In C++, you can compare two strings for equality using the `==` operator, which checks if their contents are identical.
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello";
std::string str2 = "Hello";
if (str1 == str2) {
std::cout << "Strings are equal." << std::endl;
} else {
std::cout << "Strings are not equal." << std::endl;
}
return 0;
}
Understanding C++ Strings
What Are C++ Strings?
In C++, strings are sequences of characters used to represent text. There are two primary ways to handle strings: using C-style strings (character arrays) and using the C++ `std::string` class.
C-style strings consist of an array of characters terminated by a null character (`'\0'`). While they are lightweight, they can lead to errors such as buffer overflows if not handled carefully.
On the other hand, C++ `std::string` offers a more convenient and safer way to work with strings by encapsulating memory management and providing numerous built-in methods for string manipulation. Advantages of `std::string` include:
- Dynamic sizing: Strings can grow or shrink automatically.
- Convenience methods: Built-in functions like `length()`, `append()`, `substr()`, and more.
Basic Operations on Strings
Before diving into string equality, it is important to understand the basic operations available for strings:
- Concatenation: Strings can be combined using the `+` operator.
- Length: Get the number of characters using the `length()` method.
- Indexing: Access individual characters via indexing, similar to arrays.
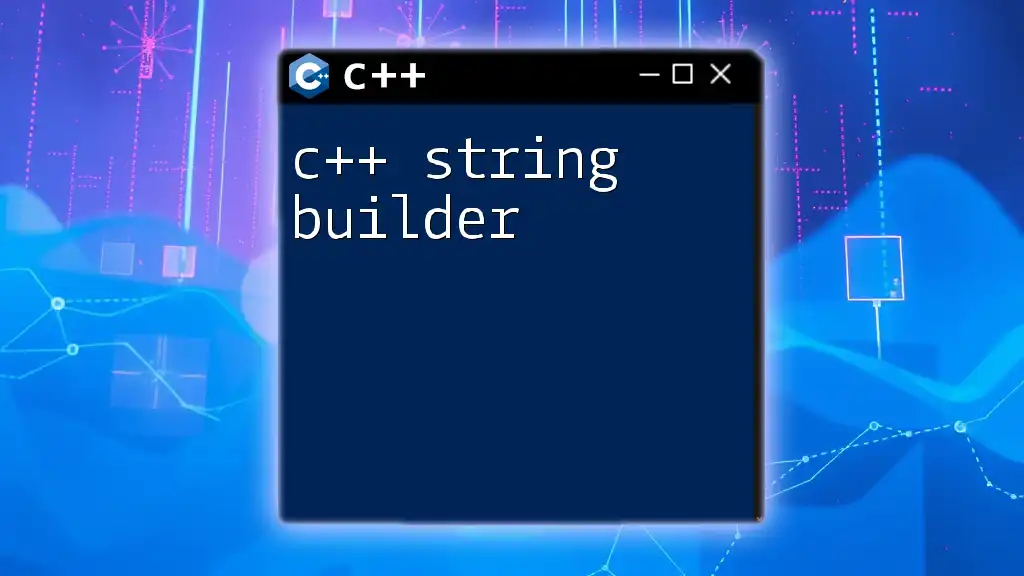
What Is String Equality?
Definition of String Equality in C++
String equality refers to the concept of determining whether two strings hold the same sequence of characters. Understanding equality is crucial in programming as it helps in making decisions and controlling the flow of a program.
Why Compare Strings?
There are numerous scenarios when you might need to compare strings, including validating user input, searching for substrings, or checking equality for configuration settings. Recognizing string equality plays a pivotal role in data processing and decision-making within a program.
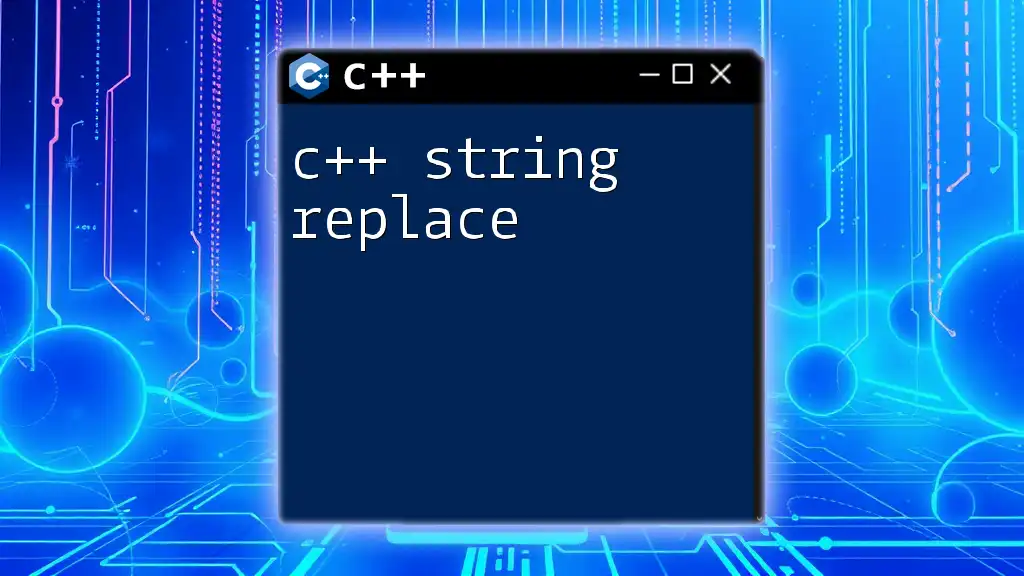
Methods to Compare Strings in C++
Using the `==` Operator
One of the simplest ways to compare two strings in C++ is using the `==` operator.
Code Snippet Example:
#include <iostream>
#include <string>
int main() {
std::string str1 = "hello";
std::string str2 = "hello";
if (str1 == str2) {
std::cout << "Strings are equal!" << std::endl;
} else {
std::cout << "Strings are not equal!" << std::endl;
}
}
In this example, the program compares `str1` and `str2` using the `==` operator. If both strings match, they are deemed equal.
This method is typically the most intuitive but should be used with care regarding performance in certain applications. While the `==` operator is straightforward, it may not be the most efficient for every scenario, especially with longer strings.
Utilizing `compare()` Method
Overview of `std::string::compare()`
The `compare()` method offers another way to compare strings and provides additional functionality over the `==` operator. Its prototype looks like this:
int compare(const std::string& str) const;
Example Usage
Code Snippet Example:
#include <iostream>
#include <string>
int main() {
std::string str1 = "hello";
std::string str2 = "world";
if (str1.compare(str2) == 0) {
std::cout << "Strings are equal!" << std::endl;
} else {
std::cout << "Strings are not equal!" << std::endl;
}
}
In this example, `compare()` returns `0` if both strings are identical, a value less than `0` if `str1` is lexicographically less than `str2`, and greater than `0` if it is greater.
Using `compare()` is advantageous because it lets you control how you want to compare strings, making it versatile for various situations.
Comparing C-style Strings
Using `strcmp` Function
For C-style strings, the `strcmp` function from the C Standard Library is used for comparison. This function takes two pointers to C-style strings and returns an integer based on their lexicographic ordering.
Example Code Snippet
Code Snippet Example:
#include <iostream>
#include <cstring>
int main() {
const char* str1 = "hello";
const char* str2 = "hello";
if (strcmp(str1, str2) == 0) {
std::cout << "Strings are equal!" << std::endl;
} else {
std::cout << "Strings are not equal!" << std::endl;
}
}
In this case, `strcmp` returns `0` if both strings are equal, just like `std::string::compare()`. However, it’s generally recommended to avoid C-style strings in modern C++ programming owing to the higher risk of errors (such as buffer overflows) involved with manual memory management.
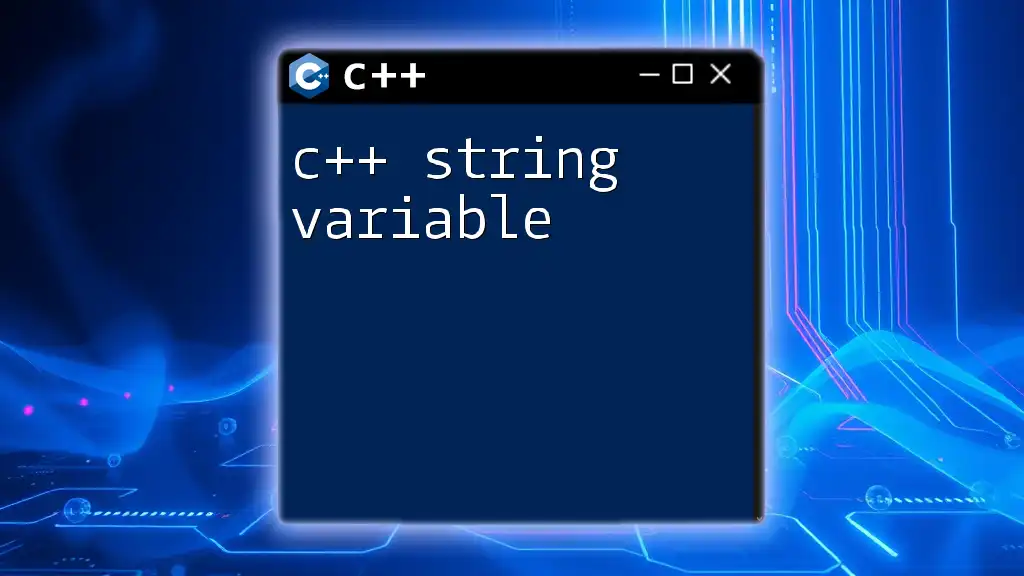
Performance Considerations
Time Complexity of String Comparison
The time complexity of string comparison depends on the length of the strings being compared. Both the `==` operator and the `compare()` function typically operate in linear time, O(n), where n is the length of the string. Thus, for very long strings, even slight differences can result in increased time taken for comparison.
Best Practices for String Comparison
To avoid common pitfalls:
- Always ensure strings are initialized before comparison, preventing undefined behavior.
- Be mindful of the performance impact on frequently invoked comparisons, especially in loops or high-frequency sections of code.
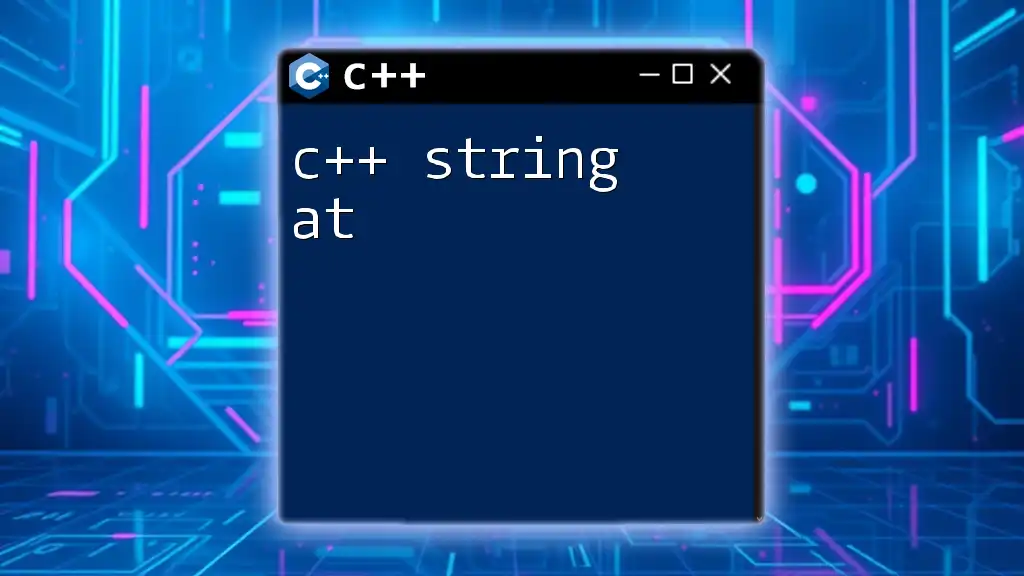
Handling Case Sensitivity in Comparisons
Case-Sensitive vs Case-Insensitive Comparison
By default, string comparisons in C++ are case-sensitive. This means that `"Hello"` and `"hello"` would not be considered equal. There are specific cases where case insensitivity is necessary, such as user input validation or searching in a case-insensitive context.
Solutions for Case-Insensitive Comparison
You can implement a custom function to compare strings without regard to case, enhancing usability.
Custom Function Example
Code Snippet Example:
#include <iostream>
#include <algorithm>
bool caseInsensitiveCompare(const std::string &str1, const std::string &str2) {
return std::equal(str1.begin(), str1.end(), str2.begin(),
[](char a, char b) { return tolower(a) == tolower(b); });
}
int main() {
std::string s1 = "Hello";
std::string s2 = "hello";
if (caseInsensitiveCompare(s1, s2)) {
std::cout << "Strings are equal (case-insensitive)!" << std::endl;
} else {
std::cout << "Strings are not equal!" << std::endl;
}
}
In this example, the `caseInsensitiveCompare` function utilizes the `std::equal` algorithm combined with a lambda function to convert both characters to lowercase before comparing them. This approach provides a flexible solution to handle variations in character cases.
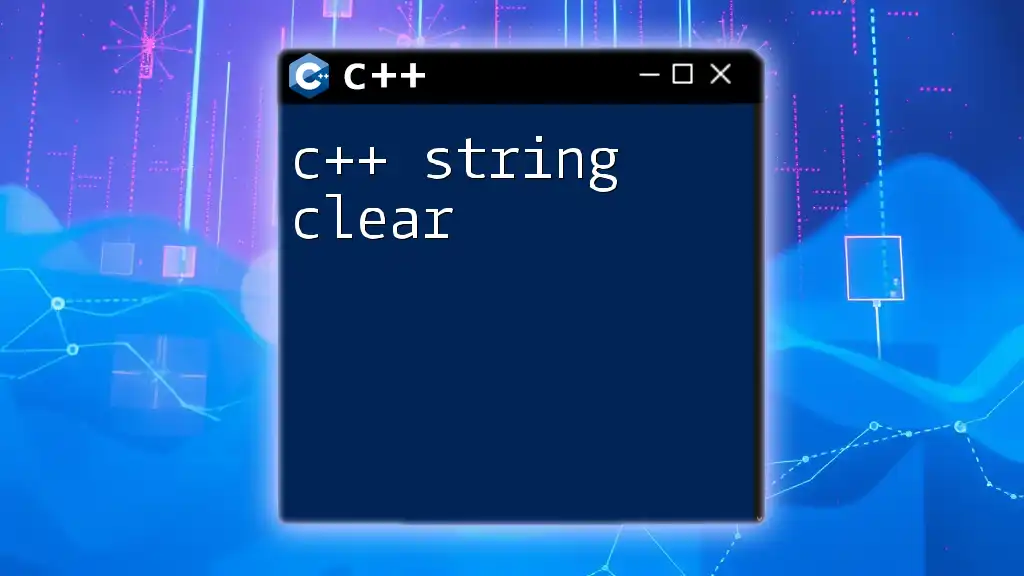
Conclusion
Understanding string equality in C++ is essential for effective programming. With various methods available—whether using the `==` operator, `compare()` method, or even C-style strings—developers have multiple options to implement string comparisons tailored to their needs.
By grasping these concepts and practicing with the examples provided, you can significantly improve your skills in string manipulation—a vital part of modern C++ programming. Join our program for more in-depth experiences and training in mastering C++.