The C++ `shuffle` function randomly reorders the elements in a container, utilizing a specified random number generator.
#include <algorithm>
#include <iostream>
#include <vector>
#include <random>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::random_device rd;
std::mt19937 g(rd());
std::shuffle(vec.begin(), vec.end(), g);
for (int n : vec) {
std::cout << n << ' ';
}
return 0;
}
Understanding the Shuffle Function
What is Shuffling?
Shuffling is the process of randomly rearranging elements in a collection, such as an array or vector. This technique is particularly useful in various applications, from card games where you want to randomize the order of cards to statistical sampling where you need a random selection of data points.
Importance of Shuffle in C++
In C++, the ability to shuffle elements lends itself to improved algorithm design and enhances randomness. This is critical for applications involving games, simulations, and any scenarios requiring randomized outputs. By utilizing the C++ shuffle function effectively, programmers can ensure that their implementations possess desirable unpredictability.
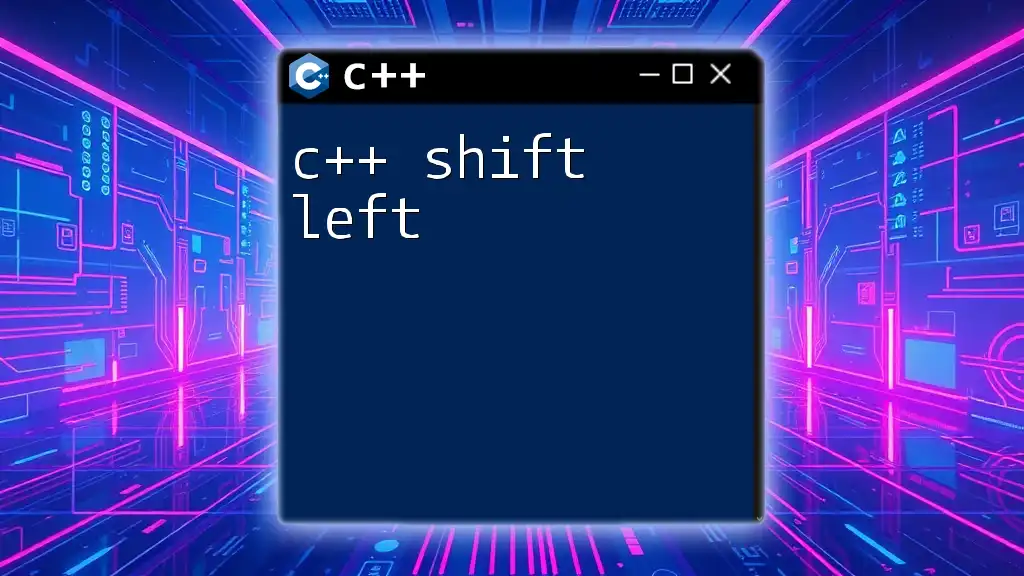
The std::shuffle Function
Definition and Syntax
The std::shuffle function is part of the `<algorithm>` header and provides an efficient way to shuffle elements in a range. The basic syntax of the function is:
std::shuffle(first_iterator, last_iterator, random_number_generator);
Where:
- first_iterator is the starting position of the range to shuffle.
- last_iterator is the ending position of the range to shuffle.
- random_number_generator is an engine responsible for creating randomness.
Parameters of std::shuffle
The parameters of std::shuffle allow for flexibility and define how shuffling is carried out.
- first_iterator: This indicates where the shuffling begins.
- last_iterator: This signifies the end of the range, which should not be included in the shuffle operation.
- random_number_generator: This is pivotal as it determines how the randomization takes place.
An example illustrating the parameter usage is as follows:
#include <algorithm>
#include <iostream>
#include <vector>
#include <random>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
std::shuffle(vec.begin(), vec.end(), std::mt19937{std::random_device{}()});
for (const auto& num : vec) {
std::cout << num << " ";
}
return 0;
}
In this example, the vector `vec` gets shuffled, and the output order will be completely random each time the program runs.
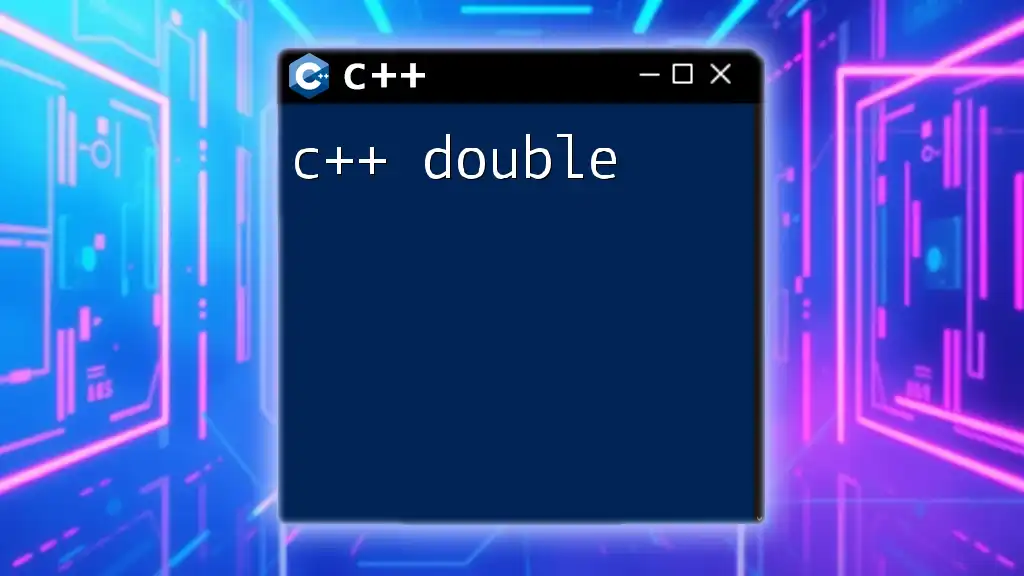
Key Concepts in Shuffling
Random Number Generators
What is a Random Number Generator?
A random number generator (RNG) produces a sequence of numbers that lack any predictable pattern, enhancing the reliability of the `std::shuffle` process. There are two main types of RNGs:
- Pseudo-random: These are generated using algorithms that produce repeatable patterns, such as std::default_random_engine.
- True random: These are generated from physical processes; for example, std::random_device.
Using std::default_random_engine
std::default_random_engine is a popular choice for casual applications. It provides a good balance of speed and randomness. Here’s how to use it:
std::default_random_engine rng;
std::shuffle(vec.begin(), vec.end(), rng);
This code snippet effectively shuffles the contents of the vector `vec`.
Utilizing std::random_device
Introduction to std::random_device
std::random_device serves as a strong source for seeding a random engine as it generates non-deterministic random values. Utilizing this method enhances the randomness in the shuffle operation.
Example of Seeding with std::random_device
Here’s an example of using std::random_device to seed the random engine:
std::random_device rd;
std::mt19937 eng(rd());
std::shuffle(vec.begin(), vec.end(), eng);
By seeding the `mt19937` engine with `rd()`, each shuffle will yield unique results, improving the unpredictability of the outcome.
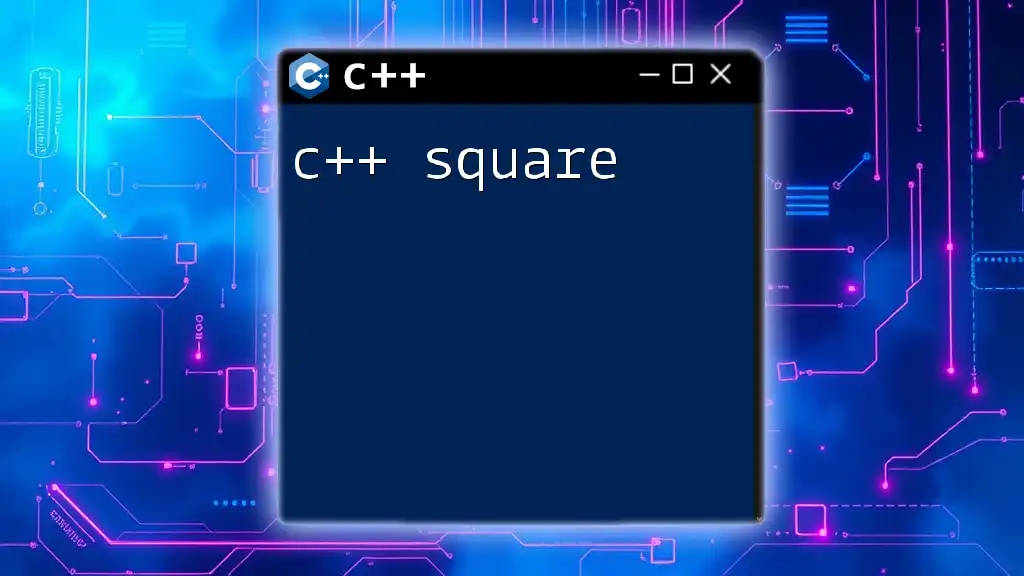
Practical Applications of Shuffling
Shuffling Arrays and Vectors
Shuffling a Simple Array
Shuffling isn’t limited to vectors; you can also shuffle simple arrays. Here’s how to shuffle a basic array:
int arr[] = {1, 2, 3, 4, 5};
std::shuffle(std::begin(arr), std::end(arr), std::mt19937{std::random_device{}()});
Shuffling a Vector of Strings
You can easily extend shuffling to vectors of different data types, such as strings. Below is an example:
std::vector<std::string> names = {"Alice", "Bob", "Charlie", "David"};
std::shuffle(names.begin(), names.end(), std::default_random_engine{});
This will shuffle the names in the `names` vector randomly.
Shuffling Custom Data Structures
Creating a Custom Data Structure
Let’s say you want to represent a card in a deck. You could create a struct as follows:
struct Card {
std::string suit;
std::string rank;
};
Shuffling Custom Structs
To shuffle a vector containing custom structs, you can do it like this:
std::vector<Card> deck = {{"Hearts", "A"}, {"Diamonds", "2"}, {"Clubs", "3"}, {"Spades", "4"}};
std::shuffle(deck.begin(), deck.end(), std::mt19937{std::random_device{}()});
Each shuffle randomly alters the order of the cards in the deck.
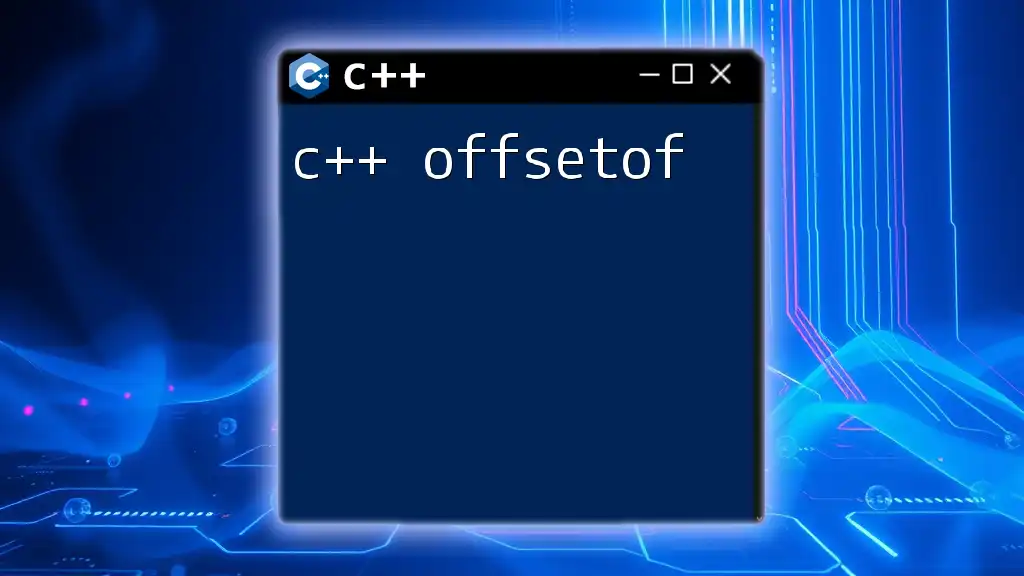
Best Practices for Using std::shuffle
Avoiding Common Pitfalls
While using std::shuffle, you need to be aware of pitfalls such as using weak or poorly implemented random number generators. To ensure a robust shuffling, always choose a high-quality PRNG like std::mt19937 seeded with std::random_device.
Performance Considerations
The performance of std::shuffle can vary based on the size of the dataset. For smaller vectors or arrays, the performance overhead is negligible. However, for larger datasets, ensure that the random number generator and the shuffling algorithm you choose are efficient.
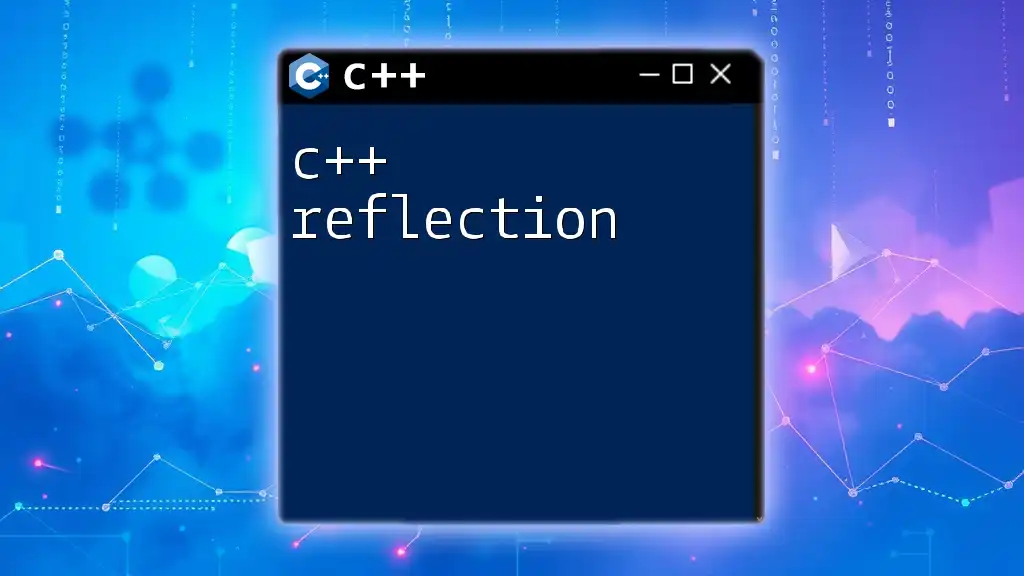
Conclusion
In summary, C++ shuffle provides a powerful and flexible tool for randomizing the order of elements within a collection. Understanding how to use it effectively can greatly enhance your programming projects that involve randomness, from games to simulations. Explore the various implementations and customization options to fully leverage this function in your applications. With practice, you’ll be able to incorporate shuffling seamlessly into your C++ projects, adding an element of unpredictability and excitement.
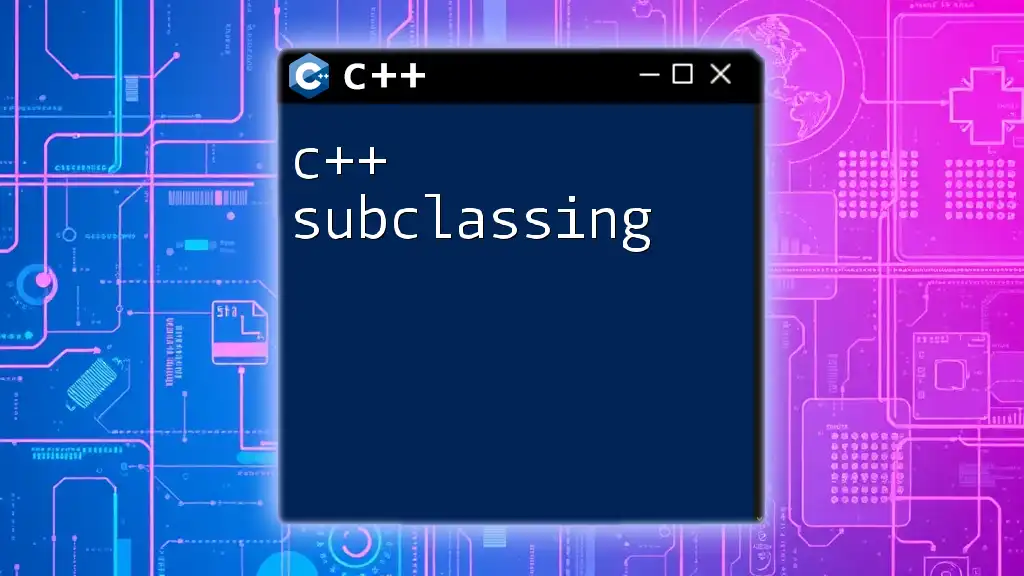
Additional Resources
For further reading, check the official documentation for std::shuffle and various random number generators available in the C++ Standard Library. Additionally, explore resources on algorithms and data structures for a deeper understanding of randomization techniques and their applications.