The `strlen` function in C++ returns the length of a null-terminated string, excluding the null character.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <cstring>
int main() {
const char* myString = "Hello, World!";
std::cout << "Length of the string: " << strlen(myString) << std::endl;
return 0;
}
Understanding `strlen` in C++
What is `strlen`?
`strlen` is a function from the C Standard Library that returns the length of a given string. This function is crucial in programming as it allows developers to determine the size of C-style strings, which are simply arrays of characters ending with a null character (`'\0'`). Knowing the length of a string is vital for various operations, such as memory allocation, string manipulation, and ensuring data integrity.
C++ Strings vs. C Strings
In C++, there are primarily two types of strings:
-
`std::string`: A part of the C++ Standard Library that manages dynamic memory automatically. It offers a range of functionalities, including size, substring operations, and safe memory handling.
-
C-Style Strings: Arrays of type `char` that require manual management of memory. `strlen` specifically operates on these strings, meaning it counts characters up to the terminating null character.
Understanding how `strlen` works with C-style strings is essential, especially when transitioning between C and C++.
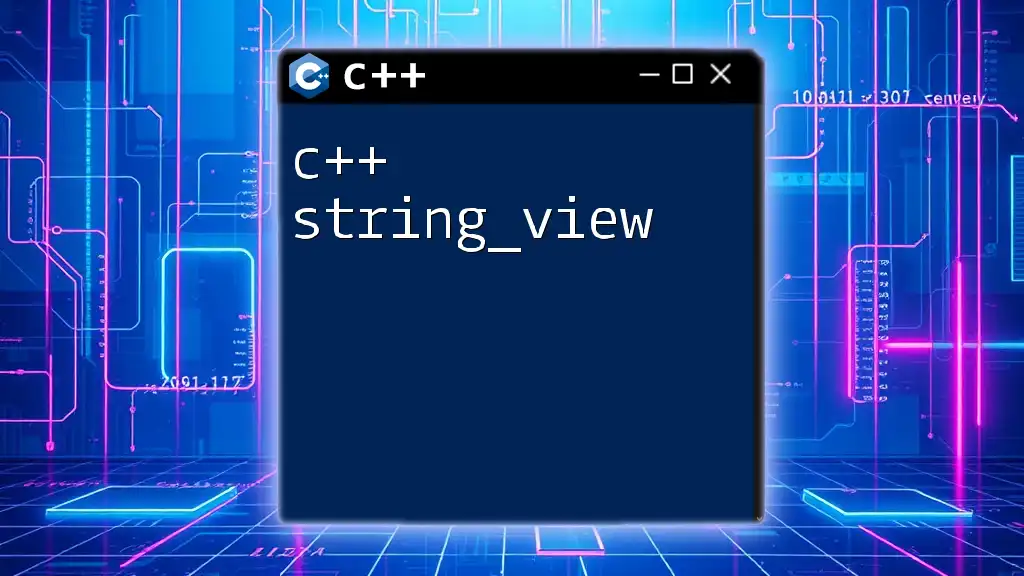
Syntax of `strlen`
Function Prototype
The signature of the `strlen` function is:
size_t strlen(const char *str);
Here, `str` is a pointer to the character array (C-style string) whose length you want to determine.
Parameters and Return Type
-
Parameters: The `const char *str` is the input string. The `const` qualifier indicates that this string should not be modified.
-
Return Type: The function returns a `size_t` data type—a type defined to represent the size of objects in bytes. It's important because it ensures that the value can represent large sizes and is compatible with various types of memory allocations.
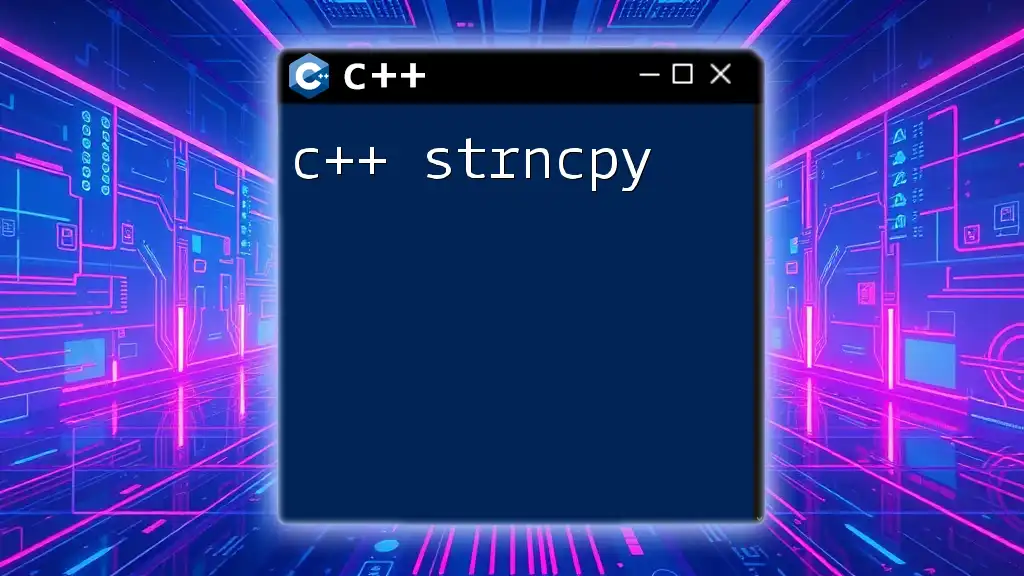
How to Use `strlen` in C++
Basic Usage Example
Using `strlen` is simple. Here's a basic illustration of its functionality:
#include <iostream>
#include <cstring> // Required header for strlen
int main() {
const char* myString = "Hello, World!";
size_t length = strlen(myString);
std::cout << "Length of the string: " << length << std::endl;
return 0;
}
In this example:
- We include the `<cstring>` header to access the `strlen` function.
- The function is called with a C-style string, and it returns the length of the string, which we print to the console.
Common Pitfalls
While `strlen` is straightforward, it can lead to errors if you're not careful:
-
Using Uninitialized Strings: If the string you pass to `strlen` is uninitialized or pointing to an invalid memory location, this will result in undefined behavior. Always ensure that your strings are valid before calling `strlen`.
-
Memory Safety: Ensure that the string is null-terminated. If not, `strlen` will continue to read memory until it finds a null character, potentially leading to program crashes or security vulnerabilities.
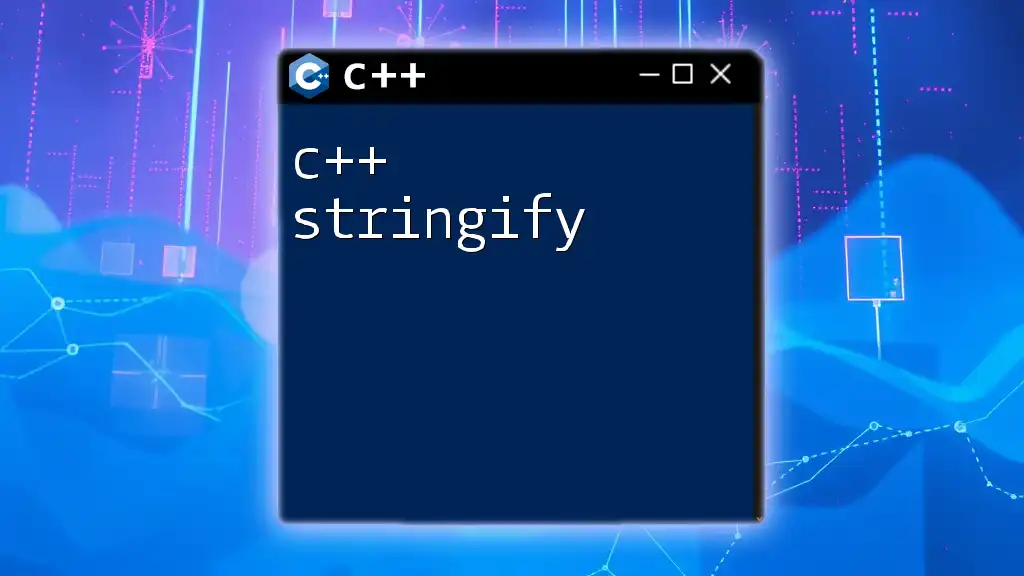
Working with `strlen` in C++
Practical Examples
Example 1: Calculating Length in a Function
You can encapsulate the `strlen` function in a user-defined function for better modularity:
size_t getStringLength(const char* str) {
return strlen(str);
}
This function simply returns the length of any C-style string you pass to it. Such encapsulation is handy for larger projects where string length calculations are frequent.
Example 2: Using `strlen` with User Input
Another practical scenario is when you want to calculate the length of user-provided input:
#include <iostream>
#include <cstring>
int main() {
char input[100];
std::cout << "Enter a string: ";
std::cin.getline(input, 100);
std::cout << "You entered: " << input << "\nLength: " << strlen(input) << std::endl;
return 0;
}
In this example:
- A character array named `input` can store user input up to 99 characters.
- The program reads a line of input and calculates its length with `strlen`, displaying the result.
Performance Considerations
It's essential to recognize that `strlen` has a time complexity of O(n), where n is the number of characters in the string. This means the function must traverse the entire string to find the null terminator. In scenarios where you need to access the string length multiple times, consider storing the length in a variable to avoid repeated calls.
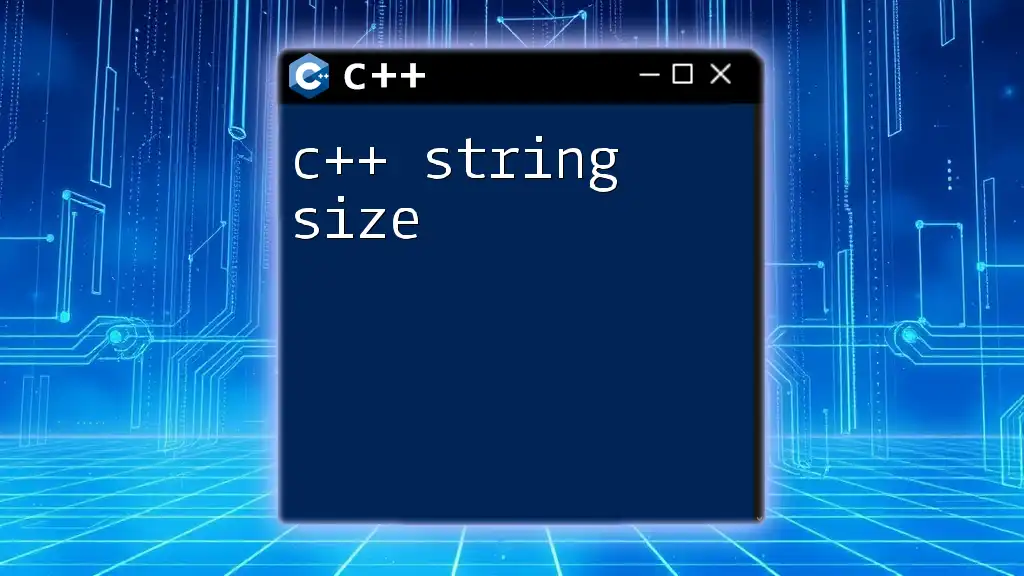
Best Practices
Handling Strings Safely
To prevent bugs and errors in your programs:
- Always ensure that the string is null-terminated when using `strlen`.
- Prefer using `std::string` over C-style strings whenever possible. `std::string` handles memory management automatically, reducing the risk of memory-related bugs.
Avoiding Overuse of `strlen`
It's also wise to consider when repeated calls to `strlen` may be unnecessary:
- If you are working with large strings and accessing their lengths multiple times, cache the result of `strlen` to improve performance.
- In performance-critical applications, consider alternatives, such as adding member functions to your custom string class that maintains the length as part of its data.
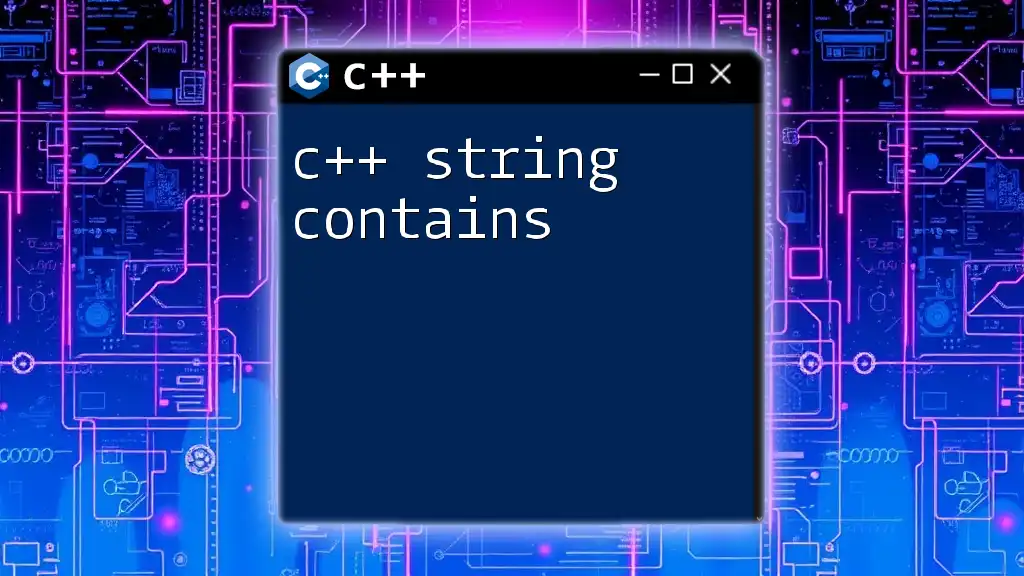
Conclusion
In summary, `strlen` is a fundamental component in C++ for measuring the length of C-style strings. Understanding its functionality, limitations, and correct usage is crucial for any developer working with string data. By implementing best practices and ensuring safe handling, you can effectively utilize `c++ strlen` in your programs.
Further Learning Resources
To enhance your skills and deepen your understanding of string handling in C++, consult the official C++ documentation for `strlen` and explore comprehensive tutorials available online. Many authoritative books on C++ also cover string manipulation extensively, which can be invaluable as you advance in your development journey.
Call to Action
Take time to experiment with `strlen` and various string manipulation techniques in your projects. Engaging with the programming community by sharing your experiences and questions can also lead to deeper insights and learning opportunities.