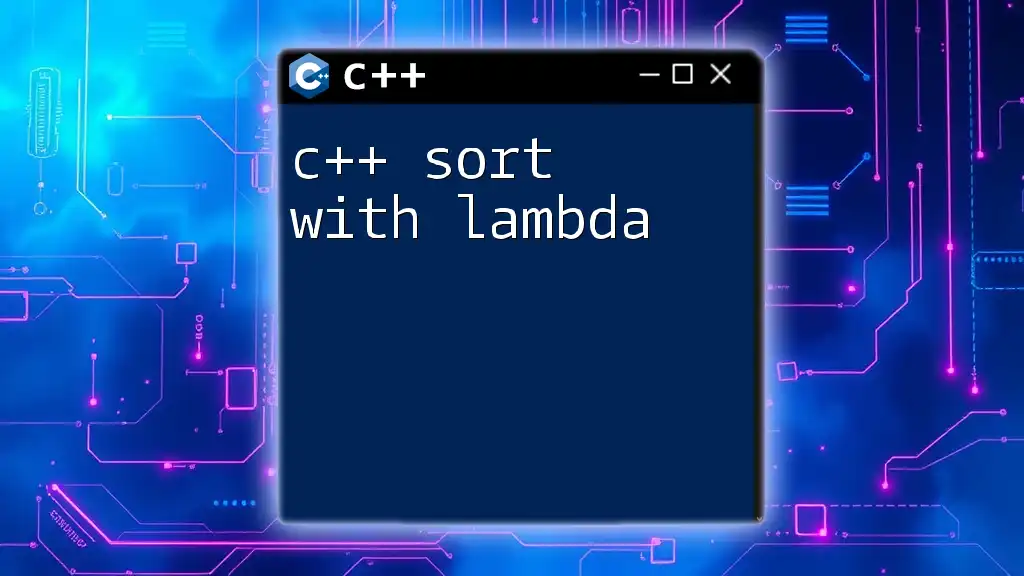
Creating Short Functions
Defining Inline Functions
Inline functions can prevent the overhead of regular function calls, especially in small functions. They allow you to write compact code while maintaining performance.
inline int square(int x) { return x * x; }
Lambda Expressions
C++ also supports lambda expressions, which reduce the code needed to define a function. They allow you to write quick, anonymous functions that can be passed around just like regular functions.
auto add = [](int x, int y) { return x + y; };
cout << add(5, 3);
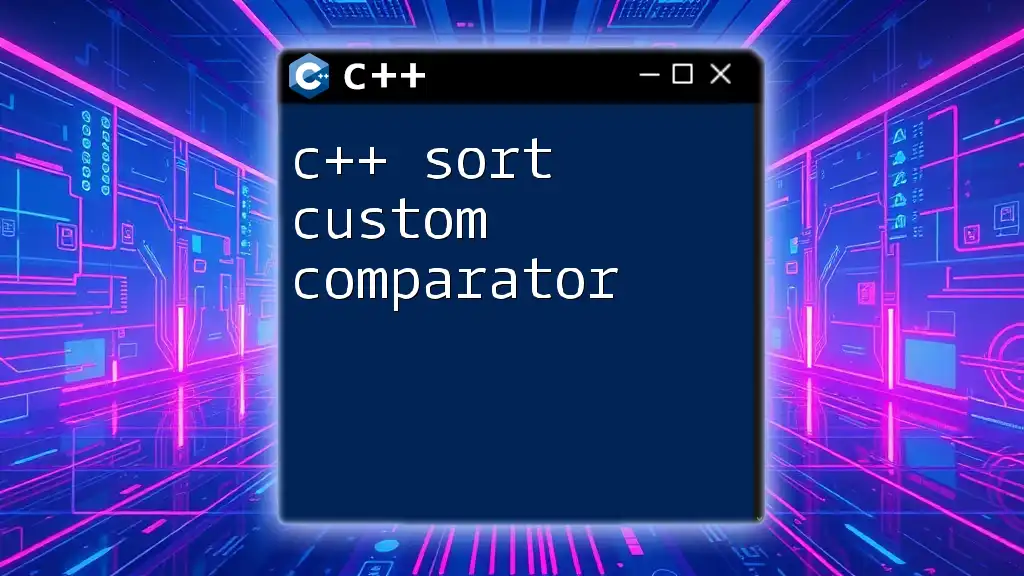
Shortening Loops and Iterations
Range-Based For Loops
Using range-based for loops can dramatically reduce the code needed to iterate through a collection, enhancing both performance and readability.
vector<int> numbers = {1, 2, 3, 4};
for (auto n : numbers) cout << n << " "; // Directly accessing each element
Using Algorithms for Shorter Code
The STL offers several algorithms like `for_each`, making your loops much shorter and cleaner. They apply a function to each element, which can significantly reduce code clutter.
for_each(numbers.begin(), numbers.end(), [](int n) { cout << n << " "; });
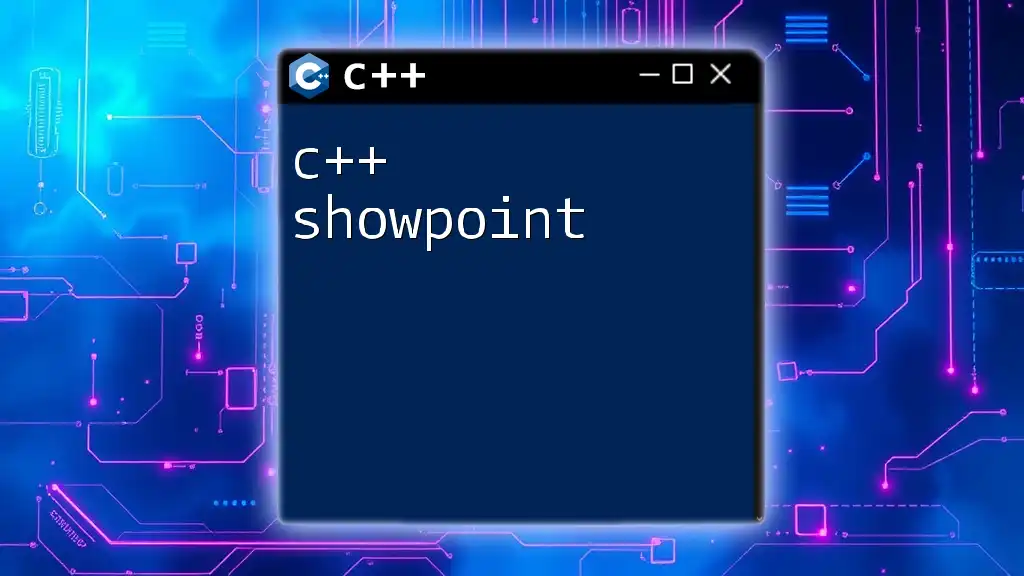
Advanced Techniques for Short Code
Template Programming
Template programming allows developers to write generic and functionally short code. Templates eliminate redundancy by enabling the same code to work with different data types.
template<typename T> T max(T a, T b) { return (a > b) ? a : b; }
Auto Keyword for Type Inference
Utilizing the auto keyword simplifies your code by letting the compiler infer types automatically. Using `auto` can significantly reduce verbosity in code, especially when dealing with complex types.
auto sum = [](auto a, auto b) { return a + b; };
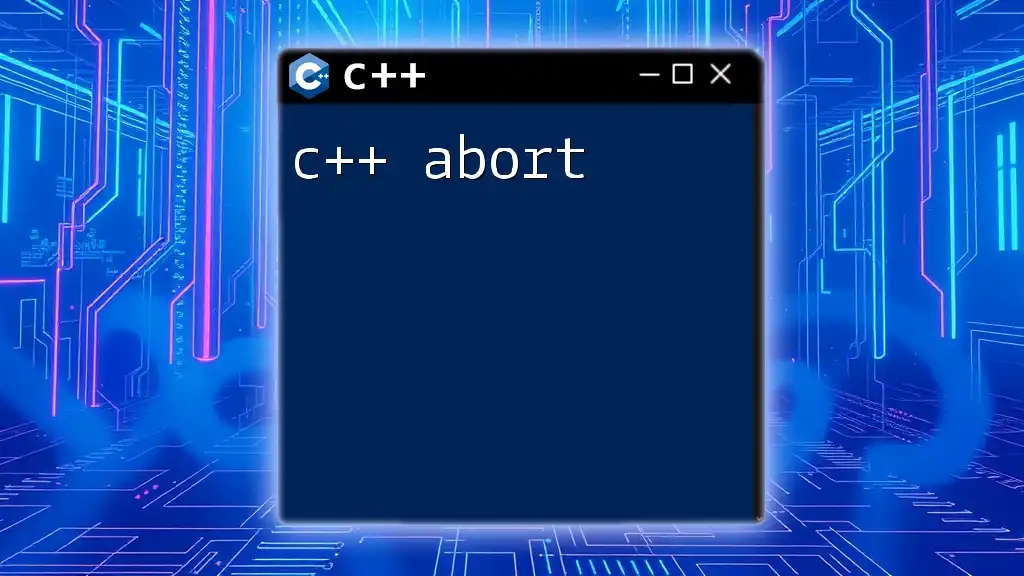
Best Practices for Writing Short C++ Code
Maintaining Readability
While writing shorter C++ code is often desirable, maintaining readability is equally important. Balancing brevity with clarity is key, and adding comments can help clarify any concise snippets that may confuse someone who reads your code later.
Avoiding Premature Optimization
Another pivotal aspect to remember is to avoid premature optimization. It's tempting to simplify code early on; however, understanding when to prioritize brevity over performance is crucial. Use short commands where they add value, but ensure your code remains functional and maintainable.
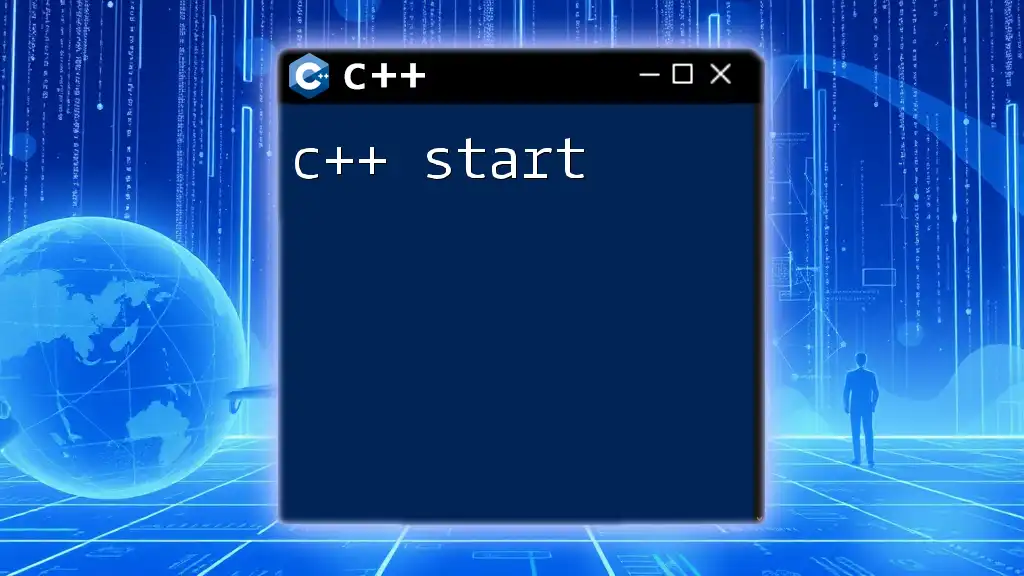
Conclusion
Utilizing "C++ short" commands can substantially enhance your programming experience and efficiency. By mastering these techniques, you not only sharpen your skills but also improve the readability and maintainability of your code. With practice, you can apply these strategies seamlessly in your projects, making your coding journey in C++ both enjoyable and fulfilling.
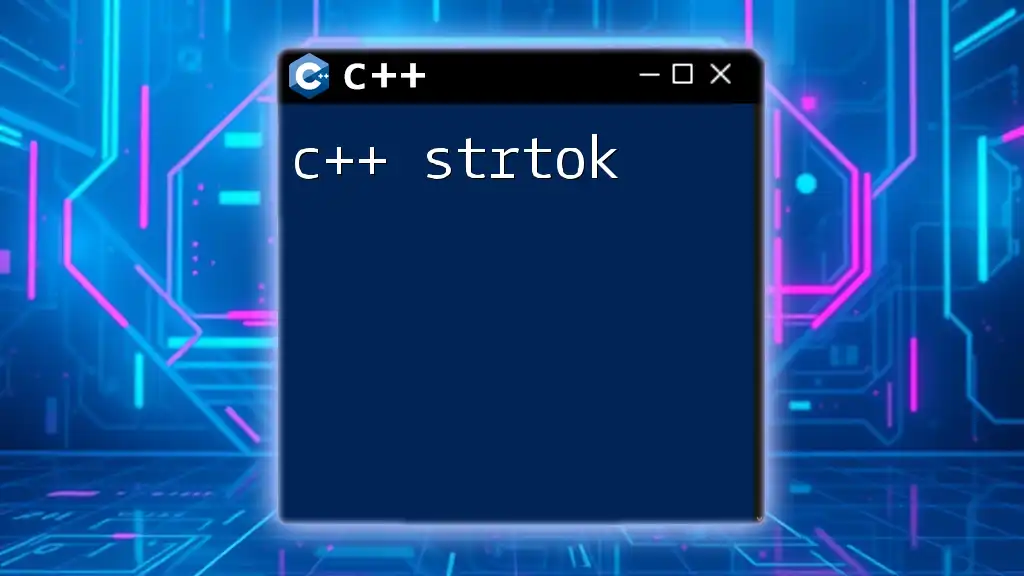
Additional Resources
For further exploration, visit the C++ documentation and reference guides. These resources can deepen your understanding of concise programming techniques, empowering you to write clean, efficient, and short code.
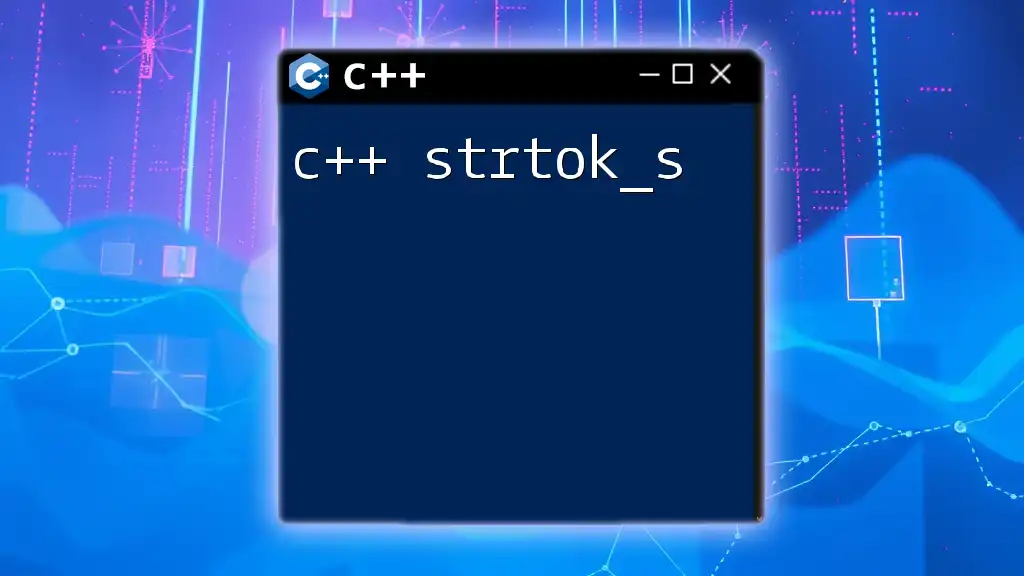
FAQs
Common Questions About Short C++ Techniques
- What are the key benefits of using short commands in C++?
- How can inline functions affect software performance?
- Is it safe to replace all output statements with a single escape sequence?
These queries touch on core aspects of C++ short techniques, reinforcing the value of concise programming in various contexts.