The `c++ sh` command provides a convenient way to execute C++ code snippets directly from the command line shell (sh), streamlining the development and testing process.
Here’s a simple example to illustrate how to compile and run a C++ program using the shell:
g++ -o hello hello.cpp && ./hello
In this example, we're compiling a `hello.cpp` file and then executing the resulting `hello` binary.
Understanding Shell Commands in C++
Shell commands are a crucial aspect of interacting with the operating system. They allow users to perform operations such as file manipulation, process control, and system monitoring directly through the command line interface. In the context of C++, these commands can serve as a bridge, enabling programs to execute shell functionalities while leveraging the power of C++.
The Role of C++ in Shell Programming
C++ provides several advantages for shell programming. While traditional shell scripts (like Bash) are effective for straightforward tasks, C++ allows for more complex operations, improving efficiency and performance. Using C++ alongside shell scripting can provide type safety, faster execution times, and easier debugging, making it exceptionally suited for tasks where performance is critical.
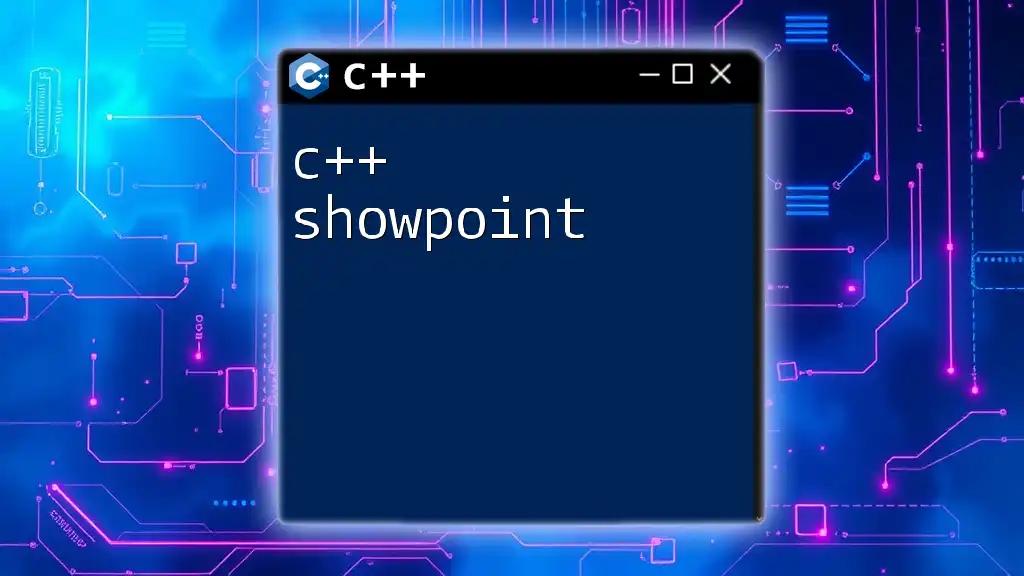
Setting Up Your C++ Environment
Installing a C++ Compiler
To get started with C++ shell programming, you must install a C++ compiler. Popular choices include GCC, Clang, and MSVC.
To install GCC, which is widely used, you can run:
sudo apt update
sudo apt install build-essential
After installation, ensure your setup is correct by checking the compiler version:
g++ --version
Essential Command-Line Tools
A few tools can enhance your command-line experience when doing C++ development. Consider using:
- Make: Automates the compilation process.
- GDB: The GNU debugger for debugging your C++ code effectively.
- Valgrind: A tool for memory debugging, memory leak detection, and profiling.
These tools can significantly improve your productivity and code reliability.
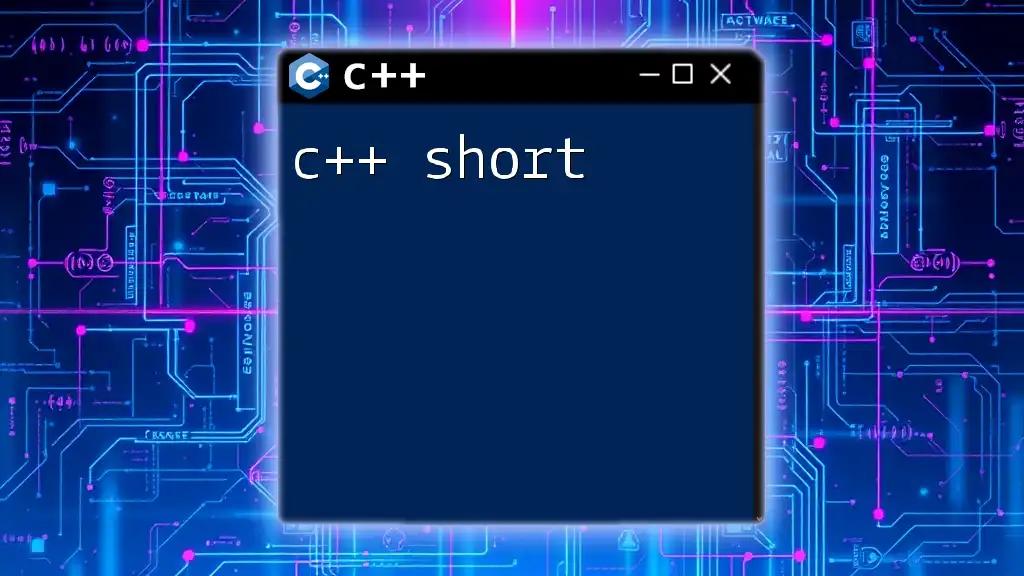
Basic C++ Command Line Usage
Writing Your First C++ Program
Every journey into programming starts with the classic "Hello, World!" program. This simple C++ code snippet serves as a testing ground for understanding the basics of writing and compiling a C++ program.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this program, we include the iostream library to utilize standard input-output functions. The `main()` function is where the program starts execution.
Compiling and Running Your Program
Compiling your C++ code is the process of converting your source code into an executable format. Once you’ve written your "Hello, World!" program in a file named `hello.cpp`, you can compile and run it with the following commands:
g++ hello.cpp -o hello
./hello
The `-o` flag followed by the desired executable name (here, `hello`) allows you to specify what the output file should be named. Running `./hello` executes the compiled program.
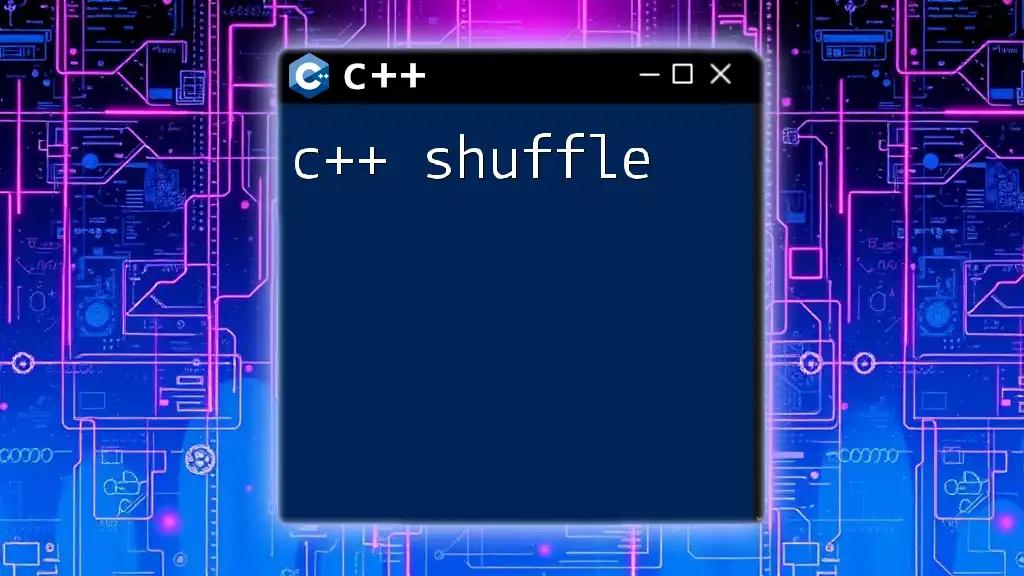
Advanced C++ Shell Techniques
Using Command-Line Arguments
C++ programs can accept input directly from the command line using command-line arguments. This feature allows users to pass dynamic values to the program at runtime. Here’s an example:
#include <iostream>
int main(int argc, char* argv[]) {
for (int i = 0; i < argc; ++i) {
std::cout << "Argument " << i << ": " << argv[i] << std::endl;
}
return 0;
}
In this snippet, `argc` counts the number of arguments, while `argv[]` holds the actual arguments. The program will print each argument passed when executed. For instance:
./my_program arg1 arg2
This command will display:
Argument 0: ./my_program
Argument 1: arg1
Argument 2: arg2
Redirecting Input and Output
In shell programming, you often need to handle files for input and output. C++ simplifies this through input/output redirection.
For example, consider an existing program that takes user input. You can run it with input sourced from a file and redirect output to another file:
./my_program < input.txt > output.txt
The `<` symbol means that `input.txt` is fed to the program as standard input. The `>` symbol saves the program's output to `output.txt`.
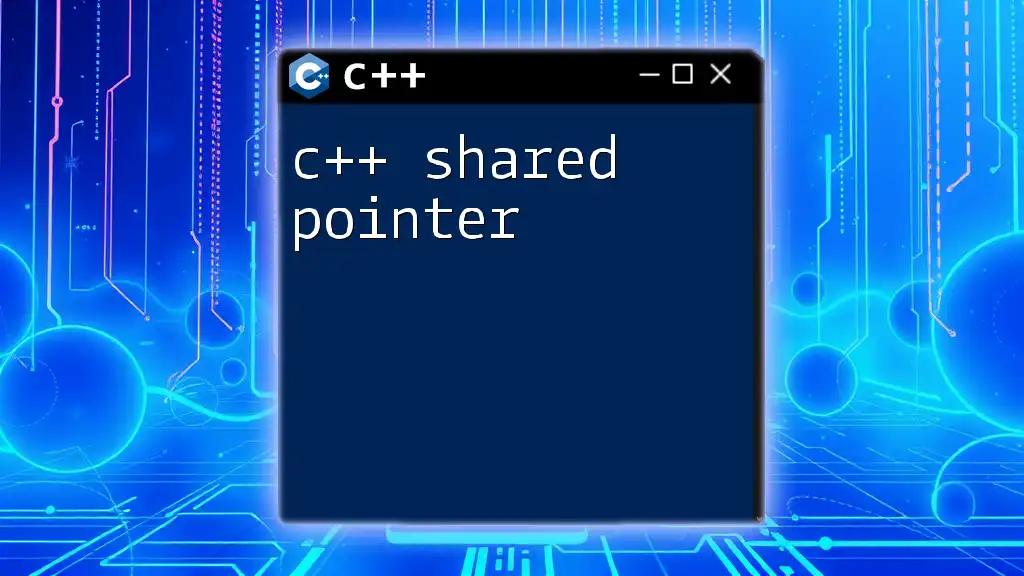
Error Handling in C++ Shell Programs
Common Errors in C++ Shell Command Usage
When writing C++ shell programs, you may encounter various errors. Syntax errors, such as missing semicolons or mismatched braces, are common and can be straightforward to troubleshoot. Logical errors, such as infinite loops or incorrect output, are often trickier to identify as they don’t result in compilation errors but produce unexpected behavior.
Handling Exceptions
C++ offers robust mechanisms for handling errors through exceptions. By using a `try` block, you can catch exceptions and respond gracefully when errors occur. Below is an example:
#include <iostream>
#include <stdexcept>
int main() {
try {
// code that may throw an exception
throw std::runtime_error("An error occurred!");
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
In this code, if an error is thrown, the catch block handles it, printing the relevant error message. This approach enhances user experience and helps in debugging.
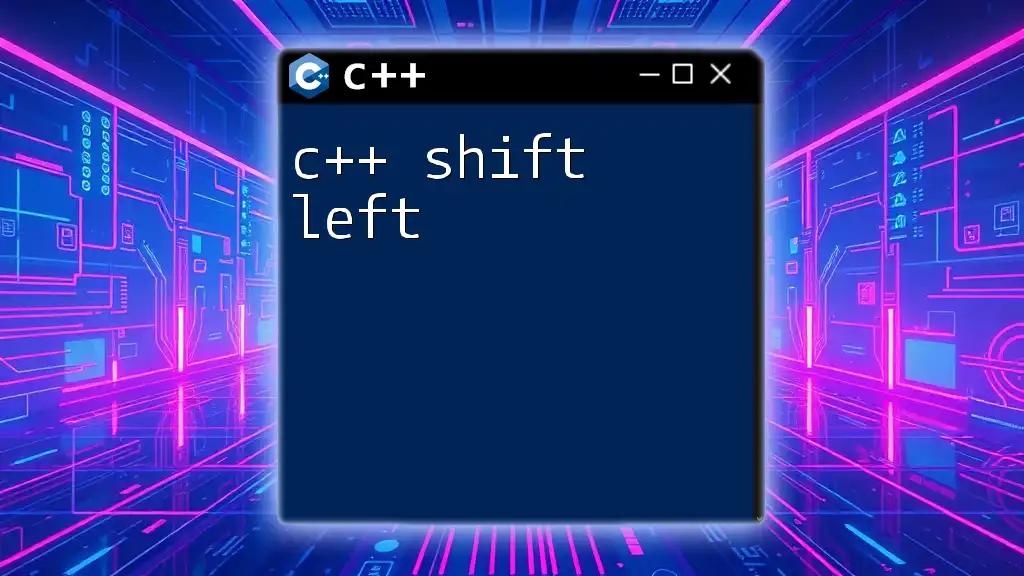
Tips and Best Practices
Writing Clean and Maintainable Code
Writing clean and maintainable C++ code is crucial for successful shell programs. Here are some practices:
- Comment Your Code: Help others (and your future self) by explaining what tricky parts of your code do.
- Organize Code Logically: Use functions and classes to encapsulate behaviors and improve readability.
- Consistent Formatting: Maintain a uniform style for indentation and spacing.
Performance Considerations
While using C++ in command-line settings can yield impressive performance benefits, it's essential to remember that optimized code can make a significant difference—especially in time-sensitive operations. Profile your code using tools like GDB and Valgrind to find and eliminate bottlenecks.
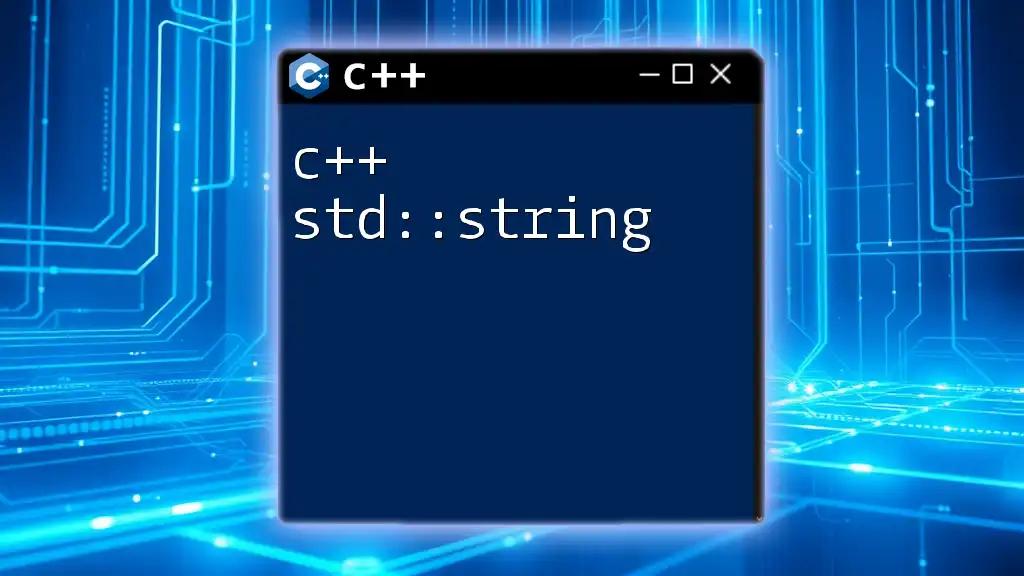
Conclusion
When effectively utilizing C++ within shell environments, you'll find that the combined capabilities can lead to powerful and efficient applications. Whether passing command-line arguments, handling input/output redirection, or managing errors, mastering these techniques will set you on a path to improve your programming proficiency and productivity. Remember, practice makes perfect—so don't hesitate to experiment with the various features C++ offers in your shell programming endeavors.
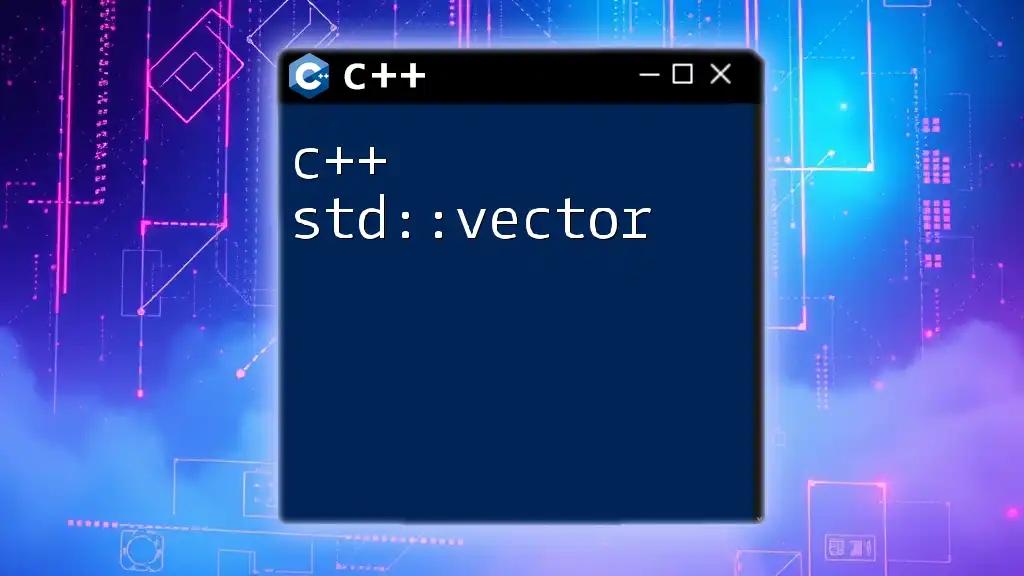
Additional Resources
For a deeper dive into C++ programming and command-line utilities, refer to official documentation, online tutorials, and community forums that offer supportive learning environments. Engaging with other programmers and sharing knowledge can greatly enhance your learning journey.